Introduction
Creating your first web app with Python and Flask is an exciting step into the world of web development. If you’re new to building websites but have some experience with Python, you’ll find this guide easy to follow. Flask is a lightweight, flexible microframework that lets you create powerful web apps without the extra complexity. It’s perfect for beginners!
In this blog post, we’ll walk you through everything you need to get started. From setting up Flask on your computer to creating a simple web app, you’ll learn the basics of Python web development. By the end, you’ll have a fully functioning app and a deeper understanding of how to use Flask to build more advanced projects.
Here’s what you’ll find:
- What is Flask? A brief introduction to the Flask microframework.
- Setting up Flask: How to get Flask running on your machine.
- Building your first app: Step-by-step instructions to create a basic web app.
- Next steps: How to expand your Flask app and add features like forms or user authentication.
Whether you’re looking to build your own portfolio or want to start creating dynamic websites, this guide will help you take that first step. So let’s get started and unlock the potential of Flask!
What is Flask? A Lightweight Python Web Framework
Flask is a microframework built using Python that allows you to build web applications quickly and easily. Unlike some larger frameworks, like Django, Flask keeps things simple and doesn’t force you to use specific tools or libraries. This makes it a perfect fit if you’re looking for a flexible, minimalistic way to start building your first web app.
The Flask framework is lightweight, which means it only comes with the essentials you need to get your app up and running. You can add features like authentication or form handling when you need them, but nothing is built-in by default, giving you the freedom to design your app exactly how you want it.
With Flask, you don’t have to deal with a steep learning curve. Many beginners love it for its simplicity, and experienced developers appreciate its flexibility for creating everything from simple projects to more complex applications.
Why Choose Flask for Web Development?
When comparing Flask vs Django, Flask is often favored by those who want more control over their app’s structure. Django is a full-stack framework that comes with everything pre-packaged, like a database system and an admin panel. This is great for big projects but might feel overwhelming if you’re just getting started.
On the other hand, Flask is a microframework. It doesn’t come with built-in components like Django, which allows you to add features only when you need them. This makes Flask easier to learn, and it gives you more flexibility to make decisions on how your Python web app is built.
Key benefits of using Flask:
- Simplicity: You only need a few lines of code to get a basic web app up and running.
- Flexibility: You can choose what tools and libraries to integrate.
- Small learning curve: If you’re new to Python web development, Flask’s lightweight nature will help you get started quickly.
- Scalability: Even though Flask is minimal, it can grow with your project by integrating additional tools and libraries when needed.
In short, if you’re building your first Web App with Python and Flask, Flask is an excellent choice for gaining confidence and understanding the basics of web development.
Prerequisites for Setting Up a Flask Application
Installing Python for Flask Development
Before creating your first web app using Flask, you must install Python on your computer. Flask is built on Python, so getting the right version is an essential first step in setting up your Flask environment. Let’s walk through how to install Python and verify that everything is working correctly.
Steps to Install Python
- Download the latest version: Visit the official Python website at python.org and download the latest version of Python 3.x. It’s important to get the most up-to-date version because Flask works best with Python 3.
- Run the installer: After downloading, run the installer and make sure to check the box that says “Add Python to PATH”. This will ensure you can run Python from any command line.
- Complete installation: Follow the prompts to complete the installation. Once it’s done, you’re ready to verify that Python is installed correctly.
Verifying Python Installation
To check if Python is installed correctly:
- Open the command line (Command Prompt on Windows, Terminal on macOS/Linux).
- Type the following command and hit Enter:
python --version
You should see something like Python 3.x.x (replace ‘x’ with your version number). If this command returns the correct version number, you’re ready to move on to setting up a virtual environment for your Flask project.
Setting Up a Virtual Environment
When building your first Web App with Python and Flask, it’s a good practice to use a virtual environment. This keeps your project dependencies isolated, so any libraries or packages you install don’t interfere with other Python projects on your computer.
Why Use a Virtual Environment?
A virtual environment ensures that your Flask project remains self-contained. This means that different projects can have their own libraries, even if they need different versions of the same package. For example, you might be working on one project that uses Flask 1.x and another project that requires Flask 2.x—a virtual environment helps avoid conflicts between these projects.
How to Create and Activate a Virtual Environment
Here’s how to set up a virtual environment for your Flask project:
- Create the virtual environment: In your command line, navigate to the folder where you want your Flask project to live. Then run the following command:
python -m venv venv
This creates a folder named venv
that contains your isolated Python environment.
Activate the virtual environment: Once created, you need to activate the environment. Use the following command based on your operating system:
- On Windows:
venv\Scripts\activate
- On macOS/Linux:
source venv/bin/activate
After activation, your command line will show the environment name in parentheses, like this: (venv)
, indicating that your virtual environment is now active.
Deactivating the virtual environment: When you’re done working, deactivate the environment by typing:
deactivate
By isolating your Flask project in a virtual environment, you keep everything organized, and future projects won’t conflict with each other.
Installing Flask in Your Environment
With Python and your virtual environment ready, it’s time to install Flask. Installing Flask is easy with pip, which is Python’s package manager.
How to Install Flask Using pip
- Install Flask: Once your virtual environment is active, you can install Flask by running this command:
pip install Flask
This will download and install Flask along with its necessary dependencies.
2. Verifying Flask Installation: To make sure Flask installed correctly, you can run the following command to check the version:
flask --version
You should see the installed Flask version, which confirms that your setup is complete.
Additional Steps for Your Flask Setup
Now that you have Flask installed, you can start building your first web app! Here’s a quick checklist of what you’ve done so far:
- Installed Python.
- Set up a virtual environment for your Flask project.
- Installed Flask using pip.
In the next steps, we’ll start building a simple web app using Flask. You’ll learn how to set up routes, create templates, and even expand the app to include features like forms and authentication. If you want to get a head start, feel free to check out our Flask Forms and Flask Authentication tutorials.
Table: Overview of Setup Commands
Step | Command | Purpose |
---|---|---|
Install Python | N/A | Download Python from official website |
Verify Python | python --version | Check Python version installed |
Create virtual environment | python -m venv venv | Set up project-specific environment |
Activate environment | source venv/bin/activate (macOS/Linux) or venv\Scripts\activate (Windows) | Activate virtual environment |
Install Flask | pip install Flask | Install Flask into the environment |
Verify Flask | flask --version | Check Flask installation |
With these steps complete, you’re on your way to creating your first Web App with Python and Flask. If you ever run into issues, be sure to check the command line for error messages—they usually point you in the right direction.
Step-by-Step Guide: Setting Up a Basic Flask Application
Creating the Flask Project Structure
To get started with your first Web App with Python and Flask, it’s important to organize your project files in a clean and efficient way. Following a good Flask folder structure will make your project more manageable, especially as it grows. Let’s walk through how to organize your Flask project directory.
Organizing Your Project Files
A typical Flask project structure looks something like this:
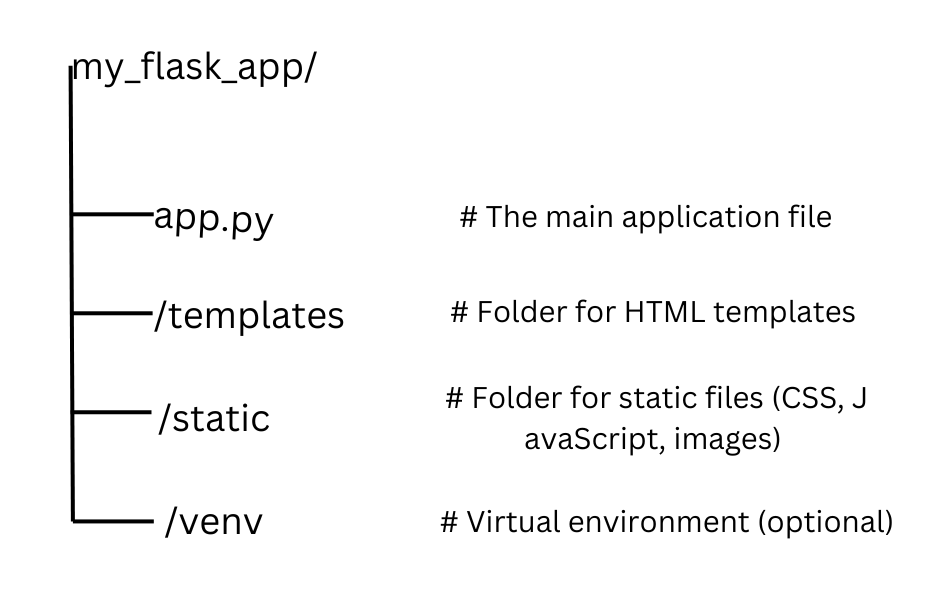
Here’s a breakdown of each element:
- app.py: This is the main file where your Flask app will live. This is where you define routes and set up the core logic.
- /templates: This folder holds all your HTML files. Flask uses the Jinja2 templating engine to dynamically render these files.
- /static: This is where you put your static assets like CSS, JavaScript, and images. Flask automatically serves these files when your app runs.
- /venv: If you’ve set up a virtual environment, you’ll see a
venv
folder, which isolates your dependencies.
By using this structure, you follow Flask best practices for organizing a project. It makes your application more scalable and easier to navigate as it grows.
Writing Your First Flask Application (app.py
)
Once your Flask project directory is set up, it’s time to create the main file for your app, app.py
. This file will contain your first web app code.
Here’s a basic example of a simple Flask app that returns “Hello, World!”:
Code Example: A Basic Flask App
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == "__main__":
app.run(debug=True)
Let’s break it down:
from flask import Flask
: This line imports the Flask class. You use this class to create your Flask app.app = Flask(__name__)
: This initializes the Flask app by passing the name of the current module (__name__
).@app.route('/')
: This is a route definition. It tells Flask to run thehello_world
function when someone accesses the root URL (/
).return 'Hello, World!'
: This function returns “Hello, World!” when the route is accessed.app.run(debug=True)
: This line starts the Flask development server. Thedebug=True
flag means Flask will reload the app whenever you make changes, which is great for development.
This is the core of a minimal Flask setup. It’s simple but powerful—you now have a Web App with Python and Flask that responds to HTTP requests.
Running Your Flask Application Locally
Now that you’ve written the code for your first web app, it’s time to run it locally. Flask has a built-in development server that makes this easy.
Steps to Run Your Flask App
- Activate your virtual environment: Before running the app, make sure your virtual environment is activated (if you set one up). On Windows:
venv\Scripts\activate
On macOS/Linux:
source venv/bin/activate
2. Run the app: With your virtual environment activated, go to your project directory and run the following command:
flask run
You should see output like this:
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
3. Testing on localhost: Open a web browser and go to http://localhost:5000/. You should see “Hello, World!” displayed on the screen. This confirms that your Flask app is running successfully.
By following these steps, you can test your Flask app on your local machine before deploying it to a live server.
Understanding Flask Routing and Views
One of the most powerful features of Flask is its routing system. Routes in Flask are URLs that map to specific view functions. These functions handle requests and return responses. Let’s break it down so you can understand how Flask routes work.
Defining Routes in Flask
Routes are defined using the @app.route()
decorator. This tells Flask which URL a specific function should respond to.
For example, in the previous “Hello, World!” app, we defined this route:
@app.route('/')
def hello_world():
return 'Hello, World!'
Here, the @app.route('/')
decorator tells Flask that the function hello_world()
should run whenever the root URL (/
) is accessed.
Defining Multiple Routes
You can define more routes to add more functionality to your first Web App with Python and Flask. For example, if you want to add a /about
page:
@app.route('/about')
def about():
return 'This is the about page.'
Now, when someone visits http://localhost:5000/about, they’ll see “This is the about page.”
Mapping URL Endpoints to View Functions
Each route in Flask maps an endpoint (URL) to a view function. The view function processes incoming requests and returns a response, which can be HTML, plain text, JSON, or any other valid HTTP response. Here’s a quick breakdown of how it works:
- User requests a URL: The user enters a URL (like
/about
) in their browser. - Flask matches the route: Flask checks if there is a route that matches the requested URL.
- View function runs: If Flask finds a matching route, it calls the associated view function and returns its result to the user’s browser.
This simple routing system is what makes Flask such an elegant framework for Python web development. You can easily define routes and build powerful web apps.
Table: Flask Routing Overview
Route | View Function | Response |
---|---|---|
/ | hello_world() | ‘Hello, World!’ |
/about | about() | ‘This is the about page.’ |
Now that you understand the basics of routing in Flask, you can start building more complex apps with dynamic routes and data handling. Your first web app is just the beginning, and there’s much more to explore, like adding forms, user authentication, and more.
Handling Templates and Static Files in Flask
Rendering HTML with Flask Templates
When you’re building your first Web App with Python and Flask, you’ll want to move beyond simply returning plain text like “Hello, World!” and start serving real HTML pages. This is where Flask’s Jinja2 templating engine comes in. Using Flask Jinja templates, you can dynamically generate HTML pages that are more interactive and personalized. Let’s explore how Flask handles this and how to pass data from Python into your HTML templates.
How to Use the Jinja2 Templating Engine
Flask uses Jinja2, a powerful templating engine that lets you insert Python code directly into HTML. This is useful for generating dynamic content based on the data you have in your app.
Here’s an example of how to render an HTML template in Flask:
- First, create an HTML file inside a /templates folder. Let’s call it
index.html
<!-- /templates/index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>First Web App</title>
</head>
<body>
<h1>Welcome to {{ app_name }}!</h1>
<p>This is your first web app using Flask!</p>
</body>
</html>
Next, in your app.py file, you’ll pass data from Python into the HTML template using the render_template()
function:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html', app_name='First Web App')
if __name__ == "__main__":
app.run(debug=True)
In this example:
render_template()
: This function loads theindex.html
template and injects theapp_name
variable from Python into the template.{{ app_name }}
: This is a Jinja2 expression that outputs the value ofapp_name
in the HTML.
Now, when you navigate to http://localhost:5000/, you’ll see the dynamically generated page: “Welcome to First Web App!” This demonstrates how Flask renders HTML with Jinja templates and passes data between Python and HTML.
Passing Data from Python to HTML Templates
In Flask, passing data from Python to your HTML templates is incredibly flexible. You can pass variables, lists, dictionaries, or even complex objects. Here’s a simple example where we pass a list of items to our HTML template:
@app.route('/items')
def show_items():
items = ['Flask', 'Python', 'HTML', 'CSS']
return render_template('items.html', items=items)
In your items.html
template:
<!-- /templates/items.html -->
<ul>
{% for item in items %}
<li>{{ item }}</li>
{% endfor %}
</ul>
This loop will generate a list of items in HTML:
- Flask
- Python
- HTML
- CSS
This illustrates the power of Flask templates in building dynamic web pages. The ability to pass data from Python to templates allows you to create more interactive and user-friendly web applications. And, of course, this is all part of building your first Web App with Python and Flask.
Using Static Files in Flask (CSS, JS, Images)
No web app is complete without some styling and interactivity. Flask makes it easy to include static files like CSS, JavaScript, and images in your project. Let’s look at how you can organize and link these static files in your Flask app.
Organizing Static Files in Flask
To organize your static assets, Flask uses a dedicated /static folder. This folder holds all your CSS, JS, and image files. Here’s what your project structure should look like:

In this structure:
- /static/css: Stores your CSS files.
- /static/js: Stores your JavaScript files.
- /static/images: Stores your image files.
Loading CSS, JavaScript, and Images into Your App
To load these static files into your templates, Flask provides a handy url_for()
function. Let’s walk through how to link your CSS, JS, and image files.
- Linking CSS: In your HTML template (
index.html
), add the following<link>
tag inside the<head>
section to load your CSS file:
<head>
<link rel="stylesheet" href="{{ url_for('static', filename='css/styles.css') }}">
</head>
2. Adding JavaScript: To load JavaScript, include the <script>
tag before the closing <body>
tag:
<body>
<!-- Your content here -->
<script src="{{ url_for('static', filename='js/scripts.js') }}"></script>
</body>
3. Displaying Images: To display an image, use the <img>
tag and point to your image in the /static/images
folder:
<img src="{{ url_for('static', filename='images/logo.png') }}" alt="Logo">
Using static files like CSS and JavaScript allows you to style your first web app and add functionality without hardcoding everything into your HTML. Flask handles these static files efficiently, ensuring they’re served to the user when they load the page.
Table: Flask Project Structure with Static Files
Folder | Purpose | Example File |
---|---|---|
/templates | Stores HTML templates | index.html |
/static/css | Stores CSS files | styles.css |
/static/js | Stores JavaScript files | scripts.js |
/static/images | Stores image files | logo.png |
As you continue developing your Web App with Python and Flask, mastering the use of Flask templates and static files will significantly enhance your app’s design and interactivity. Whether you’re building a simple landing page or a complex Web App with Python and Flask, Flask’s flexibility makes it easy to create something functional and beautiful.
Setting Up Advanced Features in Flask
Flask Forms and User Input Handling
Handling user input is a key aspect of building any interactive first web app. In Flask, working with forms is made easy through its built-in support for request handling. Whether you’re gathering user information, logging in, or submitting data, Flask forms allow you to handle form data securely and efficiently.
Creating HTML Forms
In Flask, you create forms using standard HTML. Here’s a simple example of an HTML form that allows users to submit their name:
<form method="POST" action="/submit">
<label for="name">Your Name:</label>
<input type="text" id="name" name="name">
<input type="submit" value="Submit">
</form>
In this form:
- The method is set to
POST
, meaning the form data will be sent to the server for processing. - The action attribute points to the
/submit
route in the Flask app, where the form will be handled.
Processing Form Data Using Flask’s Request Object
When the form is submitted, Flask uses the request
object to handle the incoming data. Here’s how you can process the form data in your first Web App with Python and Flask:
from flask import Flask, request, render_template
app = Flask(__name__)
@app.route('/')
def index():
return render_template('form.html')
@app.route('/submit', methods=['POST'])
def submit_form():
user_name = request.form['name']
return f"Hello, {user_name}! Thanks for submitting the form."
if __name__ == "__main__":
app.run(debug=True)
In this example:
- The
request.form['name']
retrieves the value submitted in the name field of the form. - The response dynamically greets the user by the name they submitted.
This method provides a secure way to handle form data in Flask. Flask also supports validation, but for now, we’ve kept the example simple. The same concept applies to any form you’ll use in your first web app.
Connecting Flask to a Database
To build dynamic web applications, you’ll often need to store data in a database. Flask supports several databases, but SQLite is one of the easiest to set up and is great for your first Web App with Python and Flask. Additionally, SQLAlchemy is a popular ORM (Object-Relational Mapping) library that integrates seamlessly with Flask, allowing you to interact with your database using Python.
Introduction to SQLAlchemy
SQLAlchemy acts as a bridge between Flask and the database. Instead of writing raw SQL queries, you use Python code to define tables and relationships, which makes your code more readable and easier to maintain. Let’s get started by installing SQLAlchemy:
pip install Flask-SQLAlchemy
Setting up a SQLite Database
To connect Flask to an SQLite database using SQLAlchemy, first set up your app.py:
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///mydatabase.db'
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(50))
if __name__ == "__main__":
db.create_all()
app.run(debug=True)
In this example:
- app.config[‘SQLALCHEMY_DATABASE_URI’] points to your SQLite database file.
- The
User
class defines a simple table with two fields:id
andname
.
The command db.create_all()
will generate the User
table in the database the first time the app runs.
Basic CRUD Operations
CRUD (Create, Read, Update, Delete) operations allow you to manipulate data in the database. Here’s how you can perform these actions using Flask SQLAlchemy:
- Create a new record:
new_user = User(name="John Doe")
db.session.add(new_user)
db.session.commit()
2. Read from the database:
user = User.query.filter_by(name="John Doe").first()
print(user.name)
3. Update a record:
user = User.query.filter_by(name="John Doe").first()
user.name = "Jane Doe"
db.session.commit()
4. Delete a record:
user = User.query.filter_by(name="Jane Doe").first()
db.session.delete(user)
db.session.commit()
Now that you’ve connected your first web app to a database, you can easily manipulate and store data using these CRUD operations.
Flask Blueprints for Modular Applications
As your first Web App with Python and Flask grows larger, managing routes and views in a single file can become difficult. This is where Flask Blueprints come in handy. Blueprints allow you to split your app into smaller, more manageable modules, making your project easier to maintain.
Why Use Blueprints for Large Projects
When you’re working on a larger web application, it’s important to keep things modular. Using Flask Blueprints lets you break down your app into smaller, logical pieces. For example, you can separate your user management, blog posts, and admin dashboard into different modules.
Benefits of using Blueprints:
- They make it easier to organize routes.
- Code becomes more modular and reusable.
- Teams can work on different parts of the app without stepping on each other’s toes.
Setting up Blueprints for Modular Application Structure
To use Flask Blueprints, create a separate Python file for each section of your app. Let’s walk through setting up a users blueprint.
- Create a Blueprint in a file called
users.py
:
from flask import Blueprint
users = Blueprint('users', __name__)
@users.route('/profile')
def profile():
return "This is the user profile page."
2. Register the Blueprint in your main app.py file:
from flask import Flask
from users import users
app = Flask(__name__)
app.register_blueprint(users, url_prefix='/users')
if __name__ == "__main__":
app.run(debug=True)
In this example:
- The
users
blueprint is registered under the/users
URL prefix, meaning that when you visit/users/profile
, you’ll see the profile page.
Using Blueprints is a great way to keep your first Web App with Python and Flask organized and scalable, especially as your app grows.
Summary Table: Key Flask Concepts
Topic | Description |
---|---|
Flask Forms | Creating HTML forms and handling user input using Flask’s request object. |
Flask with SQLite | Setting up a SQLite database in Flask using SQLAlchemy. |
CRUD Operations | Performing Create, Read, Update, and Delete operations on database records. |
Flask Blueprints | Modularizing your app by dividing it into smaller, reusable components. |
By handling Flask forms, managing databases with SQLAlchemy, and organizing your app using Flask Blueprints, you’re well on your way to building an organized, efficient, and scalable first Web App with Python and Flask. To take your app further, check out our related tutorials on Flask Forms and Flask Authentication to add more functionality to your web applications!
Latest Advancements and Best Practices in Flask
Asynchronous Flask with ASGI Support
In recent times, Flask has introduced async support, making it easier to handle asynchronous tasks. This is especially helpful if you’re working on real-time applications or projects with high concurrency needs, like chat apps or live data updates for your first web app. Traditionally, Flask worked synchronously, which means it would process one request at a time. With the addition of ASGI (Asynchronous Server Gateway Interface) support, Flask can now integrate with ASGI servers like Uvicorn to handle multiple requests concurrently.
Understanding Flask Async Routes
Before, Flask worked synchronously, so each request would wait for the previous one to finish. But now, with async routes, you can handle long-running tasks without blocking the entire app. Here’s an example of how you can create an async route in your Flask async app:
from flask import Flask
import asyncio
app = Flask(__name__)
@app.route('/async')
async def async_route():
await asyncio.sleep(2)
return "This is an asynchronous response!"
In this code:
- The
async
keyword is used to define an asynchronous function. await asyncio.sleep(2)
pauses the response for 2 seconds, simulating a non-blocking task.
Integrating Flask with ASGI Servers
To run your Flask async app using an ASGI server like Uvicorn, you’ll need to install the ASGI server and use the asgi.run()
method. Here’s a quick guide to setting up ASGI Flask support:
- Install Uvicorn:
pip install uvicorn
2. Modify your app.py to work with ASGI:
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="127.0.0.1", port=5000)
Now, your first Web App with Python and Flask can handle asynchronous routes and multiple requests more efficiently using ASGI support.
Flask Security Best Practices
When developing your first Web App with Python and Flask, it’s crucial to keep Flask security in mind to protect your app and its users. Flask provides several ways to secure your application from common vulnerabilities like Cross-Site Request Forgery (CSRF) and SQL injection.
Using Flask Extensions for Security
Flask offers extensions that make adding security to your app easier. Two popular ones are Flask-Login and Flask-WTF.
- Flask-Login helps manage user sessions, allowing you to authenticate users and secure specific routes. Here’s an example of how to use it in your app:
from flask_login import LoginManager, UserMixin, login_user, logout_user, login_required
app = Flask(__name__)
login_manager = LoginManager(app)
class User(UserMixin):
pass
@login_manager.user_loader
def load_user(user_id):
# Logic to load user from database
return User()
- Flask-WTF provides form validation and CSRF protection. When building forms, this extension ensures that your app is protected from CSRF attacks by automatically generating a CSRF token.
Protecting Against Common Vulnerabilities
Some of the most common vulnerabilities in web applications include:
- SQL Injection: To avoid this, always use parameterized queries or an ORM like SQLAlchemy to interact with your database. Example:
# Using SQLAlchemy to protect against SQL injection
user = User.query.filter_by(name="John").first()
- CSRF (Cross-Site Request Forgery): By using Flask-WTF, CSRF tokens are automatically added to forms to protect against malicious form submissions from other sites.
By following these practices, your first web app will be much more secure and better equipped to handle real-world security threats.
Flask and Docker: Containerizing Your Flask App
If you’re looking to make your first Web App with Python and Flask scalable and easy to deploy across different environments, Dockerizing your Flask app is the way to go. Docker allows you to package your application and all its dependencies into a container, making it easy to run anywhere.
How to Create a Dockerfile for Flask
To containerize your Flask app, the first step is to create a Dockerfile. The Dockerfile is essentially a set of instructions that tells Docker how to build your container. Here’s a basic Dockerfile for a Flask Docker setup:
# Use the official Python image from DockerHub
FROM python:3.9
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any necessary dependencies
RUN pip install -r requirements.txt
# Make port 5000 available to the world outside this container
EXPOSE 5000
# Define environment variable
ENV FLASK_APP=app.py
# Run the Flask app
CMD ["flask", "run", "--host=0.0.0.0"]
In this Dockerfile:
- The Python 3.9 image is used as a base.
- The working directory is set to
/app
, and all project files are copied into it. - Dependencies are installed via
requirements.txt
. - Port 5000 is exposed, as Flask runs on this port by default.
Building and Running Your Flask App in a Docker Container
Once your Dockerfile is ready, you can build and run the Docker container:
- Build the Docker image:
docker build -t flask-app .
2. Run the Docker container:
docker run -p 5000:5000 flask-app
Now your first Web App with Python and Flask is running inside a Docker container and can be deployed anywhere, whether on your local machine or a cloud service like AWS.
Summary Table: Key Flask Concepts
Topic | Description |
---|---|
Flask Async Support | Using ASGI servers like Uvicorn for handling async routes. |
Flask Security | Securing your app with Flask extensions and preventing vulnerabilities. |
Dockerizing Flask | Packaging your Flask app into a container for easy deployment. |
By learning how to integrate asynchronous Flask support, applying Flask security best practices, and Dockerizing your Flask app, you can make your first web app scalable, efficient, and secure.
Deploying a Flask Application
Deploying Flask on Heroku
When you’re ready to take your first Web App with Python and Flask live, deploying it to Heroku is one of the simplest ways to get your Flask app up and running. Heroku is a cloud platform that makes it easy to deploy and host web applications without needing to manage infrastructure.
Setting up a Procfile and Runtime
To deploy your Flask app to Heroku, you need two important files: the Procfile and the runtime.txt.
- Procfile: This tells Heroku how to run your app. Create a file named
Procfile
in the root directory of your project with the following content:
web: gunicorn app:app
- gunicorn is a production-level server for Flask apps, and
app:app
refers to the entry point of your Flask app (e.g.,app.py
).
runtime.txt: This file specifies the Python version for your app. For example:
With these two files in place, you’re almost ready to deploy.
Pushing Your Flask App to Heroku Using Git
- First, create a Heroku account and install the Heroku CLI.
- After that, log in using the command:
heroku login
3. Initialize a Git repository if you haven’t done so already:
git init
4. Commit your changes and push the app to Heroku:
heroku create
git add .
git commit -m "Deploying my first web app"
git push heroku master
Now, your Flask app is live on Heroku! To check it out, just visit the URL provided by Heroku after the successful deployment.
Deploying Flask on AWS (EC2)
If you’re looking for more control and flexibility for your first Web App with Python and Flask, deploying on AWS EC2 can be a great option. EC2 allows you to rent virtual servers (called instances) and customize them according to your needs.
Setting up an EC2 Instance
- Launch an EC2 instance:
- Go to the AWS Management Console, navigate to EC2, and launch a new instance.
- Choose a Linux AMI (Amazon Machine Image), and select the instance type (t2.micro works well for small Flask apps).
- Connect to your instance: Once the instance is running, connect via SSH:
ssh -i "your-key.pem" ec2-user@your-ec2-ip
Installing Flask and Setting Up Nginx
Once connected, install Flask and set up your environment:
- Install Python and Flask:
sudo yum update
sudo yum install python3
pip3 install Flask
Set up a reverse proxy with Nginx to route traffic to your Flask app:
- Install Nginx:
sudo yum install nginx
sudo systemctl start nginx
Configure Nginx to forward requests to your Flask app. Edit the Nginx configuration file located at /etc/nginx/nginx.conf
and add:
server {
listen 80;
server_name your-domain.com;
location / {
proxy_pass http://127.0.0.1:5000;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
}
}
3. Start your Flask app:
flask run --host=0.0.0.0
Now, your Flask app should be accessible via the public IP of your EC2 instance. This setup allows you to scale and manage your first Web App with Python and Flask with greater flexibility.
Using Gunicorn for Production
When you’re running your Flask app in a production environment, using Gunicorn ensures that your application handles multiple requests efficiently. Gunicorn is a Python WSGI HTTP server that’s well-suited for running Flask apps in production.
Setting up Gunicorn for Flask
- Install Gunicorn:
pip install gunicorn
2. Modify your Procfile (if using Heroku) or run Gunicorn directly for other setups. For instance, on EC2 or local development, you can run:
gunicorn --workers 3 app:app
Running Flask with Gunicorn
Once Gunicorn is installed, run it to serve your first Web App with Python and Flask efficiently:
gunicorn app:app --bind 0.0.0.0:5000
This command starts your Flask app with Gunicorn, allowing it to handle multiple requests simultaneously in a more production-ready manner.
Summary Table: Deployment Options for Flask
Deployment Option | Steps Involved |
---|---|
Heroku | Create a Procfile, push to Heroku with Git. |
AWS EC2 | Launch EC2, install Flask and Nginx, run your app. |
Gunicorn | Install Gunicorn, run it to handle multiple requests efficiently. |
Deploying your first web app is a crucial step in learning web development. Whether you choose Heroku for simplicity, AWS EC2 for flexibility, or Gunicorn for a production-ready setup, you can confidently share your Flask app with the world.
Debugging and Testing Your Flask Application
Enabling Flask Debug Mode
When you’re building your first Web App with Python and Flask, enabling debug mode is crucial for troubleshooting issues. Debug mode helps you quickly identify errors and see live updates without restarting your server. For many developers, enabling debug mode is like having an assistant that immediately points out what’s wrong.
How to Enable Debug Mode
To enable Flask debug mode, you simply need to modify the configuration of your Flask app. You can do this either programmatically or by setting an environment variable.
- Programmatically:
- Inside your Flask app script, add:
app = Flask(__name__)
app.config['DEBUG'] = True
- This line of code ensures that debug mode is active whenever the app runs.
2. Via environment variable:
- In your terminal, set the environment variable before running your app:
export FLASK_ENV=development
- This enables both debug mode and the built-in Flask development server, which is optimized for testing and local development.
Using Flask’s Built-in Debugger
Flask comes with a built-in debugger that displays detailed error messages when something goes wrong. When you encounter a bug, Flask presents a stack trace with the specific error and line number, allowing you to pinpoint the issue instantly.
Here’s an example of a stack trace in debug mode:
Traceback (most recent call last):
File "app.py", line 10, in <module>
print(1/0) # Intentional error
ZeroDivisionError: division by zero
In this case, Flask helps you identify the exact place where the error occurs, making it easier to resolve bugs quickly.
Unit Testing Your Flask Application
Testing your first Web App with Python and Flask is one of the best ways to ensure that everything works as expected. Unit tests allow you to check if individual components of your application (like routes or functions) behave as they should.
Writing Unit Tests for Flask Routes
Flask integrates seamlessly with testing tools like pytest, allowing you to write unit tests for your app’s routes. When you’re building a Web App with Python and Flask, it’s essential to test each route to ensure it’s returning the correct status code and data.
Here’s an example of how you can write a unit test for a simple route in Flask:
- Flask Route:
- Assume you have this basic route in your app:
@app.route('/hello')
def hello():
return "Hello, World!"
2. Writing the Test:
- To test the route, you can write a unit test like this using pytest:
from app import app
import pytest
@pytest.fixture
def client():
with app.test_client() as client:
yield client
def test_hello_route(client):
response = client.get('/hello')
assert response.status_code == 200
assert b"Hello, World!" in response.data
This unit test ensures that the /hello
route returns a 200 OK response and contains the correct string in the response data. Running this test using pytest will give you confidence that your Flask microframework is functioning correctly for this specific route.
Using Pytest for Testing Flask Applications
To run your tests, simply install pytest:
pip install pytest
Then, run the tests with:
pytest
Pytest will automatically detect and run your test functions. It’s a fantastic way to keep track of the quality of your first Web App with Python and Flask and ensure that everything behaves correctly as you add more features.
You can also extend the testing to cover things like:
- Form validation: Ensure your forms handle inputs correctly.
- Authentication flows: Test login and session handling.
- Database interactions: Verify data is correctly saved and retrieved from the database.
Summary Table: Flask Debug Mode vs. Unit Testing
Feature | Description |
---|---|
Debug Mode | Provides live error tracking and automatic reloads. |
Built-in Debugger | Displays stack traces to identify issues in code. |
Unit Testing with Pytest | Helps validate routes and core functionalities. |
Route Testing | Ensures individual routes return correct responses. |
Key Takeaways
- Debug mode in Flask simplifies troubleshooting by giving you real-time error feedback and automatic server reloads, essential for making your first Web App with Python and Flask development process faster.
- Writing unit tests for your Flask routes using pytest allows you to catch bugs early and verify that your app behaves as expected. This is especially useful as your app grows more complex.
- By integrating both debug mode and unit testing into your development workflow, you’ll be on the right track to building a reliable and maintainable Flask app.
Conclusion: Your Flask Application is Now Ready!
You’ve come a long way from setting up your Web App with Python and Flask to debugging, testing, and securing it. Here’s a quick recap of the key steps you’ve taken:
- Enabled Flask Debug Mode: This helped in troubleshooting errors live and ensured you could work efficiently.
- Wrote Unit Tests: Using pytest, you validated that your Flask routes and core functions behave as expected, making your app more reliable.
- Explored Flask’s Built-in Tools: Whether it’s error handling or testing, you now know how to use Flask’s powerful built-in features.
At this stage, your Flask application is fully functional, and you’re equipped with the tools to handle errors, test your app, and keep things secure. But don’t stop here! Flask has a lot more to offer, and you can continue improving your project by exploring Flask’s official documentation. There, you’ll find advanced features like asynchronous programming, extending Flask with Flask-RESTful, and learning more about database integrations.
With your first web app ready and running, it’s time to dive deeper into the world of Python web development and continue building bigger, more complex applications.
FAQ
Flask is a lightweight Python web framework that is easy to set up and perfect for building your first web application. It’s flexible and ideal for learning web development basics without too much complexity.
After setting up your Flask app, you can run it locally by using the flask run
command. By default, it will be accessible at localhost:5000
in your browser.
Yes! Flask integrates well with databases like SQLite and SQLAlchemy, which allow you to perform CRUD operations and manage data within your app easily.
You can deploy your Flask app using platforms like Heroku, AWS EC2, or by containerizing it with Docker. Each platform has a different setup process, but Flask works smoothly across them all.
External Resources
- The official Flask documentation is a great place to start. It covers everything from setting up Flask to advanced topics like authentication, deployment, and blueprints. You’ll find tutorials, explanations, and code examples to guide you through your first Flask project.
Python.org Flask Web Development Guide
- Python’s official website offers a brief guide on using Flask to create web applications. It’s a quick introduction to running Flask with Python.
Heroku Flask Deployment Documentation
- If you’re planning to deploy your Flask app, this official Heroku guide explains how to set up and push your Flask project to the cloud using Git.
Leave a Reply