Introduction
Arithmetic Operators in Python: Alright, let’s start with the basics—you need to know how to do math in Python. Don’t worry, I’ll walk you through it step by step.
When you’re writing code, you’re going to deal with numbers a lot. You’ll need to add, subtract, multiply, divide, and do a few other things like find remainders or work with powers. Python makes this easy, but you need to know the right symbols and how they work.
In this guide, I’m going to teach you everything you need to know about arithmetic operations in Python. We’ll look at real examples, and I’ll explain each one. By the end, you’ll be able to use these operations in your own projects with confidence.
Ready to learn? Let’s start with the basics.

What Are Arithmetic Operators in Python?
Arithmetic operators in Python are symbols that help you do basic math—just like the ones you use in regular math class. They let you add, subtract, multiply, divide, and more.
Definition of Arithmetic Operators
An arithmetic operator is a symbol that performs a math operation between two values or variables.
Here are the most common ones:
Operator | Meaning | Example |
---|---|---|
+ | Addition | 5 + 3 → 8 |
- | Subtraction | 9 - 4 → 5 |
* | Multiplication | 6 * 2 → 12 |
/ | Division | 10 / 2 → 5.0 |
% | Modulus (Remainder) | 7 % 3 → 1 |
** | Exponentiation (Power) | 2 ** 3 → 8 |
// | Floor Division | 7 // 2 → 3 |
These are built into Python, and you can use them directly in your code without importing anything.
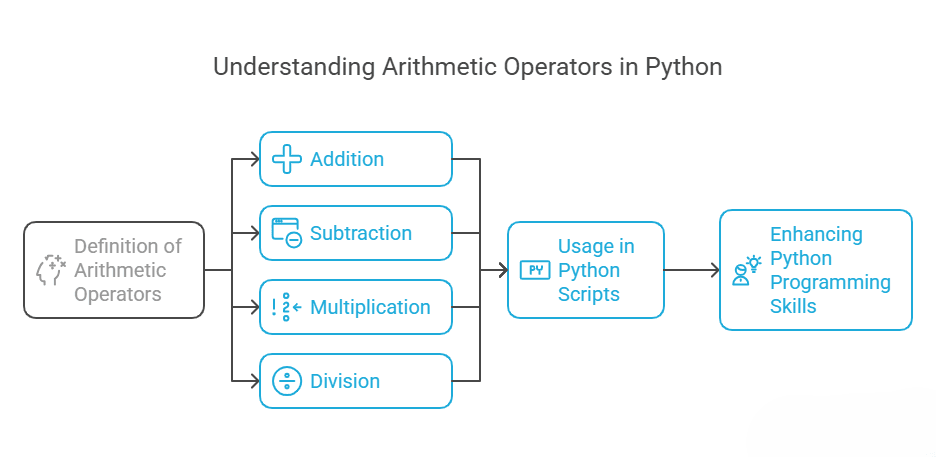
Where They Are Used in Python Scripts
You’ll use arithmetic operators everywhere when working with numbers in Python:
- Math calculations like totals, averages, or discounts
- Loops and conditions where you check or update values
- Data analysis when processing or cleaning up numbers
- Game development to handle scores, movements, or timing
- Machine learning to compute loss, accuracy, or scaling values
Basically, any time your code is doing math, you’re using arithmetic operators behind the scenes.
Using Arithmetic Operators in Python with Examples
Let’s explore how to use these operators in actual Python code. I’ll explain each one with examples so you can try them yourself.
1. Addition Operator (+
) in Python
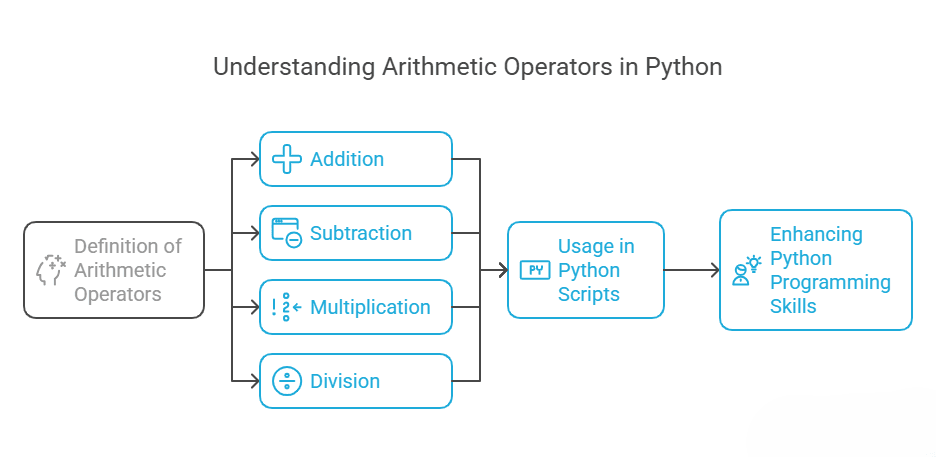
The +
operator is used to add two numbers. But it also has a bonus use—it can join strings together too. Let’s look at both.
Basic Addition in Python Code
You can add two numbers directly like this:
a = 5 + 3
print(a)
Output:
8
In this example, we’re storing the result of 5 + 3
in the variable a
, and then printing it. Simple, right?
You can also add variables together:
x = 10
y = 4
result = x + y
print(result)
Output:
14
Adding Strings with +
(Concatenation)
Here’s something cool—Python also uses the +
operator to join strings. This is called concatenation.
first_name = "John"
last_name = "Doe"
full_name = first_name + " " + last_name
print(full_name)
Output:
John Doe
So when you’re working with text instead of numbers, +
combines the strings into one. Just make sure both sides are strings, or you’ll get an error.
2. Subtraction Operator (-
) in Python
The -
operator is used to subtract one number from another. It’s just like regular math, and Python makes it super easy.
Basic Subtraction in Python Code
Here’s a simple example:
a = 10 - 4
print(a)
Output:
6
You can also subtract variables:
x = 20
y = 7
difference = x - y
print(difference)
Output:
13
This is handy whenever you need to find how much is left, how far apart two values are, or reduce a value inside a loop.
Mixing Variables and Direct Values
You can also mix both:
x = 50
result = x - 12
print(result)
Output:
38
Python is flexible with this, as long as you’re working with numbers.
Can You Use -
with Strings?
Short answer: No. The subtraction operator only works with numbers. If you try to subtract strings like this:
result = "hello" - "h"
Python will throw an error:
TypeError: unsupported operand type(s) for -: 'str' and 'str'
So stick to numbers when using -
.
3. Multiplication Operator (*
) in Python
The *
operator is used to multiply numbers. Just like you do in regular math, but here you can also use it with strings (yes, strings!).
Multiplication with Direct Values
Here’s a simple multiplication:
result = 7 * 3
print(result)
Output:
21
Python multiplies 7
and 3
and gives you the result—easy!
Multiplication with Variables
Now let’s use variables:
a = 6
b = 4
product = a * b
print(product)
Output:
24
This is great for when you’re working with dynamic values in your code.
Mixing Variables and Direct Values
You can mix both:
x = 9
result = x * 2
print(result)
Output:
18
Python handles this perfectly, no extra steps needed.
Bonus: Multiplying Strings with *
Here’s a fun trick. You can use *
to repeat a string:
word = "ha"
laugh = word * 3
print(laugh)
Output:
hahaha
Just remember: the string comes first, and you multiply it by a number.
4. Division Operator (/
) in Python
The /
operator is used to divide one number by another. It always gives you a floating-point (decimal) result, even if the numbers divide perfectly.
Division with Direct Values
Here’s a basic example:
result = 10 / 2
print(result)
Output:
5.0
Notice the result is 5.0
—not 5
. Python gives you a float whenever you divide, just in case you’re working with decimals.
Division with Variables
Let’s do the same with variables:
a = 18
b = 3
quotient = a / b
print(quotient)
Output:
6.0
Even though 18 ÷ 3
is a whole number, Python still returns it as 6.0
.
Mixing Variables and Direct Values
You can mix them like this:
x = 20
result = x / 4
print(result)
Output:
5.0
Again, always a float.
Watch Out: Division by Zero
If you try this:
print(5 / 0)
Python will give you an error:
ZeroDivisionError: division by zero
Important: You can’t divide by zero. Always make sure the number you’re dividing by is not zero.
Example with Uneven Division:
result = 7 / 2
print(result)
Output:
3.5
Here, the division doesn’t come out even, so Python gives the correct decimal result.
Using Variables
a = 9
b = 4
result = a / b
print(result)
Output:
2.25
This is useful when you’re calculating averages, rates, percentages, or anything that needs decimal accuracy.
Summary
/
always gives a float (like2.0
,5.5
,9.25
)- Even if the answer is a whole number, Python still adds
.0
- You don’t need to convert numbers to float manually—it’s automatic
If you ever want the whole number only, you can use floor division (//
), which we’ll cover next.
5. Floor Division Operator (//
) in Python
The //
operator is used for floor division. What’s cool about this operator is that it gives you the largest whole number less than or equal to the result, discarding the decimal part.
In other words, it rounds down to the nearest whole number.
Example with Direct Values
Let’s see it in action:
result = 7 // 3
print(result)
Output:
2
Here, 7 ÷ 3
gives 2.33
in regular division, but with //
, Python drops the .33
and gives you 2
(the floor of 2.33
).
Example with Variables
Now, let’s use variables:
x = 20
y = 6
result = x // y
print(result)
Output:
3
Again, even though 20 ÷ 6
is approximately 3.33
, Python rounds it down to 3
.
Mixed Values (Variables + Direct Numbers)
You can mix variables and direct values:
a = 15
result = a // 4
print(result)
Output:
3
Floor Division with Negative Numbers
Here’s an important note: floor division works with negative numbers too, but it will round down towards the more negative number.
result = -7 // 3
print(result)
Output:
-3
Notice how -7 ÷ 3
is -2.33
, but Python rounds it down to -3
.
Use Case: Getting Clean Results for Loops and Indexes with //
When you’re using loops or working with lists, sometimes you need to divide something in half, but you want a clean, whole number result—no decimals.
That’s where //
comes in.
Example 1: Finding the Middle Index of a List
Let’s say you want to split a list into two parts:
my_list = [10, 20, 30, 40, 50]
middle = len(my_list) // 2
print("Middle index:", middle)
Output:
Middle index: 2
This gives you the exact index number you can use to split the list cleanly:
first_half = my_list[:middle]
second_half = my_list[middle:]
print("First half:", first_half)
print("Second half:", second_half)
Output:
First half: [10, 20]
Second half: [30, 40, 50]
Notice how you didn’t have to worry about decimals. You got a clean index thanks to //
.
Example 2: Using //
in a Loop
Let’s say you want to run a loop only for the first half of a list:
items = ["apple", "banana", "cherry", "date", "fig", "grape"]
half = len(items) // 2
for i in range(half):
print("Item:", items[i])
Output:
Item: apple
Item: banana
Item: cherry
Using //
ensures your loop stops at the right spot, with no errors from decimal numbers.
So whenever you need a clean whole number for indexing, slicing, or splitting, //
is the go-to operator.
6. Modulus Operator (%
) in Python
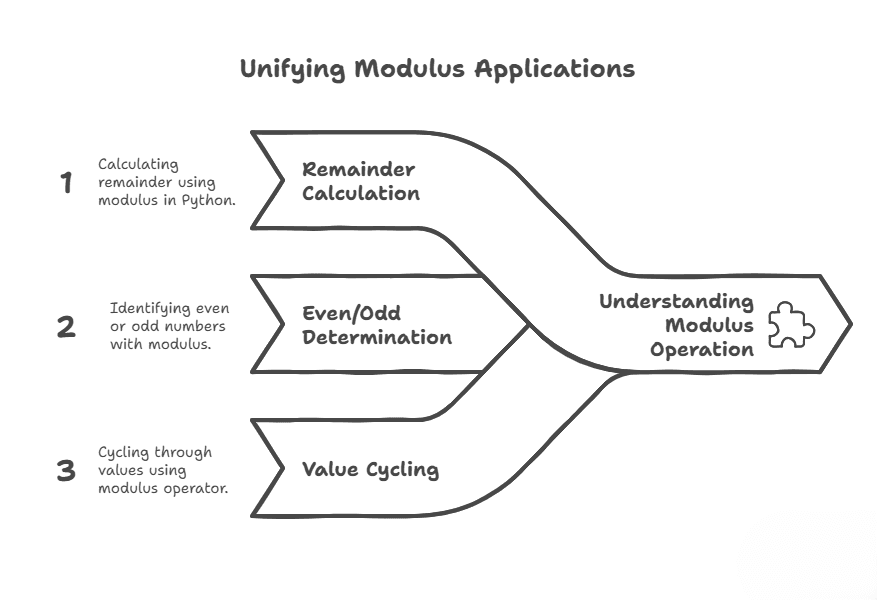
The %
operator gives you the remainder after dividing one number by another.
It’s like asking: “What’s left over?”
Basic Example with Direct Values
result = 10 % 3
print(result)
Output:
1
Here, 10 ÷ 3
is 3
with a remainder of 1—and that’s what %
gives you.
Using Variables
a = 17
b = 5
remainder = a % b
print(remainder)
Output:
2
Because 17 ÷ 5
is 3
with a remainder of 2.
Mix of Variables and Direct Numbers
x = 25
print(x % 4)
Output:
1
Python handles it the same way—it gives you what’s left over after dividing.
Use Case: Checking for Even or Odd Numbers
One of the most common uses of %
is to check if a number is even or odd:
num = 8
if num % 2 == 0:
print("Even number")
else:
print("Odd number")
Output:
Even number
If a number % 2
equals 0
, it’s even. If not, it’s odd.
Use Case: Looping with Conditions
You can also use %
in loops—for example, printing only every third item:
for i in range(1, 11):
if i % 3 == 0:
print(f"{i} is divisible by 3")
Output:
3 is divisible by 3
6 is divisible by 3
9 is divisible by 3
7. Exponentiation Operator (**
) in Python
The **
operator raises a number to the power of another number.
It’s like saying:
“Multiply this number by itself n times.”
Basic Example with Direct Values
result = 2 ** 3
print(result)
Output:
8
This means:2 × 2 × 2 = 8
Using Variables
base = 5
power = 2
result = base ** power
print(result)
Output:
25
This gives you 5 squared
(5 × 5).
Mixing Variables and Direct Values
x = 10
print(x ** 3)
Output:
1000
Python handles this just like a calculator: 10 × 10 × 10 = 1000
Use Case: Square Roots, Cubes, and More
You can also use **
for square roots by raising a number to the power of 0.5
:
num = 16
sqrt = num ** 0.5
print(sqrt)
Output:
4.0
Or cube roots:
cube_root = 27 ** (1/3)
print(cube_root)
Output:
3.0
So if you ever need to square a number, find roots, or raise a number to any power—**
is your tool.
Creating a Simple Calculator with Arithmetic Operators
Let’s walk through it step-by-step.
Step 1: Get Input from the User
We’ll ask the user for:
- First number
- Operator (
+
,-
,*
,/
,//
,%
, or**
) - Second number
Step 2: Use if-elif
to Check the Operator and Perform the Calculation
# Get user input
num1 = float(input("Enter the first number: "))
operator = input("Enter an operator (+, -, *, /, //, %, **): ")
num2 = float(input("Enter the second number: "))
# Perform calculation
if operator == '+':
result = num1 + num2
elif operator == '-':
result = num1 - num2
elif operator == '*':
result = num1 * num2
elif operator == '/':
result = num1 / num2
elif operator == '//':
result = num1 // num2
elif operator == '%':
result = num1 % num2
elif operator == '**':
result = num1 ** num2
else:
result = "Invalid operator!"
print("Result:", result)
Run
Input:
Enter the first number: 10
Enter an operator (+, -, *, /, //, %, **): //
Enter the second number: 3
Output:
Result: 3.0
Let’s upgrade our simple calculator to make it more user-friendly and error-proof.
We’ll add:
- A loop so the calculator keeps running until the user decides to quit
- Error handling for things like dividing by zero or typing the wrong operator
Full Python Script: Smart Calculator with Loop and Error Handling
while True:
print("\n=== Simple Python Calculator ===")
try:
num1 = float(input("Enter the first number: "))
operator = input("Enter an operator (+, -, *, /, //, %, **): ")
num2 = float(input("Enter the second number: "))
if operator == '+':
result = num1 + num2
elif operator == '-':
result = num1 - num2
elif operator == '*':
result = num1 * num2
elif operator == '/':
if num2 == 0:
result = "Error: Cannot divide by zero!"
else:
result = num1 / num2
elif operator == '//':
if num2 == 0:
result = "Error: Cannot divide by zero!"
else:
result = num1 // num2
elif operator == '%':
if num2 == 0:
result = "Error: Cannot use modulus with zero!"
else:
result = num1 % num2
elif operator == '**':
result = num1 ** num2
else:
result = "Invalid operator!"
print("Result:", result)
except ValueError:
print("Error: Please enter valid numbers.")
# Ask if the user wants to continue
cont = input("Do you want to calculate again? (yes/no): ").lower()
if cont != "yes":
print("Goodbye!")
break
How This Helps
- Prevents crashes when user enters wrong input
- Repeats the calculator without restarting the program
- Handles division by zero errors gracefully
- Reinforces how all the arithmetic operators work in one practical project
Using Arithmetic in Conditional Statements
You can use arithmetic operations like +
, -
, *
, /
, %
, and **
inside if
statements to make decisions based on math results.
Example: Calculating Discounts or Price Thresholds
Let’s say you run a small shop and want to give a 10% discount if a customer’s total bill is over ₹500.
bill_amount = float(input("Enter the total bill amount: "))
if bill_amount > 500:
discount = bill_amount * 0.10 # 10% discount
final_amount = bill_amount - discount
print(f"You got a discount of ₹{discount}!")
else:
final_amount = bill_amount
print("No discount applied.")
print(f"Please pay: ₹{final_amount}")
Output Example
Let’s enter:
Enter the total bill amount: 600
The output will be:
You got a discount of ₹60.0!
Please pay: ₹540.0
What’s Happening Behind the Scenes
bill_amount * 0.10
uses the multiplication operatorbill_amount - discount
uses the subtraction operator- Then we check the condition using
if
Arithmetic + condition = smart decision-making!
Common Mistakes with Arithmetic Operations in Python
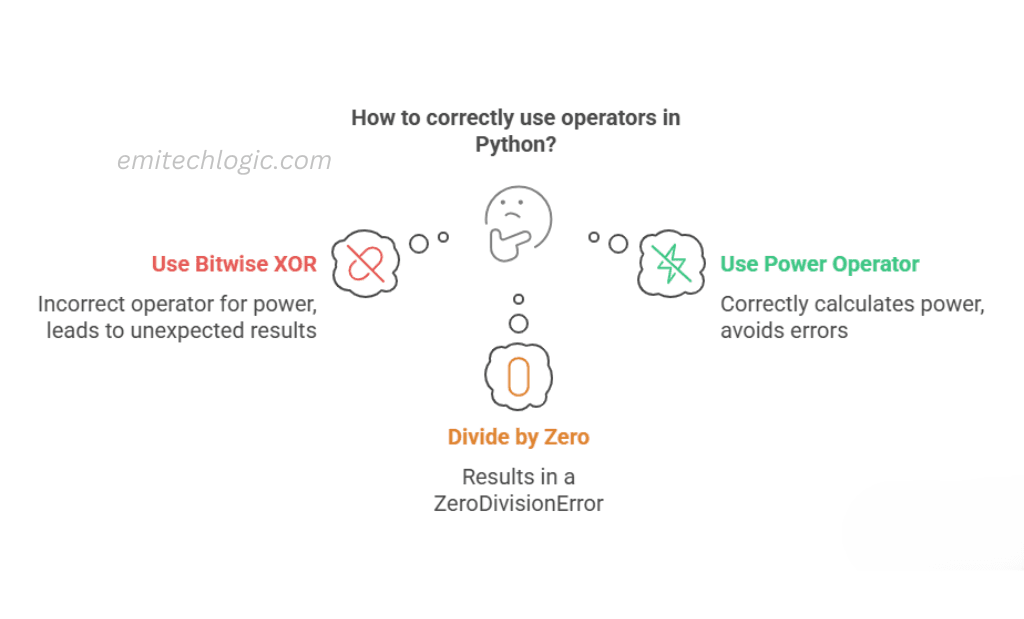
Mixing Integer and Float Data Types
Python can handle both integers (int
) and decimal numbers (float
)—but mixing them can give unexpected results if you’re not careful.
Example: 5 / 2
vs 5 // 2
print(5 / 2) # Float division
print(5 // 2) # Floor division
Output:
2.5
2
/
gives you a float result (decimal).//
gives you an integer result (it chops off the decimal part).
If you want the exact value, use /
.
If you need the whole number only, use //
.
Using the Wrong Operator for Power or Floor Division
Another common mistake is using ^
when you really meant **
.
Example:
print(2 ^ 3) # WRONG if you meant 2 to the power of 3
print(2 ** 3) # CORRECT
Output:
1 # Bitwise XOR, not power!
8 # 2 to the power of 3
^
is bitwise XOR—not exponentiation!**
is the correct operator for powers.
Just remember: use **
for math powers, not ^
.
These are the kinds of mistakes that can silently break your code. If you’re ever confused by an unexpected result, check your operators and whether you’re working with ints or floats.
Must Read
- How to Write a Python Program to Find LCM
- Complete Guide to Find GCD in Python: 7 Easy Methods for Beginners
- How to Set Up CI/CD for Your Python Projects Using Jenkins
- The Ultimate Guide to the range() Function in Python
- How to compute factorial in Python
Tips to Remember Python Arithmetic Operators
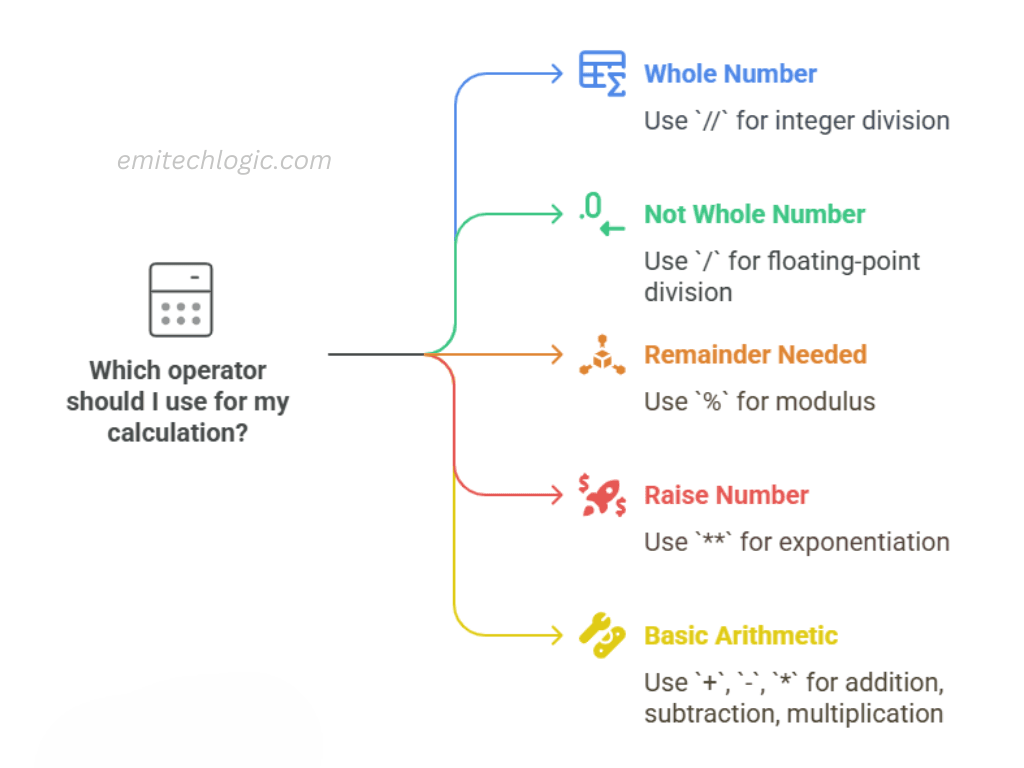
Use Mnemonics or Flashcards
Make it easier to memorize operators with short memory tricks or quick-reference cards.
Here’s a simple mnemonic:
Symbol | Operation | Mnemonic |
---|---|---|
+ | Addition | “Add up the value” |
- | Subtraction | “Take away” |
* | Multiplication | “Star means times” |
/ | Division | “Divide cleanly” |
// | Floor Division | “Double slash = whole number” |
% | Modulus | “Percent left over” |
** | Exponentiation | “Double star = power” |
You can even use flashcards to quiz yourself or others on what each one does.
Practice with Small Code Challenges
The best way to remember is to actually use them.
Try these bite-sized challenges:
- Multiply two user input values
- Write a mini tax calculator
- Print only the remainder of two numbers
- Build a script that raises numbers to powers
Hands-on practice makes things stick faster than just reading.
Understand Output Types (int
vs float
)
Always pay attention to what type of result you’re getting:
print(10 / 2) # float → 5.0
print(10 // 2) # int → 5
Knowing when your result is a decimal or a whole number helps avoid bugs—especially when working with loops, conditions, or indexing.
A little bit of daily practice goes a long way. Even 10 minutes of tinkering with arithmetic operations in Python can build muscle memory fast
Quick Reference: Python Arithmetic Operators Cheat Sheet
Operator | Name | Example | Result |
---|---|---|---|
+ | Addition | 5 + 3 | 8 |
- | Subtraction | 5 - 3 | 2 |
* | Multiplication | 5 * 3 | 15 |
/ | Division | 5 / 2 | 2.5 (float) |
// | Floor Division | 5 // 2 | 2 (int) |
% | Modulus (Remainder) | 5 % 2 | 1 |
** | Exponentiation | 2 ** 3 | 8 |
Notes:
/
always returns a float, even if the result is a whole number.//
returns the whole number part only (no decimal).%
gives the leftover after division.**
is the correct way to do powers (not^
!).
Conclusion
We’ve covered everything you need to understand and use arithmetic operators in Python with confidence.
Here’s a quick recap of what you learned:
- What arithmetic operators are and how they work in Python
- How to use operators like
+
,-
,*
,/
,//
,%
, and**
- Real-world examples like calculators and discount checks
- Common mistakes (like mixing up
^
and**
) - Tips and cheat sheets to help you remember everything
The best way to get comfortable with these operators is to practice. Try building small scripts, run quick calculations, or challenge yourself with mini coding exercises.
Want more beginner-friendly Python tutorials?
Visit emitechlogic.com for hands-on guides, examples, and projects you can actually enjoy learning from.
FAQs on Python Arithmetic Operators
/
and //
in Python? /
performs regular division and always returns a float (even if the result is a whole number).
→ 10 / 2
gives 5.0
//
performs floor division, which means it cuts off the decimal and returns an integer.
→ 10 // 3
gives 3
You can simply use arithmetic operators in your function like this:
def calculate_area(length, width):
return length * width
result = calculate_area(5, 3)
print(result) # Output: 15
You can use +
, -
, *
, /
, etc., to perform any operation you need inside the function body.
Python will raise a ZeroDivisionError if you try to divide by zero using /
, //
, or %
.
print(10 / 0) # ❌ This will cause an error
To handle it safely, use a try-except
block:
try:
result = 10 / 0
except ZeroDivisionError:
print(“Oops! You can’t divide by zero.”)
Further Reading & External Resources
Here are some useful external resources to deepen your understanding of Python arithmetic operators:
- Official Python Docs – Expressions
A detailed look at Python’s expression syntax, including all arithmetic operators. - Corey Schafer’s YouTube Video on Python Operators
A video guide that explains arithmetic and comparison operators with visuals. - Practice Problems – HackerRank Python Operators
Try solving beginner-friendly problems to solidify your knowledge.
Leave a Reply