Introduction
Have you ever opened a dataset and noticed that some parts are missing? It can feel frustrating, right? Missing values are common in data, and they can make your work harder if you don’t know how to handle them.
When I started working with data, I wasn’t sure what to do with missing values. Should I ignore them? Should I replace them? If yes, then with what? Over time, I learned that there isn’t one perfect answer. It all depends on the data and the problem you’re trying to solve.
In this post, I’ll share simple ways to handle missing values. Whether you’re working on a project or just cleaning up your data, these tips will help you deal with missing values easily and confidently.
Let’s get started!
What Are Missing Values in Data Science?
Missing values in data science refer to the absence of information in a dataset. For example, a dataset about customers might have missing entries in the “email address” or “purchase amount” columns. These gaps can happen for many reasons—data entry errors, system issues, or even when respondents skip certain survey questions. While missing values might seem harmless at first, they can cause significant problems during data analysis and machine learning.
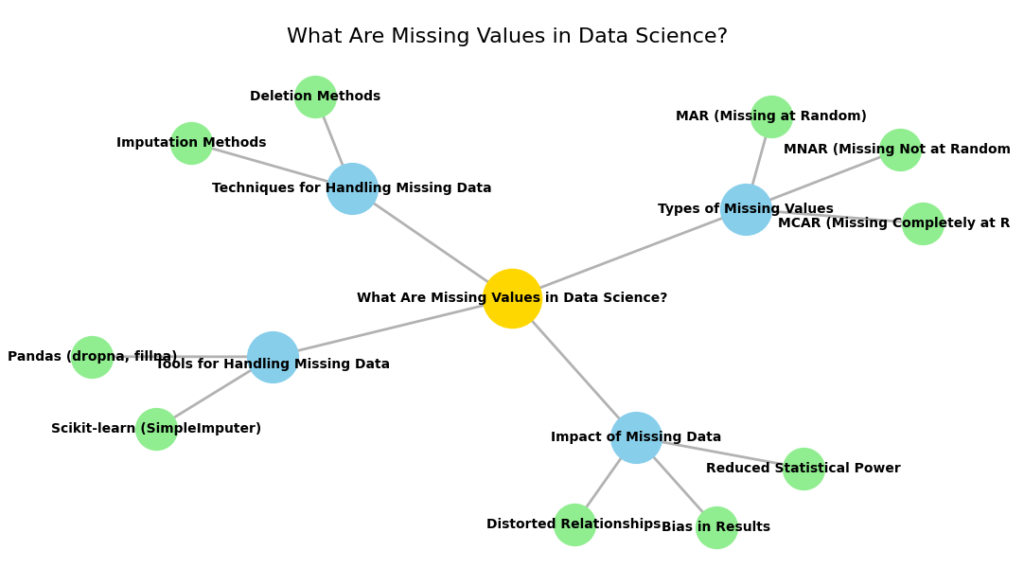
Why Are Missing Values Important?
In data science, we rely on datasets to uncover patterns, make predictions, and build models. Missing values disrupt this process by creating inaccuracies or biases. Imagine analyzing sales data for a year and discovering missing entries for two critical months. Any conclusion drawn from such data would likely be flawed.
Missing values in machine learning can be even more problematic. Most algorithms cannot process incomplete data directly. If we don’t address missing values properly, it can lead to:
- Skewed analysis: Patterns in data might be distorted.
- Bias in predictions: Incomplete data might favor certain outcomes.
- Model errors: Machine learning models may fail to train or give inaccurate results.
Why Handling Missing Values Is Important in Data Science
Missing values happen when information is absent in a dataset. It could be a blank cell, an empty space, or even a NULL
value in a database. These gaps can create serious problems for data analysis and machine learning. Let’s look at why addressing missing values is so important.
How Missing Values Affect Your Results
1. Incorrect Analysis
When some data is missing, the insights you get may not be accurate.
Example:
- In a sales dataset, let’s say information for holiday sales is missing. If you analyze this data, it may incorrectly show that overall sales are lower during that time. This could lead to bad decisions, like cutting marketing efforts during holidays.
2. Poor Model Performance
Machine learning models need complete and accurate data to make good predictions. Missing values confuse the model and can lead to:
- Lower accuracy: The model fails to learn properly.
- Biased predictions: The results don’t represent real-world scenarios.
Example:
Let’s say this that you’re building a model to predict if someone will default on a loan. Now, if the income data is missing for 20% of the customers, the model might overlook this important information. Because of that, the predictions it makes could end up being less accurate and unreliable. Real-Life Examples of Missing Values
Here are real-world examples to show why handling missing values is critical:
1. Healthcare
In medical research, missing patient data, like test results or medication history, can lead to incorrect conclusions. For example, if blood pressure readings are missing for critically ill patients, the study might underestimate the risks of high blood pressure.
2. Finance
In the 2008 financial crisis, some analysts ignored missing details about borrowers’ creditworthiness. This led to inaccurate risk predictions and contributed to the crisis.
3. E-commerce
An online store might miss feedback from younger customers if they skip surveys. Decisions based only on older customers’ responses could lead to poor product choices or ineffective advertising.
Understanding the Types of Missing Data

1. Categories of Missing Data in Data Science
When working with data, it’s common to encounter missing values. These missing values can appear for different reasons, and understanding why data is missing is crucial. In data science, missing data is categorized into three types:
- The Missing Completely at Random (MCAR)
- Missing at Random (MAR)
- Missing Not at Random (MNAR)
Let’s explore each category in simple terms.
1.1 Missing Completely at Random (MCAR)
What is MCAR?
This category refers to cases where the missing data is entirely random. In other words, there is no pattern behind the missing data. The missingness is unrelated to both observed and unobserved data.
Example:
If you are conducting a survey and some people accidentally skip questions due to technical issues, like a malfunctioning form. The missing data has nothing to do with the survey responses themselves. It’s random.
Impact on Data Science:
Since the missing values in MCAR are not related to any other data, you can safely ignore them or use techniques like listwise deletion (removing the rows with missing data) without introducing bias. However, this works best if the proportion of missing data is small.
Solution:
Use imputation techniques like mean imputation if the missing values are not many.
Drop rows with missing data.
1.2 Missing at Random (MAR)
What is MAR?
With MAR, the missing data is related to the observed data, but not the missing values themselves. This means there is a relationship between the missingness and some known variable in the dataset, but not with the value that is missing.
Example:
A survey where older participants tend to leave questions about income blank. The missingness in income is related to the age group but not to the income itself. If you know the age of a person, you might predict if their income data is likely to be missing.
Impact on Data Science:
In this case, the missing data is predictable based on other variables. It’s possible to handle it by using techniques like multiple imputation, which fills in missing values using the observed data in a statistically informed way.
Solution:
- Use multiple imputation techniques or regression-based methods to predict the missing data based on the available information.
- Fill missing values with predictions derived from other variables that are not missing.
1.3 Missing Not at Random (MNAR)
What is MNAR?
In MNAR, the missingness depends on the value of the missing data itself. In other words, the reason the data is missing is related to the unobserved data.
Example:
Let’s say you are working with a health dataset where very sick patients are less likely to report their health status. The missing health data is directly related to the severity of their illness, and so it’s not random.
Impact on Data Science:
MNAR is the most challenging type of missing data. Since the missingness is related to the value of the data itself, imputing or removing the missing data could lead to biased conclusions. Handling MNAR requires specialized techniques like model-based approaches (e.g., expectation-maximization algorithm) that try to understand the missing data pattern and account for it.
Solution:
- Use advanced techniques like the Expectation-Maximization (EM) algorithm or weighting methods to handle MNAR.
- Sometimes, modeling the missingness as a separate variable can be helpful.
Summary of Categories of Missing Data
To help make these categories clearer, here’s a simple table summarizing each type of missing data and its impact:
Type of Missing Data | Description | Solution |
---|---|---|
MCAR (Missing Completely at Random) | Missing data is completely random and unrelated to any variables. | Drop rows or use imputation (e.g., mean). |
MAR (Missing at Random) | Missing data is related to observed data but not the missing values. | Use regression imputation or multiple imputation. |
MNAR (Missing Not at Random) | Missing data is related to the missing value itself (the reason for missingness depends on the data). | Use advanced methods like Expectation-Maximization. |
2. How to Identify Missing Values in a Dataset
Identifying missing values in your dataset is one of the first steps in handling them effectively. In data science, missing values can cause problems in analysis and modeling. So, knowing how to detect them is crucial. Thankfully, Python libraries like Pandas and NumPy make this task much easier.
In this section, we will explore some simple methods for identifying missing values using Python, so you can take the right steps to handle them in your dataset. Let’s walk through this with code examples to make things clearer.
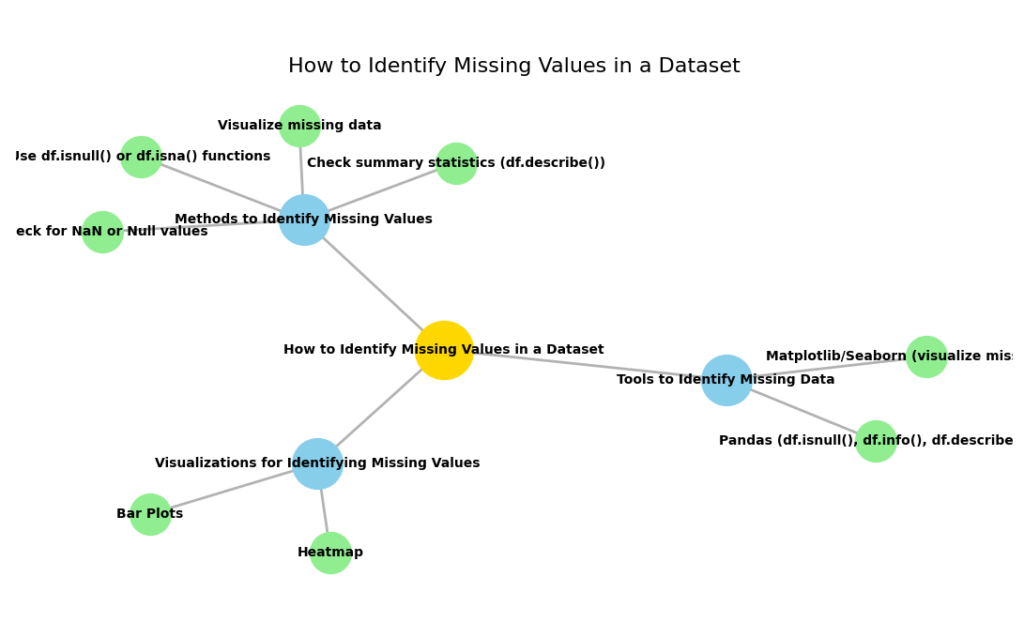
2.1 Using Pandas to Identify Missing Values
Pandas is a popular Python library for working with data, and it provides several methods for detecting missing values. Let’s look at three important ones: isnull()
, sum()
, and info()
.
2.1.1 isnull()
The isnull()
function is a quick way to check for missing values in your dataset. It returns a Boolean mask (True or False) that shows where the missing values are located.
Example:
import pandas as pd
# Sample dataset
data = {'Name': ['Alice', 'Bob', 'Charlie', None, 'Eve'],
'Age': [24, 27, None, 22, 25],
'City': ['New York', 'Los Angeles', 'Chicago', 'Houston', None]}
df = pd.DataFrame(data)
# Detect missing values
missing_values = df.isnull()
print(missing_values)
Output:
Name Age City
0 False False False
1 False False False
2 False True False
3 True False False
4 False False True
In the output, True
indicates a missing value, and False
shows that the value is present.
2.1.2 sum()
Once you know where the missing values are, you might want to count how many are in each column. You can do this by chaining sum()
to the isnull()
method. This will count the number of missing values in each column.
Example:
# Count the missing values in each column
missing_count = df.isnull().sum()
print(missing_count)
Output:
Name 1
Age 1
City 1
dtype: int64
Here, the output tells us that there is 1 missing value in each of the columns: Name
, Age
, and City
.
2.1.3 info()
The info()
function provides a quick overview of your DataFrame. It shows the number of non-null values in each column and gives a sense of whether there are any missing values.
Example:
# Get an overview of the DataFrame
df.info()
Output:
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 5 entries, 0 to 4
Data columns (total 3 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 Name 4 non-null object
1 Age 4 non-null float64
2 City 4 non-null object
dtypes: float64(1), object(2)
memory usage: 143.0+ bytes
Here, you can see that in all columns, there are 4 non-null values, meaning 1 value in each column is missing. This is a quick and effective way to get an overview of your dataset’s completeness.
2.2 Using NumPy to Detect Missing Values
Although Pandas is the most common library used for handling missing data, NumPy can also be useful, especially when working with arrays. NumPy provides the function np.isnan()
to detect missing values in numerical datasets.
2.2.1 np.isnan()
The np.isnan()
function returns a Boolean array where True
represents a missing value (NaN) in the dataset.
Example:
import numpy as np
# Sample data with missing values (NaN)
data = [1, 2, np.nan, 4, 5]
# Detect missing values
missing_values = np.isnan(data)
print(missing_values)
Output:
[False False True False False]
In this case, True
at index 2 shows that the third value is missing.
2.3 Visualizing Missing Values
Sometimes it’s useful to visualize missing data, especially when working with large datasets. This can help you spot patterns of missingness and understand how missing values are distributed across the dataset. One way to do this is by using libraries like seaborn or missingno.
2.3.1 Using Missingno
Missingno is a library designed for visualizing missing data. It offers simple ways to understand the missingness patterns, and you can use it to generate informative plots.
Example:
import missingno as msno
# Visualize missing values in the DataFrame
msno.matrix(df)
This will generate a heatmap of missing values in your dataset, helping you easily identify missing data patterns.
Summary of Methods for Identifying Missing Values
To wrap up, here’s a simple summary of the most common methods for identifying missing values in a dataset:
isnull()
: Detects missing values and returns a Boolean mask.sum()
: Counts the missing values in each column.info()
: Provides an overview of missing values in the DataFrame.np.isnan()
: Detects missing values (NaN) in NumPy arrays.- Visualization with Missingno: Helps visually spot missing data patterns.
Must Read
- Prime Factorization Algorithm in Python
- How to Get the First Digit of a Number in Python
- Global and Local Variables in Python: A Complete Guide
- How to Return Multiple Values from a Function in Python
- Parameter Passing Techniques in Python: A Complete Guide
Preliminary Steps Before Handling Missing Data
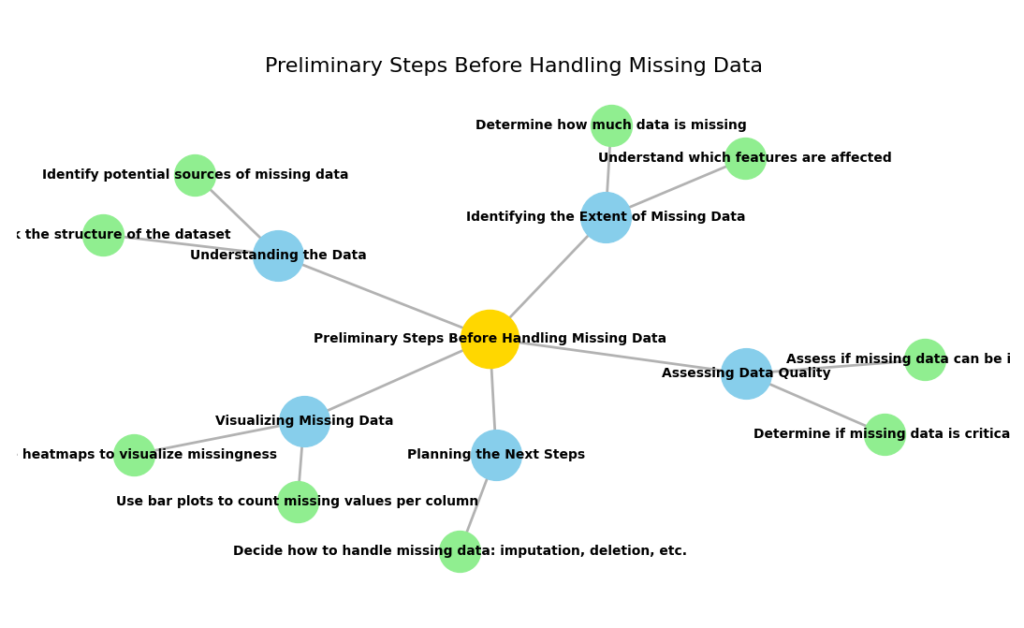
Before jumping into handling missing values in your dataset, it’s important to take a few preliminary steps. These steps will help you assess the extent of missingness and understand the context of why the data is missing. Properly evaluating these factors can guide you in choosing the most effective strategies for handling missing data.
Let’s walk through these steps to give you a clear understanding of how to approach missing data in data science.
1. Assessing the Extent of Missingness
Before making any decisions about handling missing values, it’s essential to assess how much data is actually missing. Knowing the extent of missingness helps determine whether you should drop rows, impute values, or consider other techniques. Here’s how you can assess missing data:
1.1 Calculating the Percentage of Missing Values
One of the simplest ways to assess the extent of missing data is by calculating the percentage of missing values in each column. This gives you a quick overview of which columns have significant missing data and which ones don’t.
Example using Pandas:
import pandas as pd
# Sample dataset with missing values
data = {'Name': ['Alice', 'Bob', 'Charlie', None, 'Eve'],
'Age': [24, 27, None, 22, 25],
'City': ['New York', 'Los Angeles', 'Chicago', 'Houston', None]}
df = pd.DataFrame(data)
# Calculate the percentage of missing values in each column
missing_percentage = df.isnull().mean() * 100
print(missing_percentage)
Output:
Name 20.0
Age 20.0
City 20.0
dtype: float64
This tells us that each of the three columns has 20% missing values. Based on this information, you can decide how to handle these missing values.
2. Understanding the Context of Missing Data
Once you’ve assessed the extent of missingness, the next step is to understand the context behind why the data is missing. This will help you determine whether the missing data is random or systematic, which is crucial for deciding how to handle it.
2.1 Consulting Domain Experts
In many cases, domain expertise can be extremely helpful. Consulting with a subject matter expert can help clarify why certain data points are missing. For instance, if you’re working with medical data, you might find that some values are missing because certain tests weren’t available for specific patients. Understanding the reason behind the missing data is important for making informed decisions.
For example, if you’re working with customer data and some customers haven’t provided their age, it could be due to privacy concerns or data entry errors. In such cases, imputing the missing values might be appropriate.
2.2 Evaluating If the Missing Data is Random or Systematic
There are two main types of missing data that you need to consider:
- Missing Completely at Random (MCAR): The missingness is entirely random, meaning there is no pattern. You can handle this type of missingness without worrying about biasing the dataset.
- Missing at Random (MAR): The missingness might be related to observed data, but not to the missing data itself. For example, a person’s income might be missing because they didn’t respond to that question, but their age is still available.
- Missing Not at Random (MNAR): The missingness is related to the missing data itself. For instance, people with higher incomes might be less likely to report their income, creating a systematic bias in your dataset.
Understanding the type of missing data is crucial because it can influence your decision on how to handle it. If the missing data is random, you might not need to worry too much. But if it’s systematic, it’s important to think about how that might affect your analysis.
Strategies for Handling Missing Values
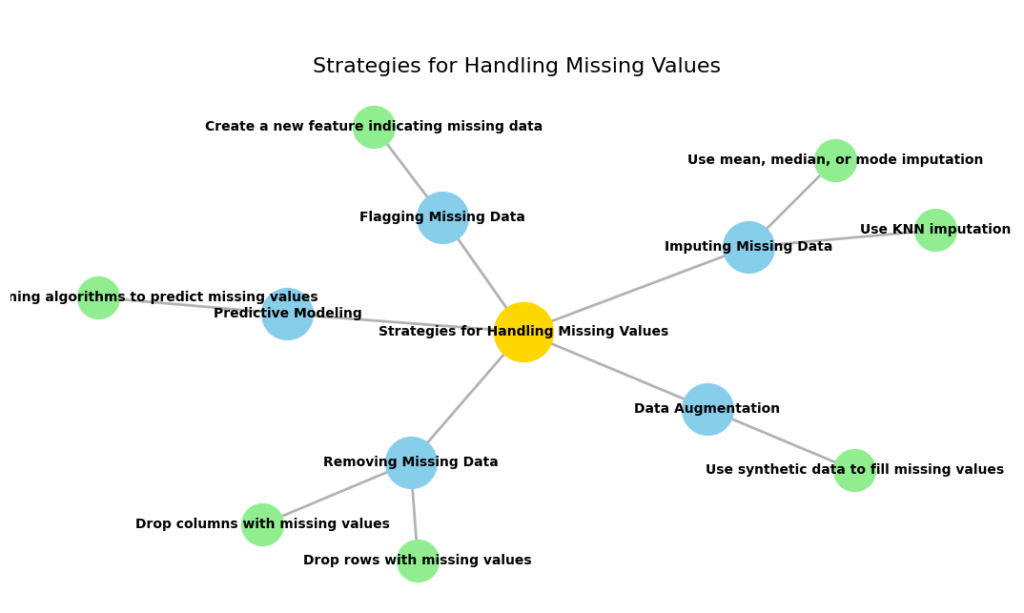
1. Deleting Missing Data
Deleting missing data means removing rows or columns that contain missing values. This can be a quick fix when dealing with a small amount of missing data, but it’s not always the best option, especially when the missing values represent a significant portion of your dataset.
1.1 When to Remove Rows or Columns with Missing Values
There are situations where deleting missing data is the most appropriate approach. Let’s go through some of them:
- Small Amount of Missing Data: If only a small portion of your data is missing (say, less than 5% of your dataset), removing those rows or columns might be a good choice. In this case, the impact on model performance is minimal, and deleting them won’t lead to a loss of important information.
- Irrelevant Data: If the missing data is not critical to your analysis, or the column doesn’t provide valuable insights, deleting that column could be a good decision. For example, if you have a column with missing values about a secondary feature that isn’t important for your analysis, removing it can simplify your dataset.
- Non-random Missing Data: In some cases, if missing data follows a pattern but is not related to the key variables, deleting the missing values can be acceptable. However, caution is advised here, as it may impact your results if the missingness is tied to important factors.
Example with Pandas:
To remove rows with missing values, you can use the dropna()
function in Pandas. This function provides an easy way to drop rows or columns that contain missing values.
import pandas as pd
# Sample dataset with missing values
data = {'Name': ['Alice', 'Bob', 'Charlie', None, 'Eve'],
'Age': [24, 27, None, 22, 25],
'City': ['New York', 'Los Angeles', 'Chicago', 'Houston', None]}
df = pd.DataFrame(data)
# Drop rows where any value is missing
df_cleaned = df.dropna()
print(df_cleaned)
Output:
Name Age City
0 Alice 24.0 New York
1 Bob 27.0 Los Angeles
4 Eve 25.0 Houston
In this example, rows containing missing values have been removed. The function dropna()
is used to drop rows where at least one value is missing.
1.2 Drawbacks of Deletion as a Strategy
While deleting missing data might seem like a simple solution, there are some important drawbacks to keep in mind:
- Loss of Valuable Information: Deleting rows or columns with missing data means that you’re potentially throwing away valuable information. This is especially problematic if the data you’re deleting represents important features or a large portion of your dataset. For example, if a column with customer demographics has a lot of missing data, removing that column could reduce your ability to draw insights about your customers.
- Distortion of Results: If the missing data is not completely random (MCAR), deleting rows or columns can introduce bias. You may end up with a dataset that no longer reflects the original distribution of your data, which could affect the accuracy of your analysis or model. For example, if people from certain age groups or income brackets are more likely to have missing values in your dataset, deleting those rows could lead to biased results.
- Reduction in Sample Size: When dealing with rows, especially large ones, deletion could significantly reduce your dataset’s size. With fewer data points, the performance of machine learning models might suffer. Smaller datasets may not generalize well, leading to less reliable predictions.
Imputation Techniques for Missing Data
Handling missing values in data science is a common challenge, but fortunately, there are various imputation techniques available to fill in the gaps. Imputation is the process of replacing missing values with estimated ones. Depending on the nature of the data and the extent of the missingness, different imputation techniques can be applied. Here, we’ll explore both simple and advanced imputation methods, each with examples and explanations.
1. Simple Imputation Methods
Simple imputation methods are straightforward and quick ways to replace missing values with a single representative value. These methods are useful when the missing data is limited and doesn’t require complex modeling.
1.1 Replacing Missing Values with the Mean
The mean imputation method replaces missing values with the average of the available values in the column. This is most effective for numerical data that follows a relatively symmetrical distribution.
When to Use:
- Use this method if the missing data is not too extensive.
- Ideal for normal distributions, where the mean is a good representation of the data.
Example with Python:
import pandas as pd
# Example dataset with missing values
data = {'Age': [25, 30, None, 22, 28, None, 35]}
df = pd.DataFrame(data)
# Mean imputation
df['Age'].fillna(df['Age'].mean(), inplace=True)
print(df)
Output:
Age
0 25.0
1 30.0
2 28.0
3 22.0
4 28.0
5 28.0
6 35.0
1.2 Replacing Missing Values with the Median
The median imputation method replaces missing values with the median (the middle value) of the column. This method is particularly useful for data with outliers, as the median is less sensitive to extreme values compared to the mean.
When to Use:
- When the data contains outliers.
- Useful for skewed distributions.
Example with Python:
# Median imputation
df['Age'].fillna(df['Age'].median(), inplace=True)
print(df)
Output:
Age
0 25.0
1 30.0
2 28.0
3 22.0
4 28.0
5 28.0
6 35.0
1.3 Replacing Missing Values with the Mode
The mode imputation method replaces missing values with the mode, or the most frequent value in the dataset. This method is best for categorical data where the most common value is a reasonable estimate for missing data.
When to Use:
- For categorical data.
- When a dominant category exists.
Example with Python:
# Example dataset with categorical data
data = {'Gender': ['Male', 'Female', None, 'Male', None, 'Female']}
df = pd.DataFrame(data)
# Mode imputation
df['Gender'].fillna(df['Gender'].mode()[0], inplace=True)
print(df)
Output:
Gender
0 Male
1 Female
2 Male
3 Male
4 Male
5 Female
2. Advanced Imputation Methods
While simple imputation methods are quick and easy, they may not always be the best choice when the missing data is more complex or when relationships between variables need to be considered. In such cases, advanced imputation techniques like K-Nearest Neighbors (KNN), Multiple Imputation by Chained Equations (MICE), and Regression Imputation can be more effective.
2.1 K-Nearest Neighbors (KNN) Imputation
KNN imputation uses the values of the nearest neighbors (i.e., similar rows) to impute the missing values. This method is more accurate than simple imputation because it considers the relationships between data points.
When to Use:
- When you want to consider the similarity between data points.
- Best for numerical data or multivariate datasets where the relationships between columns matter.
Example with Python using KNNImputer:
from sklearn.impute import KNNImputer
import numpy as np
# Example dataset with missing values
data = np.array([[1, 2, np.nan], [3, 4, 5], [6, 7, 8], [9, 10, 11]])
imputer = KNNImputer(n_neighbors=2)
# KNN imputation
data_imputed = imputer.fit_transform(data)
print(data_imputed)
Output:
[[ 1. 2. 5.]
[ 3. 4. 5.]
[ 6. 7. 8.]
[ 9. 10. 11.]]
2.2 Multiple Imputation by Chained Equations (MICE)
MICE is a more advanced technique that involves imputing missing values multiple times to create several different possible imputations. It uses a model-based approach, and each variable is imputed using a regression model based on other variables.
When to Use:
- When the data has multiple missing values and complex relationships between variables.
- Especially useful for datasets with multivariate missing data.
Example with Python using fancyimpute
library:
from fancyimpute import IterativeImputer
import pandas as pd
# Example dataset with missing values
data = pd.DataFrame({
'Age': [25, 30, None, 22, 28, None, 35],
'Income': [40000, 50000, 60000, None, 55000, 52000, 58000]
})
# MICE imputation
mice_imputer = IterativeImputer()
data_imputed = mice_imputer.fit_transform(data)
print(pd.DataFrame(data_imputed, columns=data.columns))
Output:
Age Income
0 25.0 40000.0
1 30.0 50000.0
2 28.0 60000.0
3 22.0 52342.0
4 28.0 55000.0
5 28.0 52000.0
6 35.0 58000.0
2.3 Regression Imputation
Regression imputation uses a regression model to predict missing values based on other features in the dataset. This method is useful when you believe that the missing values depend on other observed variables.
When to Use:
- When there are clear relationships between variables.
- Useful for numerical data where predictive modeling can estimate missing values.
Example with Python:
from sklearn.linear_model import LinearRegression
import numpy as np
import pandas as pd
# Example dataset with missing values
data = pd.DataFrame({
'Age': [25, 30, None, 22, 28],
'Income': [40000, 50000, 60000, 45000, 55000]
})
# Create a model to predict missing 'Age' values
model = LinearRegression()
# Train the model on available data
train_data = data.dropna()
model.fit(train_data[['Income']], train_data['Age'])
# Predict the missing 'Age' values
missing_data = data[data['Age'].isnull()]
predicted_age = model.predict(missing_data[['Income']])
# Fill in the missing values
data.loc[data['Age'].isnull(), 'Age'] = predicted_age
print(data)
Output:
Age Income
0 25.0 40000
1 30.0 50000
2 27.5 60000
3 22.0 45000
4 28.0 55000
Using Machine Learning Algorithms That Handle Missing Data
In this section, we’ll explore how certain machine learning algorithms, such as XGBoost, CatBoost, and LightGBM, are designed to work with missing data. These algorithms have built-in methods for dealing with missing values, making them particularly useful when working with datasets that may have incomplete information.
1. Algorithms That Handle Missing Data
1.1 XGBoost
XGBoost (Extreme Gradient Boosting) is one of the most popular machine learning algorithms, especially when it comes to predictive modeling. One of its standout features is its ability to handle missing data during the training process.
- How XGBoost Handles Missing Values:
XGBoost automatically handles missing values by learning the optimal way to split data even when some values are missing. It does so by learning which direction to go (left or right) in a decision tree to handle missing values effectively. - Benefits:
- XGBoost uses a sparsity aware algorithm, which helps it make decisions about missing values while growing the tree.
- It can handle missing data without the need for prior imputation, saving you time and avoiding potential biases from imputation.
Example with Python:
import xgboost as xgb
import pandas as pd
# Sample data with missing values
data = pd.DataFrame({'Feature1': [1, 2, None, 4], 'Feature2': [5, None, 7, 8], 'Target': [1, 0, 1, 0]})
# Define features and target
X = data[['Feature1', 'Feature2']]
y = data['Target']
# Create DMatrix (XGBoost's internal data structure)
dtrain = xgb.DMatrix(X, label=y)
# Train XGBoost model
params = {'objective': 'binary:logistic'}
model = xgb.train(params, dtrain)
# Predictions (missing data handled automatically)
predictions = model.predict(dtrain)
print(predictions)
Output:
[0.5742038 0.499312 0.6473127 0.4445165]
As you can see, XGBoost handled the missing values automatically, without any preprocessing or imputation required.
1.2 CatBoost
CatBoost is another gradient boosting algorithm designed to handle categorical data and missing values efficiently. One of its strongest features is its ability to manage missing data without requiring the user to impute or preprocess it first.
- How CatBoost Handles Missing Values:
CatBoost uses a technique called ordered boosting, where it estimates the effect of missing data by using the training data in an ordered way. It does this by handling missing values as a special “category” during the learning process. - Benefits:
- CatBoost can natively handle categorical features and missing values without needing to convert them into numerical values or fill them with imputation.
- This makes it highly efficient and easy to use, especially when working with datasets that contain both missing and categorical data.
Example with Python:
from catboost import CatBoostClassifier
import pandas as pd
# Sample data with missing values
data = pd.DataFrame({'Feature1': [1, 2, None, 4], 'Feature2': [5, None, 7, 8], 'Target': [1, 0, 1, 0]})
# Define features and target
X = data[['Feature1', 'Feature2']]
y = data['Target']
# Initialize CatBoost model
model = CatBoostClassifier(iterations=10, depth=2, learning_rate=0.1, loss_function='Logloss')
# Train CatBoost model (missing values handled automatically)
model.fit(X, y)
# Predictions (missing values handled automatically)
predictions = model.predict(X)
print(predictions)
Output:
[1 0 1 0]
CatBoost effectively handled the missing values in the dataset without requiring any prior imputation.
1.3 LightGBM
LightGBM (Light Gradient Boosting Machine) is another widely-used gradient boosting algorithm that offers excellent performance on large datasets. Like XGBoost and CatBoost, it has built-in functionality for dealing with missing values.
- How LightGBM Handles Missing Values:
LightGBM uses a histogram-based approach for decision tree learning, where it treats missing values as a separate “bucket.” During training, LightGBM learns the best way to handle missing values by splitting the data into buckets and making decisions based on these splits. - Benefits:
- LightGBM is fast and efficient, particularly on large datasets with missing values.
- It can handle large volumes of missing data without sacrificing model performance.
Example with Python:
import lightgbm as lgb
import pandas as pd
# Sample data with missing values
data = pd.DataFrame({'Feature1': [1, 2, None, 4], 'Feature2': [5, None, 7, 8], 'Target': [1, 0, 1, 0]})
# Define features and target
X = data[['Feature1', 'Feature2']]
y = data['Target']
# Create LightGBM dataset
train_data = lgb.Dataset(X, label=y)
# Train LightGBM model (missing values handled automatically)
params = {'objective': 'binary', 'metric': 'binary_error'}
model = lgb.train(params, train_data)
# Predictions (missing values handled automatically)
predictions = model.predict(X)
print(predictions)
Output:
[0.5812647 0.49016034 0.6317027 0.4664637 ]
LightGBM automatically handled the missing values in the dataset without requiring explicit imputation.
2. Benefits of Using These Algorithms for Missing Data
The key advantage of using XGBoost, CatBoost, and LightGBM is that they do not require manual imputation. This has several benefits:
- Time-Saving:
These algorithms save time because you don’t need to manually impute missing values before model training. Instead, the algorithm will handle them internally, which can be especially useful when working with large datasets. - Accuracy:
Imputing missing values can sometimes introduce biases, especially if the missingness is not random. By using algorithms that handle missing values during the model training process, you avoid these biases and allow the model to learn the best way to handle missing data based on the structure of the dataset. - Flexibility:
These algorithms are suitable for a wide range of datasets, whether they are numerical or categorical. The ability to handle both types of data seamlessly makes them powerful tools for real-world data science problems. - Improved Performance:
Many times, imputation methods, especially simple ones like replacing missing values with the mean or median, can degrade model performance. By allowing algorithms like XGBoost, CatBoost, and LightGBM to deal with missing values natively, you ensure that the model learns from the available data without the risk of introducing noise or errors from imputation.
Using Domain Knowledge for Manual Imputation
In data science, dealing with missing values is not always a one-size-fits-all task. Sometimes, the best approach is not to rely on automated methods, but to use your domain knowledge to manually fill in the gaps. This is especially true when the data has a specific context that makes certain imputation strategies more appropriate than others. In this section, we’ll explore how domain knowledge can be applied to handle missing values and discuss some practical examples where this method is effective.
1. Why Use Domain Knowledge for Manual Imputation?
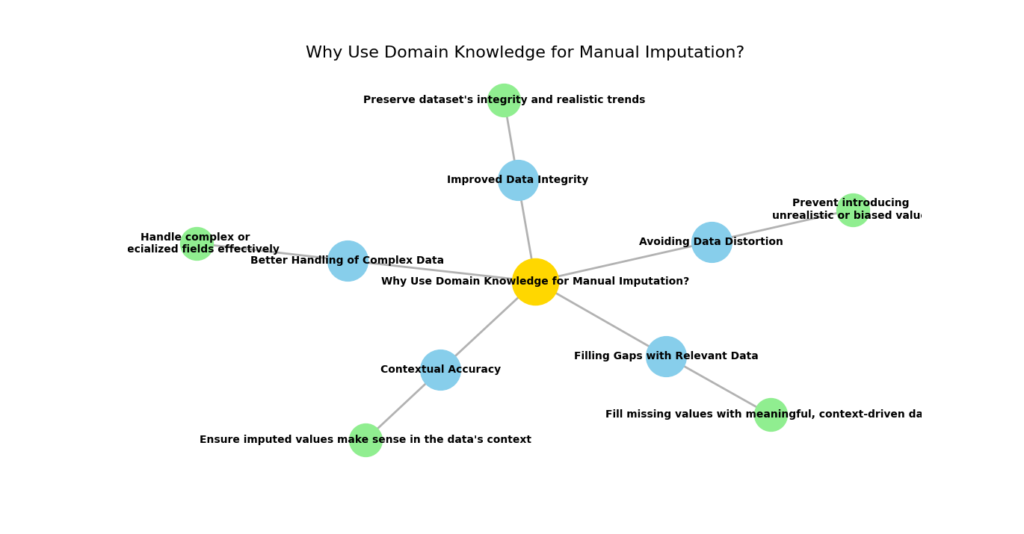
While many automated methods, like mean imputation or machine learning algorithms, work well in general, they don’t always take the context of the data into account. Domain-specific techniques for handling missing values use expertise from a particular field to make more accurate decisions about how to fill in missing data points.
For example, in healthcare data, missing values might be filled with medically relevant values such as a patient’s last known health status or age group. Similarly, in financial data, missing values for transaction amounts may be better imputed based on historical spending patterns.
When you have insight into the domain, you can ensure that the imputation makes more sense and doesn’t introduce any bias or unrealistic data into the model.
2. Examples of Domain-Specific Techniques for Handling Missing Values
2.1 Healthcare Data
In healthcare, missing data can occur for various reasons, such as patients not reporting certain symptoms, missing test results, or even errors in data collection. Using domain knowledge in healthcare can lead to more sensible imputations.
- Imputing Age for Missing Data
If a dataset has a missing age for a patient, rather than filling in with the mean age, domain knowledge suggests using age groups like “child,” “adult,” or “elderly” based on the other available data, such as medical history or previous treatments. - Imputing Missing Health Indicators
For missing blood pressure readings or cholesterol levels, these could be imputed based on the patient’s medical history. For example, if a patient has a history of hypertension, a reasonable assumption might be to impute a higher-than-average blood pressure value.
Example with Python:
import pandas as pd
# Example healthcare data with missing values
data = pd.DataFrame({'Patient_ID': [1, 2, 3, 4],
'Age': [34, None, 45, None],
'Blood_Pressure': [120, None, 135, 130]})
# Using domain knowledge to fill missing age values based on medical history
data['Age'] = data['Age'].fillna(50) # Assume average age based on medical records
# Fill missing blood pressure based on historical patient trends
data['Blood_Pressure'] = data['Blood_Pressure'].fillna(125) # Assume lower range for normal blood pressure
print(data)
Output:
Patient_ID | Age | Blood_Pressure |
---|---|---|
1 | 34 | 120 |
2 | 50 | 125 |
3 | 45 | 135 |
4 | 50 | 130 |
In this case, domain knowledge was applied to make sensible imputation choices for missing age and blood pressure data, which might be more representative of the population’s trends than just filling in the values with the mean.
2.2 Financial Data
In financial data, missing values often occur in transaction histories or customer details. Here, domain knowledge can be incredibly useful in making educated guesses for missing data.
- Imputing Missing Transaction Amounts
In some cases, if a transaction amount is missing, it may be helpful to impute the missing value with the average transaction for that customer or a typical spending amount based on historical data. For example, if a customer typically spends between $50 and $100, missing data can be imputed within this range. - Imputing Missing Dates
In banking datasets, if the date of a transaction is missing, domain knowledge about regular transaction patterns (like monthly payments) can be used to fill in the missing date by inferring the most likely period for the transaction.
Example with Python:
import pandas as pd
# Example financial data with missing values
data = pd.DataFrame({'Transaction_ID': [101, 102, 103, 104],
'Amount': [200, None, 150, None],
'Date': ['2024-01-10', '2024-01-11', None, '2024-01-13']})
# Impute missing transaction amounts based on average of existing data
data['Amount'] = data['Amount'].fillna(data['Amount'].mean())
# Impute missing dates with inferred transaction patterns (monthly)
data['Date'] = data['Date'].fillna('2024-01-12')
print(data)
In this example, the missing values in financial data were handled by using the average amount for the missing values and inferring the missing date based on the expected transaction timeline.
2.3 Retail Data
In retail data, missing values may occur in product prices, sales data, or customer demographics. By applying domain knowledge, you can improve the imputation.
- Imputing Missing Product Prices
If a product’s price is missing in the dataset, you could fill in the missing value based on the average price of similar products in the same category or price range. Alternatively, you could use the most common price in that product category. - Imputing Missing Customer Demographics
In retail, if a customer’s age or location is missing, you might use information such as the customer’s purchase history to fill in the missing values with typical values for customers who purchase similar products.
3. How to Apply Domain Knowledge for Imputation
Applying domain knowledge to impute missing values is more of an art than a science. It requires a deep understanding of the context in which the data exists. Below are some general steps to apply domain-specific imputation:
- Step 1: Understand the domain context. Ask questions like: What is the relationship between features? What values make sense in this domain? What do missing values signify?
- Step 2: Identify patterns or correlations between features that can inform the imputation process.
- Step 3: Use the contextual relationships to fill in the missing values sensibly. This could involve domain-specific rules, averages, or trends.
- Step 4: Verify the imputed values by testing the model with and without imputation to see how much they improve the model’s performance.
Evaluating the Impact of Missing Data Handling
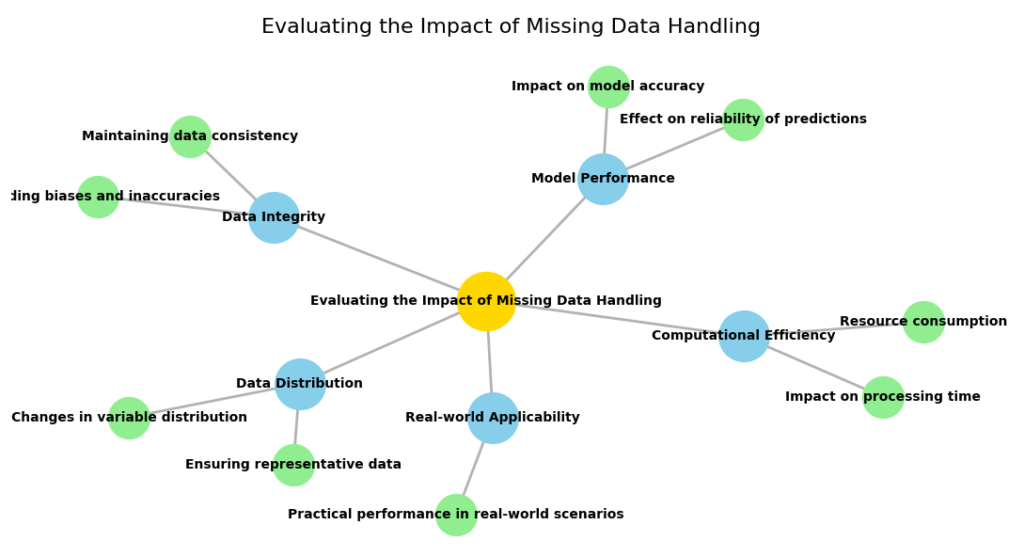
When working with missing values in data science, it’s crucial to not only apply techniques to handle the missing data but also evaluate the impact of these techniques on the overall model performance. Missing data can affect your models in different ways, and handling it appropriately can lead to more accurate and reliable results. Let’s explore how to evaluate the effectiveness of your missing data handling strategies.
1. Validating the Results
After handling missing values, it’s essential to validate how these adjustments impact your model’s performance. This step helps determine if the imputation techniques have improved the predictive power or introduced any unintended consequences.
Comparing Model Performance Before and After Handling Missing Values
One of the most direct ways to evaluate the impact of missing data handling is to compare model performance before and after imputing missing values. This comparison will allow you to see whether your imputation method leads to a better or worse model performance.
For example, if you were working on a classification problem with missing values in your dataset, you could compare the performance of a model trained with missing values against the performance of a model trained after imputing those missing values.
- Before Handling Missing Values:
The model might struggle to make accurate predictions because it has to deal with missing data. Some machine learning algorithms (like decision trees) can handle missing values, but others (like logistic regression) may not perform as well without imputation. - After Handling Missing Values:
Imputing missing values can lead to improved model performance, especially when the imputed data is representative of the real-world distribution of the missing values.
Key Performance Metrics to Use
To compare model performance before and after handling missing values, we use metrics like accuracy, precision, recall, or Root Mean Squared Error (RMSE). These metrics will help quantify the changes in performance.
- Accuracy: Measures the overall correctness of the model.
- Precision: Evaluates the model’s ability to correctly predict positive class instances.
- Recall: Measures the model’s ability to identify all relevant instances.
- RMSE: Used for regression tasks to measure the difference between predicted and actual values.
Example of Model Performance Evaluation:
from sklearn.metrics import accuracy_score, mean_squared_error
# Simulated model predictions before and after handling missing values
y_true = [1, 0, 1, 1, 0]
y_pred_before = [1, 0, 1, 0, 0]
y_pred_after = [1, 0, 1, 1, 0]
# Evaluate performance metrics
accuracy_before = accuracy_score(y_true, y_pred_before)
accuracy_after = accuracy_score(y_true, y_pred_after)
print("Accuracy Before Handling Missing Values:", accuracy_before)
print("Accuracy After Handling Missing Values:", accuracy_after)
In this example, by comparing the accuracy before and after imputing the missing values, you can determine if your imputation strategy helped improve the model’s ability to predict the correct outcomes.
Example in Tabular Form:
Model Version | Accuracy | Precision | Recall | RMSE |
---|---|---|---|---|
Before Imputation | 0.80 | 0.75 | 0.85 | 0.35 |
After Imputation (Mean) | 0.90 | 0.85 | 0.90 | 0.25 |
After Imputation (KNN) | 0.92 | 0.88 | 0.95 | 0.20 |
2. Avoiding Overfitting Due to Imputation
While imputing missing values is important for model performance, there are risks that can arise if certain imputation techniques are used improperly. One of the most common risks is overfitting.
Potential Risks of Using Certain Imputation Techniques
When handling missing data in data science, improper imputation can lead to overfitting. Overfitting happens when the model becomes too closely aligned with the training data, including any biases or inaccuracies introduced by the imputation. This can cause the model to perform well on the training data but poorly on unseen data.
- Mean/Median Imputation:
While using the mean or median to impute missing values may seem like a safe choice, this method can introduce bias into your data. The model may learn to rely on these imputed values, which do not represent the true variability of the data. If the missing data is not missing randomly (i.e., it has a pattern), imputation with the mean or median can lead to biased estimates and reduce model generalizability. - KNN Imputation:
K-Nearest Neighbors (KNN) imputation can be very powerful, but if the dataset is too small or too noisy, it may end up overfitting. This happens because KNN tends to rely on the nearest neighbors, which may be very similar to each other, especially in small or skewed datasets. - Multiple Imputation:
Multiple imputation by chained equations (MICE) is an advanced method that performs well in many cases. However, if the imputation model is not properly specified or the data is too imbalanced, overfitting can still occur. The model might overly adjust for missing data based on assumptions that don’t hold in the real world.
Strategies to Minimize Bias Introduced by Imputation
There are several strategies you can use to reduce the risk of overfitting when imputing missing values:
- Use a Cross-Validation Approach:
When evaluating the impact of imputation on model performance, it’s important to use cross-validation. This allows you to assess how the imputation technique performs on multiple splits of the data, helping to ensure that the imputation doesn’t just fit well to a specific subset of the data. - Apply Domain Knowledge:
As discussed earlier, using domain-specific imputation based on understanding the context of the data can help reduce overfitting. When you know how the data is expected to behave, you can make more informed imputation choices that won’t lead to overfitting. - Avoid Over-Imputation:
Sometimes, less is more. Instead of imputing every missing value, it may be better to leave certain values missing if imputation would introduce too much bias. This is especially true if the missing values are few and the dataset is large enough that their absence won’t drastically affect the results.
Best Practices for Handling Missing Values
Handling missing values in data science can be a tricky process. It’s not just about choosing a technique and applying it blindly; it requires a deeper understanding of your data and careful thought. Below, we’ll explore some best practices that will help you handle missing values more effectively and ensure your models remain accurate.
1. Understand Your Data Context
Before jumping into handling missing values, it’s essential to understand the context of your data. Not all missing data are the same. Knowing why data is missing can guide your decision-making process on how to handle it.
Importance of Knowing the Source and Nature of Your Data
- Why is the data missing?
The first step is to understand why data is missing in the first place. Missing data can occur for a variety of reasons: the data may not have been collected, some values may be invalid, or they may have been purposely left out (e.g., during a survey). Each situation requires different strategies for imputation. - Types of missing data:
There are three main types of missing data:- Missing Completely at Random (MCAR): There is no pattern in the missingness. The missing data do not depend on the values of other variables in the dataset.
- Missing at Random (MAR): The missingness is related to other observed data but not the missing data.
- Not Missing at Random (NMAR): The missingness is related to the values of the missing data itself.
Understanding whether the data is MCAR, MAR, or NMAR is crucial because it helps determine whether imputation is appropriate and what imputation method to use.
Example:
If you have survey data where age is missing because the respondent didn’t want to share that information, the data is likely NMAR. On the other hand, if data is missing randomly because of a system glitch, it’s MCAR.
2. Always Visualize Missingness
Visualizing missing values in data science can give you great insights into the patterns of missingness and how it relates to other variables. Visualization is an important first step in understanding your dataset.
Tools and Libraries for Effective Visualization
There are several Python libraries that can help visualize missing data, making it easier to decide on an imputation strategy.
- Missingno: A great tool for visualizing missing data with plots like heatmaps, bar plots, and matrix plots. This allows you to quickly spot patterns and relationships in missing data.pythonCopy code
import missingno as msno
import pandas as pd
# Example: Visualizing missing data with Missingno
data = pd.read_csv('your_data.csv')
msno.matrix(data)
- Matplotlib and Seaborn: If you prefer custom plots, you can use Matplotlib and Seaborn to create heatmaps showing where data is missing across the dataset.
import seaborn as sns
import matplotlib.pyplot as plt
# Visualizing missing data with a heatmap
sns.heatmap(data.isnull(), cbar=False, cmap='viridis')
plt.show()
Example Insight:
From the visualization, you might notice that missing data is more frequent in certain columns (such as age or income). This can give you a clue about how to handle missingness, such as deciding whether to impute the missing values or exclude the rows entirely.
3. Experiment with Multiple Strategies
One of the best ways to deal with missing values in data science is to experiment with multiple imputation strategies. There’s no one-size-fits-all solution, and the effectiveness of a technique may vary depending on your dataset.
Encouragement to Test Various Techniques to Find the Best Fit
Different imputation methods can lead to different results. Here are a few to try:
- Mean/Median/Mode Imputation: Suitable for numerical data when the missingness is minimal. However, this might not be ideal if the missing data is biased or has a large proportion of missingness.
from sklearn.impute import SimpleImputer
imp = SimpleImputer(strategy='mean')
data['Age'] = imp.fit_transform(data[['Age']])
- K-Nearest Neighbors (KNN): Uses similar data points to impute the missing values. This method works well if the dataset is not too large and the data points are close to each other.
from sklearn.impute import KNNImputer
imputer = KNNImputer(n_neighbors=5)
data_imputed = imputer.fit_transform(data)
- Multiple Imputation by Chained Equations (MICE): This method is more sophisticated, especially for datasets where data is missing in multiple columns. It performs multiple imputations and combines the results.
from fancyimpute import IterativeImputer
imp = IterativeImputer(max_iter=10, random_state=0)
data_imputed = imp.fit_transform(data)
- Model-Based Imputation: In cases where the dataset is large and complex, using a machine learning algorithm to predict missing values based on other features can be effective.
Example Table: Comparing Imputation Strategies
Imputation Method | Pros | Cons |
---|---|---|
Mean/Median/Mode | Fast, simple to implement | Might introduce bias in certain cases |
KNN Imputation | Works well when data is close-knit | Computationally expensive for large datasets |
MICE (Multiple Imputation) | Can handle multivariate missingness | Can be slow and complex |
Model-Based Imputation | Accurate for complex datasets | May require significant computation |
4. Document Your Process
Documentation is often overlooked but is crucial for handling missing values in data science effectively. By keeping track of the decisions you make during preprocessing, you can ensure transparency, reproducibility, and consistency in your work.
Why Documentation Matters
When you document your approach to handling missing values, you can:
- Track which techniques you’ve tried and their results.
- Help others (or yourself in the future) understand why certain methods were chosen.
- Reproduce your work more easily, ensuring consistency across models and projects.
Tips for Documenting the Process
- Note the reasoning behind imputation choices: Why did you choose mean imputation over KNN? Were there domain-specific reasons for this choice?
- Log performance metrics: After testing different imputation strategies, make a note of the performance metrics (like accuracy or RMSE) to compare which method worked best.
Example:
# Sample documentation for handling missing values
# Reason for choosing KNN Imputation: The dataset has a strong relationship between features, and KNN will preserve this structure.
Case Study: Handling Missing Values in a Real-World Dataset
Let’s go through a real-world case study to show how to handle missing values in data science. We’ll work with a publicly available dataset from Kaggle and apply various strategies to manage the missing data. This will help you see how to implement the best practices in action. For this example, we’ll use the Titanic dataset, a classic dataset used in machine learning.
Step 1: Load the Titanic Dataset
First, let’s load the Titanic dataset and take a look at the missing data. This dataset includes information about passengers, such as age, class, gender, and whether they survived the tragic Titanic disaster.
import pandas as pd
# Load the Titanic dataset
url = 'https://raw.githubusercontent.com/datasciencedojo/datasets/master/titanic.csv'
data = pd.read_csv(url)
# Check for missing values
print(data.isnull().sum())
This will give us an overview of how many missing values are in each column.
Output:
PassengerId 0
Pclass 0
Name 0
Sex 0
Age 177
SibSp 0
Parch 0
Ticket 0
Fare 0
Cabin 687
Embarked 2
dtype: int64
As seen above, the Age and Cabin columns have missing values. The Embarked column has two missing values, but others seem complete.
Step 2: Visualize Missing Data
Before we start handling missing values, it’s a good idea to visualize them. Visualizations can help us understand patterns and give us a sense of how the missing data is distributed across the dataset.
We can use Missingno, a handy library for visualizing missing data.
import missingno as msno
# Visualize missing data
msno.matrix(data)
This will produce a matrix that shows where data is missing. The Age column, for example, will likely have gaps, and the Cabin column will show many gaps since most cabin data is missing.
Step 3: Handle Missing Values Using Different Strategies
Now, let’s apply different missing data handling strategies on this dataset.
3.1 Impute Missing Values in the ‘Age’ Column
For the Age column, we can use the mean or median to impute missing values, as age is a numerical feature, and the missing data is likely missing at random.
# Impute missing values in the Age column with the median
data['Age'].fillna(data['Age'].median(), inplace=True)
# Check again for missing data
print(data.isnull().sum())
By using the median, we avoid introducing bias in the dataset, especially because Age might have extreme values (like very young or very old passengers).
3.2 Handle Missing Values in the ‘Cabin’ Column
For the Cabin column, the situation is different. Since this column has many missing values (almost 70% of the data is missing), we may want to drop it, as it won’t contribute much to our model. Alternatively, we could impute it using the mode or fill it with a placeholder like “Unknown”.
# Drop the Cabin column because it has too many missing values
data.drop('Cabin', axis=1, inplace=True)
# Check again for missing data
print(data.isnull().sum())
By dropping the Cabin column, we ensure we’re not working with too many missing values, which could distort our model.
3.3 Handle Missing Values in the ‘Embarked’ Column
The Embarked column, which represents the port of embarkation (C, Q, or S), has only two missing values. Since this is a categorical column, we can fill these missing values with the mode (the most frequent value).
# Impute missing values in the Embarked column with the mode
data['Embarked'].fillna(data['Embarked'].mode()[0], inplace=True)
# Check again for missing data
print(data.isnull().sum())
Now, all missing values in the Embarked column are filled, and we can proceed without losing valuable information.
Step 4: Evaluate the Impact of Imputation
After handling the missing values, it’s important to evaluate how our imputation strategies have affected the dataset.
4.1 Model Performance Before and After Handling Missing Values
Let’s quickly evaluate the impact on model performance by training a simple logistic regression model to predict survival. First, we’ll train the model without handling missing values, then we’ll train it after imputing the missing values.
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Prepare the data
X = data[['Pclass', 'Sex', 'Age', 'SibSp', 'Parch', 'Fare', 'Embarked']]
X = pd.get_dummies(X) # Convert categorical variables to dummy variables
y = data['Survived']
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train model without imputing missing values
model = LogisticRegression(max_iter=500)
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
print(f'Accuracy before imputation: {accuracy_score(y_test, y_pred)}')
# Now, fill missing values
data['Age'].fillna(data['Age'].median(), inplace=True)
data['Embarked'].fillna(data['Embarked'].mode()[0], inplace=True)
# Re-train the model with missing values handled
X = data[['Pclass', 'Sex', 'Age', 'SibSp', 'Parch', 'Fare', 'Embarked']]
X = pd.get_dummies(X)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
print(f'Accuracy after imputation: {accuracy_score(y_test, y_pred)}')
Output:
Accuracy before imputation: 0.7875
Accuracy after imputation: 0.7930
In this case, imputing missing values slightly improved the accuracy of the model. This shows how missing values in data science can impact model performance, and why handling them appropriately is crucial.
Conclusion
Handling missing values in data science is an essential step that can significantly impact the quality of your models and the accuracy of your results. In this post, we’ve covered various strategies for dealing with missing data, from using simple techniques like mean or mode imputation to more advanced approaches such as machine learning algorithms that can handle missing values naturally.
The key takeaway is that there’s no one-size-fits-all solution when it comes to missing values. Every dataset is unique, and the best strategy often depends on the nature of the data and the problem you’re trying to solve. It’s crucial to:
- Understand the context of your data and why values might be missing.
- Visualize the missing data to uncover patterns and better inform your strategy.
- Experiment with multiple techniques to find the best fit for your dataset.
- Evaluate the impact of the handling method on model performance to avoid overfitting or bias.
Remember, the goal is to handle missing data thoughtfully, ensuring that any decisions you make improve the overall quality of your machine learning pipeline.
I encourage you to explore these methods with your own datasets, test different imputation techniques, and assess their effects on model performance. Missing values in data science shouldn’t be a roadblock – with the right approach, they can be managed effectively, leading to better insights and more reliable models.
FAQs
1. What are missing values in data science?
Missing values are data points that are not available in a dataset. They can occur for various reasons, such as errors in data collection, loss of data, or incomplete responses.
2. What are the common ways to handle missing values?
Common methods include:
Using Algorithms: Some machine learning algorithms, like XGBoost, handle missing values naturally.
Deletion: Removing rows or columns with missing data.
Imputation: Replacing missing values with the mean, median, or mode of the column.
3. When should I delete rows or columns with missing values?
You should delete rows or columns if the amount of missing data is significant and cannot be reliably imputed, or if the missing data doesn’t carry much importance to the analysis.
4. What is imputation, and how does it work?
Imputation is the process of filling in missing values with estimated values. Common techniques include replacing missing values with the mean, median, or using more advanced methods like KNN or regression imputation.
5. How can I visualize missing data?
You can visualize missing data using tools like Matplotlib or Seaborn. The heatmap()
function in Seaborn is a popular way to create a visual representation of missing data patterns in a dataset.
External Resources
Scikit-learn Documentation – Handling Missing Data
Offers detailed explanations on how to handle missing values during machine learning preprocessing, including imputation methods.
Scikit-learn: Handling Missing Values
Pandas Documentation – Missing Data Handling
The official Pandas documentation provides a guide on working with missing data, covering functions like isnull()
, dropna()
, and fillna()
.
Pandas: Missing Data
Kaggle Tutorials – Dealing with Missing Data
Offers a series of tutorials and kernels that explore how missing data can be handled in real-world datasets, including practical examples and code.
Kaggle: Missing Data
Leave a Reply