Introduction to Voice Recording in Python
Voice recording in Python is all about capturing sounds from a microphone and turning them into digital files that you can save and listen to later. Imagine having a digital voice recorder, but instead of buying one, you’re creating it with code on your computer.
To make this work, you need to interact with your computer’s audio system. Just as you need a microphone to record your voice.
Libraries for Audio Handling
Two popular libraries for recording voices in Python are pyaudio
and wave
. Think of these as tools that make it easier for your Python program to communicate with your computer’s microphone and understand the audio it’s receiving.
pyaudio
Acts as a bridge between your Python code and your computer’s audio system. It helps your program communicate with the microphone and capture the sounds you want to record. It acts as a translator who helps you understand what the microphone is saying.
wave
This is a toolbox for working with audio files. After capturing the sounds with pyaudio
, wave
helps you save those sounds as digital audio files, specifically in the WAV format. It’s like having a set of tools to organize and store your recordings neatly on your computer.
So, when you’re building a voice recorder in Python, you’re using these tools (pyaudio
and wave
) to bridge the gap between your code and your computer’s microphone, allowing you to capture and save audio recordings with ease.
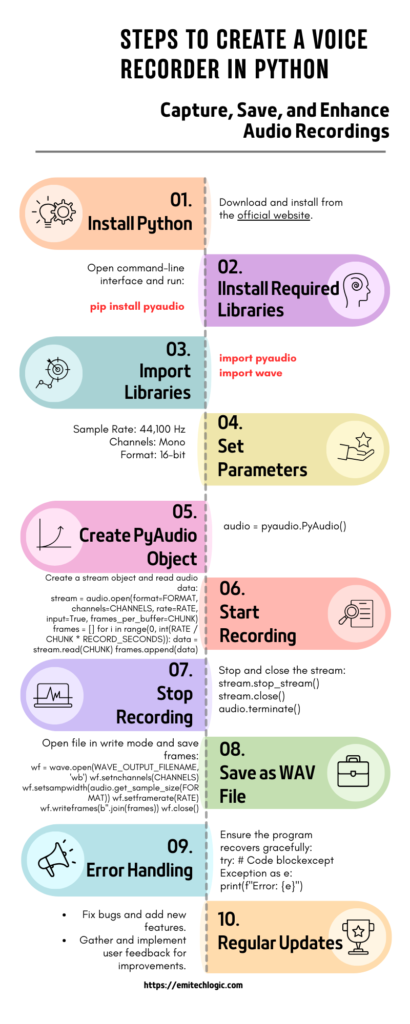
Setting Up Your Voice Recorder Development Environment
Before writing the code, you need to set up your development environment. Here’s what you need to do:
Install Python
Ensure you have Python installed on your system. Download Python from the official website and follow the installation instructions. It’s recommended to use a version of Python that is compatible with the libraries we’ll be using.
Install Required Libraries
You need to install the `pyaudio` library. Open your command-line interface (CLI) and enter the following command:
pip install pyaudio
This command will download and install the `pyaudio` library along with any necessary dependencies.
Optional Dependencies
Depending on your specific requirements, you may need to install additional libraries. For example:
numpy: For numerical operations:
pip install numpy
matplotlib: For data visualization:
pip install matplotlib
Once you have Python and the necessary libraries set up, you’re ready to write coding and start building your voice recorder!
Understanding Audio Sampling and Formats
Before recording audio, it’s important to understand some basic concepts about digital audio. Audio is a series of snapshots capturing the sound at different moments. Each snapshot, called a sample, records the strength of the sound wave at a particular point in time.
- Sampling Rate: The frequency at which these snapshots are taken. It’s measured in Hertz (Hz) and tells us how many samples are captured every second. Higher sampling rates result in more accurate representations of the original sound but require more storage space.
- Audio Formats: Just like image file formats (like JPEG or PNG) store visual data differently, audio formats specify how audio data is stored in a digital file. One popular format is WAV, short for Waveform Audio File Format. WAV files are used for storing uncompressed audio, preserving the original quality without compression, making them great for high-quality audio recordings.
Initializing the Recording Session
To start capturing audio, you need to set up a recording session using pyaudio
. This is like preparing your recording equipment before hitting the record button.
First, import the pyaudio
library into your Python script:
import pyaudio
Initialize a PyAudio object. This serves as your gateway to working with audio devices. It’s like setting up your recording studio.
audio = pyaudio.PyAudio()
Now, let’s define some parameters for our recording session:
Sample Rate
This is the number of audio samples captured each second, measured in Hertz (Hz). We’ll use a standard sampling rate of 44,100 Hz, which is typical for high-quality audio recordings, like those on CDs.
Channels
Audio can be recorded in mono or stereo. Mono recording captures sound from one source, while stereo captures sound from multiple sources, creating a sense of direction. For our purposes, we’ll use mono recording, which involves just one channel.
Format
This determines how the audio data is stored. We’ll use a 16-bit format (pyaudio.paInt16
), which is commonly used for digital audio
sample_rate = 44100 # Standard CD quality sampling rate
channels = 1 # Mono recording
format = pyaudio.paInt16 # 16-bit integer format
With these parameters set, your recording session is ready!
duration = 5 # Recording duration in seconds
Capturing Audio from the Microphone
To capture audio input, use a pyaudio.Stream
object. This is like a pipeline that connects your program to the microphone.
Open a stream for recording:
stream = audio.open(format=format, # Audio format (e.g., 16-bit integer)
channels=channels, # Number of audio channels (e.g., mono or stereo)
rate=sample_rate, # Sample rate (e.g., 44100 Hz)
input=True, # We're capturing audio input
frames_per_buffer=1024) # Number of frames per buffer (a buffer is a chunk of audio data)
This code captures audio from the microphone and stores it in the frames
list.
print("Recording...")
Now, Set up a container to hold our audio data. We can use a list called `frames` to store the audio frames.
frames = []
Start recording audio for the specified duration. We use a loop to continuously read audio data from the stream and append it to our `frames` list.
for _ in range(0, int(sample_rate / 1024 * duration)):
data = stream.read(1024) # Read audio data from the stream (1024 frames at a time)
frames.append(data) # Append the audio data to the frames list
Once the recording is complete, we print a message “Recording finished”
print("Recording finished.")
Finally, it’s important to clean up. We stop the stream and close it to release the resources.
stream.stop_stream() # Stop the audio stream
stream.close() # Close the audio stream
We’ve successfully captured audio from the microphone and stored it in the `frames` list.
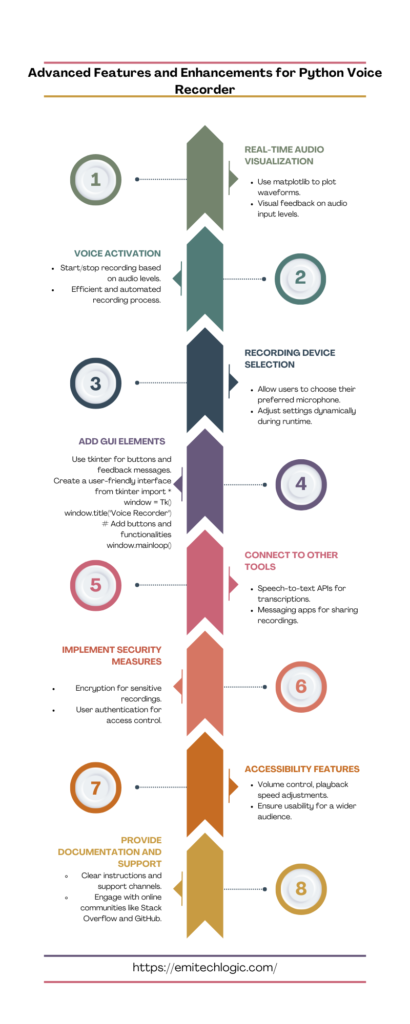
Saving the Recorded Audio
Now, save the captured audio data as a WAV file:
First, we need to decide on a name for our output file. This will be the file where our recorded audio will be stored.
output_file = "recorded_audio.wav"
Now, we open the output file in write mode using the function. This prepares the file for writing audio data.
with wave.open(output_file, 'wb') as wf:
Inside the `with` block, we need to specify the audio parameters for our WAV file. These parameters include the number of channels, sample width, and sample rate.
# Set audio parameters
wf.setnchannels(channels) # Number of audio channels
wf.setsampwidth(audio.get_sample_size(format)) # Sample width (in bytes)
wf.setframerate(sample_rate) # Sample rate (number of samples per second)
Now, we’re ready to write the audio frames. We use the `writeframes()` method to write the audio data to the file.
# Write audio frames to the file
wf.writeframes(b''.join(frames))
Finally, we print a message the audio has been saved successfully.
print("Audio saved to:", output_file)
We’ve successfully saved our recorded audio as a WAV file.
Handling Errors and Exceptions
Error handling ensure that your program can recover from unexpected situations, like when something goes wrong during audio recording.
try:
# Attempt to open the stream for recording
stream = audio.open(format=format,
channels=channels,
rate=sample_rate,
input=True,
frames_per_buffer=1024)
# Inside this block, we perform the recording...
except OSError as e:
# If an error occurs, we catch it and print a helpful message
print("Error:", e)
finally:
# Make sure to close the stream and terminate PyAudio
if stream:
stream.stop_stream()
stream.close()
audio.terminate()
By handling errors in this way, we ensure that our program remains perfect and stable, even when unexpected problems arise during audio recording.
Adding User Interface Elements
To make your voice recorder easier to use, you can add features like buttons for starting and stopping recording, as well as feedback messages. We’ll use the tkinter
library to create a simple graphical interface for this.
First, we’ll define functions to handle starting and stopping the recording. These functions will be triggered when the corresponding buttons are clicked.
import tkinter as tk
def start_recording():
print("Recording started...")
def stop_recording():
print("Recording stopped...")
Next, we create a Tkinter window, which will be the main interface for our voice recorder. We’ll set the window title to ‘Voice Recorder’ so users know exactly what the application is for.
window = tk.Tk()
window.title("Voice Recorder")
Now, let’s add buttons to the window for starting and stopping the recording. We’ll create tk.Button
instances, set the text to display on each button, and specify which function should be called when the button is clicked (command
).
start_button = tk.Button(window, text="Start Recording", command=start_recording)
start_button.pack()
stop_button = tk.Button(window, text="Stop Recording", command=stop_recording)
stop_button.pack()
“Finally, we start the Tkinter event loop with window.mainloop()
. This loop keeps the program running, listening for events like button clicks and updating the interface as needed.”
window.mainloop()
When you run this script, a window will pop up showing two buttons: ‘Start Recording’ and ‘Stop Recording’. Clicking these buttons will make the recorder start or stop, and for now, it’ll just print messages on the console.
By putting in these buttons, users can control the recording more easily, making it a better experience overall.
Complete Coding of Voice Recorder
import pyaudio
import wave
import tkinter as tk
from tkinter import filedialog, messagebox
import numpy as np
import threading
import time
import os
import struct
class VoiceRecorder:
def __init__(self):
self.FORMAT = pyaudio.paInt16
self.CHANNELS = 2
self.RATE = 44100
self.CHUNK = 1024
self.frames = []
self.is_recording = False
self.is_paused = False
self.stream = None
self.audio = pyaudio.PyAudio()
self.init_gui()
def init_gui(self):
self.root = tk.Tk()
self.root.title("Voice Recorder")
self.label = tk.Label(self.root, text="Enter Recording Duration (seconds):")
self.label.pack()
self.duration_entry = tk.Entry(self.root)
self.duration_entry.pack()
self.record_button = tk.Button(self.root, text="Record", command=self.start_recording)
self.record_button.pack()
self.pause_button = tk.Button(self.root, text="Pause", command=self.pause_recording, state=tk.DISABLED)
self.pause_button.pack()
self.resume_button = tk.Button(self.root, text="Resume", command=self.resume_recording, state=tk.DISABLED)
self.resume_button.pack()
self.stop_button = tk.Button(self.root, text="Stop", command=self.stop_recording, state=tk.DISABLED)
self.stop_button.pack()
self.play_button = tk.Button(self.root, text="Play", command=self.play_recording, state=tk.DISABLED)
self.play_button.pack()
self.visualize_button = tk.Button(self.root, text="Visualize", command=self.visualize_audio, state=tk.DISABLED)
self.visualize_button.pack()
self.save_button = tk.Button(self.root, text="Save As", command=self.save_as, state=tk.DISABLED)
self.save_button.pack()
self.root.protocol("WM_DELETE_WINDOW", self.on_closing)
self.root.mainloop()
def start_recording(self):
try:
self.RECORD_SECONDS = int(self.duration_entry.get())
if self.RECORD_SECONDS <= 0:
raise ValueError
self.is_recording = True
self.record_button.config(state=tk.DISABLED)
self.pause_button.config(state=tk.NORMAL)
self.stop_button.config(state=tk.NORMAL)
self.save_button.config(state=tk.DISABLED)
self.play_button.config(state=tk.DISABLED)
self.visualize_button.config(state=tk.DISABLED)
self.stream = self.audio.open(format=self.FORMAT,
channels=self.CHANNELS,
rate=self.RATE,
input=True,
frames_per_buffer=self.CHUNK)
self.frames = []
self.root.after(100, self.record)
except ValueError:
messagebox.showerror("Invalid input", "Please enter a valid number for duration")
def record(self):
if self.is_recording and not self.is_paused:
try:
data = self.stream.read(self.CHUNK)
self.frames.append(data)
self.root.after(100, self.record)
except Exception as e:
self.is_recording = False
messagebox.showerror("Recording Error", str(e))
def pause_recording(self):
self.is_paused = True
self.pause_button.config(state=tk.DISABLED)
self.resume_button.config(state=tk.NORMAL)
def resume_recording(self):
self.is_paused = False
self.resume_button.config(state=tk.DISABLED)
self.pause_button.config(state=tk.NORMAL)
self.record()
def stop_recording(self):
self.is_recording = False
self.stream.stop_stream()
self.stream.close()
self.audio.terminate()
self.record_button.config(state=tk.NORMAL)
self.pause_button.config(state=tk.DISABLED)
self.resume_button.config(state=tk.DISABLED)
self.stop_button.config(state=tk.DISABLED)
self.save_button.config(state=tk.NORMAL)
self.play_button.config(state=tk.NORMAL)
self.visualize_button.config(state=tk.NORMAL)
self.output_filename = "output.wav"
self.save_recording(self.output_filename)
messagebox.showinfo("Recording Finished", f"Recording saved as {self.output_filename}")
def save_recording(self, filename):
waveFile = wave.open(filename, 'wb')
waveFile.setnchannels(self.CHANNELS)
waveFile.setsampwidth(self.audio.get_sample_size(self.FORMAT))
waveFile.setframerate(self.RATE)
waveFile.writeframes(b''.join(self.frames))
waveFile.close()
def play_recording(self):
chunk = 1024
wf = wave.open(self.output_filename, 'rb')
p = pyaudio.PyAudio()
stream = p.open(format=p.get_format_from_width(wf.getsampwidth()),
channels=wf.getnchannels(),
rate=wf.getframerate(),
output=True)
data = wf.readframes(chunk)
while data:
stream.write(data)
data = wf.readframes(chunk)
stream.stop_stream()
stream.close()
p.terminate()
def visualize_audio(self):
import matplotlib.pyplot as plt
data = b''.join(self.frames)
data = np.frombuffer(data, dtype=np.int16)
plt.plot(data)
plt.title('Audio Waveform')
plt.show()
def save_as(self):
filename = filedialog.asksaveasfilename(defaultextension=".wav",
filetypes=[("WAV files", "*.wav")])
if filename:
self.save_recording(filename)
messagebox.showinfo("Saved", f"Recording saved as {filename}")
def on_closing(self):
if self.is_recording:
self.stop_recording()
self.root.destroy()
if __name__ == "__main__":
VoiceRecorder()
Implementing Advanced Features
To make your voice recorder more perfect, consider adding features like:
Real-time Audio Visualization
Use matplotlib
to plot audio waveforms in real-time.
Voice Activation
Implementing voice activation can automate the recording process by starting and stopping recording based on audio input levels.
Dynamic Recording Settings
Allow users to select their preferred recording device or adjust settings dynamically.
Conclusion
By adding these advanced features to your voice recorder, you can make it more powerful and user-friendly for a wide range of users. Enhancements like visualizing audio waveforms, implementing voice activation, and offering customizable recording settings can significantly improve your voice recorder.
Building a voice recorder in Python is an exciting project that covers various programming aspects, including audio processing, user interface design, and error handling. In this comprehensive guide, we will walk you through the step-by-step process of creating a voice recorder from scratch using Python. Along the way, you'll find explanations, code snippets, and practical tips to help you succeed.
Miscellaneous
- Audio Effects: Experiment with filters to change the pitch or speed of the recorded audio, or add echo or reverb effects.
- Integration with Other Tools: Connect your recorder to a speech-to-text API or a messaging app to expand its functionality.
- Security and Privacy: Implement measures like encryption or user authentication to protect sensitive recordings.
- Accessibility Features: Add features to make your recorder usable by a wider audience, such as adjusting volume or playback speed.
- Documentation and Support: Provide clear instructions and support channels to help users.
- Continuous Improvement: Regularly update your codebase, fix bugs, and add new features based on user feedback.
Frequently Asked Questions
External Resources
- Python Documentation: Official documentation for Python programming.
- Stack Overflow: Community-driven platform for asking and answering programming questions.
- GitHub: Platform for hosting and collaborating on software projects.
- YouTube: Tutorials and educational videos on Python programming and audio processing.
- Python Community Forums: Forums for discussing Python-related topics.
By using these resources, you can enhance your learning and create a powerful and user-friendly voice recorder with Python.
[…] MP3 converter, because these formats are used commonly. In this guide, we’ll show you how to create a Free MP4 to MP3 Converter using Python. This online converter is designed to be simple, fast, and efficient. We’ll give you […]
[…] developed by Hugging Face, has gained widespread recognition for its pre-trained models in natural language processing (NLP). […]
[…] Imagine being able to control your devices just by talking to them. Virtual assistants make this possible with voice recognition technology. […]