Introduction
Ever find yourself staring at a piece of code, trying to remember what it does or why you wrote it a certain way? You’re not alone! That’s where comments in Python come in—they’re like little notes to yourself (and anyone else who might read your code) that make your code easier to understand and maintain. But there’s a right way to use comments, and a way that can turn your code into a cluttered mess.
In this post, we’re going to talk about how to use comments in Python so they’re not just useful, but they also make your code shine. Whether you’re a beginner or someone who’s been coding for a while, knowing how to comment your code effectively can make a huge difference in how your projects turn out. By the end of this, you’ll have a few tricks up your sleeve to use comments like a pro, making your code clear, organized, and a breeze to revisit. So, let’s get started!
What Are Comments in Python?
Comments in Python are little notes or messages that you include in your code to help explain what’s happening. They don’t affect how the code runs, but they play an essential role in making your code easier to understand for yourself and others who might read it later. When writing Python code, adding comments is like leaving breadcrumbs—guiding anyone who reads your code through your thought process, explaining why certain choices were made, and clarifying complex sections.
Definition and Purpose of Comments
In Python, comments are lines of text that start with a #
symbol. Anything written after this symbol on the same line is considered a comment, meaning Python will ignore it when executing the code. The main purpose of comments is to explain what the code does and why it’s written in a particular way.
Think of comments as your way of having a conversation with whoever reads your code. Whether it’s a teammate, a future version of yourself, or even someone learning from your work, comments provide insights that make the code more understandable. They answer questions that might come up, like “What is this function supposed to do?” or “Why did I use this specific method?”
Why Are Comments Essential in Python Code?
When writing Python code, it’s easy to get caught up in the excitement of solving a problem or building a feature. However, without the right use of comments, even the most brilliantly written code can become a puzzle—difficult to read, understand, or maintain. Comments are essential in Python for a few key reasons, all of which contribute to making your code more effective and accessible.
Enhancing Code Readability
One of the main reasons to use comments in Python is to enhance code readability. Python is known for its clean and readable syntax, but even the most elegant code can benefit from a few well-placed comments. Comments provide context, explaining what a particular block of code does and why it’s necessary. This is especially important when your code includes complex logic, algorithms, or functions that might not be immediately obvious to someone else—or even to you when you revisit the code later.
When you take the time to add comments, you make your code more approachable. This means that anyone reading it, whether it’s a beginner trying to learn from your work or an experienced programmer reviewing your code, will be able to understand your intentions more quickly. Comments help to bridge the gap between what the code is doing and why it’s doing it, making the code much easier to follow.
Aiding Collaboration in Teams
In a team setting, comments are invaluable. When multiple people are working on the same project, clear communication is crucial. Comments act as a form of communication within the code itself, helping team members understand each other’s work without having to ask a lot of questions. They make it easier for new members to get up to speed, for teammates to pick up where someone else left off, and for everyone to stay on the same page.
Without comments, collaborating on code can become frustrating. Team members might struggle to understand the reasoning behind certain decisions or to figure out how a specific function works. With comments, however, these issues can be avoided. Comments help to maintain consistency and ensure that the entire team is aligned with the project’s goals and methods.
Serving as Documentation for Future Reference
Another important role of comments is serving as documentation for future reference. Over time, you may forget why certain choices were made in your code. Well-written comments will remind you—or anyone else who encounters your code in the future—about the purpose behind each section.
Comments provide a way to document your thought process, explain the purpose of complex logic, and clarify the intended use of functions and classes. This can be especially helpful when updates or bug fixes are needed long after the code was originally written. Instead of spending time trying to reverse-engineer your own work, you can rely on your comments to quickly recall what you did and why you did it.
In summary, comments are an essential part of Python programming. They enhance code readability, aid in collaboration within teams, and serve as valuable documentation for future reference. By taking the time to write thoughtful comments, you’re not just improving your code; you’re also making life easier for anyone who reads it, including your future self.
Types of Comments in Python

Single-Line Comments in Python
Single-line comments in Python are like quick notes you jot down to remind yourself—or others—of what a specific part of your code does. They’re a simple yet powerful way to make your code more understandable and maintainable. By using single-line comments effectively, you can create code that’s not only functional but also easy to read and navigate.
Syntax: Using the #
Symbol
In Python, creating a single-line comment is incredibly easy. All you need to do is start your comment with the #
symbol. Everything after this symbol on the same line will be treated as a comment, meaning Python will ignore it when running the code. The #
symbol can be placed at the beginning of a line, making the entire line a comment, or it can be added after some code on the same line to explain what that code does.
For example:
# This is a single-line comment explaining the following line of code
x = 5 # Assigns the value 5 to the variable x
In the above example, the first comment explains what’s happening in the following line of code. The second comment, placed after the code, clarifies what the assignment does. These comments don’t interfere with the code execution but add valuable context.
When and How to Use Single-Line Comments
Single-line comments should be used when you want to provide a brief explanation of a particular line or block of code. They are perfect for clarifying the purpose of a specific variable, explaining a tricky part of the logic, or noting why a certain approach was chosen. However, it’s important not to overdo it. The goal is to make the code more understandable, not to clutter it with unnecessary explanations.
Here are some tips for using single-line comments effectively:
- Place comments above the code they refer to: This helps make it clear which part of the code the comment is explaining.
- Keep comments concise: Aim for a few words or a short sentence that gets the point across without overwhelming the reader.
- Focus on the ‘why,’ not the ‘what’: The code itself often shows what is happening, so use comments to explain why a particular approach was taken or what the code is meant to achieve.
Examples of Effective Single-Line Comments
Let’s look at some examples to illustrate how single-line comments can be used effectively:
# Calculate the area of a rectangle
area = width * height
# Check if the user is logged in before displaying personalized content
if user_logged_in:
display_content()
# Set the default value for retries
retries = 3 # Allow up to 3 retry attempts
In these examples, the comments provide just enough information to clarify the purpose of each line without being overly verbose. They help anyone reading the code understand the intent behind the actions being performed.
Multi-Line Comments in Python
In Python, sometimes a single line of comment just isn’t enough to explain a complex piece of code. This is where multi-line comments come in handy. They allow you to add more detailed explanations, making your code easier to understand, especially when dealing with complicated logic or algorithms.
Syntax: Using Triple Quotes '''
or """
Python doesn’t have a specific syntax for multi-line comments like some other programming languages. Instead, you can use triple quotes—either '''
or """
—to create a block of text that spans multiple lines. This block of text can be placed anywhere in your code, and Python will treat it as a comment if it’s not assigned to a variable or used as a docstring for functions or classes.
Here’s how it looks in practice:
'''
This is a multi-line comment.
It can span several lines, allowing for more detailed explanations.
Useful for documenting complex code or providing context for a block of code.
'''
You can also use triple double quotes ("""
) in the same way:
"""
This is another example of a multi-line comment.
Using triple double quotes works just as well.
"""
In both cases, Python will ignore the text enclosed within the triple quotes during execution, making it a convenient way to add longer comments.
When to Use Multi-Line Comments
Multi-line comments are most useful when you need to explain something that can’t be covered in a single line. This could be a detailed description of a complex algorithm, an overview of what a section of the code is supposed to achieve, or even notes about potential improvements or known issues.
Use multi-line comments when:
- Explaining complex logic: If the code you’re writing involves intricate calculations or steps that might not be immediately obvious, a multi-line comment can break down the process for anyone reading it.
- Providing context: Sometimes, it’s helpful to explain why a particular approach was chosen, especially if there were other alternatives. This can be useful for future reference or when others review your code.
- Documenting large sections of code: When your code has distinct sections, you can use multi-line comments to introduce each section, explaining its purpose and how it fits into the overall project.
However, it’s important to use multi-line comments wisely. Overusing them can make your code harder to read, so they should be reserved for situations where a single-line comment wouldn’t suffice.
Examples of Multi-Line Comments in Real-World Scenarios
Let’s look at some real-world scenarios where multi-line comments can be incredibly useful:
'''
The following function calculates the Fibonacci sequence up to a given number n.
It uses a recursive approach, which can be inefficient for large values of n.
This implementation is primarily for educational purposes.
'''
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
In this example, the multi-line comment at the top of the function explains not just what the function does, but also why a particular method was chosen, and it even mentions a potential downside. This context is valuable for anyone who might use or modify the function later.
Another example:
"""
This section of code is responsible for loading the data from a CSV file,
performing necessary data cleaning, and then storing the cleaned data
in a new file. The data cleaning includes handling missing values,
converting data types, and removing duplicates.
"""
# Load the data
data = pd.read_csv('data.csv')
# Clean the data
data = data.dropna() # Remove missing values
data['date'] = pd.to_datetime(data['date']) # Convert date column to datetime
data = data.drop_duplicates() # Remove duplicate rows
# Save the cleaned data
data.to_csv('cleaned_data.csv', index=False)
Here, the multi-line comment serves as an introduction to a section of code, providing an overview of what the following lines will do. This makes it easier for someone else to understand the purpose of this code block without having to analyze each line individually.
Inline Comments in Python
Inline comments in Python can be incredibly helpful when used correctly. These are comments that sit on the same line as your code, usually to clarify what a specific part of that line does. While small and often overlooked, inline comments play a big role in making your code more accessible and easier to understand, especially when you’re working on complex projects or collaborating with others.
How to Use Inline Comments Effectively
Inline comments should be brief and directly related to the code they accompany. They are perfect for adding a quick note or explanation without breaking the flow of the code. To create an inline comment in Python, you simply place the #
symbol after a piece of code, followed by your comment.
For example:
x = 10 # Assigns the value 10 to variable x
Here, the inline comment clarifies what the code does in just a few words. However, effective inline commenting goes beyond just explaining the code—it’s about adding value without cluttering your codebase.
Here’s how to use inline comments effectively:
- Keep them short and relevant: Inline comments should be concise, usually just a few words. They are meant to clarify, not to provide lengthy explanations. If you find yourself writing a long comment, it might be better suited as a multi-line comment above the code block.
- Place them where they add value: Use inline comments to explain non-obvious parts of your code, such as a tricky calculation or a specific method used. Avoid stating the obvious, as this can make your code harder to read rather than easier.
- Update comments as you update code: Outdated comments can be more confusing than helpful. Make sure to revise your comments whenever you modify the corresponding code.
Dos and Don’ts of Inline Commenting
While inline comments can be very useful, there are best practices to follow to ensure they remain helpful rather than becoming a hindrance.
Dos:
- Do use inline comments sparingly: They should only be used when absolutely necessary. Too many inline comments can clutter your code and make it harder to read.
- Do place inline comments on the same line as the code they describe: This makes it clear which part of the code the comment is referring to.
- Do focus on the ‘why’ rather than the ‘what’: Explain why a particular approach was taken, especially if it’s not immediately obvious.
Don’ts:
- Don’t restate the code: Avoid using inline comments to explain what the code already clearly shows. For example, don’t write
x = x + 1 # Increment x by 1
—the code itself is already clear. - Don’t use inline comments for large explanations: If your comment is more than a short phrase, consider using a multi-line comment instead.
- Don’t leave outdated comments: If the code changes, make sure the comments are updated to reflect those changes.
Examples of Inline Comments in Code
Let’s take a look at some examples to better understand how inline comments can be used effectively:
total_cost = item_price * quantity # Calculate total cost by multiplying price by quantity
In this example, the inline comment clarifies the purpose of the calculation, which might be helpful if the variable names weren’t as descriptive.
discounted_price = price * 0.9 # Apply a 10% discount
Here, the comment explains the logic behind the discount calculation. Without the comment, it might not be immediately clear why the price is being multiplied by 0.9.
On the other hand, here’s an example of what not to do:
x = x + 1 # Increment x by 1
This comment is unnecessary because the code itself is clear. The comment doesn’t add any value and could be omitted to keep the code clean.
Must Read
- How to Design and Implement a Multi-Agent System: A Step-by-Step Guide
- The Ultimate Guide to Best AI Agent Frameworks for 2025
- Data Science: Top 10 Mathematical Definitions
- How AI Agents and Agentic AI Are Transforming Tech
- Top Chunking Strategies to Boost Your RAG System Performance
Best Practices for Writing Comments in Python

Keep Comments Relevant and Up-to-Date
Comments are vital for making your code easier to understand, but they only serve their purpose if they remain relevant and accurate. As your code evolves, your comments should evolve too. Outdated comments can cause confusion and lead to mistakes, especially for anyone who might be working with your code in the future.
Avoiding Outdated Comments
Outdated comments can be a serious problem. When you modify your code but forget to update the related comments, those comments can become misleading. For example, if you change the way a function works but leave the original comment intact, the comment will no longer reflect the actual behavior of the code. This can create a false sense of understanding, leading to errors and frustration.
To avoid this, make it a habit to review your comments whenever you change your code. Consider them part of the code itself. Just like you wouldn’t leave old, unused code lying around, you shouldn’t leave outdated comments.
Here’s an example of what could go wrong with outdated comments:
# Calculate the total price after applying a discount
total_price = price - (price * tax_rate)
Suppose you later change the calculation to account for tax instead of applying a discount, but you forget to update the comment:
# Calculate the total price after applying a discount
total_price = price + (price * tax_rate)
The comment now incorrectly states that a discount is being applied when, in fact, tax is being added. Anyone reading this code will be misled by the outdated comment, potentially causing errors in understanding or modifying the code.
Keeping Comments Aligned with Code Changes
Maintaining alignment between comments and code requires vigilance. Whenever you update your code, take a moment to ensure that your comments still make sense. This includes renaming variables, changing logic, or even just tweaking a line of code.
For example, if you rename a variable to better reflect its purpose, make sure you update any comments that refer to it:
# Calculate the final cost after tax
final_cost = price + (price * tax_rate) # Adjusted to match variable renaming
In this case, if you initially named the variable total_price
but later decided final_cost
was clearer, you should update both the code and the comment. This ensures that your code and comments are always in sync, making your codebase more reliable and easier to maintain.
Aligning comments with code changes also involves removing comments that no longer apply. If a piece of code is rewritten or a function is removed, be sure to clean up any associated comments to avoid leaving behind confusing or irrelevant information.
Example: Keeping Comments Relevant and Up-to-Date
Here’s an example that shows the importance of keeping comments relevant and aligned with code changes:
Original Code:
# Apply a 10% discount to the total price
total_price = price - (price * 0.10)
Updated Code:
# Add tax to the total price
final_cost = price + (price * tax_rate)
In this scenario, both the comment and the code have been updated to reflect the new logic. This ensures that anyone reading the code understands exactly what it’s doing.
Avoiding Over-Commenting
While comments are an essential part of writing clean and understandable code, it’s possible to overdo it. Too many comments can clutter your code and make it harder to read, which is the opposite of what comments are meant to achieve. The key is to find the right balance between too many and too few comments, ensuring that your code remains both readable and maintainable.
The Balance Between Too Many and Too Few Comments
When you’re adding comments to your code, think about whether they truly add value. Not every line of code needs an explanation. Comments should clarify, not restate the obvious. For instance, commenting on a line that adds two numbers together isn’t necessary because the operation is self-explanatory. Instead, save your comments for explaining why the code is doing something, not what it’s doing.
Here’s an example of over-commenting:
# Set the price to 100
price = 100
# Set the tax rate to 5%
tax_rate = 0.05
# Calculate the tax
tax = price * tax_rate
# Add the tax to the price
total_price = price + tax
These comments are unnecessary because the code is clear enough on its own. Anyone reading it can easily understand what each line does without needing a comment to explain it.
Instead, a better approach would be to use comments sparingly, focusing on the why behind the code:
# Calculate the total price, including tax, for the invoice
total_price = price + (price * tax_rate)
This comment explains the purpose of the calculation rather than describing each step, which makes it more useful to someone reading the code.
Letting the Code Speak for Itself When Possible
Well-written code often doesn’t need much commentary. If your variable names and function names are descriptive and your logic is clear, your code can often speak for itself. This doesn’t mean you should never use comments—just that you should rely on them to clarify complex or non-obvious parts of your code rather than explaining the basics.
For example:
# Good variable names and clean logic eliminate the need for excessive comments
def calculate_final_price(price, tax_rate):
return price + (price * tax_rate)
In this case, the function name calculate_final_price
and the variables price
and tax_rate
make the code self-explanatory. There’s no need for additional comments to explain what the code is doing.
Writing Clear and Concise Comments
When you do need to add comments, clarity is key. Using plain language that’s easy to understand will help others (and your future self) quickly grasp what the code is supposed to do. Avoid using jargon or overly technical terms in your comments—your goal is to make the code easier to understand, not to confuse the reader further.
Using Plain Language for Better Understanding
Comments should be written as if you’re explaining the code to someone who’s new to it. Use simple, easy language that gets the point across without being overly complicated. This makes your code more accessible to others, regardless of their experience level.
For example:
# Loop through each item in the list and print it
for item in items:
print(item)
This comment is clear and uses plain language to explain what the code does.
Avoiding Ambiguity in Comments
Ambiguity in comments can lead to misunderstandings and mistakes. When writing comments, be as specific as possible. Avoid vague statements that could be interpreted in multiple ways. Instead, clearly state what the code is doing and why.
For instance, avoid a comment like this:
# Handle the edge case
if value == 0:
print("Value is zero")
This comment doesn’t explain what the edge case is or why it’s important. A better comment would be:
# Print a message if the value is zero to handle cases where no input was provided
if value == 0:
print("Value is zero")
This version clearly explains the purpose of the code, reducing the chance of confusion.
Example: Writing Clear and Concise Comments
Here’s an example that illustrates the principles of avoiding over-commenting and writing clear, concise comments:
def calculate_discounted_price(price, discount):
# Ensure the discount is not greater than the price
if discount > price:
raise ValueError("Discount cannot be greater than the price")
# Apply the discount and return the final price
return price - discount
In this example, the comments are used sparingly and only where necessary. They clarify the purpose of the code without overwhelming the reader with unnecessary details.
Advanced Commenting Techniques

What Are Docstrings and Their Importance in Python?
Docstrings are a type of comment in Python, but they’re more than just a way to annotate your code. They are special strings that are used to explain what a function, class, or module does. Unlike regular comments, which are added to make the code easier to understand for humans, docstrings are meant to serve both humans and tools. They help in making your code more understandable and maintainable, providing a clear and concise explanation of how the code is supposed to work.
Docstrings are placed right after the definition of a function, class, or module, and they are enclosed in triple quotes '''
or """
. This placement allows tools like Sphinx or Pydoc to automatically extract these strings and generate documentation, making it easier for others (or yourself in the future) to understand what the code does without needing to read through every line of it.
Importance of Docstrings
Writing docstrings is an essential practice in Python programming, especially when working on larger projects or in teams. Here are a few reasons why docstrings are so important:
- Clarity: Docstrings provide a clear explanation of what a piece of code is supposed to do, which can be incredibly helpful for anyone reading or using your code later on. They explain the purpose, parameters, and return values of functions, which makes the code easier to use and modify.
- Documentation: Tools like Sphinx and Pydoc can automatically generate documentation from your docstrings, saving time and ensuring that your documentation is always in sync with your code. This is particularly valuable in larger projects where keeping the documentation up to date manually would be time-consuming and prone to errors.
- Collaboration: When working in a team, docstrings make it easier for everyone to understand each other’s code. They provide a standardized way of describing what functions, classes, and modules do, which can improve communication and reduce misunderstandings.
How to Write Docstrings for Functions, Classes, and Modules
Writing docstrings might seem easy, but there are best practices that can help make them more effective. Let’s break down how to write docstrings for functions, classes, and modules.
1. Writing Docstrings for Functions
A good docstring for a function should describe the function’s purpose, its parameters, and what it returns. Here’s a simple example:
def add_numbers(a, b):
"""
Add two numbers and return the result.
Parameters:
a (int or float): The first number to add.
b (int or float): The second number to add.
Returns:
int or float: The sum of the two numbers.
"""
return a + b
In this example, the docstring clearly explains what the function does, what the parameters a
and b
represent, and what the function returns.
2. Writing Docstrings for Classes
For classes, the docstring should describe what the class represents and provide a brief overview of its methods. Here’s an example:
class Calculator:
"""
A simple calculator class to perform basic arithmetic operations.
Methods:
add(a, b): Returns the sum of a and b.
subtract(a, b): Returns the difference between a and b.
multiply(a, b): Returns the product of a and b.
divide(a, b): Returns the quotient of a and b.
"""
def add(self, a, b):
"""Return the sum of a and b."""
return a + b
def subtract(self, a, b):
"""Return the difference between a and b."""
return a - b
def multiply(self, a, b):
"""Return the product of a and b."""
return a * b
def divide(self, a, b):
"""Return the quotient of a and b."""
if b == 0:
raise ValueError("Cannot divide by zero.")
return a / b
In this example, the class docstring provides a general overview of what the Calculator
class does, and each method has its own docstring explaining its specific purpose.
3. Writing Docstrings for Modules
When writing docstrings for modules, you should provide an overview of what the module does and highlight the key classes or functions within it. Here’s an example:
"""
math_operations module.
This module provides basic arithmetic operations including addition, subtraction,
multiplication, and division. It is designed to be used in simple mathematical calculations.
Classes:
- Calculator: A class to perform basic arithmetic operations.
"""
This docstring gives a brief summary of what the module is for and mentions the Calculator
class, which is the primary class within the module.
Tools and Libraries That Utilize Docstrings
One of the great things about Python docstrings is that they’re not just for humans—they can be used by various tools and libraries to automatically generate documentation, check code quality, and more.
1. Sphinx
Sphinx is a powerful tool that can automatically generate documentation from your code. It uses your docstrings to create HTML, PDF, and other formats of documentation. Sphinx is commonly used for documenting Python projects because it supports reStructuredText, a markup language that allows you to format your docstrings nicely.
# Example of generating documentation using Sphinx
sphinx-apidoc -o docs/ mymodule/
2. Pydoc
Pydoc is a built-in Python tool that can generate documentation in HTML or text format from your docstrings. It’s a quick and easy way to generate documentation without needing to install any third-party tools.
# Generate HTML documentation for a module
pydoc -w mymodule
Commenting in Collaborative Projects
When working on a collaborative project, communication is key. One of the most effective ways to ensure that everyone on your team is on the same page is through consistent commenting practices in your code. Comments are not just notes for yourself—they’re a bridge to connect your ideas with others in your team.
Let’s dive into how to make comments work for everyone involved in a collaborative project, from establishing team standards to their role in code reviews.
Establishing Commenting Standards in a Team
In any team project, it’s crucial to have clear and consistent standards for commenting. Without them, code can quickly become difficult to maintain and understand, especially as the team grows or as new members join the project. Here’s how to set up commenting standards that work for everyone:
1. Agree on a Commenting Style:
Whether it’s using single-line comments or multi-line comments, your team should agree on a common style. This ensures that the codebase remains consistent and that anyone reading the code can easily understand the comments.For instance, some teams prefer using single-line comments with the #
symbol for brief explanations:
# Initialize the list with default values
my_list = [0] * 10
Others might opt for more detailed multi-line comments when explaining complex logic:
"""
Calculate the factorial of a number recursively.
This function calls itself with the number - 1
until it reaches the base case where the number is 1.
"""
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n - 1)
2. Define When to Comment:
Not every line of code needs a comment, but critical sections—such as complex logic, workarounds, or code that’s not immediately intuitive—definitely do. Your team should agree on what constitutes a “critical section” to ensure consistency.
Example: If a particular algorithm is non-standard or if there’s a reason why a specific approach was chosen, it’s good practice to document that decision.
# Using a binary search for performance reasons, as the list is always sorted
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
3. Regularly Review Commenting Practices:
As your project evolves, so should your commenting standards. Regular check-ins during team meetings can help ensure that everyone is following the agreed-upon practices and that new team members are onboarded with the same standards.
The Role of Comments in Code Reviews
Code reviews are an integral part of any collaborative project, and comments play a significant role in making these reviews effective. Here’s how comments can enhance code reviews:
- Clarifying Intent: During a code review, the reviewer’s job is to understand the purpose of the code and ensure that it meets the project’s requirements. Well-placed comments can help the reviewer quickly grasp the intent behind specific sections of code, making the review process smoother.
Example: If a function has a non-obvious purpose or if there’s a reason why a particular approach was taken, a comment can make that clear.
# Using a binary search for performance reasons, as the list is always sorted
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
3. Regularly Review Commenting Practices: As your project evolves, so should your commenting standards. Regular check-ins during team meetings can help ensure that everyone is following the agreed-upon practices and that new team members are onboarded with the same standards.
The Role of Comments in Code Reviews
Code reviews are an integral part of any collaborative project, and comments play a significant role in making these reviews effective. Here’s how comments can enhance code reviews:
- Clarifying Intent: During a code review, the reviewer’s job is to understand the purpose of the code and ensure that it meets the project’s requirements. Well-placed comments can help the reviewer quickly grasp the intent behind specific sections of code, making the review process smoother.Example: If a function has a non-obvious purpose or if there’s a reason why a particular approach was taken, a comment can make that clear.
# This function is designed to handle edge cases in user input that we found during testing
def handle_edge_cases(input_data):
# Specific edge case handling logic
pass
2. Facilitating Discussions: Comments can also be a starting point for discussions during code reviews. If something isn’t clear, the reviewer can ask for more detailed comments, or suggest changes to the existing ones. This interaction helps improve the overall quality of the codebase.
3. Ensuring Code Maintainability: A key part of code reviews is ensuring that the code is maintainable in the long term. Comments help future-proof the code by explaining complex logic, noting assumptions, and detailing any workarounds or hacks that were necessary.
Example: If a certain function uses a workaround due to a library limitation, it should be clearly documented.
# Using a temporary workaround due to a bug in the third-party library (v1.2.3)
# This should be removed once the library is updated to v1.2.4
def workaround_function():
pass
Commenting in a collaborative project is more than just a best practice—it’s essential for maintaining code quality, enhancing communication, and ensuring that everyone on the team is on the same page.
Automating Comment Quality Checks
When working on a project, ensuring that your code is well-documented with high-quality comments is essential. But manually checking each comment can be time-consuming, especially in large codebases or when working in a team. This is where automating comment quality checks comes into play. By using the right tools and integrating them into your development workflow, you can maintain the quality of your comments without adding extra burden to your daily routine.
Tools for Checking Comment Quality
Several tools are available to help you automatically check the quality of comments in your Python code. Two popular options are Pylint and Flake8. These tools can be configured to analyze your code for various quality issues, including the quality of comments.
- Pylint: Pylint is a comprehensive tool that checks your Python code for errors, enforces a coding standard, and can be configured to check for comment quality. Pylint can ensure that comments are present where needed and that they adhere to your team’s commenting standards.
- Example: Pylint can be configured to flag missing docstrings in functions, classes, and modules, ensuring that all critical parts of your code are properly documented.
pylint --disable=C0114,C0115,C0116
In this example, C0114, C0115, and C0116 are the codes that Pylint uses for missing module, class, and function docstrings. Disabling them will ignore docstring checks, but you can enable them if you want to enforce docstring requirements.
2. Flake8: Flake8 is another popular tool that combines several Python tools (like PyFlakes, pycodestyle, and Ned Batchelder’s McCabe script) to check for errors and enforce style guides. Flake8 can be extended with plugins to check for specific commenting practices, making it a adaptable option for maintaining comment quality.
- Example: You can use the
flake8-docstrings
plugin to enforce docstring conventions in your code.
pip install flake8 flake8-docstrings
flake8 --select=D
- The
--select=D
option will check for docstring-related issues, helping you ensure that all docstrings are correctly formatted and present where needed.
Integrating Comment Checks into CI/CD Pipelines
To maintain a consistent level of quality in your project, it’s a good idea to integrate comment checks into your Continuous Integration/Continuous Deployment (CI/CD) pipeline. This way, every time code is pushed to your repository or a pull request is made, these tools automatically run, flagging any issues with comments before the code is merged.
- Setting Up CI/CD for Comment Checks: Most CI/CD services, like GitHub Actions, Travis CI, or Jenkins, can easily be configured to run tools like Pylint or Flake8 as part of the build process.
- Example using GitHub Actions:Create a
.github/workflows/lint.yml
file in your repository:
- Example using GitHub Actions:Create a
name: Lint
on: [push, pull_request]
jobs:
lint:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
pip install flake8 flake8-docstrings
- name: Run Flake8
run: |
flake8 --select=D .
- This configuration will run Flake8 every time code is pushed or a pull request is made. It will specifically check for docstring issues, helping to ensure that all necessary documentation is present and correctly formatted.
Example Code
To illustrate how automated comment checks work, here’s a simple example of a Python function that includes docstrings. Suppose we use Pylint to enforce that every function has a docstring.
def add_numbers(a, b):
"""
Add two numbers and return the result.
Parameters:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of the two numbers.
"""
return a + b
def subtract_numbers(a, b):
return a - b # This function will trigger a warning from Pylint due to the missing docstring
When you run Pylint on this code, it will flag the subtract_numbers
function for missing a docstring, reminding you to document the function’s purpose and parameters. This helps maintain a consistent level of documentation across your codebase, making it easier for others to understand and maintain the code.
Automating comment quality checks is a powerful way to ensure that your code remains well-documented, understandable, and maintainable. By using tools like Pylint and Flake8 and integrating them into your CI/CD pipeline, you can save time, reduce errors, and ensure that your project adheres to the highest standards of code quality.
Common Mistakes to Avoid with Python Comments

Writing comments in Python code is essential for creating clear, maintainable, and collaborative code. However, it’s easy to make mistakes that can reduce the effectiveness of your comments or even create confusion. Let’s explore some common mistakes and how to avoid them to keep your codebase clean and understandable.
Ignoring Comments in Code
One of the biggest mistakes a developer can make is ignoring the need for comments altogether. When code lacks comments, it can become difficult to understand, especially for someone who didn’t write it. This is particularly true when working in teams or returning to your code after some time. Not using comments can lead to misunderstandings, wasted time, and even bugs if future developers (or your future self) misinterpret the code’s logic.
- Consequences of Not Using Comments:
- Reduced Code Readability: Without comments, others (or even you) may struggle to understand the code, especially if it’s complex.
- Increased Maintenance Time: Developers may spend more time deciphering the code rather than improving or fixing it.
- Higher Risk of Errors: Misunderstanding the code can lead to incorrect modifications, causing bugs or unexpected behavior.
- Example of Poorly Documented Code:
def calculate(x, y):
return x * y + x / y
- Without comments, it’s unclear what the
calculate
function is supposed to do. Is it performing some specific mathematical operation? Or is it part of a larger calculation? Future developers would have to guess or reverse-engineer the logic, which is time-consuming and prone to errors.- Impact: If another developer doesn’t understand this function’s purpose, they might misuse it, leading to incorrect results.
Using Comments to Explain the Obvious
Another common mistake is using comments to explain what the code is already making clear. Comments should add value by explaining the “why” behind the code, not the “what” that is already obvious from the code itself.
- Why Stating the Obvious in Comments Is Unhelpful:
- Clutter: Comments that state the obvious can clutter the code, making it harder to spot important information.
- Wasted Time: Future readers may waste time reading unnecessary comments that don’t provide additional context.
- Example of an Obvious Comment:
i = 0 # Initialize i to 0
The code is already clear—i
is being initialized to 0. A comment like this doesn’t add any new information and can actually be distracting.
- Better Approach: Focus on the reasoning behind more complex logic. For instance:
# Using a counter to track iterations, as it's needed for further calculations
i = 0
- Now, the comment explains why
i
is initialized, adding context that isn’t immediately clear from the code itself.
Overusing Multi-Line Comments
While multi-line comments can be useful, overusing them can lead to distractions. If the comment is too long, it can overwhelm the reader and interrupt the flow of reading the code. Multi-line comments should be reserved for explaining complex logic or providing context that isn’t easy to understand at first glance.
- When Multi-Line Comments Become a Distraction:
- Interrupting Code Flow: Lengthy comments can make the code harder to read, as developers have to scroll past them to get to the actual code.
- Risk of Becoming Outdated: Long comments are more likely to become outdated as the code changes, leading to potential confusion.
- Alternatives to Multi-Line Comments:
- Use Clear and Descriptive Function or Variable Names: Instead of long comments, ensure that your code is self-explanatory through well-chosen names.
- Break Down Complex Logic into Smaller Functions: By splitting complex logic into smaller, well-named functions, you reduce the need for long comments.
- Example of Overused Multi-Line Comment:
"""
This function takes two numbers as input, multiplies them together,
then adds the first number divided by the second number, and finally
returns the result of this operation.
"""
def calculate(x, y):
return x * y + x / y
This comment is overly detailed and restates what the code already does, without adding much value.
Improved Version:
# Perform a custom calculation based on multiplication and division
def calculate(x, y):
return x * y + x / y
Now, the comment is concise and provides context without overwhelming the reader.
How Comments Can Improve Your Python Coding Skills
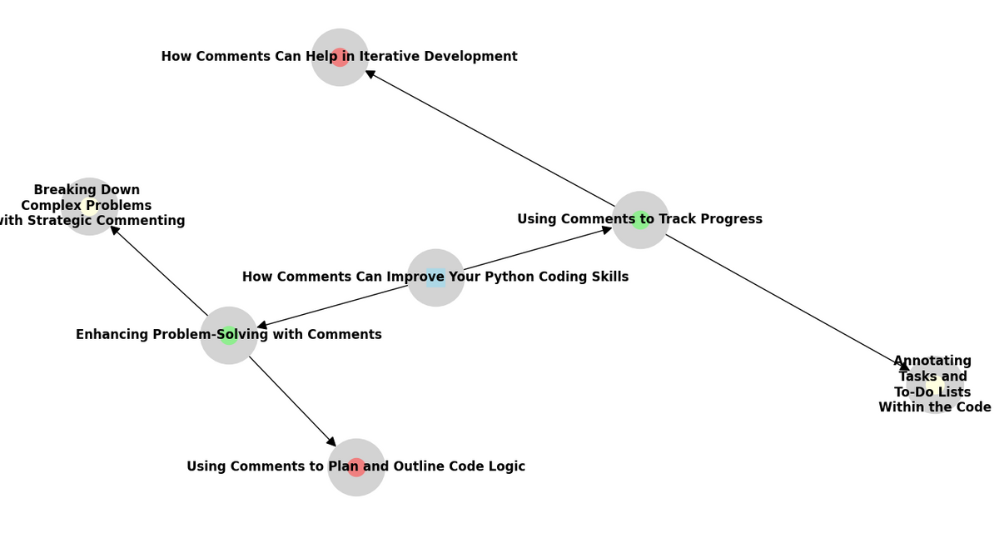
Enhancing Problem-Solving with Comments
Comments are more than just notes for future developers; they can be powerful tools to improve your problem-solving process. By using comments strategically, you can plan, outline, and break down complex code into manageable pieces, making development smoother and more efficient. Let’s explore how comments can play a crucial role in enhancing problem-solving in your Python code.
Using Comments to Plan and Outline Code Logic
When tackling a new coding problem, it’s easy to feel overwhelmed by the complexity of the task. However, using comments to outline your code logic before writing any actual code can be a game-changer. Comments can serve as a roadmap, guiding you step by step through the problem-solving process.
- Planning with Comments:
- Visualize the Solution: Before diving into the code, jot down the steps needed to solve the problem using comments. This helps in organizing your thoughts and ensuring you don’t miss any critical steps.
- Maintain Focus: Comments can help you stay focused on the task at hand, allowing you to address each part of the problem sequentially without getting sidetracked.
- Example:
# Step 1: Initialize variables
# Step 2: Loop through the data to find the target value
# Step 3: If target is found, return the index
# Step 4: If target is not found, return -1
def find_target(data, target):
# Your code here
- In this example, comments are used to outline the entire function’s logic before writing the actual code. This approach ensures that the developer understands the flow and can proceed with writing the code more confidently.
Breaking Down Complex Problems with Strategic Commenting
When faced with a complex problem, breaking it down into smaller, more manageable parts can make it less daunting. Strategic commenting allows you to divide the problem into sections, making it easier to tackle each part independently.
- Strategic Commenting:
- Identify Key Sections: Use comments to mark different sections of your code, making it easier to navigate and understand the overall structure.
- Simplify Complex Logic: By breaking down complicated logic into smaller parts, with comments explaining each step, you can reduce the chances of errors and make the code more understandable.
- Example:
def process_data(data):
# Step 1: Filter out invalid entries
valid_data = [item for item in data if item.is_valid()]
# Step 2: Sort the data based on priority
sorted_data = sorted(valid_data, key=lambda x: x.priority)
# Step 3: Process each item and collect results
results = []
for item in sorted_data:
# Check if item meets the criteria
if item.meets_criteria():
# Process the item
result = process_item(item)
results.append(result)
return results
- Here, comments break down the data processing function into clear steps, making it easier to understand and maintain. Each step is explained, so the developer can focus on one task at a time.
Using Comments to Track Progress
In iterative development, where code is frequently modified and improved, comments can be invaluable for tracking progress. They help you keep track of what’s been done and what still needs to be completed, ensuring that nothing falls through the cracks.
- Tracking Progress with Comments:
- Annotate Tasks: Comments can be used to create to-do lists within the code, allowing you to track tasks that need to be addressed in future iterations.
- Mark Progress: As you complete tasks, you can update or remove comments, keeping the codebase clean and organized.
- Example:
def analyze_data(data):
# TODO: Implement data validation
# TODO: Optimize the sorting algorithm
# Step 1: Clean the data
cleaned_data = clean_data(data)
# Step 2: Analyze the cleaned data
results = perform_analysis(cleaned_data)
# TODO: Add more analysis methods
return results
- In this example, comments are used to mark tasks that still need to be completed. This makes it easy to track what has been done and what needs attention in the next round of development.
Annotating Tasks and To-Do Lists Within the Code
Sometimes, you might encounter tasks that can’t be immediately addressed but need to be remembered for future work. Comments are perfect for annotating these tasks and creating to-do lists directly within your code.
- Annotating Tasks:
- Highlight Future Work: Use comments to mark areas that require further work or optimization. This ensures that these tasks are not forgotten and can be addressed in later iterations.
- Organize Workflows: By organizing tasks within your code using comments, you can maintain a clear workflow, helping to manage time and resources more effectively.
- Example:
def update_records(records):
# TODO: Add error handling for invalid records
# TODO: Implement logging for record updates
for record in records:
# Check if the record is valid
if validate_record(record):
# Update the record
update_record(record)
else:
# TODO: Handle invalid records
pass
# TODO: Verify that all records have been updated correctly
Here, comments are used to annotate tasks that need to be completed in the future. This helps in keeping track of what still needs to be done, ensuring that no task is overlooked.
Conclusion
The Role of Comments in Professional Python Development
Comments are essential to creating professional and maintainable Python code. They serve as a bridge between the developer’s thought process and the code itself, helping others (and even yourself in the future) understand the logic, intent, and decisions made during development. By providing clear, relevant, and timely comments, you ensure that your code remains understandable, even as it grows in complexity or changes hands in a team environment. In collaborative projects, well-commented code can reduce onboarding time for new team members, making it easier for them to contribute effectively. Additionally, comments can be a key factor in code reviews, as they provide context that might not be immediately apparent from the code alone.
How Comments Contribute to Professional, Maintainable Code
Professional code isn’t just about functionality; it’s about clarity and maintainability. Comments play a crucial role in achieving this by making your code more transparent and easier to follow. They help prevent misunderstandings and errors that can arise from unclear or ambiguous code. Moreover, comments can guide future development, reminding developers of the original intent behind a piece of code or highlighting areas that might need attention or improvement.
By making commenting a habit, you contribute to the creation of code that is not only functional but also easy to maintain and scale. This approach is especially important in large projects or teams, where multiple developers might work on the same codebase. Comments help maintain a consistent understanding of the code across the team, ensuring that everyone is on the same page.
Final Thoughts on Python Commenting Best Practices
When it comes to Python development, comments are more than just an afterthought—they are a key part of writing high-quality, maintainable code. By following best practices, such as keeping comments relevant and up-to-date, avoiding over-commenting, and using clear and concise language, you can significantly improve the readability and professionalism of your code.
Remember to use comments strategically to enhance problem-solving, track progress, and plan code logic. Tools like Pylint and Flake8 can help automate the process of checking comment quality, ensuring that your comments are as effective as possible.
Summary of Key Takeaways
- Comments are essential for maintainable code: They help make your code understandable to others (and yourself) in the future.
- Keep comments relevant and up-to-date: Always align your comments with your code to prevent confusion.
- Avoid over-commenting: Strike a balance between too many and too few comments; let your code speak when possible.
- Use clear and concise language: Avoid ambiguity in comments to ensure they are easily understood.
- Plan and outline with comments: Use comments to break down complex problems and track progress in your code.
Encouragement to Review and Improve Commenting Practices in Existing Code
As you move forward in your Python development journey, take time to review and improve your commenting practices in your existing codebase. Consider revisiting older code, and enhance comments to make them more relevant, clear, and helpful. Make commenting a habit that goes hand-in-hand with writing code, and encourage this practice within your team. By prioritizing quality comments, you contribute to a culture of professionalism and excellence in software development.
External Resources
Python’s Official Documentation on Comments: A brief overview of how comments work in Python, directly from the source.
PEP 8 – Style Guide for Python Code: The official Python style guide, which includes detailed recommendations on how to use comments effectively.
FAQs
Comments in Python are lines of text within your code that are ignored by the Python interpreter. They are used to explain what the code is doing, making it easier for others (and yourself) to understand the logic behind it. Comments improve code readability, assist in collaboration, and serve as documentation for future reference.
To write a single-line comment in Python, you use the #
symbol followed by your comment text. Anything after the #
on that line is treated as a comment. For example:
# This is a single-line comment explaining the code below
print(“Hello, World!”)
Single-line comments start with the #
symbol and apply to a single line of code. Multi-line comments, on the other hand, are typically written using triple quotes '''
or """
and can span multiple lines. They are often used for longer explanations or to comment out large blocks of code.
Multi-line comments are ideal when you need to explain a complex piece of code or provide detailed documentation within the code. They can also be used to temporarily disable a section of code during debugging or development.
Docstrings are a special type of comment used to describe the purpose of a function, class, or module. They are written using triple quotes '''
or """
and are placed immediately after the function, class, or module definition. Unlike regular comments, docstrings are stored as an attribute of the object they describe and can be accessed programmatically, making them useful for generating documentation.