Introduction: Learn How to Get User Input in Python (Step by Step)
When you first start learning Python, you might write programs that just show text on the screen. That’s a great start—but what if you want your program to ask the user a question and wait for them to type an answer?
That’s where the input()
function comes in.
In this post, I’ll teach you how to use the input() function in Python, even if you’re a complete beginner. We’ll go through easy examples so you can understand how to get user input in Python, step by step.
Whether you want to make a simple quiz, a calculator, or just greet someone by name, the input()
function is the tool that helps your program talk to the user.
By the end of this post, you’ll understand:
- What the
input()
function does - How to use it in real programs
- Why it’s important for basic Python user interaction
Let’s start learning.

What is the input()
Function in Python?
Let’s say you want your Python program to ask the user something — maybe their name or age. You want the user to type a response, and you want your program to use that response.
To do this, we use the input()
function.
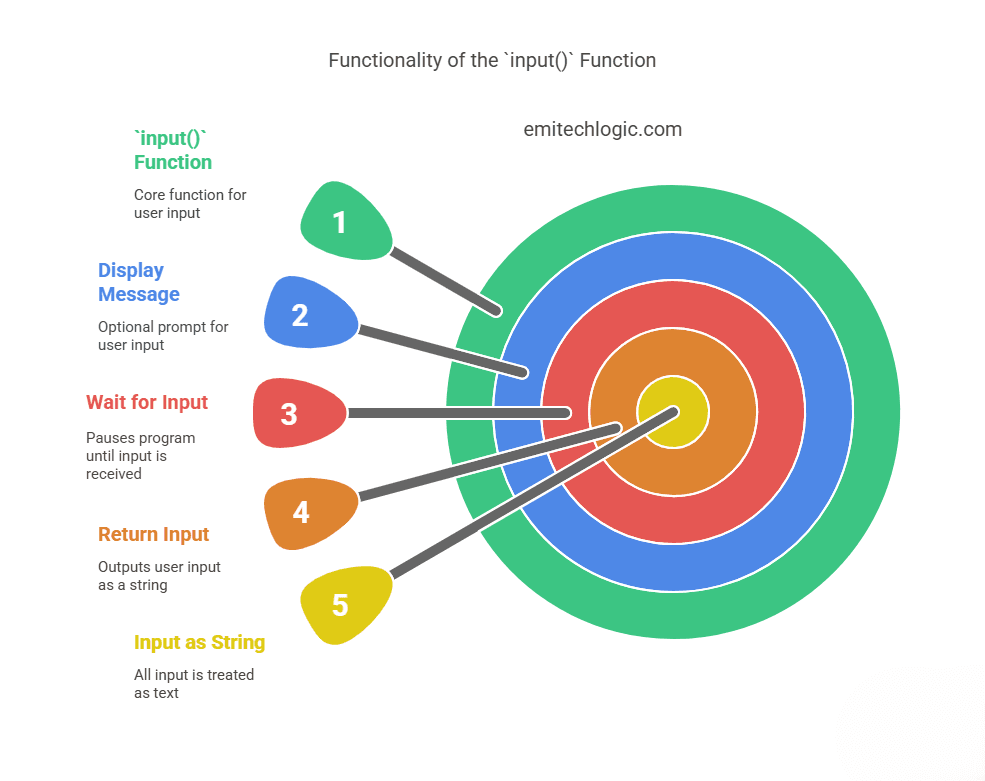
What does input()
do?
The input()
function does three simple things:
- It shows a message on the screen (if you want).
- Waits for the user to type something and press Enter.
- It gives that typed value back to your program.
Whatever the user types is stored as text — even if they type a number.
This is what people mean when they say:
“input() returns a string.”
Here’s the basic format (syntax):
input("Your message here")
That part inside the quotation marks is optional, but it’s a good way to tell the user what to type.
Now let’s try a real example.
Example: Asking the User’s Name
name = input("Enter your name: ")
print("Hello, " + name)
et me break it down for you:
input("Enter your name: ")
shows the message: Enter your name:- The program waits for the user to type something.
- Whatever the user types (let’s say
Alex
) gets saved into the variablename
. - Then the program says: Hello, Alex
Pretty cool, right?
Important: input() Always Returns a String
This is something many beginners miss.
Even if you type a number, like 25
, Python will treat it as "25"
— which is a string, not a number.
That’s why if you want to do math with input, you’ll need to convert it. But don’t worry — I’ll show you how to do that next.
Great! Now that you understand the basics of the input()
function, let’s take it a step further and learn how to work with numbers.
Remember, the input()
function always gives you text (string), even if the user types a number. So, if you want to do math with that input (like adding, subtracting, or multiplying), you need to convert the input into a number.
Let me show you how to do this.
How to Take Number Input in Python
When you’re asking for a number, you can use the int()
or float()
functions to convert the user input from a string into a number.

int()
— Convert to an Integer
If you want to ask the user for a whole number (no decimal points), you’ll use int()
to turn the input into an integer.
Here’s how you do it:
age = input("Enter your age: ")
age = int(age) # This converts the input to an integer
print("Next year, you'll be", age + 1)
Let me explain what happens here:
- The program asks: Enter your age:
- The user types their age (let’s say
25
). input()
function gives you"25"
as a string.- The
int(age)
part converts that string"25"
into the number25
. - The program adds
1
to the number and says:
➤ Next year, you’ll be 26
float()
— Convert to a Float (Decimal Number)
If you want to ask for a decimal number (like 5.5
), use float()
. This will convert the input into a float (a number with a decimal point).
Here’s an example:
price = input("Enter the price of the item: ")
price = float(price) # This converts the input to a float
print("The price with tax is", price * 1.2)
In this example:
- The program asks: Enter the price of the item:
- The user types a number with a decimal (for example,
19.99
). input()
function gives you"19.99"
as a string.- The
float(price)
part converts"19.99"
into the decimal number19.99
. - The program multiplies it by
1.2
to add tax, and shows the result.
Important Reminder: Always Convert When Doing Math!
If you forget to convert the input and try doing math directly, Python will give you an error. Here’s an example of what won’t work:
age = input("Enter your age: ")
print(age + 5) # This will cause an error
The program will try to add a string (age
) and a number (5
), which doesn’t make sense to Python.
That’s why you always need to convert the input to the correct type—either int()
for whole numbers or float()
for decimals.
Practice Time!
Let’s see how you do with a simple exercise:
# Ask for a number
num1 = input("Enter a number: ")
num2 = input("Enter another number: ")
# Convert to integers
num1 = int(num1)
num2 = int(num2)
# Add the two numbers
print("The sum of the numbers is:", num1 + num2)
This one will ask for two numbers, convert them into integers, and then add them together. Try it out!
Now, let’s take things to the next level: handling invalid user input. This is super important when you’re building real programs because users can sometimes type things that you didn’t expect—like letters when you’re asking for numbers. You don’t want your program to crash because of that!
Let’s walk through how we can handle invalid input in Python using try-except blocks. This is a technique that lets you catch errors and respond to them without crashing the program.
Handling Invalid User Input Gracefully
When you use input()
, users might type something that doesn’t match what you expect. For example, if you ask for a number and they type in letters, it will cause an error.
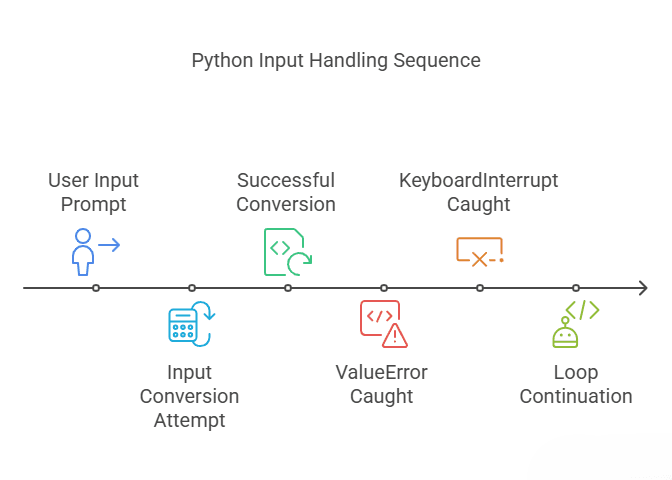
To prevent your program from crashing, you can use a try-except block.
What is a try-except block?
- try: You put the code that might cause an error here (e.g., converting input to a number).
- except: This part handles the error if it happens. You can give a message or take action to fix things.
Example: Handling Invalid Input (Number)
Let’s say you ask the user to enter a number, but they might type something like “abc” (instead of a number). You can catch that and show a helpful message.
Here’s how you do it:
try:
# Ask for a number
number = int(input("Enter a number: "))
# Print the square of the number
print("Squared:", number ** 2)
# Handle the error if the user didn't enter a valid number
except ValueError:
print("Oops! That wasn't a number.")
Let’s break this down:
- try: The program asks for a number and tries to convert it using
int()
. - If the user types a valid number (like
5
), it squares the number and prints it. - except ValueError: If the user types something like letters or any invalid input that can’t be converted to an integer, Python will raise a
ValueError
(becauseint()
can’t handle non-numeric input). - The
except
block catches that error and prints a friendly message: Oops! That wasn’t a number.
Why Use try-except for Input Validation?
Without this, if the user types something wrong (like “hello”), the program will crash. With input validation in Python using try-except
, your program keeps running smoothly, even if users make mistakes.
Example: Handling Multiple Types of Invalid Input
Let’s expand this a bit. Sometimes you want to handle multiple kinds of bad input—like asking for a float but the user enters letters or a blank space.
Here’s how you can handle more than one type of input error:
try:
# Ask for a number
number = float(input("Enter a number: "))
# Print the square of the number
print("Squared:", number ** 2)
except ValueError:
print("Oops! That wasn't a valid number.")
except KeyboardInterrupt:
print("\nYou cancelled the input.")
- ValueError: If the user types something that can’t be converted to a number.
- KeyboardInterrupt: If the user presses
Ctrl + C
to stop the program.
Now, if the user does something wrong, like typing a letter or cancelling the input, the program handles it gracefully and doesn’t crash.
Practice Time!
Try this: Let’s make a simple program that asks the user to enter their age, and if they type anything other than a number, the program tells them to try again.
while True:
try:
age = int(input("Enter your age: "))
print("Your age is:", age)
break # Exit the loop if the input is valid
except ValueError:
print("Oops! Please enter a valid number for your age.")
In this example:
- The program keeps asking until the user types a valid number.
- If the user enters something wrong, it gives a helpful message and asks again.
Now you know how to handle invalid user input in Python! By using try-except blocks, your program can stay smooth and user-friendly even if someone types the wrong thing. This is essential for making reliable programs that don’t crash unexpectedly.
Alright, let’s take this to the next level and learn how to loop with input()
for multiple entries. This is useful when you want your program to ask the user for input repeatedly until they decide to stop. You can do this with while loops or for loops.
Must Read
- Master Python String Formatting: Complete Guide
- 8 Advanced Types of AI Agents You Should Know About
- Escape Sequences and Raw Strings in Python: The Complete Guide
- Prime Factorization Algorithm in Python
- How to Get the First Digit of a Number in Python
Looping with input()
for Multiple Entries
When you want to ask the user for input multiple times—maybe a list of items, numbers, or anything else—you can use loops to keep asking until certain conditions are met.
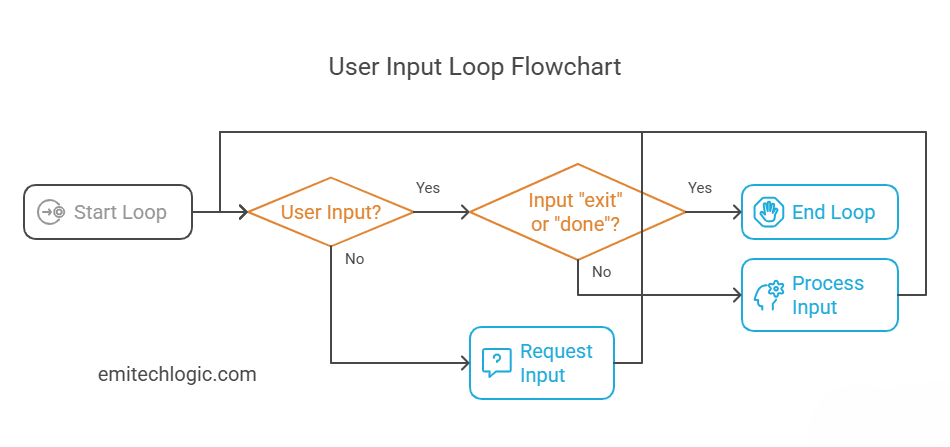
Example: Asking the User Repeatedly with a while
Loop
Let’s say we want the program to keep asking for text until the user types “exit”. This is where we use a while
loop.
while True:
# Ask for input
text = input("Type 'exit' to stop: ")
# Check if the user typed "exit"
if text == "exit":
break # Exit the loop if they typed "exit"
# Otherwise, show what they typed
print("You typed:", text)
What’s happening here?
- while True: This starts an infinite loop, meaning the program will keep asking the user for input forever unless something stops it.
- input(“Type ‘exit’ to stop: “): The program asks the user to type something.
- if text == “exit”: If the user types
"exit"
, the program stops the loop withbreak
. - print(“You typed:”, text): If they didn’t type
"exit"
, the program simply shows what they typed and asks again.
Example Flow:
- The program might ask: “Type ‘exit’ to stop: “.
- User types: “hello”.
- The program will say: “You typed: hello”.
- It will keep asking until the user types “exit”.
Another Example: Asking for Multiple Numbers with a while
Loop
Let’s take this concept and ask the user for multiple numbers to add together. The loop will stop when they type "done"
.
total = 0
while True:
number = input("Enter a number (or 'done' to stop): ")
if number == "done":
break # Stop the loop if the user types 'done'
try:
# Convert the input to an integer
total += int(number)
except ValueError:
print("Oops! That wasn't a valid number.")
print("The total is:", total)
Breakdown:
total = 0
: We start by setting the total to zero.- The program asks for a number or
"done"
. - If the input is
"done"
, the loop stops. - Otherwise, it adds the number to the total.
try-except
: We handle the case where the user types something other than a number.- When
"done"
is typed, it shows the final total.
Example Flow:
- User:
5
- Program: Adds
5
to the total. - User:
10
- Program: Adds
10
to the total. - User:
"done"
- Program: Prints the total.
Using for
Loops for Fixed Repetitions
If you know exactly how many times you want to ask for input, a for
loop can be useful. For example, let’s say we want to ask the user for their favorite 3 foods.
for i in range(3):
food = input("Enter your favorite food: ")
print("You like", food)
Breakdown:
- for i in range(3): This loop will run exactly 3 times.
- Each time, the program will ask for a favorite food and print it back.
When Should You Use While vs. For Loops?
- Use a
while
loop when you don’t know how many times you’ll need to ask the user for input (like until they type"exit"
or"done"
). - Use a
for
loop when you know the exact number of repetitions (like asking for 3 favorite foods).
Let’s take a look at how the input()
function can be used in real-world scenarios! We’ll cover a few practical examples: a simple calculator, a user login prompt, and a survey system. These examples show how to use user input to make your programs more interactive and dynamic.
Real-World Examples of Using input() in Python
1. Simple Calculator with User Input
We’re going to build a simple calculator that lets the user enter two numbers and an operator, then performs a calculation based on that input.
Key Concepts:
input()
: Used to gather input from the user.float()
: We’ll convert the input into numbers (because input frominput()
is always a string).if-else
conditions: To choose which operation to perform (add, subtract, multiply, etc.).
How to Build It:
def calculator():
print("Welcome to the interactive calculator!")
while True: # This will make the calculator keep asking for input until the user decides to stop
# Step 1: Ask the user for the first number
num1 = float(input("Enter the first number: "))
# Step 2: Ask the user for an operator
operator = input("Enter operator (+, -, *, /): ")
# Step 3: Ask the user for the second number
num2 = float(input("Enter the second number: "))
# Step 4: Perform the operation based on the operator
if operator == "+":
result = num1 + num2
elif operator == "-":
result = num1 - num2
elif operator == "*":
result = num1 * num2
elif operator == "/":
if num2 != 0: # Prevent division by zero
result = num1 / num2
else:
print("Error: Division by zero!")
continue # Skip the rest of the loop if there's an error
else:
print("Invalid operator! Please try again.")
continue # Skip the rest of the loop and ask again
# Step 5: Display the result
print(f"The result is: {result}")
# Step 6: Ask if the user wants to continue
again = input("Do you want to calculate again? (yes/no): ")
if again.lower() != 'yes':
break # Exit the loop if the user says no
calculator()
What happens in the code:
- The user is prompted to enter two numbers and an operator.
- Based on the operator entered, the appropriate calculation is performed.
- If the user types anything other than
+
,-
,*
, or/
, they’re asked to enter a valid operator. - The program keeps running until the user types “no” when asked if they want to continue.
Try it out yourself:
- Run the code in your Python environment.
- Try entering different numbers and operators to see how it behaves.
2. User Login Prompt
Now, let’s move on to creating a user login system. This is a very basic login system where the user is asked for a username and a password, and the system checks if they match pre-set values.
Key Concepts:
input()
: Used to capture user input.if-else
conditions: To check whether the username and password match.- String comparison: We compare the entered username and password with stored values.
How to Build It:
def login_system():
stored_username = "user123"
stored_password = "password456"
print("Welcome to the login system!")
# Step 1: Ask for the username
username = input("Enter your username: ")
# Step 2: Ask for the password
password = input("Enter your password: ")
# Step 3: Check if both match the stored values
if username == stored_username and password == stored_password:
print("Login successful!")
else:
print("Invalid username or password.")
login_system()
What happens in the code:
- The program asks for the username and password.
- It checks if the entered username and password match the pre-set ones (in this case,
user123
andpassword456
). - If they match, the login is successful; otherwise, an error message is shown.
Try it out yourself:
- Test the system by entering the correct and incorrect credentials.
- Try changing the values of
stored_username
andstored_password
to see how the program behaves.
3. Formatted Survey Response
Next up is a survey system where you ask users for their input (name, age, favorite language), and then format and display their responses in a clean way.
Key Concepts:
input()
: To collect responses for different questions.- String formatting: To display the responses neatly.
How to Build It:
def survey():
print("Welcome to the User Survey!")
# Step 1: Ask the user for their name
name = input("What is your name? ")
# Step 2: Ask for the user's age
age = input("How old are you? ")
# Step 3: Ask for their favorite programming language
favorite_language = input("What is your favorite programming language? ")
# Step 4: Display the survey results
print("\nSurvey Results:")
print(f"Name: {name}")
print(f"Age: {age}")
print(f"Favorite Language: {favorite_language}")
survey()
What happens in the code:
- The program asks the user for their name, age, and favorite programming language.
- Then it neatly prints out all the collected information.
Try it out yourself:
- Run the survey and answer the questions to see how the results are formatted.
What You’ve Learned:
- Interactive Calculator: We’ve seen how to ask for user input and perform calculations based on it. We used loops, conditionals, and input() to create an interactive tool.
- User Login: We learned how to use input() for user authentication (asking for username and password). This is a very common task in real-world applications.
- Survey System: We built a basic survey system using input(). This example shows how to gather multiple pieces of information and display it back in a formatted way.
Try It Out:
Now, you can take these examples and make them your own:
- Modify the calculator to add more operations (like
**
for exponentiation). - Expand the login system to allow multiple users or even include a password change feature.
- Add more questions to the survey, or save the responses to a file.
Now, let’s explore some advanced techniques for using input in Python! We’ll look at faster alternatives and how to read multiple values at once from a single line. These can be very helpful for efficient input handling in certain scenarios.
Bonus: input() Alternatives and Advanced Usage

1. Using sys.stdin.readline()
for Faster Input
The input()
function is very useful, but it might not be the most efficient when you’re working with large amounts of data. For faster input handling, especially when reading multiple lines of input, we can use sys.stdin.readline()
.
Why is it Faster?
sys.stdin.readline()
reads input as raw bytes, which is generally quicker thaninput()
, especially when you’re reading large amounts of data or working with competitive programming challenges.- It also doesn’t add a newline character at the end, so you may need to handle that manually.
How to Use It:
import sys
# Using sys.stdin.readline() to read input
name = sys.stdin.readline().strip() # .strip() removes the newline character
print(f"Hello, {name}")
How it works:
sys.stdin.readline()
reads a full line of input..strip()
is used to remove any trailing newline characters that are automatically included when reading input.- The program then prints the name entered by the user.
Key Points:
- This method is especially useful when you need to process large datasets quickly, as it avoids some of the overhead that comes with
input()
. - You can use it in cases like reading a large number of test cases in a competitive programming setting.
Try it yourself:
Try using sys.stdin.readline()
and compare it with input()
to see the difference in performance when handling large amounts of input.
2. Reading Multiple Values at Once
In many cases, you’ll want to get multiple pieces of data from the user at once. For example, asking for multiple numbers in a single line and processing them separately.
How to Read Multiple Values in One Line:
We can use split()
with input()
to handle this efficiently. This allows you to read multiple space-separated values in one go.
Example: Reading Two Numbers
# Reading multiple values in one line
x, y = input("Enter two numbers separated by space: ").split()
# Convert the strings to integers
x = int(x)
y = int(y)
# Perform some operation
print(f"The sum of {x} and {y} is {x + y}")
How it works:
input().split()
reads the entire input line as a string and splits it into a list based on spaces.- The values are then assigned to the variables
x
andy
. - We then convert these values from strings to integers (since
input()
always returns a string). - Finally, we perform the sum and display it.
Key Points:
.split()
breaks up the input string by spaces by default. You can also specify a different delimiter if needed (e.g., commas, semicolons).- This is a very common and efficient way to gather multiple inputs from the user in a single line.
Try it yourself:
Try using split()
with input()
and experiment with reading more than two values. You could read three or more numbers, process them, and perform different operations like multiplication or division.
Summary: What You’ve Learned
- Faster Input with
sys.stdin.readline()
:sys.stdin.readline()
is a great alternative toinput()
when you need faster input handling, especially in situations where performance is key (like competitive programming).- It’s quicker because it directly reads raw input without processing or adding extra newline characters.
- Reading Multiple Inputs in One Line:
- Using
input().split()
allows you to gather multiple pieces of data at once from a single line of input. - This is perfect for cases where you want to process multiple values (like numbers or words) without asking the user repeatedly for each one.
- Using
These techniques will make your programs more efficient and flexible, especially when dealing with larger or more complex input scenarios.
Conclusion
In this blog post, we explored the powerful input()
function in Python and its various uses. Whether you’re just starting out with Python or building more complex programs, understanding how to gather user input is a crucial skill.
We covered:
- Basic usage of
input()
to interact with the user. - How to handle invalid input gracefully using try-except blocks to avoid crashes.
- Ways to use loops for multiple entries, making your programs more interactive.
- Real-world examples, including a simple calculator, a user login prompt, and a survey system, that highlight how to apply
input()
in different scenarios. - Advanced techniques like faster input using
sys.stdin.readline()
and reading multiple values in one line usingsplit()
.
By mastering these techniques, you’ll be able to create programs that are not only interactive but also efficient and user-friendly. Whether you’re building simple scripts or complex applications, the ability to collect and process user input is essential for creating responsive and engaging programs.
Keep experimenting with these techniques and make your Python programs even more dynamic!
Feel free to revisit the examples and try modifying them to suit your needs
FAQs
input()
function in Python? The input()
function allows the user to enter data during the execution of a program. It reads input as a string and can be used to interact with users, asking for their input in real-time.
input()
handle different types of data? By default, input()
returns data as a string. If you need to work with numbers or other types, you must explicitly convert the input using functions like int()
or float()
.
To handle invalid input, you can use try-except blocks. This ensures that if the user enters data of the wrong type (e.g., a letter instead of a number), the program doesn’t crash, and you can display an error message instead.
You can use input().split()
to take multiple inputs in one line. This method splits the input string into separate values based on spaces, and you can then process each value individually.
Additional resources
If you’d like to explore more about handling user input in Python, here are some helpful resources you can check out:
- Python Official Documentation – input() Function
A complete reference for theinput()
function from Python’s official docs. - W3Schools – Python User Input
Simple and beginner-friendly guide with examples to practice user input in Python. - Programiz – Python Input, Output and Import
A clear explanation of user input alongside output and importing concepts in Python.
These resources will help you practice more, explore advanced examples, and strengthen your confidence in building interactive Python programs.
Want to explore more Python topics step by step?
👉 Check out more guides at emitechlogic.com for hands-on tutorials
💡 Found this helpful?
Do me a favor — share this post with your friends, colleagues, or on your social media. You never know who might need a quick Python refresher before their next interview.
Follow for Python tips & tricks
📸 Instagram: https://www.instagram.com/emi_techlogic/
💼 LinkedIn: https://www.linkedin.com/in/emmimal-alexander/
#PythonQuiz #CodingInterview #PythonInterviewQuestions #MCQChallenge #PythonMCQ #EmitechLogic #UncoveredAI #PythonTips #AIwithEmi #PythonCoding #TechWithEmi
Leave a Reply