Introduction to Inheritance and Polymorphism in Python
If you’re a Python developer looking to level up your coding skills, mastering inheritance and polymorphism is a game-changer. These two concepts lie at the heart of object-oriented programming (OOP), helping you write cleaner, more efficient, and reusable code. Whether you’re building a small project or working on large-scale software, understanding how inheritance and polymorphism work will make your code easier to manage and extend over time.
In this guide, you’ll learn everything you need to know about inheritance and polymorphism in Python, from the basics to more advanced applications. We’ll walk you through clear examples and show you how these concepts are used in real-world scenarios. If you’re new to Python or just need a refresher, don’t worry! This post breaks everything down in a simple, easy-to-follow way.
What You’ll Learn in This Guide
By the end of this guide, you’ll be able to:
- Understand how inheritance allows you to reuse code and create relationships between classes.
- Use polymorphism to make your code more flexible and dynamic.
- Learn about Python’s latest advancements related to inheritance and polymorphism, like structural pattern matching.
- Apply best practices to avoid common mistakes when working with these powerful concepts.
Why Inheritance and Polymorphism Are Key in Python Programming
Why should you care about inheritance and polymorphism? Well, they allow you to organize your code better and reduce redundancy. With inheritance, you can create new classes that inherit attributes and methods from existing ones, which saves you from rewriting code. Polymorphism, on the other hand, enables different classes to be treated as if they are the same, making your program more flexible and easier to maintain.
Whether you’re a beginner looking to deepen your understanding of Python or an experienced coder wanting to write smarter, more efficient programs, this guide will help you master these key concepts.
Let’s Start!
Understanding Inheritance in Python
When we talk about Object-Oriented Programming (OOP), inheritance is one of the most important concepts. It allows you to build relationships between classes, enabling one class to “inherit” properties and behaviors from another. This concept can help in avoiding repetitive code and makes your programs more organized. If you’re learning Python for an online course, getting a solid understanding of inheritance is essential because it builds the foundation for more advanced topics, such as polymorphism and code reusability.
In this guide, we’ll explore different types of inheritance in Python, using code snippets and examples to break things down. And yes, I’ll be sharing a few personal tips that helped me when I first learned inheritance—it can be tricky, but with the right approach, it becomes much more manageable.

What Is Inheritance in Object-Oriented Programming (OOP)?
In OOP, inheritance means that one class (called the child class) can borrow or inherit features (attributes and methods) from another class (called the parent class or base class). This makes your code more reusable and reduces redundancy.
Think of it like this: If you’re creating a game, and you have a base class called Character
with attributes like health
and power
, you could then create other classes, like Hero
or Enemy
, that inherit those attributes. You wouldn’t need to rewrite the same logic for every type of character in the game.
Here’s a simple code snippet to illustrate inheritance:
Example of Basic Inheritance:
# Base class (Parent)
class Animal:
def __init__(self, name):
self.name = name
def sound(self):
return "Some sound"
# Child class inherits from Animal
class Dog(Animal):
def sound(self):
return "Bark"
# Creating objects
animal = Animal("Generic Animal")
dog = Dog("Buddy")
print(animal.sound()) # Output: Some sound
print(dog.sound()) # Output: Bark
In the example above, the Dog
class inherits from Animal
and overrides the sound
method.
Types of Inheritance in Python
Python supports different types of inheritance, each with its unique use case. Let’s walk through them step-by-step:
Example:
- Single Inheritance
- Multiple Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
Let’s explore each type and how they function in Python programming.
Single Inheritance in Python
Single inheritance means that a child class inherits from just one parent class. This is the simplest form of inheritance and helps you reuse code from a single base class.
Example of Single Inheritance:
class Parent:
def greet(self):
print("Hello from the Parent class!")
class Child(Parent):
pass
child = Child()
child.greet() # Output: Hello from the Parent class!
Here, the Child
class inherits from Parent
and automatically gets access to the greet
method.
Multiple Inheritance in Python
In multiple inheritance, a class can inherit from more than one parent class. This feature can be powerful but requires careful handling to avoid conflicts (like when methods with the same name exist in multiple parent classes).
Example of Multiple Inheritance:
class Father:
def __init__(self):
self.father_trait = "Hardworking"
class Mother:
def __init__(self):
self.mother_trait = "Caring"
class Child(Father, Mother):
def traits(self):
print(self.father_trait, self.mother_trait)
child = Child()
child.traits() # Output: Hardworking Caring
This is where Python’s Method Resolution Order (MRO) comes in, determining which parent class is called first.
Multilevel Inheritance in Python
In multilevel inheritance, a class inherits from another child class, creating a chain of inheritance. This can be helpful when you’re building more complex hierarchies.
Example of Multilevel Inheritance:
class Grandparent:
def family_trait(self):
print("This is a family trait.")
class Parent(Grandparent):
pass
class Child(Parent):
pass
child = Child()
child.family_trait() # Output: This is a family trait.
In this example, the Child
class inherits from Parent
, which in turn inherits from Grandparent
.
Hierarchical Inheritance in Python
In hierarchical inheritance, multiple child classes inherit from a single parent class. This is useful when you have a base class that provides shared functionality to multiple sub-classes.
Example of Hierarchical Inheritance:
class Vehicle:
def start(self):
print("Vehicle is starting")
class Car(Vehicle):
pass
class Bike(Vehicle):
pass
car = Car()
bike = Bike()
car.start() # Output: Vehicle is starting
bike.start() # Output: Vehicle is starting
Both Car
and Bike
classes inherit from the Vehicle
class, allowing them to use the start
method.
Hybrid Inheritance in Python
Hybrid inheritance is a mix of two or more types of inheritance. This can sometimes be complex to manage, but Python’s MRO (Method Resolution Order) helps keep things under control.
Let’s see an example that mixes hierarchical and multiple inheritance:
Example of Hybrid Inheritance:
class Animal:
def speak(self):
print("Animal sound")
class Mammal(Animal):
pass
class Bird(Animal):
pass
class Bat(Mammal, Bird):
pass
bat = Bat()
bat.speak() # Output: Animal sound
The Bat
class is a hybrid because it inherits from both Mammal
and Bird
, which ultimately inherit from Animal
.
When I first started with inheritance in Python, the concept of polymorphism kept popping up. And if you’re like me, it might feel overwhelming at first. But the key thing I discovered is this: polymorphism in Python allows child classes to have their own implementations of methods that exist in the parent class.
For example, all animals may have a method called sound
, but each subclass (like Dog
or Cat
) can have its own version of that method. This concept is what makes inheritance flexible in Python.
Must Read
- How to Design and Implement a Multi-Agent System: A Step-by-Step Guide
- The Ultimate Guide to Best AI Agent Frameworks for 2025
- Data Science: Top 10 Mathematical Definitions
- How AI Agents and Agentic AI Are Transforming Tech
- Top Chunking Strategies to Boost Your RAG System Performance
Benefits of Inheritance in Python
Inheritance is not just a fancy feature in object-oriented programming (OOP); it’s a practical tool that solves many common programming problems. By using inheritance and polymorphism in Python, you can significantly improve your code’s structure, making it more efficient and easier to manage. If you’re working on Python projects for an online course, understanding the benefits of inheritance will enhance your ability to build scalable and maintainable software.
Let’s explore the key benefits of inheritance in Python, with examples that illustrate why it’s so valuable.
1. Code Reusability and Modularity through Inheritance
One of the primary benefits of inheritance is code reusability. You can avoid rewriting the same code over and over by having one class (the parent class) define methods and attributes that other classes (the child classes) can inherit. This not only reduces redundancy but also promotes modularity, allowing you to break your code into smaller, reusable pieces.
Imagine you’re building an application where different types of vehicles (e.g., cars, bikes, trucks) share some common features, like starting and stopping. Instead of writing the same code for each vehicle type, you can create a base Vehicle
class and then inherit those common features in the Car
, Bike
, and Truck
classes.
# Base class
class Vehicle:
def __init__(self, name):
self.name = name
def start(self):
print(f"{self.name} is starting.")
def stop(self):
print(f"{self.name} is stopping.")
# Child classes inheriting from Vehicle
class Car(Vehicle):
pass
class Bike(Vehicle):
pass
# Create objects
car = Car("Toyota")
bike = Bike("Harley")
car.start() # Output: Toyota is starting.
bike.start() # Output: Harley is starting.
As you can see, the Car
and Bike
classes automatically gain access to the start
and stop
methods from the Vehicle
class. This helps you avoid writing duplicate code, making your programs more modular and maintainable.
2. Easier Maintenance and Updates in Python Programs
Another significant advantage of inheritance is that it makes maintenance much easier. When you want to update or modify your code, you can do so in one place, rather than hunting through multiple classes to make the same changes. This centralized control makes your program more flexible and scalable, especially when working on larger projects.
Suppose you want to add a new method that’s applicable to all vehicle types in the previous example. Instead of adding that method to each child class individually, you can simply add it to the base class. Every child class will then automatically inherit that new behavior.
Example (Adding a New Method):
# Base class
class Vehicle:
def __init__(self, name):
self.name = name
def start(self):
print(f"{self.name} is starting.")
def stop(self):
print(f"{self.name} is stopping.")
# New method added
def refuel(self):
print(f"{self.name} is refueling.")
# Child classes inheriting from Vehicle
class Car(Vehicle):
pass
class Bike(Vehicle):
pass
# Create objects
car = Car("Toyota")
bike = Bike("Harley")
car.refuel() # Output: Toyota is refueling.
bike.refuel() # Output: Harley is refueling.
By updating the Vehicle
class, you automatically extend the refuel
method to both Car
and Bike
. This reduces the effort required to maintain and update your code.
This is particularly helpful when building complex systems, like web applications or large data processing scripts, where even small changes can have widespread effects.
Tabulated Comparison: With and Without Inheritance
Let’s summarize how inheritance and polymorphism in Python make a difference in code quality.
Aspect | Without Inheritance | With Inheritance |
---|---|---|
Code Reusability | Repeated code across multiple classes. | Common code is reused across classes through inheritance. |
Maintenance | Harder to update – changes must be made in many places. | Easier to update – changes in the parent class affect all child classes. |
Modularity | Classes may become bloated with similar methods. | Classes are cleaner and focused on their specific tasks. |
Scalability | Difficult to manage as project grows. | Easier to scale by extending the parent class. |
Debugging and Testing | More lines of code to debug individually. | Less code to debug and test because of centralized logic. |
How to Implement Inheritance in Python
Inheritance is a key concept in object-oriented programming (OOP), and Python makes it both intuitive and practical. Inheritance allows us to define a new class that takes on the properties and behaviors of an existing class. This concept promotes code reusability, making our programs cleaner and more organized.

Basic Syntax of Python Inheritance
At its core, inheritance lets us create a child class that inherits attributes and methods from a parent class. In Python, this is achieved with the following basic syntax:
class ParentClass:
# Parent class attributes and methods
def __init__(self, name):
self.name = name
def display(self):
print(f"Name: {self.name}")
class ChildClass(ParentClass):
# Child class inherits from ParentClass
def __init__(self, name, age):
super().__init__(name)
self.age = age
def show_age(self):
print(f"Age: {self.age}")
# Creating an object of the ChildClass
child = ChildClass("John", 25)
child.display() # Inherited method
child.show_age() # Child class method
In this example, ChildClass
inherits the display()
method from ParentClass
, while adding its own method, show_age()
. The super()
function plays an essential role here, allowing us to call the parent class’s __init__
method and reuse its logic in the child class.
The Role of the super()
Function in Python Inheritance
When working with inheritance in Python, the super()
function is your go-to tool for referring to the parent class. It is most commonly used inside the child class’s __init__
method to inherit the parent’s constructor.
But super()
isn’t just limited to constructors. You can use it to invoke any method from the parent class, allowing for smooth interaction between child and parent classes.
Let me share a personal insight. I used super()
extensively in one of my recent projects. It helped simplify my code when I was working on a multi-layered class structure. The super()
function allowed me to access parent methods with ease, without having to hardcode references to the parent class. It’s such a great way to keep your code DRY (Don’t Repeat Yourself).
Here’s a deeper look at how super()
can be used:
class Animal:
def sound(self):
print("Some generic animal sound")
class Dog(Animal):
def sound(self):
super().sound() # Call the parent class method
print("Bark!")
# Creating an object of Dog class
dog = Dog()
dog.sound()
Output:
Some generic animal sound
Bark!
As you can see, the Dog
class calls the sound()
method from the Animal
class first before adding its own behavior. This layering of functionality can be really helpful in real-world applications.
Example of Single Inheritance in Python
This was covered earlier, so there’s no need to dive back into it. However, a reminder: single inheritance involves a child class inheriting from just one parent class. It’s often used when you want to extend functionality in a simple, straightforward way.
Example of Multiple Inheritance in Python
Multiple inheritance allows a child class to inherit from more than one parent class. Again, you’ve already seen this in detail, but I’ll add a personal reflection here: When I first started learning about multiple inheritance, I found it incredibly helpful for cases where different classes shared unrelated functionality. For example, you might want to inherit from both an Animal
class and a Vehicle
class to create something like a mechanical dog!
The challenge with multiple inheritance comes from potential method resolution order (MRO) conflicts. Python uses a technique called the C3 linearization algorithm to resolve which parent method should be invoked first. The super()
function helps here, too.
If you ever find yourself confused about method order in multiple inheritance, the mro()
method can be your friend:
class A:
def method(self):
print("A's method")
class B:
def method(self):
print("B's method")
class C(A, B):
pass
c = C()
c.method() # This will print A's method because of MRO
print(C.mro()) # Check method resolution order
Output:
A's method
[<class '__main__.C'>, <class '__main__.A'>, <class '__main__.B'>, <class 'object'>]
This method resolution order ensures Python follows a predictable path when calling methods in complex inheritance scenarios.
Using Inheritance in Larger Projects
While inheritance is a powerful tool, it’s important to use it wisely. When I worked on a large-scale project involving multiple classes, I found myself questioning when and where inheritance was truly needed. Sometimes, overusing inheritance can lead to overly complex and tangled code. A better alternative in those cases might be composition—where you use objects within other objects to share functionality.
Let me give you an example:
class Engine:
def start(self):
print("Engine started")
class Car:
def __init__(self):
self.engine = Engine() # Car has an Engine
def drive(self):
self.engine.start()
print("Car is driving")
my_car = Car()
my_car.drive()
This approach avoids some of the headaches of inheritance, while still promoting code reusability. Inheritance and Polymorphism in Python can be great, but composition sometimes offers a cleaner alternative, depending on the situation.
Common Mistakes and Pitfalls in Python Inheritance
When working with Inheritance and Polymorphism in Python, especially for those new to object-oriented programming, it’s easy to make a few common mistakes. These issues can lead to unexpected behavior or harder-to-maintain code. Let’s go over some of the typical errors and how to avoid them.
1. Overusing Inheritance
One of the most common mistakes is overusing inheritance where it might not be necessary. Inheritance should be used when there is a clear is-a relationship between the child and parent class. If the relationship is forced, it can lead to tightly coupled code that’s hard to modify.
Better Alternative:
If you find yourself forcing a class into an inheritance relationship, composition might be a better choice. Rather than making a class inherit methods and attributes, use composition to embed one class into another.
Example:
class Engine:
def start(self):
print("Engine started")
class Car:
def __init__(self):
self.engine = Engine() # Car "has-a" Engine, not "is-a" Engine
def drive(self):
self.engine.start()
print("Car is driving")
Here, instead of having a Car
inherit from an Engine
, it uses composition to create a cleaner, more flexible design. This prevents unnecessary inheritance and keeps the classes independent.
2. Forgetting to Call super()
in __init__
Another common pitfall is forgetting to call super()
in the child class’s __init__
method. This means that the parent class constructor is never invoked, leading to incomplete object initialization.
For example:
class Parent:
def __init__(self):
self.name = "Parent"
class Child(Parent):
def __init__(self):
pass # No call to super()
c = Child()
print(c.name) # This will throw an AttributeError since 'name' was never set.
To avoid this, always ensure you use super()
to call the parent’s constructor in the child class:
class Child(Parent):
def __init__(self):
super().__init__() # Correct call to super()
self.age = 10
3. Inconsistent Method Overriding
Method overriding is powerful, but inconsistent overriding can cause confusion. If you’re overriding methods without using super()
to maintain the parent’s behavior, the child class may function incorrectly. Always check whether the child class should extend the parent’s behavior or completely override it.
For example:
class Animal:
def sound(self):
print("Some generic animal sound")
class Dog(Animal):
def sound(self):
print("Bark") # Overrides the parent method entirely
Now, if you wanted to extend the parent method, you could use super()
:
class Dog(Animal):
def sound(self):
super().sound() # Calls the parent class method
print("Bark")
4. Inheriting from Too Many Classes
While multiple inheritance has its place, inheriting from too many classes can lead to more harm than good. It can introduce complexity and make code harder to follow, particularly with the diamond problem.
Let’s explore that more below.
Avoiding the Diamond Problem in Multiple Inheritance
When dealing with multiple inheritance, especially when classes are derived from a common ancestor, you may run into the diamond problem. This occurs when a child class inherits from two or more classes that both derive from the same parent. The name “diamond” comes from the structure it creates:
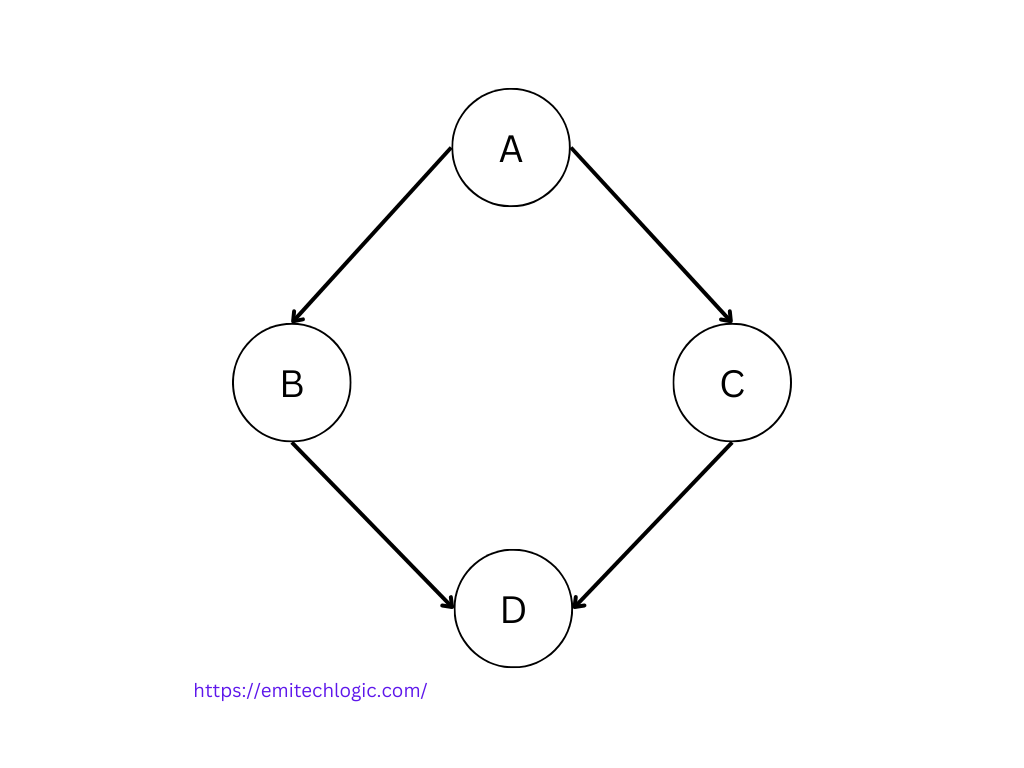
Here, D
inherits from both B
and C
, and both B
and C
inherit from A
. This can cause ambiguity—which version of the parent class’s method should D
inherit?
Example of Diamond Problem
class A:
def method(self):
print("Method in A")
class B(A):
def method(self):
print("Method in B")
class C(A):
def method(self):
print("Method in C")
class D(B, C):
pass
d = D()
d.method() # Which method gets called here?
Without careful planning, Python might invoke the wrong method or cause unexpected behavior. Fortunately, Python handles this through Method Resolution Order (MRO), which ensures that each class in the hierarchy is called only once, following the C3 linearization algorithm.
How to Avoid the Diamond Problem
- Using
super()
to Control Method Resolution: Python’ssuper()
ensures that each parent class method is called in the correct order, according to the MRO. Here’s how it works:
class A:
def method(self):
print("Method in A")
class B(A):
def method(self):
super().method()
print("Method in B")
class C(A):
def method(self):
super().method()
print("Method in C")
class D(B, C):
def method(self):
super().method()
print("Method in D")
d = D()
d.method() # This will follow the MRO and call methods in A, C, B, and D
Output:
Method in A
Method in C
Method in B
Method in D
2. Check the Method Resolution Order: Use the mro()
method to check the order in which Python will search for methods. This is especially useful when working with multiple inheritance.
print(D.mro())
Output:
[<class '__main__.D'>, <class '__main__.B'>, <class '__main__.C'>, <class '__main__.A'>, <class 'object'>]
By understanding and using the MRO and super()
, you can avoid ambiguity in multiple inheritance and ensure that methods are called in the correct order.
Understanding Polymorphism in Python
When working with Inheritance and Polymorphism in Python, polymorphism plays a crucial role in making object-oriented code flexible and reusable. Let’s break down what polymorphism is, how it works in Python, and the different types that developers often use.

What Is Polymorphism in Object-Oriented Programming?
Polymorphism, a key concept in object-oriented programming (OOP), allows objects of different classes to be treated as objects of a common superclass. This means that different classes can define methods with the same name, and Python will determine which method to execute based on the object instance. The word “polymorphism” itself means “many forms”, which reflects how an object can exhibit different behavior based on the class that implements it.
In practical terms, polymorphism allows functions and methods to process objects differently depending on their class. This provides the flexibility to use a unified interface for various types of data, making the code cleaner and easier to maintain.
Example of Polymorphism in Python:
class Bird:
def fly(self):
print("The bird is flying")
class Airplane:
def fly(self):
print("The airplane is flying")
class Superhero:
def fly(self):
print("The superhero is flying")
# Polymorphism in action
def let_it_fly(obj):
obj.fly()
bird = Bird()
airplane = Airplane()
superhero = Superhero()
let_it_fly(bird) # Outputs: The bird is flying
let_it_fly(airplane) # Outputs: The airplane is flying
let_it_fly(superhero) # Outputs: The superhero is flying
Here, even though Bird
, Airplane
, and Superhero
have nothing in common class-wise, the function let_it_fly
treats them all the same because each class implements a fly()
method. This is polymorphism at work.
Types of Polymorphism in Python
There are two main types of polymorphism in Python:
- Compile-Time Polymorphism (Method Overloading)
- Run-Time Polymorphism (Method Overriding)
1. Compile-Time Polymorphism in Python (Method Overloading)
In many languages, compile-time polymorphism (or method overloading) allows functions to have the same name but differ in the number or type of their arguments. While Python doesn’t support method overloading like some other languages (Java, C++), you can still achieve similar behavior using default arguments or handling argument types manually inside methods.
Example:
class MathOperations:
def add(self, a, b, c=0):
return a + b + c
math_op = MathOperations()
print(math_op.add(2, 3)) # Outputs: 5
print(math_op.add(2, 3, 4)) # Outputs: 9
In this example, we simulate method overloading by using a default value for the third argument (c
). This gives us the flexibility to call the same method with different numbers of parameters.
However, if you need more complex behavior, you can handle the logic within the method by checking the number of arguments or their types.
class MathOperations:
def add(self, *args):
return sum(args)
math_op = MathOperations()
print(math_op.add(2, 3)) # Outputs: 5
print(math_op.add(2, 3, 4, 5)) # Outputs: 14
class MathOperations:
def add(self, *args):
return sum(args)
math_op = MathOperations()
print(math_op.add(2, 3)) # Outputs: 5
print(math_op.add(2, 3, 4, 5)) # Outputs: 14
Here, using *args
allows us to handle a variable number of arguments, achieving a form of method overloading.
2. Run-Time Polymorphism in Python (Method Overriding)
Run-time polymorphism is achieved through method overriding. This occurs when a subclass overrides a method from its parent class to provide a specific implementation. This type of polymorphism is resolved during program execution, which is why it’s called run-time polymorphism.
In this case, when you call the overridden method, Python determines which version of the method to execute based on the object’s class at runtime.
Example:
class Animal:
def sound(self):
print("Animal makes a sound")
class Dog(Animal):
def sound(self):
print("Dog barks")
class Cat(Animal):
def sound(self):
print("Cat meows")
# Demonstrating run-time polymorphism
animal = Animal()
dog = Dog()
cat = Cat()
animal.sound() # Outputs: Animal makes a sound
dog.sound() # Outputs: Dog barks
cat.sound() # Outputs: Cat meows
In this example, both Dog
and Cat
inherit from Animal
, but each provides its own implementation of the sound()
method. When sound()
is called, Python determines which method to execute based on the object’s type at runtime.
Benefits of Polymorphism in Python
- Code Reusability: Polymorphism allows developers to write more generic and reusable code by working with objects of different types through a common interface.
- Improved Maintainability: Since different classes can have methods with the same name, developers can change a method’s implementation without affecting the overall structure, improving code maintainability.
- Extensibility: Adding new classes that follow the existing polymorphic pattern is easy, as new classes can be plugged into existing code without modification.
- Cleaner Code: Polymorphism makes code more readable and concise by allowing similar behavior across different objects without writing duplicate methods.
The Power of Polymorphism in Python
Polymorphism is one of the most powerful features in Python’s object-oriented programming paradigm. When used effectively, it can greatly enhance code flexibility and scalability, allowing developers to write more elegant and adaptable programs. With polymorphism, Python lets you design systems where the same operation can behave differently based on the object it’s acting upon. This not only makes the code reusable but also promotes cleaner and more maintainable structures. Let’s explore why polymorphism leads to flexible code and how it plays a critical role in Inheritance and Polymorphism in Python.
Why Polymorphism Leads to Flexible Code
Polymorphism allows different classes to be treated under a common interface, enabling you to build systems where components can be switched or extended with minimal effort. This kind of flexibility is especially important in large codebases, where changes or expansions can become cumbersome without such an approach.
For instance, in a software where multiple types of shapes exist (e.g., circles, rectangles, squares), polymorphism allows all these shapes to share a common draw()
method. Regardless of the specific shape, your code can interact with them uniformly without worrying about their internal details. This leads to better extensibility and the ability to modify parts of the program without breaking the overall structure.
Key benefit: Polymorphism lets you change or extend functionalities by adding new object types, without modifying existing code.
Key Differences Between Overloading and Overriding in Python
In the context of polymorphism, two important terms often come up: overloading and overriding. These terms describe how methods can exhibit different behaviors depending on the context, but they function in distinct ways.
Feature | Method Overloading | Method Overriding |
---|---|---|
Definition | Having multiple methods with the same name but different parameters in the same class. | A subclass provides a specific implementation of a method that is already defined in its parent class. |
Resolution | At compile-time (not directly supported in Python). | At run-time (Python executes the correct version of the method based on the object). |
Example | Function with default or variable arguments. | Subclass overriding a method from the superclass. |
Flexibility | Allows handling different input scenarios in the same method. | Allows for different behavior for a method based on the object instance. |
Examples of Polymorphism in Python
Let’s take a look at how polymorphism works in practice. Here’s an example where different types of classes implement a common method:
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
class Bird(Animal):
def speak(self):
return "Chirp!"
# Polymorphism in action
def animal_sound(animal):
print(animal.speak())
dog = Dog()
cat = Cat()
bird = Bird()
animal_sound(dog) # Outputs: Woof!
animal_sound(cat) # Outputs: Meow!
animal_sound(bird) # Outputs: Chirp!
In this example, the animal_sound()
function interacts with any object that has a speak()
method. Each class (Dog, Cat, Bird) provides its own implementation, demonstrating run-time polymorphism.
Polymorphism with Functions and Methods
Python allows you to implement polymorphism not just in class methods, but also with regular functions. This opens up even more ways to write flexible and reusable code. By creating a function that works with objects from multiple classes, you avoid writing redundant code.
Example:
def add(a, b):
return a + b
print(add(2, 3)) # Outputs: 5
print(add("Hello, ", "World!")) # Outputs: Hello, World!
Here, the add()
function works with both numbers and strings. Even though the +
operator behaves differently based on its arguments, Python handles it automatically. This is another instance of polymorphism in action.
Polymorphism with Classes and Inheritance
When combining Inheritance and Polymorphism in Python, you allow derived classes to inherit methods from a base class, while also enabling the derived class to override or extend those methods. This allows for more specific behavior in the child classes while maintaining a consistent interface.
In a scenario where you have a parent class Shape
, and child classes Circle
and Square
, polymorphism would allow you to work with all shape objects through the same interface (draw()
, for example), without worrying about the specific shape type.
class Shape:
def draw(self):
pass
class Circle(Shape):
def draw(self):
return "Drawing a circle"
class Square(Shape):
def draw(self):
return "Drawing a square"
# Polymorphism in action
shapes = [Circle(), Square()]
for shape in shapes:
print(shape.draw())
Dynamic Method Dispatch in Python
Dynamic method dispatch is a key mechanism in Python’s implementation of polymorphism. It refers to the process by which a call to an overridden method is resolved at runtime, not compile-time. This is what enables run-time polymorphism.
When a method is called on an object, Python determines the actual object type at runtime and calls the appropriate version of the method.
For example, if you call a method on an instance of a subclass, Python will execute the overridden method in the subclass, not the parent class’s version.
Real-Life Applications of Polymorphism in Python
Polymorphism can be found in a wide range of applications, from web development frameworks to game design and even machine learning pipelines. One common use case is creating reusable components in GUI applications, where various elements (buttons, text boxes, etc.) can share common methods but have distinct behaviors.
For example, in a game, you may have multiple character types like Player
, Enemy
, and NPC
(non-playable character), all inheriting from a base Character
class. By using polymorphism, you can handle all these characters through the same interface while allowing for specific behaviors in each subclass.
Using Polymorphism for Extensibility in Python Libraries
Many Python libraries leverage polymorphism to make their codebase extensible. For instance, libraries like matplotlib
, pandas
, and scikit-learn
allow developers to extend their functionalities by creating custom subclasses that override or extend built-in methods. This enables users to tailor the behavior of these libraries to suit their specific needs, without having to modify the original library code.
Polymorphism in Data Science and Machine Learning
In the world of data science and machine learning, polymorphism is widely used in the design of models and pipelines. Libraries like scikit-learn
use polymorphism to provide a consistent interface for different types of algorithms. For example, whether you’re working with a LogisticRegression
model or a RandomForest
model, both share common methods like fit()
, predict()
, and score()
.
This unified interface allows you to switch between different models easily within the same workflow, enhancing flexibility and making experimentation faster.
from sklearn.linear_model import LogisticRegression
from sklearn.ensemble import RandomForestClassifier
# Polymorphism in machine learning
models = [LogisticRegression(), RandomForestClassifier()]
for model in models:
model.fit(X_train, y_train)
predictions = model.predict(X_test)
In this example, both LogisticRegression
and RandomForestClassifier
implement the same interface, even though the underlying algorithms are completely different.
Inheritance and Polymorphism: A Deep Dive
Inheritance and polymorphism are foundational concepts in Python’s object-oriented programming (OOP), and when used together, they create an efficient and flexible code structure. Both concepts allow you to build systems where classes share behavior and respond in unique ways depending on their context, making your programs more extensible and maintainable.
In this section, we’ll explore how Inheritance and Polymorphism in Python work together, their best practices, and when it might be better to opt for composition over inheritance. Additionally, we’ll discuss common pitfalls and how to avoid overcomplicating your code by misusing inheritance.
How Inheritance and Polymorphism Work Together
When you combine inheritance and polymorphism, you can create systems where parent classes define common behaviors, and child classes provide specific implementations. Polymorphism, which allows objects to be treated as instances of their parent class, ensures that a child class’s unique behavior can still be accessed through a shared interface.
Consider the example of a base class Vehicle
, with two child classes: Car
and Bicycle
. The Vehicle
class might define common methods like move()
, but Car
and Bicycle
will implement this method differently:
class Vehicle:
def move(self):
pass
class Car(Vehicle):
def move(self):
return "Car is moving on the road"
class Bicycle(Vehicle):
def move(self):
return "Bicycle is pedaling down the path"
def start_movement(vehicle):
print(vehicle.move())
# Polymorphism in action
car = Car()
bicycle = Bicycle()
start_movement(car) # Outputs: Car is moving on the road
start_movement(bicycle) # Outputs: Bicycle is pedaling down the path
In this case, both Car
and Bicycle
can be treated as Vehicle
objects, but their specific implementation of the move()
method is what’s executed. This is polymorphism at work, allowing for flexibility and reusable code.
Best Practices for Using Inheritance and Polymorphism in Python
Using Inheritance and Polymorphism in Python effectively requires following certain best practices:
- Keep inheritance hierarchies shallow: Avoid deep inheritance trees, as they make the code harder to maintain and understand. The goal is to create simple, understandable hierarchies where child classes don’t get too far removed from the base class.
- Use polymorphism to promote interface consistency: If multiple classes share behavior (like in our
Vehicle
example), make sure they implement methods with the same names and parameters. This consistency allows polymorphism to work smoothly across different objects. - Avoid redundant code: Polymorphism helps eliminate redundant code. Instead of duplicating logic in multiple places, move shared functionality to the parent class and customize the behavior in child classes.
- Limit the use of the
super()
function: Whilesuper()
is useful for accessing a parent class’s method, overusing it can create hard-to-follow code, especially in deep hierarchies or when using multiple inheritance. Use it sparingly to ensure clarity.
When to Use Inheritance Over Composition
While inheritance is often a go-to solution for sharing behavior between classes, it’s not always the best choice. Composition (where objects are built using instances of other classes) can be a better alternative when you want to reuse functionality without forcing a relationship between the classes.
When to Use Inheritance:
A subclass should represent a type of its parent class. For instance, having a Dog class inherit from Animal works well because a dog falls under the category of animals. Inheritance is also useful when you need to reuse the parent class’s logic directly within the child class. Additionally, it becomes essential when enforcing a consistent interface across multiple related classes.
When to Use Composition:
A class should be used when there is a has-a relationship with another class. For example, modeling a Car class that includes an Engine is better accomplished through composition. Additionally, this approach allows for the combination of behaviors from different classes without imposing a rigid hierarchy. Flexibility takes precedence over shared interfaces in such scenarios.
Here’s an example of composition where a Car
class uses a Engine
object rather than inheriting from it:
class Engine:
def start(self):
return "Engine is starting"
class Car:
def __init__(self, engine):
self.engine = engine
def drive(self):
return self.engine.start() + " and the car is driving"
engine = Engine()
car = Car(engine)
print(car.drive()) # Outputs: Engine is starting and the car is driving
Composition keeps the Car
and Engine
classes loosely coupled, making the code more flexible to changes.
Avoiding Overuse of Inheritance for Simplicity
While inheritance is a powerful tool, overusing it can lead to complex, tightly coupled systems that are hard to manage. Before jumping into inheritance, consider whether it adds value to your design or if you’re better off using composition or even just a flat class structure.
Common Pitfalls:
- Deep inheritance trees: As you create more levels in an inheritance hierarchy, understanding how behavior flows between classes becomes harder. Keep things shallow and simple.
- Inheriting just for code reuse: If you’re using inheritance just to avoid duplicating code, it’s better to use composition or even utility functions. Inheritance should reflect logical relationships, not just code-sharing opportunities.
- The diamond problem in multiple inheritance: When multiple inheritance is involved, the diamond problem arises when two classes inherit from the same parent class. Python uses the Method Resolution Order (MRO) to resolve which parent class method to use, but this can introduce confusion.
Here’s an example of how to avoid the diamond problem:
class A:
def process(self):
return "Processing in A"
class B(A):
def process(self):
return "Processing in B"
class C(A):
def process(self):
return "Processing in C"
class D(B, C):
pass
d = D()
print(d.process()) # Outputs: Processing in B (based on MRO)
To avoid the diamond problem, you can use Python’s MRO and carefully design your inheritance structures. Composition can also help bypass this complexity entirely.
Advanced Topics in Inheritance and Polymorphism
As you continue your journey through Inheritance and Polymorphism in Python, you’ll encounter more advanced concepts that deepen your understanding and enhance your coding skills. In this section, we’ll explore abstract classes, the abc module, Method Resolution Order (MRO) in multiple inheritance, and the idea of duck typing. Each of these topics provides unique insights into how to use inheritance and polymorphism effectively.

Abstract Classes and Polymorphism
An abstract class serves as a blueprint for other classes. It cannot be instantiated on its own and is typically used to define common interfaces for derived classes. The idea behind abstract classes is to ensure that certain methods are implemented in the derived classes. This ties closely to the concept of polymorphism.
By using abstract classes, one can enforce a consistent interface while allowing flexibility in implementation. For instance, if you have a base class named Shape
, you might define an abstract method area()
. The derived classes, such as Circle
and Rectangle
, would then provide their specific implementations of this method.
Here’s an example using the abc
module in Python:
from abc import ABC, abstractmethod
import math
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return math.pi * self.radius ** 2
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
In this example, the Shape
class is abstract. It defines the area()
method without an implementation. Both Circle
and Rectangle
inherit from Shape
and implement the area()
method differently. This flexibility showcases how polymorphism allows different shapes to be handled through a common interface.
Using the abc
Module for Abstract Classes in Python
The abc
(Abstract Base Classes) module in Python provides the necessary tools to define abstract classes. By using decorators like @abstractmethod
, developers can specify which methods must be implemented in derived classes. This helps to avoid incomplete class definitions and ensures that the intended functionality is present.
Here’s a quick overview of how to create an abstract class using the abc
module:
- Import the ABC module: Start by importing
ABC
andabstractmethod
. - Define the abstract class: Inherit from
ABC
to create an abstract class. - Create abstract methods: Use the
@abstractmethod
decorator for methods that must be implemented.
Example:
from abc import ABC, abstractmethod
class Vehicle(ABC):
@abstractmethod
def start_engine(self):
pass
class Car(Vehicle):
def start_engine(self):
return "Car engine started."
class Truck(Vehicle):
def start_engine(self):
return "Truck engine started."
In this example, the Vehicle
class is abstract, and it mandates that any derived class, like Car
or Truck
, must implement the start_engine()
method. This approach provides a clear contract for developers, ensuring that all vehicles have an engine start method, promoting better organization and clarity in code.
Method Resolution Order (MRO) in Python Multiple Inheritance
When multiple inheritance is involved, the order in which classes are searched for a method or attribute can become complex. This is where Method Resolution Order (MRO) comes into play. MRO defines the order in which base classes are searched when executing a method.
Python uses the C3 linearization algorithm for determining MRO. To understand this better, consider a class hierarchy:
- Class
A
- Class
B
(inherits fromA
) - Class
C
(inherits fromA
) - Class
D
(inherits fromB
andC
)
To visualize this hierarchy, a simple diagram can be helpful:
In this case, if you call a method from class D
, Python will look for it in the following order:
D
B
C
A
You can view the MRO of a class using the __mro__
attribute or the mro()
method:
class A:
pass
class B(A):
pass
class C(A):
pass
class D(B, C):
pass
print(D.__mro__) # Output: (<class '__main__.D'>, <class '__main__.B'>, <class '__main__.C'>, <class '__main__.A'>, <class 'object'>)
Duck Typing and Polymorphism in Python
Duck typing is an important concept in Python that exemplifies polymorphism. The term comes from the phrase “If it looks like a duck and quacks like a duck, it must be a duck.” In programming, this means that the type of an object is less important than the methods it defines or the behaviors it exhibits.
For instance, consider a function that expects an object with a quack()
method. As long as the object provides this method, it can be used, regardless of its actual type. This flexibility allows developers to write more generic and reusable code.
Here’s an example demonstrating duck typing:
class Duck:
def quack(self):
return "Quack!"
class Person:
def quack(self):
return "I can mimic a duck!"
def make_it_quack(thing):
print(thing.quack())
# Creating instances
duck = Duck()
person = Person()
# Both can be passed to the function
make_it_quack(duck) # Output: Quack!
make_it_quack(person) # Output: I can mimic a duck!
In this example, both the Duck
and Person
classes implement a quack()
method. The function make_it_quack()
can accept both, demonstrating how polymorphism works in practice through duck typing.
Latest Advancements in Inheritance and Polymorphism in Python
The world of Inheritance and Polymorphism in Python is continuously evolving. With each new Python release, enhancements are introduced that refine how we can utilize these concepts in our code. This section explores some of the latest advancements, including structural pattern matching, type hints, and the @overload decorator. Each of these features contributes to making Python more expressive and type-safe, leading to cleaner and more maintainable code.
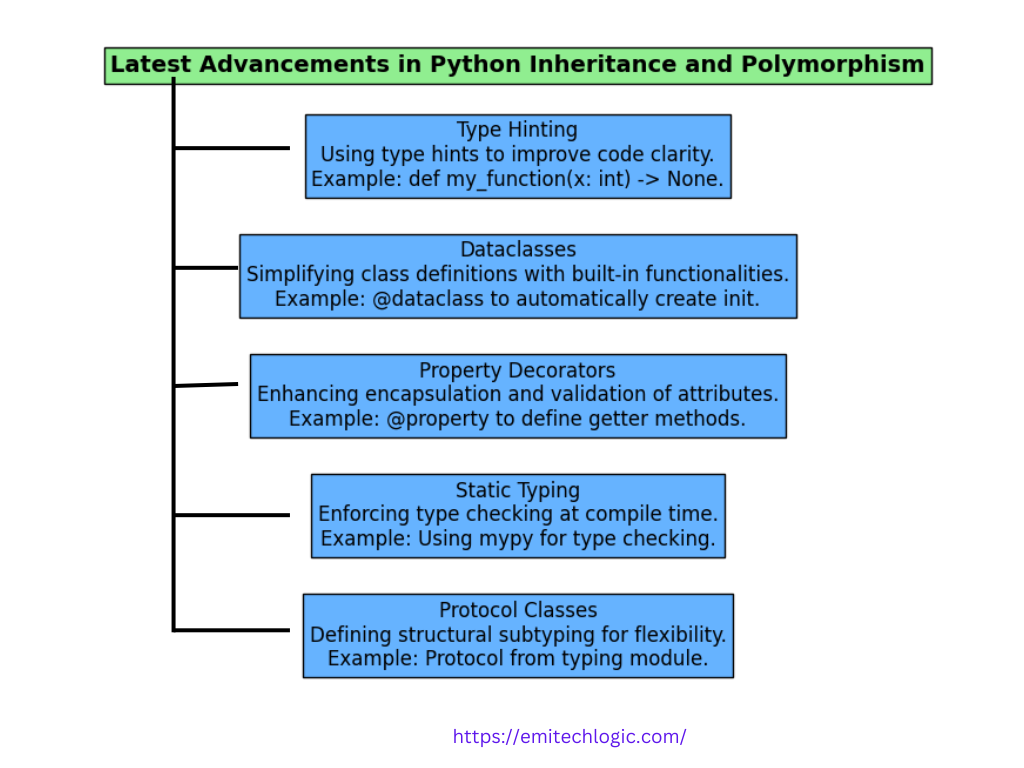
Introduction of Structural Pattern Matching in Python 3.10
One of the most exciting features introduced in Python 3.10 is structural pattern matching. This new syntax allows for more readable and concise handling of complex data structures, making it easier to implement polymorphic behavior.
What Is Structural Pattern Matching?
Structural pattern matching uses a new match
statement, which allows developers to match values against patterns. This feature enables a clearer expression of control flow based on the structure of the data rather than its type. It can simplify the code, especially in scenarios where classes and inheritance are involved.
Example of Structural Pattern Matching
Consider a scenario where different types of shapes need to be processed. The structural pattern matching can handle these types effectively.
class Circle:
def __init__(self, radius):
self.radius = radius
class Square:
def __init__(self, side):
self.side = side
def describe_shape(shape):
match shape:
case Circle(radius):
return f"A circle with radius {radius}"
case Square(side):
return f"A square with side {side}"
case _:
return "Unknown shape"
circle = Circle(5)
square = Square(4)
print(describe_shape(circle)) # Outputs: A circle with radius 5
print(describe_shape(square)) # Outputs: A square with side 4
In this example, the match
statement is used to determine the type of shape and return a corresponding description. This approach enhances readability and maintains the polymorphic nature of the shapes.
How Structural Pattern Matching Impacts Inheritance and Polymorphism
The introduction of structural pattern matching directly impacts how inheritance and polymorphism are implemented. It allows for a more concise way to handle different subclasses without resorting to numerous if-elif
statements.
By using pattern matching, classes can be treated based on their structure, enabling developers to write cleaner code. This also facilitates the handling of various inheritance hierarchies more intuitively. With pattern matching, it’s easier to add new shapes or classes without modifying existing logic, thereby promoting extensibility.
Type Hints and Static Typing Enhancements in Python 3.9+
With Python 3.9 and onwards, improvements in type hints and static typing have been made, allowing developers to specify the expected types of function arguments and return values. This addition provides better code clarity and helps prevent runtime errors.
Benefits of Type Hints
Type hints offer several benefits:
- Improved Readability: They serve as documentation, making it easier to understand the expected input and output of functions.
- Static Analysis: Tools like
mypy
can be used to catch type errors before the code is executed. - Enhanced IDE Support: Integrated Development Environments (IDEs) can provide better code completion and linting features based on type hints.
Example of Type Hints
def calculate_area(shape: Shape) -> float:
return shape.area()
rectangle = Rectangle(10, 5)
print(calculate_area(rectangle)) # Outputs: 50.0
In this example, the calculate_area
function expects a parameter of type Shape
and returns a float
. This clarity helps ensure that the correct types are used throughout the code, enhancing the implementation of polymorphism.
Using @overload Decorator for Function Overloading in Python
Python does not support traditional method overloading like some other languages. However, the @overload
decorator allows developers to indicate that a function can accept different types of arguments and behave accordingly.
How @overload Works
The @overload
decorator is used to define multiple signatures for a function. It provides a way to inform developers and tools about the various input types without enforcing strict checks at runtime. This feature can make functions more flexible and expressive.
Example of @overload
from typing import overload, Union
@overload
def process(value: int) -> str:
...
@overload
def process(value: str) -> str:
...
def process(value: Union[int, str]) -> str:
if isinstance(value, int):
return f"Processed integer: {value}"
elif isinstance(value, str):
return f"Processed string: {value}"
print(process(10)) # Outputs: Processed integer: 10
print(process("hello")) # Outputs: Processed string: hello
In this example, the process
function can accept either an integer or a string, returning a specific message based on the input type. The @overload
decorator helps clarify the expected types, improving code documentation.
Inheritance vs. Polymorphism in Python: Which to Use and When?
When learning about Inheritance and Polymorphism in Python, it can be easy to confuse these concepts. Both play essential roles in object-oriented programming, but they serve different purposes and have distinct characteristics. Understanding the differences between inheritance and polymorphism will help in making informed decisions about which tool to use in various situations.
Key Differences Between Inheritance and Polymorphism
Inheritance is a mechanism that allows a class (the child or subclass) to inherit attributes and methods from another class (the parent or superclass). This helps in creating a hierarchical relationship between classes and promotes code reusability.
Polymorphism, on the other hand, refers to the ability of different classes to be treated as instances of the same class through a common interface. In essence, polymorphism enables objects of different types to be processed in a similar way, allowing for flexibility in code.
Here’s a comparison table that outlines the key differences:
Feature | Inheritance | Polymorphism |
---|---|---|
Definition | Mechanism for creating new classes from existing ones | Ability for different classes to be treated as the same type |
Purpose | Promotes code reusability and hierarchical relationships | Enhances flexibility and allows for dynamic method calls |
Use Cases | Creating specialized classes from a base class | Implementing functions that can operate on different types of objects |
Example | Class Dog inherits from class Animal | Method sound() behaves differently in Dog and Cat classes |
Example of Inheritance
Consider a scenario where you have a general Animal
class and more specific classes such as Dog
and Cat
. The Dog
and Cat
classes can inherit common properties and methods from the Animal
class.
class Animal:
def speak(self):
return "Animal speaks"
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
dog = Dog()
cat = Cat()
print(dog.speak()) # Outputs: Woof!
print(cat.speak()) # Outputs: Meow!
In this example, Dog
and Cat
inherit the speak
method from Animal
, but they override it to provide their specific sounds. This illustrates how inheritance promotes code reuse while allowing subclasses to customize behavior.
Example of Polymorphism
Polymorphism allows the same method to be used on different objects. Here’s an example where both Dog
and Cat
can be treated as animals, and we can call the speak
method without worrying about their specific implementations.
def animal_sound(animal: Animal):
print(animal.speak())
animal_sound(dog) # Outputs: Woof!
animal_sound(cat) # Outputs: Meow!
In this code, the animal_sound
function takes an Animal
type parameter and can work with any object derived from Animal
. This demonstrates polymorphism in action.
Choosing the Right Tool for the Problem in Python
Deciding between inheritance and polymorphism depends on the specific problem you are trying to solve. Here are some guidelines to help you choose the right approach:
- Use Inheritance When:
- You have a clear hierarchical relationship between classes. For instance, if you are modeling a category of objects where subclasses share common behaviors.
- You want to reuse code across related classes. For example, if many subclasses share common attributes or methods, inheritance can minimize duplication.
- Use Polymorphism When:
- You need to design a system that requires flexibility. If different classes need to be treated uniformly without being tied to a specific type, polymorphism is ideal.
- You want to implement functions that can work with multiple types. Polymorphism allows you to create code that is more adaptable and easier to extend.
- Combine Both When:
- Complex applications often benefit from both inheritance and polymorphism. By using inheritance to establish relationships and polymorphism to enable flexible behavior, you can create a more efficient and maintainable codebase.
Debugging and Testing Inheritance and Polymorphism in Python
Understanding how to debug and test Inheritance and Polymorphism in Python is crucial for maintaining high-quality code. Debugging helps identify issues, while testing ensures that the code behaves as expected. This section will explore common errors, methods to fix them, and best practices for testing.

Common Errors in Inheritance and Polymorphism
When working with inheritance and polymorphism, several errors can arise. Being aware of these common pitfalls can save time and frustration.
- TypeError in Polymorphism: This error often occurs when an object is not of the expected type. For example, if a function expects an instance of a base class, but a subclass instance is mistakenly passed, a
TypeError
can be raised.Example:
class Animal:
def sound(self):
return "Some sound"
class Dog(Animal):
def sound(self):
return "Woof!"
def make_sound(animal: Animal):
print(animal.sound())
cat = Animal()
make_sound(cat) # Correct: Outputs: Some sound
dog = Dog()
make_sound(dog) # Correct: Outputs: Woof!
# If a list is passed instead of an instance
# make_sound([1, 2, 3]) # This raises a TypeError
2. Method Resolution Order (MRO) Conflicts: In cases of multiple inheritance, the method resolution order can lead to conflicts. Python uses the C3 linearization algorithm to determine the order in which classes are searched for methods, but this can sometimes lead to unexpected behaviors.
Example:
class A:
def greet(self):
return "Hello from A"
class B(A):
def greet(self):
return "Hello from B"
class C(A):
def greet(self):
return "Hello from C"
class D(B, C): # D inherits from B and C
pass
obj = D()
print(obj.greet()) # Outputs: Hello from B
Fixing TypeError in Polymorphism and Inheritance
To address TypeError
issues, a few strategies can be employed:
- Type Checking: Ensure that the object being passed matches the expected type using
isinstance()
. This approach can help catch errors before they occur.Example:
def make_sound(animal: Animal):
if not isinstance(animal, Animal):
raise TypeError("Expected an Animal instance")
print(animal.sound())
- Using Abstract Base Classes: Defining abstract methods in base classes can enforce a structure for subclasses, reducing the likelihood of runtime errors.
Example:
from abc import ABC, abstractmethod
class AbstractAnimal(ABC):
@abstractmethod
def sound(self):
pass
class Dog(AbstractAnimal):
def sound(self):
return "Woof!"
Resolving MRO (Method Resolution Order) Conflicts
Understanding and resolving MRO conflicts is essential for effective multiple inheritance management. Here are some techniques:
- Using
super()
: This built-in function can be used to call methods from parent classes in a controlled manner, helping to avoid conflicts.Example:
class A:
def greet(self):
return "Hello from A"
class B(A):
def greet(self):
return super().greet() + " and B"
class C(A):
def greet(self):
return super().greet() + " and C"
class D(B, C):
def greet(self):
return super().greet() + " and D"
obj = D()
print(obj.greet()) # Outputs: Hello from A and C and D
2. Reviewing the MRO: You can use the __mro__
attribute or the mro()
method to inspect the method resolution order for a class.
Example:
print(D.mro()) # Outputs the MRO for class D
Unit Testing for Inheritance and Polymorphism in Python
Testing inheritance and polymorphism ensures that the code functions as intended. Here are key points to consider:
Best Practices for Writing Unit Tests
- Test Class Behavior: Write tests that confirm the expected behavior of each class, especially when methods are overridden.
- Test for Both Base and Subclass Methods: Ensure that both the base class methods and any overridden methods in subclasses are tested.
- Use Descriptive Test Names: This practice helps in understanding what each test covers.
Using unittest and pytest Frameworks for Testing
Two popular frameworks for testing in Python are unittest
and pytest
. Here’s how to use them effectively:
Using unittest
The built-in unittest
module is a solid choice for writing tests.
import unittest
class TestAnimal(unittest.TestCase):
def test_dog_sound(self):
dog = Dog()
self.assertEqual(dog.sound(), "Woof!")
def test_cat_sound(self):
cat = Cat()
self.assertEqual(cat.sound(), "Meow!")
if __name__ == '__main__':
unittest.main()
Using pytest
pytest
is a more flexible and user-friendly testing framework.
def test_dog_sound():
dog = Dog()
assert dog.sound() == "Woof!"
def test_cat_sound():
cat = Cat()
assert cat.sound() == "Meow!"
To run the tests, simply execute the pytest
command in your terminal.
Performance Considerations for Inheritance and Polymorphism
When working with Inheritance and Polymorphism in Python, it’s essential to consider performance. As applications grow in complexity, the choices made regarding class hierarchies and method usage can significantly impact the speed and efficiency of the program. In this section, we will explore how inheritance affects program speed and how to optimize polymorphism for better performance.
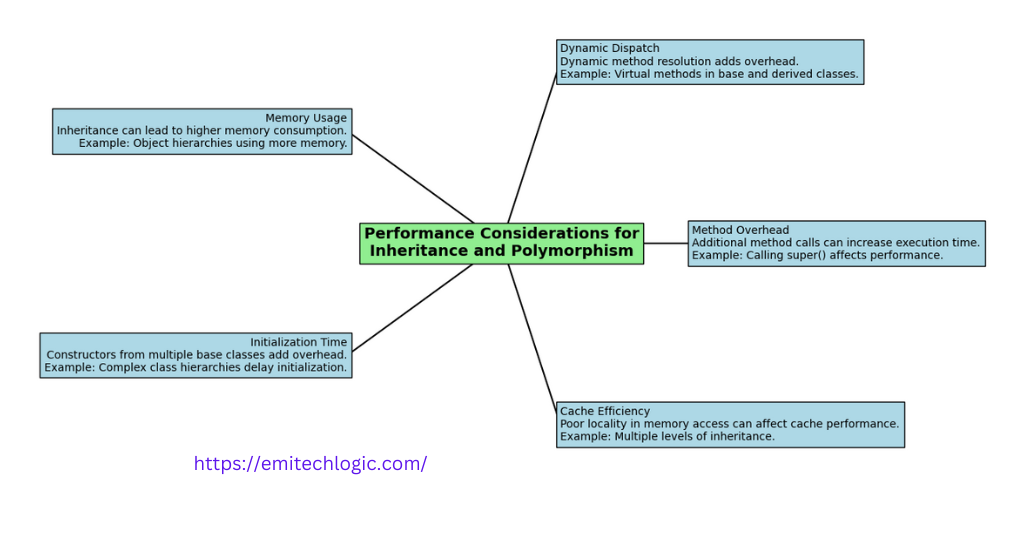
How Inheritance Affects Python Program Speed
Inheritance allows code reuse, which often simplifies development. However, it can also introduce overhead that might affect performance.
- Method Resolution Order (MRO): When a method is called on an object, Python must determine the order of classes to search for that method. This process can introduce delays, especially in complex inheritance trees. The deeper the inheritance hierarchy, the longer the resolution may take.Example:
class A:
def method(self):
return "Method in A"
class B(A):
pass
class C(B):
pass
obj = C()
print(obj.method()) # Method resolution may take longer with deeper hierarchies
2. Memory Overhead: Each subclass may introduce additional memory usage due to attributes and methods. If a large number of subclasses are created, memory consumption can increase, potentially slowing down the application.
3. Dynamic Typing: Python’s dynamic typing allows for flexible code, but it can lead to slower performance. Type checks and method lookups happen at runtime, which can introduce additional latency compared to statically typed languages.
Optimizing Polymorphism for Performance
Polymorphism allows objects of different classes to be treated as instances of the same class through a common interface. Although this feature enhances code flexibility, careful attention should be paid to optimize it for better performance.
- Use Duck Typing Wisely: In Python, duck typing allows you to use any object as long as it implements the required methods. While this feature promotes flexibility, ensuring that objects conform to expected interfaces can enhance performance.Example:
class Bird:
def fly(self):
return "Flying high!"
class Airplane:
def fly(self):
return "Flying in the sky!"
def make_it_fly(flyable):
print(flyable.fly())
# Both Bird and Airplane can be used here
make_it_fly(Bird()) # Outputs: Flying high!
make_it_fly(Airplane()) # Outputs: Flying in the sky!
2. Limit the Number of Method Overrides: While method overriding provides flexibility, excessive overriding can lead to performance issues. It can increase the complexity of the MRO and slow down method resolution. It is often beneficial to limit overriding to essential cases.
3. Utilize Built-in Functions and Libraries: Python’s built-in functions and standard libraries are optimized for performance. Using them instead of creating custom methods can significantly improve speed.
Example:
# Using built-in functions for better performance
data = [1, 2, 3, 4, 5]
print(sum(data)) # Fast and efficient
4. Avoid Unnecessary Abstraction: While abstraction helps in creating cleaner code, too many abstract layers can lead to performance degradation. It is vital to strike a balance between abstraction and direct implementation.
Conclusion: Embracing Inheritance and Polymorphism in Python
In this comprehensive guide on Inheritance and Polymorphism in Python, we’ve journeyed through the essential concepts, practical applications, and performance considerations of these powerful object-oriented programming features. By embracing inheritance, you can create flexible and maintainable code that promotes reusability. Meanwhile, polymorphism enables you to design interfaces that can work with various data types, enhancing the adaptability of your programs.
Understanding the balance between these two concepts is vital. While they provide significant advantages in code organization and functionality, careful implementation is crucial to avoid potential pitfalls such as performance issues and increased complexity. It is always advisable to analyze your specific use case to determine whether to employ inheritance, composition, or a combination of both.
As you continue your Python programming journey, keep experimenting with these concepts. Use the examples and best practices outlined in this guide to inform your approach. Remember, programming is not just about writing code; it’s about solving problems effectively and elegantly.
External Resources on Inheritance and Polymorphism
To further enhance your understanding and practical skills in Inheritance and Polymorphism in Python, here are some external resources:
Python Official Documentation: The official Python documentation offers detailed explanations of classes, inheritance, and polymorphism.
FAQ on Python Inheritance and Polymorphism
Polymorphism allows objects of different classes to be treated as instances of the same class through a common interface, enabling the same method name to be used across different classes. Inheritance, on the other hand, is a mechanism where a new class (child) derives properties and methods from an existing class (parent), promoting code reuse and hierarchical relationships.
Yes, Python supports multiple inheritance, allowing a class to inherit from more than one parent class. This enables the derived class to access methods and properties from all its parent classes.
The super()
function in Python is used to call methods from a parent class within a child class. It allows you to access inherited methods that have been overridden in the child class. This function is particularly useful for ensuring that the parent class’s initialization and other methods are properly executed.
Python does not support traditional method overloading like some other languages (e.g., Java or C++). However, it allows for method overriding and can achieve similar results using default arguments or variable-length arguments (e.g., *args
and **kwargs
) to accommodate different numbers and types of parameters.