Introduction
Let me tell you a quick story.
One rainy afternoon, you’re getting ready to go outside. You stop and think,
“Should I take my umbrella?”
Then you ask yourself,
“Is it raining and do I have my umbrella with me?”
Right there — without even knowing it — you used something called a logical operator.
In Python, we use logical operators like and
, or
, and not
to help the computer make decisions — just like you did. These are simple words that check if certain things are true or false.
Let’s say you want your program to check:
- “Is the user logged in and is their account active?”
- “Sunday or is it a holiday?”
- “Is the door not locked?”
Python needs clear answers to questions like these. That’s where logical operators come in — they connect conditions and tell your code what to do next.
You can think of them as little connectors that help your program say:
“Only do this if both things are true.”
“Do this if at least one thing is true.”
“Do this only if this one thing is not true.”
In this guide, I’m going to walk you through how these work — step by step, with real-life examples.
So pull up a chair, open your Python editor, and let’s learn how to make your code think smarter using and
, or
, and not
.
Ready? Let’s jump in.
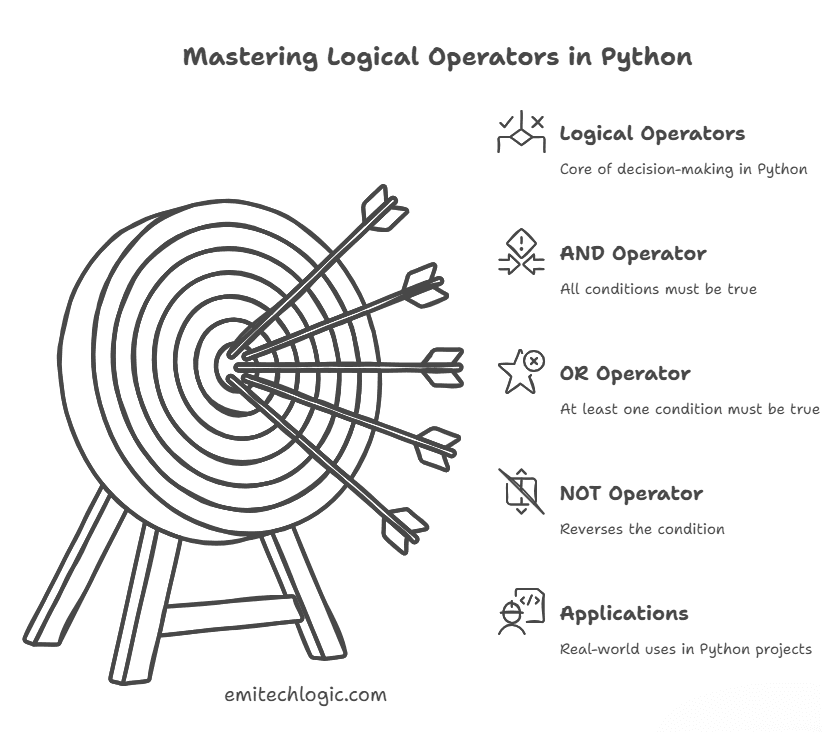
This guide breaks it down with real-life examples, truth tables, and tips to avoid common mistakes. Whether you’re just starting out or brushing up your basics, this is your go-to Python logic crash course!
What Are Logical Operators in Python?
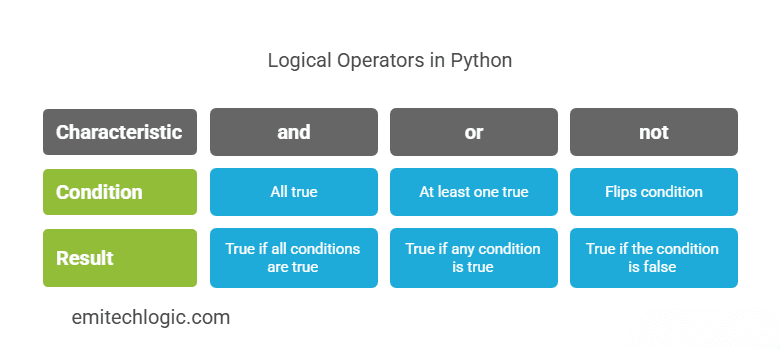
Now that you know logical operators help Python make decisions, let’s look at what they actually are.
In Python, logical operators are used to connect two or more conditions. These conditions are usually things that return either True or False — like checking if a number is greater than another, or if a variable matches a certain value.
Once Python checks those conditions, it decides what to do next based on whether the result is True or False.
Here are the three main logical operators in Python:
and
– This means all conditions must be true. If even one is false, the result is false.or
– Means only one condition needs to be true. If at least one is true, the result is true.not
– This simply flips the result. If something is true,not
makes it false. If it’s false,not
makes it true.
These operators are part of what we call Boolean logic — which is just a fancy name for working with true and false values. You’ll use Boolean logic all the time when writing programs that need to make choices, check inputs, or follow rules.
Next, let’s break down each operator with simple examples so you can see exactly how they work in Python.
Understanding the and
Operator in Python
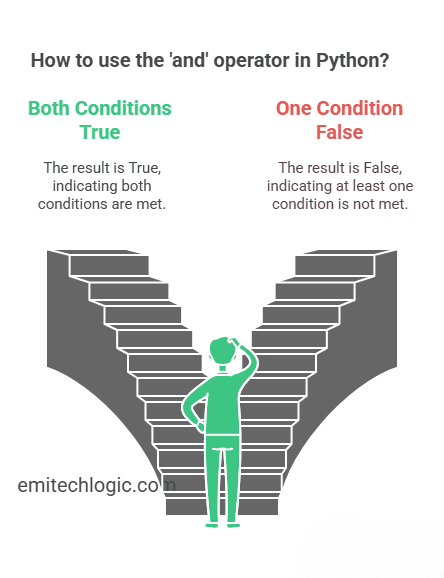
The and
operator checks if two or more conditions are true at the same time.
Let’s say you’re writing a small program to check if someone can enter a contest. The rules are simple:
- They must be at least 18 years old
- They must have a ticket
Both conditions need to be true. That’s where and
comes in.
Here’s how you’d write that in Python:
age = 20
has_ticket = True
if age >= 18 and has_ticket:
print("You can enter the contest!")
else:
print("Sorry, you can't enter.")
Let’s break this down:
age >= 18
is Truehas_ticket
is also True- Since both are true, the whole condition with
and
is True, and the message is printed.
But what if one of them isn’t true?
age = 16
has_ticket = True
if age >= 18 and has_ticket:
print("You can enter the contest!")
else:
print("Sorry, you can't enter.")
Now age >= 18
is False, so even though has_ticket
is still True, the overall result is False — and the person can’t enter.
That’s how and
works:
- True and True → True
- True and False → False
- False and True → False
- False and False → False
You’ll use and
whenever you want all conditions to be met before doing something.
Understanding the or
Operator in Python

The or
operator checks if at least one condition is true. It’s not as strict as and
, where every condition needs to be true. With or
, if one of the conditions is true, the whole condition becomes true.
Let’s go back to our contest example but with a twist. This time, you can either be 18 or older or you can have a ticket to enter. You don’t need both — just one.
Here’s how you’d write that in Python:
age = 20
has_ticket = False
if age >= 18 or has_ticket:
print("You can enter the contest!")
else:
print("Sorry, you can't enter.")
Let’s break it down:
age >= 18
is True (because 20 is greater than or equal to 18)has_ticket
is False (because the person doesn’t have a ticket)
With the or
operator, the result is True because one of the conditions is true (age >= 18
).
Now, what if neither condition is true?
age = 16
has_ticket = False
if age >= 18 or has_ticket:
print("You can enter the contest!")
else:
print("Sorry, you can't enter.")
Let’s check:
age >= 18
is False (because 16 is less than 18)has_ticket
is False
Since neither condition is true, the overall result is False, and the person can’t enter.
Here’s how the or
operator works in practice:
- True or True → True
- True or False → True
- False or True → True
- False or False → False
You can use the or
operator when you want only one of the conditions to be true for your program to do something. It’s very helpful for situations where there’s more than one way to meet a requirement.
Understanding the not
Operator in Python

The not
operator is a little different from and
and or
. Instead of combining two conditions, it flips the result of a single condition.
Think of it like a light switch. If the condition is True, not
turns it False. If the condition is False, not
turns it True. It’s like saying, “I want the opposite of what this condition is.”
Let’s take a closer look.
Example 1: Flipping a True Condition
Imagine you have a rule that says, “You can go to the park only if it’s NOT raining.”
Here’s how you’d write that:
is_raining = False
if not is_raining:
print("You can go to the park!")
else:
print("Stay indoors.")
Let’s break it down:
is_raining
is False (because it’s not raining).- The
not
operator flips it, sonot False
becomes True. - Since the result is True, the program prints “You can go to the park!”
Example 2: Flipping a False Condition
Now, what if it is raining?
is_raining = True
if not is_raining:
print("You can go to the park!")
else:
print("Stay indoors.")
Here:
is_raining
is True (because it’s raining).- The
not
operator flips it, sonot True
becomes False. - Since the result is False, the program prints “Stay indoors.”
How not
works:
- not True → False
- not False → True
You’ll use not
whenever you want to reverse the result of a condition. It’s perfect for situations like:
- “I want to do something unless a condition is true.”
- “Check if something is NOT true.”
Putting It All Together
Now you have all three logical operators:
and
checks if both conditions are true.or
checks if at least one condition is true.not
reverses the truth value of a condition.
These are powerful tools to help you control the flow of your programs and make them smarter!
Combining Logical Operators in Python
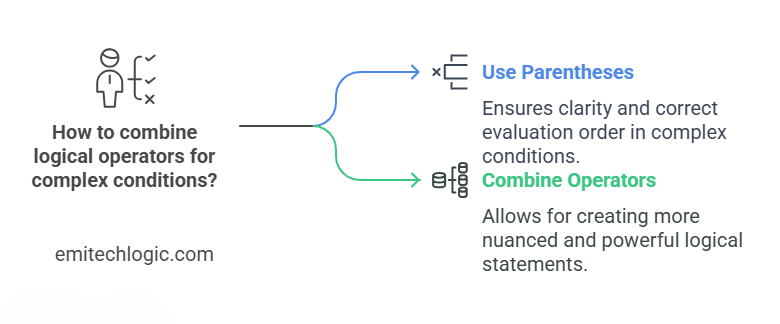
As you’ve already learned, and
, or
, and not
are powerful on their own. But what if you need to check multiple conditions that require a combination of these operators? Well, that’s where combining logical operators comes in.
You can mix and match these operators using parentheses to keep everything clear and organized.
Let’s look at an example.
Example: Checking User Permissions
Imagine you’re building a system that checks if a user can access certain admin features. The conditions for access are:
- The user must be logged in.
- The user must either be an admin or have a special permission.
You can combine and
, or
, and not
to check these conditions. Here’s how:
is_admin = True
is_logged_in = False
has_special_permission = True
# User must be logged in and either be an admin or have special permission
if is_logged_in and (is_admin or has_special_permission):
print("Access granted")
else:
print("Access denied")
Breaking it Down:
- is_logged_in is False (the user isn’t logged in).
- is_admin is True (the user is an admin).
- has_special_permission is True (the user has special permission).
We are using and
to make sure the user is logged in, and or
to check if the user is either an admin or has special permission.
- First, Python checks
(is_admin or has_special_permission)
. Sinceis_admin
is True, this part becomes True. - Then, Python checks the
is_logged_in
condition, which is False. - Finally, since both conditions are combined with
and
, and one is false, the overall result is False. So, the program prints “Access denied”.
Parentheses for Clarity:
Notice how we used parentheses around (is_admin or has_special_permission)
. This is very important because it makes sure that or
is evaluated first before and
. Without parentheses, Python would follow the default order of operations, which could lead to unexpected results.
Here’s how Python evaluates the condition:
- Check if
is_logged_in
is True (it’s not, so we go to the next step). - Evaluate the condition in the parentheses:
(is_admin or has_special_permission)
.- True or True → True
- Combine: False and True → False, so the program prints “Access denied”.
More Complex Example: Combining All Three
You can also combine all three operators to handle more complex situations. Here’s an example:
is_admin = True
is_logged_in = True
has_special_permission = False
account_suspended = False
# User must be logged in, not suspended, and either be an admin or have special permission
if is_logged_in and not account_suspended and (is_admin or has_special_permission):
print("Access granted")
else:
print("Access denied")
Breaking it Down:
- is_logged_in is True.
- account_suspended is False, so
not account_suspended
is True. - is_admin is True, so
(is_admin or has_special_permission)
becomes True.
Now we have:
- True and True and True → True, so the program prints “Access granted”.
Why Parentheses Matter:
- Parentheses ensure the correct order of operations, especially when combining different operators. In the above example, the parentheses around
(is_admin or has_special_permission)
tell Python to evaluate theor
condition first before combining it with theand
conditions.
Summary of Key Points:
and
requires all conditions to be true.or
requires at least one condition to be true.not
flips the condition.- Parentheses help keep things organized and control the order of evaluation.
By combining these operators and using parentheses, you can build more complex, powerful decision-making logic for your Python programs.
Truth Tables for Quick Reference
Here’s a quick reference for the three logical operators (and
, or
, not
), using True (T) and False (F) values for the conditions.
Truth Table for and
Operator
The and
operator returns True only if both conditions are True.
A (Condition 1) | B (Condition 2) | A and B |
---|---|---|
True | True | True |
True | False | False |
False | True | False |
False | False | False |
- True and True → True
- True and False → False
- False and True → False
- False and False → False
Truth Table for or
Operator
The or
operator returns True if at least one condition is True.
A (Condition 1) | B (Condition 2) | A or B |
---|---|---|
True | True | True |
True | False | True |
False | True | True |
False | False | False |
- True or True → True
- True or False → True
- False or True → True
- False or False → False
Truth Table for not
Operator
The not
operator simply flips the value of a condition: True becomes False and False becomes True.
A (Condition) | not A |
---|---|
True | False |
False | True |
- not True → False
- not False → True
Combining Operators (Example)
Here’s a combined truth table with and
and or
to give you a sense of how multiple operators work together:
A (Condition 1) | B (Condition 2) | A and B | A or B | (A and B) or not B |
---|---|---|---|---|
True | True | True | True | True |
True | False | False | True | True |
False | True | False | True | False |
False | False | False | False | True |
In the last column, (A and B) or not B
, the and
and or
operators are combined, and we also use not
.
Quick Reference Recap:
and
: Both conditions need to be True for the result to be True.or
: At least one condition needs to be True for the result to be True.not
: Flips the condition: True becomes False and False becomes True.
These tables should help you quickly reference how each logical operator works and how they combine together in Python!
Must Read
- How to Write a Python Program to Find LCM
- Complete Guide to Find GCD in Python: 7 Easy Methods for Beginners
- How to Set Up CI/CD for Your Python Projects Using Jenkins
- The Ultimate Guide to the range() Function in Python
- How to compute factorial in Python
Common Mistakes to Avoid with Python Logical Operators
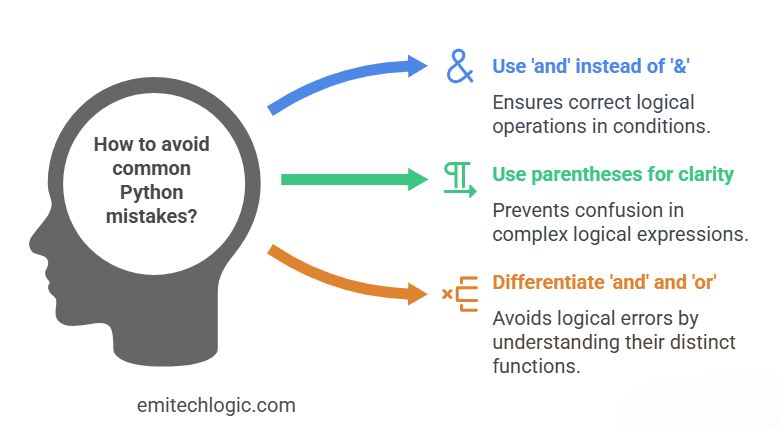
Even though Python logical operators are simple, there are a few common mistakes that can cause confusion and errors in your code. Let’s go over some of these mistakes so you can avoid them and write more reliable code.
1. Using &
Instead of and
A common mistake is using the &
operator instead of the and
logical operator. While both may seem similar, they are different.
&
is a bitwise operator, used for performing bit-by-bit operations (like on binary numbers).and
is a logical operator, used to combine two conditions and check if both are True.
Example of the Mistake:
# Wrong:
if condition1 & condition2:
print("Both conditions are True")
In this case, &
checks the individual bits of condition1
and condition2
, which might not give you the result you’re expecting.
Correct Usage:
# Right:
if condition1 and condition2:
print("Both conditions are True")
Here, and
checks if both conditions are True in a logical sense.
2. Forgetting Parentheses in Complex Logic
When combining multiple logical operators, forgetting parentheses can cause confusion and lead to unintended results.
Example of the Mistake:
# Can be confusing:
if a or b and not c:
print("Conditions met")
Python evaluates logical operators in a specific order, and and
has a higher precedence than or
. This means the expression above will actually be interpreted as:
if a or (b and not c):
Correct Usage:
To avoid confusion and ensure you’re checking the conditions in the right order, always use parentheses to make the logic clear.
# Better:
if (a or b) and (not c):
print("Conditions met")
By using parentheses, you clarify the logic and ensure the expression is evaluated as you intend.
3. Thinking and
is the Same as or
Another common mistake is assuming and
and or
behave the same way. They are used in different scenarios:
and
requires both conditions to be True for the result to be True.or
requires at least one condition to be True for the result to be True.
It’s easy to mix them up, so always double-check which one you need for your logic.
Example:
# Mistake:
if is_raining and is_sunny:
print("Strange weather!")
You might expect this to work, but for this to be True, both is_raining
and is_sunny
must be True, which doesn’t usually happen at the same time.
Correct Logic:
# Better:
if is_raining or is_sunny:
print("Strange weather!")
This uses or
because you want the message to print if either condition is true, not necessarily both.
Real-World Uses of Logical Operators in Python
Logical operators in Python are not just useful in simple examples; they play a crucial role in real-world programming scenarios. From user authentication to game development, these operators help you make decisions and control the flow of your program based on multiple conditions. Let’s explore some real-world examples where and
, or
, and not
are commonly used.
1. User Authentication and Access Control
In many systems, such as websites or applications, logical operators are used to check if a user meets certain conditions before granting access to a specific resource.
For example, in a user authentication system, you might need to check:
- If the user is logged in.
- The user has the correct role (like admin).
- If the user’s account is not suspended.
You could combine these checks using logical operators like this:
is_logged_in = True
is_admin = False
account_suspended = False
if is_logged_in and not account_suspended and is_admin:
print("Welcome, Admin! You have full access.")
else:
print("Access denied")
In this case:
- User must be logged in.
- The account should not be suspended.
- The user must be an admin.
This example uses and
and not
to ensure all conditions are met before granting access.
2. Online Shopping Cart: Applying Discounts
When you’re building an online shopping cart system, you might want to apply certain discounts based on conditions like:
- The user is logged in.
- The total purchase is above a certain amount.
- User has a special discount code.
Here’s how you can use logical operators to decide when a discount should be applied:
is_logged_in = True
total_purchase = 120 # in dollars
has_discount_code = True
if is_logged_in and (total_purchase > 100 or has_discount_code):
print("You get a discount!")
else:
print("No discount available.")
and
ensures that the user must be logged in.or
allows the user to either have a purchase above a certain amount or a discount code to qualify.
This combination of logical operators helps to determine if the discount should be applied.
3. Game Development: Player Status
In game development, you often need to check various conditions to determine a player’s status, such as whether they can perform an action, if they have enough resources, or if they’ve met certain criteria.
For example, a game might have a condition where a player can attack if:
- They have enough energy.
- Are they alive.
- They are not in a disabled state.
Here’s how logical operators can handle this:
energy = 50
is_alive = True
is_disabled = False
if energy >= 20 and is_alive and not is_disabled:
print("You can attack!")
else:
print("You cannot attack.")
and
checks that energy, life, and status all need to be in favorable conditions for the player to attack.not
ensures the player is not in a disabled state.
4. Filtering Data in Web Applications
In web applications, logical operators are frequently used to filter data based on multiple conditions. For example, you might want to display items from an online store based on:
- The category.
- The price range.
- Whether the item is available.
Let’s say a user wants to filter products that are within a certain price range and belong to a specific category, but only if the item is available. Here’s how logical operators can help:
category = "Electronics"
price = 150 # in dollars
is_available = True
if (category == "Electronics" or category == "Home Appliances") and price <= 200 and is_available:
print("Item meets your filter criteria!")
else:
print("Item does not meet your filter criteria.")
This example combines:
or
to check if the product is in one of two categories.and
to ensure the price is within the acceptable range.is_available
is checked to ensure the product is in stock.
5. Scheduling and Time-Based Logic
In a scheduling system, you might need to decide if a user can book a meeting based on:
- The availability of the user.
- If the user is working hours.
- If there are conflicting appointments.
Here’s an example using logical operators to check these conditions:
is_available = True
is_working_hours = True
has_conflicting_appointments = False
if is_available and is_working_hours and not has_conflicting_appointments:
print("You can schedule the meeting!")
else:
print("Unable to schedule the meeting.")
and
ensures the user is available and within working hours.not
ensures there are no conflicting appointments.
6. Weather Forecast System
In a weather forecasting system, you might want to display different messages or take actions depending on multiple weather conditions. For example, checking if it’s:
- Raining.
- The temperature is below a certain threshold.
- Wind speed is above a safe limit.
Let’s say you want to warn the user if it’s both raining and the wind speed is too high:
is_raining = True
temperature = 30 # in Celsius
wind_speed = 50 # in km/h
if is_raining and wind_speed > 40:
print("Warning: Heavy rain and high winds. Stay safe!")
else:
print("Weather is safe for outdoor activities.")
Conclusion
In this blog post, we’ve explored the power and simplicity of logical operators in Python. These operators—and
, or
, and not
—are fundamental tools that help you make decisions in your code based on multiple conditions. Whether you’re managing user access, filtering data, or making decisions in a game, logical operators are there to help you build clear and efficient control flow in your programs.
We’ve also seen how to combine these operators to handle more complex scenarios and avoid common mistakes like forgetting parentheses or mixing up and
and or
. With the examples and real-world use cases we discussed, you can now see how logical operators are applied in areas such as user authentication, shopping carts, game development, and much more.
By mastering these operators, you’ll be able to write cleaner, more powerful Python code that can tackle complex problems with ease.
Keep practicing with different combinations of logical operators, and soon, they’ll become second nature in your coding toolkit.
What I Wish I Knew as a Beginner
When I first started learning Python, logical operators confused me more than I expected. I thought and
and or
worked just like regular English. So I’d write:
if user == "admin" or "moderator":
…and expect it to return True
for either role. But Python doesn’t work that way. It actually always returned True
, even if the user wasn’t “admin” or “moderator”!
Why? Because "moderator"
is a non-empty string, which is always True
in Python. The right way was:
if user == "admin" or user == "moderator":
That small mistake made debugging painful. If someone had explained it like this earlier, I would’ve saved hours!
So if you’re just starting out, slow down and test each condition separately. Print values, break things into smaller steps, and use parentheses. Python will feel way less intimidating.
Quick Practice: Test Yourself
Let’s see how well you’ve understood logical operators. Try these out before looking at the answers:
Question 1:
x = True
y = False
print(x and not y)
What’s the output?
Answer:not y
is not False
→ True
.x and True
→ True and True
→ True
Question 2:
a = False
b = True
c = False
print(a or b and not c)
Tip: Remember the order of operations—not
comes first, then and
, then or
.
Answer:not c
→ True
b and True
→ True
a or True
→ False or True
→ True
Question 3:
Fill in the blank so the condition becomes True
:
is_logged_in = False
has_permission = _
print(is_logged_in or has_permission)
Answer:
To make the expression False or ___
return True
, has_permission
must be True
.
FAQs
1. What is the difference between
and
andor
in Python?and
returnsTrue
only if both conditions are True.or
returnsTrue
if at least one condition is True.2. Can I use
&
instead ofand
in Python?No,
&
is a bitwise operator, not a logical one. Useand
for conditions.3. How do I avoid confusion with complex conditions?
Use parentheses to group your logic clearly. Example:
if (a or b) and (not c):4. What is the purpose of
not
in Python?not
flips the result.not True
becomesFalse
, andnot False
becomesTrue
.
External Resources
Here are some trusted resources to help you go deeper into Python logical operators and Boolean logic:
Python Official Documentation – Boolean Operations
A great reference for how Python handles and
, or
, and not
.
Beginner-friendly guide with examples for using if
, and
, or
, and not
.
Leave a Reply