Introduction
Welcome to the second part of our in-depth guide on Python I/O operations! In this section, we shift our focus from basic input and output operations to more advanced topics, including file handling, error management, and best practices for writing efficient and secure code.
File handling is a crucial aspect of programming that allows you to manage data stored in files. We’ll explore how to open, read, and write files in Python, handling different file formats like CSV, JSON, and XML. You’ll learn practical techniques for working with these files, whether you’re building a simple log file system or processing data for analysis.
Next, we’ll explore the error handling strategies to help you deal with common issues that arise during input and output operations. You’ll discover how to use try-except
blocks to manage errors gracefully, ensuring your programs run smoothly even when unexpected problems occur.
Finally, we’ll cover best practices for writing clean and efficient I/O code. This includes optimizing your file handling, ensuring user-friendly input and output, and addressing security considerations to protect your applications from common threats.
By the end of this section, you’ll have a solid understanding of advanced file operations, effective error handling, and strategies for writing robust and secure code. Ready to take your Python skills to the next level? Let’s get started!
File Handling in Python: Integrating Input and Output
Basics of File Operations in Python
Working with files is a fundamental part of many Python applications. Whether you’re saving user data, loading configuration files, or processing large datasets, understanding how to open, read, and write files in Python is essential. This section will guide you through the basics of file operations, explaining how to handle file paths and modes, and covering the common tasks of reading, writing, and appending files.
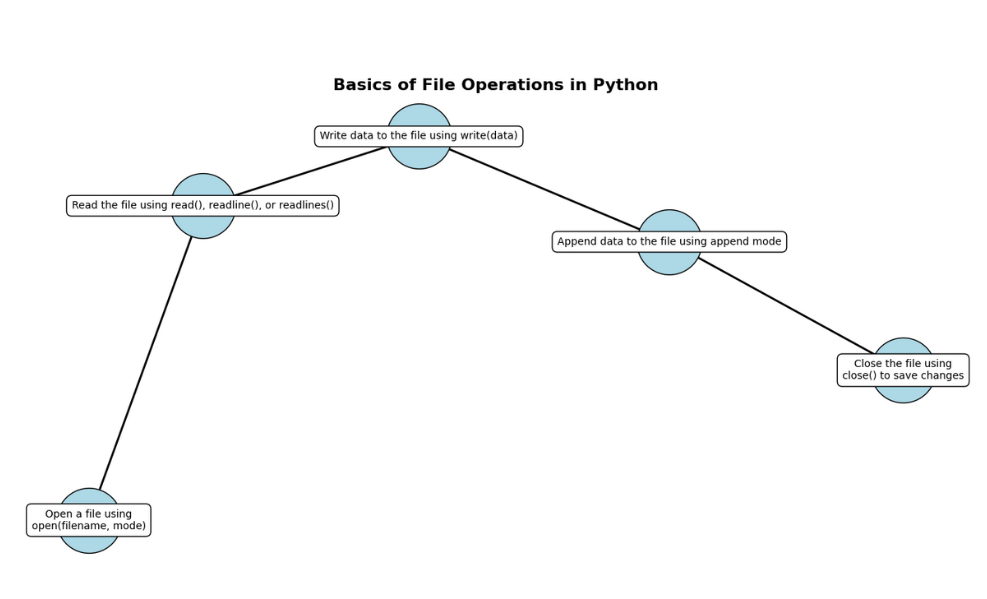
How to Open, Read, and Write Files in Python
To begin working with files in Python, the first step is to open the file. The open()
function is the most commonly used method for this. When you open a file, Python gives you access to its contents, allowing you to read from or write to the file.
Here’s a simple example:
file = open('example.txt', 'r') # Open the file in read mode
content = file.read() # Read the entire content of the file
print(content)
file.close() # Close the file
In this example, the file example.txt
is opened in read mode ('r'
). The read()
function then reads the entire content of the file and stores it in the content
variable. Finally, the file is closed using close()
to free up system resources.
If you want to write to a file instead, you can use the write mode ('w'
):
file = open('example.txt', 'w') # Open the file in write mode
file.write("Hello, world!") # Write a string to the file
file.close() # Close the file
When the file is opened in write mode, the existing content of the file is erased, and new data is written. If the file does not exist, Python will create it for you.
Writing to a file is just as easy as reading from one, making these basic file operations in Python easy to learn and apply in various projects.
Handling File Paths and Modes in Python
When working with files, it’s important to understand file paths and modes. Handling file paths and modes in Python involves specifying the location of the file and how you want to interact with it.
A file path can be absolute or relative. An absolute path specifies the full directory path to the file, starting from the root directory. A relative path, on the other hand, specifies the location of the file relative to the current working directory.
For example:
# Absolute path
file = open('/Users/username/Documents/example.txt', 'r')
# Relative path
file = open('example.txt', 'r')
In the first example, an absolute path is used, pointing to a specific file on the system. In the second example, a relative path is used, assuming that the file is in the current working directory.
Python also supports various file modes:
'r'
– Read mode (default)'w'
– Write mode (overwrites existing content)'a'
– Append mode (adds new data to the end of the file)'b'
– Binary mode (used with'r'
,'w'
, or'a'
for binary files)
By combining these modes, you can perform different types of operations on a file. For example, 'rb'
would open a file in binary read mode, which is useful for working with non-text files like images or executables.
Common File Operations: Reading, Writing, Appending
In Python, three of the most common file operations are reading, writing, and appending. Each of these operations is critical in managing data within your programs.
Reading involves accessing the contents of a file. You can read the entire file at once using read()
, or you can read it line by line using readline()
or readlines()
:
file = open('example.txt', 'r')
for line in file:
print(line.strip()) # Print each line without extra newlines
file.close()
This example demonstrates reading a file line by line, which can be more efficient for larger files.
Writing is used when you want to create or overwrite a file. As shown earlier, using the write mode ('w'
) allows you to write new content to a file, while the append mode ('a'
) lets you add to an existing file without deleting its current contents:
file = open('example.txt', 'a')
file.write("\nAdding another line.")
file.close()
In this case, the new line is added to the end of the file without affecting the existing content.
Appending is particularly useful when you need to log data or continuously update a file with new information. It’s a safe way to ensure that the existing data remains intact while new data is added.
Working with Different File Formats
In Python, working with various file formats is a common task that can enhance the functionality of your applications. Whether you need to handle data in CSV, JSON, or XML formats, knowing how to read and write CSV files, handle JSON files, and process XML files can greatly improve your ability to manage and manipulate data. This guide will explain these processes in detail, helping you become proficient in handling different file formats in Python.
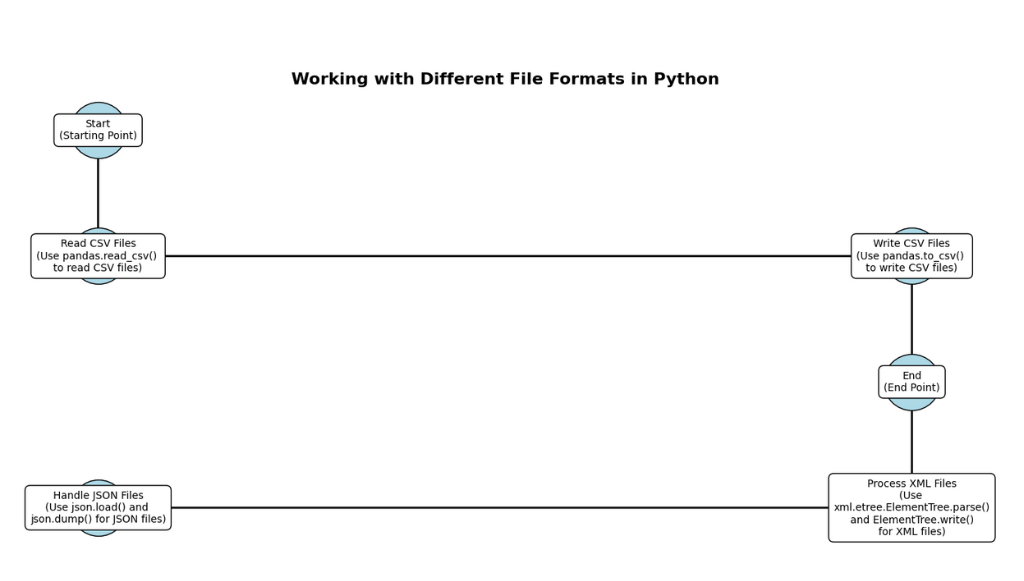
Explanation
- Start: Represents the starting point of the process.
- Read CSV Files: Step for reading CSV files using
pandas.read_csv()
. - Write CSV Files: Step for writing CSV files using
pandas.to_csv()
. - Handle JSON Files: Step for handling JSON files using
json.load()
andjson.dump()
. - Process XML Files: Step for processing XML files using
xml.etree.ElementTree
. - End: Represents the end point of the process.
Reading and Writing CSV Files in Python
CSV (Comma-Separated Values) files are a popular format for storing tabular data. They are simple to understand and use, making them a common choice for data exchange between programs. To work with CSV files in Python, you can use the csv
module, which provides functions for reading from and writing to CSV files.
Here’s how you can read and write CSV files in Python:
Reading CSV Files
To read data from a CSV file, you use the csv.reader
object. Here’s an example:
import csv
with open('data.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
In this example, the csv.reader
reads each row of the data.csv
file and prints it. Each row is returned as a list of strings, with each element representing a cell in the CSV file.
Writing CSV Files
To write data to a CSV file, you use the csv.writer
object:
import csv
data = [
['Name', 'Age', 'City'],
['Alice', '30', 'New York'],
['Bob', '25', 'Los Angeles']
]
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
In this example, the csv.writer
writes a list of lists to the data.csv
file. Each inner list represents a row in the CSV file. The newline=''
parameter is used to prevent extra blank lines in the output.
Handling JSON Files in Python
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy to read and write. It’s often used for configuration files and data exchange between servers and web applications. Python provides the json
module to work with JSON data.
Here’s how you can handle JSON files in Python:
Reading JSON Files
To read JSON data from a file, you use the json.load()
method:
import json
with open('data.json', 'r') as file:
data = json.load(file)
print(data)
In this example, json.load()
reads the JSON data from data.json
and parses it into a Python dictionary. You can then access and manipulate the data as needed.
Writing JSON Files
To write JSON data to a file, you use the json.dump()
method:
import json
data = {
'name': 'Alice',
'age': 30,
'city': 'New York'
}
with open('data.json', 'w') as file:
json.dump(data, file, indent=4)
In this example, json.dump()
writes the dictionary to data.json
. The indent=4
parameter is used to format the JSON data with indentation, making it easier to read.
Processing XML Files in Python
XML (eXtensible Markup Language) is a markup language that defines rules for encoding documents in a format that is both human-readable and machine-readable. XML is commonly used for data storage and transfer. To work with XML files in Python, you can use the xml.etree.ElementTree
module, which provides functions to parse and create XML data.
Here’s how you can process XML files in Python:
Reading XML Files
To read and parse XML data, use the ElementTree
class:
import xml.etree.ElementTree as ET
tree = ET.parse('data.xml')
root = tree.getroot()
for child in root:
print(child.tag, child.attrib)
In this example, ET.parse()
reads the data.xml
file and parses it into an ElementTree
object. The getroot()
method retrieves the root element of the XML document. You can then iterate over the child elements and print their tags and attributes.
Writing XML Files
To create or modify XML data, you can build an XML tree and write it to a file:
import xml.etree.ElementTree as ET
root = ET.Element("root")
child1 = ET.SubElement(root, "child1")
child1.text = "Data for child 1"
child2 = ET.SubElement(root, "child2")
child2.text = "Data for child 2"
tree = ET.ElementTree(root)
tree.write('output.xml')
In this example, an XML tree is created with a root element and two child elements. The ET.ElementTree()
method is then used to write the tree to output.xml
.
Practical File Handling Examples
Understanding how to handle files effectively can greatly enhance your programming skills. Whether you are creating a simple log file system, saving and loading user settings in JSON files, or processing CSV data for analysis, knowing these practical file handling techniques will help you manage data efficiently. This section will walk you through some real-world examples to illustrate these concepts.
Building a Simple Log File System in Python
A log file system is essential for keeping track of events or errors in applications. By recording activities in a log file, you can review and troubleshoot issues later. Let’s explore how to build a basic log file system using Python.
Creating a Log File
First, you need to set up a log file. This file will record messages, such as errors or status updates, as they occur in your application. Here’s an example of how to create and write to a log file:
import datetime
def log_message(message):
with open('application.log', 'a') as log_file:
timestamp = datetime.datetime.now().strftime('%Y-%m-%d %H:%M:%S')
log_file.write(f'{timestamp} - {message}\n')
# Example usage
log_message('Application started')
log_message('User logged in')
In this example, the log_message
function appends messages to application.log
. Each message is prefixed with a timestamp, which helps in understanding when each event occurred. The 'a'
mode ensures that new messages are added to the end of the file, preserving existing logs.
Reading the Log File
To review the log entries, you can read the file and print its contents:
with open('application.log', 'r') as log_file:
content = log_file.read()
print(content)
This code reads the entire log file and prints its content, allowing you to view the logged messages.
Saving and Loading User Settings in a JSON File
JSON (JavaScript Object Notation) is a lightweight format perfect for storing user settings. It is easy to read and write, making it ideal for saving configurations or preferences.
Saving User Settings
To save user settings, you first need to define the settings you want to store. Here’s how to write these settings to a JSON file:
import json
user_settings = {
'theme': 'dark',
'font_size': 12,
'notifications': True
}
with open('settings.json', 'w') as file:
json.dump(user_settings, file, indent=4)
In this example, the json.dump
function writes the user_settings
dictionary to settings.json
. The indent=4
parameter formats the JSON data with indentation, making it easier to read.
Loading User Settings
To load these settings back into your program, you use the json.load
function:
import json
with open('settings.json', 'r') as file:
settings = json.load(file)
print(settings)
This code reads the settings from settings.json
and prints them. You can now use these settings in your application.
Processing CSV Data for Analysis
CSV (Comma-Separated Values) files are often used for storing and analyzing tabular data. Python’s csv
module makes it easy to handle CSV files, allowing you to read and process data efficiently.
Reading CSV Data
To read CSV data for analysis, you can use the following approach:
import csv
data = []
with open('data.csv', 'r') as file:
reader = csv.DictReader(file)
for row in reader:
data.append(row)
# Example: Print each row
for row in data:
print(row)
In this example, csv.DictReader
reads each row from data.csv
as a dictionary, where the keys are column headers. The data is collected in a list and can be processed as needed.
Analyzing CSV Data
Once the data is read, you can perform various analyses. For instance, calculating the average of a numeric column:
import csv
total = 0
count = 0
with open('data.csv', 'r') as file:
reader = csv.DictReader(file)
for row in reader:
total += float(row['value']) # Assume 'value' is a numeric column
count += 1
average = total / count
print(f'Average value: {average}')
Here, the code calculates the average of a numeric column named 'value'
. The total
and count
variables accumulate the sum and the number of rows, respectively, and the average is calculated and printed.
Must Read
- How to Return Multiple Values from a Function in Python
- Parameter Passing Techniques in Python: A Complete Guide
- A Complete Guide to Python Function Arguments
- How to Create and Use Functions in Python
- Find All Divisors of a Number in Python
Error Handling in Python Input and Output Operations
Common Errors in Python I/O Operations
Handling input and output (I/O) operations in Python can sometimes lead to errors. Understanding these common mistakes and knowing how to resolve them is essential for writing reliable code. This guide will explain how to handle input errors, manage file I/O errors, and provide examples of typical errors along with their solutions.
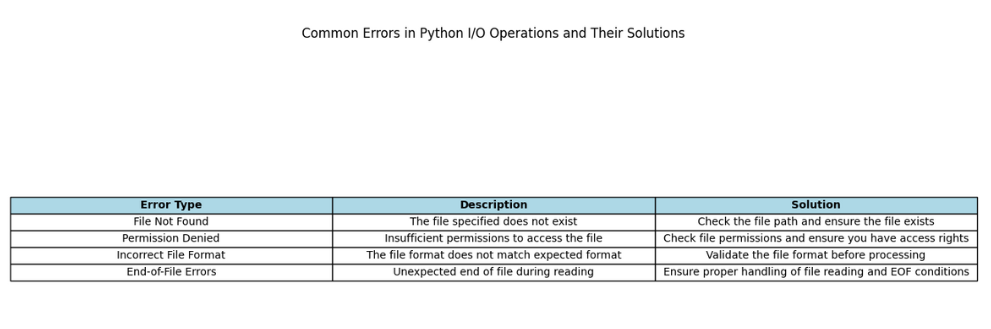
How to Handle Input Errors in Python
When dealing with user input in Python, errors can occur if the input doesn’t match the expected format. These errors often arise when converting input data or when the input is invalid. Here’s how you can handle these errors effectively:
Input Conversion Errors
When converting user input to a specific type, such as an integer or float, errors can occur if the input is not properly formatted. For instance, if a user inputs text when a number is expected, an error will be raised.
Here’s an example of handling such errors:
while True:
try:
age = int(input("Please enter your age: "))
break
except ValueError:
print("Invalid input. Please enter a numeric value.")
In this example, the try
block attempts to convert the user’s input into an integer. If the input cannot be converted, a ValueError
is caught by the except
block, which then prompts the user to enter a numeric value again.
Handling Unexpected Input
Sometimes, the input might not match the expected format, leading to unexpected behavior. To handle this, you can use validation techniques:
def get_positive_integer(prompt):
while True:
try:
number = int(input(prompt))
if number <= 0:
raise ValueError("The number must be positive.")
return number
except ValueError as e:
print(e)
# Example usage
age = get_positive_integer("Enter a positive number: ")
print(f"Your age is {age}.")
In this example, the function get_positive_integer
ensures that the user inputs a positive integer. If the input is not valid, an appropriate error message is displayed.
Managing File I/O Errors in Python
File I/O operations can also result in errors, often due to issues with file paths, permissions, or file modes. Here’s how to handle these common file-related errors:
File Not Found Errors
If you try to open a file that doesn’t exist, Python will raise a FileNotFoundError
. To manage this error, you can check if the file exists before attempting to open it:
import os
filename = 'data.txt'
if os.path.exists(filename):
with open(filename, 'r') as file:
content = file.read()
else:
print(f"The file {filename} does not exist.")
In this example, os.path.exists()
checks whether the file exists before attempting to open it. If the file doesn’t exist, a message is printed instead of raising an error.
Handling File Access Errors
Sometimes, you may encounter errors related to file permissions or other access issues. Use a try-except
block to handle these errors:
try:
with open('data.txt', 'w') as file:
file.write("Hello, world!")
except PermissionError:
print("You do not have permission to write to this file.")
In this example, if you don’t have permission to write to data.txt
, a PermissionError
is caught and a message is displayed.
Examples of Typical I/O Errors and Their Solutions
1. File Not Found Error
A common issue is trying to access a file that doesn’t exist:
try:
with open('nonexistent_file.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("The file was not found. Please check the file path.")
2. ValueError During Input Conversion
This error occurs when input cannot be converted to the expected type:
try:
number = int(input("Enter a number: "))
except ValueError:
print("Invalid input. Please enter a valid number.")
3. PermissionError When Writing to a File
This happens if you lack the necessary permissions to modify a file:
try:
with open('readonly_file.txt', 'w') as file:
file.write("Attempting to write.")
except PermissionError:
print("Permission denied. Cannot write to the file.")
Practical Error Handling Techniques
In programming, dealing with errors is essential to ensure that your code runs smoothly and handles unexpected situations gracefully. This section will cover using try-except blocks in Python I/O, with practical examples that show how to handle invalid user input and ensure that file operations are safe and reliable. Understanding these techniques will help you write code that is more resilient and user-friendly.
Using Try-Except Blocks in Python I/O
Try-except blocks are a fundamental tool in Python for handling errors. They allow you to manage exceptions, or errors, that occur during program execution. Here’s how these blocks work:
- Try Block: This is where you place the code that might cause an error. The
try
block attempts to execute the code inside it. - Except Block: If an error occurs in the
try
block, Python jumps to theexcept
block. Here, you handle the error or provide an alternative response.
Basic Syntax:
try:
# Code that might cause an error
except ErrorType:
# Code that runs if an error occurs
Example: Gracefully Handling Invalid User Input
One common scenario is handling user input that might not match the expected format. For instance, if you expect a number but the user inputs text, it’s important to catch this error and prompt the user to try again.
while True:
try:
number = int(input("Please enter a number: "))
break # Exit loop if conversion is successful
except ValueError:
print("That was not a valid number. Please try again.")
In this example, the try
block attempts to convert the user’s input into an integer. If the input is not a valid number, a ValueError
is raised, and the except
block displays an error message. The loop continues until valid input is received.
Example: Ensuring File Operations Are Safe and Reliable
File operations can also lead to errors, such as trying to read from a file that doesn’t exist or writing to a file without proper permissions. Using try-except blocks can help manage these situations effectively.
filename = 'data.txt'
try:
with open(filename, 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print(f"The file {filename} does not exist.")
except IOError:
print(f"An error occurred while trying to read {filename}.")
In this example, the try
block attempts to open and read from data.txt
. If the file is not found, a FileNotFoundError
is caught, and a message is printed. If there’s an issue with the file I/O operation (e.g., permission issues), an IOError
is handled. This approach ensures that your program can handle file-related errors gracefully.
Best Practices for Input and Output Operations in Python
Writing Clean and Efficient I/O Code
When working with input and output (I/O) operations in Python, writing clean and efficient code is essential for both performance and user experience. This guide will explore optimizing Python code for file handling and ensuring user-friendly input and output in Python applications. Understanding these practices will help you write code that is not only efficient but also easy to use and maintain.
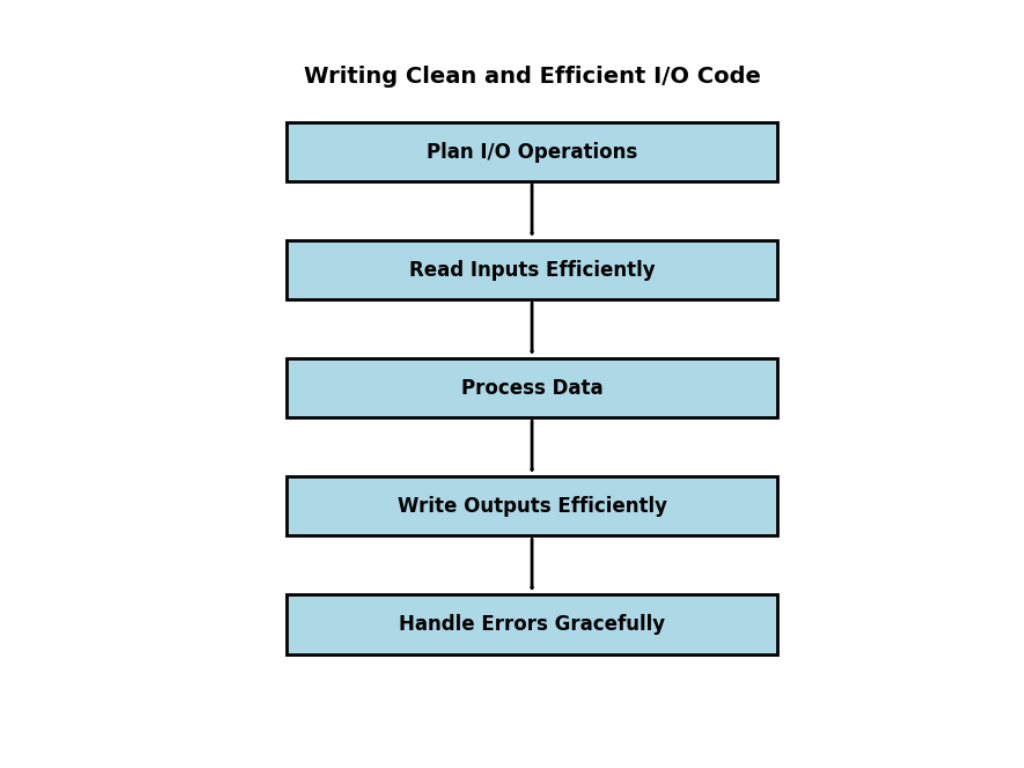
Optimizing Python Code for File Handling
Efficient file handling is important for improving the performance of your applications. Here are some techniques to optimize your Python code for file operations:
1. Using Context Managers
Context managers, implemented using the with
statement, are a great way to manage file operations efficiently. They ensure that files are properly opened and closed, even if errors occur.
with open('data.txt', 'r') as file:
content = file.read()
In this example, the file data.txt
is opened in read mode, and its content is read. The file is automatically closed when the with
block is exited, making the code cleaner and reducing the risk of leaving files open unintentionally.
2. Reading and Writing in Batches
When dealing with large files, it’s more efficient to read or write data in chunks rather than all at once. This approach can help manage memory usage and improve performance.
# Reading a large file in chunks
with open('large_file.txt', 'r') as file:
while chunk := file.read(1024): # Read 1024 bytes at a time
process(chunk)
# Writing data in chunks
with open('output.txt', 'w') as file:
for chunk in data_chunks:
file.write(chunk)
Reading or writing data in chunks prevents your program from consuming too much memory at once and can be especially useful for handling very large files.
3. Efficient File Access
Minimize the number of times you open and close files. Frequent opening and closing can be costly in terms of performance. Instead, try to handle all your file operations in a single session if possible.
# Perform multiple operations in a single session
with open('data.txt', 'r+') as file:
content = file.read()
file.seek(0) # Move the cursor to the beginning of the file
file.write("Updated content")
This approach reduces the overhead associated with file operations and can improve the overall performance of your code.
Ensuring User-Friendly Input and Output in Python Applications
Creating a positive user experience involves making your input and output operations as intuitive and clear as possible. Here’s how you can ensure your Python applications are user-friendly:
1. Validating User Input
Always validate user input to ensure it meets the expected format. This can prevent errors and guide users to provide the correct information.
def get_valid_age():
while True:
try:
age = int(input("Enter your age: "))
if age < 0:
raise ValueError("Age cannot be negative.")
return age
except ValueError as e:
print(f"Invalid input: {e}. Please enter a valid age.")
In this example, the function prompts the user to enter their age and checks that the input is a non-negative integer. If the input is invalid, an error message is displayed, guiding the user to provide correct input.
2. Providing Clear Output Messages
Make sure your output messages are clear and informative. This helps users understand the results of their actions and makes your application more intuitive.
def display_result(result):
print(f"The calculated result is: {result:.2f}")
By formatting the output clearly and providing relevant information, users will find it easier to interpret the results.
3. Handling Errors Gracefully
When errors occur, provide helpful error messages that guide users on how to resolve the issue. Avoid displaying technical jargon or error codes that might confuse them.
try:
# Code that may cause an error
except Exception as e:
print(f"An error occurred: {e}. Please try again.")
In this approach, any error encountered is caught, and a user-friendly message is displayed. This helps maintain a smooth user experience even when problems arise.
Security Considerations in I/O Operations
When working with input and output (I/O) operations in Python, it’s crucial to address security concerns to protect your applications and data. This section focuses on protecting against injection attacks in Python input and safe file handling practices in Python. By understanding and implementing these security measures, you can make your code more secure and reliable.
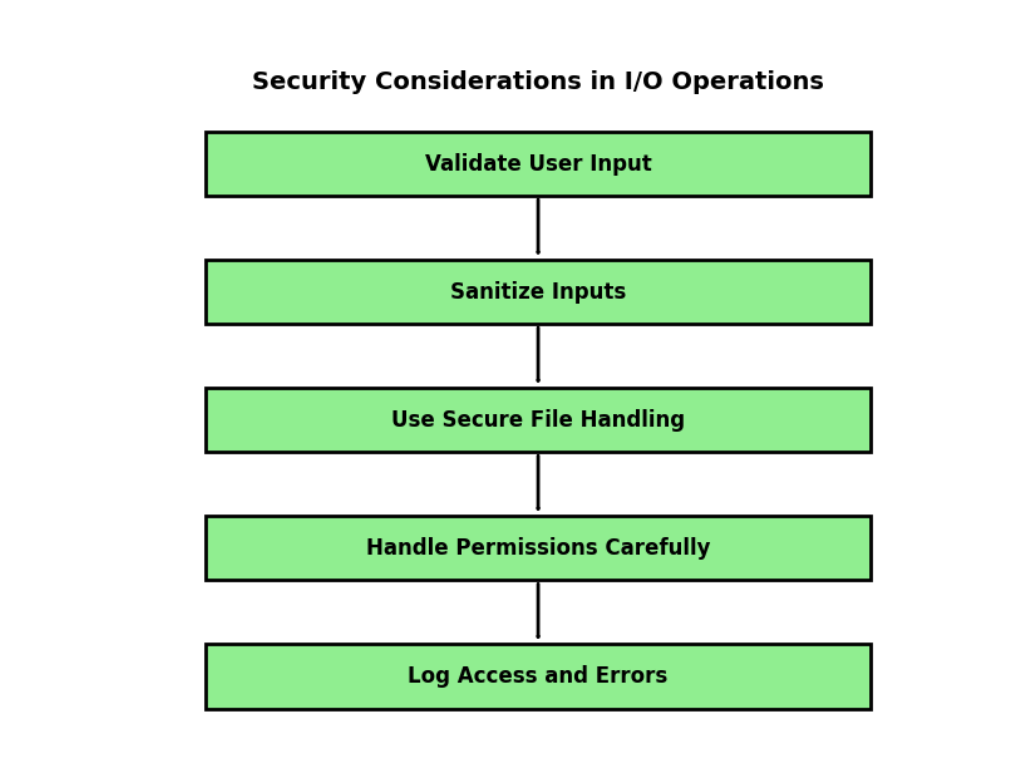
Protecting Against Injection Attacks in Python Input
Injection attacks occur when an attacker is able to insert malicious code into an application via user input. This can lead to severe security vulnerabilities, such as unauthorized access or data corruption. Here are key strategies to protect against these attacks:
1. Validate User Input
Always validate user input to ensure it meets expected criteria before processing it. For example, if expecting a numeric input, make sure the input is indeed a number and within a valid range.
def get_positive_number():
while True:
try:
number = int(input("Enter a positive number: "))
if number <= 0:
raise ValueError("Number must be positive.")
return number
except ValueError as e:
print(f"Invalid input: {e}. Please try again.")
In this function, user input is validated to ensure it is a positive integer. If the input is invalid, an error message is shown, and the user is prompted to try again. This helps prevent malicious data from being processed.
2. Use Parameterized Queries for Database Access
When interacting with databases, use parameterized queries rather than directly incorporating user input into SQL statements. This prevents SQL injection attacks by separating SQL code from user data.
import sqlite3
def fetch_user_data(user_id):
conn = sqlite3.connect('database.db')
cursor = conn.cursor()
cursor.execute("SELECT * FROM users WHERE id = ?", (user_id,))
data = cursor.fetchone()
conn.close()
return data
Here, the execute
method uses a parameterized query, where ?
acts as a placeholder for the user input. This approach ensures that the input is treated as data rather than executable code, mitigating the risk of SQL injection.
3. Sanitize Input
Sanitize user input to remove or neutralize any potentially harmful characters or code before using it in your application.
import re
def sanitize_input(user_input):
# Remove any characters that are not alphanumeric or spaces
return re.sub(r'[^a-zA-Z0-9 ]', '', user_input)
This function uses a regular expression to strip out any non-alphanumeric characters, reducing the risk of malicious code being processed by your application.
Safe File Handling Practices in Python
When working with files, following safe practices ensures that your file operations do not introduce security risks. Here’s how you can handle files securely:
1. Use Secure File Paths
Avoid hardcoding file paths and instead use functions to construct paths securely. This reduces the risk of path traversal attacks, where an attacker tries to access files outside the intended directory.
import os
def get_secure_path(filename):
base_dir = os.path.dirname(__file__) # Current directory
return os.path.join(base_dir, filename)
In this example, os.path.join
is used to build file paths securely, preventing unintended access to directories.
2. Handle File Permissions Carefully
Ensure that files are created with appropriate permissions to prevent unauthorized access. For example, avoid setting permissions that allow everyone to read or write to your files.
with open('sensitive_data.txt', 'w') as file:
file.write("Confidential Information")
# Restrict file permissions after creation
os.chmod('sensitive_data.txt', 0o600) # Read and write for owner only
Here, os.chmod
sets the file permissions to be readable and writable only by the owner, reducing the risk of unauthorized access.
3. Avoid Storing Sensitive Information in Plain Text
When storing sensitive information, such as passwords or personal data, use encryption to protect the data from unauthorized access.
from cryptography.fernet import Fernet
# Generate a key for encryption
key = Fernet.generate_key()
cipher_suite = Fernet(key)
# Encrypt data
encrypted_data = cipher_suite.encrypt(b"Sensitive Information")
# Decrypt data
decrypted_data = cipher_suite.decrypt(encrypted_data)
By encrypting sensitive data, you ensure that even if an attacker gains access to your files, they will not be able to read the information without the decryption key.
Performance Tips for Large-Scale I/O Operations
When dealing with large-scale input and output (I/O) operations in Python, efficiency becomes crucial. Managing large files and improving I/O performance can greatly affect the responsiveness and speed of your applications. In this section, we’ll explore how to handle large files efficiently in Python and improve I/O performance with buffered operations. These tips will help you write code that is not only functional but also optimized for performance.
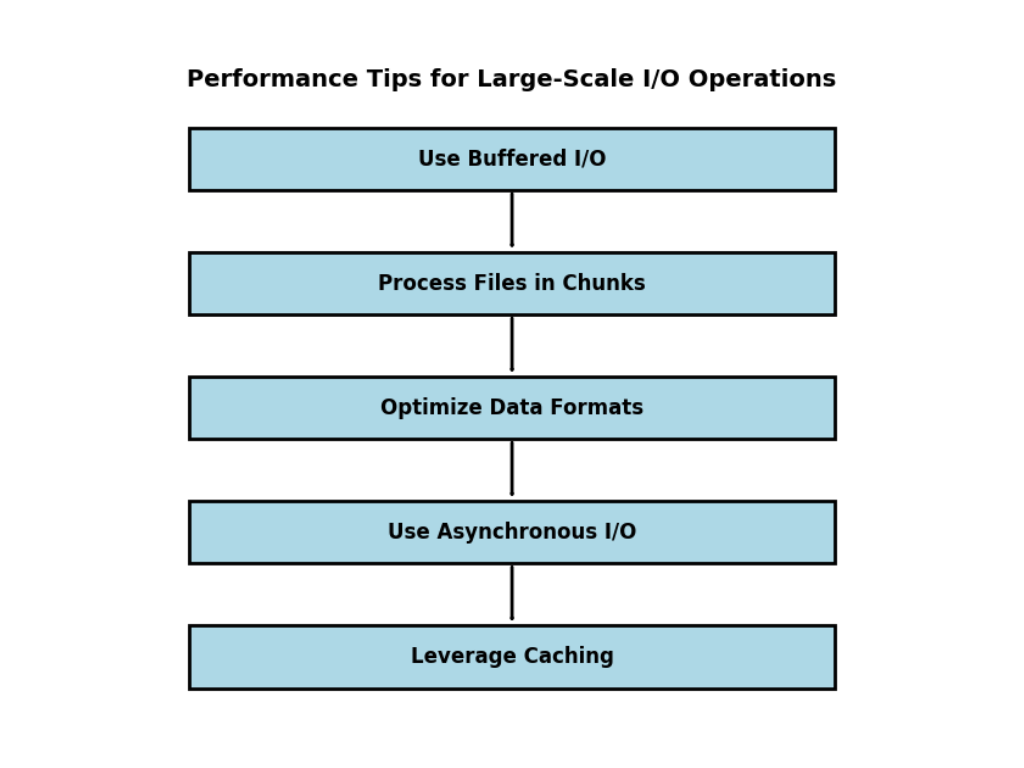
Handling Large Files Efficiently in Python
Working with large files can be challenging due to memory limitations and processing time. To handle large files efficiently, follow these strategies:
1. Process Files in Chunks
Instead of reading an entire large file into memory, process it in smaller chunks. This approach helps manage memory usage and speeds up processing.
def process_large_file(filename):
with open(filename, 'r') as file:
chunk_size = 1024 # Define the chunk size
while chunk := file.read(chunk_size):
# Process each chunk of data
print(f"Processing chunk: {chunk[:50]}...") # Example processing
In this example, the file is read in chunks of 1024 bytes. This method ensures that only a portion of the file is loaded into memory at any time, which is especially useful for very large files.
2. Use Generators for Large Data Sets
Generators allow you to iterate over data without loading it all into memory. This is particularly useful for large files or datasets.
def read_large_file(filename):
with open(filename, 'r') as file:
for line in file:
yield line # Yield each line as it is read
Here, the read_large_file
function yields lines one by one, allowing you to process each line individually without loading the entire file at once.
3. Optimize File Writing
When writing large amounts of data, buffering and flushing can help manage performance.
def write_large_file(filename, data):
with open(filename, 'w') as file:
file.writelines(data) # Write all data at once
Using writelines
for large data chunks reduces the number of write operations, which can enhance performance.
Improving I/O Performance with Buffered Operations
Buffered operations improve the efficiency of I/O operations by reducing the number of read and write operations performed. Here’s how you can leverage buffered I/O to boost performance:
1. Utilize Buffered I/O Streams
Buffered streams, such as io.BufferedReader
and io.BufferedWriter
, manage I/O more efficiently by using a buffer to temporarily hold data.
import io
def read_with_buffered_io(filename):
with io.open(filename, 'r', buffering=io.DEFAULT_BUFFER_SIZE) as file:
while chunk := file.read(io.DEFAULT_BUFFER_SIZE):
# Process each buffered chunk
print(f"Processing chunk: {chunk[:50]}...")
In this example, io.open
is used with buffering to handle data in chunks, which can speed up I/O operations by minimizing the frequency of actual read operations.
2. Use Memory-Mapped Files
Memory-mapped files allow you to access file contents directly through memory, improving access speed for large files.
import mmap
def read_with_memory_map(filename):
with open(filename, 'r+b') as file:
# Memory-map the file
mmapped_file = mmap.mmap(file.fileno(), 0)
# Access the file content through memory map
print(mmapped_file[:50])
mmapped_file.close()
Memory mapping enables you to treat file contents as if they were part of memory, which can significantly improve performance when working with large files.
3. Optimize File System Operations
Optimize file system operations by ensuring that files are stored on a fast and reliable storage medium. Using Solid-State Drives (SSDs) instead of traditional Hard Disk Drives (HDDs) can reduce read and write times.
import os
def optimize_file_storage(filename):
# Ensure the file is stored on a high-performance storage medium
if os.path.exists(filename):
print("File is stored on a reliable storage medium.")
Ensuring that files are stored on fast storage can reduce the time required for file operations, leading to better overall performance.
Conclusion
In the second part of our comprehensive guide on Python I/O operations, we explored the file handling, error management, and best practices. We began by covering the basics of file operations, including how to open, read, and write files, as well as handling file paths and modes. Understanding these basics is fundamental for effective file management in Python.
We then moved on to working with different file formats, such as CSV, JSON, and XML. By learning how to read and write these formats, you can handle various types of data efficiently, from simple text files to more complex structured data.
Our focus then shifted to practical file handling examples, including building a simple log file system, saving and loading user settings in JSON, and processing CSV data for analysis. These examples provide practical insights into how file handling can be applied in real-world scenarios.
We also covered error handling, emphasizing how to manage common input and output errors. Using try-except
blocks, we explored ways to gracefully handle invalid user input and ensure that file operations are both safe and reliable.
Finally, we discussed best practices for writing clean and efficient I/O code. This included optimizing file handling, ensuring user-friendly input and output, and addressing security considerations such as protecting against injection attacks and practicing safe file handling.
By integrating these best practices into your coding routine, you will enhance the quality and performance of your Python applications. Continue to practice these techniques through projects, and visit our blog for more advanced tutorials and updates to keep expanding your Python skills.
External Resources
- Python Documentation: File Handling
- URL: Python File Handling Documentation
- Description: The official Python documentation provides a thorough overview of file handling in Python, including file operations, modes, and examples. It’s a key reference for understanding the standard practices for file operations.
- Real Python: File Handling in Python
- URL: Real Python – File Handling
- Description: This Real Python tutorial offers an in-depth guide on working with files in Python, covering opening, reading, writing, and closing files. It also includes examples and best practices.
FAQs
Python provides several file modes for opening files:
Read ('r'
): Opens the file for reading. The file must exist.
Write ('w'
): Opens the file for writing. Creates a new file or truncates an existing file.
Append ('a'
): Opens the file for appending data. Creates a new file if it does not exist.
Binary ('b'
): Opens the file in binary mode, used in conjunction with other modes (e.g., 'rb'
, 'wb'
)
Errors in file handling can be managed using try-except
blocks. This allows you to catch and handle exceptions such as file not found errors, permission errors, or issues with reading and writing data. Providing meaningful error messages helps in diagnosing and resolving issues.
Python can handle various file formats:
CSV Files: Use the csv
module to read from and write to CSV files.
JSON Files: Use the json
module to handle JSON data for reading and writing.
XML Files: Use libraries like xml.etree.ElementTree
to parse and generate XML data.
Using file paths correctly involves:
Using absolute paths for consistent file access.
Using relative paths for files within the same project directory.
Employing the os.path
or pathlib
modules to handle file paths in a cross-platform manner.
To avoid data loss:
Always backup important files before making changes.
Use the write mode carefully to prevent overwriting existing data.
Implement error handling to manage unexpected issues during file operations.
Leave a Reply