Introduction
Welcome to the first part of our comprehensive guide on Python I/O operations! In this section, we’ll explore the essential concepts of handling input and output in Python, which are key to creating interactive and user-friendly applications.
We begin with the basics of input operations, where you’ll learn how to use the input()
function to gather data from users. This includes understanding how to read various types of input and manage different data formats.
Next, we’ll move on to advanced input handling techniques. You’ll discover how to handle multiple inputs, read data from files, and use sys.stdin
for more sophisticated input operations. These skills are vital for building more complex and responsive Python programs.
Then, we’ll explore the practical examples of Python input operations. We’ll cover how to build interactive programs, such as a simple calculator, and demonstrate how to collect and validate user data effectively. These examples will give you hands-on experience and show how these concepts come to life in real-world scenarios.
We’ll also explore the basics of output operations, including how to use the print()
function and format strings to present data clearly. You’ll learn how to display information in a way that is both informative and user-friendly.
By the end of this section, you’ll have a solid foundation in handling input and output in Python, ready to apply these techniques to your own projects. Let’s get started!
Getting Started with Input Operations in Python
Basics of Input in Python
When you’re writing a Python program, one of the first things you’ll want to do is get input from the user. This is where Python’s input()
function comes into play. It’s the go-to tool for collecting information directly from users, making your program interactive and dynamic.

Introduction to the input()
Function
The input()
function is simple yet powerful. It waits for the user to type something and hit enter, then returns whatever was typed as a string. This might seem basic, but it’s incredibly adaptable for all kinds of programs. Whether you’re asking users for their name, their favorite number, or any other piece of information, the input()
function is your friend.
Here’s a quick example:
name = input("What's your name? ")
print("Hello, " + name + "!")
In this example, the program asks for your name and then greets you. It’s as simple as that! But what if you want to do more than just collect strings?
How to Read User Input in Python
When it comes to reading user input in Python, you might need to handle different types of data. For instance, if you’re asking for a number, you’ll want to treat it as a number—not just a string. Let’s break this down.
By default, input()
gives you everything as a string. If you need a different data type, you’ll need to convert it. This is where type conversion comes in handy.
Let’s say you want to ask the user for their age. You’d start by getting their input as a string, then convert it to an integer:
age = int(input("How old are you? "))
print("You will be " + str(age + 1) + " next year.")
In this example, we’re using int()
to convert the input into an integer. This lets us do math with it—like figuring out how old the user will be next year. Just like that, your program can handle numbers, not just text.
Examples of Using input()
for Different Data Types
Python makes it easy to work with different data types. Here are some examples of how you can use input()
to handle various kinds of data.
- String Input:
city = input("Which city do you live in? ")
print("Wow, " + city + " is a great place!")
This is a basic string input, where the user enters the name of their city.
2. Integer Input:
favorite_number = int(input("What's your favorite number? "))
print("Your favorite number squared is " + str(favorite_number ** 2))
Here, we’re asking for a number and then doing some math with it.
3. Float Input:
height = float(input("How tall are you in meters? "))
print("Your height in centimeters is " + str(height * 100) + " cm.")
In this case, we use float()
to handle decimal numbers, perfect for things like height or weight.
4. Boolean Input:
is_student = input("Are you a student? (yes/no) ").lower() == 'yes'
print("Student status: " + str(is_student))
For yes/no questions, you can convert the input into a boolean value (True/False) to make your program more logical.
Each of these examples shows how you can tailor the input()
function to handle various data types, making your Python programs more flexible and user-friendly.
By understanding these basics of input in Python, you’re well on your way to creating programs that not only ask the right questions but also handle the answers in meaningful ways.
Advanced Input Handling Techniques
As you become more comfortable with Python, you’ll likely encounter situations where simple user input isn’t enough. You might need to handle multiple inputs at once, read data from files, or even use more advanced methods like sys.stdin
. These advanced input handling techniques can make your programs more efficient and adaptable.

Handling Multiple Inputs in Python
Sometimes, you might want to gather multiple pieces of information from the user in one go. Instead of asking for each input separately, Python allows you to handle multiple inputs in a single line, making your code cleaner and more efficient.
Here’s an example where we ask for both a user’s first and last name:
first_name, last_name = input("Enter your first and last name: ").split()
print("Hello, " + first_name + " " + last_name + "!")
In this example, the split()
method is used to separate the input based on spaces. If you need to handle inputs separated by something else, you can specify a different delimiter inside the split()
method, like a comma:
age, height = input("Enter your age and height (separated by a comma): ").split(',')
print("You are " + age + " years old and " + height + " tall.")
This approach is not only more efficient but also makes your program more user-friendly, as it reduces the number of prompts users have to respond to.
Reading Input from Files in Python
In many real-world applications, user input comes from files rather than being typed directly. This is common when working with large datasets or when automating tasks that require input from a predefined source. Reading input from files in Python is easy, and it opens up a lot of possibilities for more complex programs.
Here’s a simple example of how to read input from a file:
with open('input.txt', 'r') as file:
data = file.read()
print("File content:")
print(data)
In this example, we’re opening a file named input.txt
in read mode ('r'
). The with
statement ensures that the file is properly closed after reading, even if an error occurs. The read()
method reads the entire content of the file and stores it in the data
variable, which we then print out.
But what if you want to process the file line by line? Python makes that easy too:
with open('input.txt', 'r') as file:
for line in file:
print("Line:", line.strip())
This code reads the file line by line, stripping any extra spaces or newline characters at the end of each line, making it cleaner for further processing. Whether you’re reading configuration files, large data logs, or any structured input, understanding how to handle file input is a crucial skill.
Using sys.stdin
for Advanced Input Operations
For those looking to take their input handling to the next level, sys.stdin
provides more control and flexibility, especially in scenarios like competitive programming, where inputs are often provided in bulk or via command-line piping.
sys.stdin
allows you to read all input at once or line by line, similar to file reading, but from the standard input stream. Here’s how you can use it:
import sys
print("Enter some lines of text (Ctrl+D to stop):")
input_data = sys.stdin.read()
print("You entered:")
print(input_data)
This example uses sys.stdin.read()
to read everything the user types until they signal the end of input (Ctrl+D on Linux/Mac or Ctrl+Z on Windows). This method is particularly useful when dealing with large input data that needs to be processed as a whole.
If you need to handle input line by line, sys.stdin
also supports that:
import sys
print("Enter some lines of text (Ctrl+D to stop):")
for line in sys.stdin:
print("Line:", line.strip())
Here, sys.stdin
acts like a file object, letting you iterate over each line of input. This is especially handy in scripts that need to process input in a loop, making it ideal for more complex tasks.
Mastering these advanced input handling techniques—handling multiple inputs in Python, reading input from files in Python, and using sys.stdin
for advanced input operations—will give you greater control and flexibility in your programs.
Practical Examples of Python Input Operations
Understanding how to handle input in Python is essential, but applying it in real-world scenarios is where things get interesting. By building interactive Python programs, you can create applications that respond to user input in meaningful ways. In this section, we’ll explore some practical examples that demonstrate how to use Python’s input operations effectively.
Building Interactive Python Programs
Creating interactive programs is one of the most engaging aspects of learning Python. Whether it’s a simple text-based game, a data entry form, or a tool for calculations, interactive programs make your code come alive. By prompting users for input and then responding to their answers, you can make your programs more dynamic and user-friendly.
Let’s go through a couple of examples to see how this works in practice.
Example: Creating a Simple Calculator Using User Input
One of the classic beginner projects in Python is creating a simple calculator. It’s a perfect example of how to use input to build an interactive Python program. Here, we will ask the user to enter two numbers and an operation (like addition or subtraction), then perform the calculation and display the result.
# Simple Calculator in Python
# Getting user input for numbers and operation
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
operation = input("Enter an operation (+, -, *, /): ")
# Performing the calculation based on user input
if operation == '+':
result = num1 + num2
elif operation == '-':
result = num1 - num2
elif operation == '*':
result = num1 * num2
elif operation == '/':
if num2 != 0:
result = num1 / num2
else:
result = "Error: Division by zero"
else:
result = "Invalid operation"
# Displaying the result
print("The result is:", result)
In this example, the program interacts with the user by asking for numbers and the operation they wish to perform. It then uses conditional statements (if
, elif
, else
) to execute the correct operation and output the result. This basic calculator illustrates how building interactive Python programs can be both easy and rewarding.
Output
Enter the first number: 74
Enter the second number: 92
Enter an operation (+, -, *, /): /
The result is: 0.8043478260869565
Process finished with exit code 0
Example: Collecting and Validating User Data
Collecting and validating user data is a common requirement in many Python programs, especially in applications like forms, surveys, or any scenario where accurate data input is critical. Proper validation ensures that the program handles user input correctly, avoiding potential errors and improving the user experience.
Let’s create a simple example where the program asks for a user’s name, age, and email, then validates this data before proceeding.
# Collecting and validating user data
# Getting user input
name = input("Enter your name: ")
# Validating the name input
while not name.isalpha():
print("Invalid name. Please enter only letters.")
name = input("Enter your name: ")
age = input("Enter your age: ")
# Validating the age input
while not age.isdigit():
print("Invalid age. Please enter a number.")
age = input("Enter your age: ")
email = input("Enter your email: ")
# Validating the email input
while "@" not in email or "." not in email:
print("Invalid email. Please enter a valid email address.")
email = input("Enter your email: ")
# Displaying the collected data
print("\nCollected Data:")
print("Name:", name)
print("Age:", age)
print("Email:", email)
In this example, the program collects basic information from the user and validates it to ensure correctness. The isalpha()
method checks if the name contains only letters, isdigit()
ensures the age is a number, and a simple check on the email string ensures it includes the “@” and “.” characters. By repeatedly prompting the user until valid data is entered, the program prevents errors and ensures that the collected data is as accurate as possible.
Output
Enter your name: XXX
Enter your age: 22
Enter your email: XXX@gmail.com
Collected Data:
Name: XXX
Age: 22
Email: XXX@gmail.com
Process finished with exit code 0
These examples highlight the importance of practical examples of Python input operations in building useful and interactive applications.
Must Read
- How to Design and Implement a Multi-Agent System: A Step-by-Step Guide
- The Ultimate Guide to Best AI Agent Frameworks for 2025
- Data Science: Top 10 Mathematical Definitions
- How AI Agents and Agentic AI Are Transforming Tech
- Top Chunking Strategies to Boost Your RAG System Performance
Output Operations in Python
Basics of Output in Python
When learning Python, understanding how to display information is just as important as understanding how to gather it. Output is how your program communicates with the outside world—whether it’s showing the result of a calculation, displaying a message, or even debugging your code. The most fundamental tool for output in Python is the print()
function, and mastering it will make your programs more interactive and informative.

Introduction to the print()
Function
The print()
function in Python is probably the first function you’ll encounter when starting to learn the language. It’s used to display text or data to the console, making it a crucial part of your coding toolkit. The beauty of print()
lies in its simplicity and flexibility.
Here’s a basic example:
print("Hello, World!")
This simple line of code will display the text “Hello, World!” on the screen. But print()
can do much more than just display static text. It can handle variables, mathematical operations, and even more complex data structures like lists and dictionaries.
For example:
name = "Alice"
age = 30
print("Name:", name, "Age:", age)
In this example, print()
is used to display both static text (“Name:” and “Age:”) and the values of the variables name
and age
. The function automatically adds spaces between the arguments, making it easy to combine different types of information in one output.
How to Display Output in Python
Displaying output in Python isn’t limited to just text. You can use the print()
function to show the results of calculations, display data from variables, or even output formatted strings that make your data more readable. Understanding how to display output in Python effectively will make your programs more user-friendly and help you communicate information clearly.
Let’s look at some more examples:
- Displaying the result of a calculation:
num1 = 10
num2 = 5
result = num1 + num2
print("The result is:", result)
This will display: The result is: 15
.
2. Showing items in a list:
fruits = ["apple", "banana", "cherry"]
print("Fruits:", fruits)
The output will be: Fruits: ['apple', 'banana', 'cherry']
.
3. Displaying a dictionary:
person = {"name": "John", "age": 25, "city": "New York"}
print("Person Info:", person)
The output will be: Person Info: {'name': 'John', 'age': 25, 'city': 'New York'}
.
These examples show how flexible the print()
function is, allowing you to easily display a wide range of data types.
Formatting Strings for Better Output Readability
While the print()
function is powerful, there are times when you want to format your output for better readability. Python offers several ways to do this, including using f-strings (formatted string literals), the format()
method, and old-style string formatting with the %
operator. Proper formatting can make your output clearer and more professional, which is especially important when presenting data.
- Using f-strings (Python 3.6+):F-strings are one of the most efficient and readable ways to format strings in Python. They allow you to embed expressions inside string literals, using curly braces
{}
.
name = "Alice"
age = 30
print(f"Name: {name}, Age: {age}")
The output will be: Name: Alice, Age: 30
.
F-strings can also handle expressions:
print(f"5 + 3 = {5 + 3}")
This will output: 5 + 3 = 8
.
Using the format()
method:
Before f-strings, the format()
method was widely used for string formatting. It works by placing curly braces {}
as placeholders in the string, which are then replaced by the arguments passed to the format()
function.
name = "Bob"
age = 35
print("Name: {}, Age: {}".format(name, age))
The output will be: Name: Bob, Age: 35
.
You can also use positional or keyword arguments for more control:
print("Name: {0}, Age: {1}".format(name, age))
3. Old-style string formatting with %
:
This method is older and less commonly used in modern Python code, but you might still encounter it in legacy codebases.
name = "Carol"
age = 28
print("Name: %s, Age: %d" % (name, age))
The output will be: Name: Carol, Age: 28
.
Formatting strings properly not only improves readability but also helps prevent errors in complex programs. As you continue to learn Python, practicing these techniques will make you more confident in handling output in your programs.
Advanced Output Techniques
As you progress in your Python journey, you’ll find that handling output involves more than just printing to the console. Sometimes, you’ll need to save data to a file or control where your output goes. These advanced output techniques in Python allow you to manage your data more effectively and make your programs more adaptable.
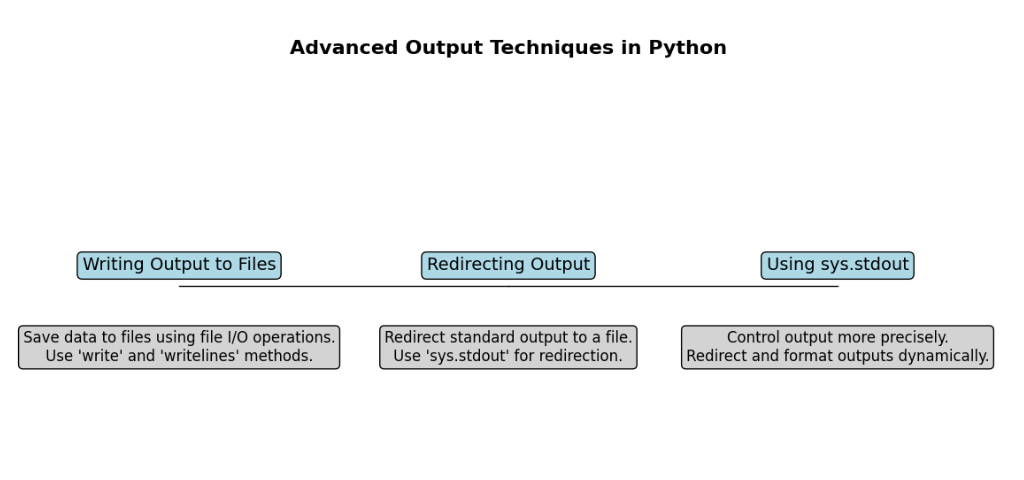
Writing Output to Files in Python
When working with Python, there will be situations where you want to write output to files rather than just display it on the screen. Saving data to a file can be useful for logging, saving the results of a computation, or simply keeping records of your program’s output.
Writing to a file in Python is easy. You start by opening the file in the desired mode—whether it’s writing ('w'
), appending ('a'
), or even reading ('r'
). Here’s how you can write to a file:
# Opening a file in write mode
with open('output.txt', 'w') as file:
file.write("Hello, World!\n")
file.write("This is a line of text.\n")
In this example, the open()
function is used to open output.txt
in write mode. The with
statement ensures that the file is properly closed after writing, which is a good practice to avoid file corruption. The write()
method is then used to output text to the file. If output.txt
does not exist, Python will create it. If it does exist, its contents will be overwritten.
If you want to add more content without erasing existing data, you can open the file in append mode ('a'
):
# Opening a file in append mode
with open('output.txt', 'a') as file:
file.write("This line will be added to the existing file.\n")
This will add new text at the end of the file without removing any existing content.
Redirecting Output in Python
Sometimes, you might want to redirect your output from the console to a file or another output stream. This can be particularly useful when running scripts that generate a lot of output or when you want to log your program’s behavior.
Python makes it easy to redirect output using the sys
module. For instance, you can redirect the output of print()
statements to a file like this:
import sys
# Redirecting output to a file
with open('output_log.txt', 'w') as file:
sys.stdout = file
print("This will be written to output_log.txt instead of the console.")
sys.stdout = sys.__stdout__ # Resetting to default
In this example, sys.stdout
is temporarily set to the file object file
. This means that any output from print()
will go into output_log.txt
rather than the console. After you’re done, it’s important to reset sys.stdout
to its original value (sys.__stdout__
) to return to normal behavior.
This technique is handy when you need to log the output of an entire program run without changing every print()
statement individually.
Using sys.stdout
for Advanced Output Control
For those who need finer control over their program’s output, using sys.stdout
directly can offer more flexibility. sys.stdout
is the file object that Python uses by default for its output. By changing or customizing sys.stdout
, you can control where and how your program’s output is displayed.
For example, you might want to redirect output to a different location or even filter the output before it’s displayed. Here’s a simple example:
import sys
class CustomOutput:
def write(self, text):
sys.__stdout__.write(text.upper()) # Convert output to uppercase
def flush(self):
pass # Handle flushing if necessary
# Redirecting sys.stdout to CustomOutput
sys.stdout = CustomOutput()
print("This text will be in uppercase.")
In this example, we create a custom class CustomOutput
that overrides the write()
method to convert all output to uppercase. By setting sys.stdout
to an instance of CustomOutput
, all subsequent output will be affected. This kind of customization can be particularly useful in specialized applications where you need to preprocess or format output in a specific way.
Practical Examples of Python Output Operations
In Python, output operations go beyond just printing a simple message to the console. The way you handle output can make your programs more user-friendly and professional. Let’s explore a few practical examples of Python output operations that will help you create well-formatted console applications, write user data to a text file, and generate reports from datasets. Each example will provide you with the necessary tools to enhance your Python projects.
Creating Well-Formatted Console Applications
Creating well-formatted console applications is a crucial skill that can improve the overall user experience. Whether you’re developing a simple script or a more complex application, the way you present information on the console can make a significant difference.
Consider a scenario where you’re creating a console application that displays a menu for users to select from. Here’s how you might format the output to make it clear and easy to read:
def display_menu():
print("Welcome to My Application!")
print("==========================")
print("Please choose an option:")
print("1. Start a new game")
print("2. Load a saved game")
print("3. Exit")
print("==========================")
display_menu()
In this example, the display_menu()
function prints a menu with clear headings and options. The use of equal signs (=
) creates a visual separation, making the menu easier to navigate. By organizing the output this way, you help users quickly understand their options, which is particularly important for applications with multiple features.
Example: Writing User Data to a Text File
Another common task in Python is writing user data to a text file. This can be useful in various scenarios, such as saving user preferences, logging information, or storing input data for later use.
Let’s look at an example where we collect a user’s name and age, and then save that information to a text file:
def save_user_data():
name = input("Enter your name: ")
age = input("Enter your age: ")
with open('user_data.txt', 'a') as file:
file.write(f"Name: {name}, Age: {age}\n")
print("Your data has been saved!")
save_user_data()
In this script, the save_user_data()
function prompts the user for their name and age. It then opens a file called user_data.txt
in append mode ('a'
), so new entries are added without overwriting existing data. The write()
method formats the data into a string and saves it to the file. Finally, a confirmation message is printed to let the user know their data has been successfully saved.
This example demonstrates how you can efficiently store user input in a file, which can be useful for logging or record-keeping purposes.
Example: Generating a Report from a Dataset
Generating reports from datasets is a common requirement in data analysis, business applications, and many other fields. Python makes it easy to process and format data into a report that can be saved and shared.
Suppose you have a dataset of sales figures and want to generate a report that summarizes the total sales for each product. Here’s how you might do it:
import csv
def generate_sales_report():
sales_data = [
{'product': 'Widget', 'quantity': 10, 'price': 2.50},
{'product': 'Gadget', 'quantity': 15, 'price': 3.00},
{'product': 'Doodad', 'quantity': 7, 'price': 1.25}
]
with open('sales_report.txt', 'w') as report:
report.write("Sales Report\n")
report.write("============\n")
total_sales = 0
for item in sales_data:
product = item['product']
quantity = item['quantity']
price = item['price']
sales = quantity * price
total_sales += sales
report.write(f"{product}: {quantity} units sold, Total Sales: ${sales:.2f}\n")
report.write("============\n")
report.write(f"Total Sales: ${total_sales:.2f}\n")
print("Sales report has been generated!")
generate_sales_report()
In this example, the generate_sales_report()
function takes a list of dictionaries representing sales data. Each dictionary contains the product name, quantity sold, and price per unit. The function calculates the total sales for each product and writes this information to a file called sales_report.txt
.
The report is formatted with clear headings and a summary of total sales at the end. This method makes the report easy to read and provides a quick overview of the data.
Conclusion
In this first part of our exploration into Python I/O operations, we’ve covered a broad range of essential topics. We started with the basics of input operations in Python, including an introduction to the input()
function and how to handle various data types. We then advanced to more complex input handling techniques, such as managing multiple inputs and reading data from files. These skills are crucial for creating interactive Python programs, like a simple calculator or user data collection tool.
We also explored the fundamentals of output operations, focusing on how to use the print()
function and format strings for clear and readable output. From there, we moved on to advanced techniques for output, including writing to files, redirecting output, and using sys.stdout
for greater control.
To wrap it up, we provided practical examples, such as generating well-formatted console applications and writing user data to text files or generating reports from datasets. These hands-on examples aim to reinforce the concepts and demonstrate their practical applications.
As you continue learning, practice these input and output techniques through real-world projects. Experiment with creating your own interactive programs and formatting output to better understand their capabilities. For more advanced Python tutorials and to deepen your knowledge, feel free to visit our blog.
External Resources
- Python Documentation: Input and Output
- URL: Python I/O Documentation
- Description: The official Python documentation provides a detailed overview of input and output operations, including examples of the
input()
andprint()
functions. It’s a great starting point for understanding the basics and advanced features of Python’s I/O system.
- Real Python: Working with Files in Python
- URL: Real Python – Working with Files
- Description: Real Python offers a comprehensive guide on file handling in Python. It covers various file operations, including opening, reading, writing, and managing different file formats like CSV and JSON.
FAQs
1. What is the purpose of the input()
function in Python?
The input()
function is used to read data entered by the user from the keyboard. It allows you to capture user input as a string and can be used in interactive programs where user interaction is required.
2. How can I read multiple pieces of data from a user?
To read multiple pieces of data from a user, you can prompt the user for input several times or ask them to enter data in a specific format that can be split into separate values. For example, asking for all inputs in one line and then separating them into individual pieces.
3. How do I handle different types of data when using input()
?
Since input()
always returns data as a string, you need to convert it to other types, like integers or floats, depending on what you need. This ensures that the data can be used correctly in your program.
4. What is the role of the print()
function in Python?
The print()
function displays output on the screen. It’s used to show text, variables, or results to users, helping them understand the output of the program or providing information.
5. How can I make my output more readable?
You can make your output more readable by formatting it properly. This can involve arranging the information in a clear and organized manner or using special formatting to highlight key details. Python provides various ways to format text to improve readability.