Introduction
Mutable vs Immutable: When you write Python code, you deal with different types of data—numbers, lists, strings, dictionaries, and more. Some of these can be changed (mutable), while others stay the same (immutable) once created. Understanding this difference is super important. Because it affects how your code works, how fast it runs, and how Python handles memory.
If you’ve ever wondered why lists behave differently from tuples or why some objects seem to change unexpectedly, you’re not alone! In this guide, we’ll break down mutable vs immutable in Python in the simplest way possible. By the end, you’ll know exactly when to use which type and how this knowledge can help you write better, more efficient code.
What Are Mutable and Immutable Types in Python?
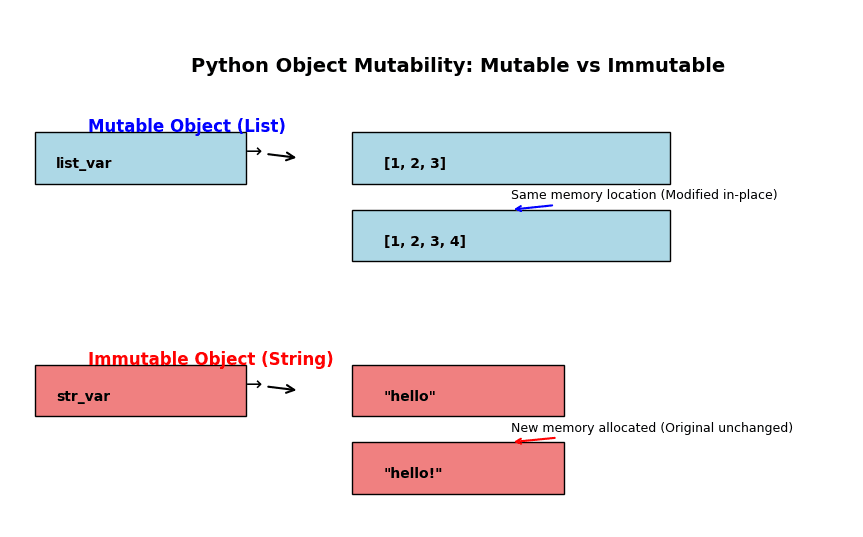
Understanding Python Data Types
In Python, everything is an object—whether it’s a number, a string, a list, or even a function. Each object has three key things:
- Type – What kind of object it is (like
int
,str
, orlist
). - Value – The actual data stored in the object.
- ID (Identity) – A unique identifier (memory address) that tells Python where the object is stored.
Now, some objects can be changed (mutable), while others cannot (immutable). This is where mutable vs immutable comes into play!
How Python Handles Memory Management
Python manages memory using something called reference counting. Every time you create a new object, Python keeps track of how many variables are referring to it. When no variables are using an object anymore, Python automatically deletes it to free up memory (this process is called garbage collection).
But here’s the catch: mutable and immutable objects behave differently when it comes to memory!
- Immutable objects (like numbers, strings, and tuples) don’t change when modified. Instead, Python creates a new object in memory.
- Mutable objects (like lists and dictionaries) can change without creating a new object. This means multiple variables can point to the same object, which can sometimes cause unexpected changes in your code!
The Role of Objects and References in Python
When you assign a variable in Python, you’re not storing the actual value—you’re storing a reference (or pointer) to the object in memory.
Example:
x = [1, 2, 3]
y = x
y.append(4)
print(x) # Output: [1, 2, 3, 4]
print(y) # Output: [1, 2, 3, 4]
Here, x
and y
both point to the same list in memory, so changing y
also changes x
. This is because lists are mutable!
But if we do the same thing with an immutable object, like a string:
a = "Hello"
b = a
b = b + " World"
print(a) # Output: Hello
print(b) # Output: Hello World
In this case, a
and b
no longer point to the same object because strings cannot be changed. Python creates a new string when we modify b
.
Understanding this difference is key to avoiding bugs and writing efficient Python programs!
What Is a Mutable Data Type in Python?
A mutable object in Python is an object that can be changed after it is created. This means you can modify its content without changing its memory address.
Mutable objects are useful when you need to update data frequently without creating new objects. But they can also lead to unexpected behavior if multiple variables reference the same object!
How Mutable Types Allow In-Place Modifications
When you modify a mutable object, Python keeps the same memory location and updates the existing object instead of creating a new one.
Example:
numbers = [1, 2, 3]
print(id(numbers)) # Memory address before modification
numbers.append(4)
print(id(numbers)) # Memory address remains the same
Even after adding 4
to the list, its memory address stays the same because lists are mutable.
Examples of Mutable Data Types in Python
1. Lists
Lists are one of the most commonly used mutable objects. You can add, remove, or change elements without creating a new list.
fruits = ["apple", "banana", "cherry"]
fruits[1] = "orange" # Modifies the list in-place
print(fruits) # Output: ['apple', 'orange', 'cherry']
2. Dictionaries
Dictionaries allow you to modify values, add new key-value pairs, and remove items without changing the dictionary’s identity.
person = {"name": "Alice", "age": 25}
person["age"] = 26 # Modifying an existing key
person["city"] = "New York" # Adding a new key
print(person) # Output: {'name': 'Alice', 'age': 26, 'city': 'New York'}
3. Sets
Sets are mutable, meaning you can add or remove elements, but since they are unordered, you can’t modify existing elements directly.
numbers = {1, 2, 3}
numbers.add(4) # Adds an element
numbers.remove(2) # Removes an element
print(numbers) # Output: {1, 3, 4}
Since mutable objects can be changed in place, you need to be careful when assigning them to multiple variables—because changes made in one place might affect another!
What Is an Immutable Data Type in Python?
An immutable object in Python is an object that cannot be changed after it is created. If you try to modify it, Python creates a new object instead of changing the existing one.
Immutable data types help keep data safe from unintended modifications and are commonly used in scenarios where data should remain constant.
Why Immutable Types Cannot Be Modified After Creation
Since immutable objects cannot be changed in place, any modification creates a new object with a different memory address.
Example:
name = "Alice"
print(id(name)) # Memory address before modification
name += " Smith" # Creates a new string
print(id(name)) # Memory address changes
Even though it looks like we modified name
, Python actually created a new string object and assigned it to name
, leaving the original "Alice"
unchanged.
Examples of Immutable Data Types in Python
1. Tuples
Tuples are like lists, but they cannot be modified after creation.
numbers = (1, 2, 3)
# numbers[1] = 4 # ❌ This will raise an error
If you need a modified tuple, you must create a new one.
new_numbers = numbers + (4, 5)
print(new_numbers) # Output: (1, 2, 3, 4, 5)
2. Strings
Strings are immutable, meaning you cannot change a string in place.
text = "hello"
# text[0] = "H" # ❌ This will raise an error
Instead, Python creates a new string when you modify it.
text = text.upper()
print(text) # Output: "HELLO"
3. Integers and Floats
Numbers in Python do not change—any operation creates a new number.
a = 10
print(id(a)) # Memory address before change
a += 5 # Creates a new integer object
print(id(a)) # Memory address changes
4. Frozen Sets
Frozen sets are like regular sets, but they cannot be changed after creation.
frozen = frozenset([1, 2, 3])
# frozen.add(4) # ❌ This will raise an error
Since immutable objects cannot be changed, they are safer to use in multi-threaded applications and more memory-efficient in some cases. But when you need flexibility, mutable objects are the way to go!
Must Read
- How to Return Multiple Values from a Function in Python
- Parameter Passing Techniques in Python: A Complete Guide
- A Complete Guide to Python Function Arguments
- How to Create and Use Functions in Python
- Find All Divisors of a Number in Python
Key Differences Between Mutable and Immutable Types in Python
How Python Uses Memory for Variables
When you create a variable in Python, Python has to store it somewhere in memory. But how it stores the variable depends on whether it’s mutable or immutable.
Let’s break it down:
- Immutable objects (numbers, strings, tuples) → Cannot change once created.
- Mutable objects (lists, dictionaries, sets) → Can be changed after creation.
This difference affects memory usage and performance.
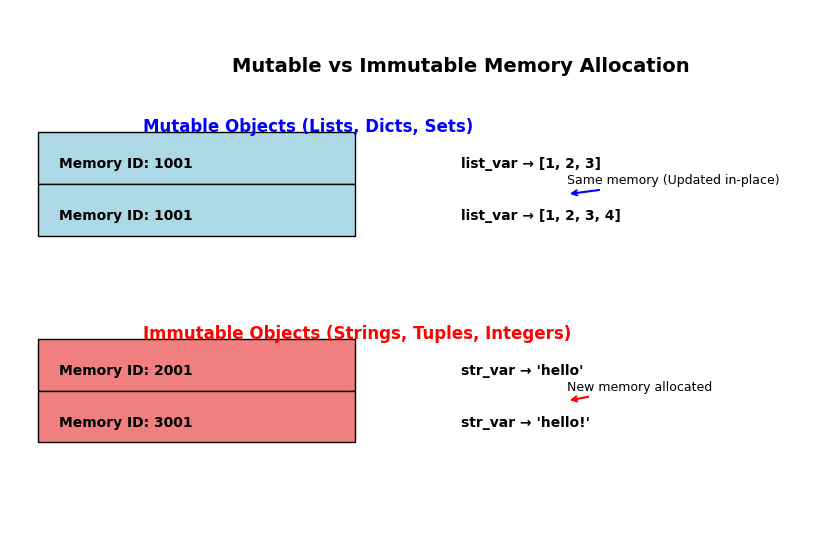
How Immutable Objects Save Memory
Let’s say you create a number:
x = 10
y = 10
Python doesn’t create two separate copies of 10
. Instead, both x and y point to the same memory location.
Check this out:
print(id(x)) # Memory address of x
print(id(y)) # Memory address of y (same as x)
print(id(x)) # Memory address of x
print(id(y)) # Memory address of y (same as x)
Since numbers never change, Python reuses the same memory to save space.
What Happens When You Change an Immutable Object?
Now, let’s change y
:
y = y + 5
Python doesn’t update the existing number. Instead, it creates a new number (15) and assigns y
to that new value. x
still points to 10
.
Check this out:
print(id(x)) # Memory address of x (still the same)
print(id(y)) # New memory address for y (now it’s 15)
This means each time you change an immutable object, Python creates a new one instead of modifying the existing one.
How Mutable Objects Use Memory Differently
Let’s do the same test with a list (which is mutable).
list1 = [1, 2, 3]
list2 = list1 # Both variables point to the same list
Now, let’s modify list1:
list1.append(4)
print(list2) # Output: [1, 2, 3, 4]
Wait… why did list2
change even though we only modified list1?
It’s because lists are mutable, so list1
and list2
both point to the same object in memory.
Check their memory addresses:
print(id(list1)) # Same memory address
print(id(list2)) # Same memory address
Since lists can change, Python doesn’t create a new copy—it just updates the existing one.
Copy-on-Write: Python’s Smart Way to Save Memory
Python tries to avoid making copies of mutable objects until it absolutely has to.
Let’s say you make a shallow copy of a list:
import copy
original = [1, 2, 3]
shallow_copy = copy.copy(original)
print(id(original), id(shallow_copy)) # Same memory address
At first, both variables share the same memory.
But if you modify shallow_copy
:
shallow_copy.append(4)
Now, Python creates a new list in memory because it sees that you changed the copy.
Check their memory addresses again:
print(id(original)) # Original stays the same
print(id(shallow_copy)) # New memory address
This is called copy-on-write. Python delays copying until a change happens to save memory.
Key Lessons from This
- Immutable objects (numbers, strings, tuples) don’t change. If you modify them, Python creates a new object.
- Mutable objects (lists, dictionaries, sets) can change in place. Be careful when assigning them to multiple variables.
- Python uses smart memory tricks like reusing immutable objects and copy-on-write for mutable objects to save space.
So, next time you write Python code, think about how your variables use memory—it can make your programs faster and more efficient!
What Happens When You Pass Variables to a Function?
When you give a variable to a function, Python handles it differently depending on whether it’s mutable or immutable.
- If the variable is immutable (like a number or a string), the function CANNOT change it directly.
- If the variable is mutable (like a list or dictionary), the function CAN change it directly.
Let’s see this in action!
Immutable Objects Inside Functions
Let’s say we pass a string to a function:
def change_text(text):
text = text + " world" # Trying to change the string
print("Inside function:", text)
message = "hello"
change_text(message)
print("Outside function:", message) # Original string is unchanged
What’s happening here?
- Inside the function,
text
gets a new value ("hello world"
). - But Python creates a new string instead of modifying the original one.
- Outside the function,
message
is still"hello"
.
Strings are immutable, so the function cannot change the original variable.
Mutable Objects Inside Functions
Now, let’s try the same thing with a list, which is mutable:
def change_list(numbers):
numbers.append(4) # Adding an item to the list
print("Inside function:", numbers)
my_list = [1, 2, 3]
change_list(my_list)
print("Outside function:", my_list) # The list has changed!
What’s happening here?
- Inside the function,
numbers.append(4)
modifies the original list. - Even outside the function,
my_list
is now[1, 2, 3, 4]
.
Since lists are mutable, the function directly changes the original list in memory.
How to Stop a Function from Changing a List?
Sometimes, you don’t want a function to change your list. You can pass a copy instead:
def safe_change(numbers):
numbers = numbers.copy() # Create a new list
numbers.append(4)
print("Inside function:", numbers)
my_list = [1, 2, 3]
safe_change(my_list)
print("Outside function:", my_list) # Original list stays the same!
Now, the original list is not changed because we made a copy inside the function.
Key Lessons
- Immutable objects (like numbers and strings) stay the same inside a function. Python creates a new object instead of modifying the original one.
- Mutable objects (like lists and dictionaries) can be changed inside a function because they are stored in memory by reference.
- If you don’t want a function to change a list, pass a copy instead of the original.
This is why Python’s behavior in functions depends on whether the object is mutable or immutable!
Why Can Some Things Be Used as Dictionary Keys and Others Can’t?
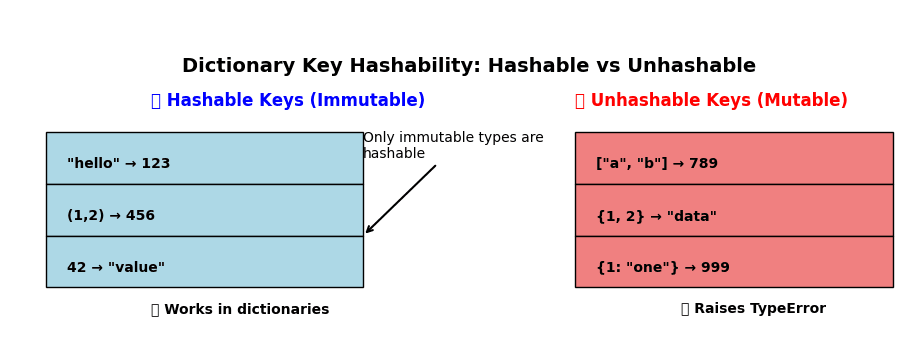
In Python, you can use some things as dictionary keys, but not others. This is because Python needs dictionary keys to be unchangeable.
- Things that NEVER change (immutable) can be used as keys.
- Things that CAN change (mutable) cannot be used as keys.
Let’s go step by step.
What Happens When You Use an Immutable Object as a Dictionary Key?
Python is happy when you use things like:
- Strings (
"apple"
) - Numbers (
42
) - Tuples (
(1, 2, 3)
)
Example:
my_dict = {
"name": "Alice",
10: "A number",
(1, 2, 3): "A tuple"
}
✔️ This works perfectly! Why? Because these things never change once they’re created.
Python remembers them using a unique code called a hash. That’s why they’re called hashable.
What Happens When You Use a Mutable Object as a Dictionary Key?
Now, let’s try using a list as a dictionary key:
my_dict = {
[1, 2, 3]: "A list as a key"
}
This gives an error:
TypeError: unhashable type: 'list'
Why does this happen?
A list can be changed at any time. Imagine if you add [1, 2, 3]
as a key and then change it to [4, 5, 6]
. Python would get confused because it wouldn’t know where to find your value anymore.
That’s why lists, dictionaries, and sets cannot be dictionary keys—because they can change!
What If You REALLY Need a List as a Dictionary Key?
There’s a trick! You can convert the list into a tuple because tuples never change.
Correct way:
my_dict = {
tuple([1, 2, 3]): "A tuple key made from a list"
}
Now Python accepts it because tuples are immutable.
Simple Rules to Remember
✔️ Immutable things (like strings, numbers, and tuples) can be dictionary keys.
❌ Mutable things (like lists and dictionaries) cannot be dictionary keys.
🔄 If you need to use a list as a key, convert it to a tuple first.
That’s it! Now you understand why some things work as dictionary keys and others don’t!
Practical Examples of Mutable vs Immutable in Python
Modifying Mutable Objects in Python (Made Simple!)
Let’s say you have a list:
my_list = [1, 2, 3]
Now, you pass it into a function:
def modify_list(lst):
lst.append(100) # Adding 100 to the list
modify_list(my_list)
print(my_list) # Output: [1, 2, 3, 100]
What Just Happened?
- You created a list:
my_list = [1, 2, 3]
. - You sent it to the function.
- Python did NOT make a copy.
- Instead, it sent the same list to the function.
- Inside the function,
lst.append(100)
changed the original list. - Now, when you print
my_list
, you see[1, 2, 3, 100]
because the function modified the same list in memory.
Why Did This Happen?
Lists are mutable, which means they can be changed in place.
When you pass a list to a function, Python doesn’t create a new list—it just gives the function a reference (or “shortcut”) to the same list in memory.
So, when you modify the list inside the function, you are actually modifying the original list.
How to Avoid Changing the Original List
If you don’t want the function to change your original list, you can pass a copy instead:
def modify_list_copy(lst):
lst = lst.copy() # Creates a new list (copy of the original)
lst.append(100)
print("Inside function:", lst)
my_list = [1, 2, 3]
modify_list_copy(my_list)
print("Outside function:", my_list) # Output: [1, 2, 3] (unchanged)
Now, the function modifies the copy, not the original list.
✔️ Safe and no accidental changes!
Simple Rule to Remember
✅ Mutable objects (like lists) change when passed to a function.
❌ If you don’t want changes, pass a copy instead.
That’s it! Now you know how functions affect mutable objects in Python.
Attempting to Modify Immutable Objects in Python (Explained Simply!)
Let’s say you have a string in Python:
my_str = "Hello"
Now, you pass it into a function:
def modify_string(s):
s += " World" # Trying to change the string
modify_string(my_str)
print(my_str) # Output: "Hello" (unchanged)
What Just Happened?
- You created a string:
my_str = "Hello"
. - You sent it to the function
modify_string(s)
.- Python DID create a copy this time (because strings are immutable).
- Inside the function, Python created a new string
"Hello World"
but did not changes
outside the function. - When you print
my_str
, it’s still"Hello"
, because the original string was never modified.
Why Are Strings Immutable?
Strings cannot be changed once they are created. Instead of modifying the original string, Python creates a new one and assigns it to s
inside the function. But since Python only changes s
inside the function, the original my_str
stays the same outside.
How Can You Modify a String?
Since strings can’t be changed, you need to return the new string and store it:
def modify_string(s):
s += " World"
return s # Return the new string
my_str = "Hello"
my_str = modify_string(my_str) # Store the new value
print(my_str) # Output: "Hello World"
Now, the original string is replaced with the new one!
Simple Rule to Remember
- Immutable objects (like strings) do not change inside functions.
- To “modify” a string, you must return a new one and store it.
That’s it! Now you understand why strings in Python are immutable and how to handle them inside functions.
Copying Mutable vs Immutable Objects in Python (Explained Simply!)
When you copy something in Python, you expect to get a new version of it, right? Well, it’s not always that simple! Some objects behave exactly how you’d expect, while others can lead to surprising changes. Let’s break it down.
Copying Immutable Objects (Safe & Simple)
Immutable objects cannot be changed, so copying them is straightforward.
x = 10
y = x # Copying an integer
y += 5
print(x) # Output: 10 (unchanged)
print(y) # Output: 15
Why does this work fine?
When you copy an immutable object (like a number or a string), Python creates a completely new one. Changing y
doesn’t affect x
.
Copying Mutable Objects (Can Be Tricky!)
Mutable objects can be changed, and copying them can lead to unexpected behavior.
Shallow Copy (Not a Full Copy!)
A shallow copy creates a new object, but it still refers to the same inner objects.
import copy
list1 = [[1, 2], [3, 4]]
list2 = copy.copy(list1) # Shallow copy
list2[0].append(99) # Changing the first sublist
print(list1) # Output: [[1, 2, 99], [3, 4]] 😲
print(list2) # Output: [[1, 2, 99], [3, 4]]
Wait, what? Why did list1
change when we only modified list2
?
💡 The issue:
list2
is a new list, but the inner lists inside it are still shared withlist1
.- When we changed
list2[0]
, it also affectedlist1[0]
because both lists point to the same inner lists in memory.
Deep Copy (A True, Independent Copy!)
A deep copy makes a brand-new copy of the object and all of its inner parts, so changes won’t affect the original.
list1 = [[1, 2], [3, 4]]
list3 = copy.deepcopy(list1) # Deep copy
list3[0].append(99) # Changing the first sublist
print(list1) # Output: [[1, 2], [3, 4]] ✅ (unchanged)
print(list3) # Output: [[1, 2, 99], [3, 4]]
Now list1
stays the same! The deep copy completely separates the two lists.
Simple Rules to Remember
✅ Immutable objects (strings, numbers, tuples) are copied safely.
❌ Shallow copies of mutable objects still share inner data, which can cause unexpected changes!
✅ Use copy.deepcopy()
when you need a full, independent copy.
Next time you copy a list or dictionary, be careful! If you notice weird changes happening, a deep copy might be what you need.
When to Use Mutable vs Immutable Types in Python
Choosing the Right Data Type in Python (Explained Simply!)
When writing Python programs, you often need to choose between mutable and immutable data types. But how do you know which one to use? Let’s break it down in a way that makes sense.
When to Use Mutable Types (Lists, Dictionaries, Sets) ✅
Mutable types can be changed after they are created. Use them when you need to modify data frequently without making new copies.
Good Situations to Use Mutable Types:
You need to update or change the data often.
You are working with large data sets, and you don’t want to keep making new copies.
If you want to pass data to a function and modify it inside the function.
Example: Using a List for Efficiency
my_list = [1, 2, 3]
my_list.append(4) # Adding an item to the same list
print(my_list) # Output: [1, 2, 3, 4]
Why is this good?
- Python does not create a new list. It just modifies the existing one.
- This makes your program faster and saves memory.
When to Use Immutable Types (Tuples, Strings, Numbers)
Immutable types cannot be changed after they are created. Use them when you need data to stay the same for safety and reliability.
Good Situations to Use Immutable Types:
Want to make sure data never changes by accident.
You need to use the data as a dictionary key (only immutable types can be used as keys).
You are working with multiple threads (immutable objects are safer in multi-threaded programs).
Example: Using a Tuple for Safety
coordinates = (10, 20)
# coordinates[0] = 15 ❌ This will cause an error!
Why is this good?
- No one can accidentally modify
coordinates
. - You avoid bugs caused by unexpected changes.
Simple Rules to Remember
- Use mutable types when you need to change data frequently.
- Use immutable types when you want data to stay the same for safety.
- If you’re unsure, go with an immutable type—it’s safer and prevents accidental changes.
By picking the right data type, you’ll write faster, safer, and easier-to-understand Python code!
Best Practices for Using Mutable and Immutable Data (Made Simple!)
When working with Python, you need to be careful when using mutable (changeable) and immutable (unchangeable) data. If you don’t handle them correctly, you can run into unexpected bugs. Let’s go through some simple rules to help you avoid problems.
![A memory diagram showing how mutable default arguments ([]) persist changes, while using None correctly prevents unintended data sharing.](https://emitechlogic.com/wp-content/uploads/2025/03/Mutable-Default-Argument-Pitfall-Step-by-Step-Memory-Change-1024x397.png)
Be Careful When Changing Mutable Objects
Mutable objects (lists, dictionaries, sets) can be changed after they are created. This is useful, but sometimes, they change when you don’t expect it.
Mistake: Changing a List Inside a Function (Without Realizing It!)
def add_item(my_list):
my_list.append(100) # This changes the original list!
numbers = [1, 2, 3]
add_item(numbers)
print(numbers) # Output: [1, 2, 3, 100] (Oops! The function changed the list!)
What went wrong?
- The function modified
numbers
directly, so the change affects the original list.
Solution: Use a Copy Instead
def add_item(my_list):
new_list = my_list[:] # Creates a copy
new_list.append(100)
return new_list
numbers = [1, 2, 3]
new_numbers = add_item(numbers)
print(numbers) # Output: [1, 2, 3] (Original list is safe!)
print(new_numbers) # Output: [1, 2, 3, 100] (Only the copy is changed)
✔ Now the original list stays unchanged!
Use Tuples Instead of Lists for Data That Should Not Change
If you have data that should stay the same, use a tuple instead of a list.
Example: Using a Tuple for Fixed Data
days_of_week = ("Monday", "Tuesday", "Wednesday", "Thursday", "Friday")
# days_of_week[0] = "Sunday" ❌ This will cause an error!
✔ Tuples protect your data from being accidentally changed.
Use Frozen Sets for Data That Must Stay Unchanged
A normal set (set()
) can be changed, so it can’t be used as a dictionary key. If you need a set that never changes, use a frozen set (frozenset()
) instead.
Example: Using Frozen Sets in a Dictionary
permissions = {frozenset(["read", "write"]): "Admin", frozenset(["read"]): "User"}
print(permissions[frozenset(["read", "write"])]) # Output: Admin
✔ Frozen sets help when you need collections that stay constant!
Final Simple Rules to Follow
- If your data should never change, use tuples instead of lists.
- If you pass a list to a function and don’t want it to change, make a copy first.
- Use frozen sets when you need a set that doesn’t change.
By following these simple tips, you’ll avoid mistakes and write better Python code!
Common Pitfalls and How to Avoid Them
Be Careful with Mutable Objects in Default Arguments!
Let’s talk about a tricky mistake that happens when using lists (or other mutable objects) as default arguments in functions. It’s a small thing, but it can cause big problems if you’re not aware of it.
What Happens When You Use a List as a Default Argument?
Look at this function:
def add_item(my_list=[]):
my_list.append(1)
return my_list
print(add_item()) # Output: [1]
print(add_item()) # Output: [1, 1] (Wait… what?)
print(add_item()) # Output: [1, 1, 1] (Why is this growing?)
What’s going wrong here?
- You might expect that every time you call
add_item()
, it creates a new list. - But Python reuses the same list every time the function is called.
- This means the list keeps growing with each function call.
The Right Way: Use None
as the Default Argument
To fix this, use None
instead of []
as the default value. Then, inside the function, create a new list only when needed.
def add_item(my_list=None):
if my_list is None: # Create a new list only when needed
my_list = []
my_list.append(1)
return my_list
print(add_item()) # Output: [1]
print(add_item()) # Output: [1] (Now, each call starts fresh!)
print(add_item()) # Output: [1]
✔ Now, every function call gets a brand-new list, so there’s no weird behavior.
Why This Happens: Python Stores Mutable Defaults Once
When Python sees my_list=[]
, it creates the list once (not every time the function is called).
So, every call to add_item()
keeps using the same list instead of making a new one.
Using None
ensures that a new list is created only when needed.
Be Careful with Immutable Objects Too!
Now, let’s talk about immutable objects (like strings and tuples). If you modify them repeatedly, you might slow down your program without realizing it.
❌ The Wrong Way: Modifying a String in a Loop
s = "Hello"
for _ in range(5):
s += "!" # Each time, Python creates a NEW string!
print(s) # Output: "Hello!!!!!"
Why is this a problem?
- Strings cannot be changed, so every time
s += "!"
runs, Python creates a new string in memory. - If you do this many times, it wastes memory and slows things down.
The Right Way: Use a List and Join It Later
s_list = ["Hello"]
for _ in range(5):
s_list.append("!") # Modify the list instead
s = "".join(s_list) # Convert back to a string
print(s) # Output: "Hello!!!!!"
✔ This is much faster because lists can be modified efficiently, and join()
creates the final string in one step.
Simple Rules to Remember
- Never use a mutable object (like a list) as a default argument. Use
None
instead. - Strings are immutable—modifying them repeatedly is slow. Use lists and
join()
instead. - Dictionaries and sets can also behave oddly as default arguments. Use the same
None
trick.
By keeping these in mind, you’ll avoid weird bugs and write faster, cleaner Python code!
Wrapping It Up on Mutable vs Immutable: What You Should Remember
Alright, let’s keep it simple. Here’s what you really need to know about mutable and immutable objects in Python:
- Some things in Python can change (mutable), and some things cannot change (immutable).
- Mutable: Lists, dictionaries, and sets can be changed after you create them.
- Immutable: Strings, tuples, and numbers cannot be changed once created.
- Be careful when using mutable objects in functions.
- If you pass a list or dictionary to a function, it can change the original data.
- This can sometimes lead to unexpected bugs if you’re not careful.
- Solution: Use
None
as a default argument and create a new object inside the function.
- Immutable objects don’t change, but modifying them repeatedly can be slow.
- Every time you change a string, Python creates a new one instead of modifying the existing one.
- Solution: If you need to update a string multiple times, use a list first and join it later.
- Copying mutable objects needs special attention.
- If you copy a list the wrong way, changes in one copy might affect the other one.
- Solution: Use
copy.deepcopy()
when needed.
- Use the right type for the job.
- If you don’t want data to change, use tuples instead of lists.
- If you need a unique, unchangeable set of values, use a frozen set instead of a regular set.
What Should You Do Next?
Now that you understand this, try it out in your own Python code.
- Pass lists and strings into functions and see what happens.
- Copy dictionaries and modify them to see the difference.
- Experiment with
join()
instead of string concatenation.
The more you practice, the easier it gets!
Mutable vs Immutable Cheat Sheet

FAQs on Mutable vs Immutable in Python
1. Why can’t I change a string after creating it?
Strings in Python are immutable, which means once they are created, they cannot be modified. If you try to change a string (e.g., s += " world"
), Python creates a new string instead of modifying the original one. This helps keep strings safe and efficient.
2. How can I prevent accidental changes to a list inside a function?
If you pass a list to a function, any modifications inside the function will affect the original list. To avoid this, you can pass a copy of the list instead:
def modify_list(lst):
lst = lst.copy() # Creates a copy
lst.append(100)
return lst
my_list = [1, 2, 3]
new_list = modify_list(my_list)
print(my_list) # Output: 1, 2, 3
print(new_list) # Output: [1, 2, 3, 100]
3. When should I use a tuple instead of a list?
Use a tuple when you have data that should not change. Tuples are faster and can be used as dictionary keys, unlike lists. If you need to modify the data, use a list instead.
Why is using a mutable default argument in a function a bad idea?
If you use a mutable object (like a list) as a default argument, Python reuses the same object across multiple function calls. This can lead to unexpected behavior:
def add_item(my_list=[]):
my_list.append(1)
return my_list
print(add_item()) # Output: [1]
print(add_item()) # Output: 1, 1
Fix: Use None
and create a new list inside the function:
def add_item(my_list=None):
if my_list is None:
my_list = []
my_list.append(1)
return my_list
External Resources on Mutable vs Immutable
If you want to explore mutable vs immutable objects in Python further, check out these resources:
- Official Python Documentation – Data Model
A deep dive into how Python handles objects, mutability, and memory management. - Deep and Shallow Copies in Python – GeeksforGeeks
Learn how copying mutable objects works and why shallow copies can sometimes cause unexpected behavior. - Python Memory Management – Towards Data Science
A detailed look at how Python handles memory allocation for different data types and how mutability plays a role.
These resources will help you understand mutability in Python at a deeper level and avoid common mistakes in your code!
Leave a Reply