Introduction to Python Attributes and Methods
When writing Python code, you’ll often hear the terms attributes and methods. These might sound technical, but they are the building blocks that make your code work smoothly. Understanding how Python attributes and methods function is key to writing code that is clean, efficient, and easy to maintain.
Attributes are like the characteristics or properties of an object in your code. For example, if you’re coding a program about cars, each car might have attributes like color, make, and model. They help define what each object looks like or how it behaves.
On the other hand, methods are actions that an object can perform. Going back to the car example, methods could include actions like starting the engine, driving, or honking the horn. Methods are functions tied to objects that allow them to do things.
By mastering how to use Python attributes and methods, you’ll write better-organized and more readable code. It’s one of those skills that can really make a difference, especially when your projects grow in size and complexity.
What Are Attributes in Python?
In Python Object-Oriented Programming, attributes are basically the data or variables attached to an object. Think of them as the qualities or characteristics that an object holds. Every object in Python is created from a blueprint called a class, and these attributes help define what makes each object unique (or sometimes, what makes them similar).
To make it clearer, let’s compare it to filling out a form. Each person who fills out a form puts down their own name and age, right? These fields are the attributes that belong to each person. In programming, objects can have their own versions of such data, which are known as attributes.
Instance Attributes vs. Class Attributes
When talking about attributes in Python, there are two important types you’ll work with: instance attributes and class attributes. Let’s break them down!
Instance Attributes
Instance attributes are like personal data for each object. Imagine you’re creating a class to represent a person. Now, every person (or object) will have a different name and age. This is where instance attributes come in—each object you create will have its own version of these details.
Here’s a quick example:
class Person:
def __init__(self, name, age):
# These are instance attributes
self.name = name
self.age = age
# Creating different person objects
person1 = Person("Alice", 28)
person2 = Person("Bob", 34)
# Accessing their attributes
print(person1.name) # Output: Alice
print(person2.age) # Output: 34
In this example, both person1
and person2
are created from the Person
class, but they each have their own name
and age
attributes. Alice is 28 and Bob is 34, which shows how instance attributes are specific to each individual object.
Class Attributes
Now, class attributes are different. These attributes are shared across all instances (or objects) of the class. It’s like something all objects have in common. Let’s say every person is a human, regardless of their name or age. That common trait can be stored as a class attribute.
Here’s how that looks in Python:
class Person:
# This is a class attribute, shared by all instances
species = "Homo sapiens"
def __init__(self, name, age):
self.name = name
self.age = age
# Checking the class attribute for different objects
print(person1.species) # Output: Homo sapiens
print(person2.species) # Output: Homo sapiens
Both person1
and person2
are humans, so their species
is the same. However, their names and ages are still unique to each of them. This is the perfect example of using both class attributes and instance attributes together in Python.
Visualizing the Difference – Python Attributes and Methods
Here’s a visual to help make it even clearer:
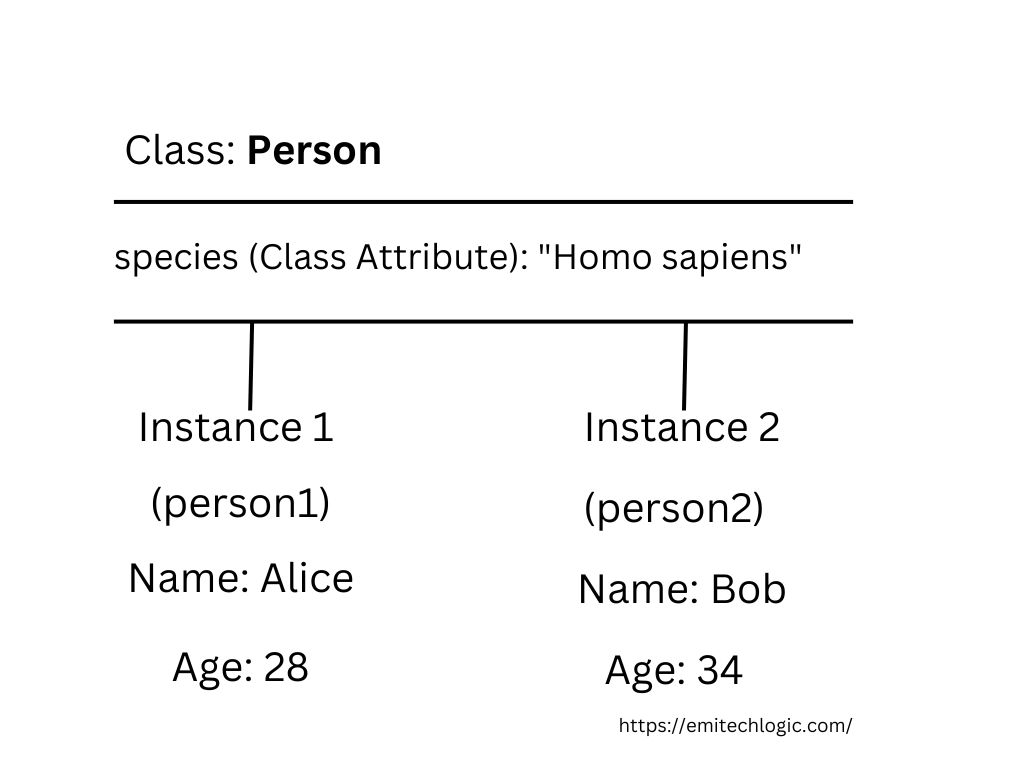
What Are Methods in Python?
In Python object-oriented programming, methods are functions that belong to an object and can perform actions on that object’s data. Simply put, methods are like commands you can give to objects—they can change or manipulate the data stored in those objects or perform operations based on that data. If you’re learning about Python attributes and methods, understanding how methods work is key to mastering how objects interact with data in Python.
Methods: Functions Attached to Objects
While a regular function in Python can exist independently, a method is always attached to an object and can access that object’s attributes (the data). So, if we have a Person
object with attributes like name
and age
, we might have methods that allow us to modify or use those attributes, like saying hello with the person’s name.
Here’s a simple example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
# Instance method
def say_hello(self):
return f"Hello, my name is {self.name}!"
# Creating an object
person1 = Person("Alice", 28)
# Calling the method
print(person1.say_hello()) # Output: Hello, my name is Alice!
In this example, say_hello
is a method because it’s a function defined inside a class and it can access the object’s name
attribute. When you call this method on person1
, it returns a message using Alice’s name.
Instance Methods vs. Class Methods – Python Attributes and Methods
There are different types of methods in Python, and it’s important to understand the difference between instance methods and class methods when learning about Python attributes and methods.
Instance Methods
An instance method is a method that belongs to an object (or an instance of a class). When you create a method inside a class, it automatically becomes an instance method unless otherwise specified. Instance methods have access to both the attributes and other methods of the object. You’ll use them a lot when building your programs, as they’re designed to manipulate or use the data specific to that object.
Here’s a deeper example:
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
self.speed = 0 # Default speed
# Instance method to start the car
def start(self):
return f"The {self.make} {self.model} is starting."
# Instance method to accelerate the car
def accelerate(self, increase):
self.speed += increase
return f"The car is now going {self.speed} mph."
# Creating an object
my_car = Car("Toyota", "Camry")
# Calling instance methods
print(my_car.start()) # Output: The Toyota Camry is starting.
print(my_car.accelerate(30)) # Output: The car is now going 30 mph.
In this code, start
and accelerate
are instance methods. They interact with the specific object my_car
, which is a Toyota Camry. Notice how the accelerate
method changes the speed
attribute of the car—this is how methods can manipulate object data.
Class Methods
Now, class methods are a little different. They are methods that belong to the class itself, not just an individual object. These methods don’t have access to instance-specific data but instead work with data that’s relevant to the class as a whole. You declare a class method using the @classmethod
decorator.
Here’s an example:
class Car:
car_count = 0 # Class attribute
def __init__(self, make, model):
self.make = make
self.model = model
Car.car_count += 1 # Increase car count when a new car is created
# Class method to get the total number of cars created
@classmethod
def get_car_count(cls):
return f"Total cars created: {cls.car_count}"
# Creating objects
car1 = Car("Toyota", "Camry")
car2 = Car("Honda", "Civic")
# Calling class method
print(Car.get_car_count()) # Output: Total cars created: 2
In this example, the get_car_count
method is a class method, and it keeps track of how many cars have been created. Notice that the class method is called on the class itself (Car.get_car_count()
), not on an individual object.
Diagram of Methods in Action – Python Attributes and Methods
Let’s break down how methods interact with objects visually:
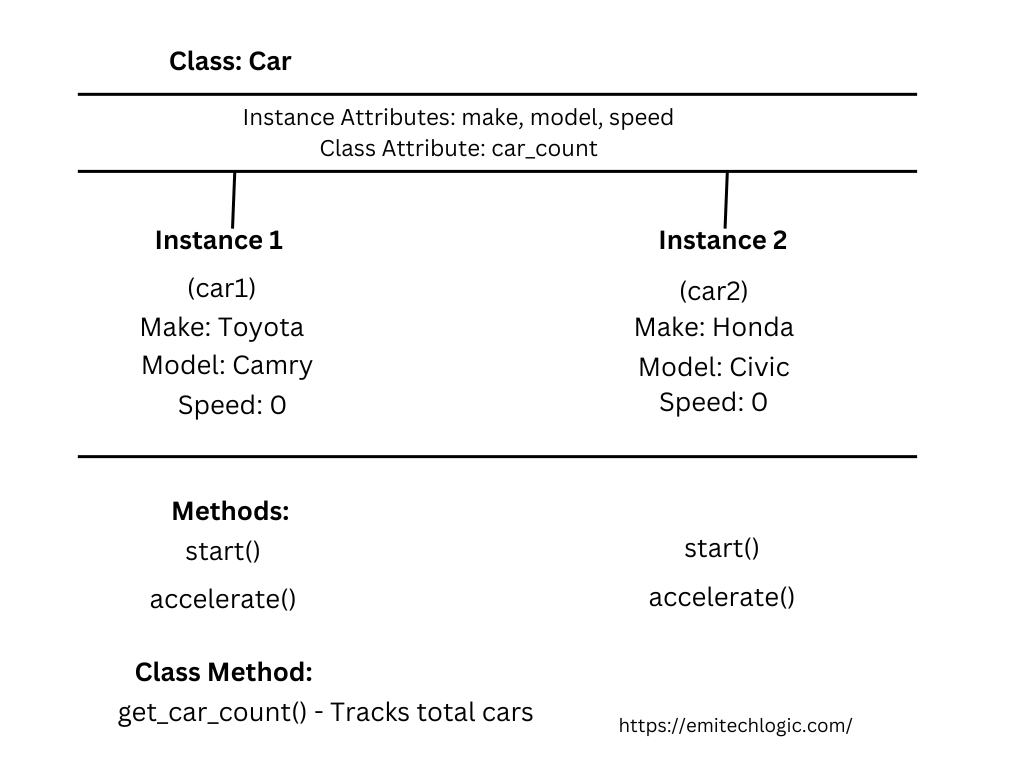
Why Python Attributes and Methods Are Key to Cleaner Code
Attributes and methods aren’t just fancy terms used in object-oriented programming; they play a critical role in keeping your code organized, readable, and easy to maintain. Python attributes and methods helps us build systems where data and actions stay neatly contained within objects. Let’s explore why these are vital to writing cleaner code and how they contribute to better code organization through encapsulation.
Encapsulation: Keeping Things Tidy
One of the key principles in object-oriented programming (OOP) is encapsulation, which simply means bundling the data (attributes) and the methods (functions) that operate on the data into one single entity—an object. This prevents outside interference with the internal workings of the object and ensures that everything related to an object stays in one place. Encapsulation not only leads to more organized code but also protects it from unnecessary complexity.
For instance, consider a BankAccount
class where attributes like balance
and methods like deposit
and withdraw
are encapsulated within the object. This way, only the account itself can manipulate its balance, making the logic easier to manage.
class BankAccount:
def __init__(self, account_holder, balance=0):
self.account_holder = account_holder
self.__balance = balance # Private attribute to prevent direct access
# Method to deposit money
def deposit(self, amount):
self.__balance += amount
return f"Deposit successful! New balance: ${self.__balance}"
# Method to withdraw money
def withdraw(self, amount):
if amount <= self.__balance:
self.__balance -= amount
return f"Withdrawal successful! Remaining balance: ${self.__balance}"
else:
return "Insufficient balance."
# Creating an object
account = BankAccount("John", 500)
# Using methods to manipulate the balance
print(account.deposit(200)) # Output: Deposit successful! New balance: $700
print(account.withdraw(100)) # Output: Withdrawal successful! Remaining balance: $600
In this example, the __balance
attribute is kept private, and all balance-related operations are handled by methods. This keeps everything clean and prevents any direct modification to the balance from outside the class.
Cleaner Code with Python Attributes and Methods
What makes Python attributes and methods so crucial for writing cleaner code? The answer lies in the structure they provide. Instead of scattering variables and functions all over your code, OOP lets you define everything within objects. Here’s how they help:
- Attributes hold the data related to the object, while methods define what actions can be performed on that data. Everything stays together, leading to fewer errors and confusion.
- Methods can be used to limit how attributes are accessed or modified. By encapsulating the data and only exposing what’s necessary, your code remains efficient and avoids accidental changes.
- The use of Python attributes and methods makes it easier to extend and modify your code in the future. You can add new features or change how the object behaves without affecting other parts of the program.
Object-Oriented Principles and Cleaner Code – Python Attributes and Methods
Another benefit of using attributes and methods is that it aligns with key OOP principles, making your code more modular and maintainable. Let’s break this down:
- Reusability: Since methods are attached to objects, they can be reused for multiple instances of the same class. For example, if you had a
Car
class, every car object could use the same method for starting or accelerating without having to rewrite the code. - Readability: Code written using attributes and methods is more intuitive to read. When someone looks at a class, they can quickly see the data and the actions associated with that data, which makes it easier to understand the logic.
Here’s another example to demonstrate the use of methods in organizing code:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
self.is_running = False
def start(self):
if not self.is_running:
self.is_running = True
return f"{self.make} {self.model} is starting!"
else:
return f"{self.make} {self.model} is already running."
def stop(self):
if self.is_running:
self.is_running = False
return f"{self.make} {self.model} has stopped."
else:
return f"{self.make} {self.model} is already off."
# Creating an object
my_car = Car("Tesla", "Model S", 2022)
# Starting and stopping the car using methods
print(my_car.start()) # Output: Tesla Model S is starting!
print(my_car.stop()) # Output: Tesla Model S has stopped.
Notice how methods help manage the state of the car (whether it’s running or not). Without them, you’d end up writing a bunch of procedural code, checking and modifying the state manually, which would make the program messy and prone to errors.
Diagram: How Methods Organize Code – Python Attributes and Methods
Here’s a simple diagram showing how methods help organize code:
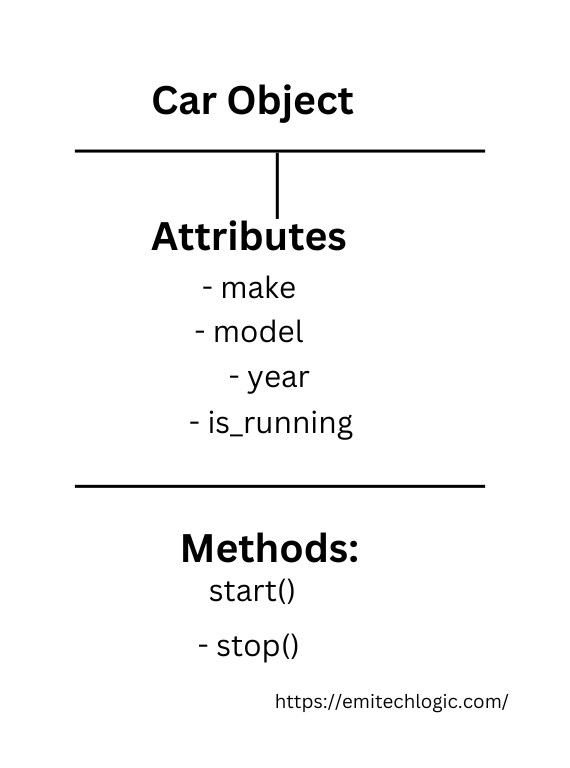
Must Read
- How to Return Multiple Values from a Function in Python
- Parameter Passing Techniques in Python: A Complete Guide
- A Complete Guide to Python Function Arguments
- How to Create and Use Functions in Python
- Find All Divisors of a Number in Python
Types of Attributes in Python
When it comes to Python attributes and methods, understanding the different types of attributes is essential for effective object-oriented programming. Attributes are the characteristics of an object, and they can be categorized into three main types: instance attributes, class attributes, and dynamic attributes. Each type has its own purpose and use cases, which will be explored in detail here.
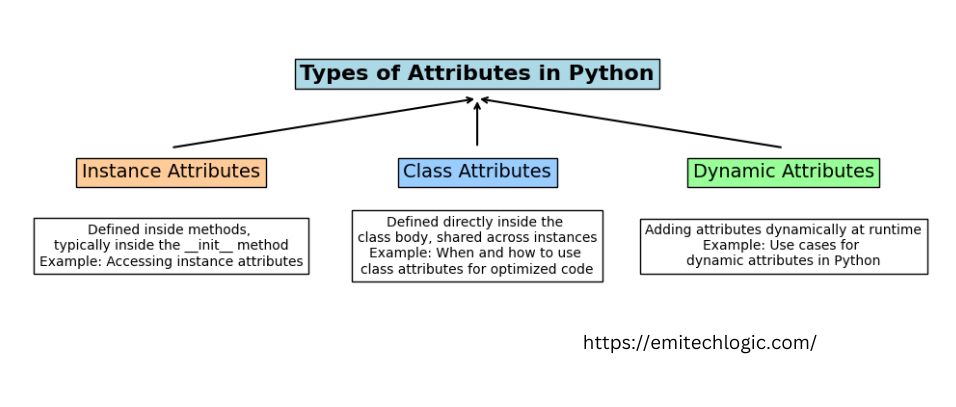
Instance Attributes
In the world of Python instance attributes in object-oriented programming, instance attributes are defined within methods, typically inside the __init__
method. This special method is called when an object is created, allowing for the initialization of attributes specific to that instance. Each object has its own set of instance attributes, making them unique to each instance.
Accessing Instance Attributes
Let’s look at an example to see how instance attributes work:
class Dog:
def __init__(self, name, age):
self.name = name # Instance attribute
self.age = age # Instance attribute
def bark(self):
return f"{self.name} says woof!"
# Creating an object
my_dog = Dog("Buddy", 3)
# Accessing instance attributes
print(my_dog.name) # Output: Buddy
print(my_dog.age) # Output: 3
print(my_dog.bark()) # Output: Buddy says woof!
In this example, name
and age
are instance attributes, unique to each Dog
object. When my_dog
is created, it has its own name
and age
, which can be accessed and modified individually. This encapsulation of data allows for cleaner code, where each dog can have its own identity.
Class Attributes
Now let’s discuss class attributes and the difference between instance and class attributes in Python. Class attributes are defined directly inside the class body and are shared across all instances of the class. This means that all objects of that class can access and modify the same class attribute, which is useful for storing information relevant to the class as a whole.
When and How to Use Class Attributes for Optimized Code – Python Attributes and Methods
Here’s an example to illustrate class attributes:
class Library:
total_books = 0 # Class attribute
def __init__(self, name):
self.name = name
Library.total_books += 1 # Increment total_books when a new library is created
@classmethod
def get_total_books(cls):
return f"Total libraries: {cls.total_books}"
# Creating Library objects
library1 = Library("City Library")
library2 = Library("County Library")
# Accessing class attribute
print(Library.total_books) # Output: 2
print(Library.get_total_books()) # Output: Total libraries: 2
In this code, total_books
is a class attribute that counts the number of Library
instances created. Whenever a new library is instantiated, the count is increased. All instances share this count, making it easy to keep track of the total number of libraries.
Dynamic Attributes – Python Attributes and Methods
Finally, let’s explore Python dynamic attributes and their uses. Dynamic attributes can be added to an object at runtime, which means that you can define new attributes on the fly. This feature provides a great deal of flexibility, allowing for objects to adapt and change as needed.
Use Cases for Dynamic Attributes in Python
Consider the following example:
class Person:
def __init__(self, name):
self.name = name
# Creating an object
john = Person("John")
# Adding a dynamic attribute
john.age = 25 # Dynamic attribute added at runtime
# Accessing the dynamic attribute
print(f"{john.name} is {john.age} years old.") # Output: John is 25 years old.
In this example, the age
attribute is added to the john
object after its creation. This flexibility can be particularly useful when working with data that may change or when building applications where attributes are not known until runtime.
Types of Methods in Python – Python Attributes and Methods
In Python’s object-oriented programming (OOP), methods play a crucial role in how objects interact with their own data or perform actions. Understanding the different types of methods can help you write more efficient and cleaner code. Let’s explore the three main types: instance methods, class methods, and static methods. These are essential concepts when working with Python attributes and methods.
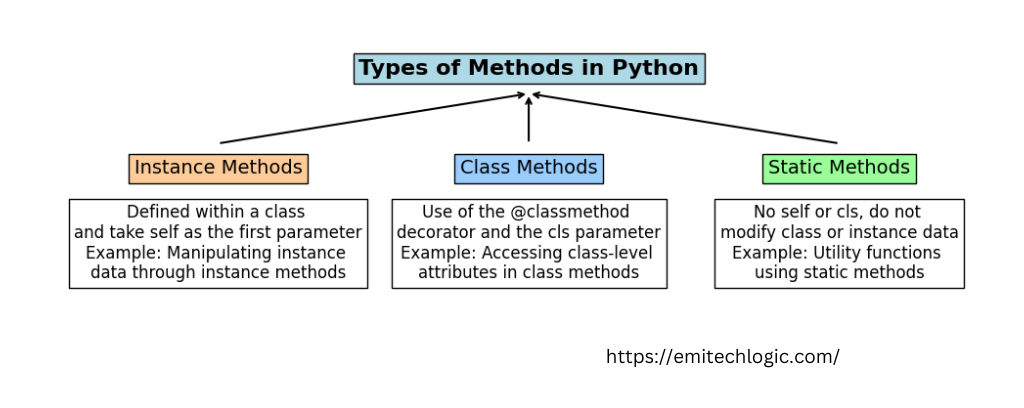
Instance Methods
The role of instance methods in Python is central to OOP because they allow objects to interact with their internal state. These methods are defined within a class and take self
as the first parameter. This parameter represents the instance of the class itself, giving access to the object’s data (or attributes). Essentially, instance methods manipulate or use the instance-specific data.
Manipulating Instance Data through Instance Methods
Let’s break down an example to see how instance methods work:
class Car:
def __init__(self, make, model, year):
self.make = make # Instance attribute
self.model = model # Instance attribute
self.year = year # Instance attribute
def start(self):
return f"The {self.year} {self.make} {self.model} is starting."
def update_model(self, new_model):
self.model = new_model # Manipulating instance data
return f"Updated model to {self.model}"
# Creating an instance of Car
my_car = Car("Toyota", "Camry", 2020)
# Calling instance methods
print(my_car.start()) # Output: The 2020 Toyota Camry is starting.
print(my_car.update_model("Corolla")) # Output: Updated model to Corolla
In this example, start()
and update_model()
are instance methods. The method update_model
manipulates the instance attribute model
, while start()
simply reads the data and returns a string.
Class Methods
Next, let’s talk about Python class methods and their significance. These methods use the @classmethod
decorator and take cls
as the first parameter, which refers to the class itself (rather than an instance). Class methods are often used when you need to work with class-level data, like modifying or accessing attributes that are shared across all instances of the class.
Accessing Class-Level Attributes in Class Methods – Python Attributes and Methods
Here’s a quick example to demonstrate class methods:
class Employee:
employee_count = 0 # Class attribute
def __init__(self, name, position):
self.name = name
self.position = position
Employee.employee_count += 1 # Modify class attribute in constructor
@classmethod
def total_employees(cls):
return f"Total employees: {cls.employee_count}"
# Creating instances
emp1 = Employee("Alice", "Engineer")
emp2 = Employee("Bob", "Designer")
# Accessing class method
print(Employee.total_employees()) # Output: Total employees: 2
In this example, the total_employees()
method is a class method. It works with the class-level attribute employee_count
, which tracks the number of employees. All instances of the Employee
class share this attribute, making class methods perfect for managing class-wide data.
Static Methods
Lastly, let’s explore static methods. These are a bit different from instance and class methods because they don’t take self
or cls
as parameters. Static methods do not modify class-level or instance-level data. Instead, they behave like regular functions but live within the class’s namespace. They’re ideal for utility functions that logically belong to the class but don’t need to modify any class or instance attributes.
What Are Static Methods in Python and When to Use Them?
Let’s look at how static methods can be used in Python:
class Calculator:
@staticmethod
def add(a, b):
return a + b
@staticmethod
def multiply(a, b):
return a * b
# Using static methods
print(Calculator.add(5, 3)) # Output: 8
print(Calculator.multiply(4, 7)) # Output: 28
In this example, add()
and multiply()
are static methods. These methods don’t interact with any instance or class data—they just perform simple mathematical operations. This makes static methods useful for utility functions that belong to a class logically but don’t require any data from the class or its instances.
Using Python Attributes and Methods Together for Cleaner Code
One of the most powerful aspects of Python’s object-oriented programming (OOP) is how attributes and methods work together. This combination helps create code that’s clean, easy to understand, and scalable. By following OOP principles such as encapsulation, abstraction, and reusability, we can maintain cleaner code and reduce the likelihood of errors. In this section, we’ll explore how these principles come together with Python attributes and methods.
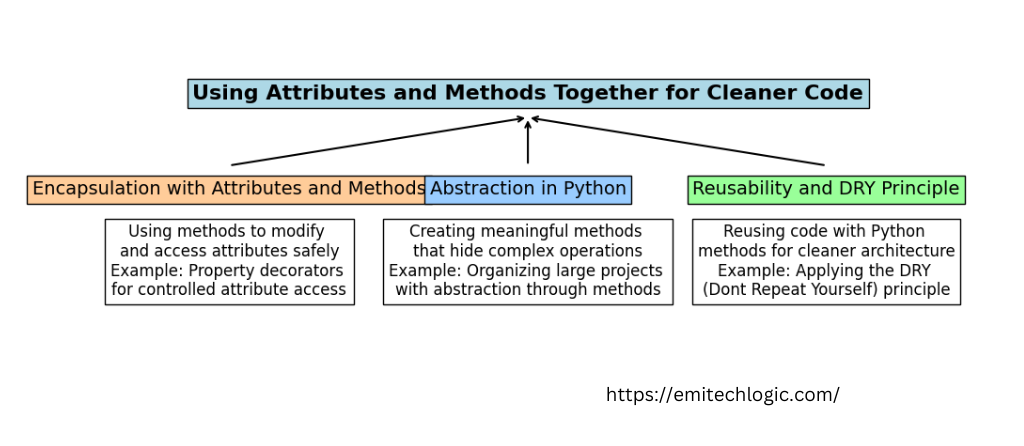
Encapsulation with Attributes and Methods
Encapsulation is a key concept in OOP that helps protect the internal state of an object. By using methods to safely modify and access attributes, Python allows for more controlled code execution and reduces unexpected changes to data. Encapsulation ensures that internal object data is hidden and can only be accessed or modified through well-defined methods, which prevents direct manipulation of attributes.
Property Decorators for Controlled Attribute Access
A common way to enforce encapsulation in Python is through the use of property decorators. This approach allows you to control access to an attribute by defining getter, setter, and deleter methods. Instead of directly accessing an attribute, you can wrap it in a method that defines how it should be accessed or modified.
Let’s take a look at an example:
class Person:
def __init__(self, name, age):
self._name = name # Private attribute
self._age = age # Private attribute
@property
def age(self):
return self._age
@age.setter
def age(self, value):
if value < 0:
raise ValueError("Age cannot be negative")
self._age = value
# Creating an instance of Person
p = Person("John", 30)
# Accessing the age using the getter method
print(p.age) # Output: 30
# Setting the age using the setter method
p.age = 35
print(p.age) # Output: 35
# Trying to set a negative age will raise an error
# p.age = -5 # Raises ValueError: Age cannot be negative
In this example, we use a getter and setter for the age
attribute. This ensures that Python encapsulation principles are followed, and the age can only be set to valid values.
Abstraction in Python: Hiding Complexity with Methods – Python Attributes and Methods
Abstraction is all about hiding unnecessary details and showing only the essential features of an object. This makes the code more readable and maintainable. By creating meaningful methods, you can hide complex operations inside those methods, so the user of the class doesn’t need to know what’s happening behind the scenes.
How Abstraction Leads to Maintainable Code
Let’s say you have a complex operation, like calculating taxes based on income, deductions, and other factors. Instead of exposing the entire calculation to other parts of the code, you can abstract it into a method that provides a clear and simple interface.
Here’s an example:
class TaxCalculator:
def __init__(self, income):
self.income = income
def calculate_tax(self):
return self._apply_deductions(self.income) * 0.25
def _apply_deductions(self, amount):
# Complex deduction logic hidden from the user
return amount - (amount * 0.1)
# Using the TaxCalculator class
tax_calculator = TaxCalculator(50000)
print(tax_calculator.calculate_tax()) # Output: 11250.0
In this example, the calculate_tax()
method abstracts away the complexity of applying deductions and calculating tax. The user doesn’t need to understand the details of how deductions work—they just call the method and get the result. This makes the code much easier to manage, especially in larger projects.
Reusability and the DRY Principle with Methods – Python Attributes and Methods
One of the golden rules of programming is DRY: Don’t Repeat Yourself. By organizing code into reusable methods, you avoid duplicating logic in multiple places, which can lead to errors and inconsistency. With Python methods, reusing code becomes easy and helps in creating a more clean architecture.
Reusing Methods Across Code to Avoid Repetition
Let’s say you need to perform a calculation across different parts of your program. Instead of rewriting the same code in multiple places, you can define a method once and use it wherever needed. This improves both readability and maintainability.
Here’s an example:
class MathOperations:
@staticmethod
def multiply(a, b):
return a * b
# Reusing the multiply method across different parts of the code
result1 = MathOperations.multiply(10, 5) # Output: 50
result2 = MathOperations.multiply(7, 3) # Output: 21
By using a method like multiply()
, you avoid repeating the same logic in different places. This is a classic example of applying the DRY principle in Python.
Advanced Techniques with Attributes and Methods – Python Attributes and Methods
In Python, working with attributes and methods can get more sophisticated as you dig deeper into advanced techniques. These techniques help developers write better, more secure, and flexible code. In this section, we’ll explore private and protected attributes, magic methods, and the use of properties. Additionally, we’ll cover the latest advancements in Python attributes and methods that you can apply to modern projects.
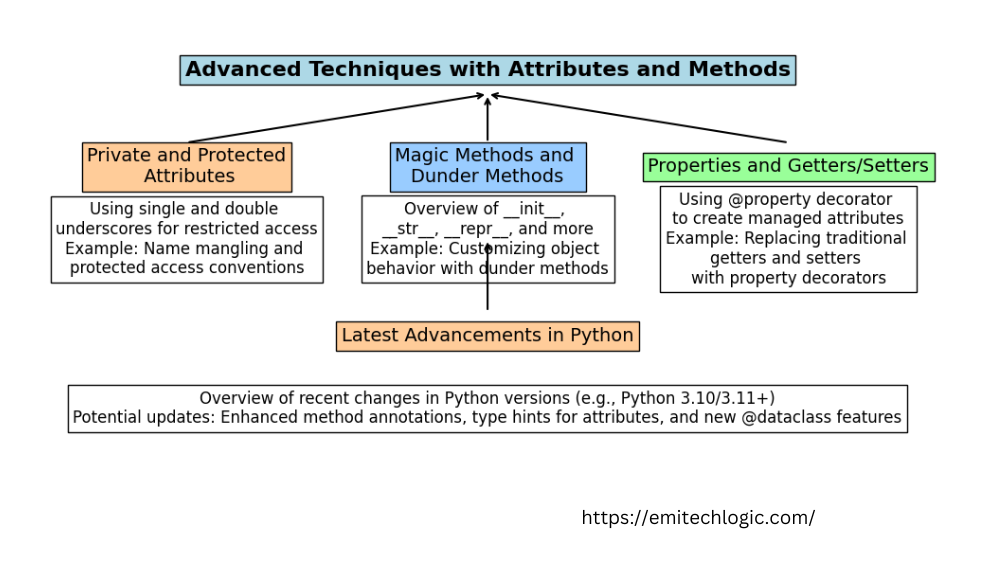
Private and Protected Attributes
In Python, private and protected attributes help manage access to an object’s internal state. While Python doesn’t enforce strict access controls like other languages (e.g., Java or C++), there are conventions that developers follow to indicate whether an attribute is meant to be private or protected.
Best Practices for Using Private and Protected Attributes in Python
- Protected attributes: A single underscore (
_
) before an attribute name indicates it’s meant to be protected, which means it shouldn’t be accessed directly from outside the class. However, it can still be accessed if necessary, as Python follows the philosophy of “we are all consenting adults here.” - Private attributes: A double underscore (
__
) before the attribute name triggers name mangling, which makes the attribute harder to access from outside the class. This is Python’s way of suggesting that you shouldn’t touch these attributes unless you know what you’re doing.
Here’s an example that demonstrates both private and protected attributes:
class MyClass:
def __init__(self, name, age):
self._name = name # Protected attribute
self.__age = age # Private attribute
def get_age(self):
return self.__age
obj = MyClass("Alice", 30)
print(obj._name) # Accessing protected attribute (allowed but not recommended)
# print(obj.__age) # Raises AttributeError due to name mangling
# Accessing private attribute through name mangling (not recommended)
print(obj._MyClass__age) # Output: 30
In this example, __age
is a private attribute, and it can only be accessed using name mangling (_MyClass__age
). Best practices suggest avoiding direct access to these attributes and using getter or setter methods when needed.
Magic Methods and Dunder Methods in Python
Magic methods, also known as dunder methods (because they start and end with double underscores), allow you to define the behavior of your custom objects when interacting with Python’s built-in operators and functions. These methods include __init__
, __str__
, __repr__
, and many more. Python magic methods are incredibly powerful tools for customizing object behavior.
Overview of __init__
, __str__
, __repr__
, and More
__init__
: This method is called when you create a new instance of a class. It initializes the object’s attributes.__str__
: Returns a user-friendly string representation of the object, which is used when printing the object.__repr__
: Returns an “official” string representation of the object that is often useful for debugging.
Here’s an example demonstrating some common magic methods:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f"Person(name={self.name}, age={self.age})"
def __repr__(self):
return f"Person({self.name!r}, {self.age!r})"
# Creating an instance of Person
p = Person("John", 25)
# Using the __str__ method
print(str(p)) # Output: Person(name=John, age=25)
# Using the __repr__ method (used for debugging)
print(repr(p)) # Output: Person('John', 25)
In this example, __str__
provides a human-readable output, while __repr__
gives a more detailed view that’s helpful during debugging.
Properties and Getters/Setters – Python Attributes and Methods
Traditionally, many programming languages use getters and setters to control how an attribute is accessed or modified. Python, however, offers a more elegant approach using properties and the @property
decorator. This technique allows you to replace traditional getters and setters with something cleaner and more Pythonic.
Implementing Python Properties with Getters and Setters – Python Attributes and Methods
Here’s a comparison between traditional getters and setters and the property decorator:
class Rectangle:
def __init__(self, width, height):
self._width = width
self._height = height
# Traditional getter and setter methods
def get_width(self):
return self._width
def set_width(self, value):
if value <= 0:
raise ValueError("Width must be positive.")
self._width = value
# Using property decorator
@property
def width(self):
return self._width
@width.setter
def width(self, value):
if value <= 0:
raise ValueError("Width must be positive.")
self._width = value
rect = Rectangle(10, 5)
rect.width = 15 # Using the property setter
print(rect.width) # Output: 15
Using the @property
decorator simplifies the syntax for accessing attributes while still allowing for validation, making the code cleaner and more intuitive.
Latest Advancements in Python Attributes and Methods
As Python evolves, new features are added that improve how we work with attributes and methods. Some of the latest enhancements in Python 3.10 and beyond include method annotations, type hints for attributes, and improvements to dataclasses.
Latest Features in Python Related to Attributes and Methods
- Enhanced method annotations: With Python 3.10+, method annotations are more flexible, allowing for complex type hints. This is especially useful when you need to ensure that attributes and method return types are correct.
- Type hints for attributes: Python’s support for type hints has made codebases more maintainable by explicitly stating the expected types of attributes and method parameters.
- New features in @dataclass: The
@dataclass
decorator, introduced in Python 3.7, continues to get improvements. It now offers features like frozen dataclasses (for immutability) and better support for default values, making it easier to manage attribute-rich classes without having to write boilerplate code.
Here’s an example using type hints and dataclasses:
from dataclasses import dataclass
@dataclass
class Employee:
name: str
age: int
position: str
def promote(self):
self.position = "Manager"
emp = Employee("Alice", 30, "Developer")
print(emp)
In this example, the @dataclass
decorator automatically generates the __init__
, __repr__
, and __eq__
methods, saving you from writing redundant code. Using type hints also makes the code more readable and self-documenting.
Best Practices for Writing Clean Python Code with Attributes and Methods
Writing clean, maintainable code in Python is essential for both small and large projects. By carefully structuring your attributes and methods, you can make your code easier to read, modify, and extend. This section will explore best practices for organizing Python code, reducing redundancy, and avoiding common pitfalls when working with Python attributes and methods.
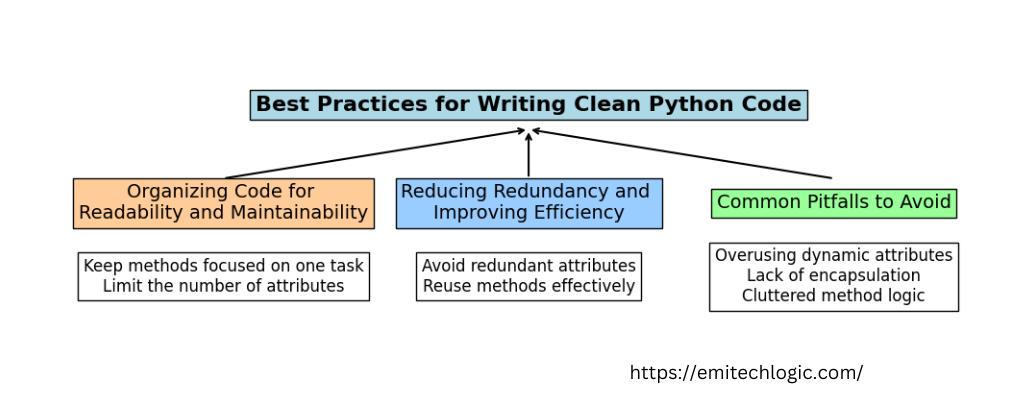
Organizing Code for Readability and Maintainability
The key to maintaining readable and clean Python code is organizing your classes, attributes, and methods thoughtfully. By ensuring that each method and attribute serves a specific purpose, you can avoid bloating your code with unnecessary complexity. Here are some practical tips for structuring your Python classes.
Tips for Structuring Python Classes with Attributes and Methods
- Limit the Number of Attributes: Having too many attributes can make your class difficult to manage. It’s best to define only the attributes that are necessary for the object’s state. Extra attributes can often lead to confusion or bugs down the line.
- Keep Methods Focused on One Task: Each method should do one thing and do it well. If you find that a method is trying to handle too many tasks, it’s often a good idea to break it into smaller, more focused methods.
- Use Meaningful Method and Attribute Names: Naming conventions are crucial for clarity. Descriptive names for methods and attributes help others understand what your code does without needing to dive into the implementation details.
Here’s an example of how to structure a simple class for better readability:
class Book:
def __init__(self, title, author, pages):
self.title = title
self.author = author
self.pages = pages
def read(self):
return f"Reading '{self.title}' by {self.author}"
def bookmark(self, page):
if page > self.pages:
return f"Page {page} exceeds the total number of pages in the book."
return f"Bookmarked page {page} in '{self.title}'"
In this example, the class Book
has only the attributes necessary for its function (title
, author
, and pages
). The methods read()
and bookmark()
are focused on their individual tasks, making the class more readable and maintainable.
Reducing Redundancy and Improving Efficiency
Redundancy in code is a common issue that makes maintaining and debugging more challenging. Writing clean, efficient code often comes down to avoiding redundant attributes and reusing methods where possible. Following the DRY principle (Don’t Repeat Yourself) is essential to keeping your code efficient.
Python Coding Best Practices for Efficient Attribute and Method Usage
- Reuse Methods Across the Class: If a certain piece of logic is used in multiple methods, refactor it into a separate method that can be reused. This eliminates code duplication and keeps your methods focused on specific tasks.
- Avoid Redundant Attributes: Be mindful of defining attributes that essentially represent the same data or that can be calculated dynamically. Having too many attributes to store similar or derived data can lead to inconsistencies and make the class harder to maintain.
Here’s an example demonstrating reusing methods and avoiding redundant attributes:
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
def perimeter(self):
return 2 * (self.width + self.height)
In this example, the Rectangle
class doesn’t store attributes like area
or perimeter
. Instead, these values are calculated dynamically using methods, reducing redundancy and ensuring the class is more efficient.
Common Pitfalls to Avoid
When working with Python attributes and methods, there are common mistakes that developers often make, especially when dealing with larger projects. By avoiding these pitfalls, you can ensure that your code remains clean, maintainable, and efficient.
Mistakes to Avoid When Working with Attributes and Methods in Python
- Overusing Dynamic Attributes: While Python allows you to add attributes dynamically at runtime, overusing this feature can lead to code that’s hard to follow and debug. Dynamic attributes can create confusion if they are not managed carefully.
- Lack of Encapsulation: Failing to encapsulate data properly by allowing attributes to be accessed or modified directly from outside the class can lead to bugs. Use property decorators or methods to control how attributes are accessed or modified.
- Cluttered Method Logic: Methods that try to do too much can be hard to test and maintain. Keep your methods focused on a single task and split complex operations into smaller, manageable pieces.
Here’s an example of poor practice that leads to messy code:
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def update_car(self, make=None, model=None):
if make:
self.make = make
if model:
self.model = model
return f"Updated to {self.make} {self.model}"
# Adding dynamic attributes on the fly
my_car = Car("Toyota", "Camry")
my_car.year = 2020 # Dynamic attribute
In this example, the update_car()
method tries to do too much by updating multiple attributes, and the use of a dynamic attribute (year
) creates a hidden dependency that could lead to confusion later.
Python Libraries and Tools That Enhance Attribute and Method Management
When working with Python, managing attributes and methods efficiently is crucial for writing clean, maintainable code. This article will explore some of the most helpful libraries and tools available for this purpose, making it easier to define attributes and optimize method performance.
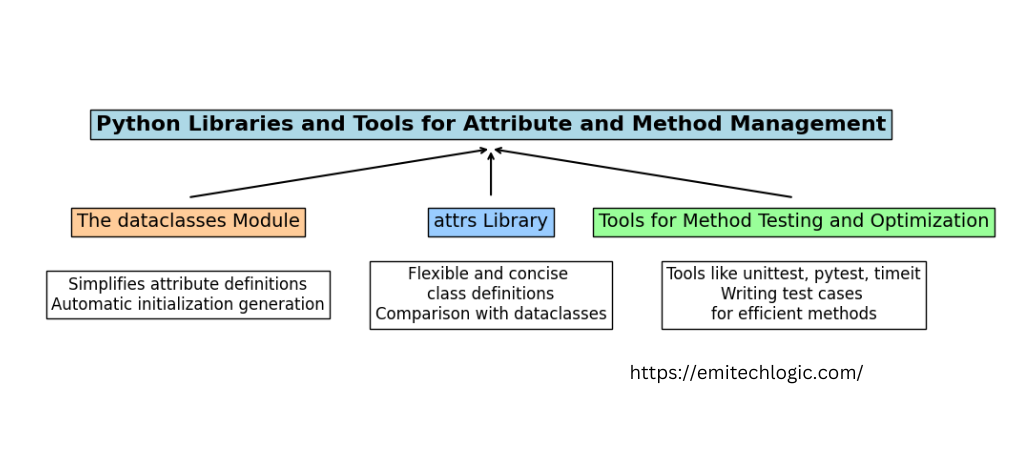
The dataclasses
Module
Cleaner Code with Attributes
One of the standout features in Python is the dataclasses
module, introduced in Python 3.7. This module simplifies the process of defining classes by automatically generating special methods, such as __init__()
, __repr__()
, and __eq__()
, based on class attributes. This not only reduces boilerplate code but also enhances readability, making your code cleaner and more intuitive.
Example: Automatic Attribute Generation
Let’s take a look at how the dataclasses
module can make attribute management easier. Consider a simple scenario where you need to represent a Book
with attributes like title and author:
from dataclasses import dataclass
@dataclass
class Book:
title: str
author: str
year: int
# Creating an instance of Book
my_book = Book(title="1984", author="George Orwell", year=1949)
print(my_book)
In this example, the @dataclass
decorator automatically creates the __init__()
method for you, allowing for straightforward instantiation of Book
objects. This approach significantly reduces the amount of code you have to write and keeps your class definitions concise.
The attrs
Library
Smarter Attribute Handling
For those looking for an alternative to the built-in dataclasses
, the attrs
library provides an even more flexible and concise way to define classes with attributes. This library is particularly useful when additional customization is required.
Example: Comparing attrs
vs. dataclass
Here’s a comparison between using attrs
and dataclasses
to illustrate their differences. First, let’s see how we can define the same Book
class using the attrs
library:
import attr
@attr.s
class Book:
title: str = attr.ib()
author: str = attr.ib()
year: int = attr.ib()
# Creating an instance of Book
my_book = Book(title="Brave New World", author="Aldous Huxley", year=1932)
print(my_book)
Both attrs
and dataclasses
make attribute management easier, but attrs
offers additional features like validators and converters, which can be incredibly beneficial in certain scenarios. For instance, you could easily add a validation step to ensure the year is a positive integer, making your code not just cleaner but also more robust.
Tools for Method Testing and Optimization
Ensuring Method Efficiency
No matter how well attributes are managed, the methods that operate on these attributes must also be efficient and reliable. Python offers several powerful tools to assist with method testing and optimization. Key tools include unittest
, pytest
, and timeit
.
Example: Writing Test Cases
Using unittest
, for example, allows you to define test cases that verify your methods work as expected. Here’s a simple illustration of how to set up tests for a method that calculates the age of a book based on its publication year:
import unittest
class TestBookMethods(unittest.TestCase):
def test_age(self):
my_book = Book(title="1984", author="George Orwell", year=1949)
self.assertEqual(my_book.age(), 75) # Assuming the current year is 2024
if __name__ == "__main__":
unittest.main()
By writing test cases, potential errors can be caught early, preventing bugs from reaching production. This testing ensures that your methods are reliable, giving you confidence in your code.
Optimizing Performance
To further enhance method efficiency, tools like timeit
can be employed. This tool measures the execution time of small code snippets, allowing for performance comparisons between different implementations of a method.
import timeit
# Example method to be tested
def calculate_age(year):
return 2024 - year
# Timing the method
execution_time = timeit.timeit('calculate_age(1949)', globals=globals(), number=1000)
print(f"Execution time: {execution_time} seconds")
Conclusion: Embracing Python Attributes and Methods for Cleaner Code
In this discussion on Python attributes and methods, we explored their crucial roles in crafting cleaner, more efficient code. By understanding attributes—variables tied to objects—and methods—functions that manipulate this data—you can leverage object-oriented programming effectively.
We highlighted the importance of differentiating between instance attributes, class attributes, and dynamic attributes, which enhance code organization and encapsulation. Similarly, recognizing the various types of methods allows for better code management and reusability.
We also examined advanced techniques like private and protected attributes, magic methods, and properties that further promote clean coding practices. Libraries such as dataclasses and attrs simplify attribute management, while testing tools like unittest and pytest ensure method reliability.
Ultimately, adopting best practices—such as reducing redundancy and avoiding common pitfalls—will lead to more maintainable code. By embracing these principles, you can enhance your coding skills and tackle complex projects with confidence.
External Resources
Python Documentation on Classes
Python Classes
This official Python documentation provides a comprehensive overview of classes, attributes, and methods, along with examples to help you understand the fundamentals.
PEP 557 – Data Classes
PEP 557
This Python Enhancement Proposal introduces data classes, explaining their purpose and implementation, which can greatly simplify attribute management in your classes.
FAQs on Python Attributes and Methods
Instance attributes are defined within a class and are unique to each instance of that class. They are typically initialized in the __init__
method. In contrast, class attributes are shared among all instances of a class and are defined directly within the class body. Class attributes can be accessed using the class name or any instance of the class.
To define a method in Python, you create a function inside a class. The first parameter should typically be self
, which refers to the instance of the class. Here’s a simple example:
class MyClass:
def my_method(self):
print(“Hello from my method!”)
Private attributes should be used when you want to restrict access to certain data within a class. By prefixing an attribute name with a single underscore (e.g., _attribute
) or double underscore (e.g., __attribute
), you signal that these attributes are intended for internal use only and should not be accessed directly from outside the class.
Yes, properties in Python provide a more elegant way to define managed attributes, allowing you to use dot notation to access attributes while still controlling their access and modification. Using the @property
decorator, you can create getter methods, and @attribute_name.setter
for setter methods, simplifying the syntax compared to traditional getters and setters.
@staticmethod
decorator? The @staticmethod
decorator is used to define a method that does not require access to the instance (self
) or class (cls
). Static methods can be called on the class itself or on instances and are often used for utility functions that perform a task in isolation from the class state. This helps keep your code organized and enhances readability.
Leave a Reply