Introduction
Welcome to The Ultimate Guide to Python Lists! Whether you’re new to Python or looking to brush up on your skills, you’ve come to the right place. Python lists are one of the most powerful and flexible tools you can have in your programming toolkit. They make it easy to store and manage collections of data, from simple numbers to complex objects.
In this guide, we’re going to break down everything you need to know about Python lists. We’ll start with the basics, like how to create a list and access its elements, and then explore the more advanced topics like list comprehensions, slicing, and sorting. Along the way, I’ll share tips, examples, and best practices that will help you write cleaner, more efficient code.
By the end of this post, you’ll not only understand how to use Python lists but also how to use their full potential in your projects. Whether you’re working on data analysis, web development, or just playing around with code, mastering lists will make your life easier and your programs more powerful.
What Are Python Lists?
When you first start learning Python, one of the most useful and flexible tools you’ll come across is the Python list. Think of a list as a container that can hold multiple items, like numbers, strings, or even other lists. What makes Python lists special is their ability to store items of different types and their flexibility in allowing you to modify, add, or remove elements as needed.
Understanding Python Lists
A Python list is an ordered collection, which means the items within the list maintain the order in which they were added. This characteristic allows you to access elements by their position, or index, starting from zero for the first item. Lists are created by placing items inside square brackets, separated by commas.
For example:
my_list = [1, "apple", 3.14, True]
Here, my_list
contains four elements: an integer, a string, a float, and a boolean. This mix of data types showcases how flexible lists can be.
Another thing to note is that lists can be nested. That means you can have a list within a list:
nested_list = [1, 2, [3, 4], 5]
In this example, the third element of nested_list
is another list.
Key Features of Python Lists
1. Mutability: Unlike strings or tuples, Python lists are mutable, which means you can change the contents after the list has been created. For instance, you can update an item, add new elements, or remove existing ones.
Example:
my_list = [1, 2, 3]
my_list[1] = "changed"
print(my_list) # Output: [1, 'changed', 3]
2. Dynamic Size: Lists in Python are dynamic. You don’t need to declare a fixed size when you create a list, and it can grow or shrink as you add or remove items.
Example:
my_list = [1, 2, 3]
my_list.append(4)
print(my_list) # Output: [1, 2, 3, 4]
3. Indexing and Slicing: Since lists are ordered, you can access individual items by their index, or get a subset of the list using slicing.
Example:
my_list = [10, 20, 30, 40, 50]
print(my_list[2]) # Output: 30
print(my_list[1:4]) # Output: [20, 30, 40]
4. Heterogeneous Elements: A list can contain elements of different data types, even other lists or objects.
Example:
my_list = [1, "two", 3.0, [4, 5]]
5. Built-in Methods: Python lists come with several built-in methods that make working with them easier, such as append()
, extend()
, remove()
, pop()
, reverse()
, and sort()
.
Why Python Lists Are Essential for Programmers
Python lists are more than just a data structure; they’re a core part of many programming tasks. Here’s why they’re so important:
1. Flexibility: Whether you’re handling data, managing a collection of objects, or passing multiple arguments to a function, lists provide a flexible way to do so.
2. Ease of Use: Lists are intuitive and easy to work with, especially for beginners. The syntax is simple, and the operations are straightforward, making them a great tool for learning and building complex programs.
3. Performance: Lists are highly optimized for common tasks. For example, accessing an item by its index is fast because Python lists are implemented as dynamic arrays.
4. Support for Iteration: Lists are iterable, meaning you can easily loop through each element using a for
loop, which is handy for processing data, performing calculations, or generating reports.
Example:
my_list = [1, 2, 3, 4, 5]
for item in my_list:
print(item)
This loop will print each element in my_list
.
Getting Started with Python Lists
Creating Lists in Python
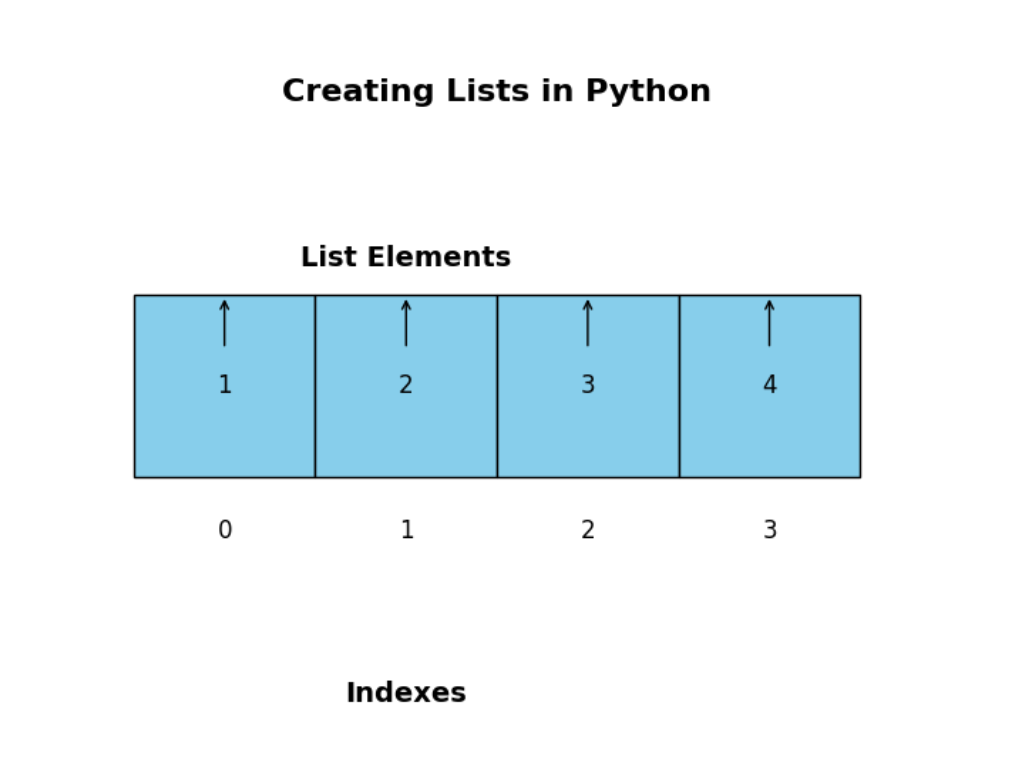
Creating a list in Python is like creating a container where you can store multiple items. These items can be of any data type, and you can change the list as your program runs. Let’s walk through the different ways you can create lists in Python.
Basic List Creation
To create a basic list, you’ll start by using square brackets []
. Inside these brackets, you can place the items you want to store in the list, separated by commas. Each item in the list is called an element. Here’s a simple example:
fruits = ["apple", "banana", "cherry"]
In this example, fruits
is a list that contains three elements: "apple"
, "banana"
, and "cherry"
. Each element in the list is a string, but lists can also store numbers, booleans, or even other lists.
Here’s another example with different data types:
mixed_list = [1, "two", 3.0, True]
This list contains an integer, a string, a float, and a boolean. Python lists are flexible enough to handle this variety without any issues.
Creating Empty Lists
Sometimes, you’ll want to create an empty list and add items to it later. An empty list is simply a list with nothing inside the brackets. Creating an empty list is straightforward and can be done like this:
empty_list = []
At this point, empty_list
doesn’t contain any elements, but it’s ready to be populated with data as needed. You might use an empty list when you’re planning to collect user inputs or data generated during the program’s execution.
Populating Lists with Data
Once you’ve created a list, you can start adding data to it. There are several ways to populate a list, depending on your needs.
1. Adding Elements Using the append()
Method: The append()
method adds a new element to the end of the list. This method is handy when you’re collecting data in a loop or adding items dynamically.
Example:
numbers = []
numbers.append(1)
numbers.append(2)
numbers.append(3)
print(numbers) # Output: [1, 2, 3]
In this example, we started with an empty list numbers
and added three elements to it using the append()
method.
2. Using the extend()
Method: The extend()
method is similar to append()
, but it’s used to add multiple elements to a list at once. This is particularly useful when you have another list or a collection of items that you want to add to an existing list.
Example:
numbers = [1, 2, 3]
more_numbers = [4, 5, 6]
numbers.extend(more_numbers)
print(numbers) # Output: [1, 2, 3, 4, 5, 6]
Here, numbers
is extended with the elements from more_numbers
, resulting in a single list containing all the elements.
3. Inserting Elements with insert()
: If you want to add an element at a specific position in the list, you can use the insert()
method. This method requires two arguments: the index where you want to insert the element and the element itself.
Example:
letters = ["a", "b", "d"]
letters.insert(2, "c")
print(letters) # Output: ['a', 'b', 'c', 'd']
In this case, "c"
is inserted at index 2, shifting the original elements to the right.
Creating and managing lists in Python is a fundamental skill that every programmer needs. Whether you’re working with a predefined set of data or collecting items dynamically, lists provide a flexible and powerful way to store and manipulate information.
List Data Types in Python
Python lists are incredibly flexible and powerful, allowing you to store a wide range of data types within a single list. This flexibility makes lists an essential tool in any Python programmer’s toolkit. Let’s explore how lists can store different data types, how to work with lists of lists (also known as nested lists), and how mixing data types in a list can be both practical and powerful.
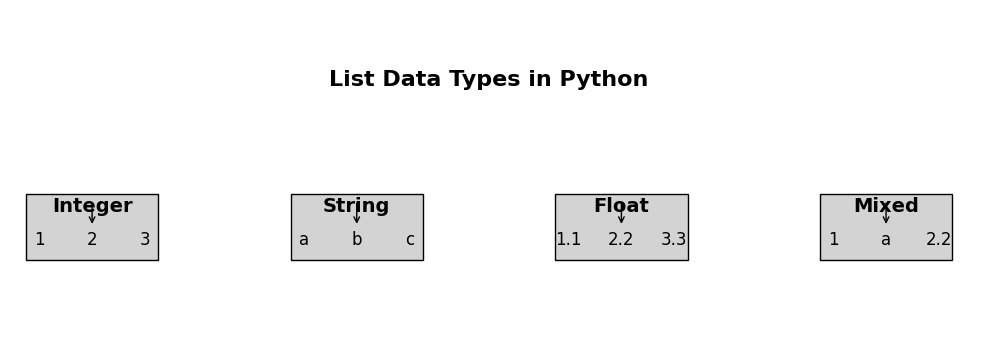
Storing Different Data Types in a List
One of the most remarkable features of Python lists is their ability to store elements of different data types. In many programming languages, arrays or lists are restricted to a single data type, but Python removes this limitation. This means you can have integers, strings, floats, booleans, and even other lists, all in one list. This flexibility allows you to group related pieces of data together, even if they are of different types.
Here’s a simple example:
mixed_list = [28, "apple", 3.14, True]
In this mixed_list
, we have an integer (28
), a string ("apple"
), a float (3.14
), and a boolean (True
). Each element is stored in the order it was added, and you can access any element by its index:
print(mixed_list[1]) # Output: apple
This feature is particularly useful when you need to store a collection of items that are related but not necessarily of the same type. For instance, if you were creating a list to store data about a book, you could have the title (a string), the number of pages (an integer), the average rating (a float), and whether it’s a bestseller (a boolean):
book_info = ["To Kill a Mockingbird", 281, 4.27, True]
This book_info
list neatly groups all the related information about the book in one place, despite the data being of different types.
Understanding Lists of Lists (Nested Lists)
A nested list (or list of lists) is exactly what it sounds like: a list that contains other lists as its elements. This structure is useful for representing more complex data, such as a table or matrix, where each sublist represents a row.
For example, let’s say you’re working on a project that requires storing data about students’ test scores. You could use a nested list where each sublist contains the scores for a different student:
students_scores = [
[85, 90, 78], # Scores for student 1
[88, 92, 80], # Scores for student 2
[84, 85, 88] # Scores for student 3
]
In this students_scores
list, each sublist represents an individual student’s scores across three tests. You can access a specific student’s scores using their index, and even access an individual score by indexing further:
print(students_scores[1]) # Output: [88, 92, 80] (scores for student 2)
print(students_scores[1][2]) # Output: 80 (third test score for student 2)
Nested lists allow you to represent and manage complex data structures within your Python programs. Whether you’re dealing with matrices in mathematics, grids in games, or tables of data, nested lists provide a simple and efficient way to organize and access your data.
Mixing Data Types in a List
Another powerful feature of Python lists is the ability to mix different data types within the same list. This can be particularly useful when you need to store diverse pieces of information that are related but differ in type.
For instance, imagine you’re creating a list to store data about a product:
product_info = ["Laptop", 999.99, 5, True]
Here’s what each element represents:
"Laptop"
is the product name (string).999.99
is the price (float).5
is the stock quantity (integer).True
indicates that the product is in stock (boolean).
Mixing data types in this way allows you to store all relevant information about a product in a single list. This approach is not only practical but also keeps your code organized and easy to manage.
Accessing and Modifying Python Lists
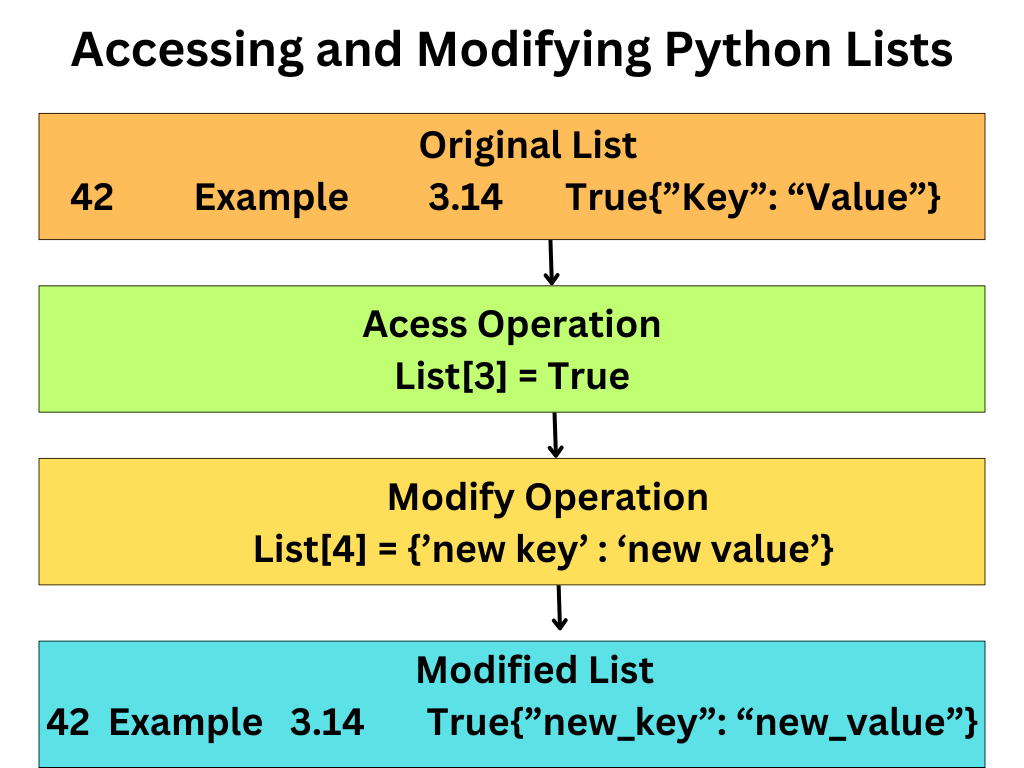
Indexing and Slicing Python Lists
Python lists are ordered collections, meaning that each element in a list has a unique position, or index. This order allows you to easily access and manipulate individual elements or subsets of the list. Let’s explore these concepts in detail.
Accessing Individual Elements
The simplest way to access an element in a Python list is by using its index. In Python, indexes start at 0, which means the first element of a list is at position 0, the second element is at position 1, and so on.
Let’s consider a list of colors:
colors = ["red", "green", "blue", "yellow", "purple"]
If you want to access the first element in this colors
list, you would do so using the index 0
:
first_color = colors[0]
print(first_color) # Output: red
Similarly, to access the third element:
third_color = colors[2]
print(third_color) # Output: blue
It’s important to note that if you try to access an index that doesn’t exist (like colors[5]
in this list), Python will raise an IndexError
.
Negative Indexing in Python Lists
Python also allows you to use negative indexing to access elements from the end of the list. This feature can be particularly handy when you want to quickly access elements without knowing the exact length of the list.
For example, if you want to access the last element in the colors
list:
last_color = colors[-1]
print(last_color) # Output: purple
Negative indexing starts from -1
for the last element, -2
for the second-to-last, and so on:
second_last_color = colors[-2]
print(second_last_color) # Output: yellow
Negative indexing is a convenient way to access elements when the length of the list might change, or when you’re working with large lists where counting from the end is easier than counting from the beginning.
Slicing Python Lists for Subsets
Sometimes, you’ll need to access a specific part of a list rather than just a single element. Python’s slicing feature allows you to create a new list that contains a subset of elements from the original list. This can be useful when you want to break down data or when you need to manipulate only a portion of a list.
The basic syntax for slicing is list[start:stop]
, where start
is the index of the first element you want to include, and stop
is the index where you want to stop (but this index itself is not included in the slice).
Let’s go back to our colors
list:
subset_colors = colors[1:4]
print(subset_colors) # Output: ['green', 'blue', 'yellow']
In this example, the slice starts at index 1
("green"
) and ends just before index 4
("purple"
), resulting in a new list containing "green"
, "blue"
, and "yellow"
.
You can also omit the start
or stop
indexes to slice from the beginning or up to the end of the list:
start_slice = colors[:3]
print(start_slice) # Output: ['red', 'green', 'blue']
end_slice = colors[2:]
print(end_slice) # Output: ['blue', 'yellow', 'purple']
Here, colors[:3]
creates a slice containing the first three elements, while colors[2:]
slices from the third element to the end of the list.
Slicing can be combined with negative indexing as well:
negative_slice = colors[-3:]
print(negative_slice) # Output: ['blue', 'yellow', 'purple']
This example slices the last three elements from the list.
Modifying Python Lists
In Python, lists are not only for storing data but also for managing and modifying it. This means you can change, add, or rearrange elements within a list to suit your needs. Understanding how to modify lists is crucial for any programming task that involves data manipulation. Let’s explore how you can efficiently make changes to your lists.
Changing Elements in a List
Sometimes, you need to update an element in a list. Python makes this easy with indexing. You simply refer to the index of the element you want to change and assign a new value to it.
Consider a list of numbers:
numbers = [10, 20, 30, 40, 50]
If you want to change the third element (index 2
) from 30
to 35
, you would do this:
numbers[2] = 35
print(numbers) # Output: [10, 20, 35, 40, 50]
This operation directly modifies the element at the specified index without affecting the other elements in the list.
Adding Elements to a List
Adding new elements to a list can be done in several ways, depending on whether you want to add a single element, multiple elements, or insert them at a specific position.
Using append()
and extend()
append()
: This method adds a single element to the end of the list. It’s great for adding individual items.
fruits = ["apple", "banana", "cherry"]
fruits.append("orange")
print(fruits) # Output: ['apple', 'banana', 'cherry', 'orange']
Here, "orange"
is added to the end of the fruits
list.
extend()
: This method is used to add multiple elements from another iterable (like another list) to the end of the list.
more_fruits = ["mango", "pineapple"]
fruits.extend(more_fruits)
print(fruits) # Output: ['apple', 'banana', 'cherry', 'orange', 'mango', 'pineapple']
- The
extend()
method takes themore_fruits
list and adds all its elements to the end of thefruits
list.
Inserting Elements at Specific Positions
If you need to insert an element at a specific position rather than just adding it to the end, you can use the insert()
method. This method allows you to specify the index at which you want the new element to be placed.
numbers = [10, 20, 30, 40, 50]
numbers.insert(2, 25)
print(numbers) # Output: [10, 20, 25, 30, 40, 50]
In this example, 25
is inserted at index 2
, shifting the other elements to the right.
Removing Elements from Python Lists
Managing lists in Python often involves not just adding and modifying elements, but also removing them when they are no longer needed. Whether you want to remove an element by its value, index, or clear the entire list, Python provides several straightforward methods to achieve this. Let’s explore how you can remove elements from your lists effectively.
Removing by Value and Index
Python offers different methods to remove elements from a list, each suitable for specific situations. You can remove elements based on their value or index, depending on what you need to accomplish.
Using remove()
The remove()
method is used to remove the first occurrence of a specified value from the list. If the value is not present, Python raises a ValueError
.
Here’s how it works:
colors = ["red", "green", "blue", "yellow", "blue"]
colors.remove("blue")
print(colors) # Output: ['red', 'green', 'yellow', 'blue']
In this example, only the first "blue"
is removed from the list. If you need to remove all occurrences of a value, you would need to use a loop or a list comprehension.
Using pop()
The pop()
method removes and returns the element at a specified index. If no index is provided, it removes and returns the last element in the list. This method is useful when you want to retrieve and remove an item simultaneously.
For example:
numbers = [10, 20, 30, 40, 50]
removed_number = numbers.pop(2)
print(removed_number) # Output: 30
print(numbers) # Output: [10, 20, 40, 50]
In this case, 30
is removed from index 2
, and the remaining elements are adjusted accordingly.
Using del
The del
statement can be used to delete an element at a specific index or to delete the entire list. It’s a more general-purpose deletion method that can be used in various ways.
To delete an element at a specific index:
fruits = ["apple", "banana", "cherry"]
del fruits[1]
print(fruits) # Output: ['apple', 'cherry']
Here, "banana"
is removed from index 1
. To delete the entire list:
numbers = [1, 2, 3, 4, 5]
del numbers
# print(numbers) # This would raise an error because the list no longer exists
Clearing Entire Lists
Sometimes, you may need to remove all elements from a list, but keep the list itself intact for further use. Python provides a method for this purpose.
Using clear()
The clear()
method removes all elements from the list, leaving it empty but still existing in memory.
Here’s an example:
items = ["apple", "banana", "cherry"]
items.clear()
print(items) # Output: []
After using clear()
, items
is now an empty list. This method is helpful when you need to reuse the list variable but start with an empty list.
Must Read
- Escape Sequences and Raw Strings in Python: The Complete Guide
- Prime Factorization Algorithm in Python
- How to Get the First Digit of a Number in Python
- Global and Local Variables in Python: A Complete Guide
- How to Return Multiple Values from a Function in Python
Advanced Python List Operations
When working with Python lists, sometimes you need to perform more sophisticated operations like sorting and reversing. These advanced operations help in organizing and manipulating data more effectively. Let’s explore how you can sort and reverse lists to make your data handling more powerful and efficient.
Sorting Lists in Ascending and Descending Order
Sorting a list is a common task that allows you to arrange elements in a specific order. Python provides built-in methods to sort lists both in ascending and descending order.
Sorting in Ascending Order
By default, the sort()
method arranges elements in ascending order. This means from the smallest to the largest value if dealing with numbers, or from A to Z if dealing with strings.
Here’s an example:
numbers = [5, 3, 9, 1, 7]
numbers.sort()
print(numbers) # Output: [1, 3, 5, 7, 9]
In this example, the sort()
method rearranges the numbers in ascending order.
Sorting in Descending Order
To sort a list in descending order, you can pass the reverse=True
parameter to the sort()
method. This sorts the elements from the largest to the smallest.
numbers = [5, 3, 9, 1, 7]
numbers.sort(reverse=True)
print(numbers) # Output: [9, 7, 5, 3, 1]
Here, the reverse=True
argument tells Python to sort the list in the opposite order.
Custom Sorting with the key
Parameter
Sometimes, you need to sort lists based on custom criteria. Python’s sort()
method allows for this by using the key
parameter. The key
parameter takes a function that specifies the criteria for sorting.
Let’s say you have a list of tuples where each tuple contains a name and age, and you want to sort the list by age:
people = [("Alice", 30), ("Bob", 25), ("Charlie", 35)]
people.sort(key=lambda person: person[1])
print(people) # Output: [('Bob', 25), ('Alice', 30), ('Charlie', 35)]
In this example, lambda person: person[1]
is a function that extracts the age from each tuple. The sort()
method then uses this age to sort the list.
Reversing List Order
Reversing a list is another useful operation. Python offers a straightforward way to reverse the order of elements in a list using the reverse()
method.
Using reverse()
The reverse()
method changes the order of elements in place, meaning it modifies the original list rather than creating a new one.
fruits = ["apple", "banana", "cherry"]
fruits.reverse()
print(fruits) # Output: ['cherry', 'banana', 'apple']
Here, the reverse()
method reverses the order of the elements in the fruits
list.
Reversing with Slicing
Alternatively, you can reverse a list using slicing. This method creates a new list that is a reversed version of the original list.
numbers = [1, 2, 3, 4, 5]
reversed_numbers = numbers[::-1]
print(reversed_numbers) # Output: [5, 4, 3, 2, 1]
In this example, [::-1]
is a slicing technique that steps through the list backward, effectively reversing it.
List Comprehensions in Python
List comprehensions in Python provide a concise way to create lists. They offer a powerful method for generating lists by transforming existing iterables with simple and readable code. Let’s break down how to use list comprehensions, from basic operations to more complex scenarios involving conditional logic.
Introduction to List Comprehensions
List comprehensions allow you to build a new list by applying an expression to each item in an existing iterable, like a list or a range. They are often used to make code more readable and succinct compared to traditional loops.
Here’s the basic syntax:
[expression for item in iterable]
- Expression: What you want to put in the new list.
- Item: Each element from the iterable.
- Iterable: The collection you are looping through.
For example, if you want to create a list of squares for numbers 1 through 5, you could use a list comprehension like this:
squares = [x**2 for x in range(1, 6)]
print(squares) # Output: [1, 4, 9, 16, 25]
In this example, x**2
is the expression applied to each item in the iterable range(1, 6)
.
Using List Comprehensions for Simple Operations
List comprehensions are particularly useful for performing simple operations and transformations on lists. They can replace multiple lines of code with a single line, making your code more concise and easier to read.
Example 1: Converting Strings to Uppercase
Imagine you have a list of names and you want to convert all names to uppercase. Using a list comprehension, this task becomes quite simple:
names = ["alice", "bob", "charlie"]
upper_names = [name.upper() for name in names]
print(upper_names) # Output: ['ALICE', 'BOB', 'CHARLIE']
Here, name.upper()
is applied to each element in the names
list, producing a new list where each name is in uppercase.
Example 2: Extracting Numbers from a String
Suppose you have a string containing numbers and text, and you want to extract all the numbers into a list. You can use a list comprehension with a conditional check:
text = "There are 3 apples, 4 oranges, and 5 bananas."
numbers = [int(word) for word in text.split() if word.isdigit()]
print(numbers) # Output: [3, 4, 5]
In this example, text.split()
breaks the string into words, word.isdigit()
checks if a word is a number, and int(word)
converts it to an integer.
Complex List Comprehensions with Conditional Logic
List comprehensions can also include conditional logic, allowing you to filter elements based on certain criteria. This adds a layer of flexibility and power to your list manipulations.
Example 1: Filtering Even Numbers
If you want to generate a list of squares for only even numbers between 1 and 10, you can include a condition in your list comprehension:
even_squares = [x**2 for x in range(1, 11) if x % 2 == 0]
print(even_squares) # Output: [4, 16, 36, 64, 100]
Here, if x % 2 == 0
filters out only even numbers before applying the expression x**2
.
Example 2: Combining Multiple Conditions
You can also combine multiple conditions in a list comprehension. For example, to create a list of positive squares of numbers between -5 and 5, excluding zero:
positive_squares = [x**2 for x in range(-5, 6) if x != 0 and x > 0]
print(positive_squares) # Output: [1, 4, 9, 16, 25]
In this example, the conditions x != 0
and x > 0
ensure that zero is excluded and only positive numbers are squared.
Using Python Lists with Other Data Structures
Python lists are incredibly flexible and can be used in conjunction with other data structures to create powerful and efficient solutions. Let’s explore how lists can be combined with dictionaries, tuples, and sets to enhance your programming capabilities.
Combining Lists and Dictionaries
Dictionaries and lists are two of the most commonly used data structures in Python. A dictionary stores key-value pairs, while a list is an ordered collection of items. Combining these two structures can be very useful, especially when you need to organize and manipulate data efficiently.
Example: Creating a Dictionary from a List
Suppose you have a list of names and you want to create a dictionary where each name is a key and the value is the length of the name. Here’s how you can do that:
names = ["Alice", "Bob", "Charlie"]
name_lengths = {name: len(name) for name in names}
print(name_lengths) # Output: {'Alice': 5, 'Bob': 3, 'Charlie': 7}
In this example, a dictionary comprehension is used to create a dictionary where each key is a name from the list, and the value is the length of that name.
Example: Extracting Values from a Dictionary into a List
If you have a dictionary and want to extract its values into a list, you can use the values()
method combined with the list()
function:
age_dict = {"Alice": 30, "Bob": 25, "Charlie": 35}
ages = list(age_dict.values())
print(ages) # Output: [30, 25, 35]
Here, age_dict.values()
returns a view of the dictionary’s values, and list()
converts this view into a list.
Using Lists with Tuples
Tuples are similar to lists but are immutable, meaning their elements cannot be changed once created. This immutability makes tuples useful for storing fixed collections of items. Lists and tuples often work together, especially when tuples are used as elements within lists.
Example: Creating a List of Tuples
Let’s say you need to store pairs of data, like names and ages, in a list. You can use tuples for this purpose:
people = [("Alice", 30), ("Bob", 25), ("Charlie", 35)]
In this list, each item is a tuple containing a name and an age. You can access and manipulate these tuples just like you would with other list elements.
Example: Converting a List of Tuples to a Dictionary
If you have a list of tuples and you want to convert it into a dictionary, where the first element of each tuple is the key and the second element is the value, you can use a dictionary comprehension:
people = [("Alice", 30), ("Bob", 25), ("Charlie", 35)]
people_dict = dict(people)
print(people_dict) # Output: {'Alice': 30, 'Bob': 25, 'Charlie': 35}
Here, dict(people)
creates a dictionary from the list of tuples, where each tuple becomes a key-value pair in the dictionary.
Interoperability Between Lists and Sets
Sets are unordered collections of unique elements. They are useful for operations that involve membership testing, removing duplicates, and performing set operations like unions and intersections. Lists and sets can be used together to handle a variety of tasks.
Example: Removing Duplicates from a List
If you have a list with duplicate items and want to remove the duplicates, you can convert the list to a set and then back to a list:
numbers = [1, 2, 2, 3, 4, 4, 5]
unique_numbers = list(set(numbers))
print(unique_numbers) # Output: [1, 2, 3, 4, 5] (order may vary)
Converting to a set removes duplicate values, and converting back to a list gives you a list of unique items.
Example: Finding Common Elements Between Two Lists
You can use sets to find common elements between two lists by converting them to sets and then finding the intersection:
list1 = [1, 2, 3, 4]
list2 = [3, 4, 5, 6]
common_elements = list(set(list1) & set(list2))
print(common_elements) # Output: [3, 4]
In this example, set(list1) & set(list2)
finds the intersection of the two sets, and list()
converts the result back to a list.
Common Python List Methods and Functions
Python lists come with a variety of built-in methods and functions that make it easier to manipulate and interact with your data. Understanding these methods will help you perform tasks more efficiently and enhance your overall programming skills. In this guide, we’ll cover some of the most commonly used list methods, including len()
, min()
, max()
, count()
, index()
, and copy()
.

Using len()
, min()
, and max()
with Lists
These built-in functions are incredibly useful for performing basic operations on lists.
len()
: Getting the Number of Elements
The len()
function returns the number of elements in a list. It is particularly handy when you need to know the size of the list or to perform operations that depend on the list’s length.
Example:
fruits = ["apple", "banana", "cherry"]
length = len(fruits)
print(length) # Output: 3
Here, len(fruits)
returns 3
, which is the number of items in the fruits
list.
min()
and max()
: Finding the Smallest and Largest Elements
The min()
and max()
functions help you find the smallest and largest elements in a list, respectively. These functions are often used with numerical data.
Example:
numbers = [10, 25, 5, 15]
smallest = min(numbers)
largest = max(numbers)
print(smallest) # Output: 5
print(largest) # Output: 25
In this example, min(numbers)
finds the smallest number in the list, while max(numbers)
finds the largest.
Counting Elements with count()
The count()
method is used to count the occurrences of a specific element in a list. This method is helpful when you need to determine how many times an item appears.
Example:
animals = ["cat", "dog", "cat", "bird", "cat"]
cat_count = animals.count("cat")
print(cat_count) # Output: 3
Here, animals.count("cat")
returns 3
because “cat” appears three times in the list.
Finding Elements with index()
The index()
method returns the index of the first occurrence of a specified element in a list. If the element is not found, it raises a ValueError
. This method is useful when you need to locate the position of an item.
Example:
colors = ["red", "blue", "green", "blue"]
first_blue_index = colors.index("blue")
print(first_blue_index) # Output: 1
In this case, colors.index("blue")
returns 1
, which is the index of the first occurrence of “blue” in the list.
Copying Lists with copy()
The copy()
method creates a shallow copy of a list. This means that a new list is created, but the elements are not copied deeply. If the original list contains other lists or mutable objects, changes to these objects will affect both the original and the copied list.
Example:
original_list = [1, 2, 3, 4]
copied_list = original_list.copy()
copied_list.append(5)
print(original_list) # Output: [1, 2, 3, 4]
print(copied_list) # Output: [1, 2, 3, 4, 5]
Here, original_list.copy()
creates a new list copied_list
that is a copy of original_list
. Appending to copied_list
does not affect original_list
.
Working with Multidimensional Lists (Matrices)
In Python, multidimensional lists, also known as matrices, are incredibly useful for representing and managing more complex data structures. They are essentially lists within lists, allowing you to organize data in a grid-like format. This guide will walk you through creating and accessing multidimensional lists, manipulating them, and exploring common use cases.
Creating and Accessing Multidimensional Lists
What Are Multidimensional Lists?
A multidimensional list is a list of lists. The most common type is a 2D list, which can be visualized as a table or grid. Each element of a 2D list is itself a list, allowing for rows and columns of data.
Example:
# Creating a 2D list (matrix)
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
In this example, matrix
is a 2D list with three rows and three columns. Each row is a list within the matrix
list.
Accessing Elements
To access an element in a multidimensional list, you use two indices: one for the row and one for the column.
Example:
# Accessing an element
element = matrix[1][2]
print(element) # Output: 6
Here, matrix[1][2]
accesses the element in the second row and third column, which is 6
.
Manipulating Multidimensional Lists
Modifying Elements
You can modify elements in a multidimensional list just like you would with a single-dimensional list. By specifying the row and column index, you can change the value of a particular element.
Example:
# Modifying an element
matrix[0][1] = 10
print(matrix) # Output: [[1, 10, 3], [4, 5, 6], [7, 8, 9]]
In this example, matrix[0][1]
changes the value in the first row and second column from 2
to 10
.
Adding and Removing Rows and Columns
You can add or remove rows and columns from a 2D list. Adding a row is as simple as using the append()
method. Removing a row can be done with the del
statement. Adding or removing columns involves more steps, such as iterating through each row.
Example:
# Adding a row
matrix.append([10, 11, 12])
print(matrix) # Output: [[1, 10, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]]
# Removing a row
del matrix[2]
print(matrix) # Output: [[1, 10, 3], [4, 5, 6], [10, 11, 12]]
To add or remove columns, you would need to iterate through each row to ensure consistency.
Common Use Cases: 2D Lists for Grids and Matrices
Grids
2D lists are often used to represent grids, such as in games or simulations. For example, a game board can be modeled as a 2D list where each cell contains information about the game state.
Example:
# Creating a grid for a game
game_board = [
[" ", "X", "O"],
["O", "X", " "],
[" ", "O", "X"]
]
Here, game_board
is a 3×3 grid where each cell represents a position on the board.
Matrices
In mathematics and data analysis, 2D lists can be used as matrices to perform operations such as matrix addition or multiplication.
Example:
# Adding two matrices
matrix1 = [
[1, 2],
[3, 4]
]
matrix2 = [
[5, 6],
[7, 8]
]
result = [
[matrix1[i][j] + matrix2[i][j] for j in range(len(matrix1[0]))]
for i in range(len(matrix1))
]
print(result) # Output: [[6, 8], [10, 12]]
This example shows how to add two matrices element-wise to produce a new matrix.
Memory and Performance Optimization with Python Lists
When working with Python lists, understanding memory usage and performance optimization can be crucial, especially when dealing with large datasets. This guide will explore how Python handles list memory, ways to optimize memory usage, and how to use generators for large datasets.
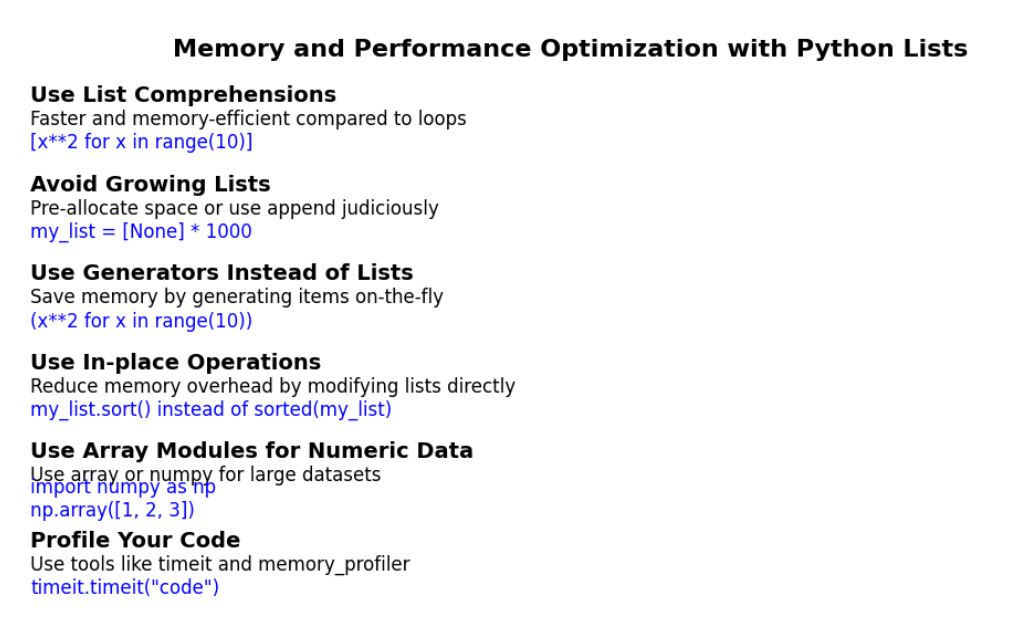
Memory Efficiency in Python Lists
Understanding List Memory Usage
Python lists are dynamic arrays, which means their size can change as you add or remove elements. This flexibility comes at a cost in terms of memory usage. Each element in a Python list is stored as a reference to the object it points to, rather than the object itself. This can lead to higher memory consumption compared to static arrays in other languages.
Example:
# Creating a list with a large number of elements
large_list = [i for i in range(1000000)]
In this example, large_list
contains a million integers. Each integer in the list is a reference to an integer object, which adds overhead.
To understand how much memory a list uses, you can use the sys
module:
import sys
# Checking the size of the list
list_size = sys.getsizeof(large_list)
print(f"Memory used by list: {list_size} bytes")
This will give you the memory usage of the list object itself, but remember that this does not include the memory used by the individual objects in the list.
Optimizing List Memory Usage
To optimize memory usage with lists, consider the following strategies:
- Use List Comprehensions: List comprehensions are generally more memory-efficient than using
append()
in a loop because they avoid the overhead of method calls.
Example:
# Using a list comprehension
squares = [x**2 for x in range(1000)]
This method creates a list of squares in a single step, reducing overhead.
2. Use Built-In Functions: Built-in functions like map()
and filter()
can be more efficient than list comprehensions in certain cases.
Example:
# Using map() to create a list of squares
squares = list(map(lambda x: x**2, range(1000)))
3. Avoid Unnecessary Copies: Be cautious when copying lists. Using slicing or the copy()
method creates a new list with the same elements, which doubles the memory usage.
Example:
# Copying a list
copied_list = large_list.copy()
If you don’t need a full copy, consider using slicing to create a smaller subset.
4. Use Lists of Fixed Size: For lists that are always of the same size, you might use arrays from the array
module or third-party libraries like NumPy, which are more memory-efficient.
Example with array module:
from array import array
# Creating an array of integers
int_array = array('i', range(1000))
Using Generators for Large Datasets
For very large datasets, traditional lists might not be practical due to their memory overhead. Generators offer a solution by producing items one at a time, rather than storing them all in memory.
Introduction to Generators
Generators are a type of iterable, like lists or tuples, but they generate items on the fly and do not store them in memory. This makes them much more memory-efficient for processing large datasets.
Example:
# Generator expression
squares_gen = (x**2 for x in range(1000))
Here, squares_gen
is a generator that yields squares of numbers from 0
to 999
one by one.
Benefits of Generators
- Memory Efficiency: Generators are much more memory-efficient because they only store the current item and generate the next one as needed.
- Lazy Evaluation: Generators compute values only when needed, which can improve performance for certain applications.
- Use Case: They are especially useful for reading large files or streaming data.
Example of using a generator to read a large file:
# Generator to read lines from a file
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line
# Processing lines from the file
for line in read_large_file('large_file.txt'):
# Process each line
print(line)
Performance Considerations for Python Lists
When working with Python lists, it’s important to understand how their performance compares to other data structures like tuples and arrays. Choosing the right structure for your task can greatly influence both speed and memory usage. Additionally, Python offers built-in functions that can speed up list operations. Let’s explore these concepts in a way that’s both easy to grasp and practical for real-world use.
List vs. Other Data Structures
Python provides several ways to store and manipulate collections of data, with lists, tuples, and arrays being among the most common. Understanding their differences can help you choose the most efficient one for your needs.
Lists
Python lists are incredibly flexible and allow for mixed data types, dynamic resizing, and various built-in methods. However, this flexibility comes at a cost in terms of speed and memory usage.
Example:
# Creating a list with mixed data types
my_list = [1, 'apple', 3.14, True]
Lists are great when you need to modify your data frequently, as they allow adding, removing, and updating elements easily. However, because lists are dynamic arrays, they can consume more memory and be slower than more specialized data structures.
Tuples
Tuples are similar to lists, but they are immutable, meaning that once you create a tuple, you cannot change its elements. This immutability makes tuples faster and more memory-efficient than lists.
Example:
# Creating a tuple
my_tuple = (1, 'apple', 3.14, True)
Tuples are ideal when you have a collection of items that should not change throughout the program, such as coordinates or fixed configurations. Their immutability ensures that they are safe from unintended modifications.
Arrays
The array
module in Python provides a more memory-efficient way to store collections of data, particularly when all elements are of the same type. Arrays are closer to the static arrays in languages like C, making them faster and more efficient for large datasets.
Example:
from array import array
# Creating an array of integers
my_array = array('i', [1, 2, 3, 4])
Arrays are perfect for numerical data that requires frequent computations, as they provide better performance than lists. However, they are not as flexible as lists, as they require all elements to be of the same type.
Choosing the Right Data Structure for the Task
Selecting the appropriate data structure for your task can significantly impact your program’s performance. Here are some guidelines to help you make the right choice:
- Use Lists when you need flexibility and the ability to modify data frequently. They are versatile but may not be the best choice for memory-sensitive applications.
- Use Tuples when you have a fixed collection of items that won’t change. They are faster and more memory-efficient, making them ideal for read-only data.
- Use Arrays when you need to store large amounts of numerical data. They provide better performance and lower memory usage for homogenous data types.
Speeding Up List Operations with Built-In Functions
Python offers several built-in functions that can speed up list operations, making your code more efficient.
Using map()
The map()
function applies a given function to all items in a list (or other iterable) and returns a map object, which can be converted to a list. This method is faster than using a for
loop to apply a function to each element.
Example:
# Using map to square each number in the list
numbers = [1, 2, 3, 4, 5]
squared = list(map(lambda x: x**2, numbers))
print(squared) # Output: [1, 4, 9, 16, 25]
Using filter()
The filter()
function filters elements from a list based on a condition. Like map()
, it returns an iterable that can be converted to a list. This function is more efficient than manually filtering elements with a for
loop.
Example:
# Using filter to get even numbers from the list
numbers = [1, 2, 3, 4, 5]
evens = list(filter(lambda x: x % 2 == 0, numbers))
print(evens) # Output: [2, 4]
Using reduce()
The reduce()
function, from the functools
module, applies a function of two arguments cumulatively to the items of a list, from left to right, reducing the list to a single value. This can be faster than manually accumulating results with a loop.
Example:
from functools import reduce
# Using reduce to calculate the product of all numbers in the list
numbers = [1, 2, 3, 4, 5]
product = reduce(lambda x, y: x * y, numbers)
print(product) # Output: 120
Latest Advancements and Features Related to Python Lists
Python continues to evolve, with each new version introducing features that make it more powerful and user-friendly. Python lists, a cornerstone of the language, have seen significant updates in recent versions. In this discussion, we’ll explore the latest advancements related to Python lists, particularly focusing on new features in Python 3.10 and beyond. These include pattern matching with lists, improved error messages, type hints, and the potential performance enhancements brought by Python 3.12.
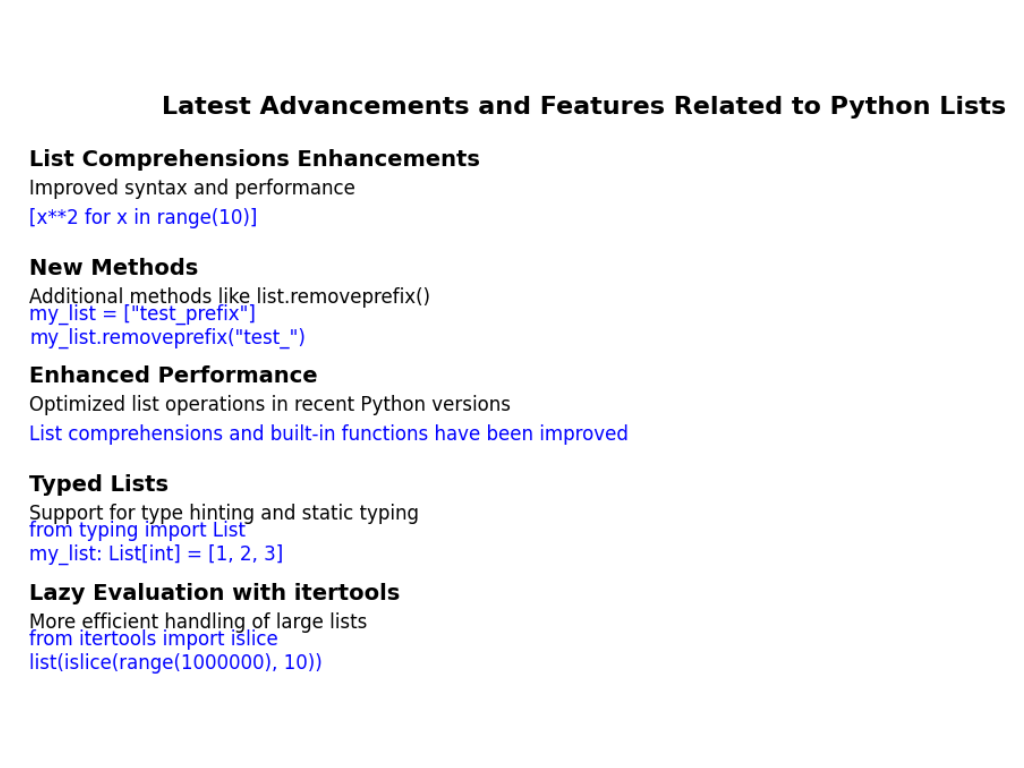
Pattern Matching with Lists
One of the most exciting features introduced in Python 3.10 is structural pattern matching, often referred to as “pattern matching.” While this feature is reminiscent of switch-case statements in other languages, it’s far more powerful and flexible. For lists, pattern matching allows you to match the structure of lists against specific patterns, making it easier to write code that processes complex data structures.
Example: Simple Pattern Matching with Lists
Let’s say you have a list and want to determine if it has exactly three elements, with the first element being a string and the second and third elements being numbers. Here’s how you can use pattern matching:
def process_list(data):
match data:
case [str(name), int(age), int(score)]:
print(f"Name: {name}, Age: {age}, Score: {score}")
case _:
print("Pattern not matched")
# Test the function
process_list(["Alice", 30, 85]) # Output: Name: Alice, Age: 30, Score: 85
process_list(["Bob", "30", 85]) # Output: Pattern not matched
Pattern matching makes it easier to work with lists that have a predictable structure, reducing the need for cumbersome conditional statements.
Improved Error Messages for List Operations
Another significant improvement in recent Python versions is the enhancement of error messages. We’ve all been there: you make a small mistake in your code, and Python’s error message leaves you scratching your head. Thankfully, the error messages have become more informative and precise, especially when working with lists.
Example: Improved Error Messages
Consider the following scenario where you mistakenly try to access an index that doesn’t exist:
my_list = [1, 2, 3]
print(my_list[5])
In earlier versions of Python, the error message might have been somewhat cryptic. However, with the latest updates, the message is more detailed:
IndexError: list index out of range
The list has only 3 elements, but you tried to access index 5.
This improved clarity can save you time when debugging, making your development process smoother.
Type Hints and Static Typing in Lists
Type hints were introduced in Python 3.5, but they’ve been continuously refined and expanded in subsequent versions. For lists, type hints allow you to specify the expected type of elements within a list, which can help catch errors before your code runs.
Example: Type Hints with Lists
Suppose you’re writing a function that processes a list of integers. You can now specify that the function expects a list of integers:
from typing import List
def sum_numbers(numbers: List[int]) -> int:
return sum(numbers)
# Test the function
print(sum_numbers([1, 2, 3, 4])) # Output: 10
Using type hints not only makes your code more readable but also allows tools like mypy to perform static type checking, catching potential errors early in the development cycle.
Exploring the Impact of Python 3.12 on List Performance
Python 3.12, the latest version in the pipeline, brings several performance improvements, some of which directly impact list operations. Although the final release notes will provide a detailed breakdown, the ongoing work hints at faster list operations and better memory management.
Example: Anticipated Performance Gains
The Python core development team has been working on optimizing the underlying algorithms and data structures that power lists. This could result in faster sorting, appending, and indexing operations. Additionally, memory optimizations may reduce the overhead associated with large lists, making Python even more competitive for high-performance applications.
Python Lists in Machine Learning and Data Science
Python lists play a crucial role in machine learning and data science, acting as a foundation for more advanced data structures and operations. They are often the starting point before transitioning to specialized tools like NumPy and Pandas, which are optimized for numerical and tabular data. In this discussion, we’ll explore how Python lists are used in machine learning and data science, focusing on their integration with NumPy arrays, Pandas DataFrames, and how they fit into more complex machine learning workflows.
Using Lists with NumPy Arrays
NumPy is a powerful library in Python, specifically designed for numerical computations. While Python lists are versatile and easy to use, they aren’t always the most efficient when working with large datasets or performing mathematical operations. This is where NumPy arrays come into play. NumPy arrays are similar to lists but are much faster and more efficient for numerical data.
However, it’s common to start with Python lists and then convert them into NumPy arrays for more advanced operations.
Example: Converting Python Lists to NumPy Arrays
Imagine you have a list of numbers and you want to perform mathematical operations on them, like finding the square of each number. You can do this more efficiently by converting the list to a NumPy array:
import numpy as np
# Start with a Python list
numbers = [1, 2, 3, 4, 5]
# Convert the list to a NumPy array
np_array = np.array(numbers)
# Perform operations
squared_array = np_array ** 2
print(squared_array) # Output: [ 1 4 9 16 25]
By converting the list into a NumPy array, you can take advantage of NumPy’s optimized operations, making your code faster and more efficient.
Integrating Python Lists with Pandas DataFrames
Pandas is another essential library in data science, particularly for working with structured data. A Pandas DataFrame is like a table in a spreadsheet or a SQL database, where you can organize your data into rows and columns. While Pandas excels with structured data, it’s common to start with lists, especially when your data is simple or unstructured.
Example: Creating a DataFrame from Python Lists
Let’s say you have two lists, one for names and one for scores, and you want to create a DataFrame:
import pandas as pd
# Lists of data
names = ['Alice', 'Bob', 'Charlie']
scores = [85, 90, 88]
# Create a DataFrame
df = pd.DataFrame({'Name': names, 'Score': scores})
print(df)
The output will be a well-structured table:
Name Score
0 Alice 85
1 Bob 90
2 Charlie 88
By integrating Python lists with Pandas DataFrames, you can quickly transition from simple lists to more complex data structures, enabling you to perform a wide range of data analysis tasks.
Advanced List Operations in Machine Learning Workflows
In machine learning workflows, lists often serve as the foundation for more complex operations. For instance, you might start with a list of features or labels and then transform these lists into the format required by machine learning models.
Example: Using Lists for Feature Engineering
Let’s consider a scenario where you have a list of raw text data, and you need to convert it into numerical features for a machine learning model. This process is known as feature engineering, and it’s a common task in machine learning workflows.
from sklearn.feature_extraction.text import CountVectorizer
# List of text documents
documents = ["I love Python", "Python is great for data science", "Data science is fun"]
# Convert the list of text documents into numerical features
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(documents)
# Convert the result into an array
X_array = X.toarray()
print(X_array)
The CountVectorizer
converts the list of text documents into a matrix of token counts, a crucial step in preparing text data for machine learning models.
Common Python List Pitfalls and How to Avoid Them
Python lists are incredibly flexible, but their mutability can sometimes lead to unexpected behavior, especially for those new to programming. Understanding the common pitfalls when working with lists—and how to avoid them—can save you a lot of headaches. Let’s explore some of these pitfalls, focusing on list mutability, common errors, and ways to avoid issues related to shared references.
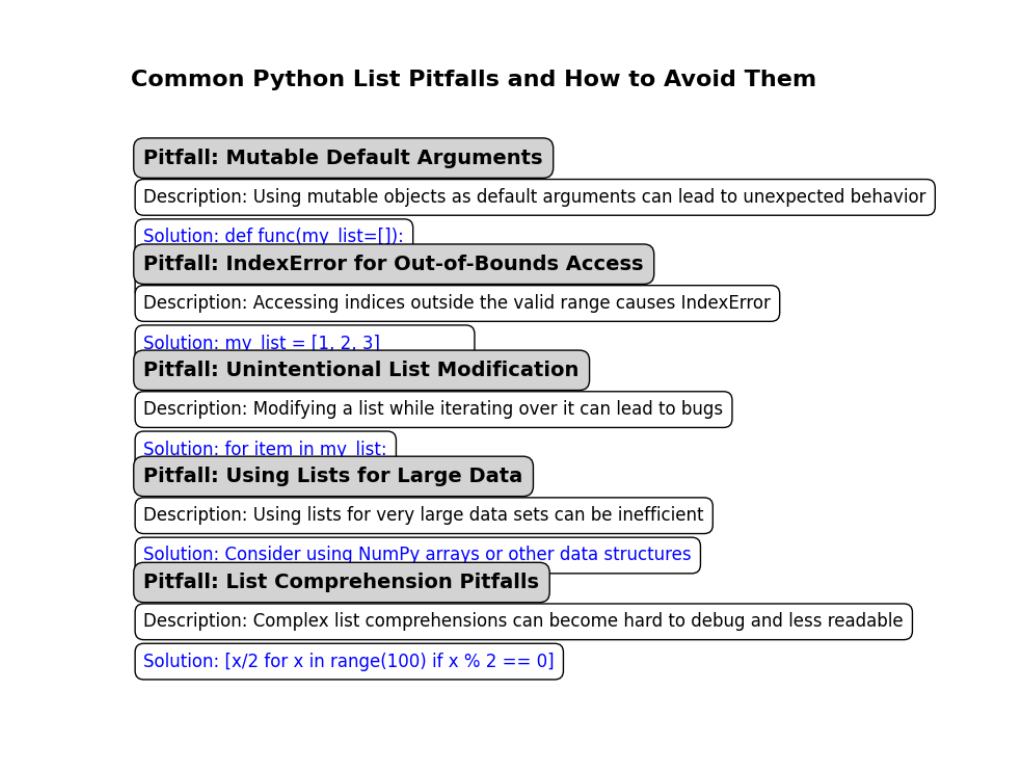
Understanding List Mutability
In Python, lists are mutable, meaning that you can change their content without creating a new list. This flexibility is one of the reasons why lists are so useful, but it also comes with its own set of challenges. When you modify a list, the changes affect the list directly, which can lead to unexpected outcomes, especially if you’re not careful.
Example: Modifying a List in Place
Let’s start with a simple example:
my_list = [1, 2, 3]
my_list.append(4)
print(my_list) # Output: [1, 2, 3, 4]
Here, the append()
method adds 4
to the end of my_list
. This is straightforward, but the mutability of lists can cause issues when you’re dealing with shared references or when you expect a list to remain unchanged.
Common Errors When Modifying Lists
One of the most common errors is unintentionally modifying a list that you intended to keep unchanged. This can happen when you copy a list by reference rather than by value.
Example: Copying by Reference
original_list = [1, 2, 3]
copied_list = original_list
copied_list.append(4)
print("Original:", original_list) # Output: [1, 2, 3, 4]
print("Copied:", copied_list) # Output: [1, 2, 3, 4]
In this example, both original_list
and copied_list
end up with [1, 2, 3, 4]
. This happens because copied_list
is not an independent copy; it’s just another reference to the same list in memory.
Avoiding Shared References Between Lists
To avoid the pitfall of shared references, you need to create a new, independent copy of the list. This can be done using methods like slicing or the copy()
method.
Example: Creating an Independent Copy
original_list = [1, 2, 3]
independent_copy = original_list.copy()
independent_copy.append(4)
print("Original:", original_list) # Output: [1, 2, 3]
print("Independent Copy:", independent_copy) # Output: [1, 2, 3, 4]
In this case, the copy()
method creates a new list that is independent of original_list
. Modifying independent_copy
doesn’t affect original_list
, which is exactly what you want when you need to preserve the original data.
Avoiding Performance Bottlenecks in Large Lists
Working with large lists in Python can sometimes lead to performance issues, especially if you don’t manage them efficiently. This can result in slowdowns or excessive memory use, which can be frustrating. Let’s explore how to handle large lists effectively, the benefits of using generators, and common pitfalls with list comprehensions.
Efficiently Managing Large Lists in Memory
When dealing with large lists, memory management becomes crucial. Lists in Python can grow large, consuming significant amounts of memory, which might lead to performance bottlenecks.
Example: Large List Creation
Creating a large list can be straightforward but memory-intensive:
large_list = [i for i in range(1000000)]
While this list is easy to create, its size can impact your system’s performance, especially if you’re working with limited memory.
Tips for Efficient Management
- Use Data Structures Wisely: Sometimes, using different data structures like arrays or sets can be more efficient for specific operations. For example, if you need to perform many lookups, a set might be a better choice than a list due to its faster membership testing.
- Optimize Memory Usage: Python lists can be memory-intensive, especially with large datasets. To minimize memory use, consider using Python’s built-in modules like
array
or third-party libraries such as NumPy, which are designed to handle large data more efficiently. - Avoid Unnecessary Copies: If you don’t need to modify a list, avoid creating unnecessary copies. Large lists copied in memory can slow down your program and use more memory.
When to Use Generators Instead of Lists
Generators are a powerful alternative to lists when dealing with large datasets. Unlike lists, which store all their elements in memory, generators yield items one at a time and do not store them in memory, which can greatly improve performance.
Example: Using a Generator
Here’s how a generator can be used instead of a list:
def large_range():
for i in range(1000000):
yield i
large_gen = large_range()
for number in large_gen:
# Process number
pass
In this example, large_range
is a generator function that yields numbers one by one. This approach doesn’t consume memory for the entire range of numbers at once, making it much more memory-efficient than a list.
Benefits of Generators
- Memory Efficiency: Generators handle large datasets efficiently by producing items on-the-fly without storing them in memory.
- Lazy Evaluation: Generators produce items only when needed, which can lead to performance improvements in your code.
Common Mistakes with List Comprehensions
List comprehensions offer a concise way to create lists but can sometimes lead to performance issues if not used carefully, especially with large data.
Example: Inefficient List Comprehension
Consider the following list comprehension:
large_list = [x * x for x in range(1000000) if x % 2 == 0]
This creates a list with 500,000 items (all even squares from 0 to 999,999). While this is efficient in terms of writing the code, it can be memory-intensive and slow if the data size is extremely large.
Tips for Avoiding Pitfalls
- Be Mindful of Data Size: For very large lists, consider using a generator expression instead of a list comprehension to save memory:
large_gen = (x * x for x in range(1000000) if x % 2 == 0)
2. Optimize Conditions: Make sure conditions in list comprehensions are optimized to avoid unnecessary computations.
Practical Examples and Use Cases of Python Lists
Data Processing and Analysis
One of the most common uses of Python lists is in data processing and analysis. Lists make it simple to store, manipulate, and analyze data, whether you’re working with a few items or handling large datasets.
Example: Filtering and Transforming Data
Let’s say you have a list of numbers, and you want to filter out the even ones and square the remaining odd numbers.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
odd_squares = [n ** 2 for n in numbers if n % 2 != 0]
print(odd_squares) # Output: [1, 9, 25, 49, 81]
In this example, list comprehension allows you to filter and transform the data in a single, readable line of code. This is incredibly useful in data analysis, where you often need to clean and manipulate data before performing any analysis.
Use Case: Analyzing Sales Data
Imagine you’re working with sales data stored as a list of dictionaries, where each dictionary represents a sale.
sales_data = [
{"date": "2024-09-01", "amount": 100},
{"date": "2024-09-02", "amount": 200},
{"date": "2024-09-03", "amount": 150},
]
total_sales = sum([sale["amount"] for sale in sales_data])
print(total_sales) # Output: 450
Here, lists help you quickly sum up sales, making it easy to perform basic data analysis tasks like calculating totals, averages, or even trends over time.
Building Custom Data Structures with Lists
Lists are also powerful tools for creating custom data structures. While Python provides built-in data structures like dictionaries and sets, sometimes you need something tailored to your specific needs.
Example: Implementing a Stack
A stack is a data structure that follows the Last In, First Out (LIFO) principle. You can implement a stack using a list in Python:
stack = []
# Push elements onto the stack
stack.append(1)
stack.append(2)
stack.append(3)
# Pop elements off the stack
print(stack.pop()) # Output: 3
print(stack.pop()) # Output: 2
Using lists to implement a stack is straightforward and demonstrates how lists can be adapted to create more complex structures.
Use Case: Task Management System
Consider building a simple task management system where tasks are stored in a list and follow a LIFO order. This system could prioritize the most recently added tasks, ensuring that they are completed first. By using lists to manage tasks, you create a flexible and easy-to-understand structure for handling priorities.
Using Lists in Web Development and APIs
In web development, lists often play a crucial role in handling collections of data, whether you’re managing user inputs, parsing JSON responses, or even generating HTML dynamically.
Example: Handling JSON Data
Let’s say you’re working with an API that returns a list of users in JSON format. You need to extract all usernames from the response.
import json
response = '[{"username": "user1"}, {"username": "user2"}, {"username": "user3"}]'
users = json.loads(response)
usernames = [user["username"] for user in users]
print(usernames) # Output: ['user1', 'user2', 'user3']
Here, the list helps you easily extract and manipulate data from the JSON response, which is a common task in web development.
Use Case: Building a Simple API
Suppose you’re building a simple RESTful API that returns a list of items in response to a GET request. You could store these items in a list and return them as JSON when requested:
from flask import Flask, jsonify
app = Flask(__name__)
items = ["item1", "item2", "item3"]
@app.route('/items', methods=['GET'])
def get_items():
return jsonify(items)
if __name__ == '__main__':
app.run(debug=True)
In this example, lists make it easy to manage and return collections of data in a web application. Lists work well with frameworks like Flask, helping you build APIs quickly and efficiently.
Python List Exercises and Challenges
Beginner Exercises: Basic List Operations
When you’re just getting started with Python lists, it’s essential to grasp the fundamentals. These exercises will help you build a strong foundation by practicing basic list operations like adding, removing, and accessing elements.
Exercise 1: Appending and Extending Lists
Start by creating a list of your favorite fruits. Then, add a new fruit to the list using the append()
method. Next, extend the list with a few more fruits using the extend()
method.
fruits = ["apple", "banana", "cherry"]
fruits.append("orange")
fruits.extend(["grape", "mango"])
print(fruits) # Output: ['apple', 'banana', 'cherry', 'orange', 'grape', 'mango']
Challenge: Try adding another list inside the fruits
list. What happens? Understanding how append()
and extend()
behave differently is key to mastering basic list operations.
Exercise 2: Removing and Popping Elements
Now, let’s practice removing elements from a list. Start with the fruits
list you just created. Remove “banana” using the remove()
method and pop the last element using the pop()
method.
fruits.remove("banana")
last_fruit = fruits.pop()
print(fruits) # Output: ['apple', 'cherry', 'orange', 'grape']
print(last_fruit) # Output: 'mango'
Tip: Pay attention to how remove()
targets specific elements, while pop()
removes elements based on their position.
Exercise 3: Indexing and Slicing Lists
Lists in Python are ordered, which means every element has an index. Try accessing the first and last elements of your fruits
list. Then, slice the list to get a sublist of the middle elements.
first_fruit = fruits[0]
last_fruit = fruits[-1]
middle_fruits = fruits[1:3]
print(first_fruit) # Output: 'apple'
print(last_fruit) # Output: 'grape'
print(middle_fruits) # Output: ['cherry', 'orange']
These exercises may seem simple, but they form the bedrock of working with lists in Python. Once you’re comfortable with these basic operations, you’ll be ready to tackle more complex challenges.
Intermediate Challenges: List Comprehensions and Sorting
As you become more familiar with Python lists, it’s time to explore more advanced techniques like list comprehensions and sorting. These challenges will help you write more concise and efficient code.
Challenge 1: Filtering Lists with List Comprehensions
Suppose you have a list of numbers, and you want to create a new list that only contains the even numbers. Instead of using a loop, try using a list comprehension.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [n for n in numbers if n % 2 == 0]
print(even_numbers) # Output: [2, 4, 6, 8, 10]
Insight: List comprehensions are powerful because they allow you to filter and transform data in a single line. This not only makes your code cleaner but also more efficient.
Challenge 2: Sorting Lists with Custom Keys
Sorting is a common operation in Python, and sometimes you need to sort lists based on custom criteria. Let’s sort a list of tuples based on the second element of each tuple.
pairs = [(1, 'one'), (2, 'two'), (3, 'three'), (4, 'four')]
sorted_pairs = sorted(pairs, key=lambda x: x[1])
print(sorted_pairs) # Output: [(4, 'four'), (1, 'one'), (3, 'three'), (2, 'two')]
Pro Tip: Understanding how to use the key
argument in sorted()
allows you to sort lists in more complex ways, making your data manipulation tasks much easier.
Challenge 3: Combining List Comprehensions and Sorting
For a more advanced challenge, combine list comprehensions with sorting. For example, create a list of squared numbers from 1 to 10 and sort them in descending order.
squares = sorted([n ** 2 for n in range(1, 11)], reverse=True)
print(squares) # Output: [100, 81, 64, 49, 36, 25, 16, 9, 4, 1]
This challenge brings together several skills—list comprehensions, sorting, and working with ranges—to produce a compact yet powerful solution.
Advanced Problems: Working with Multidimensional Lists
Once you’re comfortable with the basics and intermediate concepts, you can start working on more advanced problems involving multidimensional lists, also known as matrices. These problems require you to think about lists in two or more dimensions, which can be a bit more challenging but also very rewarding.
Problem 1: Creating and Accessing a 2D List
Start by creating a 2D list (a list of lists) representing a simple 3×3 grid. Then, access and print specific elements, such as the element in the first row and second column.
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
element = matrix[0][1]
print(element) # Output: 2
Advice: When working with 2D lists, always pay attention to the row and column indices, as they help you navigate through the matrix.
Problem 2: Transposing a Matrix
Transposing a matrix involves flipping it over its diagonal, turning rows into columns and vice versa. Try transposing the matrix from the previous problem.
transpose = [[row[i] for row in matrix] for i in range(3)]
print(transpose)
# Output:
# [
# [1, 4, 7],
# [2, 5, 8],
# [3, 6, 9]
# ]
Challenge Yourself: Implement the transpose operation manually first, then compare it to using list comprehensions. This will deepen your understanding of both approaches.
Problem 3: Working with 3D Lists
For a truly advanced challenge, create a 3D list and practice accessing elements in this more complex structure. Think of it as a list of lists of lists.
cube = [
[[1, 2], [3, 4]],
[[5, 6], [7, 8]]
]
element = cube[1][0][1]
print(element) # Output: 6
Hint: Visualizing the structure of your 3D list as layers or slices can help you better understand how to access elements within it.
Recap of Python List Essentials
At the heart of Python lists is their ability to store and manipulate data in an ordered collection. We’ve covered how to create, access, and modify lists, emphasizing the importance of understanding list mutability and common pitfalls to avoid. We’ve also dived into advanced operations like list comprehensions, sorting, and working with multidimensional lists. These concepts are foundational for any Python programmer, especially when dealing with large datasets or complex algorithms.
Key Takeaways from the Guide
- Basic Operations: Mastering appending, removing, and slicing elements is crucial for efficiently managing lists.
- List Comprehensions: These powerful one-liners can simplify your code while making it more readable and efficient.
- Sorting and Filtering: Custom sorting and filtering through list comprehensions enable more sophisticated data manipulation.
- Advanced Structures: Working with multidimensional lists requires a clear understanding of indexing and nested structures, offering deeper insights into more complex programming tasks.
- Performance Considerations: Avoiding performance bottlenecks in large lists by using generators and optimizing list comprehensions is key to writing efficient code.
Further Resources and Reading
To continue your learning journey, here are some resources that can help deepen your understanding of Python lists:
Python Official Documentation
- Python Lists
- The official Python documentation offers a comprehensive overview of lists, including their methods, usage, and best practices. It’s a great reference for understanding the intricacies of list operations and behaviors.
Encouraging Best Practices for Python Lists
As you move forward, keep these best practices in mind:
- Write Readable Code: While list comprehensions are powerful, ensure they remain readable. Avoid cramming too much logic into a single line.
- Optimize for Performance: When dealing with large datasets, consider using generators or other memory-efficient structures.
- Practice Regularly: The more you work with lists, the more intuitive they will become. Regular practice will help you recognize patterns and solutions more quickly.
- Stay Curious: Python’s ecosystem is vast, and there’s always something new to learn. Whether it’s a new list method or a different approach to solving a problem, staying curious will keep your skills sharp.
FAQs
A Python list is a versatile data structure that can store an ordered collection of items. These items can be of any data type, including integers, strings, and even other lists. Lists are mutable, meaning their elements can be modified after creation.
You can create a list in Python by placing comma-separated values inside square brackets. For example:
my_list = [1, 2, 3, ‘a’, ‘b’, ‘c’]
You can access elements in a list using indexing. For example, my_list[0]
will return the first element. Python lists use zero-based indexing, so the first element is at index 0.
Some common methods include:append()
: Adds an element to the end of the list.extend()
: Extends the list by appending elements from another list.insert()
: Inserts an element at a specified position.remove()
: Removes the first occurrence of a specified element.pop()
: Removes and returns the element at a specified position.
You can iterate over a list using a for
loop:
for item in my_list:
print(item)
Leave a Reply