Introduction to the __init__ Method in Python
If you’re learning Python, you’ve probably seen the __init__Method. At first, it can seem confusing. But it’s actually simple and important. The __init__
method helps you set up your objects in a class. It’s like a blueprint for creating something new.
In this post, we’ll explain what __init__
does in clear terms. You’ll learn how to use it, why it’s important, and how it makes your code work better. We’ll also cover some common mistakes and how to avoid them.
By the end, you’ll understand the __init__
method and be ready to use it confidently. Let’s start!
What is the __init__ Method in Python?
The__init__ Method is a special function in Python that serves as a constructor in Object-Oriented Programming (OOP). It’s called when an object is created from a class and allows you to initialize the object’s attributes. When you create a new object (or instance) of a class, the __init__ Method ensures that the object has the right properties or data attached to it.

Think of it as setting the stage before your object gets to work—assigning all the necessary attributes right from the beginning so that the object behaves correctly.
Example: How __init__
Works
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
my_car = Car('Tesla', 'Model S', 2022)
print(my_car.make) # Output: Tesla
print(my_car.model) # Output: Model S
print(my_car.year) # Output: 2022
Here, the Car
class uses the __init__
method to set up the make
, model
, and year
attributes for every new car object. Without this method, every time you create a new car, you’d have to manually assign these attributes, which could get repetitive.
Importance of the __init__ Method in Object-Oriented Programming (OOP)
The __init__ Method is vital for Python Object-Oriented Programming because it allows for initialization—assigning values when an object is created. By automatically setting the object’s initial state, it helps manage complexity and maintain a consistent structure.
Imagine you were building an online course platform where students have names, IDs, and courses they’re enrolled in. Without the__init__ Method, you’d have to write extra code each time you create a new student object, making your code messy and repetitive.
A Real-Life Example: Online Course Registration System
class Student:
def __init__(self, name, student_id, courses):
self.name = name
self.student_id = student_id
self.courses = courses
student1 = Student("John Doe", 12345, ["Python", "Data Science"])
print(student1.name) # Output: John Doe
print(student1.student_id) # Output: 12345
print(student1.courses) # Output: ['Python', 'Data Science']
This method is particularly important in real-world projects, like online course systems, where it’s essential to initialize each student with specific data like name, ID, and enrolled courses. This makes the management of large-scale systems easier to maintain, especially when new objects are constantly being created.
Python Object-Oriented Programming Concepts: A Brief Overview
Python’s Object-Oriented Programming (OOP) is a programming paradigm that revolves around objects and classes. It’s a way to structure your code, making it more intuitive by organizing related variables and functions into single units called classes.
Core Concepts of Python OOP
Concept | Description |
---|---|
Class | A blueprint for creating objects. Defines the properties (attributes) and behaviors (methods). |
Object | An instance of a class, containing data and methods that operate on that data. |
Method | Functions defined within a class that represent the behavior of the objects created by that class. |
Attribute | Variables that hold data for the object, like name, age, or in our case, make, model, year. |
Inheritance | One class can inherit attributes and methods from another class, promoting code reuse. |
Encapsulation | Bundling of data (attributes) and methods that operate on the data into one unit (class). |
Polymorphism | The ability of different classes to be treated as instances of the same class through inheritance. |
In simple terms, OOP in Python allows you to group related attributes (variables) and methods (functions) into a class. When you create an object from that class, the object gets all the attributes and methods defined in that class. It’s a great way to keep your code organized and reusable.
Example: Creating a Python Class with Methods and Attributes
class Course:
def __init__(self, course_name, instructor):
self.course_name = course_name
self.instructor = instructor
def course_info(self):
return f"{self.course_name} is taught by {self.instructor}"
python_course = Course("Python Attributes and Methods", "Prof. Jane")
print(python_course.course_info())
# Output: Python Attributes and Methods is taught by Prof. Jane
This example showcases how methods and attributes work in tandem within a class. The class Course
has both attributes (course_name
and instructor
) and a method (course_info
) that prints useful information about the course.
Understanding the Basics of the __init__ Method
What Does the __init__ Method Do?
The __init__
method in Python is all about initialization. It’s called automatically when a new instance of a class is created, and its primary job is to set up the initial state of the object by assigning values to the object’s attributes. It’s like a behind-the-scenes step that gets your object ready to function correctly.
Here’s a simple way to think about it: if your class is a blueprint for creating objects, the __init__
method is where you specify all the details for each new object, like what color a house should be, how many rooms it has, or what its layout looks like.
Explanation of Initialization in Python Classes
When an object is created in Python, the __init__ Method is triggered to initialize it. This means setting the starting values for any attributes the object needs to function properly. Without __init__
, every time you created an object, you’d have to manually assign values to its attributes afterward, which could get cumbersome and lead to errors.
How __init__
Sets Up Initial Object State
The __init__
method plays a key role in making sure that each object has a consistent and well-defined state from the very beginning. When the __init__
method is called, it assigns values to the object’s attributes and prepares it for use.
In the example above, the __init__
method sets the student’s name, ID, and enrolled courses. Once these values are assigned, you can use those attributes throughout your program, which leads to a more organized and efficient codebase.
If __init__
wasn’t used, you would have to manually assign values to each student after the object was created, leaving room for human error. That’s why __init__
is essential in ensuring your objects start off with the correct data.
Difference Between __init__
and Other Magic Methods in Python
Python has several magic methods (also called dunder methods) that start and end with double underscores. These methods control how objects behave in certain situations, like when they are created, compared, or printed.
The __init__ Method is used specifically for object initialization. However, it’s important not to confuse it with other magic methods, which serve different purposes.
Example of Magic Methods in Python:
Magic Method | Purpose |
---|---|
__new__ | Creates a new instance of the class. Rarely used directly by developers. |
__str__ | Defines the human-readable string representation of an object. |
__repr__ | Defines the official string representation of an object (useful for debugging). |
__init__
vs __new__
The key difference between __init__
and __new__
is that __new__
is responsible for creating a new instance of a class, while __init__
is responsible for initializing that instance. You almost never need to worry about __new__
, as Python handles it automatically in most cases.
Here’s how they differ:
__new__
: Creates and returns a new instance of a class.__init__
: Initializes the attributes of that instance.
In everyday Python programming, you’ll almost always be working with __init__
and will rarely need to touch __new__
unless you’re doing something more advanced with object creation.
Other Commonly Used Magic Methods (__str__
, __repr__
, etc.)
While __init__
gets a lot of attention, other magic methods like __str__
and __repr__
are also important.
__str__
: This method defines what an object looks like when you print it. It’s meant to give a more user-friendly, readable string version of the object.
class Course:
def __init__(self, name, instructor):
self.name = name
self.instructor = instructor
def __str__(self):
return f"{self.name} taught by {self.instructor}"
python_course = Course("Python Attributes and Methods", "Prof. Jane")
print(python_course) # Output: Python Attributes and Methods taught by Prof. Jane
__repr__
: This method is used for a more technical representation, which is often useful for debugging. While__str__
focuses on user-friendliness,__repr__
shows the internal details of the object.
class Course:
def __repr__(self):
return f"Course(name={self.name}, instructor={self.instructor})"
The Role of self
in the __init__ Method
The self
keyword in Python is crucial when working with classes. It refers to the current instance of the class and allows you to access its attributes and methods. Every time an object is created, self
points to that specific instance, enabling you to work with its unique data.
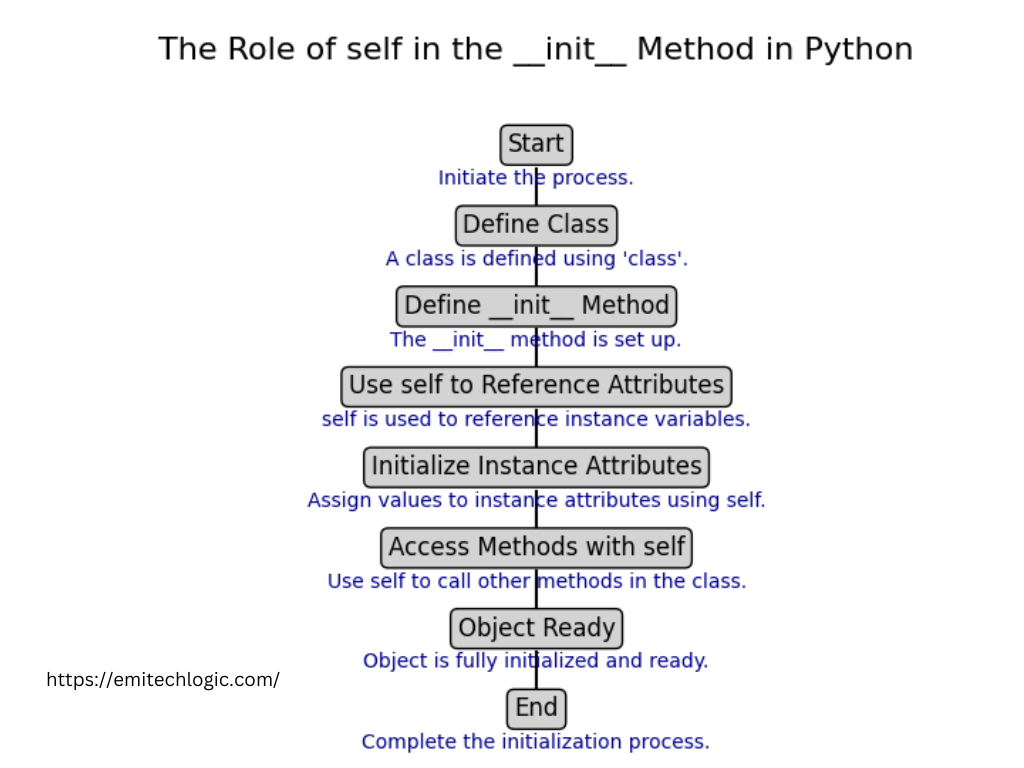
What is self
in Python Classes?
self
is automatically passed as the first argument to every method in a class, including the __init__
method. It acts like a bridge between the instance and its data. Without self
, Python wouldn’t know which instance’s attributes you’re trying to modify or access.
For example, in the earlier code snippets, you’ve seen lines like self.name = name
. The self.name
part refers to the name
attribute of the object you’re creating, and name
is the value being passed to the __init__
method when the object is initialized.
How to Use self
to Access Object Attributes
Using self
makes accessing and modifying attributes possible from within the class. It allows each instance of a class to maintain its own set of data, independent of other instances.
Let’s go back to our Student
class example:
class Student:
def __init__(self, name, student_id, courses):
self.name = name
self.student_id = student_id
self.courses = courses
def display_info(self):
return f"Student {self.name}, ID: {self.student_id}, Courses: {self.courses}"
student2 = Student("Alex", 102, ["Python Attributes and Methods", "AI Fundamentals"])
print(student2.display_info())
# Output: Student Alex, ID: 102, Courses: ['Python Attributes and Methods', 'AI Fundamentals']
In this example, self.name
, self.student_id
, and self.courses
allow us to access and work with those attributes for a specific instance (like student2
).
How to Define and Use the __init__
Method in Python
Syntax of the __init__
Method
The syntax of the __init__
method in Python is simple but crucial to understand because it’s how you define the initial attributes of any object created from a class. Here’s the basic syntax:
class ClassName:
def __init__(self, parameter1, parameter2, ...):
self.attribute1 = parameter1
self.attribute2 = parameter2
...
Let’s break this down:
def __init__(self, ...)
: The__init__
method is a special magic method that initializes the object. It always takesself
as the first parameter, which refers to the instance of the class.- Parameters: You can pass other parameters to the
__init__
method, just like any regular function. These parameters are then used to set the initial attributes of the object. - Attributes: The attributes are created using the
self
keyword. This ensures that the attributes belong to the specific instance of the class.
Basic Example of the __init__
Method in Python
Now, let’s look at a very basic example of the __init__
method. We’ll create a class to represent a book with attributes like title, author, and pages.
class Book:
def __init__(self, title, author, pages):
self.title = title
self.author = author
self.pages = pages
my_book = Book("Python Attributes and Methods", "Jane Doe", 350)
print(my_book.title) # Output: Python Attributes and Methods
print(my_book.author) # Output: Jane Doe
print(my_book.pages) # Output: 350
In this example, when we create my_book
, the __init__
method assigns values to the book’s title
, author
, and pages
. Without the __init__
method, we would need to manually set these values after creating the object, making the process more complicated.
Python Code Example for Beginners
To make this even more beginner-friendly, let’s walk through a simple use case that many students face. Imagine you want to create a student record that stores their name, grade, and subjects.
class Student:
def __init__(self, name, grade, subjects):
self.name = name
self.grade = grade
self.subjects = subjects
def show_info(self):
return f"{self.name} is in grade {self.grade} and takes the following subjects: {', '.join(self.subjects)}"
student = Student("Alice", 10, ["Math", "Science", "Python Attributes and Methods"])
print(student.show_info())
# Output: Alice is in grade 10 and takes the following subjects: Math, Science, Python Attributes and Methods
In this example, we not only used the __init__
method to initialize our Student
object, but we also added a method to display the student’s information.
This code shows how Python Attributes and Methods work hand-in-hand to make objects functional and meaningful. You can customize attributes and define actions with methods that operate on those attributes.
Common Use Cases for the __init__
Method

The __init__
method is essential whenever you want to initialize objects with unique, predefined values. Some common scenarios include:
- Creating User Profiles: When registering new users, the
__init__
method can automatically set up their username, email, and other details. - Setting Default Values: You can use
__init__
to assign default values for attributes, even if some data isn’t provided. - Managing Complex Data Structures: It helps to easily create instances of more complicated structures like graphs, trees, or databases.
Initializing Class Variables
In addition to instance variables (which belong to specific objects), Python also allows for class variables. Class variables are shared across all instances of a class, meaning they don’t change for each object unless explicitly modified.
Here’s an example that shows how class variables and instance variables differ:
class Library:
total_books = 0 # Class variable
def __init__(self, name, book_count):
self.name = name # Instance variable
self.book_count = book_count # Instance variable
Library.total_books += book_count # Modify class variable
lib1 = Library("Central Library", 300)
lib2 = Library("Westside Branch", 150)
print(Library.total_books) # Output: 450
In this example, total_books
is a class variable that tracks the total number of books across all libraries, while each library’s name and individual book count are instance variables.
Managing Default and Required Parameters
One of the great features of the __init__
method is the ability to handle both required parameters and default parameters. Required parameters must be provided when the object is created, while default parameters can be left out, as they automatically take on a default value.
Example with Default Parameters:
class Car:
def __init__(self, model, year=2020):
self.model = model
self.year = year
car1 = Car("Tesla Model S", 2022) # Providing both parameters
car2 = Car("Ford Mustang") # Only providing the model
print(car1.model, car1.year) # Output: Tesla Model S 2022
print(car2.model, car2.year) # Output: Ford Mustang 2020
In this case, if a year
isn’t provided, it defaults to 2020. This is useful when you want to make your classes more flexible and reduce the number of arguments users have to supply.
Creating Flexible Constructors with Optional Arguments
Sometimes you may want to create objects that can accept a varying number of parameters. Python allows you to create flexible constructors by using *args
and **kwargs
.
*args
allows you to pass a variable number of non-keyword arguments.**kwargs
allows you to pass a variable number of keyword arguments.
Here’s an example:
class Product:
def __init__(self, name, *args, **kwargs):
self.name = name
self.features = args
self.properties = kwargs
product = Product("Smartphone", "5G", "Dual SIM", color="Black", price=599)
print(product.name) # Output: Smartphone
print(product.features) # Output: ('5G', 'Dual SIM')
print(product.properties) # Output: {'color': 'Black', 'price': 599}
In this example, we created a Product
class that can accept any number of additional features (non-keyword arguments) and any number of properties (keyword arguments).
Must Read
- AI Pulse Weekly: December 2024 – Latest AI Trends and Innovations
- Can Google’s Quantum Chip Willow Crack Bitcoin’s Encryption? Here’s the Truth
- How to Handle Missing Values in Data Science
- Top Data Science Skills You Must Master in 2025
- How to Automating Data Cleaning with PyCaret
Advanced Concepts in the __init__
Method
Can You Have Multiple __init__
Methods in Python?
In Python, you cannot define multiple __init__
methods in the same class like you can in some other programming languages (e.g., Java or C++). If you try to define more than one __init__
method, Python will overwrite the previous one, leaving you with just the last defined version.
However, there are ways to mimic the behavior of multiple constructors by using conditional logic inside the __init__
method. This allows you to handle different kinds of inputs and still keep the initialization process flexible.
Using Conditional Logic in __init__
to Mimic Multiple Constructors
To create a flexible __init__
method that can handle different arguments, you can use conditional statements like if
, elif
, and else
. This technique allows you to perform different initializations based on the input.
Here’s an example:
class Person:
def __init__(self, name=None, age=None):
if name is not None and age is not None:
self.name = name
self.age = age
elif name is not None:
self.name = name
self.age = "Unknown"
else:
self.name = "Unknown"
self.age = "Unknown"
# Using different constructors
person1 = Person("Alice", 30)
person2 = Person("Bob")
person3 = Person()
print(person1.name, person1.age) # Output: Alice 30
print(person2.name, person2.age) # Output: Bob Unknown
print(person3.name, person3.age) # Output: Unknown Unknown
In this case, the Person
class uses a single __init__
method, but based on the arguments passed, it initializes different attributes. This gives us the flexibility of having multiple constructors without needing multiple methods.
Alternatives to Multiple __init__
Methods
If you need even more flexibility, you can use class methods or static methods to create alternative constructors. These methods can provide different ways to instantiate the class and offer more control over the object creation process.
Here’s an example using a class method:
class Circle:
def __init__(self, radius):
self.radius = radius
@classmethod
def from_diameter(cls, diameter):
radius = diameter / 2
return cls(radius)
# Two ways to create a Circle object
circle1 = Circle(5) # Using radius
circle2 = Circle.from_diameter(10) # Using diameter
print(circle1.radius) # Output: 5
print(circle2.radius) # Output: 5
This allows us to offer multiple ways to initialize a class without modifying the __init__
method. The from_diameter
class method provides an alternative constructor.
Overriding the __init__
Method in Inherited Classes
When working with inheritance, you often need to override the __init__
method of a parent class in a child class. The process of overriding allows you to modify the behavior of the parent class’s initialization while still retaining some of its properties.
For example, if you have a Vehicle
class and want to create a Car
class that inherits from it, you might want to override the __init__
method to add more attributes specific to the Car
class.
How to Use super()
in the __init__
Method
When overriding __init__
in a subclass, you often want to call the parent class’s __init__
method. This is where super()
comes into play. The super()
function lets you access methods of a parent class, allowing you to initialize the parent class’s attributes within the child class.
class Vehicle:
def __init__(self, make, model):
self.make = make
self.model = model
class Car(Vehicle):
def __init__(self, make, model, doors):
super().__init__(make, model) # Call parent class's __init__
self.doors = doors
car = Car("Tesla", "Model 3", 4)
print(car.make, car.model, car.doors) # Output: Tesla Model 3 4
In this example, Car
inherits from Vehicle
but adds an extra attribute, doors
. By using super()
, the Car
class can initialize both make
and model
from the Vehicle
class, while also setting its own unique attribute.
Example of Overriding the Parent Class Constructor
To further illustrate, let’s create a more advanced example. Imagine a base class Animal
that initializes common animal properties, and then a Dog
class that inherits from Animal
but adds a specific attribute for breed.
class Animal:
def __init__(self, species, legs):
self.species = species
self.legs = legs
class Dog(Animal):
def __init__(self, species, legs, breed):
super().__init__(species, legs)
self.breed = breed
dog = Dog("Canine", 4, "Labrador")
print(dog.species, dog.legs, dog.breed) # Output: Canine 4 Labrador
Here, Dog
calls the __init__
method of Animal
to set species
and legs
, while adding its own breed
attribute.
Best Practices for Writing __init__
Methods
Keeping your __init__
methods simple and clear is essential for writing maintainable code. Here are some best practices to follow:
- Keep Initialization Simple: Try not to overload the
__init__
method with too many responsibilities. If you have complex initialization logic, it’s better to break it into smaller methods. - Avoid Hardcoding Values: Use parameters to pass in values rather than hardcoding them inside the
__init__
method. This keeps the class flexible and reusable. - Use Default Values Carefully: Default values are helpful, but having too many can make your code harder to understand. It’s best to use them sparingly to keep your code clean.
- When overriding the
__init__
method in child classes, remember to callsuper()
. This is especially important in cases of multiple inheritance. Doing so ensures that the parent class is properly initialized.
Keeping __init__
Methods Simple and Clean
It’s easy to fall into the trap of adding too much logic to your __init__
methods. A good rule of thumb is to keep them as simple as possible by only handling the initial setup of an object. For anything more complex, create separate methods or use class methods for alternative constructors.
Avoiding Over-complicated Initialization Logic
Sometimes, trying to do too much inside the __init__
method can lead to over-complication. For instance, if your initialization requires a lot of calculations or conditional logic, it might be better to move that logic into another method. This keeps your __init__
method clean and easy to read.
Here’s a comparison:
Problematic __init__ | Cleaner __init__ |
---|---|
Overloaded with logic | Moves logic to another method |
Many conditional branches | Simple with clear intent |
Handles too many responsibilities | Focuses on initializing attributes |
By keeping your __init__
method concise, you ensure that your class is easier to understand and modify.
Common Errors in the __init__
Method
TypeErrors in the __init__
Method
When working with the __init__
method in Python, one common issue that may arise is a TypeError. This error often occurs when the number of arguments provided during the object creation does not match the parameters defined in the __init__
method. For instance, if you expect two arguments but only provide one, Python will raise a TypeError.
Here’s an example to illustrate this:
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
# Attempting to create a Book object with only one argument
book1 = Book("1984") # This will raise a TypeError
In this case, the error message will indicate that two positional arguments were expected but only one was given. It’s a helpful reminder to check your parameter requirements.
AttributeError When Using __init__
Improperly
Another common error related to the __init__
method is the AttributeError. This occurs when you try to access an attribute that has not been initialized or defined. If your __init__
method doesn’t set up certain attributes, any attempt to access those attributes later will lead to an AttributeError.
Here’s an example:
class Car:
def __init__(self, make, model):
self.make = make
# self.model is not initialized here
car1 = Car("Toyota", "Camry")
print(car1.model) # This will raise an AttributeError
In this example, the model
attribute was never defined, leading to an error when trying to access it. To avoid this, always ensure that all expected attributes are initialized in the __init__
method.
Debugging the __init__
Method in Python
Debugging the __init__
method can sometimes be tricky, especially for beginners. However, with a few strategies, it can be made easier. Here are some tips to help you debug issues effectively:
- Read Error Messages Carefully: Python’s error messages often provide a lot of information. They can guide you to the line of code causing the issue and help identify the type of error.
- Use Print Statements: Adding print statements in your
__init__
method can help you trace the flow of execution. For example, print the parameters received and the attributes being set:
class User:
def __init__(self, username, email):
print(f"Initializing User with username: {username} and email: {email}")
self.username = username
self.email = email
3. Check Parameter Types: If your method expects specific types (like integers or strings), ensure that the values being passed match those types. Using type()
can help verify this:
if not isinstance(username, str):
raise TypeError("Username must be a string")
4. Use a Debugger: Tools like Python’s built-in debugger (pdb
) can step through your code line by line. This allows you to inspect variable values and see where things might be going wrong.
Tips for Identifying and Fixing Errors
Here are some practical tips for identifying and fixing common errors related to the __init__
method:
- Consistency in Parameters: Always ensure that the number of parameters in your
__init__
method matches the number of arguments provided when creating an object. Consistent naming can also help avoid confusion. - Default Values: Use default parameter values when appropriate. This can prevent errors if an argument is not supplied:
class User:
def __init__(self, username, email="not_provided@example.com"):
self.username = username
self.email = email
3. Documentation: Clearly document what parameters the __init__
method requires. This helps users of your class understand how to use it correctly and can prevent mistakes.
4. Test Cases: Writing simple test cases for your classes can help catch errors early. Ensure you test various scenarios, including edge cases, to see how your class behaves.
5. Error Handling: Implement error handling in your __init__
method. Using try
and except
blocks can help you manage unexpected inputs gracefully:
class User:
def __init__(self, username, email):
try:
self.username = username
self.email = email
except Exception as e:
print(f"Error initializing User: {e}")
Recent Advancements Related to the __init__
Method in Python
Improvements in Python 3.x That Impact the __init__
Method
Python 3 introduced several enhancements that significantly affect how the __init__
method works in class initialization. These changes not only improve functionality but also enhance code clarity. One notable change was the introduction of dataclasses, which simplify class definitions, especially when many attributes are involved.
Python 3.8+ Features and Their Effects on Class Initialization
In Python 3.8 and later, several features were added that can impact the way you write your __init__
methods:
- Positional-only Parameters: This feature allows you to define parameters that can only be specified positionally, not as keyword arguments. This is done using a
/
in the function signature. It can lead to clearer APIs, especially in initialization methods.
class Book:
def __init__(self, title, author, /): # title and author must be positional
self.title = title
self.author = author
2. F-strings for String Interpolation: Introduced in Python 3.6 but refined in later versions, f-strings make it easier to format strings, which can be useful in the __init__
method when creating informative messages or logs.
class User:
def __init__(self, username):
self.username = username
print(f"User created: {self.username}")
Dataclasses and the __init__
Method
The dataclass module introduced in Python 3.7 has revolutionized how classes can be created. It allows for automatic generation of special methods, including __init__
, making class definitions cleaner and more concise.
Simplifying Class Initialization with Python’s Dataclass Module
With a dataclass, you no longer need to write boilerplate code for your __init__
method. Here’s how a typical class can be transformed:
from dataclasses import dataclass
@dataclass
class User:
username: str
email: str
# Automatically generated __init__ method
user1 = User("alice", "alice@example.com")
This code is simpler and focuses on the attributes rather than the boilerplate code that typically accompanies a standard class definition. The dataclass
automatically creates the __init__
method, reducing potential errors and improving maintainability.
How Dataclass Auto-Generates the __init__
Method
When you use the @dataclass
decorator, Python creates the __init__
method based on the class attributes. This method handles type hints and default values automatically, which is especially useful when dealing with multiple attributes.
Here’s an example illustrating this:
from dataclasses import dataclass
@dataclass
class Product:
name: str
price: float
quantity: int = 1 # Default value
# This automatically generated __init__ method is equivalent to:
# def __init__(self, name: str, price: float, quantity: int = 1):
# self.name = name
# self.price = price
# self.quantity = quantity
product1 = Product("Laptop", 999.99)
This simplicity encourages cleaner and more readable code, which is essential in any programming endeavor.
Type Hints in the __init__
Method (Python 3.5+)
Type hints were introduced in Python 3.5, providing a way to indicate the expected data types of parameters and return values. This feature significantly enhances code readability and debugging.
Adding Type Hints to Improve Readability and Debugging
By adding type hints to the __init__
method, you make the intentions clear. Here’s how this can be done:
class Car:
def __init__(self, make: str, model: str, year: int) -> None:
self.make = make
self.model = model
self.year = year
Using type hints allows developers and IDEs to catch type-related errors early, enhancing the overall development experience. For instance, if a string is passed where an integer is expected, a static type checker like mypy will flag the error before runtime.
Python 3.11 and Beyond: Latest Developments in Static Typing
With the introduction of Python 3.11, enhancements in static typing have continued to evolve. The focus has been on improving performance and error detection.
- Better Error Messages: The updates in 3.11 offer clearer and more informative error messages. This feature greatly helps in debugging, especially when issues arise in the
__init__
method. - Type Variables and Protocols: These features enhance flexibility in function signatures, making it easier to write generic code that can still benefit from type checking.
How to Test the __init__
Method in Python
Writing Unit Tests for the __init__
Method
Unit testing is a vital part of software development, ensuring that each component of your application works as intended. When it comes to the __init__
method, unit tests play a crucial role in verifying that your class initializes correctly.
Testing the __init__
method helps catch errors related to attribute assignments, default values, and type mismatches early in the development process. Let’s explore how to effectively write unit tests for the __init__
method.
Creating Unit Tests
To test the __init__
method, a popular framework in Python is unittest. Here’s how you can create tests for a simple class.
import unittest
class User:
def __init__(self, username: str, email: str):
self.username = username
self.email = email
class TestUser(unittest.TestCase):
def test_initialization(self):
user = User("alice", "alice@example.com")
self.assertEqual(user.username, "alice")
self.assertEqual(user.email, "alice@example.com")
if __name__ == "__main__":
unittest.main()
In this example, the test_initialization
method checks that the attributes are set correctly upon instantiation. Assertions are used to verify that the values match expected results.
Mocking and Patching the __init__
Method in Unit Tests
Sometimes, the __init__
method might contain logic that interacts with external systems, such as databases or APIs. In such cases, you may want to use mocking to isolate the tests.
The unittest.mock
module allows you to replace parts of your system under test and make assertions about how they have been used. Here’s an example of how to mock the __init__
method:
from unittest.mock import patch
class Database:
def __init__(self, connection_string: str):
self.connection_string = connection_string
class TestDatabase(unittest.TestCase):
@patch('__main__.Database.__init__', return_value=None)
def test_database_initialization(self, mock_init):
db = Database("fake_connection_string")
mock_init.assert_called_once_with("fake_connection_string")
if __name__ == "__main__":
unittest.main()
In this case, the __init__
method is mocked, allowing you to test whether it was called with the correct parameters without actually executing its code. This approach can be incredibly helpful for avoiding side effects during tests.
Best Practices for Ensuring Your __init__
Method Works Properly
When writing tests for the __init__
method, consider these best practices:
- Test Different Scenarios: Cover normal cases, edge cases, and invalid inputs. This ensures that your class behaves as expected under various conditions.
- Use Clear Assertions: Make sure your assertions clearly state what you expect. This helps maintain readability and makes it easier to identify issues later on.
- Keep Tests Independent: Each test should be able to run independently of others. This isolation makes it easier to diagnose failures.
- Document Your Tests: Include comments explaining the purpose of each test case. This aids future developers in understanding the reasoning behind each test.
Handling Edge Cases in Initialization
Edge cases often reveal bugs that might not be apparent in normal usage. Here are some examples of edge cases to consider when testing the __init__
method:
- Empty Strings: Test how the class handles empty strings or None values.
class User:
def __init__(self, username: str, email: str):
if not username or not email:
raise ValueError("Username and email must not be empty.")
self.username = username
self.email = email
class TestUserEdgeCases(unittest.TestCase):
def test_empty_username(self):
with self.assertRaises(ValueError):
User("", "test@example.com")
if __name__ == "__main__":
unittest.main()
- Invalid Types: Check how the class reacts when given incorrect data types.
def test_invalid_email(self):
with self.assertRaises(TypeError):
User("alice", 123) # email should be a string
Real-World Use Cases of the __init__
Method
Using __init__
in Django Models
In Django, a popular web framework for Python, the __init__
method plays a crucial role in defining models. Django models are classes that define the structure of your database tables. They also allow for easy interaction with the database.
When creating a model, the __init__
method can be customized to initialize attributes or perform specific actions when a model instance is created.
Example of a Django Model with __init__
Here’s a simple example of how to use the __init__
method in a Django model:
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=100)
price = models.DecimalField(max_digits=10, decimal_places=2)
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.price_with_tax = self.calculate_tax()
def calculate_tax(self):
return self.price * 1.2 # Assuming a 20% tax rate
In this code, the __init__
method is overridden to calculate the price with tax every time a Product
instance is created. This is a good example of how Python Attributes and Methods
can enhance functionality within Django.
Initialization in Python Web Frameworks
In various Python web frameworks, the __init__
method is used to set up configurations and manage the application’s behavior. Frameworks like Flask and FastAPI also utilize the __init__
method to initialize objects and manage application settings.
Applying __init__
in Machine Learning Models
In machine learning, models can be created as classes where the __init__
method is used to set hyperparameters. This approach helps in managing the configuration of your models in a clean way.
Example of a Machine Learning Model
Here’s a basic example of how you might implement a machine learning model class:
class LinearRegression:
def __init__(self, learning_rate=0.01, num_iterations=1000):
self.learning_rate = learning_rate
self.num_iterations = num_iterations
self.weights = None
def fit(self, X, y):
# Implementation for fitting the model goes here
pass
In this example, the __init__
method initializes the learning rate and the number of iterations for training. This setup ensures that your model can be configured easily before training begins.
Setting Up Model Hyperparameters Using __init__
Setting hyperparameters through the __init__
method allows for flexibility and customization. For instance, when initializing a model, users can specify values that fit their particular data set or problem domain.
Example with Hyperparameters
class DecisionTree:
def __init__(self, max_depth=None, min_samples_split=2):
self.max_depth = max_depth
self.min_samples_split = min_samples_split
def train(self, X, y):
# Code for training the decision tree goes here
pass
In this code, hyperparameters such as max_depth
and min_samples_split
can be specified when creating an instance of the DecisionTree
class. This allows users to easily adjust the behavior of the model.
__init__
Method in Flask Applications
Flask, another popular web framework, also makes use of the __init__
method to manage application settings. By initializing application settings in the __init__
method, developers can keep their code organized and easy to maintain.
Using __init__
to Manage Flask Application Settings
Here’s an example of how to use the __init__
method in a Flask application:
from flask import Flask
class MyApp:
def __init__(self, config_filename):
self.app = Flask(__name__)
self.app.config.from_pyfile(config_filename)
self.setup_routes()
def setup_routes(self):
@self.app.route('/')
def home():
return "Welcome to My Flask App!"
In this example, the __init__
method initializes the Flask application and loads configurations from a file. This organization of code improves readability and maintainability.
Common Pitfalls and How to Avoid Them
Working with the __init__
method in Python is essential for creating well-structured classes. However, several common pitfalls can lead to issues that may affect your code’s readability and functionality. Understanding these pitfalls can help you create cleaner, more effective code. Let’s explore some of the most common mistakes and how to avoid them.
Over-complicating the __init__
Method
One major pitfall is over-complicating the __init__
method. When a method becomes too complex, it becomes difficult to understand and maintain. It can be tempting to cram too much logic into __init__
, especially when trying to set up multiple attributes or conditions.
Example of an Over-complicated __init__
Method:
class User:
def __init__(self, username, email, age, is_active=True, last_login=None):
self.username = username
self.email = email
self.age = age
self.is_active = is_active
if last_login:
self.last_login = last_login
else:
self.last_login = "Never"
# Additional logic for setting up user roles
if email.endswith('@admin.com'):
self.role = 'admin'
else:
self.role = 'user'
In this example, the __init__
method has multiple responsibilities. It sets user attributes, determines the user’s role, and manages default values. This can lead to confusion, especially for others reading the code.
Solution: Keep the __init__
method focused on initialization. If more logic is needed, consider moving it to separate methods.
class User:
def __init__(self, username, email, age, is_active=True, last_login=None):
self.username = username
self.email = email
self.age = age
self.is_active = is_active
self.last_login = last_login if last_login else "Never"
self.role = self.assign_role()
def assign_role(self):
return 'admin' if self.email.endswith('@admin.com') else 'user'
This separation clarifies the responsibilities of each method, making the code easier to understand.
Misusing the __init__
Method for Non-Initialization Tasks
Another common mistake is using the __init__
method for tasks that do not belong in an initializer. The purpose of __init__
is to set up the initial state of an object. Using it to perform actions, like making API calls or starting threads, can lead to unexpected behavior and difficulties in testing.
Example of Misusing __init__
:
class DatabaseConnection:
def __init__(self, db_url):
self.connection = self.connect_to_database(db_url)
def connect_to_database(self, db_url):
# Code to connect to the database
print(f"Connecting to {db_url}...")
return True # Assume connection is successful
Here, the __init__
method initiates a connection to the database, which is not just about initializing the object. This can lead to problems, especially if the connection fails.
Solution: Instead, you should create a separate method for such actions.
class DatabaseConnection:
def __init__(self, db_url):
self.db_url = db_url
self.connection = None
def connect(self):
self.connection = self.connect_to_database(self.db_url)
def connect_to_database(self, db_url):
print(f"Connecting to {db_url}...")
return True # Assume connection is successful
In this version, the connection logic is moved to the connect
method, allowing for better control over the connection process.
Avoiding Circular Dependencies in __init__
Circular dependencies can occur when two or more classes depend on each other during initialization. This can lead to errors and can make debugging challenging.
Example of Circular Dependency:
class ClassA:
def __init__(self):
self.class_b = ClassB()
class ClassB:
def __init__(self):
self.class_a = ClassA() # This creates a circular reference
In this example, both classes attempt to create instances of each other, leading to a loop that can result in a stack overflow.
Solution: Refactor your code to avoid circular dependencies. Use design patterns like dependency injection to manage dependencies more effectively.
class ClassA:
def __init__(self, class_b):
self.class_b = class_b
class ClassB:
def __init__(self):
pass
# Example of creating instances without circular dependencies
b = ClassB()
a = ClassA(b)
In this revised code, ClassA
depends on an instance of ClassB
, breaking the circular reference and making the design cleaner.
Best Practices and Tips for Optimizing the __init__
Method
Creating effective classes in Python often starts with writing a clean and efficient __init__
method. This method plays a crucial role in initializing your objects, setting their state, and ensuring they are ready for use. Here are some best practices and tips to help you optimize your __init__
method, making it not only functional but also elegant.
Keep the Initialization Lightweight
One of the fundamental principles when writing the __init__
method is to keep it lightweight. This means avoiding heavy computations or complex logic during the initialization process. When too much work is done inside __init__
, it can slow down object creation and lead to performance issues.
Example of a Heavy __init__
Method:
class DataProcessor:
def __init__(self, data):
self.data = data
self.cleaned_data = self.clean_data(data)
self.results = self.analyze_data(self.cleaned_data)
def clean_data(self, data):
# Code for cleaning data
return data
def analyze_data(self, cleaned_data):
# Code for analyzing data
return cleaned_data
In this example, the __init__
method is overloaded with data cleaning and analysis, making object instantiation heavy and potentially slow.
Solution: Instead, focus on initializing only the necessary attributes in the __init__
method. Move the heavier operations to separate methods that can be called after the object has been created.
class DataProcessor:
def __init__(self, data):
self.data = data
self.cleaned_data = None
self.results = None
def process(self):
self.cleaned_data = self.clean_data(self.data)
self.results = self.analyze_data(self.cleaned_data)
def clean_data(self, data):
return data
def analyze_data(self, cleaned_data):
return cleaned_data
Now, the __init__
method is lightweight, allowing for faster object creation. The processing can be handled later by calling the process
method, ensuring that the object is ready for use without delays.
Use Default Parameters Wisely
Default parameters can make your __init__
method more flexible and user-friendly. They allow users to create instances with fewer arguments while still providing sensible defaults. However, it’s important to use them wisely to avoid confusion.
Example of Using Default Parameters:
class User:
def __init__(self, username, email, is_active=True):
self.username = username
self.email = email
self.is_active = is_active
# Creating a user with default is_active
user1 = User("john_doe", "john@example.com")
user2 = User("jane_doe", "jane@example.com", is_active=False)
In this example, the is_active
parameter has a default value of True
. This design allows users to instantiate a User
without explicitly specifying this parameter, making the code cleaner and more intuitive.
Tip: When using default parameters, ensure that they make sense in the context of the class. If the defaults can lead to confusion or misinterpretation, it may be better to require the parameter explicitly.
Document Your __init__
Method for Clarity
Proper documentation of the __init__
method is crucial for clarity and usability. Comments and docstrings help other developers (and future you) understand the purpose and usage of each parameter. Clear documentation can save time and prevent errors down the line.
Example of Documenting __init__
:
class Book:
def __init__(self, title, author, year_published, genre=None):
"""
Initialize a new Book instance.
:param title: The title of the book.
:param author: The author of the book.
:param year_published: The year the book was published.
:param genre: The genre of the book (optional).
"""
self.title = title
self.author = author
self.year_published = year_published
self.genre = genre if genre else "Unknown"
In this example, the docstring clearly describes what each parameter represents, making it easier for anyone to understand how to use the Book
class. Good documentation should answer questions like:
- What does this method do?
- What are the parameters, and what do they mean?
- Are there any default values?
Frequently Asked Questions About the __init__
Method
__init__
Method Mandatory in Python Classes? No, the __init__
method is not mandatory in Python classes. If it is not defined, Python will automatically create a default initializer that does nothing. However, if you need to initialize attributes when creating an object, defining __init__
is essential.
__init__
Method Manually? Yes, you can call the __init__
method manually. However, it is generally not recommended to do so. Instead, it’s best practice to create an instance of the class, which automatically calls __init__
. If you need to reinitialize an object, consider using a separate method for that purpose.
__init__
Different From __call__
? The __init__
method is used for initializing a new instance of a class, setting up its attributes. In contrast, the __call__
method allows an instance of a class to be called like a function. While __init__
is executed when an object is created, __call__
is executed when the object itself is called.
Conclusion: Mastering the __init__
Method in Python
Mastering the __init__
method is essential for any Python developer looking to build effective and efficient applications. This method serves as the cornerstone of object creation, providing a way to initialize attributes and ensure that objects are ready for use right from the start.
Key Takeaways for Python Developers
- Importance of Initialization: The
__init__
method allows you to set up initial states for your objects, making them functional and reliable. Proper initialization prevents errors and ensures that your classes behave as expected. - Design for Clarity: Keeping your
__init__
method lightweight and easy to understand is crucial. Use default parameters judiciously and provide clear documentation. This practice enhances code readability and makes it easier for others (and yourself) to work with your classes in the future. - Flexibility in Usage: While the
__init__
method is key, it’s not the only tool in your toolbox. Understand when to use it, when to call it manually, and how it interacts with other magic methods like__call__
. This flexibility is vital for creating robust Python applications.
The Role of __init__
in Building Reliable and Scalable Python Applications
In the realm of reliable and scalable applications, the __init__
method plays a critical role. By ensuring that objects are properly initialized, developers can avoid common pitfalls that lead to bugs and inefficiencies. A well-designed __init__
method sets the stage for clean, maintainable code, allowing for easier upgrades and expansions in the future.
Ultimately, mastering the __init__
method contributes to your overall skill set as a Python developer. With a solid grasp of this foundational aspect, you will be better equipped to create applications that are not only functional but also strong and easy to maintain.
External Resources
Python Documentation – Data Model
This section of the official Python documentation provides an overview of magic methods, including __init__
. It explains how __init__
is used for object initialization.
Python Data Model Documentation
Python Official Tutorial – Classes
The official Python tutorial covers the basics of classes, including the __init__
method. It offers examples and explanations to help beginners understand class construction.
Python Classes Tutorial