Introduction
Welcome back to our exploration of Data Types in Python ! In Part 1, we covered the basics, focusing on the essential numeric and sequence data types that are key to everyday programming. Now, in Part 2, we’re moving on to more advanced topics. This section will introduce you to mapping and set data types, along with some of Python’s more specialized types. By understanding these, you’ll not only improve your coding skills but also be better equipped to handle more complex programming tasks.
We’ll begin with Python’s powerful dictionary and set data types, which are great for organizing data in ways that allow for quick lookups and ensuring uniqueness. After that, we’ll explore frozensets, an immutable version of sets that adds another layer of functionality. Next, we’ll look at advanced data types like bytes and bytearrays for managing memory, NoneType for representing the absence of a value, and how to create your own custom data types.
But that’s not all! This guide also shows you how to apply these data types in real-world scenarios like web development, data science, database management, and automation. By the end of Part 2, you’ll have a solid understanding of when and how to use these data types effectively in your projects. Whether you’re building complex systems or simply fine-tuning your code, mastering these data types is essential for becoming a skilled Python programmer.
Let’s explore Python’s mapping and set data types!
Mapping and Set Data Types in Python
In Python, mapping and set data types are powerful tools for handling collections of data in a structured way. One of the most commonly used mapping types is the dictionary, while sets provide a unique way to handle collections of unique items. Let’s focus on dictionaries and how they work, as well as their practical applications.
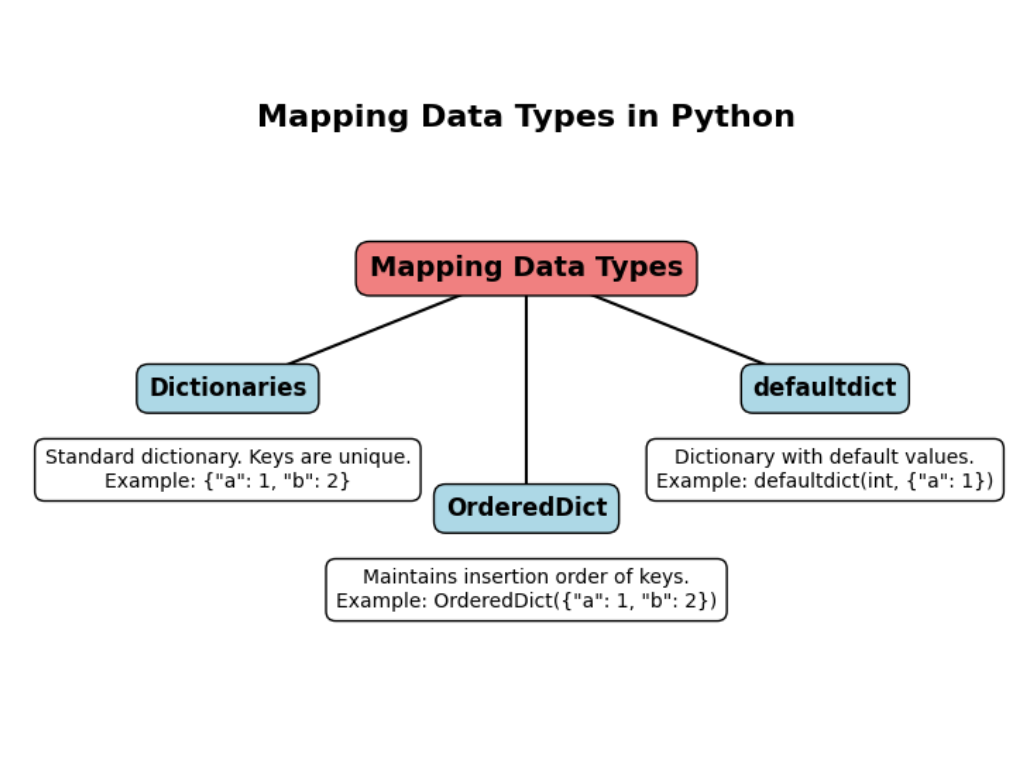
Python Dictionary Data Type
A dictionary in Python is a adaptable data structure used to store data in a format known as key-value pairs. Think of a dictionary as a real-life dictionary where you look up a word (key) to find its definition (value). In Python, this concept translates to using a key to access a specific value in a collection.
Introduction to Dictionaries and Key-Value Pairs
In Python, dictionaries are created using curly braces {}
with key-value pairs separated by colons. Each key is unique, and it maps to a specific value. This makes dictionaries incredibly useful for looking up data efficiently. Here’s a basic example:
my_dict = {
"name": "Alice",
"age": 30,
"city": "New York"
}
print(my_dict) # Output: {'name': 'Alice', 'age': 30, 'city': 'New York'}
In this example, "name"
, "age"
, and "city"
are the keys, and "Alice"
, 30
, and "New York"
are their corresponding values.
Dictionary Operations and Methods
Dictionaries come with a variety of operations and methods that make them extremely flexible. Here’s a closer look at some common dictionary operations:
- Accessing Values:You can access a value by referring to its key:
print(my_dict["name"]) # Output: Alice
2. Adding and Updating Key-Value Pairs:
You can add new key-value pairs or update existing ones:
my_dict["email"] = "alice@example.com" # Adding a new key-value pair
my_dict["age"] = 31 # Updating the value for the existing key "age"
print(my_dict) # Output: {'name': 'Alice', 'age': 31, 'city': 'New York', 'email': 'alice@example.com'}
3. Removing Key-Value Pairs:
To remove a key-value pair, use the del
statement or the pop()
method:
del my_dict["city"] # Removing a key-value pair using del
print(my_dict) # Output: {'name': 'Alice', 'age': 31, 'email': 'alice@example.com'}
email = my_dict.pop("email") # Removing a key-value pair using pop and capturing the value
print(email) # Output: alice@example.com
4. Checking for Key Existence:
You can check if a key exists in the dictionary using the in
keyword:
print("name" in my_dict) # Output: True
print("city" in my_dict) # Output: False
5. Iterating Over Dictionary Items:
Dictionaries allow you to loop through keys, values, or key-value pairs:
for key in my_dict:
print(key, my_dict[key])
# Output:
# name Alice
# age 31
for key, value in my_dict.items():
print(key, value)
# Output:
# name Alice
# age 31
6. Dictionary Methods:
.keys()
: Returns a view object of all keys..values()
: Returns a view object of all values..items()
: Returns a view object of all key-value pairs.
print(my_dict.keys()) # Output: dict_keys(['name', 'age'])
print(my_dict.values()) # Output: dict_values(['Alice', 31])
print(my_dict.items()) # Output: dict_items([('name', 'Alice'), ('age', 31)])
Example and Output for Dictionary Manipulations
Let’s put these concepts into a practical example. Suppose you are building a contact list and need to store details for each person:
contacts = {
"John": {"phone": "555-1234", "email": "john@example.com"},
"Jane": {"phone": "555-5678", "email": "jane@example.com"}
}
# Adding a new contact
contacts["Doe"] = {"phone": "555-8765", "email": "doe@example.com"}
# Updating an existing contact
contacts["John"]["email"] = "john.doe@example.com"
# Removing a contact
del contacts["Jane"]
# Iterating through the contacts
for name, info in contacts.items():
print(f"Contact: {name}")
print(f"Phone: {info['phone']}")
print(f"Email: {info['email']}")
print()
Output:
Contact: John
Phone: 555-1234
Email: john.doe@example.com
Contact: Doe
Phone: 555-8765
Email: doe@example.com
In this example:
- We started with a dictionary called
contacts
where each contact’s name is a key, and the value is another dictionary containing phone and email. - We added a new contact, updated an existing one, and removed a contact.
- Finally, we iterated through the dictionary to print out each contact’s details.
Summary
Python dictionaries are a flexible and powerful way to store and manipulate data using key-value pairs. They are ideal for scenarios where you need to quickly access, update, or manage data based on unique identifiers. Understanding how to use dictionaries effectively will enhance your ability to handle complex data structures and write more efficient Python code.
Python Set Data Type
In Python, sets are a unique and valuable data structure used to manage collections of distinct items. Unlike lists or dictionaries, sets are designed to handle only unique elements and are ideal when you need to perform operations involving collections of items without duplicates.
What Are Sets in Python?
A set in Python is an unordered collection of unique elements. This means that each item in a set appears only once, and the order of items is not guaranteed. Sets are great for operations like finding common items between collections, removing duplicates from a list, and more.
Here’s how you can create a set in Python:
my_set = {1, 2, 3, 4}
print(my_set) # Output: {1, 2, 3, 4}
In this example, my_set
contains four unique numbers. If you try to add a duplicate item, it won’t be included:
my_set.add(2)
print(my_set) # Output: {1, 2, 3, 4} (no change)
Set Operations and Methods
Sets come with a variety of operations and methods that make them useful for various programming tasks. Here’s a closer look at some of the most common set operations and methods:
1. Adding Elements:
You can add items to a set using the add()
method. Note that adding a duplicate item has no effect:
my_set.add(5)
print(my_set) # Output: {1, 2, 3, 4, 5}
2. Removing Elements:
To remove items from a set, use the remove()
method. If the item is not present, remove()
will raise an error. Alternatively, you can use the discard()
method, which will not raise an error if the item is not found:
my_set.remove(3)
print(my_set) # Output: {1, 2, 4, 5}
my_set.discard(10) # No error if 10 is not present
You can also use pop()
to remove and return an arbitrary item from the set:
item = my_set.pop()
print(item) # Output: (any item from the set)
print(my_set) # Output: (set with one less item)
3. Set Operations:
Sets support various mathematical operations such as union, intersection, and difference:
- Union (
|
orunion()
): Combines elements from two sets:
set_a = {1, 2, 3}
set_b = {3, 4, 5}
union_set = set_a | set_b
print(union_set) # Output: {1, 2, 3, 4, 5}
- Intersection (
&
orintersection()
): Finds elements common to both sets:
intersection_set = set_a & set_b
print(intersection_set) # Output: {3}
- Difference (
-
ordifference()
): Finds elements in one set but not in another:
difference_set = set_a - set_b
print(difference_set) # Output: {1, 2}
- Symmetric Difference (
^
orsymmetric_difference()
): Finds elements in either of the sets but not in both:
symmetric_difference_set = set_a ^ set_b
print(symmetric_difference_set) # Output: {1, 2, 4, 5}
4. Checking Membership:
You can check if an item is in a set using the in
keyword:
print(1 in my_set) # Output: True
print(6 in my_set) # Output: False
5. Set Methods:
.copy()
: Returns a shallow copy of the set..clear()
: Removes all items from the set
copied_set = my_set.copy()
print(copied_set) # Output: (copy of my_set)
my_set.clear()
print(my_set) # Output: set() (empty set)
Example and Output for Set Manipulations
Let’s see these concepts in action with a practical example. Suppose you want to manage a list of unique student IDs:
# Create a set of student IDs
student_ids = {101, 102, 103, 104}
print("Initial set:", student_ids) # Output: {101, 102, 103, 104}
# Add a new student ID
student_ids.add(105)
print("After adding 105:", student_ids) # Output: {101, 102, 103, 104, 105}
# Remove a student ID
student_ids.remove(102)
print("After removing 102:", student_ids) # Output: {101, 103, 104, 105}
# Check if an ID is in the set
print("Is 104 in the set?", 104 in student_ids) # Output: True
# Perform set operations
set_a = {1, 2, 3}
set_b = {3, 4, 5}
print("Union:", set_a | set_b) # Output: {1, 2, 3, 4, 5}
print("Intersection:", set_a & set_b) # Output: {3}
print("Difference:", set_a - set_b) # Output: {1, 2}
print("Symmetric Difference:", set_a ^ set_b) # Output: {1, 2, 4, 5}
In this example, you manage a set of student IDs, perform several operations, and check membership. You also use set operations to combine and compare sets of numbers.
Output
Initial set: {104, 101, 102, 103}
After adding 105: {101, 102, 103, 104, 105}
After removing 102: {101, 103, 104, 105}
Is 104 in the set? True
Union: {1, 2, 3, 4, 5}
Intersection: {3}
Difference: {1, 2}
Symmetric Difference: {1, 2, 4, 5}
Process finished with exit code 0
Python Frozenset Data Type
In Python, a frozenset is a special type of set that, unlike regular sets, is immutable. This means once you create a frozenset, you cannot modify its elements—no adding, removing, or changing items. This immutability makes frozensets a unique and valuable tool in programming, especially when you need a set of unique items that should remain constant.
Introduction to Frozensets and Their Immutability
A frozenset is similar to a regular set in Python, but with one crucial difference: it is immutable. Once a frozenset is created, it cannot be altered. This immutability ensures that the data within a frozenset remains constant throughout the program, which can be useful in various situations where data integrity is important.
Here’s how you can create a frozenset:
my_frozenset = frozenset([1, 2, 3, 4])
print(my_frozenset) # Output: frozenset({1, 2, 3, 4})
In this example, my_frozenset
contains four unique numbers. You cannot change this frozenset after its creation, which is different from a regular set where you can add or remove items.
When to Use Frozensets in Programming
Frozensets are useful in situations where you need a set-like collection of items that should not change. Here are some common scenarios where frozensets are beneficial:
- As Dictionary Keys: Since frozensets are immutable, they can be used as keys in dictionaries, unlike regular sets. This is useful when you need to associate values with unchanging collections of data.
my_dict = {frozenset([1, 2]): "Value1", frozenset([3, 4]): "Value2"}
print(my_dict[frozenset([1, 2])]) # Output: Value1
2. As Elements of Other Sets: You can include frozensets as elements within another set. This is not possible with regular sets due to their mutability.
outer_set = {frozenset([1, 2]), frozenset([3, 4])}
print(outer_set) # Output: {frozenset({1, 2}), frozenset({3, 4})}
3. Ensuring Data Integrity: Use frozensets when you need to ensure that a collection of items remains constant throughout your program. This can help prevent accidental changes and maintain consistency.
Example and Output for Frozenset Operations
Let’s see some basic operations with frozensets to understand how they work:
1. Creating a Frozenset:
numbers = frozenset([1, 2, 3, 4])
print(numbers) # Output: frozenset({1, 2, 3, 4})
2. Attempting to Modify a Frozenset:
Since frozensets are immutable, trying to add or remove elements will result in an error:
try:
numbers.add(5)
except AttributeError as e:
print(e) # Output: 'frozenset' object has no attribute 'add'
try:
numbers.remove(2)
except AttributeError as e:
print(e) # Output: 'frozenset' object has no attribute 'remove'
3. Set Operations with Frozensets:
Frozensets support set operations similar to regular sets, but since they are immutable, you cannot modify them directly:
- Union (
|
orunion()
):
set_a = frozenset([1, 2, 3])
set_b = frozenset([3, 4, 5])
union_set = set_a | set_b
print(union_set) # Output: frozenset({1, 2, 3, 4, 5})
- Intersection (
&
orintersection()
):
intersection_set = set_a & set_b
print(intersection_set) # Output: frozenset({3})
- Difference (
-
ordifference()
):
difference_set = set_a - set_b
print(difference_set) # Output: frozenset({1, 2})
- Symmetric Difference (
^
orsymmetric_difference()
):
symmetric_difference_set = set_a ^ set_b
print(symmetric_difference_set) # Output: frozenset({1, 2, 4, 5})
4. Checking Membership:
You can check if an item is in a frozenset in the same way as with regular sets:
print(1 in numbers) # Output: True
print(5 in numbers) # Output: False
5. Copying a Frozenset:
You can create a shallow copy of a frozenset:
copied_frozenset = numbers.copy()
print(copied_frozenset) # Output: frozenset({1, 2, 3, 4})
Frozensets Data Types in Python offer a unique way to handle sets of immutable data. They ensure that once created, the elements within a frozenset cannot be modified, providing stability and consistency. Use frozensets when you need unchanging sets for dictionary keys, as elements in other sets, or to maintain data integrity throughout your program. Understanding how to use frozensets can enhance your ability to manage collections of data effectively and efficiently in Python.
Must Read
- AI Pulse Weekly: December 2024 – Latest AI Trends and Innovations
- Can Google’s Quantum Chip Willow Crack Bitcoin’s Encryption? Here’s the Truth
- How to Handle Missing Values in Data Science
- Top Data Science Skills You Must Master in 2025
- How to Automating Data Cleaning with PyCaret
Advanced Data Types and Their Applications
Python Byte and Bytearray Data Types
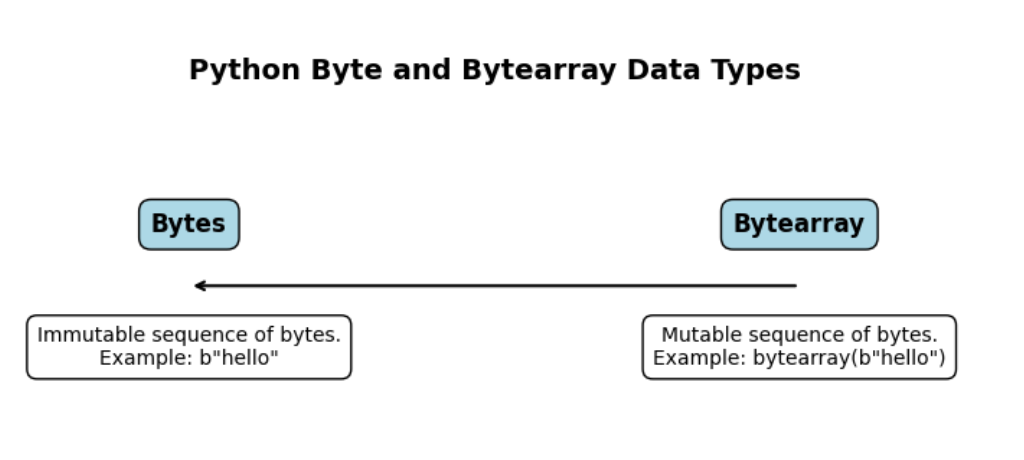
When working with Data Types in Python, especially in applications that require efficient memory management or low-level manipulation, understanding byte and bytearray data types becomes crucial. These data types are especially relevant when dealing with binary data, like reading or writing binary files, working with network protocols, or interfacing with hardware.
Understanding Byte Data and Memory Management
In Python, a byte is essentially a sequence of integers, where each integer represents a byte (8 bits) of data. Bytes are immutable, meaning once they are created, they cannot be changed. This immutability makes them similar to strings, but while strings are sequences of characters, bytes are sequences of raw data.
Here’s how you can create a byte object:
my_bytes = b'hello'
print(my_bytes) # Output: b'hello'
In the example above, b'hello'
is a byte literal. The b
prefix indicates that the following string is to be treated as a sequence of bytes.
The bytearray type is similar to bytes, but with one key difference: it is mutable. This means that you can modify its contents after creation. This mutability makes bytearrays more flexible when you need to work with data that may change over time, but it also requires more careful management of the data.
Here’s how to create a bytearray:
my_bytearray = bytearray(b'hello')
print(my_bytearray) # Output: bytearray(b'hello')
In this case, my_bytearray
allows modifications to its content, unlike the immutable my_bytes
.
Working with Bytes and Bytearrays
Bytes and bytearrays are particularly useful in situations where you need to handle raw binary data. For example, when you’re working with file I/O operations, network data, or protocols that require specific binary formats, these types offer direct control over the data.
Let’s look at some common operations you can perform with bytes and bytearrays.
Accessing and Modifying Data
You can access individual elements in a byte or bytearray just like you would in a list or string. However, you can modify elements only in a bytearray.
# Accessing data in a byte object
byte_obj = b'hello'
print(byte_obj[0]) # Output: 104 (ASCII code for 'h')
# Modifying data in a bytearray
byte_arr = bytearray(b'hello')
byte_arr[0] = 100 # Changing 'h' (104) to 'd' (100)
print(byte_arr) # Output: bytearray(b'dello')
2. Slicing
You can slice bytes and bytearrays in a way similar to lists and strings:
sliced_bytes = byte_obj[1:3]
print(sliced_bytes) # Output: b'el'
sliced_bytearray = byte_arr[:3]
print(sliced_bytearray) # Output: bytearray(b'del')
3. Concatenation
Both bytes and bytearrays can be concatenated using the +
operator:
byte_concat = b'hi' + b' there'
print(byte_concat) # Output: b'hi there'
bytearray_concat = byte_arr + bytearray(b' there')
print(bytearray_concat) # Output: bytearray(b'dello there')
4. Conversion between Types
You can convert between bytes and bytearrays, which is useful when you need to modify a byte object temporarily:
byte_obj = b'hello'
byte_arr = bytearray(byte_obj) # Convert to bytearray
byte_arr[0] = 100
byte_obj = bytes(byte_arr) # Convert back to bytes
print(byte_obj) # Output: b'dello'
5. Working with Binary Data
These types are perfect for working with binary data, such as when you’re reading or writing binary files. For example, you might read an image or an executable file, manipulate its data, and write it back to disk:
# Reading binary data
with open('example.bin', 'rb') as file:
data = file.read()
byte_data = bytes(data)
print(byte_data)
# Writing binary data
with open('output.bin', 'wb') as file:
file.write(byte_data)
Example and Output for Byte and Bytearray Operations
Let’s go through a practical example of how you can manipulate binary data using bytearrays.
Suppose you have a bytearray representing a sequence of colors in RGB format, and you want to change all red components to zero:
# A bytearray representing RGB colors: red, green, and blue
colors = bytearray([255, 0, 0, 0, 255, 0, 0, 0, 255]) # Red, Green, Blue
# Setting all red components to 0
for i in range(0, len(colors), 3):
colors[i] = 0
print(colors) # Output: bytearray(b'\x00\x00\x00\x00\xff\x00\x00\x00\xff')
In this example, the original bytearray contains three colors: red, green, and blue. After the loop runs, all the red components are set to zero, effectively removing the red color from the sequence.
Understanding the byte and bytearray data types in Python opens up powerful possibilities for working with binary data and managing memory more efficiently. Bytes are perfect for handling immutable binary sequences, while bytearrays offer the flexibility to modify data in place. These types are essential when dealing with low-level data processing tasks such as file I/O operations, network protocols, or even working with image and sound files.
Python NoneType Data Type
When you’re learning Python, you’ll quickly encounter the special value None
. Understanding what NoneType
is and how to use None
in Python is essential for writing clear and effective code.
What is NoneType in Python?
In Python, NoneType
is the type of the special value None
. None
is often used to signify “nothing” or “no value here.” It is Python’s way of representing the absence of a value or a null value. Unlike other data types like strings, lists, or integers, None
is unique—there’s only one None
object in any Python program.
Here’s a quick example:
my_variable = None
print(type(my_variable)) # Output: <class 'NoneType'>
In this example, my_variable
is assigned the value None
, and its type is NoneType
.
Common Use Cases of None in Python Programming
Understanding how and when to use None
is crucial in various programming scenarios. Here are some common use cases where None
comes into play:
1. Default Parameter Values in Functions:
When defining functions, you can use None
as a default value for a parameter. This is useful when you want to allow the caller to optionally omit certain arguments:
def greet(name=None):
if name is None:
print("Hello, Guest!")
else:
print(f"Hello, {name}!")
greet() # Output: Hello, Guest!
greet("Emmimal") # Output: Hello, Emmimal!
In this example, the function greet
checks if name
is None
and then decides how to greet the user.
2. Returning Nothing from a Function:
Sometimes, a function doesn’t need to return a value, in which case Python implicitly returns None
:
def add(a, b):
result = a + b
output = add(3, 4)
print(output) # Output: None
The add
function performs a calculation but doesn’t explicitly return a value, so None
is returned by default.
4. Indicating Missing Data:
In many applications, especially in data processing or web development, None
can be used to represent missing or unavailable data:
user_info = {"name": "John", "age": None}
if user_info["age"] is None:
print("Age is not provided.")
Here, None
indicates that the age
is not available for the user.
4. Chaining Comparisons:
Python allows chaining of comparison operators, and None
is often used to initialize variables that will later hold a value:
x = None
if x is None:
x = 10
print(x) # Output: 10
The if
statement checks whether x
is None
and assigns a value if it is.
5. Singleton Behavior:
Since None
is a singleton, meaning there’s only one instance of None
in a Python program, it’s safe to compare variables directly to None
using the is
keyword:
y = None
print(y is None) # Output: True
This ensures that you’re checking for the absence of a value in a very precise way.
Example and Output for NoneType Usage
Let’s look at a more comprehensive example to see NoneType
in action:
Suppose you’re building a function that looks up a user by their username in a database. If the user doesn’t exist, the function should return None
:
def find_user(username):
users = {"alice": 25, "bob": 30, "carol": 27}
return users.get(username)
result = find_user("dave")
if result is None:
print("User not found.")
else:
print(f"User found: Age is {result}")
Output:
User not found.
In this example, find_user("dave")
returns None
because “dave” isn’t in the users
dictionary. The if result is None
condition then triggers the appropriate message.
Understanding the NoneType data type in Python and the use of None
is fundamental for effective programming. Whether you’re setting default values in functions, representing missing data, or managing return values, None
is a tool that provides flexibility and clarity in your code. By using None
thoughtfully, you can write Python programs that are not only functional but also easy to understand and maintain.
User-Defined Data Types in Python
When working with Python, you’ll often rely on built-in data types like lists, dictionaries, or strings. But as your projects grow more complex, you might find yourself needing something more specialized. This is where user-defined data types come into play, allowing you to create custom classes tailored to your specific needs.
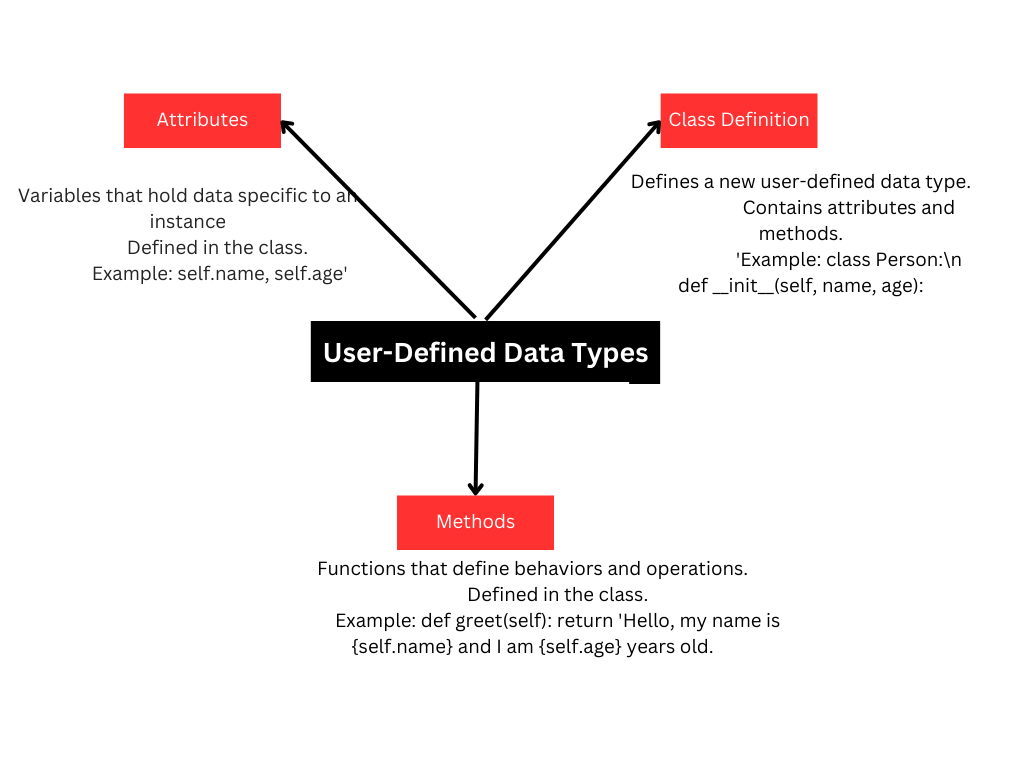
Creating Custom Classes in Python
In Python, creating a custom data type involves defining a class. A class is like a blueprint for objects—each object created from the class is an instance of that class, with its own unique data and behavior. Here’s a step-by-step breakdown of how you can create a custom class.
- Defining a Class:You start by using the
class
keyword, followed by the class name. The class name should be descriptive and follow the CamelCase naming convention.
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def start_engine(self):
return f"{self.make} {self.model}'s engine started!"
In this example, we’ve created a Car
class with three attributes—make
, model
, and year
. The __init__
method is a special method called a constructor, which initializes the attributes when a new instance of the class is created.
Creating an Instance:
Once the class is defined, you can create an instance (an object) of that class:
my_car = Car("Toyota", "Corolla", 2022)
Here, my_car
is an instance of the Car
class with specific values for make
, model
, and year
.
3. Calling Methods:
You can interact with your custom data type by calling its methods. These methods define the behavior of the class:
print(my_car.start_engine()) # Output: Toyota Corolla's engine started!
The start_engine
method is called on the my_car
object, triggering a specific action.
Using Custom Data Types in Complex Applications
User-defined data types are particularly useful in more complex applications where you need to manage multiple related pieces of data. For example, if you’re developing a vehicle management system, you could create classes like Car
, Truck
, and Motorcycle
, each with specific attributes and methods relevant to that type of vehicle.
Let’s extend the previous example to see how you might use custom data types in a more complex application:
class Car:
def __init__(self, make, model, year, fuel_level=100):
self.make = make
self.model = model
self.year = year
self.fuel_level = fuel_level
def start_engine(self):
if self.fuel_level > 0:
return f"{self.make} {self.model} is ready to go!"
else:
return f"{self.make} {self.model} cannot start due to low fuel."
def drive(self, distance):
fuel_consumed = distance * 0.2
self.fuel_level -= fuel_consumed
return f"After driving {distance} miles, fuel level is {self.fuel_level}%."
Now, when you create an instance of Car
, you can perform more complex operations:
my_car = Car("Honda", "Civic", 2023)
print(my_car.start_engine()) # Output: Honda Civic is ready to go!
print(my_car.drive(50)) # Output: After driving 50 miles, fuel level is 90.0%.
This Car
class is more sophisticated, with the ability to manage fuel levels and simulate driving.
Example and Output for User-Defined Data Types in Python
Let’s look at another example that demonstrates how user-defined data types can be used in practice. Suppose you’re working on a library management system. You might create a Book
class:
class Book:
def __init__(self, title, author, isbn):
self.title = title
self.author = author
self.isbn = isbn
self.checked_out = False
def check_out(self):
if not self.checked_out:
self.checked_out = True
return f"{self.title} has been checked out."
else:
return f"{self.title} is already checked out."
def return_book(self):
if self.checked_out:
self.checked_out = False
return f"{self.title} has been returned."
else:
return f"{self.title} was not checked out."
Now, when a book is checked out or returned, you can manage that state:
book1 = Book("1984", "George Orwell", "1234567890")
print(book1.check_out()) # Output: 1984 has been checked out.
print(book1.return_book()) # Output: 1984 has been returned.
Output:
1984 has been checked out.
1984 has been returned.
Process finished with exit code 0
User-defined data types in Python give you the flexibility to create classes that reflect real-world entities, making your code more organized and easier to maintain. By defining custom classes, you can encapsulate data and behavior in a way that aligns with the specific needs of your application. Whether you’re building a simple program or a complex system, understanding how to create and use custom data types is a fundamental skill that will enhance your ability to write clear and efficient Python code.
Practical Applications of Data Types in Python
Data Types in Web Development
Python is a favorite among web developers, and its diverse data types play a crucial role in how applications are built, managed, and interacted with. If you’re using popular web frameworks like Django or Flask, understanding how Python data types are utilized can make your development process smoother and more efficient.
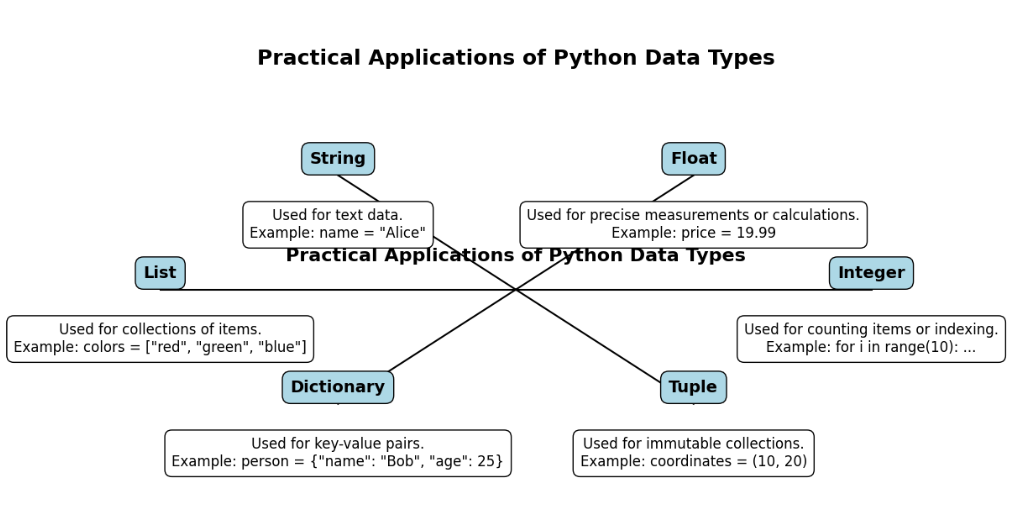
Data Types in Web Development
In web development, data types are the building blocks of your application. They help store, manage, and manipulate the data that your app works with, whether it’s user input, database records, or API responses.
For instance, strings handle text, dictionaries are perfect for key-value pairs like JSON objects, and lists or tuples are often used to manage collections of data. Let’s break down how these data types are applied in real-world web projects.
How Python Data Types Are Used in Web Frameworks
1. Strings in Web Forms and URLs:
In a web application, strings are everywhere. They manage user inputs, URLs, and even HTML templates. For example, when a user submits a form on a website, their input is captured as a string.
# Flask example for handling a form input
from flask import Flask, request
app = Flask(__name__)
@app.route('/submit', methods=['POST'])
def submit():
user_input = request.form['input_field'] # 'input_field' is the name of the input in the HTML form
return f"You entered: {user_input}"
Here, user_input
is a string that you can then process, validate, or store in a database.
2. Dictionaries for Handling JSON Data:
Web apps frequently communicate with external services using JSON. Python dictionaries map perfectly to JSON objects, making them ideal for parsing, modifying, and returning JSON data.
# Django example for handling JSON response
from django.http import JsonResponse
def get_data(request):
data = {
'name': 'John Doe',
'email': 'john@example.com'
}
return JsonResponse(data)
In this example, data
is a dictionary containing user information. The JsonResponse
function converts it to a JSON object that can be sent to the client.
3. Lists and Tuples for Managing Collections:
Whether you’re handling a list of users, products, or any other collection, Python’s lists and tuples are essential. Lists are mutable and great for scenarios where you need to add or remove items, while tuples are immutable and often used for fixed sets of data.
# Flask example for rendering a list of users
from flask import render_template
@app.route('/users')
def users():
user_list = ['Alice', 'Bob', 'Charlie']
return render_template('users.html', users=user_list)
In this example, user_list
is a Python list passed to an HTML template, where it can be iterated over and displayed.
4. Using Sets for Unique Collections:
Sometimes, you need to ensure that a collection of data contains no duplicates. Python sets are perfect for this, as they automatically enforce uniqueness.
# Django example for filtering unique values
def unique_items(request):
items = ['apple', 'banana', 'apple', 'orange']
unique_items_set = set(items)
return JsonResponse({'unique_items': list(unique_items_set)})
Here, the unique_items_set
will contain only unique items, removing any duplicates from the original list.
5. Handling NoneType in Web Development:
None
is often used to represent the absence of a value, which can be useful in various scenarios like form validation or database queries.
# Flask example for handling optional form fields
@app.route('/submit_optional', methods=['POST'])
def submit_optional():
optional_input = request.form.get('optional_field') # Returns None if 'optional_field' is not in the form
if optional_input is None:
return "No optional input provided"
else:
return f"Optional input: {optional_input}"
- In this case,
None
is used to check if a form field was filled out by the user or left empty.
Examples from Django/Flask Projects
Let’s explore a more concrete example combining these data types in a Django project.
Imagine you’re building a simple blog where users can post comments. You might use the following approach:
- Modeling Data with Classes and Lists:You could define a
Comment
class to represent each comment:
class Comment:
def __init__(self, author, content):
self.author = author
self.content = content
Then, you could store these comments in a list:
comments = [
Comment(author="Alice", content="Great post!"),
Comment(author="Bob", content="Thanks for the info."),
]
2. Using Dictionaries to Structure Data:
When rendering comments on a web page, you might structure them in a dictionary to easily pass the data to your template:
from django.shortcuts import render
def blog_post(request):
comments_data = {
'comments': comments
}
return render(request, 'blog_post.html', comments_data)
3. Leveraging Sets for Unique Tags:
Suppose your blog supports tags. You can use a set to ensure each tag is unique:
tags = set(['python', 'web', 'django', 'python']) # 'python' will only appear once in the set
By using a set, you avoid duplicate tags, ensuring that each tag appears only once.
In web development with Python, data types like strings, dictionaries, lists, tuples, sets, and NoneType
play vital roles in how you manage data within frameworks like Django and Flask. From handling user input and managing collections to structuring JSON responses and ensuring data uniqueness, these data types are the foundation of your application’s functionality. Understanding how to use them effectively can greatly enhance your ability to build strong, efficient, and maintainable web applications.
Data Types in Data Science and Machine Learning
Data types are at the core of any data science or machine learning project. Whether you’re working with Pandas, NumPy, or building machine learning algorithms, understanding how to handle data types can significantly impact the efficiency and accuracy of your work.
Handling Data Types in Libraries like Pandas and NumPy
When working with large datasets in Python, libraries like Pandas and NumPy are your go-to tools. These libraries allow you to manage and manipulate data efficiently, but it’s essential to be mindful of the data types you’re using.
- Pandas: In Pandas, data is often stored in DataFrames, which are essentially tables where each column can have a different data type. For example, you might have a column of integers, another of floats, and another of strings. Being aware of these data types is crucial because it affects how you can manipulate and analyze your data.
import pandas as pd
# Creating a DataFrame with different data types
data = {
'Age': [25, 30, 35],
'Salary': [50000.0, 60000.0, 70000.0],
'Name': ['Alice', 'Bob', 'Charlie']
}
df = pd.DataFrame(data)
Here, the ‘Age’ column is of integer type, ‘Salary’ is a float, and ‘Name’ is a string. Understanding this allows you to perform type-specific operations, like aggregations on numeric columns or string manipulations.
NumPy: In NumPy, everything is an array, and all elements within an array must be of the same data type. This is incredibly efficient for numerical operations, which is why NumPy is heavily used in scientific computing and machine learning.
import numpy as np
# Creating a NumPy array with a specific data type
ages = np.array([25, 30, 35], dtype=np.int32)
- By specifying
dtype=np.int32
, you ensure that the array uses 32-bit integers, which is optimal for large numerical computations.
Importance of Data Types in Machine Learning Algorithms
Machine learning models rely on data, and the type of data you feed into a model can significantly impact its performance. For example:
- Numerical Data: Most machine learning algorithms expect numerical input, so understanding how to convert categorical data (like names or categories) into numerical form is critical. Techniques like one-hot encoding or label encoding are often used to achieve this.
from sklearn.preprocessing import LabelEncoder
# Example of label encoding categorical data
labels = ['cat', 'dog', 'mouse']
encoder = LabelEncoder()
encoded_labels = encoder.fit_transform(labels)
Here, each label is converted into a numerical value, making it ready for training a model.
- Floating-Point Precision: In some cases, the precision of floating-point numbers can affect the performance of a model. For example, very large or very small values might need to be normalized or scaled to avoid issues during training.
from sklearn.preprocessing import StandardScaler
# Example of normalizing numerical data
salaries = np.array([50000.0, 60000.0, 70000.0]).reshape(-1, 1)
scaler = StandardScaler()
scaled_salaries = scaler.fit_transform(salaries)
- Scaling helps in ensuring that the model trains more efficiently by bringing all features to a similar range.
Data Types in Database Management
When you’re working with databases, especially SQL databases, understanding how Python data types map to SQL data types is essential for seamless integration.
Mapping Data Types in Python to SQL Data Types
When you interact with a SQL database using Python, your Python data types are converted to corresponding SQL data types. For example:
- Integer (Python) → INT (SQL)
- Float (Python) → FLOAT (SQL)
- String (Python) → VARCHAR or TEXT (SQL)
- Boolean (Python) → BOOLEAN (SQL)
This mapping is crucial when you’re inserting data into a database or querying it because mismatches can lead to errors.
Example of Database Operations Using Python
Let’s say you’re working with a SQLite database in Python:
import sqlite3
# Connecting to a SQLite database
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
# Creating a table
cursor.execute('''
CREATE TABLE employees (
id INTEGER PRIMARY KEY,
name TEXT,
age INTEGER,
salary REAL
)
''')
# Inserting data into the table
cursor.execute('''
INSERT INTO employees (name, age, salary)
VALUES ('Alice', 25, 50000.0)
''')
# Committing the transaction and closing the connection
conn.commit()
conn.close()
In this example, you’re inserting an integer (age
), a string (name
), and a float (salary
) into a SQL table. Understanding the relationship between Python and SQL data types ensures your data is stored and retrieved correctly.
Data Types in Python for Automation and Scripting
Python is often used in automation and scripting to perform repetitive tasks more efficiently. Understanding data types is key to writing scripts that are not only functional but also maintainable and scalable.
How to Effectively Use Data Types in Automation Scripts
In automation scripts, you’ll often work with data types to handle various inputs, process data, and produce outputs. For example:
- String Manipulation: Automating tasks like renaming files or processing text data requires you to work efficiently with strings.
import os
# Renaming files in a directory
for filename in os.listdir('.'):
if filename.endswith('.txt'):
new_name = filename.replace(' ', '_')
os.rename(filename, new_name)
Here, you’re manipulating string data to replace spaces with underscores in filenames.
- Lists and Dictionaries for Data Management: Automation scripts often involve collecting data in lists or dictionaries, processing it, and then taking action based on the results.
import csv
# Reading data from a CSV file into a list of dictionaries
data = []
with open('data.csv', 'r') as file:
reader = csv.DictReader(file)
for row in reader:
data.append(row)
- Using dictionaries allows you to easily access and manipulate data based on column names.
Examples of Automating Tasks with Data Types in Python
Let’s say you want to automate the process of generating reports from a dataset:
import pandas as pd
# Loading data into a Pandas DataFrame
df = pd.read_csv('sales_data.csv')
# Grouping and summarizing the data
summary = df.groupby('Product')['Sales'].sum()
# Saving the summary to a new CSV file
summary.to_csv('sales_summary.csv')
Here, you’re using a Pandas DataFrame to load and process data, then saving the result to a new file. The use of the right data types ensures that the operations are performed efficiently and the output is accurate.
Understanding and effectively using data types in Python is crucial in various fields like data science, machine learning, database management, and automation. Whether you’re processing large datasets, developing machine learning models, managing databases, or automating repetitive tasks, the right knowledge of data types can greatly enhance your work’s efficiency and accuracy. By paying attention to how these data types are applied across different scenarios, you can ensure that your Python applications are both powerful and reliable.
Best Practices for Working with Data Types in Python
When working with Data Types in Python, selecting the right data type for your tasks is crucial to writing clean, efficient, and bug-free code. Let’s dive into some best practices that can help you make informed decisions when choosing data types, avoid common pitfalls, and optimize your code.
Choosing the Right Data Type for Your Needs
Choosing the correct data type can make a significant difference in how your program runs and how easy it is to maintain. Here are some considerations to help you choose wisely:
Understand the Nature of Your Data:
The first step in choosing the right data type is to understand what kind of data you’re dealing with. For example, if you’re storing a list of items, a list is the most natural choice. But if you need to ensure the uniqueness of those items, a set might be more appropriate.
# Example of using a list and a set
fruits = ["apple", "banana", "apple"] # List allows duplicates
unique_fruits = set(fruits) # Set automatically removes duplicates
Performance Considerations:
Performance is often a critical factor when selecting data types. For instance, if you frequently need to access elements by index, a list might be the better choice because it provides O(1) access time. On the other hand, if you need to perform a lot of insertions and deletions, a deque (from the collections
module) could be more efficient than a list.
from collections import deque
# Example of using a deque for efficient insertions and deletions
d = deque([1, 2, 3])
d.appendleft(0) # Efficient insertion at the beginning
d.pop() # Efficient deletion from the end
Memory Usage:
Python offers data types like bytes
and bytearray
that are more memory-efficient than lists of integers when dealing with binary data. If your application is memory-sensitive, it’s important to choose data types that minimize memory consumption.
# Example of using bytes for memory-efficient binary data storage
data = bytes([0, 1, 2, 3])
Best Practices for Efficient Data Management
Efficient data management is about using the right data structures and keeping your code maintainable. Here are some tips:
- Use Dictionaries for Fast Lookups: Dictionaries in Python offer O(1) average time complexity for lookups, making them ideal for situations where you need to quickly retrieve values based on keys.
# Example of using a dictionary for fast lookups
employee_salaries = {"Alice": 70000, "Bob": 60000}
print(employee_salaries["Alice"]) # Fast retrieval of Alice's salary
- Leverage List Comprehensions: List comprehensions are not only more Pythonic but also often faster than traditional loops. They should be your go-to tool for creating new lists based on existing ones.
# Example of using a list comprehension for efficient data manipulation
squares = [x**2 for x in range(10)]
- Be Mindful of Mutability: Choosing between mutable and immutable types can have a big impact. For instance, tuples (immutable) can be used as keys in dictionaries, whereas lists (mutable) cannot. Using immutable types when appropriate can help avoid bugs related to unexpected changes in data.
# Example of using a tuple as a dictionary key
point = (1, 2)
distances = {point: 10.5}
Common Pitfalls to Avoid
Working with data types in Python can sometimes lead to subtle errors. Here are some common pitfalls and how to avoid them:
- Avoiding Type Errors: One of the most common errors in Python is a type error, where an operation is applied to an inappropriate type. For example, trying to add an integer and a string will result in a type error.
# Example of a type error
age = 30
name = "Alice"
# print(age + name) # TypeError: unsupported operand type(s) for +: 'int' and 'str'
To avoid such issues, ensure that your variables are of the correct type before performing operations. You can use isinstance()
to check types when necessary.
- Handling Type Conversions Carefully: Converting between types can lead to unexpected results if not done carefully. For example, converting a float to an integer will truncate the decimal part.
# Example of type conversion
price = 19.99
whole_price = int(price) # Truncates to 19
- Debugging Tips for Data Type-Related Issues: When you encounter bugs related to data types,
print()
statements and Python’stype()
function are your best friends. They allow you to check the type of a variable at any point in your code, helping you to identify where things might be going wrong.
# Example of using type() for debugging
value = "100"
print(type(value)) # <class 'str'>
Optimizing Data Type Usage in Python
Optimizing your use of data types can lead to significant performance improvements, especially in large-scale applications.
- Techniques for Improving Performance: One technique for optimization is to use
NumPy
arrays instead of Python lists when dealing with large numerical datasets.NumPy
arrays are more memory-efficient and allow for faster computations.
import numpy as np
# Example of using NumPy for efficient numerical operations
large_array = np.array([1, 2, 3, 4, 5])
Another technique is to use generators instead of lists when you only need to iterate over data once. Generators do not store all the elements in memory at once, which can save a lot of memory.
# Example of using a generator for memory efficiency
squares = (x**2 for x in range(10))
- Examples of Optimization in Real-World Scenarios: In a real-world scenario, let’s say you are processing a large log file. Instead of loading the entire file into memory, you can read and process it line by line using a generator.
# Example of reading a large file efficiently
def process_log(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
for log_entry in process_log('large_log.txt'):
# Process each log entry
pass
- This approach minimizes memory usage, making your script more efficient.
Choosing the right data types in Python is not just a matter of syntax—it’s a key part of writing efficient, maintainable code. By understanding the nature of your data, considering performance and memory usage, and avoiding common pitfalls, you can write Python code that is both powerful and reliable.
Conclusion
Conclusion: Mapping and Set Data Types in Python
In this part of “The Ultimate Guide to Python Data Types,” we’ve explored the key concepts behind Python’s mapping and set Data Types in Python. Understanding these data types is crucial for managing and manipulating collections of data effectively.
Mapping Data Types: We started with dictionaries, which use key-value pairs to store and retrieve data efficiently. Dictionaries are versatile and widely used for handling structured data. We also discussed sets, which are ideal for operations that require uniqueness, such as removing duplicates or performing mathematical set operations. Frozensets, with their immutable nature, were introduced as a useful alternative for fixed collections where data integrity is important.
Set Data Types: We examined how to use these types in various scenarios, from ensuring that data remains unique to performing set operations like unions and intersections. We also highlighted how frozensets can be employed in situations where immutability is required for reliable and consistent data handling.
Conclusion: Advanced Data Types in Python and Practical Applications
In the second part, we delved into advanced Data Types in Python and their practical applications across different fields.
Advanced Data Types: We covered byte and bytearray data types, essential for managing binary data and memory efficiently. We also explored NoneType, used to signify the absence of a value, and user-defined data types, which allow you to create custom classes customized to your specific needs. These advanced data types enhance your ability to handle complex data structures and manage various programming tasks effectively.
Practical Applications: We applied these concepts to real-world scenarios, demonstrating how Python data types are used in web development, data science, and database management. Whether you’re using Django or Flask for web development, managing data with Pandas and NumPy in data science, or mapping Python data types to SQL in database management, understanding these data types is crucial. We also discussed how these concepts are relevant in automation and scripting, providing practical examples of how to automate tasks efficiently with Python.
By mastering these advanced and practical aspects of Python data types, you’re well-prepared to write effective and efficient code, tackle complex challenges, and apply your knowledge to a range of real-world scenarios. Stay tuned for the next part of our guide, where we’ll continue to build on these foundational concepts and explore further advanced topics in Python programming.
External Resources
Python Byte and Bytearray
- Official Python Documentation: Bytes and Bytearray – Detailed information on byte and bytearray types, including methods and usage.
Python NoneType
- Official Python Documentation: None – Explanation of the
None
type and its uses
User-Defined Data Types
- Official Python Documentation: Classes – Introduction to creating and using classes in Python.
Data Types in Web Development
- Django Documentation: Django Models – How Django uses data types in its models.
FAQs
Answer: A Python dictionary is a collection of key-value pairs where each key is unique. Dictionaries are created using curly braces {}
, with each key-value pair separated by a colon. For example, my_dict = {'name': 'Alice', 'age': 30}
. You use dictionaries to store and retrieve data efficiently based on keys. Common operations include adding items, updating values, and removing items using methods like .get()
, .update()
, and .pop()
.
Answer: Python sets are unordered collections of unique elements. They are defined with curly braces {}
or the set()
function. For example, my_set = {1, 2, 3}
. Sets are useful for removing duplicates and performing operations such as union, intersection, and difference. Common methods include .add()
, .remove()
, and .discard()
.
bytes
and bytearray
in Python? Answer: bytes
represents immutable sequences of bytes, while bytearray
represents mutable sequences. Use bytes
when you need an unchangeable sequence of bytes, such as when working with binary data. Use bytearray
when you need to modify the byte data. For example, b'hello'
creates a bytes
object, while bytearray(b'hello')
creates a bytearray
object.
Answer: Choosing the right data type depends on the requirements of your application. Consider factors such as the type of data you’ll be working with, the operations you need to perform, and performance considerations. For example, use lists for ordered collections, sets for unique elements, and dictionaries for key-value mappings.