Introduction
Are you ready to explore web development? Building your first web application can feel overwhelming, but it doesn’t have to be! In this guide, we’ll show you how to create a Web Application with Flask. This popular framework for Python is both easy to use and powerful.
Flask is great for beginners. It’s lightweight and flexible. You can learn quickly while still having the tools for real projects. Whether you want to create a personal blog or a portfolio site, Flask can help you bring your ideas to life.
In this step-by-step guide, we’ll cover everything. We’ll start with setting up your environment and finish with deploying your app online. You’ll gain hands-on experience and build confidence as you create something unique. So let’s begin!
What is Flask and Why Should You Use It for Web Development?

Flask is a Python-based micro-framework. It’s great for building simple web applications quickly. It’s called a “micro” framework because it doesn’t come with the heavy features that larger frameworks like Django do. Flask is like a blank canvas. You can build your web applications exactly as you want, without enforcing a specific structure.
If you’re just starting with Python, Flask is a fantastic option for beginners. Its simplicity allows you to grasp core concepts of web development without being overwhelmed. Here are a few reasons why Flask is popular:
- It is lightweight and doesn’t come with unnecessary overhead.
- It’s flexible, so you can integrate only the tools you need.
- It encourages a hands-on approach to learning.
Flask vs. Django: Which One Should You Choose?
Many people wonder about Flask vs. Django. Both are excellent web frameworks but serve different needs.
Here’s a quick comparison:
Feature | Flask | Django |
---|---|---|
Type of Framework | Micro-framework | Full-stack framework |
Learning Curve | Easier for beginners | Steeper due to more built-in features |
Flexibility | Highly flexible | More opinionated |
Ideal for | Small to medium projects | Larger, more complex projects |
Built-in Tools | Minimal, requires third-party integrations | Comes with built-in admin panel, ORM, and more |
Speed of Development | Faster for smaller apps | Better for large apps needing many features |
If you want to build a quick prototype or an API, Flask is great for small projects. But if you need an admin panel or a more structured approach, Django may be more suitable.
When I started developing web apps, I chose Flask. It allowed me to experiment without rigid structures. Later, I transitioned to Django for larger projects where built-in tools helped simplify development.built-in features.
Key Features of Flask
Flask’s core features make it easy to use. Here are some key features of Flask:
- Lightweight and Minimal: Flask delivers only the core components to get your app started, without including any unnecessary tools.
- Routing: Flask allows you to easily define routes. Here’s a simple code snippet:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Welcome to Flask!"
if __name__ == "__main__":
app.run(debug=True)
- Jinja2 Templating: Flask uses Jinja2 templates to generate HTML. You can pass variables from your Flask app to the templates, allowing dynamic content.
- Extension Support: Flask doesn’t force you to use any particular database or form handler. You can extend it by adding only what you need.
- Blueprints: Flask supports modular structures through Blueprints. This is useful as your application grows.
Use Cases for Flask
Flask can be used in many scenarios. Here are some use cases for Flask:
- Flask for Small Projects: It shines when you need a simple web application with Flask. Whether it’s a personal blog or a portfolio website, Flask lets you quickly set things up.
- Flask for APIs: It is excellent for creating APIs. If you’re building a RESTful API, Flask is easy to work with. Here’s a quick example:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/')
def home():
return "Welcome to the Flask app!"
@app.route('/api/data')
def get_data():
return jsonify({"message": "Hello, API!"})
if __name__ == '__main__':
app.run(debug=True)
- Flask for Prototyping: If you need a quick prototype, Flask web applications can be built fast. It allows you to develop and iterate without too much setup.
- Flask for Education: Flask is used in education because of its simple syntax. It’s perfect for teaching web development to beginners.
Setting Up Your Development Environment for Flask
Setting up your Flask development environment is one of the first steps when building a web application with Flask. Whether you are working on Windows, macOS, or Linux, the process is simple. In this guide, you’ll get detailed steps to get everything ready to start building your web app. We’ll cover installing Python, setting up a virtual environment, and installing Flask with pip
.
Installing Python and Flask on Windows, macOS, and Linux
Before installing Flask, you need Python installed. Flask works on Python 3.6 and later. Here’s how you can install Python and Flask on different platforms:
Windows
- Go to the official Python website and download the installer for Windows.
- During installation, ensure that you check the box that says “Add Python to PATH”.
- Once Python is installed, open Command Prompt and verify it using:
python --version
4. To install Flask, you’ll use pip
(Python’s package installer). After Python is installed, pip
comes bundled with it:
pip install Flask
macOS
- For macOS, you can install Python using Homebrew. First, install Homebrew if you don’t have it:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
2. Then, install Python:
brew install python
3. Verify Python installation:
python3 --version
4. Now install Flask using pip
:
pip3 install Flask
Linux
On Linux, Python is usually pre-installed. To ensure you have the correct version, check:
python3 --version
If it’s not installed, use your package manager (such as apt for Ubuntu):
sudo apt update
sudo apt install python3 python3-pip
Once Python is set, install Flask:
pip3 install Flask
In my experience, setting up Flask on different operating systems doesn’t vary much. However, using the right version of Python and installing the necessary tools can save you time and prevent errors later on.
Creating a Virtual Environment for Flask
One of the best practices when working on any web application with Flask is to create a Python virtual environment. A virtual environment isolates your project’s dependencies from other Python projects, ensuring that different versions of Flask or other packages don’t interfere with each other.
Here’s how to set up a virtual environment for Flask on any platform:
Steps:
- Open your terminal or Command Prompt.
- Navigate to your project directory where you want to set up your Flask app.
- Run the following command to create a virtual environment:
python3 -m venv venv
Here, venv
is the name of the virtual environment folder.
Now, activate the virtual environment:
- Windows
venv\Scripts\activate
- macOS/Linux
source venv/bin/activate
5. Once activated, your command prompt will show the virtual environment name, which means you are now inside the isolated environment.
Installing Flask with pip
With your virtual environment set up, installing Flask becomes very easy. You can install Flask using pip, the Python package installer. Here’s how you can install Flask with pip inside the virtual environment:
Steps:
- Ensure your virtual environment is activated. You should see
(venv)
in your terminal. - Now, install Flask using:
pip install Flask
3. After installing, verify the installation by running the following command:
python -m flask --version
Your environment is now ready! You can start developing your web application with Flask.
Must Read
- How to Return Multiple Values from a Function in Python
- Parameter Passing Techniques in Python: A Complete Guide
- A Complete Guide to Python Function Arguments
- How to Create and Use Functions in Python
- Find All Divisors of a Number in Python
Creating Your First Flask Application
When you’re ready to start building a web application with Flask, it’s exciting to dive into your first project. Flask makes it simple to get up and running with just a few lines of code. In this section, we’ll walk through how to write a “Hello, World!” Flask app, set up the development server, and understand the structure of a Flask project.
Writing the Basic “Hello, World!” Flask App
To create your first Flask web application, you’ll start with a simple script that returns “Hello, World!” when you visit a page in your browser.
- First, ensure you have Flask installed and are in a virtual environment.
- Create a new Python file called
app.py
in your project directory. - Inside
app.py
, write the following code:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run()
What’s happening here?
Flask(__name__)
: This initializes the Flask application.@app.route('/')
: This is a route decorator that maps the URL/
to thehello_world
function.app.run()
: This starts the Flask development server.
This small piece of code is your first Flask app example. When you run this file, Flask will serve a simple page displaying “Hello, World!” in your browser.
Running the Flask Development Server
Once you have the code ready, you need to run the Flask development server to see your application live. Here’s how you can do it:
- Open your terminal or Command Prompt.
- Navigate to the folder where
app.py
is located. - Make sure you are inside your virtual environment (you should see
(venv)
in your terminal). - Run the app using the following command:
python app.py
Flask will start the local server and you should see output like this:
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
5. Open your browser and go to http://127.0.0.1:5000/
. You should see “Hello, World!” displayed.
Flask’s built-in development server is incredibly helpful. It allows you to make changes to your app and see updates instantly. It automatically reloads the app when it detects file changes, saving you time during development.
Understanding the Flask Application Structure
As you begin building more complex applications, understanding the basic Flask app structure is essential. A small Flask project can be managed with just one file (like app.py
), but as the app grows, organizing your project becomes important.
Here’s a typical structure for a larger Flask project layout:
Key parts of the structure:
- /static: This folder holds static files like CSS, images, and JavaScript.
- /templates: HTML templates go here, making it easy to separate logic from presentation.
- app.py: This is your main file that contains routes, views, and Flask logic.
- config.py: Store app settings and configurations here.
- requirements.txt: Use this file to list all your project dependencies for easy setup.
By structuring your web application with Flask this way, you’ll make it easier to manage as it grows.
Adding Routes and Views in Flask
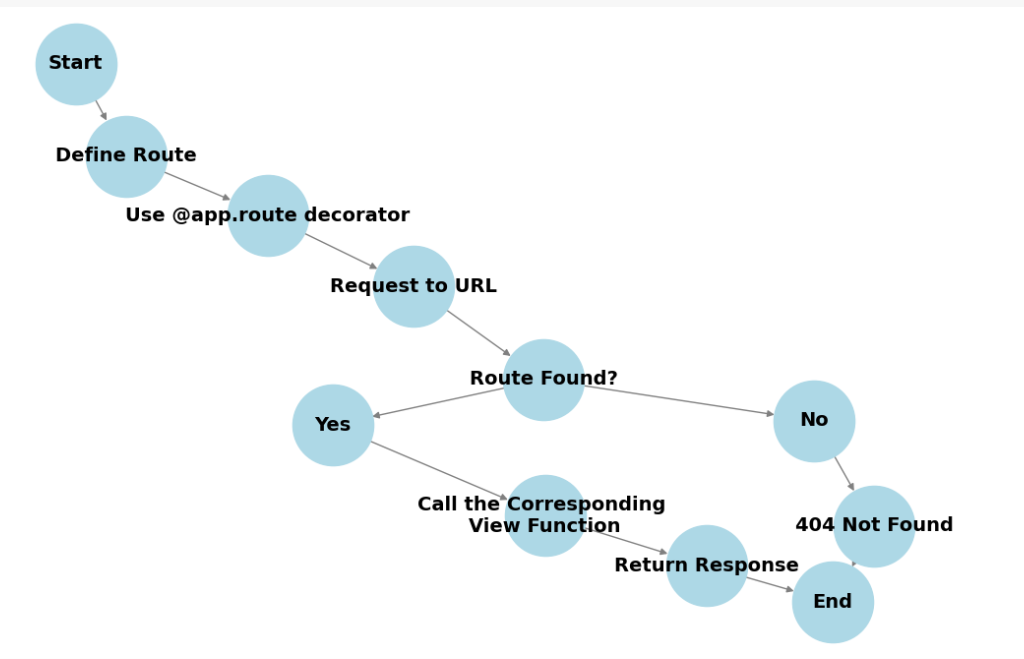
When building a web application with Flask, adding routes and views is a fundamental step. Routes are the URLs that users can visit, and views are the functions that return the content for those URLs. This section will explore how to define routes in Flask, create dynamic routes, and pass variables through them.
Defining Routes in Flask
In Flask, routes are defined using decorators. A decorator is a special type of function that modifies another function. To define a route, the @app.route
decorator is used. Here’s a simple example to illustrate this:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return 'Welcome to my Flask App!'
@app.route('/about')
def about():
return 'This is the About page.'
if __name__ == '__main__':
app.run()
Breaking It Down
@app.route('/')
: This defines the main page (home) of your application. When a user visits the root URL, thehome
function is called.@app.route('/about')
: This creates an additional route for the About page. Visiting/about
triggers theabout
function.
This basic Flask URL routing example shows how easy it is to add new pages to your application. Each route corresponds to a different function that returns a response, whether it be text, HTML, or even JSON.
Creating Dynamic Routes and Passing Variables
Dynamic routes allow you to create URLs that can accept variables. This is useful for creating pages that display content based on user input or other dynamic data.
For example, let’s create a route that greets a user by their name. Here’s how to set it up:
@app.route('/hello/<name>')
def greet(name):
return f'Hello, {name}!'
What’s Happening Here?
<name>
: This syntax in the route specifies a variable part of the URL. When a user visits a URL like/hello/Alice
, thegreet
function is called, andname
receives the value “Alice”.
This allows you to create more dynamic content. Here are some example URLs and their outputs:
- URL:
/hello/Alice
→ Output:Hello, Alice!
- URL:
/hello/Bob
→ Output:Hello, Bob!
Benefits of Dynamic Routing
- User Interaction: You can create personalized experiences for users.
- Cleaner URLs: Using variables in routes leads to more meaningful URLs.
- Scalability: Easier to manage and extend your application as it grows.
Example of Using Dynamic Routing
Here’s a more comprehensive example. Suppose you’re building a web application with Flask that showcases products. You might want to create a route to view a product by its ID:
@app.route('/product/<int:product_id>')
def show_product(product_id):
return f'Product ID: {product_id}'
Explanation
<int:product_id>
: This specifies that the variableproduct_id
must be an integer. Flask will automatically convert it before passing it to theshow_product
function.
Summary of Key Concepts
Here’s a quick recap of the key points covered:
- Defining Routes:
- Use
@app.route
to set up a route. - Each route is linked to a specific function that returns a response.
- Use
- Creating Dynamic Routes:
- Use angle brackets
< >
to define variables in your routes. - Flask allows for flexible URLs that enhance user experience.
- Use angle brackets
Rendering Templates in Flask: Using Jinja2

Rendering templates is a crucial aspect of developing a web application with Flask. Templates allow developers to separate HTML presentation from Python code, making applications easier to maintain and enhance. This section will guide you through using the Jinja2 template engine, which is integrated into Flask. We’ll cover the basics of Jinja2, how to render HTML templates, and how to pass dynamic data to your templates.
Introduction to Jinja2 Template Engine
Jinja2 is a powerful template engine for Python, and it plays a vital role in Flask applications. With Jinja2, you can create HTML files that include placeholders for dynamic content. This feature helps build more interactive web applications.
Key Features of Jinja2
- Template Inheritance: Allows creating a base template that other templates can extend. This reduces repetition in code.
- Control Structures: Supports loops and conditional statements, enabling dynamic content rendering.
- Filters: These are used to modify data before rendering, like formatting dates or transforming text to uppercase.
Example of Jinja2 Basics
Here’s a simple Jinja2 template example, saved as base.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>{% block title %}My Flask App{% endblock %}</title>
</head>
<body>
<header>
<h1>Welcome to My Flask App</h1>
</header>
<main>
{% block content %}{% endblock %}
</main>
<footer>
<p>© 2024 My Flask App</p>
</footer>
</body>
</html>
In this example, the block
tags are placeholders that other templates can fill in.
Rendering HTML Templates with Flask
To render HTML templates in Flask, the render_template
function is used. This function allows you to return a rendered template in response to a user request.
Basic Template Rendering Example
Here’s a basic example of rendering a template in Flask:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('base.html')
if __name__ == '__main__':
app.run()
In this code:
render_template('base.html')
: This line tells Flask to look for thebase.html
file in thetemplates
directory and render it.
HTML Rendering in Flask
Structure
The templates are typically stored in a folder named templates
. Flask automatically looks for templates in this directory, making it easy to organize your files.
Running the Application
To see your rendered template in action:
- Run the Flask application.
- Open your web browser and go to
http://localhost:5000/
.
Passing Data to Templates in Flask
One of the powerful features of Jinja2 is the ability to pass dynamic data to templates. This allows you to create personalized and interactive content based on user inputs or database queries.
Example of Passing Data
Here’s how to pass data to a template:
@app.route('/greet/<name>')
def greet(name):
return render_template('greet.html', user_name=name)
In this example:
- The function
greet
takes a parametername
from the URL. - The variable
user_name
is then passed to the templategreet.html
.
Creating the Template
Now, create a new template file named greet.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Greeting Page</title>
</head>
<body>
<h1>Hello, {{ user_name }}!</h1>
<p>Welcome to your personalized page.</p>
</body>
</html>
What’s Happening Here?
{{ user_name }}
: This is how you reference the variable passed from your Flask view. When the URL/greet/Alice
is accessed, it displays “Hello, Alice!” on the page.
Benefits of Passing Data
- Dynamic Content: Tailor content based on user inputs or other criteria.
- User Engagement: Personalization can lead to a better user experience, making visitors feel more connected to the application.
Summary of Key Concepts
Here’s a quick recap of the key points covered:
- Jinja2 Basics:
- A powerful template engine integrated with Flask.
- Supports features like template inheritance, control structures, and filters.
- Rendering HTML Templates:
- The
render_template
function is used to return rendered templates. - Templates are stored in the
templates
directory.
- The
- Passing Data to Templates:
- Dynamic content can be sent to templates using keyword arguments.
- Templates can reference these variables to display personalized content.
Handling Forms and User Input in Flask
Handling forms and user input is a fundamental part of building any web application with Flask. User interactions through forms enable your applications to collect data, whether it’s user registrations, feedback, or any other input. This section will guide you through using Flask-WTF for forms, validating form input, and handling form submissions effectively.
Using Flask-WTF for Forms
Flask-WTF is an extension of Flask that integrates WTForms, a flexible form handling library. This powerful tool simplifies form creation and validation, making it easier to manage user inputs.
Key Benefits of Using Flask-WTF:
- CSRF Protection: Automatically includes protection against Cross-Site Request Forgery.
- Input Validation: Provides an easy way to validate form fields.
- Customizable: Allows for the creation of complex forms with various field types.
Setting Up Flask-WTF
To get started, you need to install Flask-WTF:
pip install Flask-WTF
Then, import the necessary modules and create a form class. Here’s a simple example:
from flask import Flask
from flask_wtf import FlaskForm
from wtforms import StringField, SubmitField
from wtforms.validators import DataRequired
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your_secret_key'
class MyForm(FlaskForm):
name = StringField('Name', validators=[DataRequired()])
submit = SubmitField('Submit')
Explanation of the Code
FlaskForm
: Base class for creating forms.StringField
: Represents a text input field.DataRequired
: Validator that ensures the field is not empty.SubmitField
: Represents a submit button.
Rendering the Form in a Template
To display the form in a template, use the following code in form.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My Form</title>
</head>
<body>
<form method="POST" action="">
{{ form.hidden_tag() }}
<p>
{{ form.name.label }}<br>
{{ form.name(size=32) }}<br>
{% for error in form.name.errors %}
<span style="color: red;">[{{ error }}]</span>
{% endfor %}
</p>
<p>{{ form.submit() }}</p>
</form>
</body>
</html>
In this template:
{{ form.hidden_tag() }}
: Generates a CSRF token to protect the form.- The error messages will be displayed if the validation fails.
Validating Form Input with Flask-WTF
Validating user input is essential to ensure that the data collected is accurate and secure. Flask-WTF makes form validation straightforward with built-in validators.
Example of Validating Form Input
Building on the previous example, here’s how you can validate user input when the form is submitted:
from flask import render_template, redirect, url_for
@app.route('/form', methods=['GET', 'POST'])
def form():
form = MyForm()
if form.validate_on_submit(): # Validates the form on submission
name = form.name.data
# Process the data here (e.g., save to a database)
return redirect(url_for('success'))
return render_template('form.html', form=form)
@app.route('/success')
def success():
return "Form submitted successfully!"
Explanation of the Validation Process
validate_on_submit()
: This method checks if the form was submitted and if all validations passed.- If the form is valid, it processes the data (e.g., saving to a database) and redirects to a success page.
Handling Form Submission and Redirects
After form submission, handling the data properly is crucial for user experience. Typically, users should be redirected to a different page after a successful submission to prevent duplicate submissions.
Handling Form Submission
When a user submits a form, you can process the data and redirect them to a different route. This is important to avoid resubmission if the user refreshes the page.
Here’s how to implement this:
@app.route('/form', methods=['GET', 'POST'])
def form():
form = MyForm()
if form.validate_on_submit():
name = form.name.data
# Here, you would typically save the data to a database.
return redirect(url_for('success'))
return render_template('form.html', form=form)
Key Points in Handling Form Submission:
- POST Request: The form should be submitted using the POST method for security reasons.
- Redirect After Submission: Redirect to a success page to prevent form resubmission when the page is refreshed.
Summary of Key Concepts
Here’s a quick recap of what you’ve learned about handling forms in Flask:
Topic | Description |
---|---|
Using Flask-WTF for Forms | Integrates WTForms for easy form handling with CSRF protection. |
Validating Form Input | Built-in validators ensure user input is correct and secure. |
Handling Form Submission | Process and redirect after successful submissions to enhance UX. |
Working with Databases in Flask
In any web application with Flask, integrating a database is crucial. It allows you to store, retrieve, and manage data effectively. In this guide, you will learn how to work with databases in Flask, focusing on integrating with SQLite or PostgreSQL, utilizing Flask-SQLAlchemy for ORM (Object-Relational Mapping), and performing CRUD (Create, Read, Update, Delete) operations.
Integrating Flask with SQLite or PostgreSQL

Flask offers great flexibility in choosing databases. Both SQLite and PostgreSQL are popular choices, each with its strengths.
SQLite: A Simple Choice for Beginners
- Lightweight: SQLite is easy to set up. It runs in-process with your application, making it ideal for smaller projects or prototyping.
- File-based: All data is stored in a single file, simplifying backups and transfers.
Setting Up SQLite in Flask
To use SQLite, install Flask and create a basic application:
pip install Flask
Here’s how you can set up a simple Flask application with SQLite:
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///site.db'
db = SQLAlchemy(app)
PostgreSQL: A Powerful Option for Larger Applications
- Scalable: PostgreSQL is suitable for larger applications with high traffic.
- Advanced Features: It supports complex queries, indexing, and data types.
Setting Up PostgreSQL in Flask
To use PostgreSQL, you will need to install the necessary packages:
pip install Flask psycopg2
Configure the database URI in your Flask app:
app.config['SQLALCHEMY_DATABASE_URI'] = 'postgresql://username:password@localhost/dbname'
Replace username
, password
, and dbname
with your actual PostgreSQL credentials.
Summary of Database Integration
Database | Advantages | Use Case |
---|---|---|
SQLite | Lightweight, easy to set up | Prototyping, small projects |
PostgreSQL | Scalable, advanced features | Larger applications |
Using Flask-SQLAlchemy for ORM
Flask-SQLAlchemy is an extension that simplifies using SQLAlchemy with Flask. It provides a high-level API for database operations, making it easier to work with data models.
Why Use Flask-SQLAlchemy?
- Simplified Syntax: It reduces boilerplate code needed for database interactions.
- Built-in Migrations: It offers easy database migrations with Flask-Migrate.
Installing Flask-SQLAlchemy
To get started with Flask-SQLAlchemy, install it using pip:
pip install Flask-SQLAlchemy
Defining a Model
You can define a database model by creating a class that inherits from db.Model
. Here’s an example of a simple User model:
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(150), nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
def __repr__(self):
return f"User('{self.username}', '{self.email}')"
Key Components of the Model
db.Column
: Represents a column in the database table.primary_key=True
: Specifies that this column is the primary key.nullable=False
: Indicates that this column cannot be empty.
Creating the Database
Once your models are defined, you can create the database:
with app.app_context():
db.create_all()
This command will create the database tables based on your defined models.
Performing CRUD Operations in Flask
CRUD operations are essential for managing data in any web application with Flask. Using Flask-SQLAlchemy, these operations become straightforward.
Create: Adding a New Record
To add a new user to the database, you can do the following:
new_user = User(username='JohnDoe', email='john@example.com')
db.session.add(new_user)
db.session.commit()
Read: Querying Records
To retrieve records, you can use queries:
users = User.query.all() # Retrieves all users
first_user = User.query.first() # Retrieves the first user
Update: Modifying a Record
To update an existing user’s information, find the user first and then modify the properties:
user = User.query.get(1) # Get user with ID 1
user.username = 'JaneDoe'
db.session.commit()
Delete: Removing a Record
To delete a user from the database:
user_to_delete = User.query.get(1) # Get user with ID 1
db.session.delete(user_to_delete)
db.session.commit()
Summary of CRUD Operations
Operation | Description | Code Example |
---|---|---|
Create | Add a new record | db.session.add(new_user) |
Read | Retrieve records | users = User.query.all() |
Update | Modify an existing record | user.username = 'JaneDoe' |
Delete | Remove a record | db.session.delete(user_to_delete) |
Securing Your Flask Application
Creating a web application with Flask is an exciting journey, but it’s crucial to ensure that your application is secure. In this section, you will learn how to implement user authentication, protect your routes, secure forms, and deploy your Flask application safely.
Implementing User Authentication with Flask-Login
User authentication is vital for any web application. Flask-Login is a powerful extension that simplifies user session management.
What is Flask-Login?
- Session Management: It helps manage user sessions, making it easier to keep track of logged-in users.
- User Convenience: Users can log in and stay authenticated across multiple requests.
Setting Up Flask-Login
To get started, install Flask-Login:
pip install Flask-Login
Here’s how to set up a basic user authentication system:
from flask import Flask, render_template, redirect, url_for, flash
from flask_sqlalchemy import SQLAlchemy
from flask_login import LoginManager, UserMixin, login_user, logout_user, login_required
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your_secret_key'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///site.db'
db = SQLAlchemy(app)
login_manager = LoginManager(app)
class User(db.Model, UserMixin):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(150), nullable=False, unique=True)
password = db.Column(db.String(150), nullable=False)
@login_manager.user_loader
def load_user(user_id):
return User.query.get(int(user_id))
User Registration Example
To register a new user, create a registration form:
@app.route('/register', methods=['GET', 'POST'])
def register():
if request.method == 'POST':
username = request.form['username']
password = request.form['password']
new_user = User(username=username, password=password)
db.session.add(new_user)
db.session.commit()
flash('Registration successful!', 'success')
return redirect(url_for('login'))
return render_template('register.html')
Protecting Routes with Flask-Login
Once user authentication is set up, protecting specific routes becomes essential. This ensures that only authenticated users can access certain areas of your application.
How to Protect Routes
You can protect routes by using the @login_required
decorator. Here’s an example:
@app.route('/dashboard')
@login_required
def dashboard():
return render_template('dashboard.html')
Unauthenticated Access Handling
If an unauthenticated user tries to access a protected route, they will be redirected to the login page. This behavior can be customized:
login_manager.login_view = 'login'
login_manager.login_view = 'login'
Summary of Route Protection
Protection Type | Description |
---|---|
@login_required | Protects the route, requiring authentication. |
Redirection on Failure | Redirects unauthenticated users to the login page. |
Securing Forms with CSRF Protection in Flask
Cross-Site Request Forgery (CSRF) is a type of attack where unauthorized commands are transmitted from a user that the web application trusts. To combat this, Flask provides CSRF protection through the Flask-WTF extension.
Setting Up CSRF Protection
Install Flask-WTF:
pip install Flask-WTF
To enable CSRF protection, include it in your application:
from flask_wtf.csrf import CSRFProtect
csrf = CSRFProtect(app)
Adding CSRF Tokens to Forms
CSRF tokens are automatically included in forms created with Flask-WTF. Here’s an example:
<form method="POST">
{{ form.hidden_tag() }}
{{ form.username.label }} {{ form.username() }}
{{ form.submit() }}
</form>
Deploying Your Flask Application
Once your application is secure, the next step is deployment. This section covers how to deploy your Flask application on Heroku, AWS, and using Docker.
How to Deploy a Flask Application on Heroku
Heroku is a popular cloud platform that makes it easy to deploy applications.
Steps to Deploy on Heroku
- Create a
requirements.txt
file: Include all your dependencies.
pip freeze > requirements.txt
2. Create a Procfile
: This file tells Heroku how to run your app.
web: python app.py
Deploy the Application: Follow these commands:
heroku create
git add .
git commit -m "Initial commit"
git push heroku master
Deploying Flask on AWS with Elastic Beanstalk
AWS Elastic Beanstalk is another robust option for deploying Flask applications.
Steps for AWS Deployment
- Set Up Elastic Beanstalk CLI: Install the CLI for easier deployment.
- Create an Application: Use the following command:
eb init -p python-3.x your-app-name
3. Deploy the Application: Deploy with the following command:
eb create your-env-name
eb deploy
Summary of Deployment Options
Platform | Advantages | Ideal For |
---|---|---|
Heroku | Simple setup, free tier | Small to medium applications |
AWS Elastic Beanstalk | Scalable, robust features | Larger applications with high traffic |
Using Docker to Containerize Flask Applications
Docker is an excellent way to ensure consistency across environments.
Steps to Containerize Your Flask Application
- Create a Dockerfile: This file describes how to build your application image.
FROM python:3.x
WORKDIR /app
COPY . .
RUN pip install -r requirements.txt
CMD ["flask", "run"]
2. Build the Docker Image:
docker build -t your-flask-app .
3. Run the Container:
docker run -p 5000:5000 your-flask-app
Conclusion: Building and Scaling Your Flask Web Application
Creating a web application with Flask is an exciting journey, but the work doesn’t stop once your app is live. Scaling your Flask application to handle increased traffic and maintain performance is just as important as building it in the first place.
Key Takeaways
- Building a Solid Foundation: You’ve learned how to implement core features such as user authentication, route protection, and securing forms. These steps ensure your app is both functional and secure.
- Deploying Your Flask Application: Deploying your app on platforms like Heroku, AWS, or using Docker makes it accessible to users worldwide. Whether you’re just starting out with Heroku or looking for more scalable options with AWS Elastic Beanstalk or Docker, deployment is a critical step in bringing your project to life.
- Scaling Your Flask App: As your Flask web application grows, you’ll need to think about scaling. This could mean moving from a simple SQLite database to PostgreSQL, or implementing load balancing and container orchestration with Docker and Kubernetes.
Tips for Scaling Flask Applications
- Optimize Database Performance: If you’re starting with SQLite, it may be worth upgrading to PostgreSQL or MySQL as your user base grows. This improves performance and provides more advanced database features.
- Use Caching: Adding caching mechanisms such as Redis can significantly speed up your application by storing frequently accessed data in memory.
- Horizontal Scaling: To handle more traffic, you may want to consider horizontal scaling, which involves adding more servers to distribute the load. This can be managed easily with tools like AWS Elastic Load Balancer or Kubernetes.
- Monitoring and Analytics: Make use of tools like New Relic or Prometheus to monitor the health of your application and address any bottlenecks before they become critical issues.
Frequently Asked Questions (FAQs) About Flask Web Development
Is Flask Good for Beginners?
Yes, Flask is excellent for beginners. It’s a lightweight framework that is easy to understand, with minimal setup. The simple structure allows new developers to grasp the basics of web development quickly, while still being powerful enough for more complex projects as they grow.
Can I Use Flask for Large-Scale Applications?
Absolutely, Flask can be used for large-scale applications, though it may require some additional planning. For scalability, integrating tools like Flask-SQLAlchemy, caching solutions like Redis, and deploying on platforms like AWS or using Docker helps manage larger traffic and complex features effectively.
External Resources
Flask Official Documentation
The official docs are a great place to start. They include a thorough step-by-step guide for building your first web app and a reference to every Flask feature.
Flask Mega-Tutorial by Miguel Grinberg
This comprehensive tutorial is well-regarded in the Flask community and covers everything from creating your first Flask app to deploying it.
EmitechLogic: Python Flask Tutorial
For a clear, engaging, and in-depth explanation of building web applications with Flask, EmitechLogic’s Flask Python Tutorial covers the core concepts and gives real-world examples. It’s perfect for beginners and those looking to expand their Flask knowledge.
Leave a Reply