Introduction to Exception and Error Handling in Python
We’ve all been there. You’re working on a Python project, everything seems to be running smoothly, and then—boom! You hit an error, and suddenly your code stops working. It’s frustrating, right? But here’s the good news: errors don’t have to ruin your coding experience. In fact, they can be opportunities to make your code more resilient and strong. That’s where exception and error handling comes in.
In this complete guide, we’ll break down the essentials of error handling in Python, making it easier to understand even if you’re just starting out. From common mistakes like “ZeroDivisionError” to more advanced topics like custom exceptions, this post will walk you through everything you need to know. By the end, you’ll be equipped to handle any unexpected situation your code throws at you without breaking a sweat.
What is Error Handling in Python?
Errors are a part of coding. Whether it’s a typo, accessing something that doesn’t exist, or an unexpected input, mistakes happen. But if not managed properly, these errors can cause your program to crash. Error handling in Python is about managing these situations so your code can keep running smoothly.
In programming, you’ll face two types of problems:
- Errors: Mistakes in the code that prevent it from running. For example, dividing a number by zero causes a
ZeroDivisionError
. - Exceptions: Issues that occur during the execution of the program. These don’t always crash the program but need attention. For instance, trying to open a file that doesn’t exist raises a
FileNotFoundError
.
Why Is It Important to Handle Exceptions Gracefully?
Imagine you’re presenting a Python project, and mid-demo, the program crashes due to an unhandled error. Frustrating, right? This is why handling exceptions is crucial. It allows your code to continue running even when unexpected situations occur.
Handling exceptions ensures that:
- The program doesn’t crash unexpectedly.
- Users get clear, helpful error messages.
- You can find and fix bugs more easily.
In a professional environment, well-handled exceptions can make your code more reliable and user-friendly.
How Python Simplifies Error Handling
Python makes managing errors easy with its try-except blocks. This lets you test a block of code, catch errors, and handle them. If something goes wrong in the try
block, Python jumps to the except
block, allowing your program to recover.
Here’s a simple example:
try:
result = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
finally:
print("This code runs no matter what.")
Instead of crashing, the program catches the error and prints a message.
Common Python Errors and Exceptions
Some frequent Python errors include:
- SyntaxError: Occurs when the code’s structure is incorrect.
- TypeError: Happens when an operation is used on the wrong type.
- ValueError: Triggered when a function gets an argument of the right type but the wrong value.
- FileNotFoundError: Raised when a file you’re trying to open doesn’t exist.
Techniques for Effective Error Handling
Here are some ways to handle exceptions effectively in Python:
- Try-except blocks: Wrap potentially problematic code in
try-except
to handle errors. - Multiple except blocks: Catch different exceptions with specific
except
clauses. - Raising exceptions: Create custom exceptions when necessary.
Example:
try:
age = int(input("Enter your age: "))
if age < 0:
raise ValueError("Age can't be negative!")
except ValueError as e:
print(e)
In this case, if the user inputs a negative age, the code raises a custom ValueError
.
Best Practices for Error Handling
To ensure your error handling is effective, follow these best practices:
- Catch specific exceptions: Handle only the exceptions you expect.
- Log errors: Keep track of issues for easier debugging.
- Clean up resources: Use the
finally
block to close files or release resources.
Types of Errors in Python
Syntax Errors
What is a Syntax Error in Python?
A syntax error occurs when Python cannot understand the code you’ve written. This happens because the code does not follow the correct rules of the Python language. Think of syntax as the grammar of Python. Just like a sentence needs to be structured properly to convey meaning, your code needs the right syntax to work.
Example of Syntax Errors
Common examples of syntax errors include:
- Missing colons (
:
) after function definitions or control statements:
def my_function() # Missing colon
print("Hello")
- Incorrect indentation, which is crucial in Python:
def my_function():
print("Hello") # This line should be indented
- Mismatched parentheses:
print("Hello" # Missing closing parenthesis
When Python encounters any of these mistakes, it raises a SyntaxError. The error message usually points to the line where Python got confused, making it easier to locate the problem.
How to Avoid Common Syntax Errors
To avoid syntax errors, consider these tips:
- Always use proper indentation: Make sure your code blocks are indented correctly. In Python, indentation is essential to define the structure of your code.
- Check for colons: Ensure every function definition and control structure has a colon at the end.
- Use a code editor: Tools like VS Code or PyCharm highlight syntax errors, helping you catch them early.
- Read error messages carefully: When you encounter an error, take the time to read the message. It often gives you valuable hints about what went wrong.
By following these practices, you can significantly reduce the likelihood of syntax errors in your Python code.
Logical Errors
Definition of Logical Errors
A logical error occurs when your code runs without crashing but produces incorrect results. This type of error can be frustrating because the program behaves as if everything is correct. However, the output doesn’t match your expectations.
Examples of Logical Errors in Code
Here’s a simple example of a logical error:
def calculate_area(radius):
area = radius * 2 # Incorrect formula
return area
print(calculate_area(5)) # Outputs 10 instead of 78.5
In this example, the formula to calculate the area of a circle is wrong. Instead of multiplying by π (pi), the code multiplies by 2. The program runs fine, but the output is not what we want.
Debugging Tips to Detect Logical Errors
Finding and fixing logical errors can be challenging. Here are some tips to help you:
- Use print statements: Insert print statements throughout your code to check the values of variables at different stages. This can help you track where the logic goes wrong.
- Use a debugger: Tools like Python’s built-in debugger (pdb) allow you to step through your code line by line, making it easier to identify logical mistakes.
- Review your algorithms: Sometimes, the logic in your algorithms may be flawed. Take a moment to review the steps and calculations to ensure they align with your intentions.
- Ask for a second opinion: Sometimes, explaining your code to someone else can help you spot mistakes you might have missed. This practice is known as “rubber duck debugging.”
By being systematic and thorough in your approach, you can catch and correct logical errors in your code more effectively.
Runtime Errors
Explanation of Runtime Errors
Runtime errors occur while the program is running. These errors happen due to unforeseen circumstances that the program cannot handle. Unlike syntax errors, which are caught when the program starts, runtime errors only show up during execution.
Examples of Runtime Errors and How They Occur
Common examples of runtime errors include:
- ZeroDivisionError: Raised when trying to divide a number by zero:
result = 10 / 0 # Causes ZeroDivisionError
- TypeError: Occurs when an operation is performed on an inappropriate type, such as trying to add a string and an integer:
result = "Hello" + 5 # Causes TypeError
- IndexError: Happens when trying to access an index that is out of range in a list:
my_list = [1, 2, 3]
print(my_list[5]) # Causes IndexError
These errors can cause your program to crash unless you handle them properly.
How to Handle Runtime Errors
Handling runtime errors is crucial to ensure your program continues to run smoothly. Here’s how you can manage them:
- Use try-except blocks: Wrap the code that might raise an error in a
try
block. If an error occurs, Python jumps to theexcept
block to handle it.
try:
result = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
Provide user-friendly messages: When an error occurs, make sure to inform users in a way that’s easy to understand. Avoid technical jargon.
Log errors: Keeping a record of errors can help you understand what went wrong and why. This can be helpful for future debugging.
Test your code thoroughly: Running multiple test cases helps you identify potential runtime errors before your code goes live.
What Are Python Exceptions?
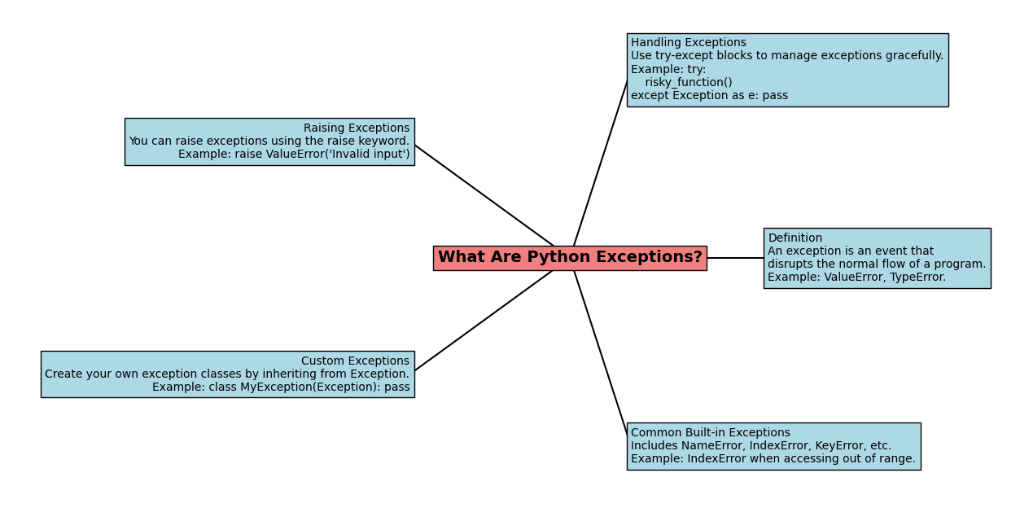
Definition of Exceptions in Python
In Python, an exception is an event that occurs during the execution of a program, interrupting its normal flow. It typically represents an error or unexpected condition that Python cannot handle on its own. Exceptions can be caught and handled using try-except blocks to prevent the program from crashing.
Differences Between Errors and Exceptions
In Python, it is essential to understand the difference between errors and exceptions. While both terms may seem similar, they refer to different concepts in programming.
- Errors are issues that occur when the program cannot execute. These can be syntax errors, which arise from incorrect code structure, or runtime errors that occur during execution, like trying to divide by zero.
- Exceptions, on the other hand, are events that disrupt the normal flow of a program. They can be anticipated and handled using specific mechanisms in Python. While errors typically indicate serious issues that need immediate attention, exceptions can often be caught and managed.
Understanding these differences is crucial for effective exception and error handling in Python. By recognizing that exceptions are part of the normal operation of a program, you can design your code to handle unexpected situations gracefully.
Importance of Managing Exceptions in Python Code
Managing exceptions is a vital part of writing strong Python code. When exceptions are handled properly, programs can:
- Prevent Crashes: By catching exceptions, you can prevent your program from crashing. This improves user experience by keeping the application running smoothly.
- Provide Useful Feedback: Proper exception handling allows developers to provide informative messages to users, making it clear what went wrong and how to fix it.
- Maintain Control Flow: Instead of stopping execution, you can direct the program to continue or take alternative actions. This control flow can enhance the functionality of applications.
For example, consider a simple program that asks for user input. If the input is invalid, instead of crashing, the program can catch the exception and prompt the user to try again.
Built-in Exception Types in Python
List of Common Built-in Exceptions
Python has several built-in exceptions that developers frequently encounter. Understanding these exceptions will help you manage them effectively. Here are some common ones:
Exception Type | Description |
---|---|
ZeroDivisionError | Raised when a division or modulo operation is performed with zero as the divisor. |
TypeError | Occurs when an operation or function is applied to an object of inappropriate type. |
IndexError | Raised when a sequence subscript is out of range. |
ValueError | Happens when a function receives an argument of the correct type but an inappropriate value. |
KeyError | Raised when trying to access a dictionary with a key that does not exist. |
FileNotFoundError | Occurs when trying to open a file that cannot be found. |
How and When These Exceptions Are Raised
Each built-in exception has specific circumstances under which it is raised. For instance:
- ZeroDivisionError: This exception occurs when the code attempts to divide a number by zero.
try:
result = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
- TypeError: This exception is raised when an operation is performed on incompatible types. For example, trying to concatenate a string and an integer.
try:
result = "Hello" + 5
except TypeError:
print("Cannot concatenate string and integer.")
- IndexError: This exception is raised when trying to access an index in a list that does not exist.
my_list = [1, 2, 3]
try:
print(my_list[5])
except IndexError:
print("Index is out of range.")
Recognizing these exceptions and knowing how to handle them is critical for effective exception and error handling in Python.
Custom Exceptions in Python
Why and How to Create Custom Exceptions
While Python’s built-in exceptions cover many scenarios, there are times when you may want to define your own exceptions. Custom exceptions allow you to handle specific situations unique to your application.
Creating a custom exception can enhance code readability and maintainability. It can provide clearer insights into what went wrong, especially in complex applications.
Example Code for Creating Custom Exceptions
Here’s how you can create and raise a custom exception in Python:
class MyCustomError(Exception):
"""Custom exception for specific error handling."""
pass
def check_value(value):
if value < 0:
raise MyCustomError("Value cannot be negative!")
else:
print("Value is acceptable.")
try:
check_value(-5)
except MyCustomError as e:
print(e)
In this example, we define a custom exception MyCustomError
that inherits from the built-in Exception
class. The function check_value
raises this custom exception when the input value is negative. By catching this specific exception, we can provide targeted feedback to the user.
Must Read
- Master Python String Formatting: Complete Guide
- 8 Advanced Types of AI Agents You Should Know About
- Escape Sequences and Raw Strings in Python: The Complete Guide
- Prime Factorization Algorithm in Python
- How to Get the First Digit of a Number in Python
How to Handle Exceptions in Python?
Using try and except Blocks
Basic Syntax of try-except Blocks
In Python, the try
and except
blocks are the foundation for handling exceptions. The try block contains the code that may potentially raise an exception. If an exception occurs, the flow of control is transferred to the except block.
Here’s the basic syntax:
try:
# Code that may raise an exception
risky_operation()
except SomeException:
# Code to handle the exception
handle_exception()
By using try
and except
, you can prevent your program from crashing when an error occurs. Instead, you can provide a meaningful response.
Example of Handling Multiple Exceptions
It is common to handle multiple exceptions in a single block. This can make your code cleaner and more manageable. For instance, if a function can raise different types of exceptions, you can catch them all at once:
try:
# Code that may raise multiple exceptions
user_input = int(input("Enter a number: "))
result = 10 / user_input
except (ValueError, ZeroDivisionError) as e:
print(f"An error occurred: {e}")
In this example, both ValueError
and ZeroDivisionError
are caught. This makes the code more efficient and allows you to manage multiple scenarios without repeating yourself.
Nested try-except Blocks
Sometimes, you may want to use nested try-except blocks. This approach is helpful when you need to handle exceptions at different levels of your code. Here’s how it works:
try:
print("Trying to read a file.")
with open("file.txt") as file:
try:
content = file.read()
number = int(content)
except ValueError:
print("Could not convert data to an integer.")
except FileNotFoundError:
print("The file was not found.")
In this example, the outer try
block attempts to open a file, while the inner block tries to read and convert its contents. If the file doesn’t exist, a FileNotFoundError
is raised. If the content cannot be converted to an integer, a ValueError
is raised. This way, different exceptions can be managed in a structured manner.
Handling Multiple Exceptions
Combining Multiple Exceptions in a Single Block
You can combine multiple exceptions in a single except
block to handle them uniformly. This approach reduces redundancy and keeps the code clean.
try:
# Code that may raise different exceptions
result = 10 / int(input("Enter a number: "))
except (ZeroDivisionError, ValueError) as e:
print(f"Error occurred: {e}")
In this example, if the user inputs zero or a non-integer, the code will handle both exceptions in the same way. This saves time and effort while maintaining clarity.
Example of Handling Multiple Exception Types
Here’s another example to illustrate handling multiple exception types:
def process_data(data):
try:
# Attempt to process the data
total = sum(data)
average = total / len(data)
except ZeroDivisionError:
print("Error: Division by zero. The data list is empty.")
except TypeError:
print("Error: Invalid data type. Ensure all elements are numbers.")
else:
print(f"The average is: {average}")
data = [1, 2, 3]
process_data(data) # Works fine
empty_data = []
process_data(empty_data) # Triggers ZeroDivisionError
mixed_data = [1, 'two', 3]
process_data(mixed_data) # Triggers TypeError
In this case, the function process_data
manages exceptions for both empty data and invalid data types, providing meaningful feedback.
Using the finally Block
What is the finally Block and Its Purpose?
The finally
block is always executed after the try
and except
blocks, regardless of whether an exception was raised or not. This is useful for cleaning up resources, such as closing files or database connections.
try:
file = open("example.txt", "r")
# Perform file operations
except FileNotFoundError:
print("File not found.")
finally:
file.close() # This will always execute
In this example, the file will be closed whether an exception occurs or not. Using the finally
block ensures that necessary cleanup is performed.
Code Examples Showing the Use of finally
Here’s a practical example of using finally
:
try:
number = int(input("Enter a number: "))
result = 10 / number
except ZeroDivisionError:
print("Error: Division by zero.")
finally:
print("Execution complete.")
In this code, the message “Execution complete.” will be printed regardless of whether an error occurred. This is a great way to inform users that the program has finished running.
Ensuring Resources are Cleaned Up
The finally
block is essential for resource management. You can use it to ensure that all resources are released properly:
import sqlite3
try:
connection = sqlite3.connect("example.db")
cursor = connection.cursor()
# Perform database operations
except sqlite3.Error as e:
print(f"Database error: {e}")
finally:
if connection:
connection.close() # Ensure the database connection is closed
print("Database connection closed.")
In this example, the database connection will always be closed, preventing resource leaks.
Using the else Block
What is the else Block in Exception Handling?
The else
block can be used after the try
and except
blocks. It executes if the code in the try
block does not raise any exceptions. This is a great way to run code that should only occur if no errors were encountered.
How and When to Use It
Using the else
block enhances code readability. It separates the error handling from the rest of the code, making it clearer to understand the flow of logic.
try:
number = int(input("Enter a number: "))
except ValueError:
print("That's not a valid number.")
else:
print(f"The number is {number}.") # This runs only if no exceptions occur
In this example, the message about the number will only display if the conversion to an integer is successful. This keeps the flow of logic simple.
Example Code to Demonstrate Else Block Functionality
Here’s another example showcasing the else
block:
def calculate_average(numbers):
try:
total = sum(numbers)
average = total / len(numbers)
except ZeroDivisionError:
print("Error: No numbers provided.")
else:
print(f"The average is {average:.2f}")
calculate_average([10, 20, 30]) # Outputs the average
calculate_average([]) # Outputs error message
In this case, the average is only calculated if there are numbers in the list. If the list is empty, the ZeroDivisionError
is handled gracefully.
Raising Exceptions in Python
What Does Raising an Exception Mean?
When we talk about raising an exception in Python, we’re referring to a way of signaling that something unexpected has happened in our code. The raise
keyword is used to throw an exception. This means that you can create your own exceptions, or use built-in exceptions, to indicate that something went wrong.
Explanation of the raise
Keyword
The raise
keyword is powerful and flexible. It allows programmers to generate exceptions intentionally, rather than waiting for Python to do it automatically. Raising exceptions is crucial in creating exception and error handling in Python, as it helps maintain control over the flow of a program.
Here’s how it works:
raise Exception("This is a custom error message.")
This line of code will immediately stop the execution of the program and raise an error with the provided message. Using raise
, you can interrupt normal processing and switch to handling an error.
How and When to Raise Exceptions
You might want to raise an exception in several situations. For instance, if a function receives an argument that doesn’t meet specific criteria, raising an exception can help prevent unexpected behavior.
Here’s a practical example:
def set_age(age):
if age < 0:
raise ValueError("Age cannot be negative.")
print(f"Your age is set to {age}.")
In this example, if someone tries to set a negative age, a ValueError
will be raised. This provides immediate feedback that something is wrong, preventing the function from continuing with an invalid value.
Customizing Exception Messages
One of the best practices in exception and error handling in Python is to provide clear and helpful messages when raising exceptions. Customizing exception messages makes it easier for users (or developers) to understand what went wrong.
Adding Custom Messages to Exceptions
When raising exceptions, you can include specific details in your messages. This can greatly aid debugging and user understanding.
For example:
def divide_numbers(x, y):
if y == 0:
raise ZeroDivisionError("Cannot divide by zero. Please provide a non-zero denominator.")
return x / y
In this function, if a user attempts to divide by zero, the error message clearly indicates what the issue is and suggests a solution. This type of clarity can make a significant difference, especially in complex applications.
Example Code for Raising Exceptions with Personalized Messages
Here’s another example that demonstrates raising exceptions with personalized messages:
def check_positive_number(num):
if num < 0:
raise ValueError(f"{num} is not a positive number. Please enter a positive value.")
print(f"The number {num} is valid.")
In this example, if the user inputs a negative number, they will receive a message that specifically indicates the problem. Personalization in error messages fosters a better user experience.
Best Practices for Raising Exceptions
Raising exceptions is not just about using the raise
keyword. It also involves knowing when and where to raise exceptions effectively. Here are some best practices to consider:
When and Where to Use raise
in Your Python Code
- Validate Input: Always validate function inputs and raise exceptions if they don’t meet expected criteria.
- Resource Management: When working with resources like files or databases, raise exceptions if there are issues with opening or accessing them.
- Critical Errors: Use
raise
for critical errors that should stop further execution, such as configuration issues or unexpected states in the program.
Ensuring Clarity in Error Reporting
When raising exceptions, clarity is paramount. Follow these tips to ensure your error reporting is effective:
- Be Specific: Use the most appropriate exception type to describe the error.
- Include Details: Provide context in the error message so users can understand what went wrong.
- Document Exceptions: In your function documentation, specify which exceptions might be raised and under what conditions.
Here’s a summary in tabulated form:
Best Practice | Description |
---|---|
Validate Input | Check input values and raise exceptions for invalid data. |
Resource Management | Raise exceptions when accessing files or databases fails. |
Critical Errors | Use raise for issues that halt execution, like config errors. |
Be Specific | Select appropriate exception types for clarity. |
Include Details | Add helpful messages to guide users in resolving errors. |
Document Exceptions | Clearly state which exceptions your functions may raise. |
Exception Hierarchy in Python
Understanding the Exception Class Hierarchy
Python uses a structured hierarchy to manage exceptions, making it easier to handle different types of errors in a consistent way. At the top of this hierarchy is the BaseException
class, and every exception in Python inherits from it. This structure allows you to catch broad types of errors or specific ones depending on your needs.
Overview of Python’s Exception Hierarchy
In Python, exceptions are organized into a hierarchy of classes, and this helps categorize errors based on their type. All exceptions, including built-in ones like ValueError
or TypeError
, inherit from the BaseException
class. Below this is the Exception
class, which serves as a base for most error types you encounter in everyday programming.
Here’s a simple diagram to visualize this hierarchy:
In this diagram:
BaseException
is at the top, followed byException
.- Under
Exception
, you see specific error types likeArithmeticError
orLookupError
. - These categories further branch into more specific errors like
ZeroDivisionError
orIndexError
.
By organizing exceptions this way, Python makes it easier for developers to write error handling code that is both precise and efficient. You can choose to handle a broad category of exceptions or catch very specific ones based on your application’s needs.
How Exceptions Inherit from Base Classes
In Python’s exception hierarchy, every class inherits properties and behaviors from its parent class. This means that when you catch an exception, you are also catching all of its derived exceptions. For example, if you catch an Exception
, it will also catch errors like ZeroDivisionError
or IndexError
, because these are subclasses of Exception
.
Here’s an example:
try:
x = 1 / 0
except Exception as e:
print(f"Caught an exception: {e}")
In this case, the ZeroDivisionError
is a subclass of Exception
, so it gets caught by the except Exception
block. This is useful if you want to handle many types of errors with a single block of code.
However, if you want more control over which specific exceptions to catch, you can target the child classes directly:
try:
x = 1 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
This level of specificity can help you provide more meaningful feedback to users or log more detailed error information in your programs.
BaseException vs Exception: What’s the Difference?
Although most exceptions you’ll encounter in Python inherit from the Exception
class, there’s another class at the very top of the hierarchy called BaseException
. Understanding the difference between these two can help you avoid common mistakes and write more effective error-handling code.
Explanation of BaseException
and Exception
Base Classes
- BaseException: This is the root class for all exceptions in Python. It is rarely caught directly and is usually reserved for system-level exceptions like
SystemExit
,KeyboardInterrupt
, orGeneratorExit
. These exceptions typically indicate that something critical has happened, such as the program being stopped by the user. - Exception: This is a subclass of
BaseException
and is the base class for all standard errors that a programmer might want to catch in their code. Most exceptions, such asValueError
,TypeError
, orIndexError
, inherit from this class. When writing error-handling code, you will usually catch exceptions at this level or lower.
Here’s a quick comparison in tabulated form:
Class | Use Case | Examples |
---|---|---|
BaseException | System-level errors, rarely caught directly | SystemExit , KeyboardInterrupt |
Exception | Programmer-defined errors and typical runtime errors | ValueError , TypeError , KeyError |
When to Use BaseException
vs Exception
In most cases, you will only need to catch exceptions derived from the Exception
class. Catching BaseException
is generally discouraged because it can also capture critical system-level exceptions that you may not want to handle, such as KeyboardInterrupt
. These exceptions are often better left to propagate naturally so the system can exit cleanly.
Here’s an example:
try:
while True:
pass
except BaseException:
print("Caught a system-level exception")
In this case, even pressing Ctrl+C
(which raises KeyboardInterrupt
) would be caught, preventing the program from exiting. This is usually not what you want.
On the other hand, catching exceptions at the Exception
level allows you to handle most programming errors without interfering with system-level events.
try:
x = int(input("Enter a number: "))
except Exception as e:
print(f"An error occurred: {e}")
In this case, we only catch standard errors like ValueError
or TypeError
, but the program will still exit cleanly if the user presses Ctrl+C
to stop it.
Best Practices for Exception Handling
Avoid Using Bare Except Blocks
Bare except blocks are often tempting for developers, especially when they want to catch any and all errors without specifying what kind of exception may occur. But using bare except can lead to unintended consequences and make debugging much harder.
Why Using Bare Except is Risky
When you write a block like this:
try:
# Some code
except:
print("An error occurred")
You are essentially telling Python to catch every possible exception, including ones you might not expect or want to handle, such as system-level exceptions like KeyboardInterrupt
or SystemExit
. These are critical exceptions that should usually not be caught, as they control the system’s shutdown process or stop a program during execution.
The risk lies in hiding important errors, making it difficult to understand what went wrong in your code. Instead of getting specific feedback, you’ll end up with generic messages that don’t point you toward the actual problem.
How to Catch Specific Exceptions Instead
To avoid this issue, you should always catch specific exceptions. Not only does this make your code clearer, but it also ensures that you’re handling only the errors you expect.
try:
result = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
except ValueError:
print("Invalid input!")
In this case, specific exception handling allows you to respond to different errors appropriately. You can even combine multiple exceptions in one block to handle them similarly:
try:
result = int(input("Enter a number: ")) / 0
except (ZeroDivisionError, ValueError) as e:
print(f"Error: {e}")
This approach improves both clarity and error handling efficiency in your code. You’ll be catching the right errors without accidentally interfering with system-level behavior.
Using Logging for Better Error Handling
Logging exceptions is crucial for understanding what went wrong in your application, especially in more complex projects or when debugging production code. Python’s logging
module makes it easy to log exceptions and other useful messages without affecting the program’s flow.
How to Use Python’s Logging Module to Log Exceptions
Logging provides a record of errors that happen while the program runs, without stopping execution like print()
would. For example, in a web application, logging an error can help the development team track down bugs without crashing the user experience.
Here’s how to log exceptions using Python’s logging
module:
import logging
logging.basicConfig(level=logging.ERROR)
try:
result = 10 / 0
except ZeroDivisionError as e:
logging.error(f"An error occurred: {e}")
In this example, the error is recorded with the ERROR level in the logs, so it’s easy to trace back the issue later. You can configure the logging output to write to a file, the console, or both, depending on the environment you’re working in.
Best Practices for Logging Exceptions
- Log the full traceback: It’s often helpful to log the full stack trace so you can see where the error originated in the code. You can do this by adding
exc_info=True
to the logging call:
logging.error("An error occurred", exc_info=True)
- Choose the correct logging level: Not all errors are created equal. Use different logging levels (
DEBUG
,INFO
,WARNING
,ERROR
,CRITICAL
) to prioritize which messages need immediate attention. - Avoid over-logging: Logging too much can clutter your logs and make it difficult to find meaningful information. Only log critical information that will help diagnose issues.
Avoiding Overuse of Exceptions
Exceptions in Python are powerful, but they should not be overused. While it’s easy to catch errors with exception blocks, sometimes it’s better to rely on if-else logic to handle situations where you expect things could go wrong.
When to Rely on If-Else Logic Instead of Exceptions
Instead of waiting for an exception to happen, it’s often smarter to check for potential issues upfront using simple if-else conditions. For example, instead of letting a division by zero exception happen, you could check the denominator before performing the division:
denominator = 0
if denominator == 0:
print("Cannot divide by zero!")
else:
result = 10 / denominator
By using conditional checks, you avoid triggering exceptions unnecessarily and improve the clarity of your code. It’s better to prevent an error than to rely on catching it later.
Avoid Using Exceptions for Control Flow
A common mistake is to use exceptions to control the flow of a program, especially when checking user input or validating conditions. This can make your code less efficient and harder to maintain.
Here’s an example of incorrect control flow with exceptions:
try:
result = 10 / int(input("Enter a number: "))
except ValueError:
print("Invalid input!")
Instead, handle the validation with if-else logic to ensure better readability and performance:
user_input = input("Enter a number: ")
if user_input.isdigit():
result = 10 / int(user_input)
else:
print("Invalid input!")
This way, your program runs more predictably, without relying on exceptions to control its logic.
Advanced Python Error Handling Techniques
Context Managers for Resource Management
Managing resources like files or database connections can be tricky, especially when errors occur. Context managers provide a way to handle exceptions while automatically taking care of resource management in Python. They help make your code cleaner and more predictable.
How Context Managers Work to Handle Exceptions
When using a context manager, Python makes sure that a certain block of code is always executed, whether an exception is raised or not. This is especially useful for things like file handling or database connections, where resources need to be closed or cleaned up properly after being used.
A common way to use a context manager is with the with
statement, which ensures resources are released, even if an error occurs in the code block. The beauty of the with
statement lies in its simplicity and reliability.
Example of Using the with
Statement for File Handling
Let’s consider file handling. If we open a file without using a context manager, we may forget to close it, which can lead to memory leaks or locked resources. With a context manager, Python guarantees the file will be closed, no matter what.
try:
with open("example.txt", "r") as file:
data = file.read()
print(data)
except FileNotFoundError as e:
print(f"Error: {e}")
In this code, the with
statement ensures the file is properly closed after reading. Even if an exception like FileNotFoundError
is raised, the file is still closed automatically.
By using context managers, you can also avoid cluttering your code with explicit try-finally
blocks, making it cleaner and more reliable.
Exception Chaining (__cause__
and __context__
)
Python supports exception chaining, allowing developers to handle multiple exceptions while keeping track of the original error. This feature helps provide better error traceability, making debugging easier.
Explanation of Exception Chaining in Python
Exception chaining refers to the process where one exception causes another. There are two key attributes for chaining exceptions: __cause__
and __context__
.
__cause__
: This is used when one exception is directly raised by another. You explicitly set the cause by usingraise ... from ...
. This makes it clear that one exception led to another.__context__
: This is used when an exception occurs while handling another exception but isn’t directly caused by it. Python automatically sets the__context__
attribute when an exception is raised in theexcept
block of another exception.
Examples of Raising Chained Exceptions for Better Traceability
Here’s an example to show how exception chaining works:
try:
result = 10 / 0
except ZeroDivisionError as e:
raise ValueError("A value error occurred") from e
In this example, ValueError
is raised after catching a ZeroDivisionError
, with the from e
making it clear that the ZeroDivisionError
caused the ValueError
.
If you check the traceback, Python will clearly show both exceptions, giving better traceability.
Working with Asynchronous Code and Exceptions
Handling exceptions in asynchronous code can be a bit more complex than in regular synchronous code. Python’s asyncio
module makes it easier to work with asynchronous tasks and catch exceptions in coroutines.
How to Handle Exceptions in Async Code Using asyncio
When working with async code, we use async
and await
to define and run asynchronous tasks. However, exceptions can still occur inside these tasks, and they need to be handled properly. Just like in regular code, we use try-except
blocks, but the challenge is that these exceptions might occur asynchronously.
Example of Catching Exceptions in Coroutines
Let’s see how you can handle exceptions in asynchronous code:
import asyncio
async def divide(a, b):
try:
result = a / b
print(f"The result is {result}")
except ZeroDivisionError as e:
print(f"Error: Cannot divide by zero - {e}")
async def main():
await divide(10, 0)
asyncio.run(main())
In this code, divide
is an asynchronous function that performs division. If b
is zero, it raises a ZeroDivisionError
, which we catch using the try-except
block inside the coroutine.
By using asyncio.run()
, we can run the asynchronous code in Python. This example shows that exception handling works similarly in async functions, but you must be aware of when and where the exceptions can be raised.
Debugging Tools and Techniques
Using Python’s Built-In Debugger (pdb)
Debugging your code is an essential part of developing reliable Python programs. One of the most powerful yet often underused tools is Python’s built-in debugger, pdb. If you’ve ever struggled with figuring out why your code isn’t working as expected, pdb can be your best friend.
How to Debug Python Code with pdb
The pdb module is Python’s standard debugger, allowing you to pause code execution at certain points, examine variables, and step through the code line by line. This is especially helpful when you’re trying to track down bugs that don’t produce obvious errors.
Here’s how you can use pdb to debug your code:
- Import the
pdb
module: In your script, import thepdb
module to start using it. - Set a breakpoint: You can insert
pdb.set_trace()
at any line in your code where you want execution to stop. This gives you control to inspect the state of your program at that point. - Step through your code: Once the program hits the breakpoint, you can step through the code line by line, check the value of variables, and even modify them if needed.
Example of Setting Breakpoints and Inspecting Variables
Let’s say you’re trying to debug a simple Python function that divides two numbers, but it’s not working correctly.
import pdb
def divide(a, b):
pdb.set_trace() # This is where the debugger will stop
return a / b
result = divide(10, 0)
In this code, you might not immediately see that the division by zero is causing an error. When you run the code, it will pause at the pdb.set_trace()
line. From there, you can use pdb commands like n
(next) to move to the next line, or p
(print) to inspect the value of variables:
> divide(10, 0)
(Pdb) p a
10
(Pdb) p b
0
(Pdb) n
ZeroDivisionError: division by zero
By stepping through the code and inspecting variables, you can catch mistakes early and understand why your program is misbehaving. This method is especially helpful for more complex issues where print statements just aren’t enough.
The traceback
Module for Detailed Error Information
Another tool that can help with debugging, especially when dealing with complicated code, is Python’s traceback module. When an error occurs, Python typically shows a simple error message. However, in more complex scenarios, you might need a detailed stack trace to fully understand what went wrong.
How to Use the traceback
Module for Error Analysis
The traceback module allows you to extract, format, and display error details in a more structured way. You can use it to log errors to a file or output them in a more readable format.
To use traceback
, you need to import the module and call its functions in your exception handling code. It’s particularly useful when you’re trying to debug exceptions in larger codebases or when running scripts that don’t have an interactive debugger attached.
Extracting Detailed Stack Traces for Complex Debugging
Let’s look at an example where using the traceback
module can be beneficial:
import traceback
def faulty_function():
try:
result = 10 / 0
except ZeroDivisionError:
print("Oops, an error occurred!")
traceback.print_exc() # Print the detailed traceback
faulty_function()
In this code, the traceback.print_exc()
function prints the full stack trace of the exception, which includes information about what line of code caused the error, along with the type of exception and the error message.
The output might look something like this:
Oops, an error occurred!
Traceback (most recent call last):
File "example.py", line 7, in faulty_function
result = 10 / 0
ZeroDivisionError: division by zero
This detailed traceback provides more context about the error than just the basic print()
statement. It’s especially helpful in Exception and Error Handling in Python, where having a clear view of the problem can save hours of debugging time.
Common Mistakes in Python Exception Handling
Swallowing Exceptions Silently
One of the biggest pitfalls in Exception and Error Handling in Python is silently swallowing exceptions—when your code catches an exception but doesn’t handle it properly. This can lead to unpredictable behavior, making debugging a nightmare because you might not even realize that something went wrong.
Why Silently Ignoring Exceptions is Dangerous
When exceptions are ignored without any reporting or logging, you lose crucial insights into the state of your program. Imagine working on a complex application where a critical function fails, but instead of raising an error, the program quietly continues, leaving you confused about why the results are off. This is why swallowing exceptions can be so harmful—it obscures the root cause of problems, and small issues can escalate into major ones.
Consider this example:
try:
result = 10 / 0
except ZeroDivisionError:
pass # Silent failure, nothing happens here
Here, the ZeroDivisionError
is caught, but the pass
statement ensures that no action is taken. The program moves on, but the root problem—dividing by zero—remains unresolved.
Why is this risky?
- Silent failures lead to bugs that are hard to detect and fix.
- You miss out on valuable error information that could have been logged for later review.
- Critical issues might go unnoticed, affecting the program’s output and logic.
How to Avoid it with Proper Logging and Error Reporting
A better way to handle exceptions is to ensure that they are logged or reported, even if you’re not actively resolving the issue right away. Using Python’s logging
module is an excellent way to capture exceptions.
import logging
logging.basicConfig(level=logging.ERROR)
try:
result = 10 / 0
except ZeroDivisionError as e:
logging.error(f"Error occurred: {e}") # Log the error with context
This way, even though the error is caught, it’s not swallowed silently. Instead, it’s logged with useful information, helping you identify issues later when debugging.
Key points to remember:
- Always log exceptions with meaningful error messages.
- Even if you don’t fix the issue immediately, ensure there’s a record of it for future troubleshooting.
- Silent failure is almost always a bad practice in Exception and Error Handling in Python.
Using Exceptions for Control Flow
Another common issue in Exception and Error Handling in Python is overusing exceptions for control flow. While exceptions are designed to handle unexpected situations, using them to direct the flow of your program is generally considered an anti-pattern.
Why Overusing Exceptions for Logic Flow is an Anti-Pattern
The primary purpose of exceptions is to handle errors, not to replace regular logic structures like if-else
. Using exceptions for control flow can make your code harder to understand and maintain. Exceptions should signal that something unusual has occurred—not that the next step in your program should execute.
Here’s an example of bad practice where exceptions are used to control the program’s flow:
try:
result = some_list[5] # May raise an IndexError
except IndexError:
result = None # Handle out-of-bounds with an exception
Instead of relying on the IndexError
to handle the out-of-bounds scenario, it would be better to use a logical check:
if len(some_list) > 5:
result = some_list[5]
else:
result = None
In this example, checking the length of the list before accessing an index is much clearer and prevents unnecessary exceptions. Exceptions should only be used for truly exceptional situations, not routine logic.
Alternatives to Using Exceptions in Your Code
Here are some alternatives to using exceptions for control flow:
- Conditional Statements: Use
if-else
logic for expected scenarios, like checking the length of a list before accessing an index. - Built-in Methods: Functions like
get()
for dictionaries can prevent the need for exceptions. For example,my_dict.get('key')
returnsNone
if the key doesn’t exist, instead of raising aKeyError
. - Try-Except for Truly Unexpected Scenarios: Reserve exceptions for situations where something unexpected happens, such as I/O errors or network issues.
By avoiding overuse of exceptions in logic flow, your code becomes:
- Easier to read: Readers can follow the logic without having to mentally track possible exceptions.
- More efficient: Exceptions come with overhead, so reducing their use can improve performance.
- Less prone to hidden errors: You’ll reduce the chances of catching and swallowing errors unintentionally.
Conclusion on exception and error handling
To recap, Exception and Error Handling in Python is an essential skill for writing clean, reliable, and maintainable code. Throughout this article, we explored several key concepts:
- Avoid using bare
except
blocks: They obscure the source of errors and make debugging much harder. Always specify the type of exception you’re catching. - Logging for better error handling: Logging exceptions ensures that you capture useful information when something goes wrong without silently swallowing errors.
- Avoiding overuse of exceptions: Exceptions should be reserved for truly exceptional situations. Use regular control flow structures like
if-else
for expected logic. - Swallowing exceptions silently: Ignoring exceptions can lead to undetected bugs, which can escalate over time.
- Using exceptions for control flow: Overusing exceptions as part of normal logic is considered bad practice and should be avoided.
Importance of Mastering Exception Handling in Python
Mastering these concepts not only improves the readability and efficiency of your code but also reduces the likelihood of unintended errors. Proper exception handling ensures that your programs behave predictably even when things go wrong. This can save time, both in debugging and maintaining code, and build more trust with users and collaborators.
Encouragement to Follow Best Practices
As you continue to work with Python, always strive to follow best practices in error handling:
- Log errors rather than hiding them.
- Catch only the exceptions you’re prepared to handle.
- Keep your code clean by not overusing exceptions for normal control flow.
By avoiding common pitfalls and adopting these practices, you’ll be well on your way to writing resilient and maintainable Python code.
FAQs on Python exception and error handling
Errors are issues that occur at runtime, like syntax errors, which stop the program from running. Exceptions, on the other hand, are events that disrupt the program flow but can be caught and handled, allowing the program to continue.
You can handle multiple exceptions by specifying them in a single except
block using a tuple. For example:
try:
# code that may raise an exception
except (ValueError, KeyError):
# handle both ValueError and KeyError
Yes, you can create custom exceptions by subclassing the Exception
class. This allows you to define specific error types for your program.
class MyCustomError(Exception):
pass
The finally
block is used to execute code, regardless of whether an exception occurred. It’s typically used for cleaning up resources like closing files or database connections.
try:
# code that might raise an exception
finally:
# cleanup code
External Resources on exception and error handling
Python Official Documentation – Errors and Exceptions
The official Python tutorial provides an in-depth guide on how errors and exceptions work in Python, including try-except
blocks, raising exceptions, and creating custom exceptions.
Python Logging Module Documentation
This documentation explains how to use Python’s built-in logging module to log exceptions and errors effectively.
Python pdb – Python Debugger Documentation
The official guide to pdb
, Python’s built-in debugger, which helps trace issues and inspect code execution in case of exceptions or runtime errors.
Leave a Reply