Introduction to GitHub Copilot and Its Significance in AI Software Development
Brief Introduction to AI in Software Development
Artificial Intelligence (AI) is changing the way we develop software by making the process faster and easier. GitHub Copilot AI-powered tool helps developers by taking care of repetitive tasks, suggesting smart code snippets, and improving overall code quality. These changes are especially noticeable in tools that help with code completion and generation. These tools use machine learning algorithms to assist developers as they write code, offering real-time support and suggestions.
Overview of GitHub Copilot and Its Significance
GitHub Copilot is a remarkable AI-powered tool created to help developers by offering real-time code suggestions and completions. Developed by GitHub in partnership with OpenAI, Copilot works smoothly with popular Integrated Development Environments (IDEs) like Visual Studio Code. This means it fits right into the tools developers are already using, providing helpful code recommendations as they type.
What makes GitHub Copilot special is how it boosts productivity. It not only speeds up the coding process but also helps ensure that the code is high-quality and consistent. This is crucial for developers who want to write better code faster. Copilot uses AI to understand the context of what you’re working on, making suggestions that are relevant and useful. This can be a real time-saver and can help reduce errors in your code.
For example, if you’re working on a Python project, Copilot can suggest entire functions or even detect common patterns in your code and offer completions that match those patterns. This makes the development process smoother and more efficient, allowing developers to focus on solving complex problems rather than getting bogged down with repetitive coding tasks.
Understanding GitHub Copilot
What is GitHub Copilot?
GitHub Copilot is a smart tool that helps you write code more efficiently. It uses artificial intelligence to understand what you’re working on and gives you suggestions to complete your code. This tool is integrated directly into your coding environment, meaning you don’t have to switch between different applications. GitHub Copilot is trained on a large amount of publicly available code, so it can offer helpful and intelligent code suggestions.
Features of GitHub Copilot
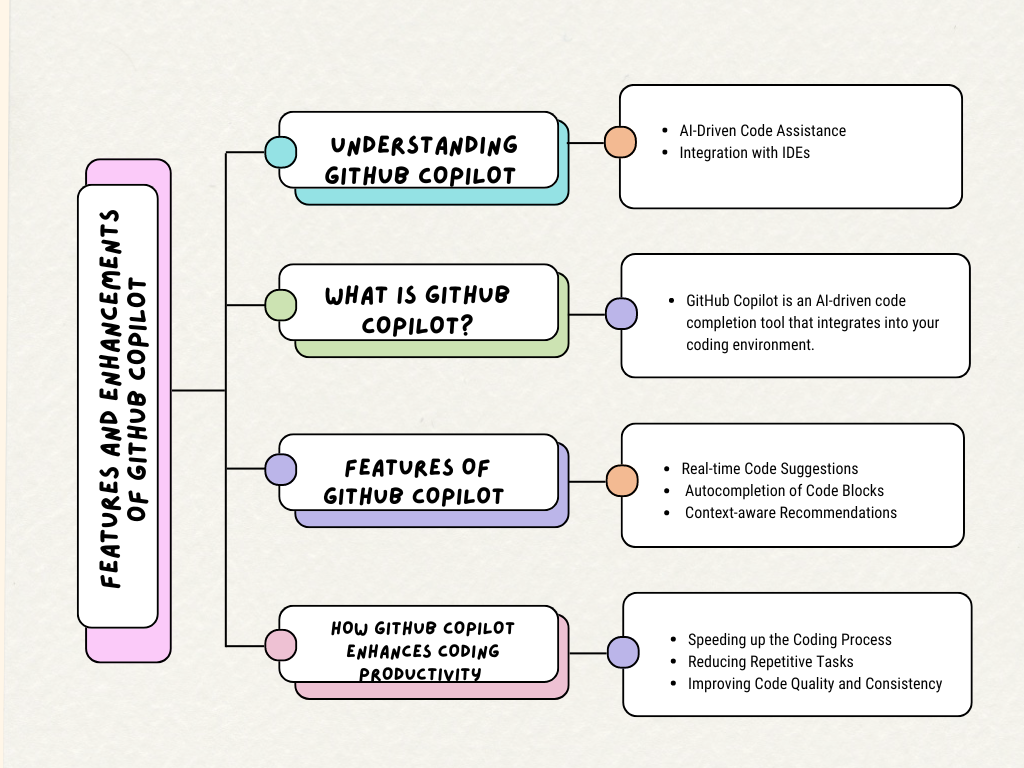
Real-time Code Suggestions
One of the best things about GitHub Copilot is that it gives you immediate suggestions while you type. Imagine you’re writing a function and you’re not sure how to complete it. Copilot can step in and suggest the next lines of code. This can help you write code faster and reduce the chances of making mistakes. By enhancing coding productivity with AI-powered assistance, Copilot makes your work easier and more efficient.
Autocompletion of Code Blocks
GitHub Copilot can also complete whole blocks of code for you. For example, if you start writing a loop or a function, Copilot can recognize what you’re trying to do and finish the entire block for you. This is incredibly useful because it saves time and ensures that the code follows best practices. Whether you’re writing a simple function or working on a more complex algorithm, this feature can significantly improve your coding efficiency.
Context-Aware Recommendations
Another powerful feature of GitHub Copilot is its ability to provide context-aware recommendations. It understands the project you’re working on and gives suggestions that fit the specific context of your code. For instance, if you’re working on a web application, it might suggest relevant code snippets that are commonly used in web development. This makes the development process smoother and helps you stay focused on your project goals.
How GitHub Copilot Enhances Coding Productivity with AI-Powered Assistance
Speeding Up the Coding Process
GitHub Copilot can significantly speed up the coding process by offering instant code suggestions. This means you spend less time typing and searching for the right code snippets, and more time focusing on complex tasks that require your expertise.
Example
Suppose you’re writing a Python function to calculate the factorial of a number. Instead of typing out the entire function, you start by typing def factorial(n):
, and Copilot can suggest the rest of the function for you:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
This immediate suggestion saves you time and ensures you have a correctly structured function.
Reducing Repetitive Tasks
One of the biggest benefits of using GitHub Copilot is its ability to automate repetitive coding tasks. This allows you to focus on more creative and complex aspects of your project.
Example
Imagine you’re creating a new class in JavaScript. Copilot can help by automatically filling in the constructor and common methods, like toString
and equals
, which you often need:
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
toString() {
return `${this.name} is ${this.age} years old`;
}
}
With Copilot handling these boilerplate tasks, you can focus on developing the unique features of your class.
Improving Code Quality and Consistency
Copilot’s suggestions are based on best practices and widely accepted coding standards. This helps maintain high code quality and consistency across your projects.
Example
When writing SQL queries, Copilot can suggest well-structured queries that follow best practices. For instance, if you’re writing a query to select employee names from a specific department, Copilot can provide a suggestion like this:
SELECT first_name, last_name
FROM employees
WHERE department = 'Sales'
ORDER BY last_name;
This not only saves time but also ensures that your queries are correctly formatted and efficient.
Personalized Experience
GitHub Copilot isn’t just a generic code completion tool. It learns from the context of your project and the coding patterns you frequently use, making its suggestions more customized and relevant to your specific needs.
Benefits of Using GitHub Copilot for AI-Powered Code Completion
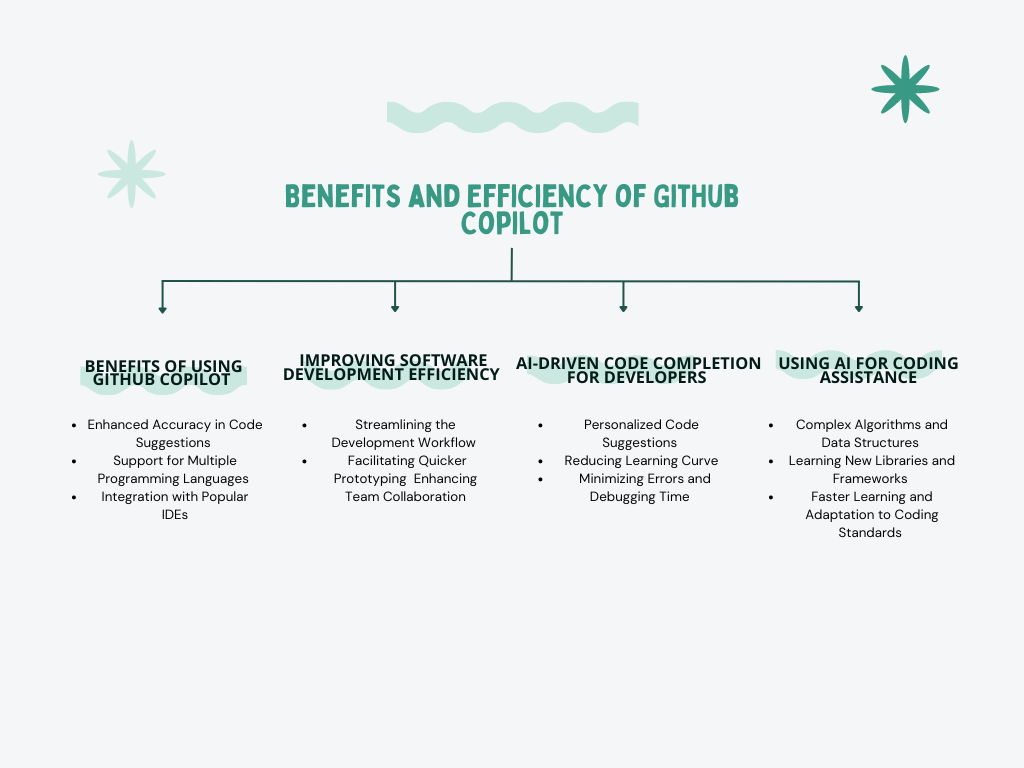
Enhanced Accuracy in Code Suggestions
GitHub Copilot provides accurate code suggestions, reducing the chances of errors and bugs in your code. This feature is incredibly helpful when writing complex code that requires precision.
Example
Let’s say you’re writing a regular expression in Python to validate email addresses. Copilot can suggest the correct pattern, saving you from the hassle of looking up the syntax:
import re
pattern = r'^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}$'
email = "example@example.com"
if re.match(pattern, email):
print("Valid email")
else:
print("Invalid email")
This accurate suggestion helps ensure that your regular expression works correctly, reducing potential bugs.
Support for Multiple Programming Languages
GitHub Copilot supports a wide range of programming languages, making it a versatile tool for developers working on different types of projects.
Example
Whether you’re working in Python, JavaScript, Java, or Ruby, Copilot can provide relevant code suggestions.
Integration with Popular IDEs
GitHub Copilot integrates well with popular Integrated Development Environments (IDEs) like Visual Studio Code. This integration enhances your overall development experience by providing instant code suggestions as you type.
Example
To start using Copilot in Visual Studio Code, you can install the Copilot extension from the extensions marketplace. Once installed, you’ll start receiving suggestions immediately as you code. Here’s how to do it:
- Open Visual Studio Code.
- Go to the Extensions view by clicking on the Extensions icon in the Activity Bar on the side of the window.
- Search for “GitHub Copilot” in the search bar.
- Click on the “Install” button next to the GitHub Copilot extension.
- After installation, follow the prompts to sign in to GitHub and enable Copilot.
Now, as you type, Copilot will provide helpful suggestions right in your editor, improving your coding efficiency and accuracy.
Improving Software Development Efficiency with GitHub Copilot AI Tools
Smootheing the Development Workflow
GitHub Copilot helps smoothens the development workflow by providing real-time code suggestions. This reduces the need for manual coding, allowing you to focus more on solving problems and building features.
Example
When building a REST API with Flask in Python, Copilot can suggest route handlers and boilerplate code, saving you time and effort:
from flask import Flask, jsonify, request
app = Flask(__name__)
@app.route('/api/data', methods=['GET'])
def get_data():
data = {"key": "value"}
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
This code snippet includes everything you need to start a simple API endpoint that returns JSON data. Copilot’s suggestions help you set up the basic structure quickly, allowing you to move on to more specific features of your API.
Facilitating Quicker Prototyping
By speeding up the coding process, GitHub Copilot enables quicker prototyping and iteration of ideas. This is especially useful when you need to test concepts and get feedback rapidly.
Example
When creating a new React component, Copilot can suggest the component structure and common methods:
import React, { Component } from 'react';
class MyComponent extends Component {
render() {
return (
<div>
<h1>Hello, world!</h1>
</div>
);
}
}
export default MyComponent;
This basic React component sets up a simple “Hello, world!” message. Copilot helps you quickly scaffold the component, so you can start experimenting with different ideas and features right away.
Enhancing Team Collaboration
Copilot’s consistent code suggestions help maintain coding standards within a team, making collaboration more efficient. By ensuring that everyone follows the same coding conventions, the codebase remains clean and easy to understand.
Example
When multiple team members are working on a project, Copilot can help maintain consistent code style and conventions across the codebase. For instance, if your team is working on a JavaScript project, Copilot can suggest standardized function signatures and variable naming conventions:
function fetchData(url) {
return fetch(url)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error fetching data:', error));
}
By providing consistent suggestions, Copilot helps ensure that all team members write code in a similar style, making it easier for everyone to read and understand each other’s work.
AI-Driven Code Completion with GitHub Copilot for Developers
Personalized Code Suggestions Based on Developer’s Style
GitHub Copilot learns from your coding style and provides personalized suggestions that match your preferences. This means that over time, it can adapt to the way you like to write your code, making the coding process even smoother and more efficient.
Example
If you prefer a particular way of writing loops or conditionals, Copilot will adapt its suggestions to match your style. For instance, if you often use for
loops in a specific format, Copilot will provide suggestions in that format:
# Preferred loop style
for i in range(10):
print(i)
Reducing the Learning Curve for New Developers
New developers can benefit greatly from Copilot’s suggestions, which help them learn best practices and coding standards quickly. By seeing common patterns and well-structured code, they can improve their skills faster.
Example
A new developer writing their first Django view can get immediate suggestions for common patterns, helping them understand how to structure their code correctly:
from django.shortcuts import render
from .models import Item
def item_list(request):
items = Item.objects.all()
return render(request, 'items/item_list.html', {'items': items})
This example shows how to create a simple view in Django that retrieves items from the database and renders them in a template. Copilot’s suggestions guide the new developer through the process, making it easier for them to learn and follow best practices.
Minimizing Errors and Debugging Time
By providing accurate and context-aware suggestions, Copilot helps reduce coding errors and the time spent on debugging. This is particularly useful when writing complex or error-prone code.
Example
When writing multi-threading code in Java, which can be prone to synchronization issues, Copilot can suggest correct patterns to avoid common pitfalls:
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
In this example, Copilot suggests using the synchronized
keyword to ensure that the increment
and getCount
methods are thread-safe. This helps prevent common concurrency issues and reduces the time spent debugging such problems.
Leveraging AI for Coding Assistance with GitHub Copilot
Using Copilot for Complex Algorithms and Data Structures
GitHub Copilot is great at assisting with writing complex algorithms and data structures by providing relevant code snippets and suggestions. This can save a lot of time and help you get your code right the first time.
Example: Writing a Binary Search Tree in JavaScript
When implementing a binary search tree, Copilot can suggest the structure and methods needed to create and manipulate the tree:
class Node {
constructor(data) {
this.data = data;
this.left = null;
this.right = null;
}
}
class BinarySearchTree {
constructor() {
this.root = null;
}
insert(data) {
const newNode = new Node(data);
if (this.root === null) {
this.root = newNode;
} else {
this.insertNode(this.root, newNode);
}
}
insertNode(node, newNode) {
if (newNode.data < node.data) {
if (node.left === null) {
node.left = newNode;
} else {
this.insertNode(node.left, newNode);
}
} else {
if (node.right === null) {
node.right = newNode;
} else {
this.insertNode(node.right, newNode);
}
}
}
}
This code provides the basic functionality for inserting nodes into a binary search tree, with Copilot helping you through the process.
Assistance in Learning and Implementing New Libraries and Frameworks
Copilot is also helpful when learning and implementing new libraries and frameworks by providing examples and usage patterns. This can accelerate your learning curve and allow you to implement new technologies faster.
Example: Using TensorFlow to Build a Simple Neural Network
When using TensorFlow for the first time, Copilot can suggest how to build and compile a simple neural network model:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
model = Sequential([
Dense(128, activation='relu', input_shape=(784,)),
Dense(64, activation='relu'),
Dense(10, activation='softmax')
])
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
This example shows a basic neural network model with layers and an activation function, demonstrating how Copilot can guide you through setting up and compiling the model.
Enabling Faster Learning and Adaptation to New Coding Standards
With Copilot’s help, developers can quickly adapt to new coding standards and practices, enhancing their coding efficiency and ensuring they follow best practices.
Example: Transitioning to TypeScript in a React Project
When switching to TypeScript in a React project, Copilot can suggest type annotations and interfaces, making the transition smoother:
interface Props {
name: string;
age: number;
}
const MyComponent: React.FC<Props> = ({ name, age }) => {
return (
<div>
<p>{name} is {age} years old.</p>
</div>
);
}
This example shows how Copilot can help you define interfaces and use them in your components, ensuring your code is type-safe and follows TypeScript standards.
Must Read
- How to Design and Implement a Multi-Agent System: A Step-by-Step Guide
- The Ultimate Guide to Best AI Agent Frameworks for 2025
- Data Science: Top 10 Mathematical Definitions
- How AI Agents and Agentic AI Are Transforming Tech
- Top Chunking Strategies to Boost Your RAG System Performance
Comparing GitHub Copilot with Competitor Tools
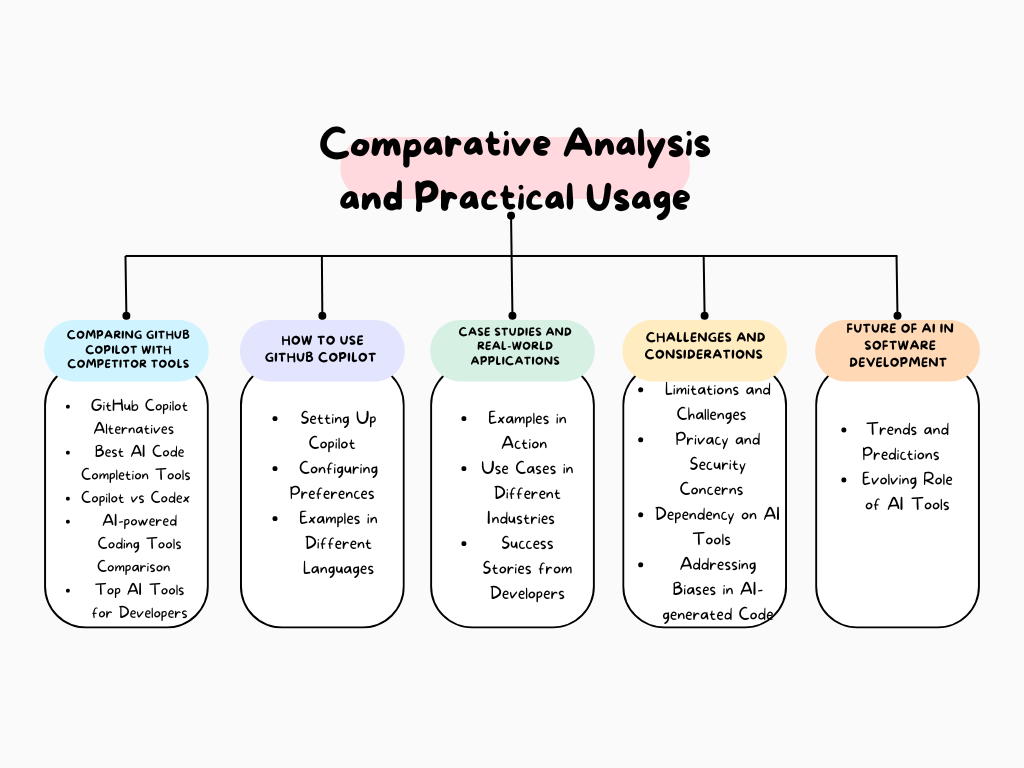
GitHub Copilot Alternatives and Competitors
While GitHub Copilot is a popular AI-powered code completion tool, there are several other alternatives worth considering, such as Kite and TabNine. These tools also offer intelligent code suggestions and can be valuable assets for developers.
Best AI Code Completion Tools
GitHub Copilot is known for its high accuracy and strong integration capabilities with popular Integrated Development Environments (IDEs). Its ability to provide relevant and context-aware code suggestions makes it one of the best AI code completion tools available.
Copilot vs Codex
GitHub Copilot is built using OpenAI’s Codex. While Copilot is designed for integration with coding environments like Visual Studio Code, Codex can be used for a wider range of applications. Both tools have unique strengths and can complement each other well in different scenarios.
AI-Powered Coding Tools Comparison
Comparing various AI-powered coding tools helps developers understand the strengths and weaknesses of each, enabling them to choose the best tool for their specific needs. Let’s look at some key features and examples to illustrate these points.
Example: Using GitHub Copilot, Kite, and TabNine
GitHub Copilot Example
Here’s how GitHub Copilot assists in writing a function to check if a number is prime in Python:
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(n ** 0.5) + 1):
if n % i == 0:
return False
return True
Copilot provides the complete function based on a simple prompt, helping you write accurate code quickly.
Kite Example
Kite also offers intelligent code suggestions. Here’s how it helps in writing a similar function:
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(n ** 0.5) + 1):
if n % i == 0:
return False
return True
Kite’s suggestions are similar, focusing on helping you write the function efficiently.
TabNine Example
TabNine uses deep learning models to predict and suggest code completions. Here’s how it assists in the same task:
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(n ** 0.5) + 1):
if n % i == 0:
return False
return True
TabNine provides quick and accurate completions, streamlining the coding process.
Top AI Tools for Developers
Among the top AI tools for developers, GitHub Copilot stands out due to its strong features, wide support for different programming languages, and smooth integration with popular IDEs. Whether you’re a beginner or an experienced developer, Copilot can significantly enhance your coding productivity.
How to Use GitHub Copilot
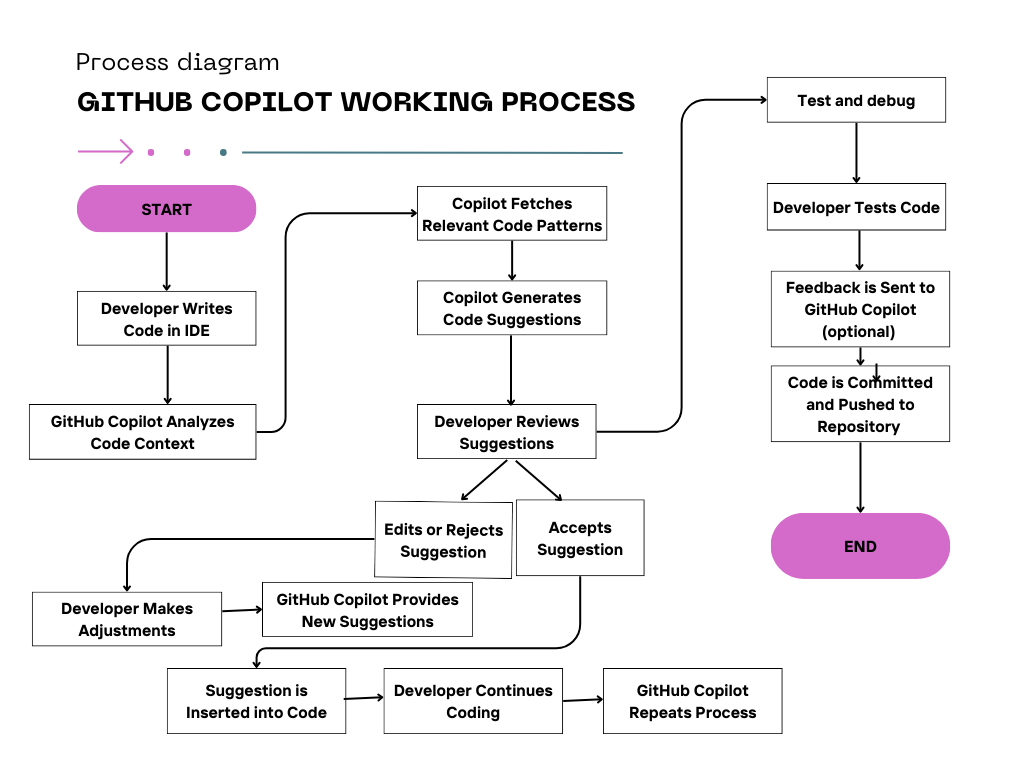
Step-by-Step GitHub Copilot Tutorial
Setting Up Copilot in Your IDE
To get started with GitHub Copilot, you’ll need to install the Copilot extension in your Integrated Development Environment (IDE). Here’s how you can set it up in Visual Studio Code (VS Code):
- Install the Extension:
- Open Visual Studio Code.
- Go to the Extensions Marketplace by clicking on the Extensions icon in the sidebar or pressing
Ctrl+Shift+X
. - In the search bar, type “GitHub Copilot.”
- Click on the “Install” button next to the GitHub Copilot extension.
- Sign In to GitHub:
- After installation, you’ll need to sign in with your GitHub account.
- A prompt will appear asking you to log in. Follow the instructions to authorize the extension with your GitHub credentials.
Configuring Preferences and Settings
Once GitHub Copilot is installed, you can customize its settings to fit your coding style and preferences:
- Access Settings:
- Go to the settings in Visual Studio Code by clicking on the gear icon in the lower-left corner and selecting “Settings” or pressing
Ctrl+,
.
- Go to the settings in Visual Studio Code by clicking on the gear icon in the lower-left corner and selecting “Settings” or pressing
- Adjust Copilot Settings:
- In the Settings menu, navigate to Extensions > GitHub Copilot.
- Here, you can configure various options such as:
- Suggestion Delay: Adjust how quickly Copilot provides suggestions.
- Suggestion Visibility: Set how often suggestions appear and how they are displayed.
Examples of Using Copilot in Different Programming Languages
GitHub Copilot is versatile and works with multiple programming languages. Here’s how it helps with a few examples:
Example: Python
- Create a New Python File:
- Open a new file and save it with a
.py
extension.
- Open a new file and save it with a
- Start Typing a Function Definition:
- Type
def
followed by the function name. For instance
- Type
def greet(name):
- Copilot will automatically suggest a complete function definition based on common patterns.
Example suggestion:
def greet(name):
return f"Hello, {name}!"
- This helps you quickly write functions without having to remember all the syntax.
Example: JavaScript
- Create a New JavaScript File:
- Open a new file and save it with a
.js
extension.
- Open a new file and save it with a
- Start Typing a Function Definition:
- Type
function
followed by the function name. For example
- Type
function calculateArea(radius) {
- Copilot will provide suggestions for completing the function.
Example suggestion:
function calculateArea(radius) {
return Math.PI * radius * radius;
}
- This can speed up the coding process by providing commonly used code patterns.
Example: TypeScript
- Create a New TypeScript File:
- Open a new file and save it with a
.ts
extension.
- Open a new file and save it with a
- Start Typing a Function Definition:
- Type
function
followed by the function name. For instance:
- Type
function addNumbers(a: number, b: number): number {
- Copilot will suggest the function body based on TypeScript best practices.
Example suggestion:
function addNumbers(a: number, b: number): number {
return a + b;
}
This helps ensure your TypeScript code adheres to type safety and best practices.
Case Studies and Real-World Applications
Real-World Examples of GitHub Copilot in Action
GitHub Copilot has proven to be a powerful tool across various industries, enhancing productivity and fostering innovation. Here are some real-world examples of how Copilot is used in different fields:
Use Cases in Different Industries
- Web Development:
- Streamlining Front-End Development: Developers working on web applications often use Copilot to generate HTML, CSS, and JavaScript code quickly. For example, when building a user login form, Copilot can suggest complete code snippets for form validation and styling.Example:
<form id="loginForm">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
<button type="submit">Login</button>
</form>
With Copilot’s suggestions, developers can avoid repetitive tasks and focus on designing user-friendly interfaces.
2. Data Science:
- Accelerating Data Analysis: Data scientists use Copilot to quickly write data processing and analysis scripts in Python. For instance, when performing exploratory data analysis, Copilot can suggest code for loading datasets and generating summary statistics.
Example
import pandas as pd
# Load the dataset
df = pd.read_csv('data.csv')
# Display basic statistics
print(df.describe())
This allows data scientists to spend more time interpreting results rather than writing boilerplate code.
3. Machine Learning:
- Speeding Up Model Training: In machine learning projects, Copilot helps by suggesting code for model training and evaluation. For example, when setting up a neural network with TensorFlow, Copilot can offer code for defining and compiling models.
Example
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
# Define the model
model = Sequential([
Dense(128, activation='relu', input_shape=(784,)),
Dense(64, activation='relu'),
Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
This helps machine learning engineers focus on tuning hyperparameters and improving model performance.
Challenges and Considerations
While GitHub Copilot offers numerous advantages for developers, it’s important to be aware of certain limitations and challenges associated with using this AI-powered tool. Understanding these challenges can help you use Copilot more effectively and responsibly.
Privacy and Security Concerns
When using GitHub Copilot, privacy and security are important considerations. Here’s a closer look at potential issues:
- Exposure of Sensitive Code: Copilot generates code based on a broad dataset of public code. This means there’s a possibility that sensitive or proprietary code could be suggested, especially if similar code exists in the training data.
Example: If you are working on a private project that includes custom encryption algorithms, Copilot might inadvertently suggest similar code snippets from public repositories, raising concerns about intellectual property.
- Data Privacy: Ensure that you are not inadvertently sharing confidential information through Copilot. Avoid entering sensitive data directly into Copilot, as there is a risk of the tool learning from and potentially exposing this information.
Best Practice: Use Copilot for general coding tasks but review and sanitize any code suggestions carefully before integrating them into your projects.
Dependency on AI Tools
Over-reliance on GitHub Copilot can pose some challenges:
- Reduced Skill Development: Relying heavily on Copilot for coding suggestions might impede the development of your problem-solving skills and coding proficiency. Developers might become too dependent on Copilot, leading to a decline in their ability to write code independently.
Example: If you always use Copilot to generate code for algorithms, you might miss out on learning how to design and implement these algorithms yourself, which can be crucial for understanding complex programming concepts.
- Code Understanding: Developers may not fully understand the code suggested by Copilot, which can lead to issues when troubleshooting or modifying code. It’s essential to review and comprehend the code Copilot provides.
Example: If Copilot suggests a function for handling user authentication, make sure to understand how it works and why it’s structured that way, rather than just copying and pasting it into your project.
Addressing Potential Biases in AI-Generated Code
AI tools, including Copilot, can sometimes produce biased code due to biases in the training data:
- Bias in Suggestions: AI models like Copilot are trained on large datasets that may contain biases. This can result in suggestions that reflect those biases, potentially affecting fairness and accuracy in the code generated.
Example: When generating code for user interface elements, Copilot might suggest designs that are not inclusive or accessible, which could affect the usability of your application for diverse users.
- Ensuring Fairness: To address potential biases, carefully review the code Copilot generates to ensure it meets ethical and fairness standards. Consider testing and validating code in various scenarios to ensure it performs well across different user groups.
Best Practice: Supplement Copilot’s suggestions with your own knowledge and best practices to ensure that the code adheres to inclusive design principles and ethical standards.
Future of AI in Software Development
Trends and Predictions for AI in Coding
Artificial Intelligence (AI) is rapidly transforming the landscape of software development. As AI technology continues to advance, its role in coding and development is becoming increasingly significant. Here’s a look at some trends and predictions for the future of AI in software development:
The Evolving Role of AI Tools like GitHub Copilot in the Developer Community
Enhanced Coding Productivity:
AI-Driven Assistance: Tools like GitHub Copilot are expected to become even more advanced, offering more precise and context-aware code suggestions. This will help developers write code faster and more efficiently, allowing them to focus on solving complex problems rather than repetitive tasks.
Example: Future versions of Copilot might include features that better understand project-specific contexts, providing suggestions tailored to the particular needs of your project.
Greater Integration with Development Environments:
IDE Integration: AI tools are likely to integrate more deeply with Integrated Development Environments (IDEs) and other development tools. This means that AI could become a more integral part of the coding workflow, offering real-time support and enhancements directly within the development environment.
Example: Imagine an AI assistant within your IDE that not only suggests code but also helps with project management, debugging, and testing by analyzing your entire codebase.
Increased Focus on Collaborative Coding:
Team Collaboration: AI tools will increasingly support collaborative coding efforts by ensuring that team members adhere to consistent coding standards and practices. This can help improve code quality and streamline teamwork, especially in large projects with multiple contributors.
Example: Copilot could provide suggestions that are aligned with the team’s coding guidelines and automatically adjust recommendations based on collective coding practices.
Advancements in AI Learning and Adaptation:
Personalized Code Recommendations: As AI tools evolve, they will become better at learning from individual developers’ coding styles and preferences. This personalization will make code suggestions more relevant and helpful, enhancing the overall coding experience.
Example: Future AI tools might analyze your previous code and suggest improvements based on your coding habits, making it easier to maintain consistency and quality.
Ethical and Bias Considerations:
Addressing Bias: The industry will increasingly focus on ensuring that AI-generated code is fair and unbiased. Developers and tool creators will work together to identify and mitigate biases in AI suggestions to promote more inclusive and accurate coding practices.
Example: AI tools could include features that highlight potential biases in code or suggest alternative approaches to ensure fairness and accessibility.
Expansion into New Areas of Development:
Broader Application: AI’s role in software development will expand into new areas, such as automated testing, code refactoring, and even software design. This will provide developers with a comprehensive suite of tools to enhance every stage of the software development lifecycle.
Example: AI might assist in generating test cases based on your code, automatically refactor code to improve performance, or suggest design patterns that align with best practices.
Conclusion
The future of AI in software development looks promising, with AI tools like GitHub Copilot set to play an increasingly important role in enhancing productivity and innovation. As AI technology continues to advance, we can expect more personalized, integrated, and collaborative tools that will transform how we write, test, and manage code. Keeping an eye on these trends and adapting to new developments will be key for developers looking to stay ahead in this evolving landscape.
External Resources
GitHub Copilot Official Documentation
- GitHub Copilot Documentation
The official GitHub Copilot documentation provides a comprehensive guide on how to set up and use Copilot, along with troubleshooting tips and FAQs.
GitHub Copilot Blog Posts
- Introducing GitHub Copilot: Your AI Pair Programmer
An announcement from GitHub about the launch of Copilot, its features, and how it aims to assist developers.
Kite: An AI Code Completion Tool
- Kite Official Website
Learn more about Kite, a popular alternative to GitHub Copilot, offering AI-powered code completions and suggestions.
TabNine: AI Code Completion Tool
- TabNine Official Website
Explore TabNine, another AI code completion tool, and compare its features with GitHub Copilot.
OpenAI Codex
- OpenAI Codex Overview
A detailed overview of OpenAI’s Codex, the underlying model that powers GitHub Copilot, including its capabilities and research insights.
Frequently Asked Questions
1. What is GitHub Copilot?
GitHub Copilot is an AI-powered tool developed by GitHub and OpenAI. It helps developers by providing real-time code suggestions and completions as you type, making coding faster and more efficient.
2. How does GitHub Copilot enhance coding productivity?
GitHub Copilot enhances coding productivity by offering instant code suggestions, completing code blocks, and providing context-aware recommendations. This speeds up coding, reduces repetitive tasks, and helps maintain consistent code quality.
3. What are some key features of GitHub Copilot?
Key features of GitHub Copilot include real-time code suggestions, autocompletion of code blocks, and context-aware recommendations based on your current coding context. It supports multiple programming languages and integrates with popular IDEs.
4. How can I set up GitHub Copilot in my IDE?
To set up GitHub Copilot, install the GitHub Copilot extension in your IDE, such as Visual Studio Code. Sign in with your GitHub account and configure your preferences in the IDE settings. Once installed, Copilot will start providing code suggestions as you type.
5. Are there any alternatives to GitHub Copilot?
Yes, there are alternatives to GitHub Copilot, such as Kite and TabNine. These tools also offer AI-powered code completion and suggestions, each with its own unique features and capabilities.