In today’s digital world, customer service has moved beyond phone calls and emails. More and more businesses are using chatbots to give customers instant answers and personalized interactions. Chatbots are like smart helpers that understand how people talk and can have real conversations. They make customer support easier and make customers happier by quickly solving their problems. This guide will show you how to create these helpful Customer Service Chatbots, step by step.
Introduction to Customer Service Chatbots
Customer service chatbots are advanced computer programs designed to talk like humans. They help customers by understanding what they need and giving answers quickly using advanced technologies such as Natural Language Processing (NLP) and machine learning. These chatbots are crucial in modern customer service strategies, managing common questions and directing complex issues to human agents when needed.

Benefits of Using Customer Service Chatbots
Implementing customer service chatbots offers many benefits for businesses:

- Always Available: Chatbots are there 24/7 to help customers, even outside of regular hours.
- Instant Answers: Customers get quick responses to their questions, making them happier with faster service.
- Handle More Customers: Chatbots can talk to many people at once, so they’re great for growing businesses without needing more staff.
- Consistent Help: Chatbots always give the same answers based on rules and AI, which means fewer mistakes and better service.
- Learn from Customers: Chatbots collect information and feedback from customers, so businesses can use that to make products and services even better.
Getting Started: Setting Up Your Development Environment
To start making your customer service chatbot, you’ll need a setup where you can use the right tools and software. Here’s how to get started:
- Get Rasa and Flask: Rasa helps build AI chatbots, and Flask is good for making the backend of websites.
- Start Your Rasa Project: Use “rasa init” to set up the first parts of your chatbot project.
Building Blocks of Customer Service Chatbots
Natural Language Processing (NLP)
NLP is what makes chatbots understand how people talk. It includes:
- Tokenization: Breaking sentences into words or parts.
- Finding Important Details: Figuring out specific things like product names or dates.
- Understanding What Someone Wants: Figuring out why someone is messaging, like saying hi or asking for help.
Dialog Management
Dialog management guides how the chatbot talks with users. This includes:
- Keeping Track of What’s Talked About: Remembering what’s been said so the chat stays on topic.
- Getting the Information Needed: Asking for details to understand what the user wants, like information about a product.
Integration with Backend Services
Connecting with backend services allows chatbots get information from databases or other apps. Here’s how:
Integrating with backend services allows chatbots to get information from databases or other apps. Here’s how:
- Implementing Custom Actions: Writing code to perform specific tasks like finding product details or handling payments.
- Webhooks: Establishing communication between the chatbot and external services via HTTP requests.
User Interface Design
User interface (UI) design aims to make using the chatbot easy and smooth for users. This involves:
- Web Interface: Creating a simple place for users to type messages and see replies.
- Message Formatting: Styling messages which helps to differentiate between user inputs and bot responses.
- Accessibility: Making sure the chatbot works well on all devices and screen sizes.

Step-by-Step Guide to Building a Customer Service Chatbot
Defining Intents and Entities
version: "2.0"
nlu:
- intent: greet
examples: |
- hi
- hello
- hey
- intent: goodbye
examples: |
- bye
- goodbye
- intent: ask_product_info
examples: |
- Can you tell me about [product A](product)?
- I need details on [product B](product)
Explanation
Understanding Chatbot Configuration
In the chatbot configuration:
- Version: This specifies the format version of the configuration file, which in this case is version 2.0.
- NLU Section: Here, you define how the chatbot understands what users say.
- Intents: These represent the different reasons or purposes behind what users say. For example, “greet” is used when a user says something like “hi” or “hello”.
- Examples: These are specific sentences or phrases that show what users might say for each intent. For instance, examples for “greet” could be “hi”, “hello”, or “hey”.
- Entities: These are specific details within the user’s message that the chatbot needs to pay attention to. For example, in “ask_product_info”, the entity could be a product name like “[product A]”. The chatbot should recognize and extract this information to provide relevant answers.
This setup helps the chatbot understand the different ways users communicate and what they’re asking for, making interactions smoother and more useful.
Designing Responses and Stories
Create responses for various intents and design conversation flows (stories) to guide how interactions will proceed. For example:
version: "2.0"
intents:
- greet
- goodbye
- ask_product_info
responses:
utter_greet:
- text: "Hello! How can I help you today?"
utter_goodbye:
- text: "Goodbye! Have a nice day."
utter_ask_product:
- text: "Which product do you want to know about?"
utter_product_info:
- text: "Sure, here is the information about {product}."
stories:
- story: greet path
steps:
- intent: greet
- action: utter_greet
- story: goodbye path
steps:
- intent: goodbye
- action: utter_goodbye
- story: product info path
steps:
- intent: ask_product_info
- action: utter_ask_product
- slot_was_set:
- product: "product A"
- action: action_product_info
Understanding Chatbot Interaction
In the chatbot’s setup:
- Story: product info path: This illustrates a more detailed interaction where a user asks about a product.
- Steps: These outline the sequence of events in this interaction path.
- Intent: ask_product_info: Represents the user’s request for details about a product.
- Action: utter_ask_product: Guides the chatbot to ask the user which specific product they want information on.
- Slot_was_set: Shows that a memory slot called “product” is now set to “product A” based on what the user said.
- Action: action_product_info: This action typically triggers a custom process in the chatbot’s backend. It’s designed to fetch and provide detailed information about the product the user asked about.
In summary, this setup in the configuration file helps the chatbot understand specific things users want to do. It allows the chatbot to respond with set messages for basic greetings and farewells. It also sets paths for more complex interactions, like when users want details about products. This structure helps the chatbot handle conversations smoothly and give helpful answers based on what users say.
Must Read
- How to Return Multiple Values from a Function in Python
- Parameter Passing Techniques in Python: A Complete Guide
- A Complete Guide to Python Function Arguments
- How to Create and Use Functions in Python
- Find All Divisors of a Number in Python
Implementing Custom Actions
Write custom actions in Python to perform specific tasks such as fetching product information from a backend service.
from typing import Any, Text, Dict, List
from rasa_sdk import Action, Tracker
from rasa_sdk.executor import CollectingDispatcher
import requests
class ActionProductInfo(Action):
def name(self) -> Text:
return "action_product_info"
def run(self, dispatcher: CollectingDispatcher,
tracker: Tracker,
domain: Dict[Text, Any]) -> List[Dict[Text, Any]]:
product = tracker.get_slot('product')
response = requests.get(f"http://localhost:5000/product/{product}")
product_info = response.json().get("info", "I'm not sure about that product.")
dispatcher.utter_message(text=product_info)
return []
Understanding Custom Action in a Rasa Chatbot
Imports
- Imports: These lines bring in necessary tools for Python to handle different kinds of information.
- from rasa_sdk import Action, Tracker: This imports tools from Rasa that help the chatbot perform actions and keep track of conversations.
- from rasa_sdk.executor import CollectingDispatcher: This imports a tool from Rasa that helps the chatbot send messages back to users.
- import requests: This brings in a tool that lets Python ask other programs or websites for information over the internet.
ActionProductInfo Class
- class ActionProductInfo(Action): This sets up a special action in the chatbot called ActionProductInfo. It uses special features from Rasa to do its job.
- def name(self) -> Text: This tells the chatbot what to call this action when it needs to use it. Here, it’s named “action_product_info”.
- def run(self, dispatcher: CollectingDispatcher, tracker: Tracker, domain: Dict[Text, Any]) -> List[Dict[Text, Any]]: This part is where the action actually happens when the chatbot needs to use this action.

Functionality
- product = tracker.get_slot(‘product’): This gets the name of a product that the user asked about during the chat.
- response = requests.get(f”http://localhost:5000/product/{product}“): This asks another program, running at http://localhost:5000, for details about the product the user wants to know about.
- product_info = response.json().get(“info”, “I’m not sure about that product.”): This reads the answer that the other program gives back. If the program doesn’t know about the product, it’ll say “I’m not sure about that product.”
- dispatcher.utter_message(text=product_info): This tells the chatbot to tell the user what it found out about the product.
- return []: This tells the chatbot that it finished doing what it needed to do.
Usage
This special action (ActionProductInfo) helps a Rasa chatbot when someone asks about a specific product. When the chatbot sees that someone wants to know about a product, it uses this action. The action then goes out and asks another program for information about the product. After it gets that information, it tells the user what it found out.
In short, this special action shows how a Rasa chatbot can use information from other programs to give answers to users’ questions about products.
Integrating Backend Services
Set up a Flask backend to handle requests and provide data to the chatbot.
from flask import Flask, jsonify
app = Flask(__name__)
products = {
"product A": "Product A is a great choice for...",
"product B": "Product B has the following features..."
}
@app.route('/product/<product_name>', methods=['GET'])
def get_product_info(product_name):
info = products.get(product_name, "I'm not sure about that product.")
return jsonify({"info": info})
if __name__ == '__main__':
app.run(debug=True)
Understanding Flask Backend for Product Information
Imports
- Imports: These lines bring in tools needed to build a web application using Flask.
Flask
sets up the web server, whilejsonify
helps convert data into a format that can be easily sent over the internet.
Flask Application Setup
- app = Flask(name): This line creates a web application using Flask. Think of it like setting up a place where the server can run and respond to requests.
__name__
represents the name of the current script.
Products Data
- products: This is a dictionary that holds information about different products. It acts like a pretend database in this example, storing product names and their descriptions.
Route Definition
- @app.route(‘/product/<product_name>’, methods=[‘GET’]): This line defines a special spot on the web server (a route) where it listens for requests. When someone asks for
/product/<product_name>
, it’s set up to handle that request.
get_product_info Function
- def get_product_info(product_name): This function runs when someone asks for information about a specific product (
<product_name>
). - info = products.get(product_name, “I’m not sure about that product.”): Inside the function, it looks up the product name in the
products
dictionary. If it finds the product, it gives back the description. If not, it says it doesn’t know about that product. - return jsonify({“info”: info}): This part converts the product information into a format called JSON, which is easy for computers to send and read. It then sends that information back as a response to the person who asked for it.
Main Execution Block
- if name == ‘main’: This line checks if this script is being run directly by itself (not as part of another program).
- app.run(debug=True): If it’s being run directly, this starts up the web server using Flask. It turns on a special feature called debugging, which helps find and fix problems while the server is running. The server starts up at
http://localhost:5000/
, which means it’s ready to respond to requests.
Usage
This Flask application acts as a simple server that stores and provides information about products. When someone asks for details about a specific product by visiting /product/<product_name>
, the server checks its records and sends back the product’s description in a structured format called JSON.
For example, in the context of a chatbot (like the Rasa example earlier), the chatbot’s custom action (ActionProductInfo
) would use this Flask server to get live updates about products when users ask questions.
This setup shows how Flask can create a straightforward backend service that gives information to different applications, including chatbots, through the internet.
Creating a User Interface
Develop a simple HTML and JavaScript interface for users to interact with the chatbot.
<!DOCTYPE html>
<html>
<head>
<title>Customer Service Chatbot</title>
<style>
.chat-container {
width: 300px;
height: 400px;
border: 1px solid #ccc;
padding: 10px;
overflow-y: scroll;
}
.message {
padding: 5px;
margin: 5px 0;
}
.user-message {
text-align: right;
background-color: #d4edda;
}
.bot-message {
text-align: left;
background-color: #f8d7da;
}
</style>
</head>
<body>
<div class="chat-container" id="chat-container"></div>
<input type="text" id="user-input" placeholder="Type your message here...">
<button onclick="sendMessage()">Send</button>
<script>
async function sendMessage() {
const inputElement = document.getElementById('user-input');
const message = inputElement.value;
inputElement.value = '';
const chatContainer = document.getElementById('chat-container');
chatContainer.innerHTML += `<div class="message user-message">${message}</div>`;
chatContainer.scrollTop = chatContainer.scrollHeight;
const response = await fetch('http://localhost:5005/webhooks/rest/webhook', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ sender: 'user', message })
});
const data = await response.json();
const botMessage = data[0]?.text || "I'm not sure how to respond to that.";
chatContainer.innerHTML += `<div class="message bot-message">${botMessage}</div>`;
chatContainer.scrollTop = chatContainer.scrollHeight;
}
</script>
</body>
</html>
Testing and Iterating Your Chatbot
Before launching your chatbot, thoroughly test its functionality and responses. Utilize tools like Rasa’s interactive learning mode to refine dialog flows and improve accuracy based on real user interactions.

Testing Functionality
- Check how well the chatbot understands users: Test if the chatbot correctly recognizes what users mean when they greet, ask questions, or request information.
- Handle unexpected situations gracefully: Make sure the chatbot responds well when users say something unexpected or unclear, asking for more details or giving helpful responses.
- Verify data accuracy from backend services: If your chatbot uses information from other systems, ensure it fetches and shows the right data.
Testing Responses
- Evaluate clarity and relevance: See if the chatbot’s answers are easy to understand and directly related to what users asked.
- Consistency in tone: Check if the chatbot keeps a consistent style and tone in its replies, matching what users expect.
- Adapt to different situations: Test how the chatbot reacts in different scenarios and adjusts its responses based on what’s happening in the conversation.
Using Rasa’s Interactive Learning
- Correct mistakes in real-time: When testing interactively, fix any wrong answers the chatbot gives or add more examples so it understands better.
- Add new examples: Teach the chatbot new ways users might ask questions to improve its understanding.
- Refine how conversations flow: Adjust how the chatbot leads conversations based on what you see people actually do and say.
Iterating Based on Feedback
- Get feedback from testers and users: Listen to what people say about how the chatbot works and where it could be better.
- Make changes to improve: Use feedback to change how the chatbot decides what people mean, what it says back, and how it leads conversations.
- Keep making it better: Test and change the chatbot often to make sure it works well and is easy for people to use.
Getting Ready to Launch
- Do final checks: Make sure everything works right before you start using the chatbot for real.
- Write down what still needs work: Keep track of what the chatbot could do better so you can make it even better after it starts.
- Plan to watch and learn: Think about how you’ll check how well the chatbot does once it’s being used, so you can make it better later.
By testing and fixing your chatbot carefully with tools like Rasa’s interactive way of learning, you can make sure it does what people want, is right a lot, and gives people a good time. This way makes sure your chatbot does what people need and helps your business.
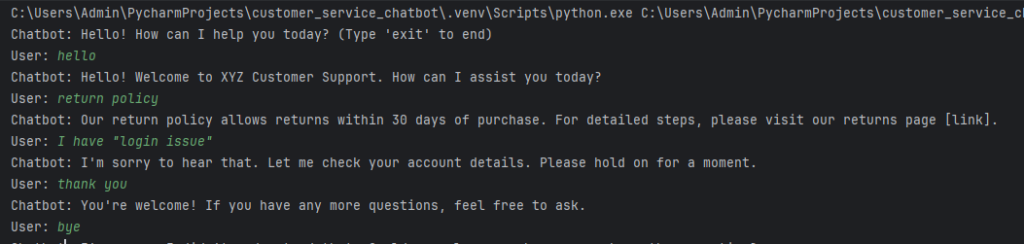
Deployment and Maintenance
Deployment and maintenance of a chatbot involve several key steps to ensure it runs smoothly and evolves over time:

Deployment
- Choose a Cloud Platform: Pick a place where your chatbot can live, like Amazon Web Services (AWS) or Google Cloud. These places can run programs like Python, which your chatbot uses.
- Get Ready to Deploy: Make sure everything your chatbot needs is packed up and ready to go. This means putting all the pieces together in a way that makes it easy to start up, like putting everything in a box (package) or a big container (Docker).
- Put It Out There: Once everything’s ready, send your chatbot to the cloud platform or server you picked. Set it up so it can handle a lot of people and keep everything safe. Make sure all the things it needs, like settings and permissions, are set up right.
- Watch What Happens: After your chatbot starts working, keep an eye on how it does. Look at how many people use it, how it’s working, and if there are any problems that come up.
Sure, here’s a simplified and humanized version of the deployment and maintenance steps for a chatbot:
Deploying and Maintaining Your Chatbot
Deployment:
- Choose a Cloud Platform: Pick a place where your chatbot can live, like Amazon Web Services (AWS) or Google Cloud. These places can run programs like Python, which your chatbot uses.
- Get Ready to Deploy: Make sure everything your chatbot needs is packed up and ready to go. This means putting all the pieces together in a way that makes it easy to start up, like putting everything in a box (package) or a big container (Docker).
- Put It Out There: Once everything’s ready, send your chatbot to the cloud platform or server you picked. Set it up so it can handle a lot of people and keep everything safe. Make sure all the things it needs, like settings and permissions, are set up right.
- Watch What Happens: After your chatbot starts working, keep an eye on how it does. Look at how many people use it, how it’s working, and if there are any problems that come up.
Maintenance
- Keep It Up to Date: Keep changing your chatbot to make it better. Add new things people want, fix anything that’s wrong, and make it work better all the time.
- Fix Things Fast: When people tell you something’s not right, fix it right away. Make sure your chatbot always works well and does what people need.
- Make It Faster: Look at how fast your chatbot works and how much it uses. Change it to make it work better based on how people use it and what they say.
- Keep It Safe: Make sure your chatbot is always safe. Change the things it uses to make sure no one can hurt it or the people who use it. Follow the best ways to keep it safe and make sure it does what it should.
- Plan for Problems: Make a plan to keep your chatbot safe from losing things. Have a plan to make it work again fast if something bad happens and it can’t work.
Listen to People and Make It Better
- Hear What People Say: Ask people what they think and what they want. Use what they say to make your chatbot better.
- Look at the Numbers: Use what you know about how people use your chatbot to make smart choices about what to do next.
- Keep Making It Better: Change your chatbot all the time to make it better. Make sure it does what people need and helps your business grow.
By sending your chatbot to a good place and changing it a lot, you can make sure it does a good job and keeps getting better. This helps your chatbot do what your business needs and make people happy in the digital world.
Best Practices for Creating Effective Customer Service Chatbots
- Understand Your Audience: Customize your chatbot’s language and tone to match your target audience.
- Provide Clear Instructions: Guide users on how to interact with the chatbot effectively.
- Offer Human Handoff: Make it easy to switch to a human agent for complex issues.
- Monitor Performance: Check chatbot metrics to find ways to improve and enhance user experience.
Case Studies: Successful Implementations of Customer Service Chatbots
In this section, we will look at real-life examples of businesses that have used chatbots to improve customer service and make their operations more efficient. These stories show how chatbots can help different industries by providing quick, personalized support and streamlining processes.
Example 1: E-Commerce
Company: XYZ Online Retailer
Challenge: XYZ Online Retailer was getting more and more customer questions about order statuses, product details, and return policies. This was causing long response times and overloading their support staff.
Solution: They set up a chatbot to answer common customer questions. This chatbot connected with their order management system to give real-time updates and product information.
Results
- Faster Responses: Customers got instant answers, which cut down their waiting time a lot.
- Better Efficiency: The chatbot handled 70% of inquiries, so human agents could focus on more complicated issues.
- Happier Customers: Customer satisfaction scores went up because of the faster and more accurate responses.
Example 2: Banking
Company: ABC Bank
Challenge: ABC Bank wanted to offer customer support 24/7 for inquiries about account balances, transaction histories, and branch locations.
Solution: They deployed a chatbot on their website and mobile app to answer frequently asked questions, provide account info, and guide customers to relevant services.
Results
- Always Available: Customers could get support any time, which made things more convenient for them.
- Saved Money: The chatbot reduced the need for more support staff, which cut costs.
- Better Engagement: The chatbot also gave personalized financial advice, which increased customer engagement.
Example 3: Healthcare
Company: HealthFirst Clinic
Challenge: HealthFirst Clinic wanted to make it easier to schedule appointments and give patients quick access to medical information.
Solution: They introduced a chatbot on their website and patient portal to help with booking appointments, checking symptoms, and answering questions about treatments.
Results
- Easier Access: Patients could book appointments and get information even outside regular office hours.
- Less Work for Staff: Administrative staff had fewer routine questions to handle, so they could focus more on in-person patient care.
- Better Patient Experience: The chatbot’s instant responses and easy access to information improved the overall experience for patients.
Example 4: Telecommunications
Company: GlobalTel
Challenge: GlobalTel had a high volume of support requests related to billing, service outages, and technical troubleshooting.
Solution: They implemented a chatbot to automate responses to common issues and provide troubleshooting guides.
Results:
- Quick Resolutions: The chatbot could solve simple problems right away, reducing the time customers spent waiting for support.
- Increased Efficiency: Automating routine inquiries made better use of human resources.
- Higher Customer Retention: Better support experiences helped keep customers loyal.
Summary
These case studies show how chatbots can be used in various industries to provide instant, personalized support, improve efficiency, and boost customer satisfaction. By implementing chatbots, businesses can enhance their operations and better meet customer needs.
Future Trends in Customer Service Chatbots
Stay updated on emerging technologies such as AI advancements, voice-enabled assistants, and omnichannel integration, which are shaping the future of customer service chatbots.
Conclusion
Building a customer service chatbot means using AI and NLP technologies to create a system that grows with your business, making customer interactions smoother and more efficient. This guide will help you start developing your own chatbot, improving how customers experience your services.
Having a chatbot doesn’t just make customers happier—it also lets your team spend more time on important jobs while still giving great support. This helps your business grow and keeps customers coming back in today’s digital world.
Source Code
Project Structure
customer_service_chatbot/
│
├── actions/
│ ├── bot.py
│ └── actions.py # Custom actions for handling intents
│
├── data/
│ ├── nlu.yml # Training data for NLU model
│ ├── responses.yml # Responses for different intents
│ └── stories.yml # Sample conversations
│
├── models/ # Trained Rasa models will be saved here
│
├── config.yml # Rasa configuration file
├── credentials.yml # Credentials for external integrations (optional)
├── domain.yml # Domain file defining intents, entities, responses, actions
├── endpoints.yml # Endpoint configuration for actions server
├── bot.py # Flask app to integrate Rasa with web
└── README.md # Project documentation
data/nlu.yml
version: "2.0"
nlu:
- intent: greet
examples: |
- Hello
- Hi
- Hey
- intent: goodbye
examples: |
- Goodbye
- Bye
- See you later
- intent: thanks
examples: |
- Thanks
- Thank you
- Thanks a lot
- intent: help
examples: |
- Help me
- Can you help?
- I need assistance
- intent: query_order_status
examples: |
- What is the status of my order?
- Can you check my order?
- intent: cancel_order
examples: |
- I want to cancel my order
- Cancel my order
- Stop my order
- intent: inform
examples: |
- My order number is [12345](order_id)
- I ordered [product X](product) yesterday
entities:
- order_id
- product
data/responses.yml
responses:
utter_greet:
- text: "Hello! How can I assist you today?"
utter_goodbye:
- text: "Goodbye! Have a great day."
utter_thanks:
- text: "You're welcome!"
utter_help:
- text: "Sure, I'm here to help. How can I assist you?"
utter_query_order_status:
- text: "Let me check the status of your order."
utter_cancel_order:
- text: "I will assist you with canceling your order."
utter_default:
- text: "I'm sorry, I didn't understand that. Could you please repeat?"
actions/actions.py
from typing import Any, Text, Dict, List
from rasa_sdk import Action, Tracker
from rasa_sdk.executor import CollectingDispatcher
class ActionQueryOrderStatus(Action):
def name(self) -> Text:
return "action_query_order_status"
def run(self, dispatcher: CollectingDispatcher,
tracker: Tracker,
domain: Dict[Text, Any]) -> List[Dict[Text, Any]]:
# Logic to query order status using tracker.latest_message['entities']
order_id = next((e['value'] for e in tracker.latest_message['entities'] if e['entity'] == 'order_id'), None)
if order_id:
dispatcher.utter_message(text=f"The status of your order {order_id} is processing.")
else:
dispatcher.utter_message(text="I couldn't find your order. Could you please provide the order number again?")
return []
domain.yml
intents:
- greet
- goodbye
- thanks
- help
- query_order_status
- cancel_order
- inform
responses:
utter_greet:
- text: "Hello! How can I assist you today?"
utter_goodbye:
- text: "Goodbye! Have a great day."
utter_thanks:
- text: "You're welcome!"
utter_help:
- text: "Sure, I'm here to help. How can I assist you?"
utter_query_order_status:
- text: "Let me check the status of your order."
utter_cancel_order:
- text: "I will assist you with canceling your order."
utter_default:
- text: "I'm sorry, I didn't understand that. Could you please repeat?"
actions:
- action_query_order_status
entities:
- order_id
- product
bot.py
from flask import Flask, request, jsonify
import requests
from rasa.core.agent import Agent
from rasa.core.utils import EndpointConfig
app = Flask(__name__)
# Load your Rasa model and agent
model_path = "./models"
endpoint = EndpointConfig("http://localhost:5005/webhook")
agent = Agent.load(model_path, action_endpoint=endpoint)
@app.route('/webhook', methods=['POST'])
def webhook():
data = request.get_json()
response = agent.handle_text(data.get('message'))
return jsonify(response)
if __name__ == '__main__':
app.run(debug=True)
config.yml
language: "en"
pipeline:
- name: "WhitespaceTokenizer"
- name: "RegexFeaturizer"
- name: "CRFEntityExtractor"
- name: "CountVectorsFeaturizer"
- name: "EmbeddingIntentClassifier"
Train and Run
Train the Rasa model using:
rasa train
Then, start the Rasa server:
rasa run --enable-api --cors "*"
Finally, run the Flask app
python bot.py
You can now interact with your customer service chatbot through HTTP requests to http://localhost:5000/webhook
. For a complete deployment, you would need to handle more intents, create appropriate actions, and integrate with backend systems to fetch real data like order statuses.
External Resources
- Rasa Documentation: Rasa is a tool you can use to create chatbots that can talk like humans. Their guides and examples show you how to build chatbots for customer service and other uses.
- Dialogflow Documentation: Dialogflow, part of Google Cloud, helps you make chatbots that can understand and respond to people. Their tools and guides are useful for creating chatbots for customer service.
- IBM Watson Assistant: IBM Watson Assistant lets you build and manage chatbots with artificial intelligence. They provide tutorials and guides to help you create chatbots that can help customers.
- Microsoft Bot Framework: Microsoft Bot Framework gives you tools and code to make chatbots for different places people use. Their guides cover everything from creating to putting chatbots into use for customer service.
These resources are great for learning how to create chatbots that can improve customer service and interact more like humans.
[…] AI-powered application that can interact with users through text or voice. They are widely used in customer service, personal assistance, and other applications to provide quick responses and automate […]