Introduction
Format Strings in Python: Let me show you something really useful in Python—string formatting.
When you’re working with Python, you’ll often need to print messages to the screen. Maybe you’re showing a user’s name, printing a calculation, or just making your program’s output look clean and clear.
To do all that properly, you need to understand how Python string formatting works—especially using the print()
function and the format()
method.
Now, don’t worry if this sounds technical. I’m going to walk you through it step by step. We’ll start with the basics and build up from there.
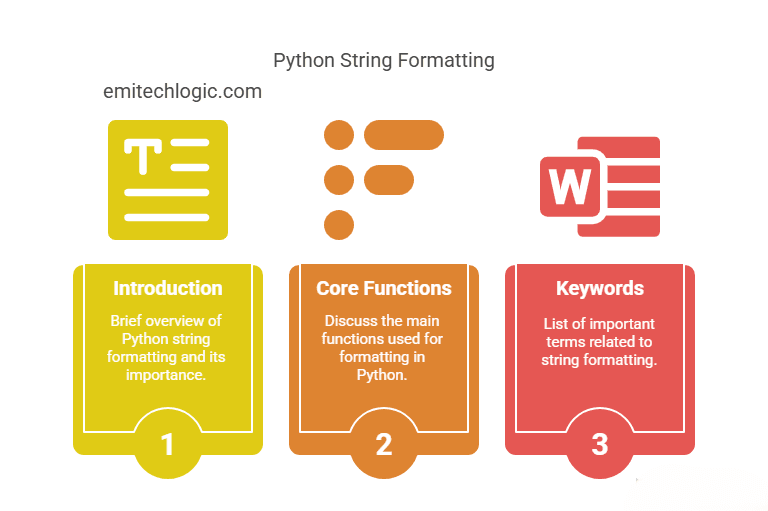
By the end of this guide, you’ll know:
- How to use
print()
in Python to show messages with variables. - How the
format()
method helps you insert values into strings. - To make your output look better by aligning text, rounding numbers, and adding spaces.
- And how to avoid common formatting mistakes that can confuse beginners.
This isn’t just a list of examples—it’s a hands-on way to learn Python string formatting, so you can understand why it works the way it does.
So open up your Python editor, and let’s start learning how to make your output look clean, professional, and easy to read.
What Is the print()
Function in Python?
The print()
function in Python is one of the very first things you’ll learn when writing code. It’s used to display messages or results in the console. Whenever you want your program to show something to the user, you use a print statement.
What’s the purpose of print()
?

The main purpose of the print()
function is to output information. This can be:
- A simple message like
"Hello, World!"
- The result of a calculation
- A combination of text and variables
- Or even formatted output that looks clean and easy to read
So any time you want your program to “speak,” print()
is how you do it.
Basic usage
Here’s the most basic way to use print()
:
print("Hello, World!")
When you run this, Python will display:
Hello, World!
This is a classic example. It’s simple, but it shows the core idea of the print statement: sending output to the screen.
Why is this important?
You’ll use print()
all the time—for testing, debugging, or just showing results. As you go further, you’ll also learn how to use print()
for formatted output, which means making the printed text look nice, organized, and clear.
And that’s exactly what we’ll start working on next—how to make your output more meaningful using formatting.
Understanding format()
: The Key to Python String Formatting
Now that you’re comfortable with the print()
function, let’s take it a step further. One of the best ways to make your output cleaner and more dynamic is by using the format()
function in Python.
This is where string formatting becomes really useful—especially when you want to include variables in your messages.
How format()
Works in Python
The format()
method lets you insert values directly into a string by using placeholders. A placeholder is just a pair of curly braces {}
inside your string. Wherever you put {}
, Python will fill it with a value you provide using .format()
.
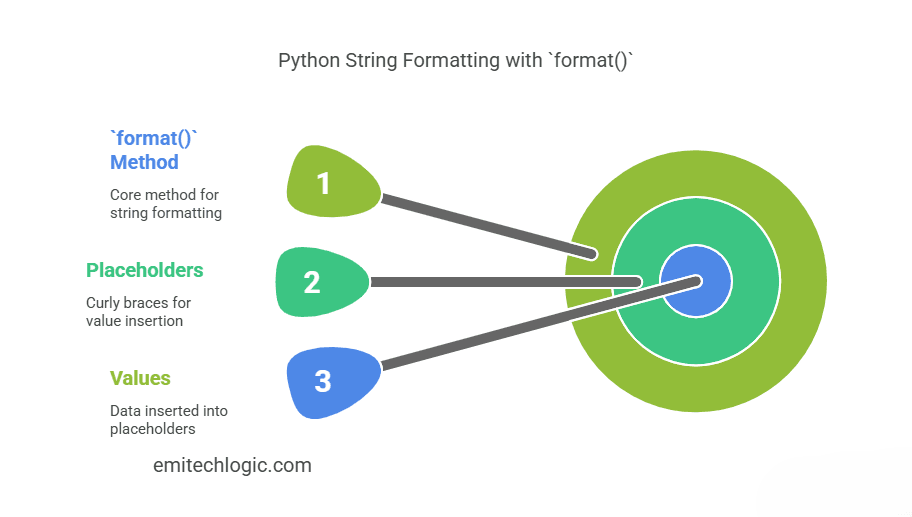
Let me show you a simple example:
name = "Alex"
print("Hello, {}".format(name))
Here’s what’s happening:
"Hello, {}"
is the string template, and{}
is the placeholder..format(name)
tells Python to insert the value ofname
into the{}
.- The output will be:
Hello, Alex
This technique is called string interpolation, because you’re inserting (or interpolating) values into a string.
Why this is so helpful
Using the Python format function makes it easy to:
- Combine text and variables in a clean way
- Keep your code organized
- Avoid confusing string concatenation with
+
It works with more than one value, too! We’ll explore that shortly.
Multiple Placeholders and Positional Arguments
When you’re formatting strings in Python, you’re not limited to just one value. You can insert multiple values into a single string using more than one placeholder.
Example: Multiple Placeholders
Here’s a simple example with two values:
print("Name: {}, Age: {}".format("Alice", 30))
What’s happening here?
{}
and{}
are placeholders for values..format("Alice", 30)
sends"Alice"
to the first{}
and30
to the second{}
.- The output will be:
Name: Alice, Age: 30
This is clean, readable, and way easier than trying to join strings manually.
Positional Arguments with Indexes
You can also control the order of the values by using index-based placeholders. Here’s how:
print("{1}, {0}".format("first", "second"))
Let’s break this down:
{1}
refers to the second item in.format()
, which is"second"
.{0}
refers to the first item,"first"
.
So the output will be:
second, first
This is helpful when you want to reuse values or arrange them differently in your output.
Using multiple placeholders and index-based formatting gives you more control over how your output looks. And don’t worry—we’ll soon explore named placeholders and more advanced tricks too.
Named Placeholders with format()
Instead of using {}
or index numbers like {0}
, you can use named placeholders inside your string. This means you label each placeholder with a name, and then pass values using keyword arguments inside the format()
function.
Here’s an example:
print("Name: {name}, Age: {age}".format(name="Bob", age=25))
Let’s break it down:
{name}
and{age}
are named placeholders.- Inside
.format()
, you pass values usingname="Bob"
andage=25
. - Python matches the names and fills in the values.
The output will be:
Name: Bob, Age: 25
Why this is helpful
- It’s much easier to read and understand, especially when you’re formatting long strings.
- You don’t have to remember which value goes where—you just use the name.
- You can reuse values by referencing the same name more than once in the string.
Example with reuse:
print("{name} is {age} years old. {name} loves Python.".format(name="Bob", age=25))
Output:
Bob is 25 years old. Bob loves Python.
Named placeholders are a big help when your output gets more detailed. Want to learn how to format numbers (like decimal places or padding)? We can jump into that next if you’re ready!
Must Read
- Global and Local Variables in Python: A Complete Guide
- How to Return Multiple Values from a Function in Python
- Parameter Passing Techniques in Python: A Complete Guide
- A Complete Guide to Python Function Arguments
- How to Create and Use Functions in Python
Using Format Specifiers for Precise Output
Sometimes, you don’t just want to print a value—you want to format it nicely. Maybe you want to round a number, control how many decimal places show, or align values for cleaner output.
That’s where format specifiers come in.
Formatting Numbers, Floats, and Decimals
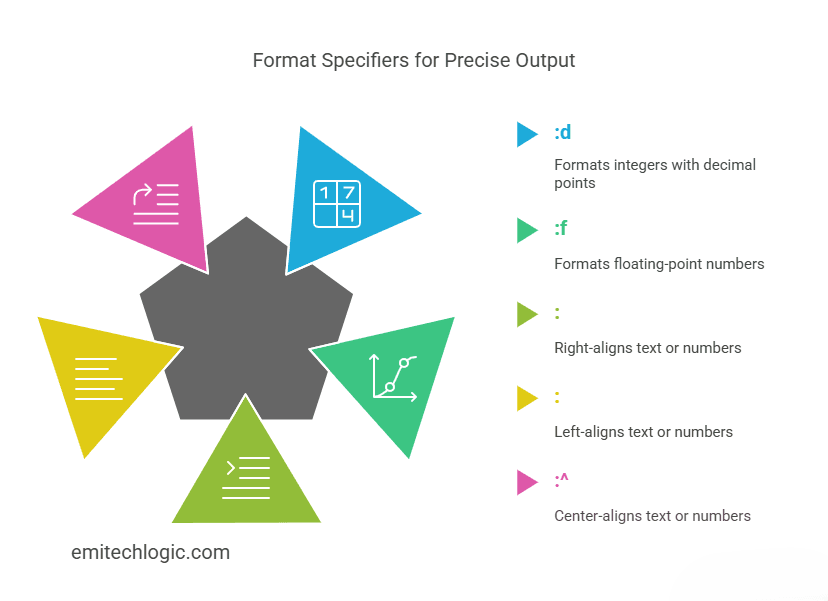
Let’s say you have a float value like Pi, and you want to show only 2 decimal places. Here’s how you do it:
value = 3.14159
print("Pi is approximately {:.2f}".format(value))
Explanation:
:.2f
is a format specifier.:
tells Python you’re starting a format instruction..2f
means “2 digits after the decimal point” as a float.
Output:
Pi is approximately 3.14
Common Format Specifiers
Here are a few you’ll use often:
Specifier | Meaning |
---|---|
:d | Format as an integer (whole number) |
:f | Format as a float |
:.2f | Float with 2 decimal places |
:> | Right-align the text |
:< | Left-align the text |
:^ | Center-align the text |
Example with alignment:
print("{:<10} | {:^10} | {:>10}".format("Left", "Center", "Right"))
Output:
Left | Center | Right
Each column is 10 characters wide:
<
makes it left-aligned,^
centers the text,>
makes it right-aligned.
This is super useful when you’re printing tables or reports and want everything to line up neatly.
Creating a Formatted Table with Variables and Calculations
Let’s say you’re building a small program to display product details: name, quantity, price, and total cost. We’ll use everything you’ve learned to format it neatly.
Example:
print("{:<10} {:>10} {:>10} {:>12}".format("Item", "Qty", "Price", "Total"))
print("-" * 44)
item1 = "Apple"
qty1 = 5
price1 = 0.99
total1 = qty1 * price1
item2 = "Banana"
qty2 = 12
price2 = 0.35
total2 = qty2 * price2
print("{:<10} {:>10} {:>10.2f} {:>12.2f}".format(item1, qty1, price1, total1))
print("{:<10} {:>10} {:>10.2f} {:>12.2f}".format(item2, qty2, price2, total2))
Output:
Item Qty Price Total
--------------------------------------------
Apple 5 0.99 4.95
Banana 12 0.35 4.20
Let’s break this down:
:<10
→ Left-align the item name in a 10-character space.:>10
→ Right-align quantity and price in 10 characters.:>10.2f
→ Right-align and format float with 2 decimal places.:>12.2f
→ Wider column for total, 2 decimal places."-" * 44
→ A simple line to separate the header and the data.
This gives you a clean layout that looks almost like a printed receipt.
Why this matters
- Readable output = better user experience.
- Great for console apps, reports, or debugging data.
- Makes your code feel polished—even if it’s just a practice project.
Introducing f-Strings: Cleaner Python String Formatting

Starting from Python 3.6, you can use f-strings (formatted string literals). Instead of calling .format()
, you just add an f
before the string and write your variables directly inside {}
.
Basic Example:
name = "Alice"
age = 30
print(f"Name: {name}, Age: {age}")
Output:
Name: Alice, Age: 30
No need for .format()
—just use the variables straight inside the curly braces. Much cleaner, right?
Formatting Numbers with f-Strings
You can use the same format specifiers with f-strings as you did with format()
.
Example: Float formatting
pi = 3.14159265
print(f"Pi rounded to 2 decimal places: {pi:.2f}")
Output:
Pi rounded to 2 decimal places: 3.14
Same result, but less typing.
Alignment with f-Strings
Let’s recreate part of the table example using f-strings:
item = "Orange"
qty = 3
price = 1.25
total = qty * price
print(f"{'Item':<10} {'Qty':>5} {'Price':>10} {'Total':>10}")
print("-" * 40)
print(f"{item:<10} {qty:>5} {price:>10.2f} {total:>10.2f}")
Output:
Item Qty Price Total
----------------------------------------
Orange 3 1.25 3.75
Why use f-strings?
- Cleaner syntax
- Faster performance
- Easier to debug and edit
- Still supports all formatting options like
.2f
,:>10
,:<15
, etc.
Why use f-strings?
- Cleaner syntax
- Faster performance
- Easier to debug and edit
- Still supports all formatting options like
.2f
,:>10
,:<15
, etc.
Now let’s take your formatting skills a step further by using format()
inside loops. This is especially useful when you’re working with dynamic output, like creating logs, showing results, or printing data reports.
Using format()
in Loops and Dynamic Output
When you’re looping through data, format()
lets you insert values directly into strings for clean, readable output—even when the content changes every time.
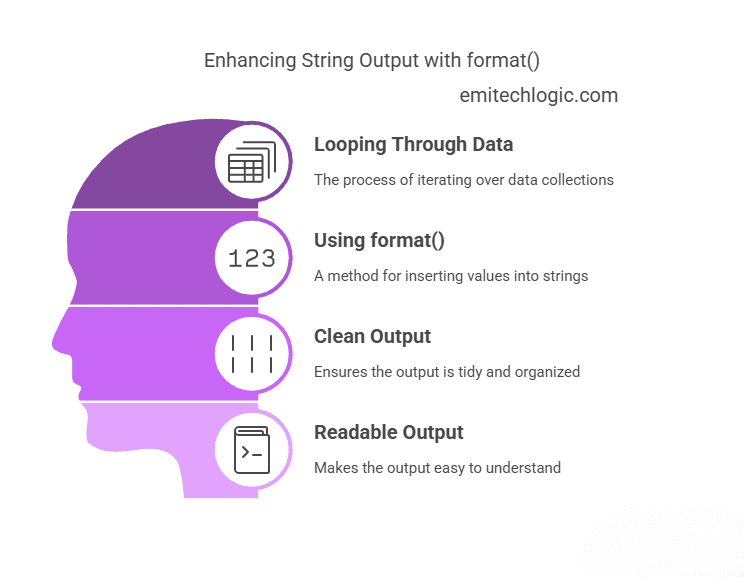
Example: Print Index and Square
for i in range(5):
print("Index: {}, Square: {}".format(i, i * i))
Output:
Index: 0, Square: 0
Index: 1, Square: 1
Index: 2, Square: 4
Index: 3, Square: 9
Index: 4, Square: 16
Why this is useful
- Perfect for debugging, generating logs, or reporting results.
- Keeps your print statements neat and consistent, no matter how large your dataset.
- You can easily adjust formatting later (e.g., align columns, format numbers) without changing your core loop logic.
You can also add format specifiers
for i in range(1, 6):
print("Index: {:>2}, Square: {:>3}".format(i, i * i))
Output:
Index: 1, Square: 1
Index: 2, Square: 4
Index: 3, Square: 9
Index: 4, Square: 16
Index: 5, Square: 25
This uses :>2
and :>3
to right-align the numbers in consistent-width columns, which makes the output much easier to read—especially when you have larger values.
Let’s take that same loop example and upgrade it using f-strings. This is the cleaner and more modern way to handle dynamic output in loops, and it works just as well—if not better—for logs, reports, or any data processing.
Using f-Strings in Loops and Dynamic Output
You don’t need .format()
anymore—just add an f
before the string and write your variables directly inside {}
. You can even use format specifiers right there.
Example: Using f-strings in a loop
for i in range(5):
print(f"Index: {i}, Square: {i * i}")
Output:
Index: 0, Square: 0
Index: 1, Square: 1
Index: 2, Square: 4
Index: 3, Square: 9
Index: 4, Square: 16
Same result, but the code is easier to read and write.
Now, with Alignment and Precision
Let’s improve readability by aligning the numbers:
for i in range(1, 6):
print(f"Index: {i:>2}, Square: {i * i:>3}")
Output:
Index: 1, Square: 1
Index: 2, Square: 4
Index: 3, Square: 9
Index: 4, Square: 16
Index: 5, Square: 25
:>2
right-aligns the index to 2 spaces.:>3
right-aligns the square to 3 spaces.
Why use f-strings in loops?
- Clean and readable, even with multiple variables.
- Supports all formatting options (
:.2f
,:>10
, etc.). - Ideal for logging, data tables, and quick reports.
If you’re building anything that involves data rows, progress tracking, or even debugging output, f-strings in loops can really clean things up.
Let’s level up with a real-world use case: looping through a list of dictionaries—like product data, user info, or logs—and printing everything cleanly using f-strings.
Formatting Complex Data in Loops with f-Strings
Let’s say you’re working with a list of user records. Each record is a dictionary with name
, email
, and age
.
Example: Loop Through a List of Dictionaries
users = [
{"name": "Alice", "email": "alice@example.com", "age": 28},
{"name": "Bob", "email": "bob@example.com", "age": 34},
{"name": "Charlie", "email": "charlie@example.com", "age": 22},
]
print(f"{'Name':<10} {'Email':<25} {'Age':>3}")
print("-" * 40)
for user in users:
print(f"{user['name']:<10} {user['email']:<25} {user['age']:>3}")
Output:
Name Email Age
----------------------------------------
Alice alice@example.com 28
Bob bob@example.com 34
Charlie charlie@example.com 22
Why this works well:
:<10
aligns the name to the left in a 10-character column.:<25
ensures email is readable and spaced out.:>3
right-aligns the age for a clean, tabular look.
Add Timestamps for Log Output
Let’s simulate a simple log system where each message is printed with the current timestamp:
from datetime import datetime
events = ["User logged in", "File uploaded", "Error occurred", "User logged out"]
for event in events:
timestamp = datetime.now().strftime("%Y-%m-%d %H:%M:%S")
print(f"[{timestamp}] {event}")
Output (example):
[2025-04-09 14:32:12] User logged in
[2025-04-09 14:32:12] File uploaded
[2025-04-09 14:32:12] Error occurred
[2025-04-09 14:32:12] User logged out
You can use this to format debug logs, activity feeds, or execution reports with clarity.
📝 Bonus: print()
vs .format()
vs f-Strings (With Examples)
Feature | print() (basic) | .format() | f-Strings |
---|---|---|---|
Basic Usage | print("Name:", "Alice") | print("Name: {}".format("Alice")) | name = "Alice" print(f"Name: {name}") |
Multiple Values | print("Name:", "Bob", "Age:", 25) | print("Name: {}, Age: {}".format("Bob", 25)) | name = "Bob" age = 25 print(f"Name: {name}, Age: {age}") |
Readability | Okay, can get messy | Harder with many placeholders | ✅ Very clean: print(f"Hello, {name}") |
Formatting Numbers | ❌ Not built-in | value = 3.14 print("Pi: {:.2f}".format(value)) | value = 3.14 print(f"Pi: {value:.2f}") |
Positional Arguments | ❌ Not supported | print("{1}, {0}".format("first", "second")) | a, b = "first", "second" print(f"{b}, {a}") |
Named Placeholders | ❌ Not supported | print("{name}".format(name="Alex")) | name = "Alex" print(f"{name}") |
Works in Loops | ✅ for i in range(3): print(i) | ✅ for i in range(3): print("Index: {}".format(i)) | ✅ for i in range(3): print(f"Index: {i}") |
Alignment & Padding | ❌ Manual spacing only | print("{:<10}".format("Item")) | print(f"{'Item':<10}") |
Speed / Performance | Fast enough for most uses | Slower than f-Strings | ✅ Fastest method (preferred in large scripts) |
Python Version | All versions | Python 2.7+ and 3.x | ✅ Python 3.6+ only |
Best For | Beginners, quick checks | Compatible formatting with older code | ✅ Clean scripts, logging, dynamic data |
Common Mistakes with print()
and .format()
— and How to Fix Them

1. Missing Arguments in .format()
If you use placeholders {}
but don’t provide enough values, Python will raise an error.
# ❌ Incorrect:
print("Name: {}, Age: {}".format("Alice"))
# ❗ Error:
# IndexError: Replacement index 1 out of range for positional args tuple
Fix: Provide all required arguments.
print("Name: {}, Age: {}".format("Alice", 30))
2. Misordered Placeholders
Using {1}
and {0}
means you’re specifying the order manually. If you mix it up, the output becomes confusing.
# ❌ Incorrect:
print("{1}, {0}".format("first"))
# ❗ Error:
# IndexError: tuple index out of range
Fix: Make sure you provide the right number of values, in the correct order.
print("{1}, {0}".format("first", "second"))
# Output: second, first
3. Forgetting to Call .format()
This happens when you write the string with {}
but forget to use .format()
at the end.
# ❌ Incorrect:
print("Hello, {}")
# Output: Hello, {}
Fix: Call .format()
with the value you want to insert.
print("Hello, {}".format("Alex"))
# Output: Hello, Alex
Bonus Tip: Watch Out for Mixing Styles
Using f-Strings and .format()
together won’t work:
# ❌ Incorrect:
name = "Sam"
print(f"Hello, {}".format(name)) # Won't work
Fix: Use one or the other, not both.
# f-String
print(f"Hello, {name}")
# OR .format()
print("Hello, {}".format(name))
Conclusion: Mastering Python String Formatting with print()
and format()
By now, you’ve seen how powerful and flexible string formatting in Python can be. From the basic print()
statement to more dynamic output using the format()
function, you’ve learned how to:
- Use placeholders and positional arguments
- Improve readability with named arguments
- Control output with format specifiers like
:.2f
,:<
,:^
, and more - Apply formatting in loops and real-world use cases
- Avoid common mistakes, like missing arguments or forgetting the
.format()
call
You also got a side-by-side comparison of print()
, .format()
, and f-Strings, so you know exactly when to use each one.
Whether you’re just starting out or brushing up your Python basics, understanding how to use print()
and format()
the right way helps you write cleaner, clearer, and more professional code.
💡 Found this helpful?
Do me a favor — share this post with your friends, colleagues, or on your social media. You never know who might need a quick Python refresher before their next interview.
✍️ For more Python tricks, breakdowns, and real-world coding insights, head over to emitechlogic.com. I post new tutorials regularly!
Thanks for reading
📲 Follow for Python tips & tricks
📸 Instagram: https://www.instagram.com/emi_techlogic/
💼 LinkedIn: https://www.linkedin.com/in/emmimal-alexander/
#PythonQuiz #CodingInterview #PythonInterviewQuestions #MCQChallenge #PythonMCQ #EmitechLogic #UncoveredAI #PythonTips #AIwithEmi #PythonCoding #TechWithEmi
FAQs on Format Strings in Python
print()
and .format()
in Python? The print()
function in Python is used to display output on the console, while .format()
is a method used to insert values into strings in a controlled way. You can use .format()
inside a print()
statement to create formatted output. For example:
print(“Hello, {}”.format(“Alice”))
This prints: Hello, Alice
— combining both print()
and .format()
.
format()
function? You can use multiple curly braces {}
to define placeholders for each value, then pass those values in order inside .format()
. This is helpful when creating formatted output in Python using multiple values:
print(“Name: {}, Age: {}”.format(“Bob”, 25))
If you want to control the order, use index-based placeholders:
print(“{1}, {0}”.format(“first”, “second”)) # Output: second, first
.format()
placeholder not working in the Python print statement? One of the most common Python string formatting errors is forgetting to actually call the .format()
method. If you write:
print(“Hello, {}”)
You’ll just get: Hello, {}
Fix it by adding .format()
:
print(“Hello, {}”.format(“Alex”))
.format()
or f-Strings for formatting strings in Python? If you’re using Python 3.6 or later, f-Strings are usually better than .format()
because they are faster and easier to read. For example:
name = “Emma”
print(f”Hello, {name}”)
But if you’re writing code for older Python versions, the format()
function is still the best choice for string interpolation in Python.
External Resources for Format Strings in Python
🔗 Python Official Documentation: str.format()
This is the official Python documentation with detailed syntax, format specifiers, and use cases.
Leave a Reply