Practical Exercises on Conditional Statements in Python
Master If-Elif-Else Logic with Fun and Interactive Examples
Introduction to Python Conditional Statements
What are conditional statements in Python?
Think of conditional statements like a smart traffic light. They help your program make decisions. Just like you decide whether to wear a jacket based on the weather, Python uses conditional statements to choose what to do next.
A conditional statement asks a simple question. If the answer is yes, it does one thing. If the answer is no, it does something else. This is how computers think and make choices.
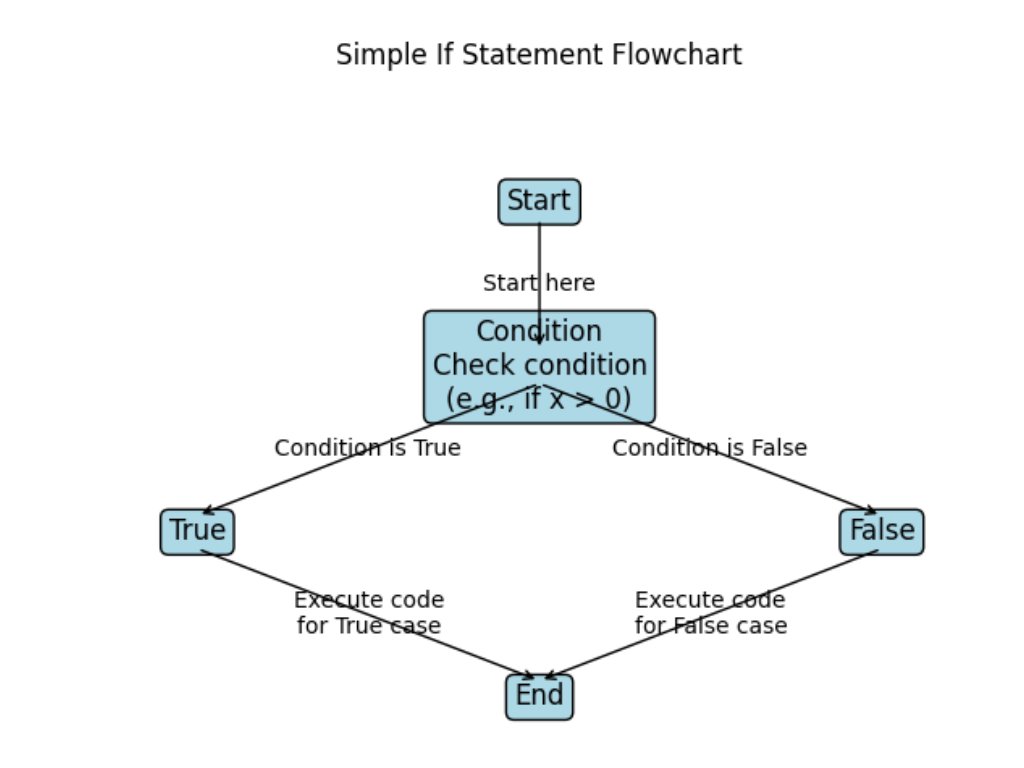
Importance of mastering if-elif-else in Python
Learning conditionals is like learning to drive. You need to know when to stop, go, or turn. Every program you write will use conditionals. They are the brain of your code.
Without conditionals, your programs would be boring. They would do the same thing every time. With conditionals, your programs become smart and interactive.
How conditional logic powers decision-making in programs
Imagine you are a robot butler. Your owner says “If it’s raining, bring an umbrella. Otherwise, don’t.” This is exactly how conditional logic works in programming.
Programs use conditionals to:
- Check if a user entered the right password
- Decide what message to show
- Calculate different prices for different customers
- Control game characters
Before we dive deeper, you might want to check out our guide on Python data types to understand the basics better.
Getting Started: Python If Statement Practice for Beginners
Basic If Statement Exercises in Python
Let’s start simple. An if statement is like asking “Is this true?” If yes, do something. If no, do nothing.
The basic structure looks like this:
if condition: # Do something here print("This will run if condition is true")
Let’s write a program that checks if a number is positive. A positive number is greater than zero.
# Exercise 1: Check if a number is positive number = 5 if number > 0: print("This number is positive!")
How it works:
- We store the number 5 in a variable called ‘number’
- We check if number is greater than 0
- Since 5 > 0 is true, the print statement runs
- If we used -3 instead, nothing would print
In most countries, you can vote when you turn 18. Let’s check if someone can vote.
# Exercise 2: Check voting eligibility age = 20 if age >= 18: print("You can vote!")
How it works:
- We store the age 20 in a variable called ‘age’
- We check if age is greater than or equal to 18
- Since 20 >= 18 is true, the message prints
- The >= symbol means “greater than or equal to”
Python If-Else Practice Problems

Sometimes you want to do something when the condition is false. This is where ‘else’ comes in. Think of it as “if this, otherwise that.”
if condition: # Do this if condition is true print("Condition is true") else: # Do this if condition is false print("Condition is false")
Let’s write a program that tells us if a number is odd or even. We use the % operator to find the remainder when dividing by 2.
# Exercise 3: Check if number is odd or even number = 7 if number % 2 == 0: print("This number is even") else: print("This number is odd")
How it works:
- We use % to find the remainder when dividing by 2
- If the remainder is 0, the number is even
- If the remainder is not 0, the number is odd
- 7 divided by 2 gives remainder 1, so it’s odd
Let’s check if a student passed or failed based on their score. We’ll say 60 or above is a pass.
# Exercise 4: Check pass or fail score = 75 if score >= 60: print("Congratulations! You passed!") else: print("Sorry, you failed. Try again!")
How it works:
- We check if the score is 60 or higher
- Since 75 >= 60 is true, it prints the pass message
- If the score was 45, it would print the fail message
- The else part only runs when the if condition is false
Try it yourself: Height checker for roller coaster
Create a program that checks if someone is tall enough for a roller coaster (minimum height 120 cm).
Hands-On Python Elif Exercises for Intermediate Learners
Using Elif for Multi-Condition Checks
Sometimes you have more than two choices. This is where ‘elif’ (else if) comes in handy. It’s like having multiple questions to ask.
Think of elif like a multiple choice question:
- If choice A is true, do this
- Else if choice B is true, do that
- Else if choice C is true, do something else
- Otherwise, do the default thing
if condition1: # Do this if condition1 is true elif condition2: # Do this if condition2 is true elif condition3: # Do this if condition3 is true else: # Do this if all conditions are false
Let’s convert number scores into letter grades. This is perfect for elif practice!
# Exercise 5: Convert score to letter grade score = 85 if score >= 90: print("Grade: A - Excellent!") elif score >= 80: print("Grade: B - Good job!") elif score >= 70: print("Grade: C - Not bad!") elif score >= 60: print("Grade: D - You passed!") else: print("Grade: F - Try harder next time!")
How it works:
- Python checks each condition from top to bottom
- 85 is not >= 90, so it skips the first condition
- 85 is >= 80, so it prints “Grade: B” and stops
- It doesn’t check the remaining conditions
- This is called “short-circuit evaluation”
Let’s determine the season based on the month number. This shows how elif can handle multiple ranges.
# Exercise 6: Determine season from month month = 7 if month in [12, 1, 2]: print("It's Winter!") elif month in [3, 4, 5]: print("It's Spring!") elif month in [6, 7, 8]: print("It's Summer!") elif month in [9, 10, 11]: print("It's Fall!") else: print("Invalid month number!")
How it works:
- We use the ‘in’ operator to check if month is in a list
- Month 7 (July) is in the summer list [6, 7, 8]
- So it prints “It’s Summer!” and stops checking
- The else handles invalid month numbers (like 13 or 0)
Python Elif Ladder Exercise with Real-World Example
Let’s create a traffic light system. This is a perfect real-world example of elif usage.
# Exercise 7: Traffic light system light_color = "yellow" if light_color == "red": print("STOP! Do not move!") elif light_color == "yellow": print("CAUTION! Slow down and prepare to stop!") elif light_color == "green": print("GO! Drive safely!") else: print("Unknown signal! Be careful!")
How it works:
- We check the exact color of the traffic light
- The == operator checks if two things are exactly equal
- Since light_color is “yellow”, it matches the second condition
- Each color gives a different instruction to the driver
Grade Calculator Demo
Enter a score to see what grade it gets:
Nested If-Else Practice Problems in Python

Understanding Nested Conditional Statements with Practice
Nested if statements are like Russian dolls. You have one if statement inside another if statement. This lets you ask multiple questions in a row.
Think of it like this: “If it’s raining, then if I have an umbrella, I’ll go outside. Otherwise, I’ll stay home.”
if outer_condition: # Check another condition inside if inner_condition: # Both conditions are true print("Both conditions met") else: # Only outer condition is true print("Only outer condition met") else: # Outer condition is false print("Outer condition not met")
Let’s create a login system that first checks if the password is correct, then checks what type of user it is.
# Exercise 8: Login system with role check username = "admin" password = "secret123" user_role = "admin" if password == "secret123": print("Password correct!") if user_role == "admin": print("Welcome Admin! You have full access.") elif user_role == "user": print("Welcome User! You have limited access.") else: print("Welcome Guest! Read-only access.") else: print("Wrong password! Access denied.")
How it works:
- First, we check if the password is correct
- Only if password is right, we check the user role
- This prevents unauthorized users from even seeing role options
- The inner if-elif-else only runs when outer if is true
Let’s create a shopping system that gives different discounts based on customer type and purchase amount.
# Exercise 9: Discount calculation system customer_type = "premium" purchase_amount = 150 if customer_type == "premium": print("Premium customer detected!") if purchase_amount >= 100: discount = 20 # 20% discount print(f"Big purchase! {discount}% discount applied!") else: discount = 10 # 10% discount print(f"Small purchase! {discount}% discount applied!") elif customer_type == "regular": print("Regular customer!") if purchase_amount >= 200: discount = 15 # 15% discount print(f"Large purchase! {discount}% discount applied!") else: discount = 5 # 5% discount print(f"Standard discount: {discount}%") else: print("New customer - no discount this time!") discount = 0 final_price = purchase_amount * (1 - discount/100) print(f"Final price: ${final_price:.2f}")
How it works:
- First level checks customer type (premium, regular, or new)
- Second level checks purchase amount for each customer type
- Different discounts apply based on both conditions
- Finally calculates the actual price after discount
Common Pitfalls in Nested Conditional Exercises
Indentation Issues
The biggest mistake beginners make with nested if statements is wrong indentation. Each level of nesting needs 4 more spaces.
# WRONG - Bad indentation if condition1: if condition2: # Should be indented! print("Hello") # Should be indented more! # CORRECT - Good indentation if condition1: if condition2: print("Hello")
Logical Error Traps
Another common mistake is putting conditions in the wrong order. Remember, Python checks from top to bottom.
# WRONG - This will never work correctly if score >= 0: print("You got a grade!") elif score >= 90: print("A grade!") # This never runs! # CORRECT - Most specific first if score >= 90: print("A grade!") elif score >= 0: print("You got a grade!")
Advanced Python If-Else Practice Projects

Mini Projects Using Conditional Logic in Python
Now let’s build some real projects! These will help you practice everything you’ve learned so far.
Let’s build a simple ATM machine that can check balance and handle withdrawals.
# Project 1: ATM Simulator account_balance = 1000 pin = "1234" entered_pin = "1234" action = "withdraw" withdraw_amount = 200 print("Welcome to Python ATM!") if entered_pin == pin: print("PIN correct! Access granted.") if action == "balance": print(f"Your balance is: ${account_balance}") elif action == "withdraw": if withdraw_amount <= account_balance: if withdraw_amount > 0: account_balance = account_balance - withdraw_amount print(f"Withdrew ${withdraw_amount}") print(f"New balance: ${account_balance}") else: print("Invalid amount! Must be greater than 0.") else: print("Insufficient funds!") else: print("Unknown action!") else: print("Wrong PIN! Access denied.")
Let’s create the classic game using conditional statements to determine the winner.
# Project 2: Rock-Paper-Scissors Game player_choice = "rock" computer_choice = "scissors" print(f"You chose: {player_choice}") print(f"Computer chose: {computer_choice}") if player_choice == computer_choice: print("It's a tie!") elif player_choice == "rock": if computer_choice == "scissors": print("You win! Rock crushes scissors!") else: # computer chose paper print("You lose! Paper covers rock!") elif player_choice == "paper": if computer_choice == "rock": print("You win! Paper covers rock!") else: # computer chose scissors print("You lose! Scissors cut paper!") elif player_choice == "scissors": if computer_choice == "paper": print("You win! Scissors cut paper!") else: # computer chose rock print("You lose! Rock crushes scissors!") else: print("Invalid choice! Please choose rock, paper, or scissors.")
Using Conditional Logic in Menu-Driven Python Programs
Let’s build a calculator that shows a menu and performs different operations.
# Project 3: Simple Calculator print("=== Python Calculator ===") print("1. Add") print("2. Subtract") print("3. Multiply") print("4. Divide") choice = 1 num1 = 10 num2 = 5 if choice == 1: result = num1 + num2 print(f"{num1} + {num2} = {result}") elif choice == 2: result = num1 - num2 print(f"{num1} - {num2} = {result}") elif choice == 3: result = num1 * num2 print(f"{num1} * {num2} = {result}") elif choice == 4: if num2 != 0: result = num1 / num2 print(f"{num1} / {num2} = {result}") else: print("Error: Cannot divide by zero!") else: print("Invalid choice! Please select 1-4.")
Let’s create a system that recommends movies based on user preferences.
# Project 4: Movie Recommendation System age = 25 genre_preference = "action" mood = "exciting" print("=== Movie Recommendation System ===") if age < 13: print("Recommended: Family-friendly movies only!") if genre_preference == "animation": print("Try: Toy Story, Finding Nemo") else: print("Try: The Lion King, Frozen") elif age < 18: print("Recommended: Teen-appropriate movies") if genre_preference == "action": print("Try: Spider-Man, The Avengers") elif genre_preference == "comedy": print("Try: School of Rock, The Princess Bride") else: print("Try: Harry Potter series") else: # Adult movies if mood == "exciting": if genre_preference == "action": print("Try: John Wick, Mad Max: Fury Road") elif genre_preference == "thriller": print("Try: Inception, The Dark Knight") else: print("Try: Fast & Furious series") elif mood == "relaxing": if genre_preference == "romance": print("Try: The Notebook, Titanic") else: print("Try: Forrest Gump, The Shawshank Redemption") else: print("Try: Popular movies from this year!")
Condition-Based Python Programs for Practice
Create an alarm system that sets different wake-up times for weekdays and weekends.
# Exercise 10: Smart alarm system day_of_week = "Saturday" is_holiday = False has_important_meeting = True if day_of_week in ["Saturday", "Sunday"]: if has_important_meeting: alarm_time = "8:00 AM" print("Weekend but you have a meeting!") else: alarm_time = "10:00 AM" print("Weekend sleep-in time!") else: if is_holiday: alarm_time = "9:00 AM" print("Holiday! Sleep a bit longer.") else: alarm_time = "7:00 AM" print("Weekday - time for work!") print(f"Alarm set for: {alarm_time}")
Create a restaurant billing system that applies different discounts based on time and day.
# Exercise 11: Restaurant billing system bill_amount = 50 time_of_day = "lunch" # breakfast, lunch, dinner day_type = "weekday" # weekday or weekend is_senior = False print(f"Original bill: ${bill_amount}") if time_of_day == "breakfast": discount = 15 # 15% breakfast discount print("Early bird breakfast discount!") elif time_of_day == "lunch": if day_type == "weekday": discount = 10 # 10% weekday lunch discount print("Weekday lunch special!") else: discount = 5 # 5% weekend lunch print("Weekend lunch - small discount") else: # dinner discount = 0 # No dinner discount print("Dinner - no time discount") # Additional senior discount if is_senior: discount += 10 # Extra 10% for seniors print("Senior citizen additional discount!") final_amount = bill_amount * (1 - discount/100) print(f"Total discount: {discount}%") print(f"Final bill: ${final_amount:.2f}")
Create a BMI calculator that provides health advice based on the calculated BMI.
# Exercise 12: BMI Calculator with feedback weight = 70 # kg height = 1.75 # meters # Calculate BMI bmi = weight / (height * height) print(f"Your BMI is: {bmi:.2f}") if bmi < 18.5: category = "Underweight" advice = "Consider eating more nutritious foods and consult a doctor." elif bmi < 25: category = "Normal weight" advice = "Great! Maintain your current lifestyle." elif bmi < 30: category = "Overweight" advice = "Consider regular exercise and a balanced diet." else: category = "Obese" advice = "Please consult a healthcare professional for guidance." print(f"Category: {category}") print(f"Advice: {advice}") # Additional specific advice if bmi > 25: print("Recommended: 30 minutes of exercise daily") elif bmi < 18.5: print("Recommended: Focus on protein-rich foods") else: print("Keep up the good work!")
Create a quiz system that calculates scores and provides feedback based on performance.
# Exercise 13: Quiz scoring system total_questions = 10 correct_answers = 8 time_taken = 15 # minutes difficulty_level = "medium" # easy, medium, hard # Calculate percentage percentage = (correct_answers / total_questions) * 100 print(f"You got {correct_answers}/{total_questions} correct ({percentage}%)") # Determine grade based on percentage if percentage >= 90: grade = "A" feedback = "Outstanding!" elif percentage >= 80: grade = "B" feedback = "Great job!" elif percentage >= 70: grade = "C" feedback = "Good work!" elif percentage >= 60: grade = "D" feedback = "You passed, but try harder next time." else: grade = "F" feedback = "Study more and retake the quiz." print(f"Grade: {grade} - {feedback}") # Bonus points for difficulty and speed bonus_points = 0 if difficulty_level == "hard" and percentage >= 70: bonus_points += 5 print("Bonus: +5 points for completing hard quiz!") if time_taken <= 10 and percentage >= 80: bonus_points += 3 print("Speed bonus: +3 points for quick completion!") if bonus_points > 0: final_percentage = percentage + bonus_points print(f"Final score with bonus: {final_percentage}%")
How to Practice Python Conditional Statements Effectively
Tips for Practicing Python If-Else Logic
Break problems into smaller conditions
Don’t try to solve everything at once. Start with one simple condition, then add more. This is like building with blocks – start with one block, then stack more on top.
For example, instead of writing a complex grade calculator all at once:
- First, check if score is above 60 (pass/fail)
- Then add A, B, C, D grades
- Finally add bonus features
Use flowcharts or pseudocode to structure logic
Before writing code, draw out your thinking. A flowchart shows the path your program should take. Pseudocode is like writing instructions in plain English first.
Example pseudocode for a weather app:
# Pseudocode - plan before coding # IF it's raining # IF temperature is cold # Suggest: "Wear a jacket and take umbrella" # ELSE # Suggest: "Take an umbrella" # ELSE # IF temperature is hot # Suggest: "Wear light clothes" # ELSE # Suggest: "Perfect weather!"
Online Platforms to Practice Python Conditionals
Here are some great places to practice what you’ve learned. Start with easy problems and work your way up!
HackerRank offers a structured approach to learning. They have specific sections for conditional statements with problems ranging from beginner to advanced.
- Start with “30 Days of Code” challenge
- Focus on “If-Else” and “Loops” sections
- Try “Problem Solving” track for real challenges
LeetCode has problems that real companies ask in interviews. Filter by “Easy” difficulty and look for problems involving conditions.
- Search for problems with tags like “simulation”
- Practice “Easy” level problems first
- Focus on understanding logic before speed
These sites offer interactive exercises where you can practice right in your browser.
- W3Schools has “Try it Yourself” examples
- Programiz offers step-by-step explanations
- Both have online Python editors for practice
Also check out our guides on Python overview and applications and input and output operations to expand your Python knowledge.
Debugging Python Conditional Statement Errors
Common Logical Mistakes in If-Else Conditions
Unreachable code in elif chains
This happens when you put a general condition before a specific one. Python checks conditions from top to bottom and stops at the first true condition.
# WRONG - Unreachable code score = 95 if score >= 60: print("You passed!") elif score >= 90: print("Excellent! A grade!") # This NEVER runs! # CORRECT - Most specific first if score >= 90: print("Excellent! A grade!") elif score >= 60: print("You passed!")
Misplaced conditions and wrong comparisons
Using the wrong comparison operators or comparing the wrong values can break your logic.
# WRONG - Common mistakes age = "18" # String, not number! if age >= 18: # Comparing string to number print("Can vote") password = "secret" if password = "secret": # Using = instead of == print("Correct password") # CORRECT - Fixed versions age = 18 # Number, not string if age >= 18: print("Can vote") password = "secret" if password == "secret": # Using == for comparison print("Correct password")
Using Print Statements and IDEs to Trace Errors
How to visualize the flow of execution
Add print statements to see what your program is actually doing. This is like leaving breadcrumbs to follow your program’s path.
# Debug version with print statements age = 16 has_license = True print(f"Debug: age = {age}, has_license = {has_license}") if age >= 18: print("Debug: Age check passed") if has_license: print("Debug: License check passed") print("You can drive!") else: print("Debug: No license") print("Get a license first!") else: print("Debug: Age check failed") print("Too young to drive!")
Importance of writing test cases
Test your code with different inputs to make sure it works in all situations. Think of edge cases – unusual situations that might break your code.
# Test different scenarios def test_grade_calculator(): # Test normal cases test_cases = [ (95, "A"), # High score (85, "B"), # Medium score (75, "C"), # Low passing (55, "F"), # Failing # Edge cases (90, "A"), # Exactly 90 (89, "B"), # Just below 90 (0, "F"), # Zero score (100, "A") # Perfect score ] for score, expected_grade in test_cases: # Your grade calculation logic here if score >= 90: grade = "A" elif score >= 80: grade = "B" elif score >= 70: grade = "C" elif score >= 60: grade = "D" else: grade = "F" print(f"Score {score}: Expected {expected_grade}, Got {grade}")
For more advanced debugging techniques, check out our guide on error management in Python.
Bonus: Python Conditional Statements Quiz
Test Your Python Conditional Logic Skills
Let’s see how much you’ve learned! Try to answer these questions without looking back at the examples.
Question 1: What will this code print?
x = 15 if x > 10: if x < 20: print("Medium") else: print("Large") else: print("Small")
Question 2: Which operator checks if two values are equal?
Question 3: What’s wrong with this code?
score = 85 if score >= 70: print("Pass") elif score >= 80: print("Good") elif score >= 90: print("Excellent")
Question 4: Fill in the blank to check if a number is even:
number = 8 if number _____ 2 == 0: print("Even")
Question 5: What does elif stand for?
Conclusion: Mastering Python If-Else Through Practice
Recap of covered exercises
Congratulations! You’ve completed a comprehensive journey through Python conditional statements. Let’s recap what you’ve learned:
Basic Concepts Covered:
- Simple if statements – Making basic decisions
- If-else statements – Choosing between two options
- Elif chains – Handling multiple conditions
- Nested conditionals – Making complex decisions
- Real-world projects – Applying concepts practically
13 Exercises You Completed:
- Positive number checker
- Voting eligibility checker
- Odd or even number detector
- Grade pass or fail system
- Letter grade calculator
- Season detector from month
- Traffic light interpreter
- Login validation system
- Multi-level discount calculator
- Smart alarm system
- Restaurant billing system
- BMI calculator with feedback
- Quiz scoring system
4 Advanced Projects You Built:
- ATM simulator with balance checking and withdrawals
- Rock-Paper-Scissors game with win/loss logic
- Calculator with menu-driven interface
- Movie recommendation system with multiple criteria
How repeated practice improves logic building
Every exercise you completed has strengthened your logical thinking. Programming is like learning a musical instrument – the more you practice, the more natural it becomes.
Each time you wrote an if statement, you were training your brain to:
- Break down complex problems into simple yes/no questions
- Think about all possible scenarios (edge cases)
- Structure your thoughts in a logical flow
- Debug and fix logical errors
Encouragement to try custom real-world problems
Now that you’ve mastered the basics, it’s time to create your own projects! Think about problems in your daily life that could be solved with conditional logic:
- Personal Finance Tracker: Categorize expenses and suggest budget adjustments
- Study Schedule Optimizer: Recommend study times based on difficulty and deadlines
- Workout Planner: Suggest exercises based on fitness level and available time
- Recipe Recommender: Suggest meals based on ingredients you have
- Travel Advisor: Recommend destinations based on budget and preferences
- Smart Home Controller: Automate lights and temperature based on time and occupancy
Start small, think big, and most importantly – have fun coding! Every expert programmer started exactly where you are now.
FAQs on Practicing Conditional Statements in Python
The best way is through hands-on practice with real examples. Start with simple if statements, then gradually work up to complex nested conditions. Don’t just read about it – write code every day!
Here’s a proven learning path:
- Master basic if statements first
- Practice if-else with different data types
- Learn elif for multiple choices
- Try nested conditions carefully
- Build real projects that interest you
Python has three main conditional statements:
- if statement: Executes code only if condition is true
- if-else statement: Chooses between two code blocks
- if-elif-else statement: Handles multiple conditions
You can also use:
- Nested conditionals: If statements inside other if statements
- Conditional expressions (ternary operator): Short one-line conditions
- Boolean operators: and, or, not for complex conditions
Conditional statements are everywhere in software! Here are some examples:
Web Applications:
- User authentication (login/logout)
- Shopping cart discounts
- Content filtering by age or region
- Form validation
Mobile Apps:
- GPS navigation (route selection)
- Camera apps (auto-focus, flash)
- Weather apps (alert conditions)
- Game logic (winning/losing conditions)
Business Systems:
- Payroll calculations (overtime, taxes)
- Inventory management (reorder alerts)
- Customer service (ticket routing)
- Financial risk assessment
AI and Machine Learning:
- Decision trees
- Data preprocessing
- Model validation
- Automated trading systems
Here are the most common mistakes and how to avoid them:
1. Using = instead of ==
Remember: = assigns a value, == compares values
2. Wrong indentation
Python is strict about indentation. Use 4 spaces for each level
3. Unreachable elif conditions
Put most specific conditions first, general ones last
4. Comparing different data types
Make sure you’re comparing numbers to numbers, strings to strings
Conditional statements are fundamental and should be learned early. You should know these basics first:
- Variables and data types – numbers, strings, booleans
- Basic operators – +, -, *, /, %, ==, !=, <, >
- Input and output – print() and input() functions
After mastering conditionals, you can move on to:
- Loops (for and while)
- Functions
- Lists and dictionaries
- File handling
External Resources for Further Learning
Official Python Documentation
For a deeper dive into Python’s control flow statements, including detailed explanations and advanced examples, visit the official Python documentation:
Python Control Flow Documentation
This resource covers if statements, for loops, while loops, and more advanced control flow tools.
Continue Your Python Journey
Ready to expand your Python skills? Check out these related tutorials on our site:
- The Ultimate Guide to Python Data Types – Master strings, numbers, and booleans
- Getting Started with Python – Overview and real-world applications
- Mastering Input and Output Operations – Handle user input like a pro
- File Handling and Error Management – Build robust applications
Leave a Reply