Introduction
If you’re familiar with Python, you’ve probably worked with plenty of modules already. From simple math functions to complex data manipulations, Modules in Python make it easy to keep things organized and reusable. But have you ever thought about creating your own Python modules? Whether you’re working on a small project or building something more complex, custom modules can help you break your code into manageable pieces.
Creating your own Python modules might sound a bit technical, but it’s actually much simpler than you think. Not only does it make your code cleaner, but it also saves time by allowing you to reuse your functions and classes in different projects. Plus, it’s a skill every Python developer should have in their toolbox!
In this guide, we’ll walk you through everything you need to know about building custom Python modules. From setting up your development environment to organizing your code into packages, we’ll cover all the essentials. By the end of this post, you’ll feel confident creating modules that can be shared across your projects—or even published for others to use.
Ready to take your Python skills to the next level? Let’s get started!
Introduction to Python Modules
Python is a powerful programming language, and one of its best features is how organized and reusable you can make your code. At some point, you’ll likely want to break your code into smaller, reusable parts, and that’s where Python modules come into play. When you’re working on larger projects or even simple programs, understanding how to create and use Python modules is key to keeping your code clean and organized.
What is a Python Module?
A Python module is simply a file containing Python definitions and statements. It’s essentially a .py file that can contain variables, functions, and classes. Imagine a scenario where you write a function and need to reuse it across different scripts. Instead of copying and pasting it, you can write it once in a module and import it wherever it’s needed.
In real-life programming, I often use modules to avoid rewriting functions for tasks like data processing or file handling. With a module, I can build a library of tools to use across multiple projects. Trust me, it saves a ton of time!
Definition of Python Modules
At its core, a module is just another Python file, but it’s used in a specific way. You could define it as:
“A Python module is a file that contains Python code, allowing you to reuse that code in other scripts by importing the module.” – Emmimal Alexander
Modules can either be standard library modules (which come with Python) or user-defined modules (which you create yourself). For example, Python’s math module comes built-in and gives you access to various mathematical functions. Similarly, you can create your own module for custom functionality.
Difference Between Scripts and Modules in Python
Now, let’s clear up the difference between Python scripts and Python modules because people often mix these up.
- A Python script is any Python file you run directly. For example, if you create a file named
hello.py
and run it with the commandpython hello.py
, that’s a script. - A Python module, on the other hand, is a file you don’t necessarily run directly but import into another script or module.
Think of a script as a standalone program, whereas a module is a piece of code that supports other scripts. Here’s a simple example:
# script.py
import mymodule
mymodule.greet("Emmimal")
# mymodule.py (this is the module)
def greet(name):
print(f"Hello, {name}!")
In this example, mymodule.py is a module that contains a greet()
function. The script.py imports this module and calls the greet()
function. Instead of rewriting the greet()
function in script.py, I can import it whenever needed.
What Are Python Modules and How to Create Them?
Creating a Python module is as easy as saving a Python file. Here’s how you can create and use one in your projects:
- Write a Python file – Create a file with a
.py
extension. For example, let’s create a file calledmy_module.py
. - Add functionality – Define your functions, classes, or variables within that file.
# my_module.py
def add_numbers(a, b):
return a + b
3. Import it into other scripts – Once you’ve defined the functionality in the module, you can import it into another Python file using the import
statement.
# another_script.py
import my_module
result = my_module.add_numbers(5, 10)
print(result) # Output will be 15
That’s it! You’ve just created a module and imported it into another script. It’s a simple yet powerful way to keep your code organized and maintainable. The more you work with Python, the more you’ll appreciate how modules help you reuse your code effectively.
Why Create Your Own Python Modules?
As you progress in Python programming, you’ll soon realize that creating custom Python modules becomes an invaluable part of your toolkit. Whether you’re working on personal projects or collaborating with a team, there are several advantages to writing your own modules. They’re not only handy for code reusability but also help you organize large projects in a cleaner, more efficient way. Let’s walk through why this matters, and why you should consider making custom modules a habit.
Advantages of Custom Python Modules
Creating your own Python modules offers multiple benefits that can elevate the quality of your code and simplify the way you work. Below are some key reasons to take the plunge into building custom modules.
Code Reusability
One of the biggest advantages of creating your own Python modules is reusability. Let’s say you’re working on several projects that require similar functionality—like data processing or file handling. Instead of rewriting the same code over and over, you can write it once in a module and use it wherever it’s needed. This saves time and reduces the risk of introducing errors from copying and pasting code across multiple files.
Here’s an example:
# data_validation.py (the module)
def validate_data(data):
if not data:
raise ValueError("No data provided!")
# Further validation logic here
return True
Now, instead of copying that function into every script, I can just import it like this:
# project_script.py
import data_validation
data = []
if data_validation.validate_data(data):
print("Data is valid")
Not only does this make your work more efficient, but it also helps ensure that your validation logic stays consistent across projects.
Modularity for Large Projects
If you’ve ever worked on a large project, you know how difficult it can be to manage all the code in one place. This is where modularity comes into play. Breaking your project into smaller, more manageable parts (modules) makes it easier to focus on individual components without losing track of the bigger picture.
Imagine a scenario where you’re building an e-commerce platform. You might have one module for user authentication, another for handling payments, and a separate one for product management. By splitting up the functionality into these focused modules, it becomes much easier to work on specific parts of the project, debug problems, and maintain the codebase over time.
Here’s a simplified view:

By organizing your project this way, not only do you reduce clutter, but you also create a logical structure that makes your code easier to understand and maintain, especially as the project grows.
Easy Collaboration Across Teams
Another huge benefit of creating your own Python modules is how they facilitate collaboration. When working in a team, everyone has different parts of the project to handle. By breaking the project into self-contained modules, team members can focus on their specific areas without stepping on each other’s toes.
From my experience working on a collaborative project, having modules allowed different team members to work independently on their pieces of the system. For example, while I was working on the data analysis module, someone else could focus on the user interface module without worrying about conflicts.
This method also makes it much easier to test individual parts of the project before integrating everything together. Each team member can create unit tests for their module, ensuring everything works correctly before merging it into the main codebase.
# Example of importing a module for collaboration
from user_management import signup
from payments import process_payment
# Call methods from different team members’ modules
signup.create_user("username", "password")
process_payment("credit_card_info")
By creating and organizing your own Python modules, you create clear boundaries between different parts of the project, making it much easier for others to collaborate.
Setting Up Your Python Development Environment
Before you jump into creating your own Python modules, it’s essential to set up your Python development environment correctly. Having the right tools and libraries makes a world of difference, whether you’re building simple scripts or more complex modules. I’ll walk you through how to install Python, choose the best IDE (Integrated Development Environment), and set up a virtual environment to ensure your module development goes smoothly.
Tools and Libraries for Module Creation
When you’re ready to start working on custom modules, having the right setup is key. Without a good environment, you might face issues that could slow down your development process. Here are the essential tools you’ll need:
- Python: Of course, the first step is installing Python itself.
- IDEs: You’ll need a good editor where you can write and organize your code easily. IDEs like VS Code or PyCharm are popular choices.
- Virtual Environment: This is crucial to keep your projects isolated, so each one uses its own set of dependencies.
In my experience, setting this up early on avoids a lot of headaches later, especially when working on multiple projects.
Installing Python
To create Python modules, the first step is to have Python installed on your computer. Head over to the official Python website at python.org and download the latest version of Python. It’s important to ensure you’re installing a version compatible with most modern libraries. Python 3.x is recommended for nearly all new projects.
Once the installation is complete, you can check if Python was installed correctly by opening your terminal (or command prompt) and typing:
python --version
You should see something like Python 3.10.5
(or whatever the latest version is at the time). This confirms that Python is successfully installed.
Best Tools and IDEs for Python Module Development
When it comes to writing and organizing Python code for module creation, choosing the right IDE can significantly boost your productivity. IDEs give you features like code auto-completion, error detection, and integrated terminal access. Let’s look at two of the most widely used Python IDEs.
VS Code
Visual Studio Code (VS Code) has quickly become a favorite among Python developers. It’s lightweight, fast, and can be customized with extensions to suit your workflow. When I first started using VS Code, I loved how easy it was to install extensions like Python linting and code formatting tools. Plus, the integrated terminal allows you to run Python commands without leaving the editor.
Setting up Python in VS Code is a breeze:
- Install VS Code from code.visualstudio.com.
- Install the Python extension for VS Code by Microsoft.
- Open a new Python file, and VS Code will prompt you to install the necessary tools like pylint or autopep8 for linting and formatting.
Here’s an example of how the interface might look once you have VS Code set up with Python:

VS Code’s intuitive interface helps you stay organized and focus on writing clean, efficient code.
PyCharm
PyCharm, developed by JetBrains, is another excellent option for Python module development. It’s a full-featured IDE that comes with integrated tools like version control, debugging, and even database access. PyCharm can be a bit heavier compared to VS Code, but it’s packed with features tailored for Python developers.
To start using PyCharm:
- Download PyCharm from jetbrains.com.
- Install the Community Edition (free) or Professional Edition (paid, but more features).
- Once installed, you can create a new project, and PyCharm will guide you through setting up your Python interpreter and environment.
For many developers working on larger projects or needing more advanced debugging and testing tools, PyCharm becomes the go-to IDE.
Creating a Simple Python Module
Step-by-Step Guide to Building a Python Module
Let’s get started by creating a simple Python module. We’ll cover everything from defining functions and classes to saving and importing the module into another script.
1. Defining Functions and Classes in Your Module
First, you need to create a file where you will define your functions and classes. Think of this file as a toolbox where you store your useful tools (functions and classes) for later use.
Here’s how to do it:
- Create a New File: Open your preferred text editor or IDE (like VS Code or PyCharm). Create a new file and save it with a
.py
extension. For instance, let’s call itmath_tools.py
. - Write Your Functions and Classes: In
math_tools.py
, you can start by defining some functions and classes. Here’s a simple example:
# math_tools.py
def add(a, b):
"""Add two numbers."""
return a + b
def subtract(a, b):
"""Subtract two numbers."""
return a - b
class Calculator:
"""A simple calculator class."""
def multiply(self, a, b):
"""Multiply two numbers."""
return a * b
def divide(self, a, b):
"""Divide two numbers."""
if b == 0:
raise ValueError("Cannot divide by zero!")
return a / b
In this module, we have two functions (add
and subtract
) and a class (Calculator
) with methods for multiplication and division. These are basic examples, but you can add as many functions and classes as needed.
2. Saving Your Python Module with .py Extension
After writing your code, save the file with a .py
extension. This tells Python that the file contains code that can be imported and used in other Python scripts.
For instance:
- File Name:
math_tools.py
- Location: Save it in a directory where you will later import it.
Ensure the file is saved in the same directory as the script where you plan to import it, or place it in a directory that is in your Python path.
3. Importing the Module into Another Script
Now that you have your math_tools.py
module ready, you can use it in other scripts. Importing a module allows you to access the functions and classes defined within it.
Here’s how you can import and use the math_tools
module:
- Create Another Script: In the same directory, create a new Python file, say
main.py
. - Import the Module: In
main.py
, you can import themath_tools
module and use its functions and classes like so:
# main.py
# Import the module
import math_tools
# Use the functions and classes
result1 = math_tools.add(10, 5)
result2 = math_tools.subtract(10, 5)
calc = math_tools.Calculator()
result3 = calc.multiply(10, 5)
result4 = calc.divide(10, 2)
print(f"Addition: {result1}")
print(f"Subtraction: {result2}")
print(f"Multiplication: {result3}")
print(f"Division: {result4}")
When you run main.py
, you should see the output from the functions and class methods:
Addition: 15
Subtraction: 5
Multiplication: 50
Division: 5.0
This demonstrates how to access the add
and subtract
functions as well as the Calculator
class from the math_tools
module.
Organizing Your Python Code into Packages
When working on larger Python projects, you’ll quickly realize that keeping your code organized becomes crucial. One of the best ways to manage multiple modules is by organizing them into packages. This approach not only keeps your project tidy but also makes it easier to manage and scale. In this guide, I’ll walk you through the process of organizing Python modules into packages. We’ll cover the folder structure, creating the __init__.py
file, and importing modules from a package.
Understanding Python Packages
A Python package is essentially a directory that contains multiple modules and a special file called __init__.py
. Packages help in organizing related modules into a single directory, making it easier to manage and maintain your codebase.
Think of a package like a folder in your computer that holds several files related to a specific project. In Python, this “folder” can also contain subfolders (which are also packages) and modules (files with Python code). This hierarchical structure helps in keeping the codebase clean and structured.
Folder Structure of Python Packages
To create a Python package, you’ll need to follow a specific folder structure. Here’s a simple example to illustrate this:
- Create a Main Package Directory: This directory will contain all your modules and sub-packages.
- Add Modules and Sub-Packages: Inside this directory, you can add multiple modules and additional directories for sub-packages.
- Include an
__init__.py
File: This file indicates that the directory should be treated as a package. It can be empty or contain initialization code.
Here’s a visual representation of the folder structure:
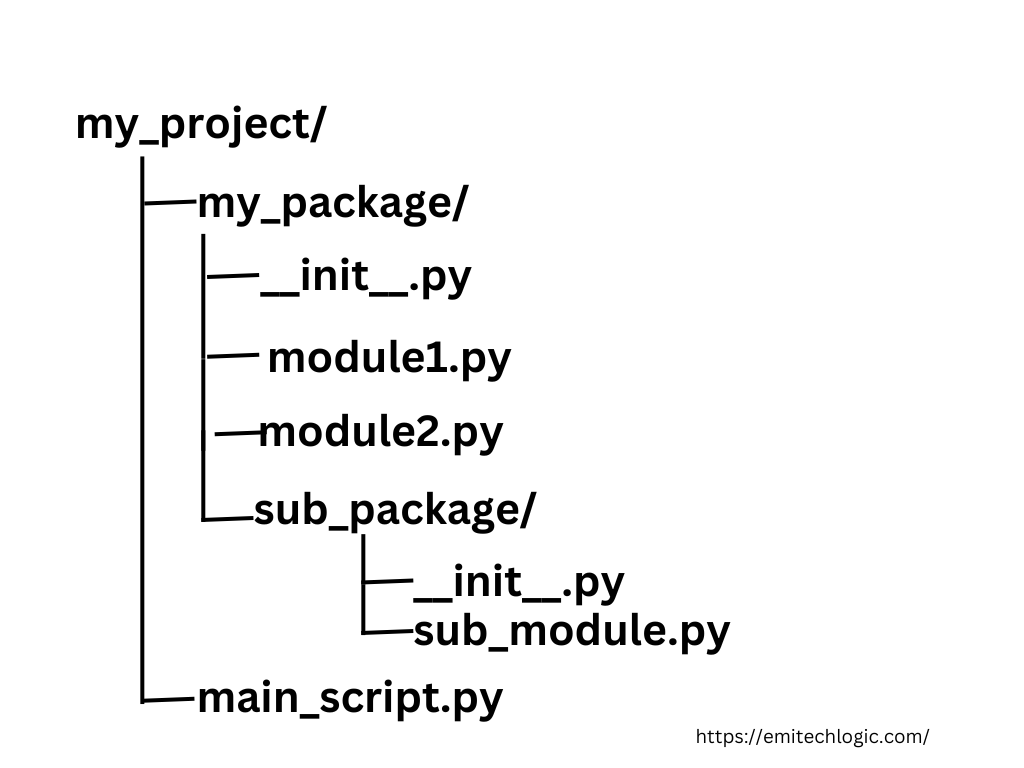
Importing Modules in Python: Best Practices
Importing modules effectively is crucial for writing clean and maintainable Python code. Whether you’re working on a small script or a large project, understanding how to import modules correctly will help keep your code organized and avoid common pitfalls. In this guide, we’ll explore how to import custom Python modules effectively, covering the different ways to import modules, the syntax for custom modules, and how to handle namespace conflicts using aliases.
Using import
, from ... import
, and Aliases
Python provides several ways to import modules and functions, each suited to different needs. Let’s explore these methods in detail:
1. Using import
The import
statement is the most common way to bring in a module. When you use this approach, you import the entire module, and you access its functions and classes using dot notation.
Example:
# Import the entire module
import math
# Use functions from the math module
result = math.sqrt(16)
print(result) # Output: 4.0
In this example, math.sqrt(16)
accesses the sqrt
function from the math
module. This approach is straightforward and works well when you need to use multiple functions or classes from a module.
2. Using from ... import
If you only need specific functions or classes from a module, you can use the from ... import
syntax. This method allows you to import only what you need, which can make your code cleaner and reduce the amount of typing required.
Example:
# Import specific functions from the math module
from math import sqrt, pi
# Use the imported functions directly
result = sqrt(25)
print(result) # Output: 5.0
print(pi) # Output: 3.141592653589793
Here, sqrt
and pi
are imported directly from the math
module, so you can use them without needing the math.
prefix.
3. Using Aliases
Aliases allow you to rename a module or function during import. This is especially useful for long module names or to avoid conflicts with existing names in your code.
Example:
# Import a module with an alias
import numpy as np
# Use the alias to access functions
array = np.array([1, 2, 3])
print(array) # Output: [1 2 3]
In this example, numpy
is imported as np
, which is shorter and often used in the community. This can make your code more concise and readable.
Syntax for Importing Custom Modules
When you create custom modules, you can use the same import statements to bring them into your scripts. Here’s how you can do it:
- Assuming you have a module named
my_module.py
with a functiongreet
:
# my_module.py
def greet(name):
return f"Hello, {name}!"
2. To import and use this module in another script:
# main.py
import my_module
message = my_module.greet("Alice")
print(message) # Output: Hello, Alice!
This example shows how to import the entire my_module
and use its greet
function.
3. If you want to import only the greet
function directly:
# main.py
from my_module import greet
message = greet("Bob")
print(message) # Output: Hello, Bob!
Here, only the greet
function is imported, so you don’t need to prefix it with my_module.
.
Handling Namespace Conflicts with Aliases
When importing multiple modules that have functions or classes with the same name, you can use aliases to avoid conflicts.
Example:
Suppose you have two modules, module1.py
and module2.py
, both containing a function named process
.
# module1.py
def process(data):
return f"Processing {data} in module1"
# module2.py
def process(data):
return f"Processing {data} in module2"
In your main script, you can use aliases to distinguish between these functions:
# main.py
import module1 as m1
import module2 as m2
result1 = m1.process("data1")
result2 = m2.process("data2")
print(result1) # Output: Processing data1 in module1
print(result2) # Output: Processing data2 in module2
Using aliases (m1
and m2
) ensures that you can clearly identify which process
function you are calling.
Must Read
- How to Return Multiple Values from a Function in Python
- Parameter Passing Techniques in Python: A Complete Guide
- A Complete Guide to Python Function Arguments
- How to Create and Use Functions in Python
- Find All Divisors of a Number in Python
Handling Module Dependencies and Imports
When working on Python projects, one of the challenges you might face is managing dependencies between your modules. This becomes even trickier when you encounter circular imports, a common issue that can disrupt your code. In this article, we’ll walk through what circular imports are, why they occur, and most importantly, how to resolve circular import issues in Python modules.
What Are Circular Imports?
Circular imports happen when two or more Python modules depend on each other. In other words, module A imports module B, and module B, in turn, imports module A. This can create an infinite loop that prevents Python from successfully loading the modules.
Circular imports often result in an error like this:
ImportError: cannot import name 'X' from partially initialized module 'moduleA' (most likely due to a circular import)
This error occurs because when Python tries to import module A, it sees that module A is trying to import module B, but module B also needs module A to complete its import. Python can’t complete the import process, and it throws an error.
How to Fix Circular Import Errors
When it comes to resolving circular import issues in Python, there are a few methods that can help you avoid or fix these errors. Let’s break them down with examples to make things clearer.
1. Refactor Your Code to Avoid Circular Imports
One of the most effective ways to fix circular imports is by refactoring your code. Often, circular imports indicate that your modules are too dependent on each other. You can reorganize your code so that dependencies flow in one direction.
For instance, instead of having both moduleA
and moduleB
depend on each other, you can create a new module, moduleC
, that handles the shared functionality.
Example:
# moduleA.py
from moduleC import common_function
def func_a():
return common_function() + " from moduleA"
# moduleB.py
from moduleC import common_function
def func_b():
return common_function() + " from moduleB"
# moduleC.py (new module)
def common_function():
return "This is a common function"
By moving the common functionality into a separate module, you eliminate the circular dependency between moduleA
and moduleB
.
2. Use Local Imports
Another method to resolve circular import issues is by using local imports instead of top-level imports. In Python, when you place an import statement inside a function or a method, the module is only imported when the function is called. This delays the import until it’s necessary, preventing circular import errors.
Example:
# moduleA.py
def func_a():
from moduleB import func_b # Local import
return func_b() + " from moduleA"
# moduleB.py
def func_b():
from moduleA import func_a # Local import
return func_a() + " from moduleB"
In this example, instead of importing moduleB
at the top of moduleA
(and vice versa), the import is placed inside the function. This prevents the import loop because moduleB
is only imported when func_a
is called.
3. Break the Circular Import with Conditional Imports
Sometimes, refactoring or local imports might not be possible. In such cases, you can use conditional imports to control when certain modules are imported. Conditional imports are useful when you only need certain functionality in specific cases.
Example:
# moduleA.py
def func_a():
if condition:
import moduleB # Conditional import
return moduleB.func_b()
return "No import needed"
In this example, moduleB
is only imported if a certain condition is met. This can help you avoid circular import issues while keeping your code flexible.
4. Use Importlib for Dynamic Imports
If you encounter complex circular dependencies that can’t be easily solved with local or conditional imports, you can use the importlib module to perform dynamic imports. This gives you finer control over when and how imports are handled.
Example:
# moduleA.py
import importlib
def func_a():
moduleB = importlib.import_module('moduleB')
return moduleB.func_b() + " from moduleA"
In this case, importlib.import_module
dynamically imports moduleB
during the execution of func_a
. This method is particularly useful when you need to delay imports in more sophisticated use cases.
Writing Readable and Maintainable Python Modules
1. Using Docstrings for Functions and Classes
One of the best ways to ensure your code is clear and easy to understand is by using docstrings for your functions and classes. A docstring is a brief explanation of what a function or class does, and it’s placed right below the function or class definition. Docstrings help other developers—and even your future self—quickly understand the purpose of your code.
Here’s a simple example:
def add_numbers(a, b):
"""
Adds two numbers and returns the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of the two numbers.
"""
return a + b
In this example, the docstring provides a concise explanation of what the function does, what arguments it expects, and what it returns. This small addition can make a huge difference when you or someone else needs to quickly grasp the functionality of your code.
2. Commenting and Organizing Your Code
Writing clean code also means commenting where necessary. However, it’s important to strike a balance: comment enough to clarify, but don’t overwhelm your code with unnecessary details. Use comments to explain why you’re doing something, especially if it’s not immediately obvious.
Here’s an example of good commenting practice:
def process_data(data):
"""
Processes the input data by normalizing and filtering it.
"""
# Normalize the data to a 0-1 range
normalized_data = [x / max(data) for x in data]
# Filter out any values below 0.1
filtered_data = [x for x in normalized_data if x > 0.1]
return filtered_data
In this case, the comments provide context about the two key steps: normalizing the data and filtering it. The comments clarify the intention behind each operation, making the function easier to understand and maintain.
3. Following the PEP 8 Style Guide
For Python, PEP 8 is the go-to style guide. It lays out conventions for writing Python code that is both consistent and easy to read. While it’s not mandatory to follow every single rule in PEP 8, adhering to most of its guidelines will make your code cleaner and easier to maintain.
Here are some of the most important tips from PEP 8:
- Indentation: Use 4 spaces per indentation level (no tabs!).
- Line Length: Keep your lines of code under 79 characters.
- Blank Lines: Use blank lines to separate functions and classes, as well as between blocks of code inside functions to improve readability.
- Naming Conventions: Use descriptive names for variables, functions, and classes. For example, use
calculate_average
instead ofcalc_avg
.
Here’s a quick example of code that follows PEP 8 conventions:
class DataProcessor:
"""
A class used to process and filter data.
"""
def __init__(self, data):
self.data = data
def normalize(self):
"""Normalizes the data to a 0-1 range."""
return [x / max(self.data) for x in self.data]
def filter(self, threshold=0.1):
"""Filters out values below the threshold."""
normalized_data = self.normalize()
return [x for x in normalized_data if x > threshold]
In this code, the class and function names are descriptive, there are docstrings explaining the purpose of each method, and the code structure follows PEP 8 guidelines. Following these conventions makes the code more readable and ensures it adheres to common Python standards.
Distributing Your Python Module
At some point, you might want to share your Python module with the world or simply make it available to colleagues or a wider community. The process of packaging and distributing a Python module can seem tricky, but it’s a rewarding step. Whether you’re an experienced developer or just starting out, publishing your code on PyPI (Python Package Index) can help others use and benefit from your work.
In this guide, we’ll cover everything from setting up your module to distributing it on PyPI, including creating the essential setup.py
file, using setuptools for packaging, and uploading your project online.
1. Creating a setup.py
File
The setup.py
file is crucial because it defines how your Python module will be packaged. This file contains all the metadata for your project—like its name, version, description, and dependencies. It’s essentially the “recipe” for how your module is built and distributed.
Here’s a basic example of a setup.py
file:
from setuptools import setup, find_packages
setup(
name='my_cool_module', # Replace with your module's name
version='0.1',
description='A cool Python module for doing cool things',
author='Your Name',
author_email='your.email@example.com',
packages=find_packages(), # Automatically find and include all packages
install_requires=[
'numpy', # List dependencies here
'requests'
],
)
In this example, the setup.py
file does a few important things:
name
: The name of your module (as it will appear on PyPI).version
: The version number of your module. It’s important to follow semantic versioning like0.1
,1.0
, etc.description
: A brief description of what your module does.author
andauthor_email
: Information about who created the module.packages
: This usesfind_packages()
to automatically include all packages in your module.install_requires
: Here, you list any dependencies that your module needs to run, likenumpy
orrequests
.
Adding this setup.py
file is the first step toward packaging your module for distribution.
2. Using setuptools
for Module Packaging
Once your setup.py
file is ready, the next step is to package your module using setuptools. Setuptools is the standard tool for packaging Python projects, and it simplifies the whole process.
You can install setuptools with:
pip install setuptools
After installing, you can run the following command in the same directory as your setup.py
file to build your module:
python setup.py sdist
This command creates a source distribution of your module, which packages everything up into a .tar.gz
file. This file contains all the code and resources that others will download.
Next, you’ll want to test your package to ensure it installs correctly. You can install your package locally by running:
pip install .
If everything works, you’re ready to upload your package to PyPI!
3. Uploading Your Module to PyPI
Now comes the exciting part—uploading your module to PyPI, where others can discover and install it. PyPI is the official repository for Python modules, and it’s as simple as running a few commands.
But first, you’ll need to create an account on PyPI. Once you have an account, follow these steps:
Step 1: Install twine
twine
is a tool that securely uploads your package to PyPI. Install it using:
pip install twine
Step 2: Upload your package
Now, run the following command to upload your package to PyPI:
twine upload dist/*
This command looks in the dist/
folder (created when you ran python setup.py sdist
) and uploads the package to PyPI. You’ll be prompted for your PyPI credentials, and once it’s done, your package will be live on PyPI!
To install your package, others can now use:
pip install my_cool_module
Example: Publishing a Simple Python Module
Let’s walk through a real example of packaging and distributing a simple module:
- Create a module: Create a file called
cool_math.py
with a simple function:
def add_numbers(a, b):
return a + b
2. Create a setup.py
: In the same directory, add the following setup.py
:
from setuptools import setup, find_packages
setup(
name='cool_math',
version='0.1',
description='A module for basic math operations',
author='Your Name',
author_email='your.email@example.com',
packages=find_packages(),
install_requires=[],
)
3. Package the module: Run:
python setup.py sdist
4. Upload to PyPI: After installing twine
, upload the module:
twine upload dist/*
Now, your simple math module is available for anyone to install with pip
!
Latest Advancements in Python Module Creation
With the release of Python 3.11, we’ve seen some exciting new developments that have made creating Python modules even more efficient and fun. The new features in Python 3.11 not only improve performance but also enhance modularity, allowing developers to write cleaner, more maintainable code.
Let’s explore what’s new in Python 3.11 for module creation, how the introduction of type hints and pattern matching impacts development, and finally, we’ll cover some best practices for testing your modules using unittest
and pytest
.
1. Exploring Python 3.11 and its Impact on Modules
One of the major focuses of Python 3.11 is performance improvements, which are particularly noticeable when dealing with large modules or applications. Faster startup times and reduced memory usage mean that importing modules and executing them is quicker, which makes a difference when working on bigger projects.
Here are a few key features from Python 3.11 that directly affect module creation:
a. Performance Improvements
Python 3.11 introduces several under-the-hood changes that significantly boost the execution speed of Python programs. This includes optimized bytecode and interpreter improvements, which means your modules now run faster without any extra effort from your side.
Let’s say you’ve written a module that handles some data processing:
def process_data(data):
result = []
for item in data:
result.append(item * 2)
return result
In Python 3.11, the performance of even basic loops like this one has been enhanced. This means your module’s functions will execute faster, especially when dealing with large datasets.
b. Use of Typing and Type Hints for Better Modularity
Python has been gradually introducing type hints since version 3.5, but in Python 3.11, the use of type hints has become more powerful and flexible. Type hints improve the modularity of your code by making it easier for others (or yourself) to understand what types are expected in functions and classes.
Here’s an example of how you can use typing to make your module more maintainable:
from typing import List
def process_numbers(numbers: List[int]) -> List[int]:
return [n * 2 for n in numbers]
By explicitly stating that process_numbers
takes a list of integers and returns a list of integers, you reduce potential bugs and make your module easier to use.
c. Pattern Matching
A feature that caught everyone’s attention in Python 3.11 is pattern matching. It allows for clearer and more readable code, especially in cases where you need to check for multiple conditions. This is a game-changer for module development, as it simplifies the logic in many use cases.
Here’s an example where pattern matching can enhance the readability of a function in a module:
def process_value(value):
match value:
case int():
return value * 2
case str():
return value.upper()
case _:
return "Unsupported type"
By using pattern matching, you can handle different input types more cleanly and efficiently, making your modules more modular and easier to maintain.
Testing Your Python Modules
Testing is a critical part of module development, especially if you’re aiming to distribute your module or use it in larger applications. Python provides built-in tools like unittest
and popular third-party libraries like pytest
to make writing and running tests easy.
Unit Testing for Python Modules
Unit tests focus on testing individual functions or components of your module. By testing each function in isolation, you can ensure that everything works as expected without relying on other parts of the code. Let’s walk through the two most common ways to test Python modules.
Writing Tests Using unittest
and pytest
Both unittest
and pytest
are excellent tools for testing Python modules, but they have slightly different philosophies. unittest
comes built-in with Python and is great for those who want a simple, no-frills testing solution. On the other hand, pytest
provides more flexibility and is often preferred by developers who want a cleaner syntax and additional features.
a. Writing Tests with unittest
Here’s a simple example of testing a module with unittest
:
import unittest
from my_module import process_data
class TestProcessData(unittest.TestCase):
def test_process_data(self):
self.assertEqual(process_data([1, 2, 3]), [2, 4, 6])
if __name__ == '__main__':
unittest.main()
This example shows how to test the process_data
function. You define a class that inherits from unittest.TestCase
and then write individual test methods that check the output of your functions. In this case, we’re checking if process_data([1, 2, 3])
returns [2, 4, 6]
.
b. Writing Tests with pytest
For those who prefer pytest
, here’s how you can write a similar test:
from my_module import process_data
def test_process_data():
assert process_data([1, 2, 3]) == [2, 4, 6]
The syntax with pytest
is cleaner, and you don’t need to inherit from any class. Additionally, pytest
offers more powerful tools for parametrizing tests, handling fixtures, and generating detailed reports.
Version Control for Python Modules
Managing versions and collaborating effectively on your Python modules is essential when working on long-term projects or with multiple contributors. Git and GitHub are two tools that help you do just that—track changes, collaborate with others, and automate tasks like testing. If you’re serious about creating a Python module, learning how to use Git for version control is a must.
1. Using Git and GitHub for Module Development
At its core, Git is a version control system that allows you to keep track of every change made to your code. Whether you’re working alone or with others, it ensures that nothing gets lost, and you can always go back to a previous version if something breaks.
Here’s a brief guide on how to use Git and GitHub for managing your Python module development:
a. Creating a Repository for Your Module
The first step is to create a GitHub repository for your module. A repository is essentially a folder where all the files, commits, and changes related to your project are stored.
- Initialize Git locally: If your project isn’t already a Git repository, you can initialize it by running the following command inside your project folder:
git init
2. Create a GitHub repository: On GitHub, create a new repository. This is where your module will live in the cloud. After creating it, connect your local repository to the GitHub one by running:
git remote add origin https://github.com/username/repository.git
b. Committing, Pushing, and Collaborating with Git
Once your repository is set up, the next steps involve committing your changes and pushing them to GitHub.
- Commit your changes: Whenever you’ve made significant updates to your module, you can commit those changes with a descriptive message. For example:
git add .
git commit -m "Added new function for processing data"
2. Push changes to GitHub: After committing, you’ll push the changes to GitHub where other collaborators can see them:
git push origin main
Collaboration becomes easier using GitHub because it allows multiple people to work on the same codebase simultaneously, without stepping on each other’s toes.
2. Using GitHub Actions for Automated Testing
One of the most powerful features of GitHub is GitHub Actions. This is a CI/CD (continuous integration/continuous deployment) tool that allows you to automate tasks like testing your module every time you make a commit.
You can create a simple action for testing your Python module whenever changes are pushed:
name: Python Package
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: 3.x
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install pytest
- name: Run tests
run: |
pytest
This YAML file sets up an automated process that runs tests on every push, ensuring that any new code doesn’t break your module.
Optimizing and Debugging Python Modules
Developing a Python module often involves more than just writing functional code. Debugging and optimizing are critical steps to ensure your module runs efficiently and error-free. Knowing how to debug and optimize Python modules will save you a lot of headaches later in the development process.
1. Profiling and Debugging Python Modules
When your code isn’t working as expected, or it’s running too slowly, debugging and profiling tools come to the rescue. Profiling helps identify performance bottlenecks, while debugging tools allow you to trace and fix issues in your code.
a. Using pdb and Other Debugging Tools
The built-in pdb (Python Debugger) tool is a simple but effective way to step through your code, inspect variables, and find out exactly where things go wrong.
Here’s a basic example of using pdb:
import pdb
def process_data(data):
pdb.set_trace() # Debugger will start here
result = []
for item in data:
result.append(item * 2)
return result
process_data([1, 2, 3])
By adding pdb.set_trace()
at the point where you want the debugger to start, you can step through the code line by line. You’ll be able to examine the value of variables and see exactly how your program is executing.
b. Other Debugging Tools
While pdb is great, there are other tools like ipdb (a richer version of pdb) or IDE-based debuggers in tools like PyCharm or Visual Studio Code. These offer more visual interfaces for debugging, making the process even smoother.
2. Profiling for Performance Bottlenecks
If your module is running slower than expected, profiling it can help you understand why. Python’s cProfile module is a great tool for identifying slow parts of your code.
Here’s how you can profile your Python module:
python -m cProfile my_module.py
This will output detailed statistics about how much time each function in your module takes to execute, helping you identify which parts of the code need optimization.
For example, if you discover that a certain loop is taking up most of the runtime, you can refactor or optimize that section for better performance.
Best Practices for Documentation in Python Modules
Documentation is crucial for any Python module. It not only helps others understand how to use your module but also aids you in maintaining and extending it over time. Proper documentation can make the difference between a module that’s widely adopted and one that’s barely used.
Here’s a guide on how to create effective documentation for Python modules and common mistakes to avoid.
Documenting Python Modules with Sphinx
Sphinx is a powerful tool for generating documentation. It converts reStructuredText files into HTML, PDF, and other formats, making it easier to create and maintain comprehensive documentation for your Python modules.
Generating Documentation with Sphinx
To get started with Sphinx, follow these steps:
- Install Sphinx: You can install Sphinx using pip:
pip install sphinx
2. Set Up Your Documentation: In your project directory, run:
sphinx-quickstart
This command will guide you through creating the initial setup for your documentation. You’ll be asked a series of questions to configure your documentation, such as the project name and author.
Write Your Documentation: Sphinx uses reStructuredText to write documentation. Create .rst
files in the docs
directory that include descriptions of your module’s functions, classes, and methods.
Example of documenting a function:
.. function:: add_numbers(a, b)
Adds two numbers together.
:param a: The first number.
:param b: The second number.
:return: The sum of the two numbers.
Build Your Documentation: To generate the documentation, run:
make html
This will create HTML files that can be viewed in a web browser, making it easy to share your documentation with others.
Writing README Files for Module Users
A well-written README file is often the first thing users see when they explore your module. It should provide a concise overview of your module, installation instructions, usage examples, and how to contribute.
Here’s a basic structure for a README file:
- Project Title: A clear and concise title for your module.
- Description: What does your module do? Why is it useful?
- Installation: Instructions on how to install your module. For example:
pip install your_module
4. Usage Examples: Show how to use your module with some sample code:
from your_module import add_numbers
result = add_numbers(5, 3)
print(result) # Output: 8
5. Contributing: If you’re open to contributions, provide guidelines on how others can contribute to your project.
6. License: Mention the licensing terms for your module.
Common Mistakes to Avoid When Creating Python Modules
Creating a Python module involves more than just writing code. Avoiding common pitfalls can save you from headaches later. Here are some common mistakes to avoid when building Python modules:
1. Overcomplicating Code Structure
It’s tempting to build complex structures to handle every possible scenario, but this can make your module harder to understand and maintain. Keep your module simple and focus on solving a specific problem efficiently.
For example, instead of creating multiple classes for a single function, use a simple approach if it suits the problem. Here’s a simplified example:
# Complex approach
class DataProcessor:
def __init__(self, data):
self.data = data
def process(self):
# Complex processing
pass
# Simpler approach
def process_data(data):
# Simple processing
return data
2. Neglecting Testing and Documentation
Skipping testing and documentation is a common mistake. Without tests, you can’t be sure that your module works as expected, and poor documentation will make it difficult for users to understand and use your module.
Make it a habit to write unit tests for your functions and methods. Use tools like unittest or pytest to automate testing. Similarly, keep your documentation up-to-date and comprehensive.
3. Ignoring Namespace Collisions
Namespace collisions occur when two modules or packages use the same names for functions, classes, or variables. This can lead to unexpected behavior and bugs that are hard to trace.
To avoid this, use clear and descriptive names for your functions and classes. If you’re importing functions from multiple modules, consider using aliases to prevent name clashes.
For example:
import module_a as a
import module_b as b
result_a = a.function()
result_b = b.function()
This helps avoid confusion and keeps your code organized.
Conclusion: Building Efficient Python Modules
Creating Python modules is a journey that combines thoughtful design, clear documentation, and ongoing maintenance. As we wrap up our exploration of building efficient Python modules, let’s reflect on the key insights and takeaways.
Summary and Final Thoughts
Building Python modules is not just about writing functional code; it’s about crafting components that are reusable, maintainable, and scalable. Here’s a summary of what we’ve covered:
- Creating and Organizing Modules: From defining simple functions to organizing code into packages, we’ve seen how to structure Python modules effectively. This organization helps in managing complex projects and improves code readability.
- Documentation and Testing: Proper documentation using tools like Sphinx and writing clear README files are essential for making your module accessible and user-friendly. Testing with frameworks such as unittest and pytest ensures that your module performs as expected and helps catch potential issues early.
- Version Control: Using Git and GitHub for version control aids in tracking changes, collaborating with others, and managing different versions of your module. This practice keeps your development process organized and transparent.
- Optimization and Debugging: Profiling and debugging are crucial for improving performance and fixing issues in your modules. Tools like pdb for debugging and various profiling techniques help ensure that your code runs efficiently.
- Avoiding Common Mistakes: Awareness of common pitfalls, such as overcomplicating code or neglecting testing, is vital for developing reliable modules. Clear code structure and comprehensive documentation can prevent many issues.
Importance of Modular Code for Scalability
Modular code forms the backbone of scalable software development. By breaking down a project into smaller, manageable modules, you can work on individual components independently, making it easier to expand and maintain your codebase. This modularity not only enhances code organization but also facilitates collaboration among team members, as each module can be developed and tested separately.
Encouraging Experimentation with Python Module Creation
The world of Python modules is rich with opportunities for innovation and creativity. Don’t be afraid to experiment with new ideas and techniques. Create custom modules, explore advanced features, and contribute to open-source projects. Each experiment will deepen your understanding and improve your coding skills.
Key Takeaways for Creating Python Modules
- Design with Clarity: Focus on creating clear, concise, and reusable code. Good module design simplifies maintenance and enhances usability.
- Document Thoroughly: Comprehensive documentation makes your module easier to use and understand. Use tools like Sphinx to generate professional-quality documentation.
- Test Rigorously: Implement robust testing to ensure your module functions correctly. Automated tests help catch bugs early and maintain code quality.
- Use Version Control: Manage your module’s development and track changes effectively using Git and GitHub.
- Optimize and Debug: Continuously profile and debug your code to improve performance and resolve issues.
Building efficient Python modules requires a blend of good practices, attention to detail, and a willingness to learn and adapt. By applying these principles, you’ll create modules that are not only functional but also robust and scalable. Keep exploring and experimenting, and you’ll find that creating Python modules can be both a rewarding and exciting endeavor.
FAQs
A Python module is a file containing Python code that can define functions, classes, and variables. It allows you to organize and reuse code efficiently.
To create a basic Python module, write your code in a .py
file. For example, save a file as mymodule.py
with functions and classes defined inside. You can then import this module into other scripts using the import
statement.
You can import functions from your Python module using the from module_name import function_name
syntax. For example, from mymodule import myfunction
imports myfunction
from mymodule
.
__init__.py
file in a Python package? The __init__.py
file is used to mark a directory as a Python package. It can also initialize package-level variables or execute package initialization code.
Leave a Reply