Introduction
If you use Python, you’ve likely heard a lot about Python packages and PyPI. But what exactly are they. And why are they so important? Understanding how Python packages work and how the Python Package Index (PyPI) helps you manage them. It is key to becoming more confident in your Python programming journey.
Think of Python packages like neatly packed toolkits that save you time and effort. PyPI? That’s like the ultimate library where you can find thousands of these packages. Which are ready for you to install and use in your projects.
In this guide, we’ll break down everything you need to know—what Python packages are, how they work, and how to get the most out of PyPI. Whether you’re looking to install a popular package with pip, upload your own to PyPI, or even troubleshoot common issues, we’ve got you covered. Plus, we’ll take a look at some of the latest updates in Python packaging, so you’re always in the loop with the newest advancements.
By the end of this post, you’ll not only understand how to use Python packages like a pro but also feel confident exploring the vast library of PyPI. Ready to level up your Python skills? Let’s get started!
Understanding Python Packages: An Essential Component of Python Programming
What is a Python Package?
When you’re working on Python projects, packages help you organize your code in a clean, logical way. A Python package is essentially a collection of Python modules bundled together. A module, on the other hand, is just a single Python file containing code that you can reuse. But when your project grows, you might have several modules working together. That’s where a package comes into play—it’s like a folder that holds your modules and makes your code more manageable.
Think of it this way: Instead of having one giant file with all your code, you can split it into smaller, manageable files (modules), and then use a package to keep them organized. It’s like organizing a drawer full of tools into separate boxes so you can easily find what you need.
Definition and Structure of Python Packages
A Python package is simply a directory (or folder) that contains modules along with an additional file called __init__.py
. This special file lets Python know that the folder should be treated as a package. Without it, Python won’t recognize the folder as a package.
Let’s look at the structure of Python packages for beginners with an example:

In this example:
- my_project/ is the root folder of the project.
- my_package/ is the package that contains two modules:
module_one.py
andmodule_two.py
. - The
__init__.py
file allows the code in bothmodule_one.py
andmodule_two.py
to be used as part ofmy_package
.
Inside your main.py
, you can import and use these modules like this:
from my_package import module_one, module_two
module_one.some_function()
module_two.another_function()
This structure makes it easy to write modular code in Python, which keeps everything organized and easier to maintain, especially in larger projects.
Differences Between Python Modules and Packages
People often get confused between modules and packages, so let’s break it down simply:
- A module is a single Python file. For example,
module_one.py
is a module. - A package, on the other hand, is a directory that contains multiple modules and the
__init__.py
file. A package allows you to group related modules together, so you can work on bigger projects without everything getting messy.
To sum up, if a module is like a tool, a package is the toolbox that holds those tools together.
Advantages of Using Packages in Python Projects
Why bother using packages in Python? Let’s explore some of the key advantages:
- Organization: With packages, you can easily group related modules together. This keeps your project folder neat and organized.
- Modularity: When your code is split into smaller parts (modules), it becomes easier to test, debug, and reuse. You don’t need to search through a huge file to find the code you’re looking for. You can simply work on a specific module.
- Collaboration: If you’re working with a team, it’s easier for each team member to handle different parts of the project when the code is organized into packages.
- Reusability: Once you’ve created a package, it can be reused across multiple projects. It’s like having a library of tools ready to go.
For example, if you write a package for handling user authentication, you can reuse that package in any future project that needs authentication. This saves time and ensures consistency across projects.
Let’s take a quick example. Say you’re writing a package to manage user authentication, like logging in and logging out:

Now, in your main application, you could import the package and use its functions like this:
from auth_package import login, logout
login.login_user("username", "password")
logout.logout_user("username")
How Python Packages Help in Code Reusability and Maintenance
As you dive deeper into Python, one of the first things you’ll appreciate is how Python packages contribute to code reusability and make maintaining code so much easier. Whether you’re working on small scripts or large-scale applications, Python packages can save you from repeating yourself and help you keep your code clean and well-structured.
Let’s break this down step by step so it’s easier to understand.
Code Reusability Through Python Packages
When we talk about code reusability, we’re referring to the ability to use existing code in multiple places without having to rewrite it. Python packages make this possible by allowing you to group together useful functions, classes, or entire modules and reuse them whenever needed.
Here’s a quick example to illustrate this:
Let’s say you wrote a module for calculating tax on products in one of your projects. Without using a package, you might find yourself copying and pasting that tax calculation code into every new project that requires it. That’s not only time-consuming but also error-prone. Instead, you could bundle the tax calculation code into a package and reuse it in any project.
Example:

In calculate.py
:
def calculate_tax(price, tax_rate):
return price + (price * tax_rate)
Now, in any future project where you need to calculate tax, you can import this package instead of rewriting the entire logic. For example, in another project:
from tax_calculator import calculate
total_price = calculate.calculate_tax(100, 0.05)
print(total_price)
This way, the Python package keeps your tax calculation logic reusable across multiple projects. By organizing your code into packages, you’re saving yourself from rewriting code—making your life easier.
How Packages Allow Developers to Avoid Rewriting Code
Once you create a package, it becomes an asset. You can import it into any new project as long as the functionality it provides is still relevant. This avoids the headache of rewriting the same code multiple times and eliminates potential errors from copying code across projects.
For example, if you’re building several projects that involve user authentication (like logging in and out), you might find yourself repeatedly writing similar code. By creating a user authentication package, you could simply reuse it every time you need it.
Example:

In auth.py
:
def login(username, password):
# Authentication logic
print(f"User {username} logged in.")
def logout(username):
# Logout logic
print(f"User {username} logged out.")
Now, in your future projects, instead of writing the login/logout logic over and over, you can simply import and use it:
from auth_package import auth
auth.login("JohnDoe", "password123")
auth.logout("JohnDoe")
By using this method, you’re avoiding code duplication and focusing on building new features rather than rewriting old ones.
Improving Code Maintenance with Python Packages
One of the biggest challenges developers face is maintaining and updating their code over time. As projects grow, it can become hard to keep track of where specific functions are or how they interact with other parts of the project. Maintaining Python code using packages helps solve this problem by organizing code into logical, manageable pieces.
With packages, you can update one module without worrying about breaking other parts of the project. For example, if you need to update the tax calculation logic, you can make that change inside the package, and all projects that use it will automatically benefit from the update.
Example:
Suppose you need to change the tax rate calculation from the original logic in our tax_calculator
package. Instead of going into every project and updating it, you just need to change it in the package:
def calculate_tax(price, tax_rate):
return price * (1 + tax_rate) # New logic
After this small update, any project using this package will now use the updated tax calculation without you having to modify each individual project. Packages make maintaining code simpler and help you avoid bugs that come from inconsistent updates.
Step-by-Step Guide to Creating a Python Package
Here’s a step-by-step guide to creating a Python package from scratch:

- Create the Package Directory: First, create a directory for your package. This will be the root of your package where all your modules will reside.Example:
mkdir math_operations
cd math_operations
2. Add the __init__.py
File: Inside your package directory, create the __init__.py
file. This file tells Python that the directory should be treated as a package. It can be left empty, or you can add initialization code.
Example:
touch __init__.py
3. Create Modules: Now, add your Python files (modules) inside the package. These files will contain the functions, classes, or variables you want to include.
Example:
touch addition.py
touch subtraction.py
4. Write Your Code: Inside each module, write the necessary functions or classes that will be part of your package.
Example (inside addition.py
):
def add(a, b):
return a + b
5. Test Your Package: After setting up your package, create a Python script outside of the package directory to test it.
Example:
from math_operations import addition
print(addition.add(2, 3)) # Output: 5
6. Package Distribution (Optional): If you want to share your package with others or use it across different projects, you can upload it to PyPI (Python Package Index). But this is an optional step for most personal projects.
Naming Conventions and Best Practices
When creating a Python package, following naming conventions and best practices is essential to keep your code readable and maintainable.
- Use lowercase letters and underscores for naming your package and modules. For example,
math_operations
is a better name thanMathOperations
. - Keep your package name descriptive yet concise. It should reflect the purpose of the package.
- Avoid common names that could clash with other packages or standard libraries. For instance, avoid naming your package something generic like
math
.
Another important best practice is keeping your code modular. Each module inside your package should focus on one specific functionality. This makes it easier to debug, update, and reuse.
Adding Metadata to Your Package
When you want to distribute your package or share it with others, it’s important to include metadata so others know what your package does. This is done by creating a setup.py
file. This file contains all the information about your package, like the version, author, and description.
Here’s a basic setup.py
example:
from setuptools import setup, find_packages
setup(
name="math_operations",
version="0.1",
packages=find_packages(),
description="A simple package for basic math operations",
author="Your Name",
author_email="your.email@example.com",
)
- name: The name of your package.
- version: The current version of your package.
- packages: A list of modules or packages included.
- description: A short explanation of what your package does.
- author and author_email: Information about you, the package creator.
By adding this metadata, you’re giving your package context, which becomes essential if you’re uploading it to a platform like PyPI.
Must Read
- AI Pulse Weekly: December 2024 – Latest AI Trends and Innovations
- Can Google’s Quantum Chip Willow Crack Bitcoin’s Encryption? Here’s the Truth
- How to Handle Missing Values in Data Science
- Top Data Science Skills You Must Master in 2025
- How to Automating Data Cleaning with PyCaret
Getting Started with PyPI: Your First Steps
If you’ve been developing in Python for a while, you’ve probably heard of PyPI (Python Package Index). It’s the hub where the Python community shares, distributes, and installs packages. Whether you’re working on a small personal project or a large application, understanding the role of PyPI in Python development can make your life easier. In this guide, we’ll take you through the basics of getting started with PyPI and show you why it’s the go-to source for Python packages.
What is the Role of PyPI in Python Development?
To put it simply, PyPI acts like a marketplace for Python packages. It’s where developers can upload their packages and where others can download and install them. Think of it as a giant library filled with pre-built tools that can help you speed up your Python development. Instead of reinventing the wheel, you can search for packages that already do what you need and incorporate them into your project.
Example:
Let’s say you’re building a web app and need a way to handle HTTP requests. Instead of writing your own HTTP request functions from scratch, you can install the widely-used requests package from PyPI with a single command:
pip install requests
Now, you can use this package to handle requests in your app without needing to write the code yourself.
Why PyPI is the Go-To Source for Python Packages
For many Python developers, PyPI is an essential part of the development process. It’s the largest repository of Python packages, offering solutions for nearly every problem you can imagine. Whether you’re looking for a package to help with web scraping, data analysis, or even machine learning, PyPI is where you’ll find it.
Some key reasons PyPI stands out include:
- Accessibility: PyPI is open to everyone, and you can access it easily using the
pip
command. - Variety: With over 400,000 packages available, you’ll rarely need to write your own solution from scratch.
- Community-driven: Many packages on PyPI are created, maintained, and improved by the Python community.
Understanding the Role of PyPI in Python Development
When it comes to understanding the role of PyPI in Python development, it’s important to see how it fits into the broader ecosystem. Python’s simplicity and readability are major reasons for its popularity, but PyPI extends its power by allowing developers to share reusable packages. The collaborative nature of PyPI makes Python a great language for both beginners and seasoned developers. Newcomers can easily find packages to learn from, while experienced coders can contribute their own packages, building on each other’s work.
How PyPI Facilitates Sharing and Installing Python Packages
One of the main features of PyPI is how easy it makes sharing and installing Python packages. Let’s walk through both of these processes.
Sharing a Python Package on PyPI
If you’ve created a package you’d like to share with others, PyPI makes distribution a breeze. Here are the basic steps:
- Package Your Code: First, ensure your package is structured properly (with an
__init__.py
file) and create asetup.py
file with the necessary metadata, as we discussed in a previous section. - Register on PyPI: Sign up for a free account on PyPI.
- Upload Your Package: Use
twine
(a utility that helps in uploading) to upload your package to PyPI:
twine upload dist/*
4. Make It Available to the World: Once uploaded, anyone can install your package using pip
.
Installing a Package from PyPI
For users, installing a package from PyPI is as simple as opening a terminal and running the following command:
pip install package_name
For example, if you want to install the NumPy package, which is widely used for numerical computations:
pip install numpy
In a few seconds, the package is downloaded, and you can immediately start using it in your code.
Exploring the PyPI Interface: Searching and Downloading Packages
The PyPI website (https://pypi.org/) has a simple interface that lets you search for packages, read documentation, and see the latest releases. Let’s break down some key features of the PyPI interface:
- Search Bar: The most common way to find packages. If you’re looking for a specific package, just type its name in the search bar.
- Package Details: Each package has a page with useful information like installation instructions, documentation, and a list of recent versions. This helps you decide whether the package fits your needs.
- Download Statistics: PyPI shows the number of times a package has been downloaded, giving you a sense of how popular or reliable a package might be.
How to Install Python Packages Using pip
If you’re new to Python development, you’ve probably heard about pip — the tool that makes installing Python packages a breeze. It’s the package manager for Python and is essential for anyone who wants to work with external libraries. This guide will show you how to use pip to install Python packages from PyPI and handle upgrades, removals, and dependencies with ease.
Using pip to Install Python Packages from PyPI
At its core, pip is your gateway to the vast world of Python libraries available on the Python Package Index (PyPI). With just a few simple commands, you can bring a wealth of functionality into your project.
Example:
Let’s say you want to install requests, one of the most popular Python libraries for making HTTP requests. Here’s the command you’d use:
pip install requests
This command will download the latest version of the requests
package from PyPI and install it in your Python environment. After that, you can easily import it into your script:
import requests
response = requests.get('https://example.com')
print(response.status_code)
And just like that, you’ve added powerful functionality to your project without needing to write the HTTP handling code yourself.
How to Use pip to Install Python Packages from PyPI
When it comes to how to use pip to install Python packages from PyPI, it’s not just about adding new libraries. It’s also about managing them effectively. The pip command makes it easy to keep everything up-to-date and working together smoothly in your Python project.
Upgrading and Removing Packages with pip
As your project evolves, you’ll likely need to upgrade some of your packages to newer versions or even remove those you no longer need. pip handles both tasks with ease.
Upgrading Packages
You can upgrade a package to its latest version with a simple command:
pip install --upgrade package_name
For example, if you wanted to upgrade the NumPy package:
pip install --upgrade numpy
This will download the latest release of the package from PyPI and update it in your project. It’s important to stay on top of upgrades, especially for security updates and bug fixes.
Removing Packages
If you no longer need a package in your project, you can remove it with:
pip uninstall package_name
For example, to remove NumPy:
pip uninstall numpy
This ensures your project stays clean and lightweight, without unnecessary libraries cluttering your environment.
Managing Dependencies in Python Projects
One of the best things about using pip is how it manages your project’s dependencies. Dependencies are other libraries your package needs to work correctly. When you install a package using pip, it automatically installs all the required dependencies, saving you a ton of manual work.
Example:
Let’s go back to the requests package. When you install requests
, pip checks if there are any additional packages it relies on. If so, it will install those automatically. This ensures that your package works right out of the box without needing to manually track down and install its dependencies.
Dependency Management with requirements.txt
As your project grows, managing these dependencies can become a bit overwhelming. This is where the requirements.txt
file comes in handy. It’s a simple text file where you list all the packages and their versions that your project depends on.
To create a requirements.txt
file, you can use the following command:
pip freeze > requirements.txt
This will generate a list of all the packages installed in your environment along with their versions. Your file might look something like this:
requests==2.25.1
numpy==1.21.0
pandas==1.3.1
Later, if you or someone else wants to set up the project in a different environment, you can install all the necessary dependencies with one command:
pip install -r requirements.txt
This command reads the requirements.txt
file and installs all the listed packages in one go, making it incredibly easy to manage complex projects.
Diagram: pip workflow
Here’s a quick diagram to illustrate how pip works:

This simple workflow shows how pip not only installs the package you request but also handles its dependencies.
How to Upload Your Python Package to PyPI
Uploading your Python package to PyPI (Python Package Index) is a significant milestone in your development journey. It’s not just about sharing your work with others; it’s about contributing to the Python community and making your project easily accessible to other developers. This guide will walk you through the step-by-step process of publishing your package on PyPI, from preparing your package to successfully uploading it using Twine.
Preparing Your Package for Distribution
Before you can upload your package to PyPI, it needs to be properly prepared for distribution. This process involves organizing the files, writing the necessary metadata, and making sure everything is in order.
Step 1: Organizing Your Package
Your package should follow a clear structure. A basic package might look like this:

- The
__init__.py
file is essential for Python to recognize your directory as a package. setup.py
is the most important file for distribution. It contains metadata about your package, such as the name, version, author, and dependencies.
Step 2: Writing setup.py
Your setup.py
file provides critical information about your package to PyPI. Here’s a simple example of what it might look like:
from setuptools import setup, find_packages
setup(
name="my_package",
version="0.1",
author="Your Name",
author_email="your.email@example.com",
description="A short description of the package",
long_description=open('README.md').read(),
long_description_content_type="text/markdown",
url="https://github.com/yourname/my_package",
packages=find_packages(),
install_requires=[
'requests',
],
)
In this example:
name
: The name of your package as it will appear on PyPI.version
: The current version of your package.author
andauthor_email
: Information about the creator of the package.install_requires
: Any dependencies your package needs.
Creating a PyPI Account
Before you can upload your package, you’ll need an account on PyPI. Creating one is straightforward:
- Go to PyPI and sign up.
- After verifying your email, you can log in and start publishing your packages.
Using Twine to Upload Your Package
Once your package is prepared, the next step is uploading it to PyPI. This is where Twine comes in — a Python utility that makes it easy to publish your package. Here’s how you can do it:

Step 1: Install Twine
If you don’t have Twine installed yet, you can do so with this command:
pip install twine
Step 2: Build Your Package
Before uploading, you’ll need to create distribution files. Run the following command to build the package:
python setup.py sdist bdist_wheel
This will generate the necessary files in a directory called dist/
.
Step 3: Uploading Python Packages Using Twine
Now it’s time to upload your package. Run this command:
twine upload dist/*
Twine will prompt you to enter your PyPI username and password. Once authenticated, your package will be uploaded to PyPI, and it will be available for everyone to download and use.
Publishing a Python Package on PyPI
The process of publishing a Python package on PyPI using Twine is relatively simple but very powerful. It enables you to share your work with other developers around the world, ensuring that your package can be easily installed with a single pip command.
Common Mistakes to Avoid When Uploading a Python Package
While the process seems straightforward, there are a few common mistakes that developers encounter when uploading packages to PyPI. Let’s look at some of them and how to avoid them:
1. Errors in Package Metadata
A lot of beginners miss the importance of filling out the metadata correctly in the setup.py
file. Omitting important fields like the license or the version number can lead to upload failures or, worse, confusion for users.
Tip: Always double-check your setup.py
to ensure all the required fields are filled in.
2. Incorrect Package Structure
Another common mistake is having the wrong package structure, which can make the package unusable once installed. Be sure to include a __init__.py
file in every package directory, even if it’s empty.
3. Missing README or License File
Your package should include a README and a LICENSE file. The README file provides an overview of your project, and the license ensures that others know how they’re allowed to use your code.
Tip: PyPI displays the content of your README.md on your package’s page, so make sure it’s clear and informative.
Example of a Good README.md
# My Package
My Package is a Python library that simplifies HTTP requests.
## Installation
You can install the package using pip:
```bash
pip install my_package
Usage
import my_package
my_package.do_something()
### Diagram: Package Upload Process
Here’s a simple diagram of the steps involved in uploading a Python package to PyPI:

Latest Advancements in PyPI and Python Package Management (2023 Updates)
Python Package Index (PyPI) plays a crucial role in Python development, being the go-to repository for developers to share and install packages. With Python growing rapidly, PyPI has also evolved to meet the needs of the community. In 2023, we’ve seen new features and security enhancements introduced, making Python package management even smoother and safer. This article will explore these updates, including Poetry for managing dependencies, pipx for isolated installations, and the latest security features in PyPI for Python packages.
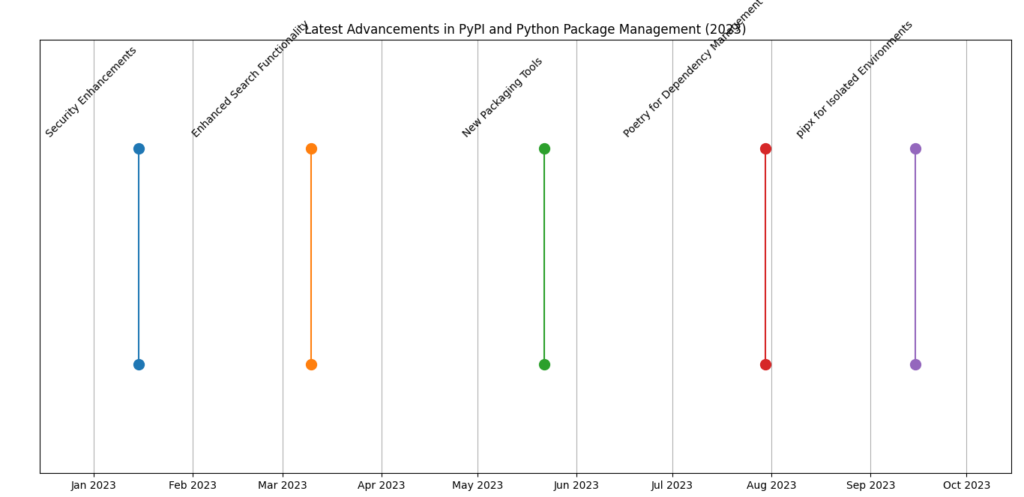
New Features and Enhancements in PyPI
PyPI continues to refine its features to support developers. In 2023, PyPI introduced several enhancements that make it easier for developers to upload, search, and manage their packages.
Security Enhancements in PyPI
Security has always been a priority for PyPI, but with recent advancements, it has been significantly strengthened. One of the most notable updates is the two-factor authentication (2FA) requirement for all critical projects. This ensures that sensitive packages cannot be tampered with or hijacked, giving developers and users more peace of mind.
Latest security features in PyPI for Python packages
Some of the latest security features in PyPI for Python packages include:
- Mandatory 2FA for critical projects: If your package is widely used or essential, enabling two-factor authentication becomes a requirement.
- API tokens for package uploads: Instead of using basic credentials, you can now create API tokens that are safer and easier to manage.
- Dependency vulnerability warnings: PyPI warns developers if the packages they are depending on have known security vulnerabilities.
Enhanced Package Search Functionality
New search options in PyPI
Searching for the right Python package has always been an essential part of the PyPI experience. In 2023, PyPI enhanced its search options, making it easier for developers to find the most relevant packages. These new search options in PyPI include:
- Improved filters: You can now filter by metadata such as project license, Python version compatibility, and more.
- Relevance ranking: PyPI’s search engine now uses better algorithms to ensure that the most relevant results appear at the top.
- Advanced keyword suggestions: As you type, PyPI now offers smarter suggestions to help you narrow down your search.
For example, if you’re searching for a package that works with a specific Python version, you can now easily filter results to show only those compatible packages.
Example: Searching for a Package
Suppose you’re working on a project that needs a library compatible with Python 3.10. In PyPI’s new interface, you can simply enter the package name and filter by Python version, saving you time.
New Python Packaging Tools and Libraries
In 2023, some new tools have emerged in the Python packaging ecosystem, making it easier to manage packages, dependencies, and isolated environments. Let’s take a look at two tools that are gaining popularity this year.
Poetry: For Better Dependency Management
How to use Poetry for Python package management
Poetry has been gaining traction as a more modern tool for managing Python projects and their dependencies. Unlike the traditional pip
and virtualenv
, Poetry simplifies the process of creating and managing your Python projects by:
- Handling both dependencies and virtual environments.
- Managing project metadata in a
pyproject.toml
file, reducing clutter. - Offering an easy way to publish your package to PyPI.
To install Poetry, you can run:
curl -sSL https://install.python-poetry.org | python3 -
Poetry is perfect for developers who want a clean, straightforward tool to manage both dependencies and package distribution. With its dependency resolution feature, you won’t run into conflicts as often as you might when using just pip
.
pipx: Installing Python Applications in Isolated Environments
While pip
is great for managing libraries, pipx comes into play when you want to install Python applications in isolated environments. This tool is a lifesaver if you need to install command-line tools without worrying about polluting your global Python environment.
pipx allows you to install and run Python applications in their own virtual environment, ensuring that they don’t interfere with your system’s packages.
To install pipx:
pip install pipx
Then, you can install Python applications with:
pipx install some-python-app
This tool is perfect for developers who frequently work with different Python applications and want to avoid version conflicts.
Upcoming Features in PyPI and Python Packaging
As Python continues to grow, PyPI and packaging tools are constantly evolving. Some exciting features are already on the horizon for 2024:
- Package metadata improvements: More comprehensive metadata to help users understand package dependencies better.
- Built-in vulnerability scanning: PyPI is planning to introduce automatic scanning for packages to detect vulnerabilities and provide warnings.
- Enhanced developer profiles: A new profile system that allows developers to showcase their work and contributions to the Python community.
Best Practices for Python Package Management
Managing Python packages effectively is a skill that every developer must master, especially when working on larger projects or distributing your code. Package management involves organizing dependencies, ensuring security, and maintaining consistency throughout a project. Following some best practices for Python package management can help make your development process smoother and avoid common pitfalls.
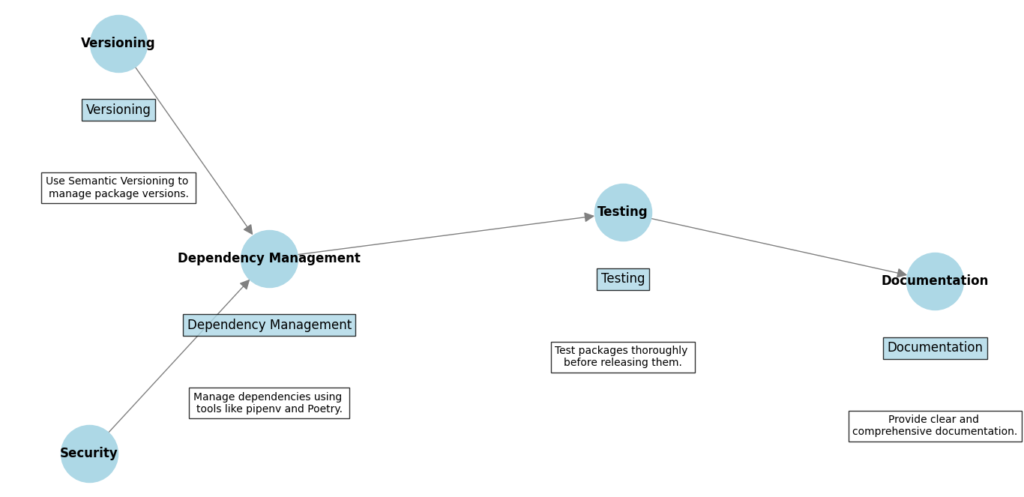
Versioning Your Python Packages Correctly
Versioning is the backbone of package management, helping you track changes, improvements, and bug fixes in your project. Without proper version control, managing multiple releases can quickly turn chaotic. The industry standard for Python packages is Semantic Versioning (also known as SemVer).
Semantic Versioning: A Detailed Explanation
Long-tail keyword: “how to version Python packages using semantic versioning”
Semantic versioning follows a three-number system, structured as MAJOR.MINOR.PATCH
. Here’s what each of these numbers represents:
- MAJOR: Increment this number when you make incompatible changes to your API.
- MINOR: Increase this number for adding new features in a backward-compatible manner.
- PATCH: Use this number to release backward-compatible bug fixes.
For example, if your package version is 2.1.4
, it means:
- Version
2
introduces some breaking changes compared to previous major releases. 1
means you’ve added features without breaking any existing functionality.4
indicates that four bug fixes have been applied since the last minor release.
Example: Let’s say you’re maintaining a package for handling data visualization. Your package was at version 1.0.0
. You add support for a new chart type, but without altering the way existing users interact with the package. You would then change the version to 1.1.0
. However, if you remove support for an old feature, breaking backward compatibility, the version would go up to 2.0.0
.
Ensuring Package Security: What Every Developer Should Know
When managing Python packages, security cannot be ignored. While open-source packages provide great flexibility, they can also introduce vulnerabilities if you’re not careful. Here are some essential best practices for ensuring security in Python packages.
Using Trusted Sources for Package Downloads
best practices for ensuring security in Python packages
Always use trusted sources like PyPI (Python Package Index) when downloading or installing Python packages. PyPI ensures a level of scrutiny and security that third-party sources may lack. To further protect yourself:
- Enable two-factor authentication (2FA) on your PyPI account to prevent unauthorized access.
- Use API tokens instead of username/password credentials for package uploads, making the process safer.
Verifying Package Integrity with Hashes
When installing packages, it’s a good idea to verify the integrity of the files you download. Many developers use hashes to ensure that the downloaded package hasn’t been tampered with. You can check the integrity of your package during installation using:
pip install <package-name> --hash=<hash-value>
This method adds an extra layer of security to your installations by ensuring the downloaded file matches the hash you expect.
Optimizing Python Projects with Dependency Management Tools
Managing dependencies in Python projects can become a hassle, especially when your project grows and includes multiple libraries. Tools like pipenv and Poetry simplify this process, ensuring that your dependencies are handled correctly without conflicts.
Using Virtual Environments to Isolate Project Dependencies
To avoid dependency clashes, it’s essential to use virtual environments. These virtual environments create isolated spaces where each project can have its own dependencies, separate from other projects. This is crucial when different projects require different versions of the same package.
For example, one project might require Flask v1.1.2
while another requires Flask v2.0.1
. Virtual environments make sure these packages don’t conflict.
You can create a virtual environment using venv
:
python3 -m venv my_project_env
source my_project_env/bin/activate
Tools like pipenv and Poetry for Dependency Management
Semantic keyword: “how pipenv and poetry improve dependency management”
Both pipenv and Poetry go beyond traditional virtual environments by providing more features to manage dependencies and streamline your project workflow.
- Pipenv is a popular tool that brings together
pip
andvirtualenv
, simplifying dependency installation and project management. With Pipenv, you can manage your Python projects’ dependencies, environment variables, and virtual environments in one place.
To install a package using Pipenv, simply run:
pipenv install <package-name>
Pipenv will handle the virtual environment creation and manage your Pipfile
for version control.
- Poetry, on the other hand, is considered by many to be a more modern solution, offering dependency management and package distribution all in one. Unlike
pip
orpipenv
, Poetry uses thepyproject.toml
file to define your project’s dependencies. This file ensures your project’s structure is clean, and dependency conflicts are less likely.
To install a package with Poetry:
poetry add <package-name>
Poetry resolves dependencies and locks versions, so you can avoid conflicts down the road.
Common Issues and Troubleshooting with Python Packages and PyPI
Managing Python packages and dealing with PyPI can sometimes be tricky, especially when unexpected errors or conflicts arise. Whether you’re working on a small project or managing a large-scale application, running into dependency conflicts, upload errors, or security vulnerabilities is not uncommon. Let’s break down some common issues and troubleshooting techniques to help you work through these challenges.

Resolving Dependency Conflicts in Python Projects
One of the most common issues developers face when managing Python packages is dependency conflicts. These occur when different libraries in your project require different versions of the same package, which can result in version clashes.
How to Handle Version Conflicts in Python Packages
troubleshooting dependency conflicts in Python projects
When two or more packages depend on different versions of a third-party library, it leads to a version conflict. For instance, if one package requires numpy==1.18
and another needs numpy==1.21
, this can cause compatibility issues. Here are some ways to resolve this:
- Virtual environments: By using virtual environments, you can create isolated spaces for each project, preventing dependency conflicts between projects.Example of setting up a virtual environment:
python -m venv my_env
source my_env/bin/activate
- Dependency management tools: Tools like pipenv and Poetry help resolve dependency conflicts by locking specific versions of packages. If you’re working on a larger project, Poetry is a great option to keep your dependencies in check.
For example, when using Poetry, it automatically resolves conflicts and creates a pyproject.toml
file to manage dependencies:
poetry add <package-name>
- Upgrading dependencies: If a conflict arises, you can try upgrading one or both conflicting packages to versions that work together. Tools like
pipdeptree
can help visualize your project’s dependency tree and identify conflicts:
pip install pipdeptree
pipdeptree
Fixing Issues When Uploading Packages to PyPI
If you’re uploading your Python package to PyPI for distribution, you might encounter some common errors. These errors typically relate to packaging, authentication, or metadata issues. Let’s look at how to fix them.
Common Upload Errors and Their Solutions
Semantic keyword: “how to fix common PyPI upload errors”
- Error: “400 Bad Request” This error often occurs due to an issue with your package’s metadata, such as an incorrect version number or missing required fields. Make sure your
setup.py
file is properly formatted and includes all necessary metadata. - Error: “403 Forbidden” If you see a 403 Forbidden error, it usually means that there is an issue with your authentication when uploading to PyPI. To fix this, make sure you’re using an API token instead of your PyPI password. API tokens are more secure and should be used in place of credentials.You can configure Twine to use an API token for uploads:
twine upload --repository pypi dist/* -u __token__ -p <your-token>
3. Error: “File already exists” This happens when the same version of a package has already been uploaded. You need to update your package version in setup.py
before uploading it again.
For example:
setup(
name='mypackage',
version='0.2.0', # update this version
...
)
4. Missing README or License File Ensure you include all necessary files, such as a README.md and a LICENSE file, as PyPI requires them. You can specify these files in your setup.py
using the long_description
argument:
with open("README.md", "r") as fh:
long_description = fh.read()
setup(
long_description=long_description,
long_description_content_type="text/markdown",
)
Handling Security Vulnerabilities in Third-Party Packages
Even with trusted packages, security vulnerabilities can creep in, especially in open-source libraries. Being proactive in managing these vulnerabilities is critical to maintaining a secure project.
What to Do If a Python Package Has a Vulnerability
how to manage security vulnerabilities in Python packages
- Stay updated: Regularly update your dependencies to the latest versions, as they often contain security patches for known vulnerabilities.
- Check vulnerability databases: Tools like Safety and Snyk allow you to scan your dependencies for known vulnerabilities.You can install and use Safety as follows:
pip install safety
safety check
This will scan your dependencies and notify you if any packages contain vulnerabilities.
- Consider alternatives: If a package you’re using has a vulnerability, and no fix is available, look for alternative libraries that provide similar functionality without the security risk.
Verifying Package Integrity with Hashes
When you install a package, verify its integrity by comparing its hash to a known value. This ensures the package hasn’t been tampered with. You can do this using pip
:
pip install <package-name> --hash=<hash-value>
This provides extra security, ensuring you’re installing the exact version of the package you expect.
Conclusion: Mastering Python Packages and PyPI for Efficient Python Development
Mastering Python package management and understanding PyPI are essential for anyone serious about Python programming. The ability to efficiently manage dependencies, upload your packages to PyPI, and troubleshoot common issues is critical for scaling projects and ensuring smooth development. Whether you’re handling version conflicts, securing your packages, or sharing your work with the community, having a strong grip on Python packages and PyPI tools will make your coding life much easier.
Why Understanding Python Packages and PyPI is Critical for Python Programmers
Understanding Python packages and the role of PyPI is more than just a nice-to-have skill; it’s a cornerstone of Python development. Packages allow you to reuse and share code, reducing the time and effort needed for repeated tasks. Meanwhile, PyPI provides the infrastructure to distribute and install these packages efficiently. Without mastering these, you may struggle with common issues like dependency conflicts, outdated libraries, or security vulnerabilities, all of which can slow down development.
By using tools like pip, Poetry, and pipenv, you gain control over dependencies, ensuring that your project is always running with compatible and secure packages. And when it comes to publishing your work, understanding how to upload packages to PyPI ensures your contributions are shared with the larger community, further establishing your presence in the Python ecosystem.
Future of PyPI and Python Package Management
Looking ahead, the future of PyPI and Python package management is bright, with continuous improvements in security features, search functionality, and dependency management tools. As Python grows in popularity, new tools like Poetry and pipx will continue to emerge, providing developers with even better ways to handle dependencies and package distribution. We’re already seeing enhanced security measures like API tokens and hash verification becoming the standard, ensuring safer downloads and installations.
In the coming years, expect PyPI to further improve its infrastructure with faster search capabilities, enhanced package metadata, and better tooling for handling complex project dependencies. These advancements will make Python development more efficient, secure, and scalable, allowing developers to focus more on writing great code and less on managing packages.
By staying up-to-date with the latest PyPI features and package management tools, you’ll be better equipped to handle the evolving needs of Python projects, ensuring that your development process remains both smooth and efficient.
FAQs
A Python package is essentially a collection of modules (Python files) that are grouped together in a directory. These modules can be reused across different projects, making it easier to organize and maintain code.
You can use the pip
tool to install a package from PyPI. For example, to install the popular requests
package, you can run:
pip install requests
PyPI (Python Package Index) is the official repository for Python packages. It allows developers to publish their packages and for others to install them easily, ensuring a streamlined way to share and manage code.
A module is a single Python file, while a package is a collection of multiple modules stored in a directory. Packages allow for better code organization, especially in larger projects.
To create your own package, you need to:Organize your code into a directory structure.
Add an __init__.py
file in your package directory (this can be empty).
Optionally, add a setup.py
file for easier distribution.
Once done, you can upload it to PyPI using tools like Twine.