Introduction to While Loops in Python
Now that you’ve gotten comfortable with for
loops in Python, it’s time to explore another essential loop: the while
loops. If you’re familiar with looping through a sequence a fixed number of times using for
loops, you might wonder when and why you’d use a while
loop instead.
What is a While Loops in Python?
A while
loop in Python is a control flow statement that allows you to execute a block of code repeatedly as long as a certain condition is true. Unlike for
loops, which iterate over a sequence (like a list or a range of numbers) a specific number of times, while
loops continue running until the condition you’ve set becomes false.
Here’s a simple analogy: Imagine you’re at the gym doing push-ups. You don’t have a set number of push-ups in mind; instead, you plan to keep going as long as you don’t feel too tired. The moment you feel exhausted, you stop. That’s how a while
loop works. You keep performing an action (like doing push-ups) until a condition (your energy level) tells you to stop.
Let’s see a basic while
loop in action:
# Example of a simple while loop
count = 0
while count < 5:
print("This is push-up number", count + 1)
count += 1
In this example, the loop will print out a message for each “push-up” until the count reaches 5. The loop checks the condition count < 5
, and as long as it’s true, the loop keeps running. Once count
hits 5, the condition is no longer true, and the loop stops.
Understanding the Importance of While Loops in Python Programming
You might be wondering, “Why not just use a for
loop for everything?” Well, the while
loop shines in scenarios where you don’t know in advance how many times you need to repeat an action. The flexibility of while
loops is especially useful when the number of iterations depends on factors that change during runtime.
For example, let’s say you’re building a simple guessing game where the user has to guess a number between 1 and 10. You don’t know how many guesses the user will need, so a while
loop is perfect here:
import random
number_to_guess = random.randint(1, 10)
user_guess = None
while user_guess != number_to_guess:
user_guess = int(input("Guess the number between 1 and 10: "))
if user_guess < number_to_guess:
print("Too low, try again!")
elif user_guess > number_to_guess:
print("Too high, try again!")
print("Congratulations! You guessed it right!")
In this game, the loop continues until the user guesses the correct number. Since we can’t predict how many attempts it will take, a while
loop is the ideal choice.
When and Why to Use a While Loop in Python
Choosing between a for
loop and a while
loop depends on your specific needs. Here are some scenarios where while
loops are particularly useful:
- Unknown Iterations: When the number of iterations isn’t fixed or predictable. For instance, looping until a user provides valid input, like in a menu-driven program.
- Waiting for a Condition: When you need to wait for something to happen or a condition to change before moving on. An example could be a program that keeps checking the status of a file download.
- Potentially Infinite Loops: When the condition for exiting the loop may not be met quickly, or when you want the loop to run indefinitely until a certain external condition is met. For example, a server that waits for incoming connections might use a
while True
loop.
Here’s a quick example of using a while True
loop to create an infinite loop that waits for a user to decide when to stop:
while True:
response = input("Type 'exit' to stop, or anything else to continue: ")
if response.lower() == 'exit':
break
print("You chose to continue.")
In this case, the loop keeps running until the user types “exit.” Using while True
is a common way to create a loop that could, in theory, run forever—unless interrupted by a specific condition.
Adding While Loops to Your Toolkit
As you continue learning Python, you’ll find that while
loops are a powerful tool, especially in situations where flexibility is key. They’re not just another way to repeat actions; they’re your go-to solution when the path ahead isn’t entirely clear.
Remember to be mindful of the conditions you set in your while
loops. An infinite loop can happen if the condition never becomes false, which can cause your program to hang or crash. It’s always a good idea to include a break
statement or some other fail-safe mechanism to exit the loop if needed.
Basic Syntax and Structure of While Loops in Python
Now that you’ve been introduced to the concept of while
loops in Python, let’s break down the syntax and structure so you can confidently write and understand these loops. As you explore Python, mastering the while
loop will become essential, especially when dealing with conditions that don’t have a predetermined number of iterations.

Explaining the Syntax of a While Loop in Python
The syntax of a while
loop in Python is quite simple. It consists of the while
keyword, followed by a condition, and then a block of code that will be executed as long as that condition remains true. Here’s what the basic structure looks like:
while condition:
# code block to be executed
Let’s break this down step-by-step:
while
Keyword: This tells Python that you’re about to start a loop that will continue running as long as the specified condition is true.- Condition: This is the heart of the loop. The condition is a boolean expression (something that evaluates to
True
orFalse
). As long as this condition isTrue
, the loop will keep running. - Code Block: This is the set of instructions that will be executed each time the loop runs. The code block is indented under the
while
statement, which is how Python knows which lines of code belong to the loop.
Here’s a simple example to illustrate:
counter = 0
while counter < 5:
print("Counter is at:", counter)
counter += 1
In this example, the loop will print the current value of counter
and then increase it by 1. The loop runs as long as counter
is less than 5. Once counter
hits 5, the condition counter < 5
becomes False
, and the loop stops.
How the While Loop Works: Step-by-Step Explanation
Understanding how a while
loop operates in practice is key to using it effectively in your programs. Let’s walk through the process step by step.
- Initial Condition Check: Before the loop starts, Python checks the condition. If the condition is
True
, the loop begins; if it’sFalse
, the loop is skipped entirely. - Executing the Code Block: If the condition is
True
, Python runs the indented code block under thewhile
statement. - Re-evaluating the Condition: After executing the code block, Python checks the condition again. If the condition is still
True
, the loop runs another iteration. If it’sFalse
, the loop exits. - Loop Continues Until Condition is False: This process repeats until the condition becomes
False
. When that happens, Python exits the loop and moves on to the next part of your program.
Here’s an example that shows how a while
loop continues running until a condition changes:
password = ""
while password != "EmiTechLogic":
password = input("Enter the password: ")
if password != "EmiTechLogic":
print("Incorrect password, please try again.")
print("Access granted!")
In this example, the loop continues asking for a password until the correct one is entered. The loop only stops when the condition password != "EmiTechLogic"
is no longer true.
Indentation Rules in Python While Loops
Indentation is crucial in Python, and nowhere is this more important than in loops. Unlike many other programming languages, Python uses indentation (spaces or tabs) to define the blocks of code. This means that every line of code inside a while
loop must be indented to show that it belongs to that loop.
Here’s what happens if you don’t indent correctly:
counter = 0
while counter < 3:
print("This will cause an error")
counter += 1
The code above will throw an IndentationError
because the print
statement is not indented under the while
loop. Python expects all the code within the loop to be indented.
When writing while
loops, it’s also important to maintain consistent indentation throughout your code. Whether you choose to use spaces or tabs, stick to one method to avoid errors.
Here’s a corrected version of the above example:
counter = 0
while counter < 3:
print("This is indented correctly")
counter += 1
In this example, the loop will run smoothly because the code is properly indented.
When I first started coding, I struggled with while
loops because I often found myself accidentally creating infinite loops. That’s when the importance of the condition really hit home. It’s essential to ensure that your condition will eventually become False
; otherwise, your loop will keep running forever.
For example, forgetting to increment a counter inside the loop is a common mistake:
counter = 0
while counter < 5:
print("This loop will run forever!")
In this case, counter
never increases, so counter < 5
is always true, leading to an infinite loop. To avoid this, I now double-check that my condition will change and eventually stop the loop.
As you work with while
loops, take your time to understand how the condition affects the loop. Play around with different examples, and don’t be afraid to make mistakes. That’s how you learn!
Must Read
- How to Design and Implement a Multi-Agent System: A Step-by-Step Guide
- The Ultimate Guide to Best AI Agent Frameworks for 2025
- Data Science: Top 10 Mathematical Definitions
- How AI Agents and Agentic AI Are Transforming Tech
- Top Chunking Strategies to Boost Your RAG System Performance
Examples of Using While Loops in Python
Now that you’re familiar with the syntax and structure of while
loops in Python, let’s explore some practical examples. These examples will help you understand how while
loops can be applied to solve real-world problems and avoid common pitfalls.
Simple While Loop Example: Counting from 1 to 10
Let’s start with a basic example: counting from 1 to 10. This is a great way to see how a while
loop works in action. The idea here is to use a counter that starts at 1 and increments by 1 with each iteration of the loop until it reaches 10.
Here’s how you can write this in Python:
counter = 1
while counter <= 10:
print(counter)
counter += 1
In this example:
counter = 1
: We initialize the counter variable to 1.while counter <= 10:
: The loop will keep running as long as the counter is less than or equal to 10.print(counter)
: This line prints the current value of the counter.counter += 1
: After printing, we increment the counter by 1.
What’s great about this example is that it’s easy to follow. You start with a number and keep increasing it until the condition counter <= 10
is no longer true. Once the counter hits 11, the loop stops, and the program moves on.
This is a classic use case for a while
loop, especially when you want to perform an action multiple times based on a condition that changes over time.
Using While Loops to Process User Input
While loops are particularly useful when dealing with user input, especially in cases where you don’t know how many times the user will interact with the program. Let’s look at an example where we use a while
loop to keep asking the user for input until they provide a valid response.
Here’s a scenario: you want the user to enter a positive number. If they enter a negative number or zero, you’ll keep asking them until they get it right.
number = int(input("Enter a positive number: "))
while number <= 0:
print("That's not a positive number!")
number = int(input("Please enter a positive number: "))
print(f"Thank you! You entered {number}.")
In this example:
- The program asks the user to enter a positive number.
- The
while
loop checks if the number is less than or equal to 0. If it is, the loop continues asking for input. - Once the user enters a positive number, the loop ends, and the program thanks the user.
This example highlights how while
loops can be effectively used to validate user input. It’s a practical way to ensure your program behaves correctly, even if the user makes a mistake.
Creating Infinite Loops with While Loops (and How to Avoid Them)
An infinite loop is a while
loop that never ends. While these loops can be useful in certain scenarios, like running a server that should always be active, they can also cause your program to hang or crash if you’re not careful.
Let’s first look at how you might accidentally create an infinite loop:
counter = 1
while counter <= 10:
print("This loop will never end!")
In this example, the loop will print “This loop will never end!” endlessly because there’s no code inside the loop to change the value of counter
. The condition counter <= 10
will always be true, so the loop keeps running forever.
To avoid infinite loops, always make sure the condition in your while
loop will eventually become False
. Here’s a fixed version of the loop above:
counter = 1
while counter <= 10:
print("This loop will end.")
counter += 1
Now, the loop will stop after printing “This loop will end.” ten times because the counter increases by 1 with each iteration, eventually making the condition counter <= 10
false.
Sometimes, infinite loops are intentional. For example, if you’re writing a program that should keep running until manually stopped, you might write something like this:
while True:
user_input = input("Type 'exit' to stop: ")
if user_input == 'exit':
break
In this example, the loop will continue running until the user types “exit.” The break
statement is used to exit the loop when the condition inside the loop is met.
Controlling the Flow of a While Loop
When working with while
loops in Python, there are moments when you might want to have more control over how the loop behaves. For instance, you may want to exit the loop before it completes all its iterations, skip over certain parts of the loop, or include a placeholder for future code. Python offers three useful statements for these situations: break
, continue
, and pass
.

Let’s break down each one with examples to see how they work and why they’re useful.
Using Break in While Loops to Exit Early
The break
statement is used to exit a loop prematurely. It’s particularly handy when you’ve achieved what you need, and there’s no reason to continue looping.
Imagine you’re searching for a specific number in a sequence, and once you find it, you want the loop to stop. Here’s how you can do that with a break
statement:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
target = 7
index = 0
while index < len(numbers):
if numbers[index] == target:
print(f"Found the target: {target} at index {index}")
break
index += 1
In this example:
- The
while
loop goes through each number in thenumbers
list. - If the number matches the
target
, the loop prints a message and immediately exits, thanks to thebreak
statement. - If the
break
statement wasn’t there, the loop would continue to run, even after finding the target.
Using break
makes your code more efficient by stopping the loop as soon as the desired outcome is achieved.
How to Use Continue in a While Loop to Skip Iterations
The continue
statement allows you to skip the rest of the current iteration and move on to the next one. This can be useful when you want to bypass certain conditions but still continue looping.
For example, let’s say you’re processing a list of numbers, but you want to skip over any negative numbers:
numbers = [5, -1, 8, -3, 10, -7]
index = 0
while index < len(numbers):
if numbers[index] < 0:
index += 1
continue
print(f"Processing number: {numbers[index]}")
index += 1
In this scenario:
- The
while
loop iterates over each number in thenumbers
list. - If the number is negative, the
continue
statement is triggered, which skips the print statement and immediately jumps to the next iteration. - Positive numbers are processed and printed, while negative ones are simply ignored.
This approach is useful when you want to exclude certain items or conditions from processing without stopping the loop entirely.
Understanding the Pass Statement in While Loops
The pass
statement might seem odd at first because it doesn’t do anything. However, it’s an important tool in Python, especially when you’re planning your code and need a placeholder for future logic.
Let’s say you’re writing a while
loop, but you’re not ready to implement the logic just yet. You can use pass
to avoid syntax errors:
index = 0
while index < 5:
pass # Logic to be added later
index += 1
In this example:
- The
pass
statement is there to fill the space where the loop logic will eventually go. - The loop will run as usual, but it won’t do anything until you replace
pass
with actual code.
I’ve found the pass
statement incredibly useful when I’m sketching out the structure of my code. It allows me to outline loops and functions without worrying about the details right away. As your program evolves, you can go back and replace pass
with the necessary logic.
The Else Clause in While Loops
While loops are an essential tool in Python, allowing you to repeat a block of code as long as a condition remains true. But did you know that you can also pair a while
loop with an else
clause? This feature isn’t just an add-on; it can enhance your code’s logic and readability, especially when you want to handle situations where a loop completes its iterations without any interruptions.
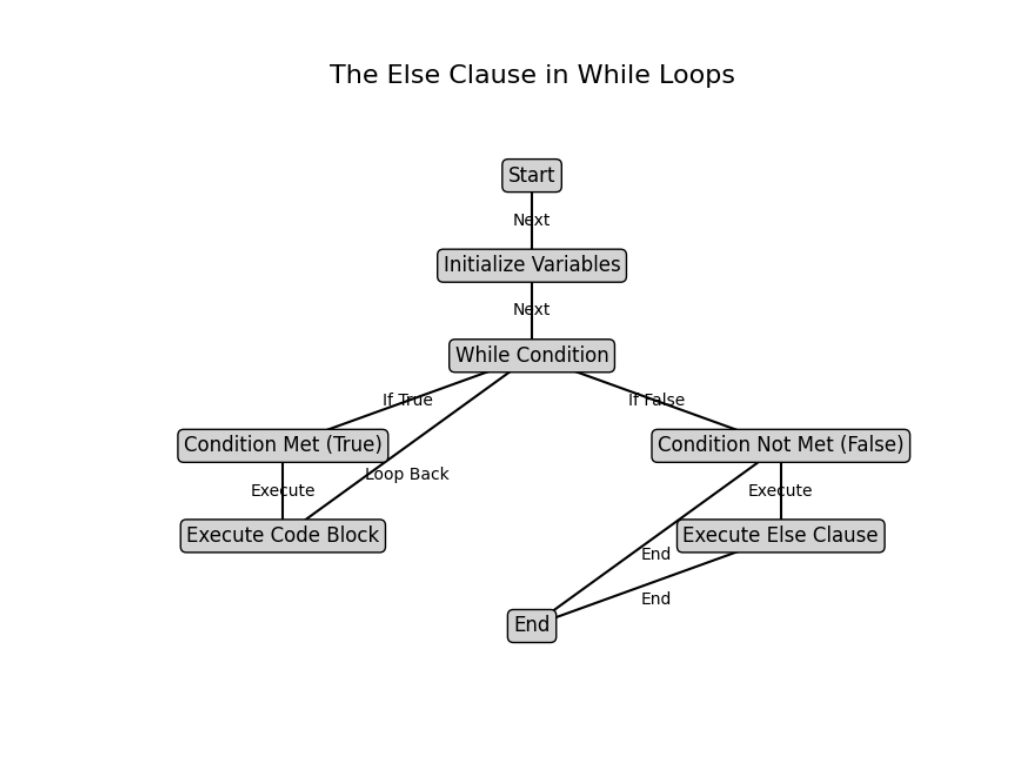
Let’s explore the else
clause in while
loops, why you might want to use it, and how it can make your code more effective.
Introduction to the Else Statement in While Loops
The else
statement in Python is typically associated with if
conditions. However, it also has a place in loops. When used with a while
loop, the else
clause will only execute if the loop completes all its iterations without hitting a break
statement.
This might sound a bit abstract, so let’s break it down with an example:
index = 0
while index < 5:
print(f"Index is now {index}")
index += 1
else:
print("The loop completed without interruption.")
In this code:
- The
while
loop runs as long asindex
is less than 5. - Each time the loop runs, the current value of
index
is printed, and thenindex
is incremented. - Once the loop finishes (when
index
reaches 5), theelse
clause is triggered, printing a message that confirms the loop completed normally.
If a break
statement had been used inside the loop, the else
clause would have been skipped entirely. This is the crucial difference: else
only executes if the loop wasn’t exited early.
When to Use Else with While Loops in Python
You might wonder when it makes sense to use an else
clause with a while
loop. In my experience, it’s particularly useful in scenarios where you’re searching for something within a loop, and you want to know if the loop ended naturally or if it was interrupted by a break
.
Let’s say you’re searching through a list to find a specific item. If the item is found, the loop should break immediately. If it isn’t found, you might want to notify the user that the item wasn’t present in the list. Here’s how you can do that:
numbers = [1, 3, 5, 7, 9]
target = 4
index = 0
while index < len(numbers):
if numbers[index] == target:
print(f"Found the target: {target}")
break
index += 1
else:
print(f"Target {target} not found in the list.")
In this example:
- The
while
loop searches fortarget
in thenumbers
list. - If the
target
is found, the loop prints a message and breaks out of the loop. - If the loop completes without finding the
target
, theelse
clause runs, letting you know the item wasn’t found.
Using an else
clause in this way helps to clearly separate the outcomes: what happens if the loop finds the item and what happens if it doesn’t. It makes your code easier to understand and maintain.
Practical Examples of Using Else with While Loops
To illustrate the else
clause further, let’s look at a few more practical examples.
1. Checking for Prime Numbers:
Let’s say you want to write a function that checks if a number is prime. You can use a while
loop with an else
clause to make the logic clear:
def is_prime(number):
if number < 2:
return False
divisor = 2
while divisor * divisor <= number:
if number % divisor == 0:
return False
divisor += 1
else:
return True
print(is_prime(11)) # Output: True
print(is_prime(10)) # Output: False
In this example:
- The
while
loop checks for divisors up to the square root ofnumber
. - If a divisor is found, the function returns
False
immediately. - If no divisors are found, the loop completes, and the
else
clause returnsTrue
, indicating that the number is prime.
2. Searching for a String in a File:
Imagine you’re searching through a text file for a specific keyword. If the keyword is found, you might want to stop searching and report the line number. If the keyword isn’t found after scanning the entire file, the else
clause can handle that scenario:
keyword = "Python"
line_number = 0
with open("example.txt", "r") as file:
while True:
line = file.readline()
if not line:
break
line_number += 1
if keyword in line:
print(f"Keyword found on line {line_number}")
break
else:
print("Keyword not found in the file.")
Here:
- The
while
loop reads the file line by line. - If the keyword is found in a line, the loop prints the line number and exits.
- If the loop reaches the end of the file without finding the keyword, the
else
clause prints a message.
This example demonstrates how the else
clause can provide a clear, logical way to handle different outcomes in a loop.
Nested While Loops in Python
Nested while loops are a concept that can add a layer of complexity to your Python programs but also unlock powerful possibilities. They allow you to create loops within loops, which can be incredibly useful in specific situations. But with great power comes the need for caution—nested loops can be tricky if not handled properly.
Let’s break down what nested while loops are, how they can be used effectively in real-world applications, and some common pitfalls to watch out for.

Understanding Nested While Loops: Concept and Use Cases
A nested while loop in Python simply means having a while loop inside another while loop. The inner loop will run entirely before the outer loop iterates to its next step. This can be useful when you need to perform repeated actions in multiple dimensions, like processing a grid or matrix of data.
Example: Nested While Loop for a Multiplication Table
Let’s say you want to generate a multiplication table for numbers 1 through 5. A nested while loop can help you do this:
i = 1
while i <= 5:
j = 1
while j <= 5:
print(f"{i} * {j} = {i * j}", end="\t")
j += 1
print() # Move to the next line after the inner loop completes
i += 1
In this example:
- The outer loop runs through the numbers 1 to 5 (
i
). - For each value of
i
, the inner loop also runs through numbers 1 to 5 (j
). - The result is a multiplication table where each combination of
i
andj
is calculated and printed.
This is just one example of how nested while loops can be used. They’re also common in scenarios like working with multi-dimensional arrays, nested data structures, or performing repetitive tasks that involve multiple layers.
Examples of Nested While Loops in Real-World Applications
Nested while loops are not just a theoretical concept—they are used in various real-world applications to solve complex problems. Here are a few scenarios where nested while loops can be particularly useful:
1. Processing Multi-Dimensional Data:
In data science, you often work with multi-dimensional arrays or matrices. For example, if you have a 2D array representing a grid of pixel values, you might need to process each pixel in the grid. Nested while loops make it possible to iterate over both the rows and columns of the grid.
Example:
image = [
[255, 0, 0],
[0, 255, 0],
[0, 0, 255]
]
row = 0
while row < len(image):
col = 0
while col < len(image[row]):
print(f"Pixel at ({row},{col}) has value {image[row][col]}")
col += 1
row += 1
In this case, the outer loop iterates over the rows, and the inner loop iterates over the columns, allowing you to access each pixel in the grid.
2. Building Complex Patterns:
Nested loops are also helpful when generating complex patterns or shapes. For instance, if you need to print a pattern of stars or numbers, a nested loop can simplify the process.
Example:
n = 5
i = 1
while i <= n:
j = 1
while j <= i:
print("*", end=" ")
j += 1
print()
i += 1
This code prints a right-angled triangle of stars. The outer loop controls the number of rows, while the inner loop controls the number of stars in each row.
3. Navigating Nested Data Structures:
When working with nested data structures like lists within lists or dictionaries within dictionaries, nested while loops can be essential. They allow you to drill down into each layer of the structure and process the data accordingly.
Common Pitfalls When Using Nested While Loops
While nested while loops can be powerful, they also come with potential pitfalls. If not used carefully, they can lead to problems such as infinite loops, performance issues, or code that’s difficult to read and maintain. Here are some common mistakes to watch out for:
1. Infinite Loops:
One of the most common issues with nested loops is accidentally creating an infinite loop. This can happen if the condition for ending the loop is not correctly set or if the loop variables are not updated as expected.
Example of a Potential Infinite Loop:
i = 1
while i <= 5:
j = 1
while j <= 5:
print(f"{i} * {j} = {i * j}")
# Forgot to update j, so the inner loop never ends
i += 1
In this example, the inner loop would run indefinitely because j
is never incremented. Always ensure that all loop variables are correctly updated within the loop.
2. Complexity and Readability:
Nested loops can quickly become complex, especially if you’re dealing with more than two levels of nesting. This can make your code harder to read and understand. It’s important to keep your loops as simple as possible and to add comments or break down complex logic into functions when necessary.
3. Performance Issues:
Nested loops can also lead to performance issues, particularly when dealing with large datasets. Since the inner loop runs for each iteration of the outer loop, the total number of iterations can grow quickly. In some cases, optimizing your logic or using more efficient algorithms may be necessary.
4. Use of Multiple Loop Variables:
When using nested loops, it’s common to use multiple loop variables (e.g., i
and j
). However, it’s crucial to ensure these variables don’t interfere with each other. It’s easy to accidentally overwrite a loop variable or use the wrong variable in a condition, leading to unexpected behavior.
Insights on Nested While Loops
In my experience, nested while loops are a double-edged sword. They can be incredibly useful for solving complex problems, but they can also make your code more complicated if not used carefully. I’ve found that the key to using nested loops effectively is to keep the logic as clear and simple as possible.
For instance, when I first started programming, I would sometimes get carried away with nesting loops to solve problems. But I quickly realized that this approach could lead to tangled, difficult-to-read code. Over time, I learned to ask myself whether a nested loop was really necessary or if there was a simpler way to achieve the same result.
In many cases, breaking the problem down into smaller functions or using more advanced data structures can help reduce the need for deep nesting. When nesting is necessary, I’ve found that carefully planning the logic and adding comments can make a big difference in keeping the code manageable.
Advanced Techniques with While Loops
While loops are a fundamental part of Python, offering a way to repeat a block of code as long as a condition is true. Once you’ve mastered the basics, you can explore more advanced techniques that allow you to create more complex and powerful loops. These advanced techniques include combining while loops with conditional statements, using while loops with complex conditions, and implementing time-dependent while loops in Python.

Let’s explore each of these techniques and see how they can be applied in real-world scenarios.
Combining While Loops with Conditional Statements
Combining while loops with conditional statements is a powerful way to control the flow of your loops. By using if
, elif
, and else
statements within a while loop, you can create more dynamic and responsive code. This approach is particularly useful when you need to handle different cases or conditions within the same loop.
Example: Password Validation
Let’s consider a situation where you want to validate a user’s password. You can use a while loop to repeatedly ask for the password until it meets certain criteria, and use conditional statements to check each criterion.
password = ""
while True:
password = input("Enter your password: ")
if len(password) < 8:
print("Password must be at least 8 characters long.")
elif not any(char.isdigit() for char in password):
print("Password must contain at least one digit.")
elif not any(char.isupper() for char in password):
print("Password must contain at least one uppercase letter.")
else:
print("Password is valid.")
break # Exit the loop when the password is valid
In this example:
- The while loop continues until the user enters a valid password.
- The conditional statements inside the loop check for specific password requirements, providing feedback if any are not met.
- Once all conditions are satisfied, the loop exits with the
break
statement.
This method allows you to handle multiple conditions in a single loop, making your code more efficient and easier to manage.
Using While Loops with Complex Conditions
While loops can also be used with more complex conditions, allowing you to create loops that respond to multiple factors simultaneously. Complex conditions might involve multiple variables, logical operators, or even nested conditions.
Example: User Authentication System
Imagine you’re building a user authentication system that locks an account after three failed login attempts. You can use a while loop with a complex condition to handle this.
In this example:
- The while loop runs as long as the user has made fewer than three attempts.
- The condition within the loop checks both the username and password. If either is incorrect, the loop continues, and the number of attempts is incremented.
- If the user fails three times, the loop ends, and the account is locked.
This approach showcases how complex conditions can be used within while loops to create more sophisticated logic.
Implementing Time-Dependent While Loops in Python
Sometimes, you might need a while loop that runs for a specific amount of time or until a certain time has passed. Implementing time-dependent while loops in Python involves using the time
module to control how long the loop runs.
Example: Countdown Timer
Let’s create a countdown timer that runs for a specified number of seconds.
import time
countdown = 10 # Countdown from 10 seconds
start_time = time.time()
while time.time() - start_time < countdown:
remaining_time = countdown - int(time.time() - start_time)
print(f"Time left: {remaining_time} seconds")
time.sleep(1) # Pause for 1 second
print("Time's up!")
In this example:
- The while loop runs as long as the difference between the current time and the start time is less than the countdown duration.
- The
time.sleep(1)
function pauses the loop for one second, creating a ticking effect. - Once the countdown reaches zero, the loop exits, and the program prints “Time’s up!”
Time-dependent loops are useful in scenarios like creating timers, scheduling tasks, or implementing real-time systems.
Advanced techniques with while loops can open up a world of possibilities in Python. By combining while loops with conditional statements, using complex conditions, and implementing time-dependent loops, you can create more sophisticated and dynamic programs.
As you continue to explore these techniques, remember to keep your code clear and test it thoroughly. With practice, you’ll find that these advanced methods become second nature, allowing you to tackle even more challenging programming tasks with confidence.
Avoiding Common Errors in While Loops
When learning about while loops in Python, it’s not uncommon to encounter some bumps along the way. These loops can be incredibly powerful, but they also come with their own set of challenges. From infinite loops to subtle bugs, while loops can be tricky to master. In this section, we’ll discuss some common mistakes to avoid, how to detect and fix infinite loops, and tips for debugging your while loops effectively.

Let’s explore these aspects in a way that’s easy to understand and apply in your own coding projects.
Common Mistakes to Avoid When Writing While Loops
While loops are simple in concept, but it’s easy to make mistakes, especially when you’re just starting out. Let’s look at some common pitfalls:
- Forgetting to Update the Condition: One of the most common mistakes is forgetting to update the variable that controls the loop condition. If the condition never changes, the loop can run indefinitely.
Example:
i = 0
while i < 5:
print(i)
# Forgot to increment i
In this example, i
is never updated, so the loop will run forever. The correct approach would be:
i = 0
while i < 5:
print(i)
i += 1 # Increment i
2. Incorrect Condition: Writing an incorrect loop condition can lead to unexpected behavior. It’s important to ensure that your condition is logically sound and will eventually become false.
Example:
i = 10
while i > 0:
print(i)
i += 1 # This should be i -= 1
Here, the condition i > 0
is correct, but the loop increments i
instead of decrementing it, causing the loop to run infinitely.
3. Off-by-One Errors: These errors occur when the loop condition is off by one, leading to either an extra iteration or one too few.
Example:
i = 0
while i <= 5:
print(i)
i += 1
- This loop will print numbers 0 through 5, but if the requirement is to print only up to 4, the condition should be
i < 5
instead ofi <= 5
.
Understanding Infinite Loops: How to Detect and Fix Them
An infinite loop occurs when the loop’s condition is always true. While infinite loops can sometimes be intentional (e.g., in a server that runs continuously), they are often the result of a mistake. Detecting and fixing infinite loops is crucial for ensuring your programs run as expected.
How to Detect an Infinite Loop:
- Program Freezes: If your program seems to hang or stop responding, it might be stuck in an infinite loop.
- Unusual Output: If you notice the same output repeatedly without stopping, your loop might be running endlessly.
Example of an Infinite Loop:
i = 0
while i >= 0:
print(i)
i += 1
In this example, the loop condition i >= 0
will always be true because i
is incremented with each iteration. This loop will never terminate on its own.
Fixing Infinite Loops: To fix an infinite loop, ensure that the loop’s condition will eventually become false. This typically involves correctly updating the loop control variable.
i = 10
while i > 0:
print(i)
i -= 1 # Now the loop will end when i becomes 0
In this fixed example, the loop will terminate after i
is decremented to 0.
Tips for Debugging While Loops in Python
Debugging while loops can be challenging, especially when dealing with more complex logic. Here are some tips to help you debug your loops more effectively:
- Print Statements: Inserting print statements inside the loop can help you track the values of variables and understand how the loop is behaving.
Example:
i = 0
while i < 5:
print(f"i is now: {i}")
i += 1
2. Use a Debugger: Python’s built-in debugger (pdb
) or an IDE with debugging features allows you to step through each iteration of the loop, inspect variables, and understand the flow of your program.
3. Break Down Complex Conditions: If your loop condition is complex, try breaking it down into simpler parts. This can make it easier to identify where the logic might be going wrong.
Example:
condition_a = i < 5
condition_b = j > 10
while condition_a and condition_b:
# Do something
i += 1
j -= 1
Breaking the conditions into separate variables can help clarify the logic and make debugging easier.
4. Test with Small Input: Start by testing your loop with small, manageable input values. This makes it easier to track the loop’s behavior and identify issues.
5. Check Loop Boundaries: Make sure your loop’s start and end conditions are correctly defined. Off-by-one errors are common and can often be caught by carefully reviewing the loop’s boundaries.
6. Watch for Logical Errors: Logical errors in the condition or within the loop body can cause unexpected behavior. Double-check the logic to ensure everything is functioning as intended.
Using While Loops with Python Data Structures
While loops are a fundamental part of Python programming, and their flexibility makes them suitable for working with various data structures like lists, dictionaries, tuples, and strings. In this section, we’ll explore how to effectively use while loops with these data structures, providing practical examples to make these concepts easy to understand.

While Loops with Lists: Practical Examples
Lists are one of the most commonly used data structures in Python. They’re ordered, mutable, and can contain a mix of different data types. While loops are particularly useful when you need to process elements in a list until a certain condition is met, rather than just iterating through every item.
Example: Summing Numbers in a List
Let’s say you have a list of numbers and you want to sum them until the sum reaches a certain limit. A while loop would be perfect for this task.
numbers = [3, 5, 8, 13, 21, 34]
sum_numbers = 0
index = 0
while sum_numbers <= 20:
sum_numbers += numbers[index]
index += 1
print(f"Sum: {sum_numbers}")
In this example, the while loop continues to add numbers from the list until the total sum exceeds 20. Notice how the index is used to access list elements, and how it’s incremented with each iteration to move through the list. This approach is particularly useful when you need to process only part of the list based on a dynamic condition.
Example: Removing Elements from a List
Sometimes, you may need to remove certain elements from a list while processing it. Here’s how you can do that with a while loop.
fruits = ["apple", "banana", "cherry", "date", "fig", "grape"]
while "banana" in fruits:
fruits.remove("banana")
print(f"Fruits: {fruits}")
This loop removes all instances of “banana” from the list. The condition checks if “banana” is still in the list, and if so, it removes it. The loop continues until there are no more “banana” elements left.
Using While Loops with Dictionaries and Tuples
Dictionaries and tuples are also important data structures in Python, each with its unique characteristics. Let’s see how while loops interact with these structures.
Using While Loops with Dictionaries
Dictionaries are unordered collections of key-value pairs. While loops can be used to iterate through a dictionary’s keys, values, or both, depending on what you need to do.
Example: Iterating Over a Dictionary
student_grades = {"Alice": 85, "Bob": 92, "Charlie": 78}
keys = list(student_grades.keys())
index = 0
while index < len(keys):
key = keys[index]
print(f"{key}: {student_grades[key]}")
index += 1
In this example, we first convert the dictionary keys into a list, which allows us to use a while loop to iterate over them. This approach is useful when you need more control over the iteration process, such as skipping certain keys or breaking the loop early.
Using While Loops with Tuples
Tuples are similar to lists but are immutable, meaning their elements cannot be changed after creation. While loops with tuples work similarly to lists, though since you can’t modify tuples, you often use while loops to process or extract data rather than altering the tuple.
Example: Accessing Tuple Elements
coordinates = (10, 20, 30, 40, 50)
index = 0
while index < len(coordinates):
print(f"Coordinate {index+1}: {coordinates[index]}")
index += 1
This loop goes through each element in the tuple, printing out the coordinates. Since tuples are immutable, this kind of loop is typically used for reading data rather than modifying it.
Handling Strings in While Loops: Iterating and Processing
Strings in Python are sequences of characters, much like lists but immutable like tuples. You can use while loops to iterate through each character in a string or to perform operations such as searching for a substring or replacing characters.
Example: Counting Vowels in a String
Here’s an example of using a while loop to count the number of vowels in a string.
text = "While loops in Python are powerful"
vowels = "aeiou"
index = 0
count = 0
while index < len(text):
if text[index].lower() in vowels:
count += 1
index += 1
print(f"Number of vowels: {count}")
In this example, the loop iterates through each character in the string. It checks if the character is a vowel by seeing if it exists in the string vowels
, and if so, increments the count
. This is a simple but effective way to process strings using while loops.
Example: Reversing a String
Let’s reverse a string using a while loop.
text = "Python"
reversed_text = ""
index = len(text) - 1
while index >= 0:
reversed_text += text[index]
index -= 1
print(f"Reversed Text: {reversed_text}")
In this example, the while loop starts from the end of the string and works its way to the beginning, adding each character to the reversed_text
string. This is a great example of how you can manipulate strings with while loops.
While Loop vs. For Loop in Python
In Python programming, loops are essential for repeating tasks efficiently. Among the looping constructs, the while
loop and the for
loop are the most commonly used. Understanding their differences, advantages, and ideal use cases can significantly impact how effectively you write your code. In this section, we will explore the key differences between while loops and for loops, discuss when it’s best to use one over the other, and compare their performance.

Key Differences Between While Loops and For Loops
1. Control Flow
The primary difference between while loops and for loops is how they control the flow of iteration.
- While Loop: A
while
loop continues to execute as long as its condition remains true. The loop does not have a built-in mechanism to determine how many times it will run, which means it can run indefinitely if the condition never becomes false.
count = 0
while count < 5:
print(count)
count += 1
In this example, the loop will keep running until count
reaches 5. The condition is checked before each iteration, and the loop executes based on this condition.
For Loop: A for
loop is generally used when the number of iterations is known beforehand or when iterating over a sequence (like a list, tuple, or dictionary). The for
loop iterates over each element in the sequence.
for i in range(5):
print(i)
- Here, the
for
loop will iterate through the numbers 0 to 4. The range function specifies how many times the loop will run.
2. Initialization and Termination
- While Loop: Requires manual initialization and update of the loop variable and relies on a condition to terminate.
index = 0
while index < 10:
print(index)
index += 1
In this case, you must manage the initialization (index = 0
), the condition (index < 10
), and the update (index += 1
) yourself.
- For Loop: Automatically handles initialization and termination. The loop variable is initialized and updated by the
for
loop itself.
for number in range(10):
print(number)
- The
for
loop takes care of initializing the loop variable (number
), setting the termination condition, and updating the variable after each iteration.
3. Use Cases
- While Loop: Best used when you need a loop that depends on a condition and the number of iterations is not predetermined. It is useful for scenarios where you do not know in advance how many times you will need to iterate.Example: Reading lines from a file until the end is reached.
with open('example.txt') as file:
line = file.readline()
while line:
print(line.strip())
line = file.readline()
- For Loop: Ideal for iterating over a sequence or range when the number of iterations is known or when you are working with iterable data structures.
Example: Summing values in a list.
numbers = [1, 2, 3, 4, 5]
total = 0
for num in numbers:
total += num
print(total)
When to Use While Loops Instead of For Loops
Choosing between a while loop and a for loop often depends on the specific needs of your program.
- Use a While Loop When:
- The number of iterations is unknown and depends on a condition.
- You need more complex conditions for continuing or breaking out of the loop.
- You are dealing with scenarios where the loop might run indefinitely until a specific condition is met.
Example: Waiting for user input until a valid response is received.
user_input = ''
while user_input.lower() not in ['yes', 'no']:
user_input = input("Please enter 'yes' or 'no': ")
Use a For Loop When:
- You have a defined sequence or range to iterate over.
- The number of iterations is predetermined or fixed.
- You are iterating over elements in a data structure like lists, tuples, or dictionaries.
Example: Iterating over a list of user names to greet each user.
users = ["Alice", "Bob", "Charlie"]
for user in users:
print(f"Hello, {user}!")
Performance Comparison: While Loops vs. For Loops
In terms of performance, both while loops and for loops are generally efficient and their performance difference is usually negligible for most use cases. The choice between them should primarily be based on readability and suitability for the task at hand rather than performance.
1. Execution Time
Both types of loops have similar execution times for equivalent tasks. However, for loops might have a slight edge in scenarios where the number of iterations is known beforehand, as they are often more optimized by Python’s internals.
2. Code Readability
For loops can often make the code more readable when iterating over sequences or ranges, as they clearly indicate the number of iterations and the data being iterated over.
Example of Readable For Loop:
for number in range(10):
print(number)
Example of Equivalent While Loop:
index = 0
while index < 10:
print(index)
index += 1
In this case, the for loop provides a more concise and readable approach, especially when iterating through a known sequence or range.
3. Overhead
While loops can potentially incur more overhead due to manual condition checking and updating loop variables. For loops, with their built-in iteration mechanisms, can be slightly more efficient in these scenarios.
Both while loops and for loops are powerful tools in Python, each suited to different scenarios. While loops offer flexibility for condition-based iteration, while for loops provide a clear structure for iterating over sequences. Understanding when to use each type of loop can enhance the readability and efficiency of your code, making your programming tasks smoother and more intuitive.
Recent Advancements and Updates Related to While Loops in Python
In the world of Python, where new versions and libraries are constantly evolving, it’s essential to stay updated on how these changes impact the core structures we use daily. While loops, a fundamental part of Python, have seen some interesting updates and enhancements in recent versions of Python 3.x. In this section, we’ll explore what’s new for while loops, the latest libraries that can enhance their capabilities, and how while loops have evolved in recent Python versions.
What’s New in Python 3.x for While Loops?
Python 3.x brought numerous updates that, while not directly altering the fundamental nature of while loops, have improved how developers can use them in modern coding practices. These enhancements focus on making loops more efficient, readable, and versatile, particularly when combined with other Python features.
1. The Walrus Operator (:=
)
One of the more notable additions in Python 3.8 was the introduction of the walrus operator (:=
), which allows you to assign values within expressions. This operator has subtly enhanced how while loops are written, making them more concise and often easier to read.
Before Python 3.8, you might have written a while loop like this:
data = input("Enter data (or 'quit' to stop): ")
while data != 'quit':
process(data)
data = input("Enter data (or 'quit' to stop): ")
With the walrus operator, the loop can be simplified:
while (data := input("Enter data (or 'quit' to stop): ")) != 'quit':
process(data)
This change reduces redundancy by allowing the assignment to occur directly within the while loop’s condition, making the code cleaner and easier to follow.
2. Type Hinting and Static Analysis
Python 3.x versions, particularly from 3.5 onward, introduced and refined type hinting. This feature helps with static analysis tools like mypy
, enabling developers to catch potential errors before running the code. While loops benefit from this, as you can now clearly specify expected types within the loop, leading to fewer runtime errors.
Example:
def process_data(data: str) -> None:
while data != "stop":
print(f"Processing {data}")
data = input("Enter data (or 'stop' to end): ")
Type hinting enhances readability and provides additional documentation, helping developers understand the expected types in the loop.
Latest Python Libraries that Enhance While Loop Capabilities
While loops are versatile on their own, but several modern Python libraries and tools have emerged that extend their functionality, allowing for more efficient and advanced looping mechanisms.
1. itertools
The itertools
library, a part of Python’s standard library, provides powerful tools for iteration that can be combined with while loops for more complex tasks. For example, itertools.count
can generate an infinite sequence of numbers, which can be very useful in while loops that need to iterate indefinitely under certain conditions.
Example:
import itertools
counter = itertools.count()
while next(counter) < 10:
print("This will run 10 times")
itertools
can be a lifesaver when you need to handle large datasets or perform repetitive tasks efficiently within a while loop.
2. more_itertools
For even more advanced iteration techniques, the more_itertools
library extends the standard itertools
with additional functionalities like chunked
, side_effect
, and consumer
. These can be particularly useful in scenarios where while loops are dealing with large, complex data structures.
Example:
from more_itertools import chunked
data = range(20)
chunks = chunked(data, 5)
while chunks:
print(next(chunks))
Using libraries like these can simplify the code inside your while loops, making them easier to manage and understand.
How While Loops Have Evolved in Recent Python Versions
While the basic structure of while loops has remained consistent, the surrounding ecosystem of Python has evolved, making while loops more powerful and flexible in modern coding environments.
1. Enhanced Error Handling
With Python 3.x’s enhanced exception handling and the introduction of context managers (with
statements), while loops have become more robust when dealing with errors or managing resources like file I/O.
Example:
try:
with open('data.txt') as file:
while (line := file.readline()):
process(line)
except FileNotFoundError:
print("File not found.")
This integration allows for safer code, particularly in scenarios where external resources are involved, making while loops more dependable.
2. Improved Debugging with f-strings
Introduced in Python 3.6, f-strings have significantly improved the debugging experience. When used within while loops, they allow for quick, readable output that can help trace the loop’s behavior without adding much overhead.
Example:
count = 0
while count < 5:
print(f"Current count: {count}")
count += 1
F-strings provide a clear, concise way to monitor values during each iteration, making the debugging process smoother.
Best Practices for Writing Efficient While Loops
Optimizing While Loops for Better Performance
Performance is a key concern when writing while loops, especially in scenarios involving large datasets or time-sensitive operations. Even a small inefficiency in a loop can cause a noticeable delay when the loop runs thousands or millions of times.
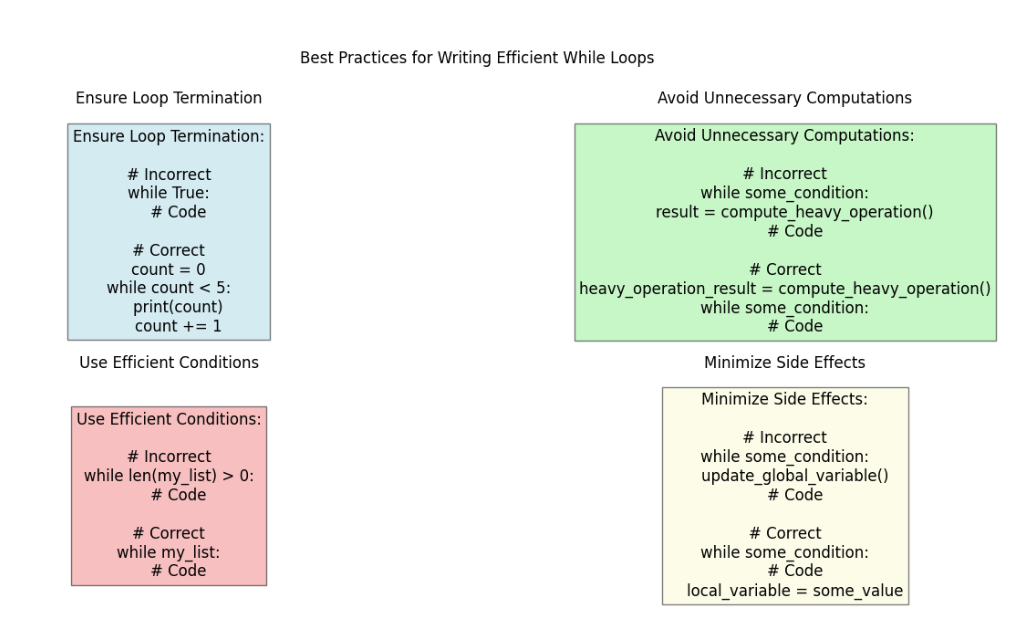
1. Avoid Unnecessary Operations
Every operation inside a while loop adds to its execution time. To optimize, ensure that only essential operations are performed within the loop. If a calculation or an operation can be done before entering the loop, move it outside.
Example:
# Inefficient loop
i = 0
while i < len(data):
value = complex_calculation(data[i]) # Avoid repeating this calculation
i += 1
# Optimized loop
length = len(data)
i = 0
while i < length:
value = complex_calculation(data[i])
i += 1
In the first version, len(data)
is recalculated in every iteration, while the optimized version calculates it once, reducing unnecessary overhead.
2. Minimize Function Calls
Function calls inside while loops can slow down the loop, especially if the function is complex or resource-intensive. Where possible, replace the function call with its direct computation or use a more efficient function.
Example:
# Inefficient
i = 0
while i < n:
process_item(get_next_item(i)) # Function call inside loop
i += 1
# More efficient
i = 0
next_item = get_next_item # Cache the function
while i < n:
process_item(next_item(i))
i += 1
By caching the function reference outside the loop, you can slightly improve performance, especially in tight loops.
3. Use Efficient Data Structures
Choosing the right data structure can make a significant difference in while loop performance. Lists, dictionaries, and sets all have different performance characteristics. For example, searching for an item in a set is generally faster than searching in a list due to how sets are implemented.
Example:
# Using a list (slower for large n)
elements = [1, 2, 3, 4, 5]
while some_condition:
if item in elements:
process(item)
# Using a set (faster for large n)
elements = {1, 2, 3, 4, 5}
while some_condition:
if item in elements:
process(item)
In this case, using a set improves the efficiency of the loop when checking membership, especially with larger datasets.
Refactoring While Loops for Improved Readability
Readability is often sacrificed for performance, but with careful refactoring, you can maintain both. Clean, readable code is easier to maintain, debug, and extend, especially in large-scale projects.
1. Simplify the Loop Condition
Complicated loop conditions can make your while loop hard to understand. Simplify the condition by breaking it down into smaller, well-named variables or functions.
Example:
# Complex condition
while (x > 0 and y < 100) or (z == 5 and check_condition(a, b)):
process_data()
# Simplified condition
valid_range = x > 0 and y < 100
special_case = z == 5 and check_condition(a, b)
while valid_range or special_case:
process_data()
By breaking the condition into named variables, the loop becomes much easier to read and understand.
2. Use Comments Wisely
Adding comments inside while loops helps others (and your future self) understand the logic. However, over-commenting can clutter the code. Aim for concise, meaningful comments that explain why something is done, not what is done.
Example:
# Process data while in valid range or under special condition
while valid_range or special_case:
# Process each item in the list
process_data()
This keeps the code clean while still providing context.
3. Refactor Long Loops into Functions
If a while loop is doing too much, consider refactoring it into smaller functions. This makes the loop itself more readable and the overall code more modular.
Example:
while some_condition:
process_data_step1()
process_data_step2()
process_data_step3()
Each step could be a function that handles a specific part of the loop’s work, making the loop itself a high-level overview of what’s happening.
Using While Loops in Large-Scale Python Projects
In large-scale projects, while loops can become bottlenecks if not managed properly. Efficiently using while loops in such projects requires careful planning and awareness of the potential pitfalls.
1. Consider Loop Unrolling
In scenarios where performance is critical, you might consider loop unrolling, a technique where you manually expand the loop body to reduce the loop’s control overhead.
Example:
# Regular loop
while i < n:
process_item(i)
i += 1
# Unrolled loop
while i < n:
process_item(i)
if i + 1 < n:
process_item(i + 1)
i += 2
This reduces the number of iterations, which can save time, especially in tight loops. However, loop unrolling should be used judiciously, as it can make the code harder to maintain.
2. Using Asynchronous Programming
In large-scale applications, consider using asynchronous programming to handle loops that involve I/O operations. asyncio
in Python can help manage long-running loops more efficiently.
Example:
import asyncio
async def process_data():
while some_condition:
await asyncio.sleep(1) # Simulate I/O-bound task
process(item)
# Running the async loop
asyncio.run(process_data())
Using asyncio
allows other tasks to run while waiting, making your program more responsive and efficient.
3. Monitor Performance with Profiling Tools
Regularly profiling your code helps identify while loops that may be causing performance issues. Tools like cProfile
can show you where the most time is being spent, allowing you to target optimization efforts where they are most needed.
Example:
import cProfile
def main():
while some_condition:
process_data()
cProfile.run('main()')
Profiling provides a clear picture of your loop’s performance, guiding further optimization.
Practical Applications of While Loops
Automating Repetitive Tasks with While Loops
One of the most common uses of while loops is automating repetitive tasks. Whether it’s cleaning up files, managing system operations, or even iterating over user input, while loops provide a way to handle tasks that need to be repeated until a specific condition is met.
Example: Renaming Files in a Directory
Suppose you have a directory full of files that need renaming based on a certain pattern. A while loop can automate this task, ensuring every file gets processed without missing a beat.
import os
# Get a list of files in the directory
files = os.listdir('/path/to/directory')
index = 0
# Loop until all files are renamed
while index < len(files):
old_name = files[index]
new_name = f"renamed_file_{index}.txt"
os.rename(old_name, new_name)
index += 1
In this example, the while loop continues renaming files until every file in the directory has been processed. This is much more efficient than manually renaming each file.
Example: Monitoring System Health
Another practical use is in monitoring system health or services, where a while loop can continually check the status of a service and trigger alerts if something goes wrong.
import time
# Monitor service status
service_running = True
while service_running:
status = check_service_status()
if not status:
alert_admin("Service is down!")
service_running = False
time.sleep(5) # Wait for 5 seconds before checking again
Here, the loop runs indefinitely, checking the status of a service every 5 seconds until an issue is detected. Automating this kind of monitoring helps in maintaining system reliability without manual intervention.
Using While Loops for Real-Time Data Processing
In real-time applications, such as data streaming or sensor data analysis, while loops play a crucial role. They allow continuous data processing as new data becomes available, making them ideal for situations where the data flow is constant and needs immediate handling.
Example: Processing Sensor Data
Imagine working on a project that involves reading data from a temperature sensor. The data needs to be processed as it arrives to trigger warnings if the temperature crosses a threshold.
import random
import time
# Simulate real-time sensor data
def read_temperature_sensor():
return random.uniform(20.0, 30.0)
while True:
temperature = read_temperature_sensor()
print(f"Current temperature: {temperature:.2f} °C")
if temperature > 28.0:
print("Warning: High temperature detected!")
time.sleep(1) # Wait for 1 second before reading the sensor again
This loop continues to read the temperature sensor indefinitely, processing each reading in real-time. If a temperature exceeds 28°C, a warning is printed. Such loops are essential in environments where quick responses to data changes are necessary.
Example: Handling Real-Time Stock Data
For financial applications, real-time stock data needs to be processed quickly to make trading decisions. A while loop can handle the incoming data, allowing for immediate analysis and action.
def fetch_stock_price(symbol):
# Simulate fetching stock price
return random.uniform(100.0, 150.0)
while True:
price = fetch_stock_price("AAPL")
print(f"Apple stock price: ${price:.2f}")
if price < 105.0:
print("Buy signal: Apple stock price is low!")
elif price > 145.0:
print("Sell signal: Apple stock price is high!")
time.sleep(2) # Check every 2 seconds
In this example, the while loop processes stock prices in real-time. It provides immediate buy or sell signals based on predefined price thresholds, automating part of the trading decision-making process.
Examples of While Loops in Web Scraping and Data Collection
Web scraping is another area where while loops shine. They allow for continuous data extraction from websites, handling multiple pages, and collecting data until there’s no more to retrieve. This is particularly useful for gathering large datasets or monitoring websites for updates.
Example: Scraping Multiple Pages of a Website
When scraping a website, you often need to navigate through multiple pages to collect all the necessary data. A while loop can manage this by checking if the next page exists and continuing to scrape until the end.
import requests
from bs4 import BeautifulSoup
url = "http://example.com/page/"
page = 1
while True:
response = requests.get(f"{url}{page}")
if response.status_code != 200:
break # Exit loop if no more pages
soup = BeautifulSoup(response.text, 'html.parser')
# Extract and process data from the page
print(f"Scraping page {page}")
page += 1
In this example, the while loop continues to scrape each page until the server returns a status code other than 200, indicating there are no more pages to scrape. This method automates the data collection process across multiple pages.
Example: Monitoring a Website for Updates
If you need to monitor a website for updates, such as checking when a new blog post is published, a while loop can automate this by periodically requesting the page and comparing the content.
import hashlib
def get_page_hash(url):
response = requests.get(url)
return hashlib.md5(response.text.encode('utf-8')).hexdigest()
url = "http://example.com/blog"
last_hash = get_page_hash(url)
while True:
current_hash = get_page_hash(url)
if current_hash != last_hash:
print("New update detected!")
last_hash = current_hash
time.sleep(60) # Check every 60 seconds
Here, the loop continually checks the page’s content hash and compares it to the previous hash. If there’s a change, it detects that the page has been updated. This approach is useful for scenarios where manual checking would be tedious and time-consuming.
While loops are far more than just a basic tool in a programmer’s toolkit. They offer the flexibility and power needed to automate repetitive tasks, handle real-time data processing, and scrape websites for valuable information. By understanding how to apply while loops effectively, you can unlock new possibilities in your Python projects, making your code not only functional but also efficient and reliable.
Conclusion
Recap: Key Points to Remember About While Loops
Throughout this course, we’ve covered a wide range of topics related to while loops in Python. Here’s a quick recap of the key points:
- Understanding While Loops: While loops continue to execute as long as the given condition is true. They’re versatile for situations where the number of iterations isn’t known in advance.
- Common Pitfalls: We explored common mistakes, such as forgetting to update the loop condition, which can lead to infinite loops. Understanding these errors and how to avoid them is crucial for writing reliable code.
- While Loops with Python Data Structures: You learned how to use while loops with lists, dictionaries, tuples, and strings. Each data structure offers unique ways to loop and process data.
- While Loop vs. For Loop: We discussed the differences between while loops and for loops, including when to use each type. While loops are best for conditions-based iteration, while for loops are ideal when the number of iterations is known.
- Recent Advancements: Python’s evolution has brought subtle enhancements to while loops, such as more efficient libraries that complement their use, making them more powerful in specific scenarios.
- Best Practices: Optimizing and refactoring while loops can significantly improve code performance and readability. This is particularly important in large-scale projects where efficiency and maintainability are key.
- Practical Applications: We explored real-world applications of while loops, from automating repetitive tasks to processing real-time data and web scraping. These examples demonstrate the practical utility of while loops in various Python projects.
Final Thoughts on Mastering While Loops in Python
Mastering while loops is an essential step in becoming proficient in Python programming. They might seem simple at first, but their ability to handle complex, condition-based scenarios makes them indispensable in many applications. Whether you’re automating a task, handling real-time data, or navigating the intricacies of web scraping, while loops provide the control and flexibility needed to write efficient and effective code.
As you’ve seen, while loops can be powerful tools when used correctly. Understanding how and when to use them, avoiding common mistakes, and applying best practices will make you a more confident and capable Python programmer.
Encouragement to Practice and Apply While Loops in Projects
Now that you have a solid understanding of while loops, I encourage you to put this knowledge into practice. Experiment with different use cases in your own projects. The best way to truly master while loops is through hands-on experience.
Try automating a repetitive task you do often, or perhaps set up a script to monitor real-time data or scrape a website. The more you practice, the more intuitive writing while loops will become. As you apply these concepts to real-world problems, you’ll find that while loops can greatly enhance your programming capabilities.
Remember, programming is as much about problem-solving as it is about writing code. While loops are one of the many tools in your toolkit that can help you tackle complex challenges with ease. So, keep practicing, stay curious, and don’t hesitate to push the boundaries of what you can achieve with while loops in Python.
External Resources
Links to Python Documentation and Developer Forums
To continue building your Python skills and deepening your understanding of while loops, here are some valuable resources you can explore:
- Python Official Documentation: While Loops
The Python documentation provides a comprehensive guide to while loops, covering syntax, examples, and advanced usage. - Real Python: While Loops
Real Python offers a beginner-friendly tutorial on while loops, complete with examples and explanations. - Stack Overflow: Python While Loop Questions
Stack Overflow is a go-to resource for developers seeking answers to specific programming questions. You can search for while loop-related topics or post your own questions. - Reddit: Learn Python Subreddit
The Learn Python subreddit is a helpful community where you can ask questions, share knowledge, and find resources related to Python programming.
FAQs
A while loop in Python is a control flow statement that allows you to repeatedly execute a block of code as long as a specified condition remains true. It’s particularly useful when you don’t know in advance how many times you need to repeat the action, such as waiting for user input until they provide a correct response.
To avoid infinite loops, you need to ensure that the condition controlling the while loop will eventually become false. This typically involves updating the variables or conditions being checked within the loop so that the loop can terminate properly. Forgetting to do this is a common cause of infinite loops.
You should use a while loop when the number of iterations is not known before the loop starts. While loops are best for scenarios where you need to keep checking a condition until it becomes false. On the other hand, a for loop is more appropriate when you know the exact number of iterations in advance, such as iterating over a list of items.
Yes, you can use an else clause with a while loop in Python. The else block will execute only if the loop terminates normally (i.e., the condition becomes false). However, if the loop is exited via a break statement, the else block will not execute. This feature can be useful for adding additional logic that should run after a loop finishes its regular execution.