Introduction to For Loops in Python
When you start learning Python, one of the first things you’ll encounter is the concept of loops, specifically the for loop. Now, if you’re anything like most beginners, you might be wondering what exactly a for loop is and why it’s so important. Well, you’re in the right place! In this section, we’ll break it down in a way that’s easy to understand, so by the end of this course, you’ll feel confident using for loops in your own Python projects.
What is a For Loop in Python?
A for loop in Python helps you repeat a task multiple times without writing the same code again and again. It’s useful when you need to go through a list of items, process data, or automate repetitive work.
Let’s say you have a list of names and you want to print each name one by one. Without a for loop, you’d have to write a print statement for each name manually. But with a for loop, you can do this in just a few lines of code:
names = ["Alice", "Bob", "Charlie", "Diana"]
for name in names:
print(name)
Output:
Alice
Bob
Charlie
Diana
Here’s what’s happening: the for loop goes through each item in the list names
, assigns it to the variable name
, and then runs the print statement. So, you only write the code once, and the loop does the rest for you. It’s simple, but powerful.
Importance of For Loops in Python Programming
Now, you might be wondering, “Why are for loops so important in Python?” The answer is that they save you time and make your code cleaner and easier to read. Instead of repeating yourself, you can let the for loop handle the repetition for you. This not only makes your code shorter but also reduces the chances of making mistakes.
For loops are especially useful when working with large datasets or when you need to process a lot of information. For example, if you’re working with a list of thousands of numbers and you need to calculate the total sum, doing it manually would be a nightmare. But with a for loop, it’s a breeze:
numbers = [2, 4, 6, 8, 10]
total_sum = 0
for number in numbers:
total_sum += number
print(total_sum)
Output:
30
In this example, the for loop goes through each number in the list, adds it to total_sum
, and at the end, you get the sum of all the numbers. It’s quick, efficient, and easy to understand.
When and Why to Use For Loops in Python
So, when should you use a for loop? The simple answer is: whenever you need to repeat something. Whether you’re processing data, automating tasks, or just trying to make your code cleaner, for loops are your go-to tool. They’re flexible enough to handle a wide range of tasks, from the very simple to the complex.
For example, let’s say you’re building a simple text-based game where the player has three chances to guess the correct number. A for loop is perfect for this because you can set it to run exactly three times:
correct_number = 7
for attempt in range(3):
guess = int(input("Guess the number: "))
if guess == correct_number:
print("Congratulations! You guessed the right number.")
break
else:
print("Try again.")
Output:
Guess the number: 5
Try again.
Guess the number: 6
Try again.
Guess the number: 7
Congratulations! You guessed the right number.
In this example, the for loop runs three times, giving the player three chances to guess the correct number. If they guess correctly, the loop stops early thanks to the break
statement. If not, it moves on to the next attempt. This is just one of many situations where a for loop can come in handy.
Must Read
- How to Return Multiple Values from a Function in Python
- Parameter Passing Techniques in Python: A Complete Guide
- A Complete Guide to Python Function Arguments
- How to Create and Use Functions in Python
- Find All Divisors of a Number in Python
Basic Syntax and Structure of For Loops in Python
Learning the basic syntax of a for loop in Python is like getting the hang of a simple recipe. Once you know the ingredients and the steps, you can create something really powerful with very little effort. Let’s break it down, so you can confidently write for loops in your Python projects.
Understanding the Basic Syntax of a For Loops in Python
A for loop in Python is simple and easy to read. It helps you write cleaner and more understandable code. The basic structure looks like this:
for item in sequence:
# code to execute for each item
Here’s what’s happening in this code:
for
is the keyword that tells Python you’re starting a loop.item
is a variable that takes the value of each element in the sequence, one at a time.in
is another keyword that connects the loop variable with the sequence you’re going to loop through.sequence
is the collection of items you want to loop over, like a list, tuple, or string.- The colon (
:
) indicates that what follows is the block of code that will be repeated.
This structure makes Python’s for loop easy to read, even for beginners. You don’t need to worry about complex syntax—everything is laid out in a way that feels logical and straightforward.
For Loop Syntax with Simple Examples
Let’s make this more concrete with a couple of simple examples. Suppose you want to print each number in a list:
numbers = [1, 2, 3, 4, 5]
for number in numbers:
print(number)
In this example:
- The for loop goes through each number in the list
numbers
. - The variable
number
takes the value of each list item, one by one. - The
print(number)
line inside the loop prints each number as it’s accessed.
When you run this code, the output will be:
1
2
3
4
5
This is a classic use of a for loop—iterating through a list and performing an action with each item. The structure is simple, yet it’s powerful enough to handle all kinds of tasks.
Indentation in Python For Loops: Why It Matters
In Python, indentation is not just for readability—it’s a key part of the language. The way you use spaces or tabs determines which code belongs inside a for loop and which code doesn’t. If indentation is incorrect, Python will show an error or your code won’t work as expected.
Let’s look at this with an example:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
print("This is a fruit")
print("End of loop")
Here’s how indentation affects this code:
- The two
print
statements inside the loop are indented, meaning they are part of the for loop. Python knows to repeat these lines for each item in thefruits
list. - The
print("End of loop")
line is not indented, meaning it’s outside the loop. It will only run once, after the loop has finished.
The output will be:
apple
This is a fruit
banana
This is a fruit
cherry
This is a fruit
End of loop
Without proper indentation, Python will either give you an error or produce unexpected results. So, when writing for loops, always pay close attention to your indentation—it’s not just about making your code look nice; it’s about making sure your code works correctly.
Iterating Over Different Data Types with For Loops
A for loop is one of the most useful tools in Python. It helps you work with different types of data like lists, strings, and tuples by letting you access and manipulate each item easily.
In this section, we’ll look at how for loops work with different data types, using examples and clear explanations to make it easy to understand.
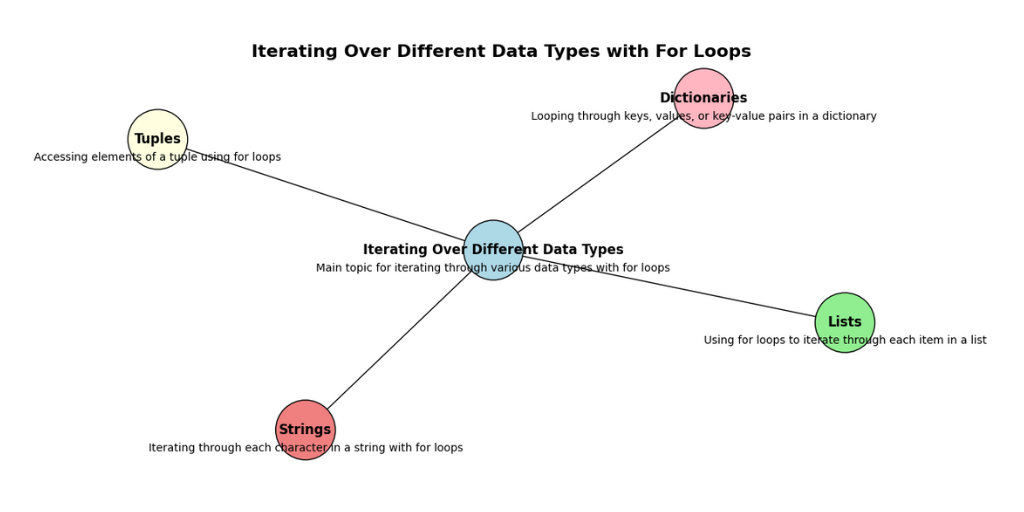
For Loops with Lists in Python
Let’s start with lists, one of the most common data types you’ll work with in Python. A list is a collection of items that can be anything from numbers to strings. What makes lists so powerful is that you can store multiple items in one variable and then use a for loop to access and process each item individually.
Here’s a simple example:
fruits = ["apple", "banana", "cherry", "date"]
for fruit in fruits:
print(fruit)
In this code:
- The for loop goes through each item in the
fruits
list. - The variable
fruit
takes the value of each item, one at a time. - The
print(fruit)
statement outputs each fruit to the console.
The output will be:
apple
banana
cherry
date
This approach is perfect when you need to perform the same operation on every item in a list. Whether you’re printing values, modifying them, or doing something more complex, for loops give you the control you need.
Iterating Through Strings Using For Loops
Now, let’s talk about strings. A string in Python is a sequence of characters. You might not realize it, but a for loop can treat a string much like a list. Each character in the string can be accessed individually using a for loop.
Here’s an example:
message = "Hello"
for letter in message:
print(letter)
In this example:
- The for loop iterates over each character in the
message
string. - The variable
letter
takes the value of each character. - The
print(letter)
statement prints each character on a new line.
The output will be:
H
e
l
l
o
This approach is useful when you need to work with individual characters in a string. Whether you’re counting letters, finding patterns, or printing each character, a for loop makes the task easy and efficient.
Using For Loops with Tuples in Python
Finally, let’s talk about tuples. A tuple is similar to a list, but the main difference is that tuples are immutable, meaning once you create a tuple, you can’t change its contents. However, for loops work just as easily with tuples as they do with lists, allowing you to iterate through their items without any issues.
Here’s how you can use a for loop with a tuple:
coordinates = (10, 20, 30)
for coordinate in coordinates:
print(coordinate)
In this example:
- The for loop iterates over each item in the
coordinates
tuple. - The variable
coordinate
takes the value of each item. - The
print(coordinate)
statement prints each coordinate.
The output will be:
10
20
30
Even though tuples are immutable, for loops allow you to access and use the data they contain, making them just as flexible as lists for many tasks.
How to Iterate Over Dictionaries with For Loops in Python
Dictionaries in Python store data in pairs, where each key is linked to a value. This makes it easy to find specific information using the key (like looking up someone’s name or ID). When working with dictionaries, for loops help you go through each key-value pair, so you can do things like modify or check the data.
Iterating Through Keys in a Dictionary
Let’s begin with the basics: iterating through the keys of a dictionary. A key in a dictionary is used to find the value associated with it. Python makes it simple to loop through these keys with a for loop, allowing you to access each key one by one.
Here’s an example:
student_ages = {
"Alice": 22,
"Bob": 24,
"Charlie": 23
}
for student in student_ages:
print(student)
In this example:
- The for loop goes through each key in the
student_ages
dictionary. - The variable
student
takes the value of each key (e.g., “Alice”, “Bob”, “Charlie”). - The
print(student)
statement outputs each key.
The output will be:
Alice
Bob
Charlie
Iterating Through Values in a Dictionary
Sometimes, you might want to work with the values in a dictionary instead of the keys. You can easily modify your for loop to do this by using the values()
method.
Here’s how you do it:
for age in student_ages.values():
print(age)
In this case:
- The for loop iterates over each value in the
student_ages
dictionary. - The variable
age
takes the value of each entry (e.g., 22, 24, 23). - The
print(age)
statement outputs each value.
The output will be:
22
24
23
Iterating Through Key-Value Pairs in a Dictionary
Often, you’ll want to work with both the key and the value at the same time. Python allows you to do this using the items()
method, which returns each key-value pair as a tuple.
Here’s an example:
for student, age in student_ages.items():
print(f"{student} is {age} years old.")
In this example:
- The for loop iterates over each key-value pair in the
student_ages
dictionary. - The variables
student
andage
take the values of each key and corresponding value. - The
print(f"{student} is {age} years old.")
statement formats and outputs a string that includes both the key and value.
The output will be:
Alice is 22 years old.
Bob is 24 years old.
Charlie is 23 years old.
This method is incredibly handy when you need to perform operations that involve both the keys and the values in your dictionary.
For Loops and Sets: Unique Data Iteration
Now, let’s talk about sets. In Python, sets are collections of unique items. Unlike lists or tuples, sets don’t allow duplicate elements. This makes them great for situations where you want to ensure all items are unique, like keeping track of event participants or counting distinct words in a document.
Iterating Over Sets with For Loops
Using a for loop to iterate over a set is simple. Since sets are unordered, the order in which you access the items may vary each time, but every item will be accessed exactly once.
Here’s a basic example:
unique_numbers = {1, 2, 3, 4, 5}
for number in unique_numbers:
print(number)
In this example:
- The for loop iterates over each item in the
unique_numbers
set. - The variable
number
takes the value of each item. - The
print(number)
statement outputs each number.
The output will display all the numbers in the set, though the order may vary:
1
2
3
4
5
Practical Example: Removing Duplicates from a List
Sets are often used to remove duplicates from a list. You can convert a list to a set, which automatically removes any duplicate items, and then iterate over this set using a for loop.
Here’s how you can do it:
numbers = [1, 2, 2, 3, 4, 4, 5]
unique_numbers = set(numbers)
for number in unique_numbers:
print(number)
In this example:
- The
numbers
list contains duplicate values. - Converting
numbers
to a set removes the duplicates, leaving only unique values. - The for loop then iterates over the
unique_numbers
set and prints each unique number.
The output will be:
1
2
3
4
5
This method is incredibly useful when you need to ensure that all items in a collection are unique.
Advanced Techniques with For Loops
For loops are incredibly powerful tools in Python, and once you’re comfortable with the basics, there are some advanced techniques that can take your coding skills to the next level. These techniques include nested for loops and using for loops with the range function.

Nested For Loops in Python
A nested for loop is simply a for loop inside another for loop. It’s useful when you need to work with data that has multiple levels, like a list of lists or a grid. The outer loop handles one level, and the inner loop works through the items in that level. For example, in a list of lists, the outer loop goes through each list, and the inner loop goes through each item in that list.
Here’s a simple example to illustrate:
# Let's create a multiplication table
for i in range(1, 4):
for j in range(1, 4):
print(f"{i} * {j} = {i * j}")
In this example:
- The first for loop (with
i
) runs through numbers 1 to 3. - For each value of
i
, the second for loop (withj
) also runs through numbers 1 to 3. - The
print(f"{i} * {j} = {i * j}")
statement outputs the multiplication ofi
andj
.
The output will be:
1 * 1 = 1
1 * 2 = 2
1 * 3 = 3
2 * 1 = 2
2 * 2 = 4
2 * 3 = 6
3 * 1 = 3
3 * 2 = 6
3 * 3 = 9
This method is particularly useful for generating grids, tables, or working with multidimensional data structures.
Using For Loops with the Range Function
The range()
function in Python is useful when you want to loop through a specific number of times or generate a sequence of numbers. You can customize the range by specifying a start, stop, and step. The start tells where the sequence begins, the stop tells where it ends (but doesn’t include it), and the step determines the difference between each number in the sequence. For example, range(1, 10, 2)
would generate numbers from 1 to 9, counting by 2 each time.
Let’s start with a basic example:
for i in range(5):
print(i)
In this case:
- The range function generates numbers from 0 to 4 (not including 5).
- The for loop iterates over these numbers, and the
print(i)
statement outputs each number.
The output will be:
0
1
2
3
4
Now, let’s explore a more customized range:
for i in range(1, 10, 2):
print(i)
In this example:
- The range function starts at 1, stops before 10, and increments by 2.
- The for loop iterates over these numbers, and
print(i)
outputs each number.
The output will be:
1
3
5
7
9
This is especially helpful when you want to control the order of iteration, like processing every other item in a list or dealing with data that isn’t in a continuous sequence.
Practical Example: Countdown Timer
Let’s look at a practical example where the range function is used to create a simple countdown timer:
import time
for seconds in range(10, 0, -1):
print(f"Time left: {seconds} seconds")
time.sleep(1)
print("Time's up!")
In this example:
- The for loop uses
range(10, 0, -1)
to start from 10 and count down to 1. - The
time.sleep(1)
function pauses for one second between each iteration. - The loop prints a countdown message for each second, and finally prints “Time’s up!” when the countdown ends.
This technique is not only useful for countdowns but also for situations where you need to step through a process in reverse.
Enumerate in For Loops: Accessing Index and Value Simultaneously
When you’re working with lists or any iterable in Python, you often need to access both the index and the value of each item simultaneously. Python makes this task easier with the enumerate
function. Using enumerate()
in a for loop allows you to get both the index and the value of items in a sequence at the same time. This is helpful when you need to know the position of each item while also accessing its value. For example, when iterating over a list, enumerate()
provides a convenient way to work with both the item and its index without needing a separate counter.
Let’s break this down with a simple example:
fruits = ['apple', 'banana', 'cherry', 'date']
for index, value in enumerate(fruits):
print(f"Index: {index}, Value: {value}")
In this example:
- The
enumerate
function takes thefruits
list as input and returns both the index and value for each item in the list. - The for loop then iterates over these pairs, and the
print
statement outputs the index and corresponding value.
The output will be:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
Index: 3, Value: date
Why Use Enumerate?
Using enumerate
is especially helpful when you need to keep track of your position in the loop. For instance, you might be iterating through a list and want to perform a specific action when you reach a certain index. Here’s a practical example:
numbers = [10, 20, 30, 40, 50]
for index, value in enumerate(numbers):
if index % 2 == 0: # Check if index is even
print(f"Index {index} is even, value: {value}")
In this example:
- The for loop iterates through the
numbers
list usingenumerate
. - The
if
statement checks if the index is even (i.e., divisible by 2 without a remainder). - If the index is even, the
print
statement outputs the index and value.
The output will be:
Index 0 is even, value: 10
Index 2 is even, value: 30
Index 4 is even, value: 50
Looping with Conditional Statements Inside For Loops
Another powerful technique is using conditional statements inside for loops. This allows you to filter data, perform specific actions only when certain conditions are met, or manage control flow in a loop. Let’s explore this concept with some practical examples.
Example 1: Filtering Even Numbers
Let’s say you have a list of numbers and you want to print only the even ones. You can do this easily with a for loop combined with an if
statement:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for number in numbers:
if number % 2 == 0: # Check if the number is even
print(f"Even number: {number}")
In this example:
- The for loop iterates through the
numbers
list. - The
if
statement checks if the number is even (i.e., divisible by 2 without a remainder). - If the condition is met, the
print
statement outputs the even number.
The output will be:
Even number: 2
Even number: 4
Even number: 6
Even number: 8
Even number: 10
Example 2: Handling Specific Values Differently
Imagine you have a list of grades and you want to print a special message for failing grades. You can do this with a for loop and an if-else
statement:
grades = [85, 62, 90, 45, 78]
for grade in grades:
if grade < 60: # Check if the grade is failing
print(f"Grade {grade}: Failing")
else:
print(f"Grade {grade}: Passing")
In this example:
- The for loop iterates through the
grades
list. - The
if
statement checks if the grade is below 60, indicating a failing grade. - If the grade is failing, it prints “Failing”; otherwise, it prints “Passing.”
The output will be:
Grade 85: Passing
Grade 62: Passing
Grade 90: Passing
Grade 45: Failing
Grade 78: Passing
Example 3: Combining Enumerate and Conditional Statements
You can also combine enumerate
with conditional statements inside a for loop to create more complex logic. Here’s an example where you track both the index and the value, and perform an action based on a condition:
colors = ['red', 'blue', 'green', 'yellow', 'purple']
for index, color in enumerate(colors):
if color == 'green':
print(f"Found green at index {index}")
else:
print(f"Color {color} at index {index}")
In this example:
- The for loop uses
enumerate
to iterate over thecolors
list. - The
if-else
statement checks if the current color is “green.” - If it is, the loop prints a special message; otherwise, it prints the color and index.
The output will be:
Color red at index 0
Color blue at index 1
Found green at index 2
Color yellow at index 3
Color purple at index 4
Python For Loops with Else Statements
Understanding the Else Clause in For Loops
In Python, the else
clause isn’t just for if
statements; it can also be used with for loops. This might surprise those who are new to the language or even those who have been coding in Python for a while. The else
clause in a for loop adds a special touch to your code, allowing you to execute a block of code after the loop finishes iterating through all items in the sequence. However, there’s a small catch: the else
block will only execute if the loop completes without encountering a break
statement.
Let’s break this down with an example:
numbers = [2, 4, 6, 8, 10]
for num in numbers:
if num % 2 != 0: # Check if the number is odd
print(f"Found an odd number: {num}")
break
else:
print("All numbers are even")
In this code:
- The for loop iterates through the
numbers
list. - The
if
statement checks if a number is odd. - If an odd number is found, the loop stops early with
break
, and theelse
block is skipped. - If no odd number is found, the
else
block runs, printing “All numbers are even.”
The output in this case would be:
All numbers are even
Because the list contains only even numbers, the else
block executes after the loop ends naturally.
When and How to Use Else with For Loops
The else
clause is helpful when you want to run a block of code only if the loop completes without being interrupted by a break
. This pattern is useful in situations like searching for an item in a list, validating data, or ensuring that a condition holds true for every iteration.
Let’s explore another example where this feature shines:
names = ['Alice', 'Bob', 'Charlie', 'David']
for name in names:
if name == 'Eve': # Looking for the name 'Eve'
print("Found Eve!")
break
else:
print("Eve was not found in the list")
Here’s what happens:
- The loop searches for the name “Eve” in the
names
list. - If “Eve” is found, the loop stops with
break
, and theelse
block is skipped. - If “Eve” isn’t found after checking all names, the
else
block runs, printing “Eve was not found in the list.”
The output would be:
Eve was not found in the list
This approach allows you to perform a search and handle the case where the item isn’t found, all within the loop structure.
Practical Example: Validating a List of Passwords
Let’s say you have a list of passwords and want to ensure all of them meet a certain security standard (e.g., a minimum length of 8 characters). If you find any password that’s too short, you want to stop the validation process immediately and raise a flag. Otherwise, you want to confirm that all passwords are secure.
passwords = ['password123', 'admin', 'letmein', 'qwertyuiop']
for pwd in passwords:
if len(pwd) < 8: # Check if the password is too short
print(f"Password '{pwd}' is too short!")
break
else:
print("All passwords are secure")
In this example:
- The loop checks each password’s length.
- If a password is found to be too short, it prints a warning and stops checking further passwords.
- If all passwords meet the length requirement, the
else
block confirms their security.
The output here would be:
Password 'admin' is too short!
Since the second password is too short, the loop stops, and the else
block doesn’t run.
List Comprehensions as a Concise Alternative to For Loops
Introduction to List Comprehensions in Python
List comprehensions provide a more concise and readable way to create lists in Python. Instead of using a for loop to append items to a list, you can do it in a single line. This makes your code cleaner and easier to understand. For example, if you want to create a list of squares from 1 to 5, you could use a list comprehension instead of a loop:
squares = [x**2 for x in range(1, 6)]
This one-liner replaces a longer for loop and gives you the same result, making your code more elegant and efficient.
Converting For Loops to List Comprehensions
Let’s look at how a basic for loop can be transformed into a list comprehension. Consider a simple example where you want to create a list of squares from a list of numbers:
Here’s how you might write it using a for loop:
numbers = [1, 2, 3, 4, 5]
squares = []
for number in numbers:
squares.append(number ** 2)
print(squares)
Output:
[1, 4, 9, 16, 25]
This code works perfectly, but it takes a few lines to accomplish a simple task. Now, let’s see how you can achieve the same result using a list comprehension:
numbers = [1, 2, 3, 4, 5]
squares = [number ** 2 for number in numbers]
print(squares)
Output:
[1, 4, 9, 16, 25]
In this example, the list comprehension condenses the for loop into a single line. This not only saves space but also makes the code more readable.
List comprehension syntax is simple:
[expression for item in iterable]
expression
: What you want to include in your new list (in this case,number ** 2
).item
: A variable representing each element in the iterable.iterable
: The collection you’re looping over (in this case,numbers
).
Examples of List Comprehensions for Common For Loop Tasks
List comprehensions aren’t just about shortening code—they also open up possibilities for more complex operations with ease. Let’s explore some common for loop tasks and how they can be rewritten using list comprehensions.
1. Filtering Elements in a List
Suppose you want to create a new list that contains only the even numbers from an existing list:
Using a for loop:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = []
for number in numbers:
if number % 2 == 0:
even_numbers.append(number)
print(even_numbers)
Output:
[2, 4, 6, 8, 10]
Using a list comprehension:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [number for number in numbers if number % 2 == 0]
print(even_numbers)
Output:
[2, 4, 6, 8, 10]
The list comprehension simplifies the process by including the filtering condition directly within the comprehension.
2. Transforming Elements in a List
Imagine you need to convert a list of strings to uppercase:
Using a for loop:
words = ['hello', 'world', 'python', 'rocks']
uppercase_words = []
for word in words:
uppercase_words.append(word.upper())
print(uppercase_words)
Output:
['HELLO', 'WORLD', 'PYTHON', 'ROCKS']
Using a list comprehension:
words = ['hello', 'world', 'python', 'rocks']
uppercase_words = [word.upper() for word in words]
print(uppercase_words)
Output:
['HELLO', 'WORLD', 'PYTHON', 'ROCKS']
Again, the list comprehension makes the transformation more direct and readable.
3. Combining Multiple Lists
If you want to combine two lists into a list of tuples, where each tuple contains corresponding elements from the original lists:
Using a for loop:
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
combined = []
for i in range(len(list1)):
combined.append((list1[i], list2[i]))
print(combined)
Output:
[(1, 'a'), (2, 'b'), (3, 'c')]
Using a list comprehension:
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
combined = [(list1[i], list2[i]) for i in range(len(list1))]
print(combined)
Output:
[(1, 'a'), (2, 'b'), (3, 'c')]
This example shows how list comprehensions can efficiently combine elements from multiple lists into a new list.
Handling Errors and Exceptions in For Loops
When working with for loops in Python, it’s not uncommon to encounter errors or exceptions, especially when dealing with unpredictable data. Errors can disrupt the flow of your program, leading to unexpected results or even crashes. That’s why it’s crucial to know how to handle these situations gracefully.
Let’s dive into how to handle errors and exceptions within for loops effectively, ensuring that your code remains robust and reliable.
Common Errors in Python For Loops and How to Avoid Them
For loops are powerful, but they can sometimes trip you up if you’re not careful. Let’s explore some common errors that occur in for loops and how to avoid them.
1. IndexError
An IndexError occurs when you try to access an index in a list (or any sequence) that doesn’t exist. For example:
numbers = [1, 2, 3, 4, 5]
for i in range(6): # The range goes beyond the list's length
print(numbers[i])
In this code, the for loop attempts to access numbers[5]
, but since the list only has indices 0 to 4, an IndexError will be raised.
How to avoid it: Make sure your loop’s range matches the length of the list:
for i in range(len(numbers)):
print(numbers[i])
2. TypeError
A TypeError often occurs when you’re trying to perform an operation on incompatible data types. For instance:
mixed_list = [1, 'two', 3, None]
for item in mixed_list:
print(item + 1) # This will cause a TypeError for non-integers
Here, adding 1
to a string or None
will cause a TypeError.
How to avoid it: Check the type of each item before performing operations:
for item in mixed_list:
if isinstance(item, int):
print(item + 1)
else:
print(f"Cannot add 1 to {item} (type: {type(item)})")
3. KeyError
A KeyError occurs when trying to access a dictionary key that doesn’t exist. For example:
my_dict = {'a': 1, 'b': 2}
for key in ['a', 'b', 'c']:
print(my_dict[key]) # KeyError will occur for 'c'
How to avoid it: Use the get
method, which returns None
(or a specified default) if the key is not found:
for key in ['a', 'b', 'c']:
print(my_dict.get(key, 'Key not found'))
Using Try-Except Blocks within For Loops
To handle errors gracefully in your for loops, you can use try-except blocks. These allow you to catch exceptions and decide how to handle them, preventing your program from crashing.
For example, let’s say you’re reading numbers from a list and dividing a constant by each number:
numbers = [10, 5, 0, 2, 'a']
for number in numbers:
try:
result = 10 / number
print(f"10 divided by {number} is {result}")
except ZeroDivisionError:
print("Cannot divide by zero")
except TypeError:
print(f"Invalid type: {number} is not a number")
In this example:
- A ZeroDivisionError is caught when attempting to divide by
0
. - A TypeError is caught when trying to divide by a string (
'a'
).
By using try-except blocks within your for loops, you can handle each error specifically, allowing your program to continue running even when encountering problematic data.
Breaking Out of For Loops: Break, Continue, and Pass
For loops in Python are powerful for iterating over elements, but there are times when you might want to control how the loop behaves. That’s where the break, continue, and pass statements come in. These statements allow you to:
- break: Exit the loop entirely, even if there are more items to iterate over.
- continue: Skip the current iteration and move on to the next one.
- pass: Do nothing and move on, useful as a placeholder or when you want to skip a loop body without any action.
These statements provide more control over the loop’s flow and help you handle different situations more efficiently.
Let’s explore each of these statements in detail, with examples to make the concepts more relatable and easy to understand.
Using the Break Statement to Exit For Loops Early
The break
statement is like an emergency exit in a for loop. It allows you to stop the loop entirely, regardless of whether you’ve reached the end of the sequence. This is particularly useful when you’ve found what you’re looking for or when continuing further would be unnecessary or even problematic.
For instance, let’s say you’re searching for a specific number in a list:
numbers = [3, 8, 12, 7, 9, 15, 2]
for number in numbers:
if number > 10:
print(f"Found a number greater than 10: {number}")
break
In this example, the loop stops as soon as it finds the first number greater than 10. The break
statement ensures that we don’t waste time checking the remaining numbers, which can be particularly important in larger datasets.
Understanding the Continue Statement in For Loops
The continue
statement is like a “skip” button for your for loop. When Python encounters continue
, it skips the rest of the code inside the loop for that particular iteration and moves on to the next item. This can be handy when you want to skip over certain values but still complete the loop.
Consider this scenario: You’re looping through a list of numbers but want to skip any negative numbers:
numbers = [5, -3, 8, -1, 12]
for number in numbers:
if number < 0:
continue
print(f"Processing number: {number}")
Here, the loop skips over -3
and -1
, continuing to the next numbers. The continue
statement is a clean way to avoid processing values that don’t meet your criteria.
When to Use the Pass Statement in For Loops
The pass statement in Python may seem confusing because it does nothing at all. However, it’s quite useful in certain situations. It acts as a placeholder when you need to define a block of code, like a loop or a function, but aren’t ready to implement the actual logic yet. It allows your program to run without errors, even if that part of the code is incomplete.
Here’s an example where you might use pass
:
numbers = [4, 7, 10, 13]
for number in numbers:
if number % 2 == 0:
pass # Placeholder for future logic
else:
print(f"Odd number: {number}")
In this code, pass
is used to temporarily “fill in” the loop where you might later add logic to handle even numbers. The loop continues to run without any interruptions, and the pass
statement allows you to keep your structure intact.
Understanding how to use break
, continue
, and pass
in for loops can significantly improve your control over loop behavior in Python. The break
statement allows you to exit a loop early when you’ve found what you need. The continue
statement lets you skip over parts of the loop that don’t meet your criteria. And pass
acts as a placeholder, allowing you to outline your code and fill in the details later.
Latest Advancements in Python For Loops
Python has consistently evolved to keep up with the changing needs of developers, and for loops are no exception. Over the years, especially with Python 3.x, these loops have seen significant updates that make them more efficient, flexible, and easier to use. Whether you’re a seasoned developer or just starting, understanding these advancements can help you write better code.
Let’s explore the recent updates in Python 3.x that have impacted for loops, new libraries that enhance their functionality, and how for loops have evolved.
Recent Updates in Python 3.x Affecting For Loops
With Python 3.x, for loops became even more powerful and efficient, particularly when working with large datasets. One of the major improvements is the better support for iterators and generators. In earlier versions, handling large datasets could be tricky and memory-heavy, often requiring complex solutions. However, Python 3.x simplifies this by allowing for loops to work more efficiently with large sequences, enabling your program to handle vast amounts of data without running into memory problems. This improvement makes Python 3.x much more suitable for tasks like processing large files or iterating through big collections.
For example, using the range()
function in Python 2.x would generate a list, which could be memory-intensive. In Python 3.x, range()
creates an iterator instead:
# Python 3.x
for i in range(1000000):
# Efficient iteration without generating a large list in memory
print(i)
This improvement makes for loops more efficient, especially when dealing with large numbers of iterations.
Another important update is the introduction of the enumerate()
function, which allows you to loop over a sequence and get both the index and the value in one go:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits):
print(f"Index {index} has the fruit: {fruit}")
This function simplifies code and reduces the need for manually tracking indexes, making for loops cleaner and more intuitive.
New Python Libraries that Enhance For Loop Functionality
Python’s ecosystem of libraries continues to grow, and several new libraries have emerged that extend the capabilities of for loops. One such library is tqdm
, which allows you to add progress bars to your loops with minimal code changes. This is particularly useful when working with long-running loops, giving you visual feedback on the progress.
Here’s how you can use tqdm
in a for loop:
from tqdm import tqdm
import time
for i in tqdm(range(100)):
time.sleep(0.1) # Simulating a task
In just a few lines, you get a clear progress bar that updates in real-time, making it easier to monitor your loop’s execution.
Another useful library is itertools, which provides a range of functions for creating more advanced and efficient loops. These functions simplify complex looping tasks that would otherwise be tricky or time-consuming to implement manually. For instance, itertools.product() allows you to iterate over all possible combinations of multiple sequences, making it perfect for generating Cartesian products (all combinations of items from different lists). This is especially helpful when solving problems that involve exploring every possible combination or systematically processing multiple choices.
import itertools
colors = ['red', 'green']
sizes = ['S', 'M', 'L']
for color, size in itertools.product(colors, sizes):
print(f"Color: {color}, Size: {size}")
This powerful tool extends the reach of for loops, enabling you to perform advanced iterations with ease.
How For Loops Have Evolved in Python 3.x Versions
The evolution of for loops in Python 3.x is closely tied to the language’s overall push towards better efficiency, readability, and functionality. The shift from list-based iteration to iterator-based iteration with range()
was a significant step forward. It not only reduced memory usage but also encouraged a more generator-based approach to looping, which is often more efficient in Python.
Additionally, the language has introduced list comprehensions and generator expressions, which offer more concise alternatives to traditional for loops. These constructs allow you to create new lists or generators in a single line, often replacing more verbose for loop code:
# Traditional for loop
squares = []
for i in range(10):
squares.append(i * i)
# List comprehension
squares = [i * i for i in range(10)]
This evolution reflects Python’s commitment to making the language more accessible and expressive, encouraging developers to write code that is both efficient and easy to read.
The advancements in for loops in Python 3.x reflect the language’s ongoing evolution toward efficiency, simplicity, and power. Whether it’s the enhanced support for iterators, the introduction of useful functions like enumerate()
, or the rise of new libraries like tqdm
and itertools
, Python’s for loops are more capable than ever. By understanding these changes and incorporating them into your coding practices, you can write more efficient and readable code, staying ahead in the ever-evolving world of Python programming.
Best Practices for Using For Loops in Python
For loops are a fundamental tool in Python, and while they’re simple to use, getting the most out of them requires a bit of strategy. In this guide, we’ll explore the best practices for using for loops in Python, focusing on optimizing them for performance, avoiding common pitfalls, and refactoring them for readability and efficiency.
Optimizing For Loops for Performance
When working with for loops in Python, especially in large-scale projects or data-heavy applications, performance matters. Here are a few ways to optimize your loops:
1. Avoid Unnecessary Computations Inside Loops:
Every time a loop iterates, it repeats whatever instructions are inside. So, if you’re performing calculations or accessing data that doesn’t change with each iteration, it’s better to move those computations outside the loop. This small change can significantly speed up your code.
# Example: Unoptimized loop
result = 0
for i in range(1000000):
result += len("constant_string") # Unnecessary computation inside loop
# Example: Optimized loop
result = 0
string_length = len("constant_string")
for i in range(1000000):
result += string_length
2. Use Built-in Functions and Libraries:
Python’s built-in functions and libraries are often implemented in C, making them much faster than writing the equivalent functionality in Python. For example, if you need to sum a list, using Python’s sum()
function is more efficient than manually summing the elements in a loop.
# Example: Using built-in sum function
numbers = [1, 2, 3, 4, 5]
total = sum(numbers) # More efficient than a loop
3. Use Generators and Iterators:
Generators and iterators are memory-efficient because they yield items one at a time instead of generating the entire list at once. Using them in for loops can save memory and make your code run faster, especially with large datasets.
# Example: Using a generator in a for loop
def square_numbers(numbers):
for number in numbers:
yield number * number
for square in square_numbers(range(1000000)):
pass # Process each square number
4. Avoid Nested Loops When Possible:
Nested loops, where one loop is placed inside another, can often lead to performance issues, especially when dealing with large datasets. If you notice your code becoming slow or inefficient, it’s worth exploring alternative methods. For example, list comprehensions can sometimes replace nested loops with more concise and faster solutions. Additionally, external libraries like itertools can provide optimized functions that avoid the need for multiple nested loops, making your code both more efficient and easier to read.
# Example: Refactor nested loop with itertools.product
import itertools
colors = ['red', 'blue', 'green']
sizes = ['S', 'M', 'L']
for color, size in itertools.product(colors, sizes):
print(f"Color: {color}, Size: {size}")
Avoiding Common Pitfalls with For Loops
Even experienced developers sometimes fall into common traps when working with for loops. Here are some pitfalls to watch out for:
- Modifying a List While Iterating Over It: Modifying a list while iterating over it can cause unexpected results in Python. As you loop through a list, altering it during iteration may skip some elements or lead to errors. To avoid this, it’s best to either loop through a copy of the list or create a new list to store any changes you make. This ensures that the iteration works as expected without any unintended consequences.
# Example: Avoid modifying list during iteration
numbers = [1, 2, 3, 4, 5]
for number in numbers[:]: # Iterate over a slice copy of the list
if number % 2 == 0:
numbers.remove(number)
2. Confusing Range Boundaries: Python’s range()
function is half-open, meaning it includes the start value but excludes the end value. This can sometimes lead to off-by-one errors, especially for those new to Python. It’s important to remember how range()
works to avoid unexpected results.
# Example: Correct use of range
for i in range(5): # Iterates over 0, 1, 2, 3, 4
print(i)
3. Using for
Instead of while
: Not all loops should be for loops. If you don’t know in advance how many iterations you’ll need, a while
loop might be more appropriate. A for loop is ideal when iterating over a known sequence, but if the loop’s continuation depends on a condition, a while
loop can be more efficient and clearer.
# Example: Using a while loop instead of a for loop
count = 0
while count < 5:
print(count)
count += 1
Refactoring For Loops for Readability and Efficiency
Readability is just as important as efficiency when it comes to for loops. Code that’s easier to read is also easier to maintain and debug. Here are some tips on how to refactor for loops for better readability:
- Use Descriptive Variable Names: While it might be tempting to use single-letter variable names in for loops, more descriptive names make your code easier to understand at a glance.
# Example: Using descriptive variable names
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
2. Keep Loop Bodies Small: When a for loop becomes too complex or lengthy, it can be hard to follow. If you find yourself writing a lot of code inside the loop, consider breaking it up into smaller functions. This approach makes the loop easier to read and understand, while also improving the reusability of your code. By organizing your code into functions, you can keep things clean and manageable.
# Example: Refactoring a large loop body into a function
def process_fruit(fruit):
print(f"Processing {fruit}")
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
process_fruit(fruit)
3. Use List Comprehensions Where Appropriate: List comprehensions can often replace a for loop with a single, concise line of code. However, this should only be done if it improves clarity—if the list comprehension becomes too complex, a traditional for loop might be better.
# Example: Refactoring a for loop into a list comprehension
fruits = ['apple', 'banana', 'cherry']
fruit_lengths = [len(fruit) for fruit in fruits]
Practical Applications of For Loops in Real-World Projects
For loops in Python are not just a basic programming concept; they are the backbone of many real-world applications. Whether you’re processing large datasets, scraping data from websites, or automating repetitive tasks, for loops can be your go-to tool for getting the job done efficiently.
Using For Loops for Data Processing and Analysis
In data processing and analysis, for loops are incredibly useful. Let’s say you have a large dataset with thousands of customer records, and you need to calculate the average purchase amount. A for loop lets you go through each record, add up the purchase amounts, and then divide by the total number of customers to get the average. Here’s a simple example to show how it works:
# Example dataset: List of customer purchase amounts
purchase_amounts = [100, 200, 150, 300, 250]
# Calculate the total sum and count of customers
total_amount = 0
total_customers = len(purchase_amounts)
# Loop through each purchase amount and add it to the total
for amount in purchase_amounts:
total_amount += amount
# Calculate the average purchase amount
average_amount = total_amount / total_customers
print("Average purchase amount:", average_amount)
In this example, the for loop goes through each purchase amount in the purchase_amounts
list and adds it to total_amount
. Then, the average is calculated by dividing the sum by the number of customers.
For Loops in Web Scraping with Python
Web scraping is another area where for loops shine. Suppose you want to gather information from multiple pages of a website, like product prices or blog post titles. A for loop can help you iterate through each page, send requests, and extract the required data. Here’s an example using the requests
and BeautifulSoup
libraries:
import requests
from bs4 import BeautifulSoup
urls = [
'https://example.com/page1',
'https://example.com/page2',
'https://example.com/page3'
]
for url in urls:
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
titles = soup.find_all('h2', class_='post-title')
for title in titles:
print(title.text)
In this snippet, the for loop iterates through a list of URLs, fetching the HTML content of each page and then extracting the titles of blog posts. This is a simplified version, but you can imagine scaling this up to scrape thousands of pages or even automating the process to run daily.
Automating Tasks with For Loops in Python
Automation is another practical application where for loops can save you a lot of time and effort. Whether it’s renaming files, sending out personalized emails, or updating records in a database, for loops allow you to automate repetitive tasks with minimal code.
For instance, if you have a folder full of files that need renaming according to a specific pattern, a for loop can help:
import os
files = os.listdir('/path/to/your/folder')
for index, file in enumerate(files):
new_name = f'file_{index}.txt'
os.rename(f'/path/to/your/folder/{file}', f'/path/to/your/folder/{new_name}')
In this code, the for loop goes through each file in the directory, renames it according to the specified pattern, and saves the changes. This approach can be adapted to other tasks, such as batch processing images, cleaning up data files, or even interacting with APIs.
These examples illustrate just a few ways for loops can be applied in real-world projects.
Conclusion
Recap: Key Takeaways on Using For Loops in Python
As we’ve explored, for loops are an important part of Python programming, allowing you to iterate over sequences efficiently and effectively. We discussed optimizing for loops for better performance, such as by avoiding unnecessary computations inside loops and using Python’s built-in functions. We also highlighted the importance of using for loops wisely, like steering clear of modifying lists during iteration and ensuring that your loops are clear and readable by using descriptive variable names and keeping loop bodies concise.
Final Thoughts on the Importance of Mastering For Loops
Mastering for loops in Python is more than just understanding how to write them—it’s about writing them well. As you continue to code, you’ll realize that for loops are not just a tool but a foundation for building more complex logic. They help you break down tasks into manageable steps, make your code more readable, and ultimately, more powerful. The ability to write efficient, clean, and error-free loops is a skill that will serve you well, whether you’re automating tasks, analyzing data, or developing software.
External Resources
Python Official Documentation: The official Python documentation is the best place to start. It provides comprehensive coverage of all Python features, including for loops.
For Loop Documentation: This section of the Python tutorial specifically explains for loops and how they are used in Python.
List Comprehensions: Learn more about list comprehensions, a concise way to create lists using for loops.
Python’s Enumerate Function: This page explains the enumerate()
function, which allows you to loop over something and have an automatic counter.
Exception Handling in Python: Understand how to handle errors and exceptions, especially within for loops.
FAQs
A for loop in Python is a control flow statement that allows you to iterate over a sequence (such as a list, tuple, string, or range) and execute a block of code for each element in that sequence.
A for loop iterates over a sequence of elements, whereas a while loop continues to execute as long as a certain condition is true. For loops are generally used when the number of iterations is known beforehand, while while loops are used when the number of iterations is not known.
You can exit a for loop early using the break
statement. This will terminate the loop as soon as the break
statement is encountered.
continue
statement in a for loop? The continue
statement skips the current iteration of the loop and moves on to the next iteration. It’s useful when you want to skip certain conditions within a loop.
If the sequence in a for loop is empty, the loop body will not execute, and the loop will simply terminate.
Leave a Reply