Introduction to Conditional Statements in Python
When learning Python, one of the most important concepts you’ll encounter is conditional statements, If, Elif, and Else. These are the backbone of decision-making in your code, allowing your program to react differently based on varying conditions. Whether you’re just starting out or brushing up on your Python skills, understanding conditional statements is crucial.
What Are If, Elif, and Else from conditional statements?
Let’s break down these fundamental components:
- If Statement: This is where the magic begins. The
if
statement allows your program to test a specific condition. If the condition is true, the block of code under theif
statement will execute. If not, it will skip to the next part of the code. - Elif Statement: The
elif
(short for ‘else if’) conditional statements comes into play when you have multiple conditions to check. If theif
statement’s condition is false, the program moves on to check the condition specified in theelif
statement. You can have as manyelif
statements as you need, making your program more flexible and adaptable. - Else Statement: Finally, the
else
conditional statements is your safety net. If none of theif
orelif
conditions are true, theelse
statement will execute. It’s like the program saying, “Well, none of these conditions were met, so let’s do this instead.”
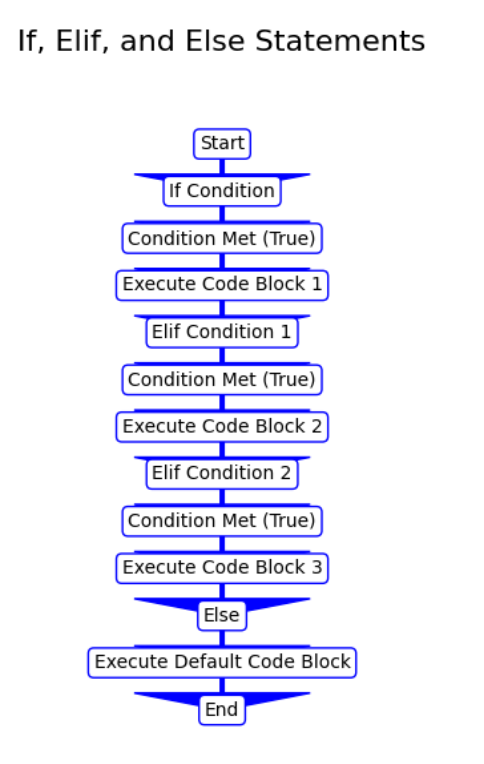
Overview of conditional statements in Programming
conditional statements is the essence of control flow in any programming language, not just Python. It’s what allows programs to make decisions, much like how we do in everyday life. When you wake up and decide what to wear based on the weather, you’re using conditional logic. Similarly, in programming, conditional statements guide the program on what to do next depending on specific criteria.
In Python, this control flow is primarily handled by if
, elif
, and else
conditional statements. Mastering these statements will empower you to create programs that can handle a wide variety of scenarios, making your code more efficient and dynamic.
Importance of Control Flow in Python
Why is control flow so important? Without it, your code would be linear and unresponsive to different situations. Control flow allows your program to take different paths based on the input it receives or the conditions it encounters. This is especially vital in creating interactive applications, where the user’s input directly influences the outcome.
In Python, the simplicity and readability of if
, elif
, and else
conditional statements make them an essential tool for anyone learning to code. They are your go-to tools for making your program responsive and intelligent.
Basic Syntax of If, Elif, and Else conditional statements
Understanding the syntax of these conditional statements is the first step to using them effectively. Here’s a basic example:
weather = "sunny"
if weather == "sunny":
print("Wear sunglasses!")
elif weather == "rainy":
print("Take an umbrella!")
else:
print("Have a great day!")
In this example, the program checks the value of the weather
variable. When the weather is “sunny,” the program advises you to wear sunglasses. On a “rainy” day, it suggests taking an umbrella. For any other weather, it simply wishes you a great day. This is a simple yet powerful demonstration of how conditional statements work in Python.
By regularly practicing with if
, elif
, and else
statements, you’ll soon master the ability to manage complex decision-making in your code. This skill is invaluable, not just in Python, but across any programming language you choose to learn, as it allows you to control the program’s flow based on different conditions.
As you progress in your coding journey, using conditional statements will become second nature, enabling you to build more complex and interactive programs effortlessly.
Must Read
- AI Pulse Weekly: December 2024 – Latest AI Trends and Innovations
- Can Google’s Quantum Chip Willow Crack Bitcoin’s Encryption? Here’s the Truth
- How to Handle Missing Values in Data Science
- Top Data Science Skills You Must Master in 2025
- How to Automating Data Cleaning with PyCaret
The Role of If conditional statements in Python
How to Use If Statements for Conditional Execution
An if
statement in Python allows your program to execute specific blocks of code only when certain conditions are met. This makes your scripts more dynamic and responsive to the data they process. It’s a bit like setting rules for your code: if a condition is true, the program performs one action; if not, it takes a different action.
For those new to Python, writing if
conditional statements is easy. Begin with the keyword if
, followed by a condition and a colon. Then, place the code that should execute when the condition is true on the following indented line.
Let’s break this down with an example.
Example: Simple If conditional statements in Python
Here’s a basic example that illustrates how to use an if
statement:
temperature = 30
if temperature > 25:
print("It's a hot day! Stay hydrated.")
In this example, the program checks if the temperature is greater than 25 degrees. If it is, it prints a message advising you to stay hydrated. This is a very simple example, but it shows how you can use if
statements to make your program react to different conditions.
When I began learning Python, I found it incredibly satisfying to watch my code come to life as it responded to various inputs. This interactive experience made learning feel more engaging, and I quickly grasped the practical uses of what I was studying.
When to Use If conditional statements in Python Scripts
Understanding when to use if
statements in Python scripts is just as important as knowing how to write them. Whenever your program needs to make decisions—like checking user input, validating data, or controlling the flow of your application—if
statements are your go-to tool.
For example, if you’re building a simple weather app, you might use if
conditional statements to suggest what users should wear based on the current temperature or weather conditions. Similarly, in a game, you might use if
statements to determine whether a player has won or lost, or to trigger different events based on the player’s actions.
As you gain more experience with Python, you’ll start to see how powerful if
conditional statements are in controlling the logic of your programs. They enable you to create scripts that can handle a wide variety of scenarios, making your code more flexible and adaptive to real-world situations.
In your journey to mastering Python, getting comfortable with if
statements is a critical step. These statements are not just about making decisions; they are about giving your code the ability to react and adapt, just like you do in everyday life.
Common Mistakes to Avoid When Using If conditional statements
As you start using if
statements in Python, it’s natural to run into a few bumps along the way. Even experienced coders sometimes make common errors in Python if statements. Recognizing and understanding these mistakes can save you a lot of time and frustration. By knowing what to watch out for, you’ll be able to troubleshoot if statement errors more effectively and write cleaner, more efficient code.
Common Errors in Python If conditional statements
One of the most common mistakes is forgetting to properly indent your code. Python relies on indentation to define the blocks of code that belong to each if
, elif
, or else
statement. If the indentation is off, Python will throw an IndentationError
, and your code won’t run as expected.
Here’s an example of improper indentation:
temperature = 30
if temperature > 25:
print("It's a hot day!") # This line is not indented properly
The mistake here is easy to miss. The print
statement should be indented so that Python knows it belongs to the if
block. The correct version looks like this:
temperature = 30
if temperature > 25:
print("It's a hot day!") # Now it's properly indented
I remember when I first started coding, indentation errors were my biggest headache. I spent hours trying to figure out why my code wasn’t working, only to realize it was all because of a missing indent. These errors might seem small, but they can stop your code from running.
Another common error is forgetting to use the double equals sign (==
) when comparing values. In Python, a single equals sign (=
) is used for assignment, while the double equals (==
) is used to check equality. Mixing these up can lead to unexpected behavior in your code.
For example:
user_input = "yes"
if user_input = "yes": # Oops! This should be '=='
print("User agreed.")
This code will cause a SyntaxError
because Python interprets user_input = "yes"
as an assignment, not a comparison. The corrected code should be:
user_input = "yes"
if user_input == "yes":
print("User agreed.")
Troubleshooting If Statement Errors in Python
When you run into errors with if
statements, the best approach is to carefully read the error message provided by Python. These messages often point you directly to the line causing the issue. Take your time to analyze what might be going wrong—whether it’s an indentation problem, a typo, or a logic error.
A handy tip is to insert print statements at different points in your code to check if it’s reaching certain parts of the logic. For instance, if an if
statement isn’t triggering as expected, you can add a print("Checking condition...")
before the condition to confirm that your program is evaluating the if
statement.
user_input = "no"
print("Checking condition...")
if user_input == "yes":
print("User agreed.")
else:
print("User disagreed.")
This way, you can trace the flow of your program and better understand where things might be going wrong.
Best Practices for Writing If Statements
To avoid common mistakes, it’s important to follow some best practices when writing if
statements. Here are a few tips:
- Keep Your Conditions Simple: Complex conditions can be harder to read and debug. If possible, break down your conditions into smaller, more manageable pieces. This will make your code easier to follow.
- Use Meaningful Variable Names: Naming your variables clearly can help you and others understand what the condition is checking for. Instead of using generic names like
x
ory
, use descriptive names liketemperature
oruser_input
. - Test Your Code Frequently: After writing a few lines of code, test it to make sure it works as expected. This habit can help you catch errors early before they become harder to track down.
- Watch Out for Logical Errors: Sometimes your code runs without any syntax errors, but the logic isn’t quite right. For example, using an
and
operator when you meant to useor
can change the outcome of your condition completely.
By keeping these practices in mind, you’ll avoid many of the pitfalls that can trip up both beginners and experienced programmers alike. Learning from mistakes is part of the journey, and by addressing these common errors, you’ll become more confident in writing and troubleshooting if
statements in Python.
Mastering Elif conditional statements in Python
The Purpose of Elif Statements in Conditional Logic

When you’re coding in Python, you often encounter situations where a single condition isn’t enough to make decisions in your program. This is where the elif
statement comes into play. Understanding how to use elif
statements in Python for multiple conditions is essential for writing flexible and efficient code. The elif
statement allows your program to evaluate multiple conditions in a sequence, making it a powerful tool for controlling the flow of your script.
How to Use Elif Statements in Python for Multiple Conditions
The elif
statement, short for “else if,” comes into play when you have more than two possible outcomes to consider. With elif
, you can chain together multiple conditions, and Python will check each one in the order you’ve written them. The moment a true condition is found, Python executes the corresponding block of code and skips the rest. If none of the conditions are true, the program will move on to the optional else
statement, if you’ve included one.
Here’s how it looks in practice:
temperature = 15
if temperature > 25:
print("It's a hot day!")
elif temperature > 15:
print("It's a warm day.")
elif temperature > 5:
print("It's a cool day.")
else:
print("It's a cold day.")
In this example, the program checks the temperature and prints a message based on which condition is true. When the temperature exceeds 25 degrees, the program prints, “It’s a hot day!” If this condition isn’t met, it then checks whether the temperature is above 15 degrees, and so on. Should none of the if
or elif
conditions apply, the else
block executes, signaling that it’s a cold day.
Differences Between If, Elif, and Else conditional statements
Understanding the differences between if
, elif
, and else
conditional statements is crucial for effectively using them in your Python scripts. Here’s a quick breakdown:
- If Statement: The
if
statement is used to check the first condition. If this condition is true, the code block under it will execute, and the rest of the conditions (if any) will be ignored. - Elif Statement: The
elif
statement follows theif
statement and is used to check additional conditions if the previous ones were false. You can have as manyelif
statements as needed to cover all possible scenarios. - Else Statement: The
else
statement is the final fallback. It executes only if all the precedingif
andelif
conditions are false. It’s optional but useful for handling cases that don’t fit any specific condition.
Example: Using Elif to Check Multiple Conditions
Let’s look at another example that shows how to use elif
statements in Python for multiple conditions:
score = 85
if score >= 90:
print("Grade: A")
elif score >= 80:
print("Grade: B")
elif score >= 70:
print("Grade: C")
elif score >= 60:
print("Grade: D")
else:
print("Grade: F")
In this case, a student’s score determines their grade. The program checks each elif
condition in order, from the highest grade down to the lowest. The first true condition it encounters dictates the grade that gets printed.
Using elif
statements in this way simplifies your code and makes it easier to follow. Instead of writing separate if
statements for each grade range, the elif
structure allows all related conditions to be grouped together in a logical, readable manner.
Nested Elif conditional statements and Their Applications
When coding in Python, you’ll sometimes encounter scenarios where a simple chain of if-elif-else
statements isn’t enough to handle all the conditions your program needs to check. This is where nested elif
statements in Python come into play. They allow you to structure more complex conditional logic by placing if-elif-else
blocks inside other if-elif-else
blocks. While this can make your code more intricate, it also gives you the flexibility to manage multiple layers of conditions, ensuring that your program behaves exactly as you want it to.
How to Structure Complex Conditional Logic with Elif
Nested elif
statements are like decision trees. You start with a broad condition and then, depending on the outcome, narrow down to more specific conditions. This technique is particularly useful when the outcome of one condition influences the evaluation of subsequent conditions.
Let’s break it down with an example. Suppose you’re writing a program to determine the clothing recommendations based on both temperature and weather conditions.
temperature = 20
weather = "rainy"
if temperature > 25:
if weather == "sunny":
print("Wear sunglasses and light clothing.")
elif weather == "rainy":
print("Take an umbrella and wear light clothing.")
else:
print("Just wear light clothing.")
elif temperature > 15:
if weather == "sunny":
print("Wear sunglasses and a light jacket.")
elif weather == "rainy":
print("Take an umbrella and wear a jacket.")
else:
print("Wear a light jacket.")
else:
if weather == "sunny":
print("Wear warm clothing, and maybe sunglasses.")
elif weather == "rainy":
print("Wear warm clothing and take an umbrella.")
else:
print("Just bundle up!")
In this code, the first if
statement checks the temperature. Inside each temperature condition, a nested if-elif-else
block further checks the weather. This structure allows the program to make more nuanced decisions based on a combination of factors.
When I first started coding, I remember being a bit overwhelmed by the idea of nesting conditions. It felt like opening a set of Russian dolls—each condition revealing another layer underneath. However, once I grasped the concept, it became much easier to organize my thoughts and code in a way that made complex decisions feel manageable.
Example: Python Code with Nested Elif conditional statements
Let’s consider another practical example, where you want to decide which transport mode to use based on both distance and urgency.
distance = 10 # in kilometers
urgency = "high"
if distance > 20:
if urgency == "high":
print("Take a taxi or drive your car.")
else:
print("Take a bus or drive your car.")
elif distance > 5:
if urgency == "high":
print("Ride a bike or take a taxi.")
else:
print("Take a bus or walk.")
else:
if urgency == "high":
print("Walk quickly or take a taxi.")
else:
print("Just walk.")
In this example, the program first evaluates the distance. Depending on whether the distance is above 20 kilometers, between 5 and 20 kilometers, or below 5 kilometers, the program then checks the urgency. This nested structure allows the program to select the most appropriate mode of transport based on the combined conditions of distance and urgency.
Nested elif
conditional statements might seem intimidating at first, but they’re incredibly useful for handling situations where multiple layers of decision-making are required. The key to using them effectively is to keep your code organized and ensure that each condition is necessary and relevant. By breaking down complex logic into smaller, nested pieces, you make your program both more powerful and easier to understand.
Practical Examples of Elif Statements in Python
Elif
conditional statements in Python are highly valuable for managing scenarios where multiple conditions must be checked. They might appear a bit abstract initially, but observing them in action can make their purpose and importance in programming much clearer. Let’s look at some practical examples of elif
in Python to understand how these statements can be effectively utilized.
Real-World Use Cases for Elif in Python
One of the most common scenarios where elif
conditional statements are used is in determining outcomes based on user input or external data. For example, if you’re building a simple weather app, you might want to provide users with specific advice depending on the weather conditions.
Example 1: Weather-based Outfit Suggestions
Imagine you want to create a program that suggests what to wear based on the weather:
weather = "cloudy"
temperature = 18 # degrees Celsius
if weather == "sunny":
print("Wear sunglasses and a hat.")
elif weather == "rainy":
print("Don't forget your umbrella!")
elif weather == "cloudy" and temperature > 20:
print("A light jacket should be enough.")
elif weather == "cloudy" and temperature <= 20:
print("Wear a warm jacket.")
else:
print("Just dress comfortably!")
Here, the elif
statements allow the program to check multiple conditions before deciding what advice to give. This makes the program more flexible and responsive to different scenarios, providing more personalized suggestions.
Example 2: Grading System Based on Scores
Another practical use of elif
is in educational applications, such as grading systems. Suppose you want to assign letter grades based on a student’s score:
score = 85
if score >= 90:
grade = "A"
elif score >= 80:
grade = "B"
elif score >= 70:
grade = "C"
elif score >= 60:
grade = "D"
else:
grade = "F"
print(f"The student's grade is: {grade}")
In this example, the elif
statements allow the program to evaluate different score ranges and assign the appropriate grade. This is a common pattern in educational software and demonstrates how elif
can be used to handle multiple potential outcomes efficiently.
Python Code Snippets Demonstrating Elif Usage
Let’s take a look at a more complex scenario where elif
helps manage multiple conditions. Suppose you’re developing a basic chatbot that responds to user greetings in different ways depending on the time of day.
greeting = "hello"
time_of_day = "afternoon"
if greeting.lower() in ["hello", "hi", "hey"]:
if time_of_day == "morning":
print("Good morning! How can I assist you today?")
elif time_of_day == "afternoon":
print("Good afternoon! What can I do for you?")
elif time_of_day == "evening":
print("Good evening! How can I help you tonight?")
else:
print("Hello! How can I help you?")
else:
print("Greetings! How may I assist you?")
In this example, elif
allows the chatbot to provide different responses based on the time of day. This type of conditional logic is essential in creating more dynamic and user-friendly applications. It’s a real-world example of how elif
statements can enhance the functionality of a program by introducing more nuanced responses.
The Else Statement: Handling All Other Cases

Why and When to Use Else conditional statements in Python
In Python, else statements play an essential role in controlling the flow of your code. When you’re writing a program that needs to handle different scenarios, else statements ensure that no situation gets overlooked. But when should you use an else statement, and how does it fit into the broader picture of conditional logic in Python?
When to Use Else Statements in Python Programs
The else statement is a safety net for your code. After you’ve checked all your specific conditions using if
and elif
statements, else
steps in to catch any cases that haven’t been explicitly handled. This is particularly useful when you want to make sure that your code doesn’t miss any potential outcomes.
For example, let’s say you’re writing a program to classify temperatures:
temperature = 12
if temperature > 30:
print("It's a hot day.")
elif temperature > 20:
print("It's a warm day.")
elif temperature > 10:
print("It's a cool day.")
else:
print("It's a cold day.")
In this code, the else statement handles any temperature that’s 10 degrees or below. Without the else
, temperatures of 10 degrees or lower would be ignored, potentially causing confusion or errors in your program’s output.
When should you use an else statement in your Python programs? The answer is whenever you need to ensure that your code covers all possible scenarios. If there’s a chance that none of your if
or elif
conditions will be met, adding an else
will make sure that your program still produces a valid and meaningful output.
The Role of Else in Ensuring Code Coverage
Ensuring code coverage is a vital aspect of writing reliable programs. Code coverage means making sure that every possible input or situation is accounted for. The else statement plays a crucial role here, as it ensures that even the unexpected or less common cases are handled appropriately.
For instance, consider a program that categorizes the age of a user:
age = 45
if age < 13:
category = "child"
elif age < 20:
category = "teenager"
elif age < 60:
category = "adult"
else:
category = "senior"
print(f"The user is classified as: {category}")
In this example, the else
statement ensures that anyone 60 years old or older is categorized as a senior. If you removed the else
and the user’s age was 60 or above, the program would fail to assign them to any category, leading to incomplete results.
Effectively using else
statements ensures your code remains predictable, even when it encounters unexpected inputs. This is crucial in real-world applications, where failing to handle a condition properly could lead to incorrect data processing or user experience problems.
Example: Python if-elif-else Statement for Comprehensive Condition Checking
Let’s break down a more complex example where an if-elif-else structure ensures comprehensive condition checking. Suppose you’re creating a program that offers different greetings based on the time of day:
hour = 16
if hour < 12:
print("Good morning!")
elif hour < 18:
print("Good afternoon!")
else:
print("Good evening!")
In this case, the else statement ensures that the program can handle any hour that isn’t covered by the morning or afternoon conditions. If the time is 6 PM or later, the program will greet the user with “Good evening!”
Combining If, Elif, and Else conditional statements in Python
When it comes to writing Python code that needs to handle multiple conditions, combining if
, elif
, and else
statements is a powerful approach. This combination allows you to create clear and efficient conditional logic that can adapt to various scenarios. Let’s explore how to use these statements together effectively, and look at an example to illustrate their use.
How to Write Clean and Efficient Code with Combined Conditional Statements
Combining if, elif, and else statements helps you create a structured way to handle different conditions in your code. Here’s a simple guide to making your code clean and efficient using these statements:
- Start with an
if
Statement: This is where you handle the first condition. It checks if a specific condition is true, and if so, executes the corresponding block of code. - Add
elif
Statements: These statements allow you to check additional conditions if the previousif
condition was not met. You can have as manyelif
statements as needed to handle different scenarios. - Finish with an
else
Statement: This is the catch-all for any cases not covered by theif
orelif
conditions. It ensures that there’s always a fallback action, which helps in managing unexpected scenarios.
By structuring your code in this way, you ensure that all possible conditions are considered, and your program behaves predictably. This approach also makes your code easier to read and maintain.
Example: Full If-Elif-Else Chain in Python
To see how this works in practice, let’s look at a detailed example. Suppose you’re creating a simple grading system that assigns letter grades based on a student’s score:
score = 85
if score >= 90:
grade = "A"
elif score >= 80:
grade = "B"
elif score >= 70:
grade = "C"
elif score >= 60:
grade = "D"
else:
grade = "F"
print(f"The student's grade is: {grade}")
In this example, the if
, elif
, and else
statements work together to handle all possible ranges of scores:
if score >= 90
: If the score is 90 or above, the student gets an “A”.elif score >= 80
: If the score is between 80 and 89, the student gets a “B”.elif score >= 70
: If the score is between 70 and 79, the student gets a “C”.elif score >= 60
: If the score is between 60 and 69, the student gets a “D”.else
: For scores below 60, the student gets an “F”.
This full chain ensures that every possible score is accounted for, and the output is always meaningful. It’s an example of how combining these statements provides a clear and comprehensive way to handle multiple conditions.
Advanced Techniques with If, Elif, and Else conditional statements
Short-Circuit Evaluation in Python If Statements
When you’re working with multiple conditions in Python, you might notice that the code doesn’t always evaluate every single condition you write. This behavior is known as short-circuit evaluation. Understanding this concept can help you write more efficient code and avoid unnecessary computations. Let’s explore how Python handles multiple conditions in if
statements and see some examples of short-circuit behavior.

How Python Handles Multiple Conditions in If Statements
In Python, when you use logical operators like and
and or
in your if
statements, the language uses short-circuit evaluation to determine the result. This means that Python doesn’t always evaluate every condition. Instead, it stops as soon as the result is determined. This can save time and resources, especially in complex conditions.
Short-circuit evaluation works differently with the and
and or
operators:
- With
and
: Python evaluates the conditions from left to right. If it finds any condition that isFalse
, it immediately concludes the entire expression isFalse
and doesn’t check the remaining conditions. This is because, withand
, if one condition isFalse
, the whole expression can’t possibly beTrue
. - With
or
: Python evaluates the conditions from left to right as well. If it finds any condition that isTrue
, it immediately concludes the entire expression isTrue
and doesn’t check the remaining conditions. This is because, withor
, if one condition isTrue
, the whole expression is guaranteed to beTrue
.
Example: Short-Circuit Behavior with Logical Operators
Let’s look at how short-circuit evaluation works with some code examples:
Example 1: Using and
Operator
def is_even(num):
return num % 2 == 0
def is_positive(num):
return num > 0
number = 5
if is_even(number) and is_positive(number):
print("The number is even and positive.")
else:
print("The number does not meet the conditions.")
In this example, is_even(number)
returns False
because 5 is not an even number. Due to short-circuit evaluation with the and
operator, Python doesn’t even check is_positive(number)
. Since the first condition is False
, it already knows the whole expression cannot be True
. Therefore, it directly executes the else
block.
Example 2: Using or
Operator
def is_even(num):
return num % 2 == 0
def is_positive(num):
return num > 0
number = -4
if is_even(number) or is_positive(number):
print("The number is either even or positive.")
else:
print("The number does not meet either condition.")
Here, is_even(number)
returns True
because -4 is an even number. Thanks to short-circuit evaluation with the or
operator, Python doesn’t evaluate is_positive(number)
. Since the first condition is True
, the whole expression is already True
, so it skips the rest and prints “The number is either even or positive.”
Short-circuit evaluation is a powerful feature in Python that can enhance the performance of your code by avoiding unnecessary evaluations. By understanding how Python handles multiple conditions with and
and or
, you can write more efficient and effective conditional logic.
As you continue to work with Python, keep in mind how short-circuit evaluation can affect your code. It’s a subtle but important aspect that can help you write better, faster code. Embrace this concept, and use it to make your Python programs even more efficient!
Using Ternary Conditional Operators as an Alternative
When you’re looking to simplify your code and make it more concise, Python’s ternary conditional operator can be a great tool. This operator allows you to write conditional expressions in a more compact form, which can be especially handy in situations where you need to make quick decisions within a single line of code. Let’s explore how to use the ternary conditional operator in Python and how it can replace more verbose if-else
logic.

How to Write Concise Conditional Expressions with Ternary Operators
The ternary conditional operator provides a way to condense an if-else
statement into a single line. The general syntax for this operator is:
value_if_true if condition else value_if_false
Here’s a breakdown of the syntax:
condition
: This is the condition you want to check.value_if_true
: This is the value or expression that will be used if the condition evaluates toTrue
.value_if_false
: This is the value or expression that will be used if the condition evaluates toFalse
.
Using this syntax allows you to create a more readable and concise version of simple conditional statements. It’s particularly useful for assigning values based on conditions.
Example: Simplifying If-Else Logic with Python’s Ternary Operator
To illustrate how the ternary conditional operator works, let’s simplify a common if-else
scenario:
Traditional If-Else Statement
Suppose you want to determine if a number is positive or not:
number = 10
if number > 0:
result = "Positive"
else:
result = "Non-positive"
print(result)
This code checks if number
is greater than 0. If it is, it assigns "Positive"
to result
; otherwise, it assigns "Non-positive"
. It works perfectly but can be a bit verbose.
Using Ternary Conditional Operator
You can simplify the above if-else
statement using Python’s ternary operator:
number = 10
result = "Positive" if number > 0 else "Non-positive"
print(result)
Here, the ternary operator condenses the logic into a single line. It’s much shorter and can be easier to read at a glance. The condition number > 0
determines which value gets assigned to result
, making the code more concise without losing clarity.
The ternary conditional operator in Python is a handy tool for writing concise and readable conditional expressions. By understanding how to use this operator effectively, you can replace more verbose if-else
statements with cleaner, single-line expressions. This not only makes your code more compact but also enhances its readability.
Latest Advancements in Python Conditional Statements
The Introduction of Pattern Matching in Python 3.10
With the release of Python 3.10, a new feature called pattern matching was introduced, bringing a fresh approach to handling conditional logic. This feature offers a more powerful and flexible way to manage complex conditions compared to the traditional if-elif-else
chains. Let’s explore how pattern matching enhances conditional logic in Python and see some practical examples of how it can be used.

How Pattern Matching Enhances Conditional Logic in Python
Pattern matching allows you to write more readable and maintainable code by matching patterns in data structures. Unlike if-elif-else
statements, which check conditions one by one, pattern matching provides a more declarative approach. It enables you to describe the structure and content of data in a clear and concise manner.
Here’s how pattern matching can enhance your code:
- Simplified Syntax: Pattern matching introduces a new syntax that can be more intuitive than multiple
if-elif
statements. It reduces the need for repetitive condition checks. - Improved Readability: The pattern matching syntax is designed to be more readable. It can be easier to understand at a glance compared to a long chain of
if-elif-else
conditions. - Flexible Matching: You can match various data types and structures, including tuples, lists, and custom classes, making it a powerful tool for handling diverse conditions.
Example: Using Pattern Matching as an Alternative to If-Elif-Else Chains
To illustrate the benefits of pattern matching, let’s compare it to a traditional if-elif-else
chain. Suppose you need to check the type of a variable and act accordingly:
Using If-Elif-Else Chains
Here’s how you might handle different types of variables using if-elif-else
:
def check_variable(value):
if isinstance(value, int):
return "Integer"
elif isinstance(value, str):
return "String"
elif isinstance(value, list):
return "List"
else:
return "Other"
print(check_variable(42)) # Output: Integer
print(check_variable("hello")) # Output: String
print(check_variable([1, 2, 3])) # Output: List
print(check_variable(3.14)) # Output: Other
This code works well but can become cumbersome if you have many conditions.
Using Pattern Matching
With pattern matching, the same logic becomes more concise:
def check_variable(value):
match value:
case int():
return "Integer"
case str():
return "String"
case list():
return "List"
case _:
return "Other"
print(check_variable(42)) # Output: Integer
print(check_variable("hello")) # Output: String
print(check_variable([1, 2, 3])) # Output: List
print(check_variable(3.14)) # Output: Other
In this example, the match
statement checks the type of value
using patterns. Each case
specifies a pattern to match, and the _
pattern serves as a catch-all for any values that don’t match the previous patterns. This syntax is cleaner and easier to follow, especially as the number of conditions grows.
The introduction of pattern matching in Python 3.10 provides a powerful alternative to traditional if-elif-else
chains. It simplifies the process of handling complex conditions, improves readability, and enhances flexibility in matching various data structures.
Performance Optimization Techniques for Conditional Statements
When working with conditional statements in Python, performance can be an important consideration, especially when dealing with large datasets or complex logic. Optimizing your if-elif-else
blocks can make your code run faster and more efficiently. Let’s explore some practical tips for improving the performance of your conditional statements and how to measure the impact of these optimizations.
Tips for Improving the Efficiency of If-Elif-Else Blocks
1. Order Conditions by Likelihood:
The order in which you write your conditions can affect performance. Place the most frequently true conditions at the top. This way, the program checks the most likely conditions first, potentially skipping over less likely ones.
Example:
def categorize_age(age):
if age < 18:
return "Minor"
elif age < 65:
return "Adult"
else:
return "Senior"
If most of your inputs are likely to be adults, place the elif age < 65
condition before the else
. This ensures the function checks the most common condition first.
2. Use Boolean Logic Wisely
Combine conditions that share the same outcome. This avoids redundant checks and makes your code more efficient.
Example:
def is_eligible(age, has_id):
if age >= 18 and has_id:
return True
else:
return False
Here, combining age >= 18
and has_id
into a single if
statement improves efficiency by reducing the number of checks.
3. Minimize Expensive Operations
Avoid placing complex operations or function calls directly in the condition. Perform these operations outside the conditional logic if possible.
Example:
def process_data(data):
if len(data) > 1000: # expensive operation
return "Large dataset"
else:
return "Small dataset"
In this case, you can cache the result of len(data)
to avoid recalculating it multiple times.
4. Leverage Dictionary Lookups
For scenarios with multiple discrete conditions, using a dictionary to map keys to functions or values can be more efficient than a long if-elif
chain.
Example:
def handle_command(command):
commands = {
'start': start_engine,
'stop': stop_engine,
'status': check_status
}
# Execute command if it exists, else handle invalid command
return commands.get(command, handle_invalid_command)()
This method avoids multiple if-elif
checks by directly mapping commands to their corresponding functions.
Python Code Profiling and Performance Measurement
To truly understand how optimizations impact your code, you need to measure its performance. Python provides tools to profile and analyze code execution:
1. Use the time
Module:
For simple timing, you can use the time
module to measure how long your code takes to run.
Example:
import time
start_time = time.time()
# Code to measure
end_time = time.time()
print(f"Execution time: {end_time - start_time} seconds")
This basic timing can give you a rough idea of performance improvements.
2. Profile with cProfile
For more detailed analysis, the cProfile
module can help identify which parts of your code are consuming the most time.
Example:
import cProfile
def my_function():
# Code to profile
cProfile.run('my_function()')
This will provide a detailed report on function calls and their execution times.
3. Analyze with timeit
The timeit
module is useful for timing small code snippets and comparing performance before and after optimizations.
Example:
import timeit
code_to_test = """
# Code snippet to test
"""
execution_time = timeit.timeit(code_to_test, number=1000)
print(f"Execution time: {execution_time} seconds")
This helps in assessing how changes affect performance on a small scale.
Optimizing Python conditional statements can boost your code’s speed and efficiency. Start by ordering conditions wisely. Minimize costly operations and use dictionary lookups to enhance performance. Profile your code to measure the impact of these changes and refine your approach. These techniques will help you write faster, more efficient Python code and improve your programming skills.
Best Practices for Writing If, Elif, and Else Statements in Python
Writing Readable and Maintainable Code
When crafting code, especially when working with if-elif-else
statements, ensuring that your code is readable and maintainable is crucial. This becomes even more important in collaborative projects where multiple people may be working on the same codebase. Let’s explore some best practices for writing clear and maintainable conditional logic, along with tips for refactoring complex conditions.
Importance of Code Readability in Collaborative Projects
Code readability refers to how easily others (or even yourself in the future) can understand your code. Good readability ensures that your code is easy to follow, debug, and update. This is particularly important in collaborative projects where team members need to understand and work with each other’s code.
Imagine working on a team project where the codebase is a tangled mess of if-elif-else
statements. It can quickly become overwhelming and difficult to manage. By following best practices for writing clear conditional logic, you can make your code more accessible and easier to maintain.
Best Practices for Writing If-Elif-Else Statements
1. Keep It Simple and Organized:
Break down complex conditions into smaller, more manageable parts. Keeping your logic simple helps avoid confusion.
Example:
def categorize_age(age):
if age < 0:
return "Invalid age"
elif age < 18:
return "Minor"
elif age < 65:
return "Adult"
else:
return "Senior"
This example handles age categorization in a clear and straightforward manner.
2. Use Descriptive Variable Names
Choose variable names that clearly indicate their purpose. Descriptive names make your code more intuitive.
Example:
def check_voting_eligibility(age):
if age >= 18:
return "Eligible to vote"
else:
return "Not eligible to vote"
Here, age
and check_voting_eligibility
make the code’s intent clear.
3. Avoid Deep Nesting
Deeply nested if-elif-else
blocks can be hard to read. Try to keep nesting to a minimum by using early returns or breaking up complex logic into functions.
Example:
def process_order(order_amount):
if order_amount <= 0:
return "Invalid order amount"
if order_amount < 50:
return "Standard shipping"
if order_amount < 100:
return "Free shipping"
return "Free expedited shipping"
By avoiding deep nesting, the logic remains more readable and easier to manage.
4. Use Functions to Encapsulate Logic
Encapsulate conditional logic into functions to improve readability and reuse code. This helps keep your if-elif-else
statements clean and focused.
Example:
def is_valid_age(age):
return age >= 0
def categorize_age(age):
if not is_valid_age(age):
return "Invalid age"
if age < 18:
return "Minor"
elif age < 65:
return "Adult"
else:
return "Senior"
Encapsulating the age validation logic in a separate function improves clarity.
Refactoring Tips for Complex Conditional Logic
Refactoring involves reorganizing your code to improve its structure and readability without changing its functionality. Here are some tips for refactoring complex if-elif-else
statements:
1. Use Guard Clauses:
Instead of nesting multiple conditions, use guard clauses to handle edge cases and exit early.
Example:
def categorize_age(age):
if age < 0:
return "Invalid age"
if age < 18:
return "Minor"
if age < 65:
return "Adult"
return "Senior"
Here, each condition is handled immediately, reducing nesting.
2. Apply the Strategy Pattern
For scenarios with many different conditions and outcomes, consider using a strategy pattern or lookup table to map conditions to results.
Example:
def handle_command(command):
commands = {
'start': start_engine,
'stop': stop_engine,
'status': check_status
}
return commands.get(command, handle_invalid_command)()
This approach replaces a long if-elif
chain with a dictionary lookup.
3. Simplify with Logical Operators
Combine multiple conditions using logical operators when possible to reduce complexity.
Example:
def check_eligibility(age, has_id):
if age >= 18 and has_id:
return "Eligible"
return "Not eligible"
Using and
to combine conditions simplifies the logic.
Writing readable and maintainable code is essential, especially when using if-elif-else
statements. By keeping your logic simple, using descriptive names, avoiding deep nesting, and encapsulating conditions in functions, you can improve code clarity. Refactoring techniques like using guard clauses, the strategy pattern, and logical operators can further enhance readability and maintainability. These practices will make your code easier to work with, both for you and your team.
Testing and Debugging Conditional Statements
When working with conditional statements like if-elif-else
in Python, it’s important to ensure that your code behaves as expected. Testing and debugging are crucial steps in this process. They help identify and fix issues, ensuring your code runs smoothly. Let’s explore some tools and techniques for debugging Python code and writing effective unit tests for conditional statements.
Tools and Techniques for Debugging Python Code
Debugging is the process of finding and fixing errors in your code. Here are some helpful tools and techniques for debugging if-elif-else
statements in Python:
1. Using Print Statements:
A straightforward method to debug your code is to insert print
statements. These can help you understand which parts of your code are executed and what values your variables hold.
Example:
def check_temperature(temp):
print(f"Temperature: {temp}") # Debug statement
if temp > 30:
print("It's hot!")
return "Hot"
elif temp > 20:
print("It's warm.")
return "Warm"
else:
print("It's cold.")
return "Cold"
By running the code and observing the output, you can see how different values affect the logic.
2. Using Python Debugger (pdb)
The pdb
module is a built-in Python debugger that allows you to step through your code, set breakpoints, and inspect variables. This tool provides more control than print statements.
Example:
import pdb
def check_temperature(temp):
pdb.set_trace() # Set a breakpoint
if temp > 30:
return "Hot"
elif temp > 20:
return "Warm"
else:
return "Cold"
Running this code will pause execution at pdb.set_trace()
, letting you inspect the current state and step through the code line by line.
Using Integrated Development Environment (IDE) Debuggers
Many IDEs, such as PyCharm or VSCode, come with built-in debuggers. These graphical tools provide features like breakpoints, variable inspection, and step execution, making debugging easier.
Example:
- In PyCharm, you can set breakpoints by clicking in the gutter next to the line number.
- Run your script in Debug mode, and the IDE will pause execution at breakpoints, allowing you to inspect variables and step through code.
4. Using Logging
For more persistent debugging, especially in production code, use the logging
module instead of print statements. Logging provides a way to record what happens in your code, which can be reviewed later.
Example:
import logging
logging.basicConfig(level=logging.DEBUG)
def check_temperature(temp):
logging.debug(f"Temperature: {temp}")
if temp > 30:
return "Hot"
elif temp > 20:
return "Warm"
else:
return "Cold"
The logging
module allows you to set different logging levels and record messages to various outputs.
Writing Unit Tests for If-Elif-Else Statements
Unit testing is a method of testing individual parts of your code to ensure they work correctly. Writing unit tests for if-elif-else
statements helps verify that each condition is handled properly. Here’s how you can approach it:
1. Using the Unittest Framework:
Python’s unittest
module is a built-in library for writing and running tests. You can create test cases for different conditions your code should handle.
Example:
import unittest
def check_temperature(temp):
if temp > 30:
return "Hot"
elif temp > 20:
return "Warm"
else:
return "Cold"
class TestTemperature(unittest.TestCase):
def test_hot(self):
self.assertEqual(check_temperature(35), "Hot")
def test_warm(self):
self.assertEqual(check_temperature(25), "Warm")
def test_cold(self):
self.assertEqual(check_temperature(15), "Cold")
if __name__ == '__main__':
unittest.main()
This code defines a test case for each possible outcome of the check_temperature
function.
2. Using Assertions
Assertions are statements that check if a condition is true. In unit tests, assertions are used to compare the expected and actual output of your code.
Example:
def test_temperature():
assert check_temperature(35) == "Hot", "Test failed for hot temperature"
assert check_temperature(25) == "Warm", "Test failed for warm temperature"
assert check_temperature(15) == "Cold", "Test failed for cold temperature"
Each assertion verifies that the function returns the correct result for a given input.
3. Testing Edge Cases
It’s important to test edge cases—values that are at the boundary of what your code should handle. This helps ensure that your code behaves correctly in all scenarios.
Example:
def test_edge_cases():
assert check_temperature(30) == "Warm", "Test failed for edge case at 30"
assert check_temperature(20) == "Cold", "Test failed for edge case at 20"
Testing edge cases helps catch issues that might not be evident with typical inputs.
Testing and debugging are essential skills for any Python programmer. By using print statements, debuggers, and logging, you can effectively track down and resolve issues in your if-elif-else
statements. Writing unit tests ensures that your conditional logic works correctly across various scenarios. These techniques will help maintain code quality and reliability, making your development process more strong and enjoyable.
Conclusion
Summary of Key Takeaways
Understanding how to use if
, elif
, and else
statements in Python is crucial for managing conditional logic in your programs. These statements allow you to make decisions within your code, directing it to execute different blocks of code based on specific conditions. Here’s a recap of why these conditional statements are important:
- Control Flow:
If
,elif
, andelse
statements help control the flow of your program, ensuring that different actions are taken based on varying conditions. - Complex Logic: Using
elif
allows for handling multiple conditions in a clean and manageable way, reducing the need for lengthy and nestedif
statements. - Default Behavior: The
else
statement ensures that there’s a default block of code executed when none of the preceding conditions are met, providing a fallback option.
Practicing with these statements helps in developing a deeper understanding of how to handle various scenarios and refine your problem-solving skills. Experimenting with different conditions and logic structures will enhance your coding abilities and make your programs more robust.
Encouragement to Practice and Experiment with Conditional Logic
Applying what you’ve learned about if
, elif
, and else
statements in real-world projects will solidify your understanding and improve your coding skills. Don’t hesitate to create small projects or solve coding challenges that involve conditional logic. For instance:
- Create a simple calculator that performs different operations based on user input.
- Develop a weather app that suggests clothing based on the temperature.
- Build a quiz application that provides feedback based on the user’s answers.
These exercises will not only reinforce your knowledge but also make coding more enjoyable and practical. Experiment with different scenarios to see how changing conditions affect the behavior of your program. This hands-on approach is invaluable for mastering conditional logic.
Additional Resources for Learning Python Conditional Statements
To further enhance your understanding of Python conditional statements, you can explore the following resources:
- Official Python Documentation: The official Python documentation offers detailed explanations and examples of conditional statements.
FAQs
if
, elif
, and else
statements in Python? Answer: If
, elif
, and else
statements are fundamental tools in Python used to control the flow of a program based on conditions. The if
statement checks whether a condition is true and executes a block of code if it is. If the if
condition is not met, elif
allows you to check additional conditions. The else
statement provides a default block of code that runs when none of the conditions in if
or elif
are true.
if
statement? Answer: An if
statement evaluates a condition, and if that condition is true, the code following it will run. It’s the starting point of conditional logic in a program. For example, you might use an if
statement to determine if a number is positive.
elif
? Answer: Use elif
when you have multiple conditions to check, and you want to handle each one differently. It allows you to add more checks after the initial if
statement. Each elif
condition is only checked if the previous conditions were false.
else
statement do? Answer: The else
statement provides a final option that executes when none of the if
or elif
conditions are true. It acts as a fallback to handle any cases that weren’t addressed by the earlier conditions.
elif
statements? Answer: Yes, you can include multiple elif
statements to handle various conditions. Each elif
allows you to test a new condition, and only the first true condition’s block of code will be executed.